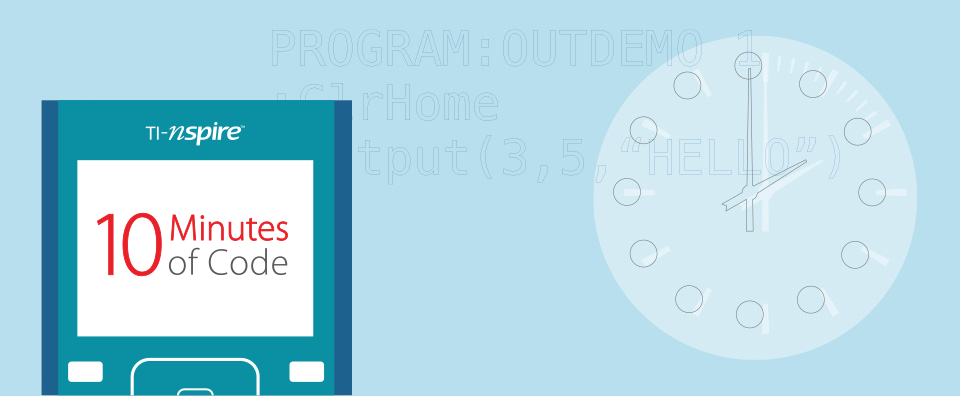
TI Codes: TI-Nspire™ programming activities
Engage students in short activities that build understanding of math concepts, programming logic and coding skills
*Some of the features used in these lessons require TI-Nspire™ CX OS version 4.5
**Unit 6 requires the TI-Nspire™ CX II with OS 5.0 or higher.
Unit 1: Program Basics
Skill Builder 1 Introducing the Program Editor
Download Teacher/Student DocsIn this first lesson for Unit 1, you will learn about the TI-Nspire™ CX Basic Program Editor and how to write, store and run your first program.
Objectives:
- Launching the TI-Nspire Program Editor
- Write your first program
- Store and run a program
Step 1
Introducing the Program Editor
- Start a New Document and add select Add Program Editor > New...
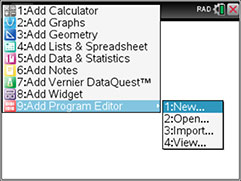
Step 2
- In the Name field, type the name of your first program, hello.
- Leave the Type as Program and the Library Access as None. We'll discuss Type in a later lesson.
- Click OK, or press enter.
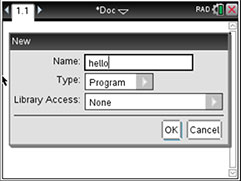
Step 3
Your screen should now look like the image to the right. Your program code belongs inside the Prgm...EndPrgm block within the editor. You cannot type anything outside this area (with one exception we'll discuss later). These keywords cannot be edited.
Note: The single page program editor is a feature in the TI-Nspire CX OS version 4.5 and beyond. Your screen will appear as a split screen by default if you are on another platform or OS version.
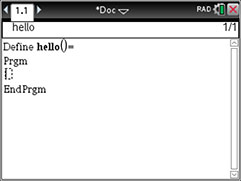
Step 4
All programming commands are found in the menu.
- With your text cursor inside the dotted box in the editor, select menu > I/O > Disp. The keyword Disp is pasted into your program.
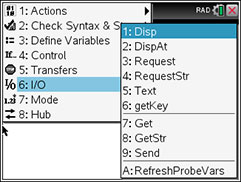
Step 5
- Next, type the quotation (literal string) template “ “ by pressing ctrl and then the multiplication key.
- Inside the quotes, type the text Hello, World! Use the shift key for capital letters, and press the
key to select the exclamation point.
Note: You must type the quotes before typing the text.
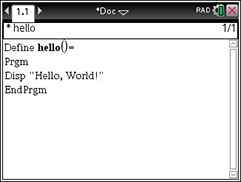
Step 6
- Before running any program, you must first ‘store’ the program to memory. To store the program, select menu > Check Syntax & Store > Check Syntax & Store (or use the shortcut ctrl-B.) You should see '"<program name>" stored successfully' at the top of the screen.
Note: An asterisk (*) in front of the program name indicates that the program has changed but has not been stored.
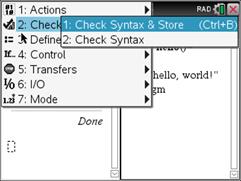
Step 7
- To run the program press ctrl+R. This is a shortcut for menu > Check Syntax & Store > Run which checks syntax (grammar), stores the program, adds a Calculator app on a new page after the Program Editor page, and pastes the program name into the Calculator app. Press enter to run the program.
The word Done indicates that the program has completed.
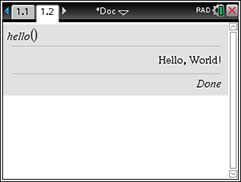
Step 8
- To view or edit the program, switch to the previous page by pressing ctrl+left arrow or by clicking the page number tab at the top of the screen.
- Save the document as hello program. This ensures that you will have access to this program in the future.
Congratulations! You've written your first TI-Nspire™ CX Basic program!
Skill Builder 2 Arguments and Expressions
Download Teacher/Student DocsIn this second lesson for Unit 1, you will learn about sending arguments into a program and displaying results of expressions.
Objectives:
- Use arguments in a program
- Use expressions in Disp statements
Step 1
Why does a program name need parentheses?
The parentheses after a program name are always required, and they allow a program to accept arguments or initial values. There are two forms of arguments: the formal arguments which are always variables within the parentheses when viewing the Program Editor and the actual arguments which are values, defined variables, or expressions that are entered into the parentheses when running the program on the Calculator app.
Define hypotenuse(a, b)
a and b are formal arguments (or parameters) that will receive values when the program runs.
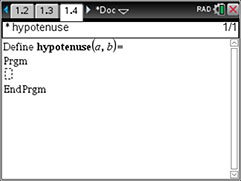
Step 2
Let’s write a program that uses arguments to compute the length of the hypotenuse of a right triangle.
- If you are continuing work in a document, you can insert a page by pressing ctrl+doc, and selecting Add Program Editor > New...
Alternatively, start a new document by pressing, and selecting New Document. Select Add Program Editor > New...
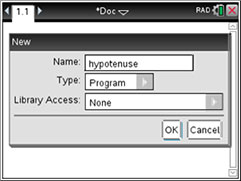
Step 3
- Name the program hypotenuse, and select OK or press enter.
- In the Program Editor, press the up arrow to move inside the parentheses after the program name. Type the formal arguments a, b (note the comma) inside the parentheses. Then, move the cursor into the Prgm…EndPrgm block by pressing the down arrow.
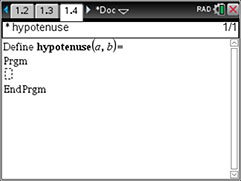
Step 4
The Code
In this program, one statement is used to display the value of the hypotenuse of a right triangle whose leg lengths are the arguments to the program.
- Enter Disp √(a^2 + b^2) by selecting menu > I/O > Disp and then typing the expression.
- 'Check Syntax & Store' the program by selecting menu > Check Syntax & Store > Check Syntax & Store (or use the shortcut ctrl-B)
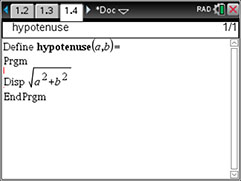
Step 5
Run the program
- Press ctrl+R to prepare to run the program. Before pressing enter, type two values separated by a comma inside the two parentheses provided. These values are used by the arguments a and b in the program. Then press enter. Be sure to use values for which you know the answer to test that the program is working properly.
You can also use expressions in place of numbers, such as:
hypotenuse(2*7, 9-5)
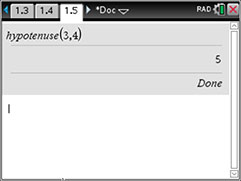
Step 6
- To run the program again, press var, and select the program name. Type two values, and press enter. Alternatively, arrow up to highlight the program name and the values in parentheses, press enter to paste to the command line, edit the values in the parentheses, and press enter to run the program.
- Test your program thoroughly with various values as arguments. Do any values cause errors?
- Save your document by pressing ctrl+S.
Skill Builder 3 Programs and Functions
Download Teacher/Student DocsIn this third lesson for Unit 1, you will learn the basic difference between a program and a function.
Objectives:
- Write a program and a function that appear to do the same thing.
- Explore the differences between a program and a function.
Step 1
What is a function?
The purpose of a function is to represent, or return a value. In the image on the right, the program hypotenuse and the function hypot perform the same task. Note the change from Disp in the program to Return in the function. The function ‘represents’ a value which can be used by other operations, even graphing.
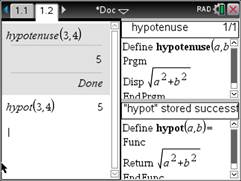
Step 2
Creating a Function
- In a Calculator app, select menu > Functions & Programs > Program Editor > New…
- Enter hypot for the name, and change the Type to Function. Select OK or press enter to create the new program.
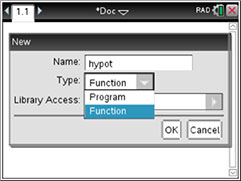
Step 3
You will see a split page with the Calculator app on the left and the Function Editor on the right. This makes it easy to edit and test the function on the same page.
- Add the arguments a,b inside the parentheses. Inside the function, add the Return statement by selecting menu > Transfers > Return. Complete the statement by adding the square root of a²+b².
Return √(a² + b²) - 'Check Syntax & Store' the program by selecting menu > Check Syntax & Store > Check Syntax & Store (or use the shortcut ctrl+B.)
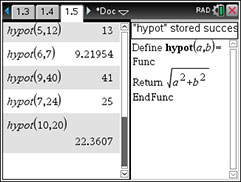
Step 4
- In the Calculator app, test the function. Use the example hypot(3,4). Also try some expressions using this function such as:
2*hypot(5,12) hypot(4,5) + hypot(6,7) (hypot(7,24))^2
Functions are similar to programs but are not the same. Functions can have many statements and look like other programs in the Program Editor. Functions are different from programs because their purpose is to return a value. The value can be a number, list, string, matrix, or any other built-in data type. Programs are limited in scope (where they can be used). Functions are more versatile because they can be used anywhere a built-in function is used. Programs can only be run (executed) from a Calculator app or in a Math Box in a Notes app. Functions represent a value that can be used as part of a larger expression.
Step 5
On a TI-Nspire™ CX CAS, a function will return an algebraic expression when undefined variables are used as arguments. It could also return a symbolic expression such as √(13) for hypot(2,3).
On a non-CAS TI-Nspire™ CX, the use of undefined variables produces an error message. For a symbolic expression such as √(13), the function returns an approximate numeric value.
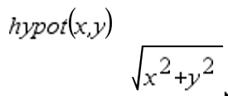
Step 6
Graphing the hypot(x,b) function
User-defined functions have the advantage of being available just like any built-in function.
After you've written and stored the hypot(a,b) function above, add a Graphs app, enter f1(x)=hypot(x,3) and select enter.
What shape is this function?
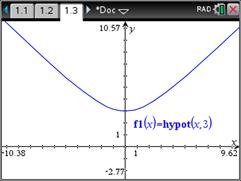
Application Evaluate a Formula
Download Teacher/Student DocsIn this Application for Unit 1, you will discover the versatility of the Disp statement and develop your own program. Skill Builders for Unit 1 should be completed prior to this activity.
Objectives:
- Embellish Disp statements to produce meaningful information using literal strings
- Write your own formula program
Step 1
A split-screen can be useful to compare program code with the output of the program on a Calculator app.
To set up a split-screen page with a Calculator app and a Program Editor:
- Add a Calculator app to your document, or open a New Document and add a Calculator app.
- Press menu > Functions & Programs > Program Editor > New….
When working on a split-screen page, press ctrl+tab to move from one application to the other. Alternatively, click in an application to make it the ‘active’ application. The border of that app becomes a bold rectangle.
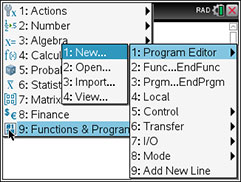
Step 2
The Disp statement can display more than one item at a time.
Study the image at the right in which the hypotenuse program has been modified. The program ‘echoes’ the arguments a and b with appropriate labels and then displays the calculated hypotenuse length, also appropriately labeled.
Note: The items in quotes are called ‘literal strings’. A string is a collection of characters ‘strung’ together.
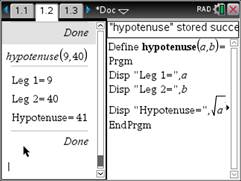
Step 3
When writing the program, remember to use the comma as a separator between the items to be displayed. There should be a comma in between the text in quotes and the value a in the expression
Disp “Leg 1 =”, a
After you edit your program, press ctrl+R to ‘Check Syntax & Store’ the program, and prepare to run the program in the Calculator app.
When you run the program, remember to provide two values inside the parentheses as arguments for the program.
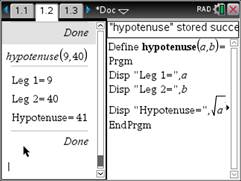
Step 4
Your Task
Write a program that takes one or more arguments and then displays the result of a calculation based on those argument(s).
The calculation can be any formula. Here are a few suggestions:
Area of a geometric shape:
Square: side²
Triangle: ½*b*h
Circle: pi*r²
Trapezoid: ½*(b1+b2)*h
Volume of a solid:
Cube: side³
Square Pyramid: 1/3*side²*height
Sphere: 4/3*pi*r³
Simple interest: A=P+P*R*T
Compound interest: A=P*(1+r/n)n*t
The program should clearly label the values of the arguments (input) and the result of the calculation (output) using strings.
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 2: Assigning Values to Variables
Skill Builder 1 Global Variables
Download Teacher/Student DocsIn this first lesson for Unit 2, you will use variables in a program and discover their effect in the document.
Objectives:
- Use a variable in a program
- Learn the impact of creating a variable in a program to the rest of the document
- Explore the ‘scope’ of the arguments to a program
Step 1
Storing a Value in a Variable Using :=
Often you need to assign a value to a variable within your program. Our example will use Heron’s formula for calculating the area of a triangle from the lengths of its three sides, a, b, and c. This is a two-step formula and the computations will be assigned to variables:
Determine half the perimeter, and assign the value to variable s:
s:=1/2(a+b+c)
Calculate the area, and assign the value to variable area:
area:=(s*(s-a)*(s-b)*(s-c))0.5
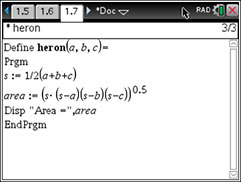
Step 2
The program is in the image to the right. Notice how the symbol is used to assign the value of a computation to a variable; it is not just an equals sign.
means “gets the value of…” or, more simply, “gets”, so the statement s:= ½ * (a + b + c) is read “s gets ½ the perimeter of the triangle.”
On the TI-Nspire™ CX, this symbol , is accessed by pressing
.
On a computer or the handheld, you can also type the two characters (colon and equals sign) separately. On the handheld, press to access the colon. For the equals sign, press
. On a handhld, it is simpler to just select symbol
.
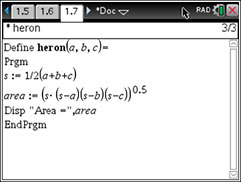
Step 3
On the TI-Nspire™ CX, this symbol , is accessed by pressing
.
On a computer or the handheld, you can also type the two characters (colon and equals sign) separately. On the handheld, press to access the colon. For the equals sign, press
. On a handhld, it is simpler to just select symbol
.
Step 4
Storing a Value in the Variable Using ➔
You can use the store operator (➔) in place of the gets operator () but the order is a bit different. See the program shown at the right. Notice the reverse order of the statements in which the computation comes first, then the store operator ➔ (ctrl+var), and then the variable. This is the order in which the statement is executed (left-to-right).
<expression> ➔ <variable>
Either the gets operator () or the store operator (➔) can be used in any assignment statement. Just remember the order of the statement for each method.
Both methods ‘assign’ a value (usually the result of a computation) to a variable so these are called assignment statements.
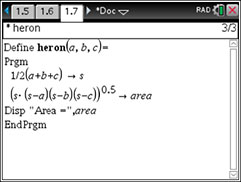
Step 5
- Enter the complete heron( ) program as shown in the image to the right.
- When the code has been entered, ‘Check Syntax & Store’ the program by selecting menu > Check Syntax & Store > Check Syntax & Store (or use the shortcut ctrl+B).
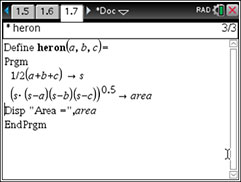
Step 6
Be sure to use a multiplication sign after the first s in the area formula. Without that multiplication sign, the TI-Nspire™ treats the expression s( as a function, and the error message ‘Invalid implied multiply’ appears when you ‘Check Syntax & Store’ (ctrl+B or ctrl+R).
- Test the program with the known values of 3, 4, 5 to make sure you get a result of 6 for the area. (Why is the area 6?)
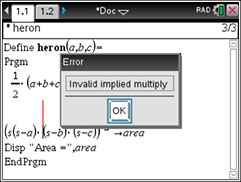
Step 7
- Next, in the Calculator app, select the var key and notice the list of variables. The variables area, heron and s appear in the list.
heron is the program name.
The variables area and s were created by the program.
a, b, and c were also used in the program. Why are they not listed? The answer lies in the fact that a, b, and c are arguments to the program and, as arguments, they only exist within the program and are not created in the document. In fact, we really do not want the variables area and s in the document either. We’ll learn how to fix this ‘side effect’ in the next lesson.
- Remember to save the document (ctrl+S) so that you save the program. We will use this program in the next lesson.
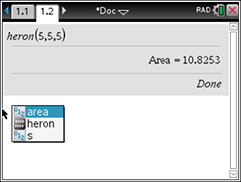
Skill Builder 2 Local variables
Download Teacher/Student DocsIn this second lesson for Unit 2, you will learn about declaring local variables in a program
Objectives:
- Understand the need for local variables
- Use local variables in programs
Step 1
- Begin by opening the document containing the heron program you wrote in Skill Builder 1 as seen in the image to the right.
Recall that in this program creates the variables s and area in the current problem of the document. This is undesirable, and we’ll learn how to prevent this issue now.
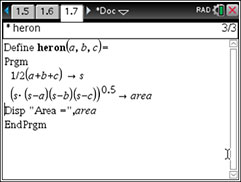
Step 2
- Below the Prgm keyword, add the statement Local s, area
Note: The Local statement is located in menu > Define Variables
- When this line of code has been entered, use the shortcut ctrl+B to check and store the program.
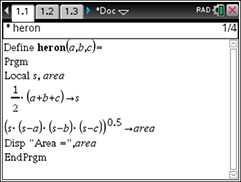
Step 3
- In the Calculator app, before running the program, use the command DelVar s, area to delete the variable from the problem.
Delete Variable is located in menu > Actions in a Calculator app.
- Now, run the heron program.
- When the program is done, select var, and notice that the only variable listed is the program heron itself.
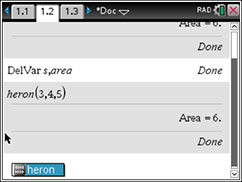
Step 4
What happened?
When you state or declare that a variable is ‘Local’, it tells the TI-Nspire™ CX to create the variable while executing the program and to delete the variable when the program ends so that it does not exist anywhere else in the current problem. This eliminates the issue of variables being created in the problem where they are not needed. If the problem already uses a variable that the program refers to, then declaring it Local within the program will not change the problem variable’s value since the program makes its own (temporary) variable instead.
Step 5
Summary
The ‘scope’ of a variable is the location(s) where the variable exists. In the TI-Nspire™ CX, variables live in the current problem. Adding a problem to a document gives you a ‘clean slate’ of variables. Problem 2 in a document knows nothing about the variables in problem 1 and vice-versa.
If programs in a problem use the same variables as the problem, then the programs can inadvertently (or intentionally) create or use those variables. Sometimes it might make sense to link the program variables and the problem variables but keep that in mind when creating programs.
Declaring variables as Local stops the program from creating or affecting those variables in the problem.
Functions, on the other hand, deal with the ‘scope’ principle in a different way as we’ll see in the next lesson.
Skill Builder 3 Functions and global variables
Download Teacher/Student DocsIn this third lesson for Unit 2, you will learn about a function's impact on global variables.
Objectives:
- Compare the impact of a function on global variables with that of programs
- Copy and Paste code from one program to another or within a program
Step 1
- Open the document that contains the heron program seen at the right.
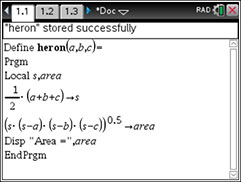
Step 2
- Add another Program Editor to the document by pressing ctrl+doc and selecting Add Program Editor. Create a new program using a unique name, and change the Type to Function.
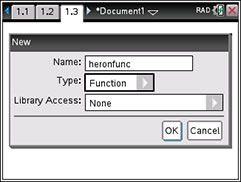
Step 3
- Add the arguments a, b, c in the parentheses.
- Copy the two assignment statements from the program heron to the new function.
To Copy and Paste on a handheld:
- Hold the SHIFT key down while moving the text cursor over the desired text.
- Press ctrl+C to copy the selection to the clipboard.
- Move from the program to the function, and place the cursor in the desired position.
- Press ctrl+V to paste the selection.
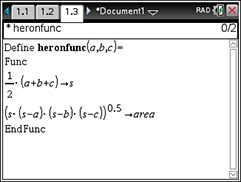
Step 4
Recall from Unit 1 that a function must Return a value.
- Add the statement
Return area
at the end of the function.
Do not use the Disp statement in the function.
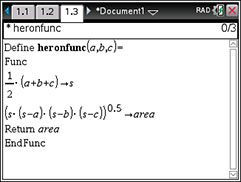
Step 5
- Prepare to run the function by selecting ctrl+R. Be sure to supply the three argument values. Press enter.
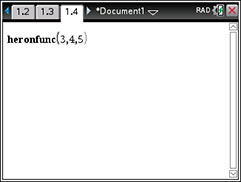
Step 6
An error message is displayed.
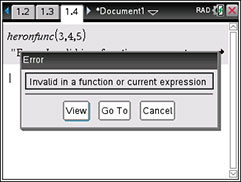
Step 7
What is wrong?
The variables s and area are global variables since they are not declared Local. Global (problem) variables are not permitted in a function though global variables are permitted in a program. Recall that the purpose of a function is to return a value. To protect global variables from inadvertent changes (‘side effects’), functions cannot modify global variables.
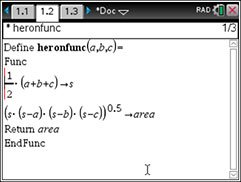
Step 8
- To fix this error, add the statement Local s, area at the top of the function.
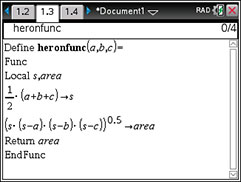
Step 9
- Store, and run the function again.
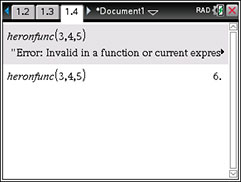
Application A Mystery
Download Teacher/Student DocsIn this Application for Unit 2, you will write a program that uses some simple assignment statements and arithmetic to perform some coding ‘magic’. Skill Builders for Unit 2 should be completed prior to this activity.
Objectives:
- Write a program from given instructions.
- Use the assignment statement.
- Discover the mystery inside a program.
Step 1
Program mystery
Write a program that takes in two arguments, a and b, then:
- Adds a to b and stores the result in b
- Subtracts a from b and stores the result in a
- Subtracts a from b and stores the result in b
- Displays a and b
Store and test your program. What is the effect? Does it work with any two numbers?
At first glance it appears that the same value (see the subtraction instructions) is being stored in a and b. But, is it?
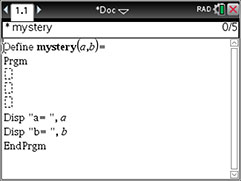
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 3: Conditional Statements
Skill Builder 1 Request and If
Download Teacher/Student DocsIn this first lesson for Unit 3, you will learn about Request(ing) input from the user while the program is running, strings, and the most basic (primitive) of conditional statements: If
Objectives:
- Use Request and RequestStr for input
- Investigate string variables and concatenation
- Write statements using If and conditions
- Use DispAt for better control of output
Step 1
Input Overview
Up to now, we’ve only been able to provide values in a program or function by using arguments. In the TI-Nspire™ CX, there are two statements that allow you to enter values into a program while it is running. These are known as ‘input’ commands:
Request “message”, variable (for numeric input)
RequestStr “message”, variable (for string input)
These statements are found on the I/O menu of the Program Editor.
.jpg?rev=abbda372-0cd9-487e-8eaf-424c4ab1f6b5&la=en&h=181&w=242&hash=E2558AD18ED341FCE9043CFFC3180FFE)
Step 2
Types of Variable input
- A numeric variable can contain a real or complex number, a list, or even a matrix. It can be used in algebraic expressions, and its value is used during the computation of the expressions.
- A string variable can contain any text including letters, digits, and most punctuation marks. It cannot be used in algebraic expressions. Note that numbers are allowed in strings.
- The “message” is called the ‘prompt’. It describes to the user what is supposed to be entered during the command’s operation.
- The variable can be any alphabetic character, including most Greek characters or a word, such as hours, that is not a reserved word. You will recognize a reserved word when the font style changes from italic to normal as you type it.
Step 3
String Concatenation
Two strings (variables or literals or a combination) can be combined into one longer string (concatenation) using the ‘&’ operator. This operator is accessed by pressing ctrl + .
Example on a Calculator app:
fname:=”John”
lname:=”Smith”
full:=fname & ” “ & lname
This results in the variable full to contain the string “John Smith”
.jpg?rev=49f760e8-05d8-49d4-b0c4-8106b595ac70&la=en&h=181&w=240&hash=CB489EA037945236AEE4CAAD52E816F4)
Step 4
Request and RequestStr
The program to the right contains no arguments and two Local variables name and age. There is no distinction between numeric and string variable names but there are two different statements for entering numeric and string data.
The RequestStr statement contains a prompt (“Name ?”) that is displayed so that the user know what to enter.
The Request statement (for age) concatenates the name from the first statement with the text “ ’s age?” using the & symbol. A space is not needed in front of the ‘s. Some refer to string concatenation as string ‘addition,’ but it is not a mathematical operation.
Note: Any variable name can contain an underscore character (e.g., can_vote). The underscore character is accessed by pressing .
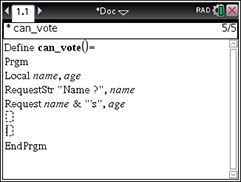
Step 5
RequestStr and Request each produce a dialog box containing the prompt and a field for entering your data.
To the right is the effect of the statement:
RequestStr “Name ?” , name
The user has entered ‘Mich’ so far.
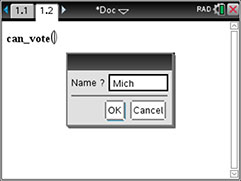
Step 6
Conditions
A condition is an expression that evaluates to true or false. Conditions use the relational operators. Select to display the relational operators selection box. For the equals sign, simply press
.
Conditions can also use the logical operators, and, or, not, and xor. These operators are found only in the Catalog. Also included are nand, nor, implies (⇒), if and only if (⇔), and an isPrime( ) function.
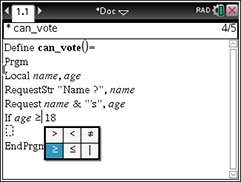
Step 7
Examples of Conditions:
- x > 0 and y > 0 (which is true when x is greater than 0 and y is greater than 0; note the spaces before and after the word ‘and’)
- hours > 40
- time ≤ 0
- age ≥ 18
- c² = a² + b²
- isPrime(n) (a built-in function)
Step 8
The ‘Primitive’ If Statement
The simplest form of all the If statements is
If condition
do only this statement
An example of this structure is found in the can_vote() program. When the value of the age variable is greater than or equal to 18, then the DispAt statement below it is executed; otherwise, it is skipped. This form of the If statement is useful for simple operations that do not require multiple statements or alternative actions (using else). Then is not used in this statement.
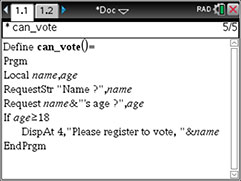
Step 9
DispAt
The DispAt statement (introduced in OS version 4.5) gives programmers greater control of the output in the Calculator app. When running a program, there are 8 lines of output area with which to work. Use the structure:
DispAt <line number> , <expression to display>
Note the comma after the line number:
DispAt 4, “Please register to vote, ” & name
The result of using DispAt in this program is seen to the right. Concatenation is used to append the user’s name to the message.
The two Request statements are echoed on lines 1 and 2 of the output area, and the “Please register…” message is displayed on line 4. Note the blank area, which is line 3.
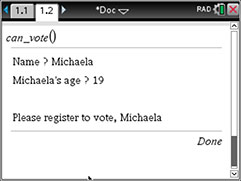
Step 10
Indenting
Note that the DispAt statement is indented. Indenting statements is permitted on the TI-Nspire™ CX. Indenting helps to make the code more readable. The DispAt statement has to be on the line directly below the If statement in this program.
Step 11
Running the Program
- After entering the program, select ctrl+R to prepare to run the program, and then select enter.
- The RequestStr statement displays a dialog box. Type a name, and select enter or click OK on the screen.
- The Request statement displays a message consisting of the user’s name and the word ‘age’ and expects a number as shown to the right. Type an age number, and select enter or click OK.
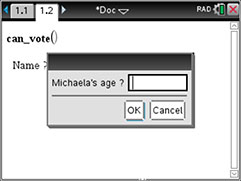
Step 12
The results of the program are displayed as output in the Calculator app. If the age is greater than 18, then the text ‘Please register…’ is displayed. Otherwise, as seen to the right, it is not displayed.
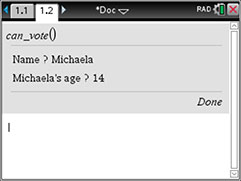
Skill Builder 2 If...Then statements
Download Teacher/Student DocsIn this second lesson for Unit 3, you will learn about If…Then…EndIf statements.
Objectives:
- Examine the If…Then…EndIf structure
- Make compound conditions with the relational and the logical operators
- Write a program using the If…Then…EndIf structure that examines the regions of the coordinate plane
- Use DispAt to control the location of output
Step 1
Up to now you have been writing programs that have been processing statements from top to bottom. This type of program is called a ‘sequence’ structure because the statements are processed in the order in which they are written. We now introduce programming statements that allow the program to follow one of two or more alternative paths through the code. This is sometimes known as ‘branching’ and is implemented with several types of If…Then statements.
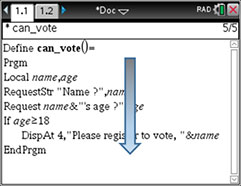
Step 2
- Start a new program, and enter a name for the program.
- Select menu > Control. As you can see from the image at the right, there are four different types of If statement templates provided in the TI-Nspire™ CX (items 1 through 4 in the menu). They are used to conditionally process statements.
.jpg?rev=cbcb4ed8-9fd4-4398-99ac-07064225c94e&la=en&h=181&w=239&hash=7B1C720B769BE1E7273A83D0BEFBF8E9)
Step 3
If…Then…EndIf
It is advisable to select these structures from the menu because all the needed components will be inserted into the code in the correct places. You will then go back and ‘fill in the blanks.’ The image to the right shows the immediate result of selecting If…Then…Endif from the Control menu:
If Then
EndIf
- After selecting If…Then…EndIf, your next task will be to fill in the condition (between If and Then) and the action (between Then and Endif).
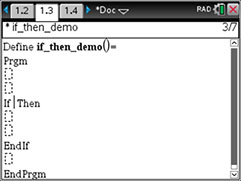
Step 4
A ‘Which Quadrant?’ Program
We’re going to write a more detailed program that lets the user enter values for the variables x and y, then determines in which quadrant the point (x,y) lies, and finally determines the signs of the coordinates in that quadrant.
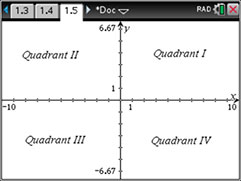
Step 5
The first part of the program is shown to the right.
- Above the If statement you will need to add two Request statements (one for x and one for y).
- Enter the condition (after If…) and action (between Then and EndIf) for Quadrant I as shown to the right.
Remember to separate the keyword ‘and’ from the surrounding text with spaces before and after the word. Select ‘and’ from the catalog or just type the keyword.
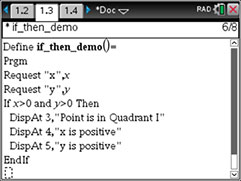
Step 6
- Modify the condition (after If…) and action (between Then and EndIf) for each of the other three quadrants. Y
You can copy and paste the entire If…EndIf structure, and then edit the block of code for each Quadrant.
Select text by holding down the shift key while arrowing over the text. Then press ctrl+C to copy, move the cursor, and press ctrl+V to paste the code just like on a computer. - After entering the conditions and actions for each Quadrant, test your program with many different values for x and y.
Are there any values that do not give the desired results? How would you address any issues?
Step 7
Remember to save your file so that your program is saved. The code for the entire program is shown below:
Define if_then_demo()= Prgm Request "x=",x Request "y=",y If x>0 and y>0 Then DispAt 3, "Quadrant I" DispAt 4, "x is positive" DispAt 5,"y is positive" EndIf If x<0 and y>0 Then Disp At 3, "Quadrant II" Disp At 4, "x is negative" Disp At 5, "y is positive" EndIf |
If x<0 and y<0 Then Disp At 3, "Quadrant III" Disp At 4, "x is negative" Disp At 5, "y is negative" EndIf If x>0 and y<0 Then Disp At 3, "Quadrant IV" Disp At 4, "x is positive" Disp At 5, "y is negative" EndIf EndPrgm |
Skill Builder 3 Using Else and Elself
Download Teacher/Student DocsIn this third lesson for Unit 3 you will learn about the Else clause and the use of ElseIf.
Objectives:
- Develop If…Then…Else statements so that there are actions for both the true and false values of the condition.
- Use the ElseIf clause to handle several different possible conditions in one block structure
Step 1
It is sometimes necessary to have one set of actions occur when a condition is true and some other set of actions when that same condition is false. (An example is the can_vote program from Skill Builder 1). This is where the Else clause comes into play.
After naming a new program, select the If…Then…Else…EndIf structure from the Control menu. All four keywords are inserted into the program. You will fill in the missing pieces of code.
.jpg?rev=9eda1a29-5768-4732-beb2-7a8afc13b0d0&la=en&h=181&w=240&hash=70E76795FDEF25C83DAA3E95D75F4172)
Step 2
The Pass/Fail Program:
In a college course there are three exams, and the course is pass/fail. An average grade of 65 is needed to pass. Write a program that accepts three test scores, computes the average of the scores, and finally displays either a “Pass” or “Fail” message.
- First, consider which method will be used to get the three scores into the program: arguments or Request statements?
- How will the average grade be computed?
Step 3
- For this program, enter three arguments for the three test scores.
.jpg?rev=5735bc31-99e8-4637-81d8-210ec8baae2a&la=en&h=71&w=240&hash=A74479F335305E0D845E3F69BDE0A593)
Step 4
- The average of the three scores is defined as the sum of the scores divided by 3:
avg:= (t1 + t2 + t3) / 3.
Use a decimal point after the 3 to ensure that the result is displayed in decimal form. Without a decimal point, the average may be displayed as a fraction.
- Use two DispAt (from menu > I/O) statements to show the scores and the average:
DispAt 1, ”Scores:”, t1, t2, t3
DispAt 2, ”Average:”, avg
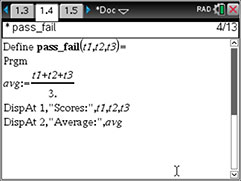
Step 5
- Select the If…Then…Else…EndIf structure from menu > Control. Complete the If structure to determine if “Pass” or “Fail” will be displayed.
- Use DispAt from menu > I/O for each result to enter the appropriate message.
If avg≥65 Then
DispAt 3,"- - - -"
Else
DispAt 3,"- - - -"
EndIf
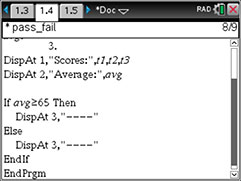
Step 6
The Discriminant Program
- Write a program that takes the values of a, b, and c in the standard form of a quadratic function, f(x) = a*x² + b*x + c, and
- Computes the discriminant: d := b² – 4*a*c
- Displays the number of distinct real roots:
If d < 0, there are no real roots
If d = 0, there is one real root
If d > 0, there are two real roots
Step 7
- To build the structure using ElseIf…Then:
First, place the
If Then
Else
EndIf
structure.
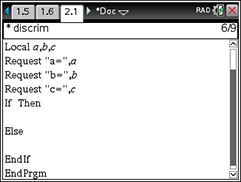
Step 8
- Next, place your cursor inside the Then…Else block, and paste the ElseIf…Then statement. This ensures that all the necessary components are in the correct place.
- Complete the program as described above.
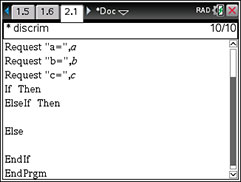
Application ZODIAC
Download Teacher/Student DocsIn this Application for Unit 3, you will learn about the signs of the zodiac and write a program that will tell your sign. Skill Builders for Unit 3 should be completed prior to this activity.
Objectives:
- Use If… Then… Elseif… Then… Else… Endif in a program.
- Work with date conversions (dbd() function)
Step 1
The Zodiac
In astronomy and astrology, the zodiac is a division of the sky into 12 equal regions. The regions are named by the constellations that are approximately within these regions. The Babylonians developed this division around 1000 - 500BC, and they began their calendar year with the vernal equinox (the first day of Spring).
The Program
In this activity, we will write a program that prompts the user to enter a month number and a day number (according to our calendar). The program will determine the day number of the year (e.g., April 14 is the 104th day of the year) and use If statements to determine the correct Zodiac sign. The program then displays the sign of the zodiac for that date.
Step 2
We first need to convert the dates in the table below into day-of-year numbers. On the TI-Nspire™ CX, we can use the dbd( ) function:
dbd(12.3117,03.2118) returns the number of days from
Dec 31, 2017 (12.3117) to March 21,2018 (03.2118).
As seen in the image to the right, March 21 is the 80th day of the year.
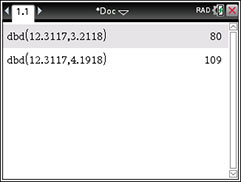
Step 3
Complete the two day# columns of the table below using your TI-Nspire™ CX and the dbd( ) function. This function returns the number of days between day 1 and day 2.
The date intervals shown for each sign of the zodiac correspond to those found in many horoscopes:
Sign | Interval | From day# | To day# |
---|---|---|---|
Aries: | March 21 – April 19 | 80 | 109 |
Taurus: | April 20 – May 20 | 110 | |
Gemini: | May 21 – June 20 | ||
Cancer: | June 21 – July 22 | ||
Leo: | July 23 – August 22 | ||
Virgo: | August 23 – September 22 | ||
Libra: | September 23 – October 22 |
Step 4
(Continuation of table from previous page)
Sign | Interval | From day# | To day# |
---|---|---|---|
Libra: | September 23 – October 22 | ||
Scorpio: | October 23 – November 21 | ||
Sagittarius: | November 22 – December 21 | ||
Capricorn: | December 22 – January 19 | ||
Aquarius: | January 20 – February 18 | ||
Pisces: | February 19 – March 20 |
Step 5
In our program we need to convert a user-entered month and day into the format MM.DDYY that the dbd( ) function requires.
You can do this by:
daycode := month + day/100 + .0018 (for 2018)
Then we can determine the day number by:
daynum := dbd(12.3117, daycode) (for # of days since 12/31/3017)
To the right is a test program that only displays the daycode and the daynum values. This test assures us that these expressions are working properly.
Optional: Input the year as well and use that variable in the two formulas above.
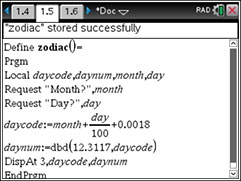
Step 6
We’re now ready to write the rest of the zodiac program. The program utilizes the If…Then…Else…EndIf and ElseIf…Then structures.
Build one long If…Then…ElseIf…Then…ElseIf… statement to decide which sign is correct. Complete the missing parts of the program.
If daynum >= 80 and daynum <= 109 Then
zodsign := "Aries"
ElseIf daynum >= ... and daynum <= ... Then
zodsign := "Taurus"
ElseIf.... Then
…
EndIf
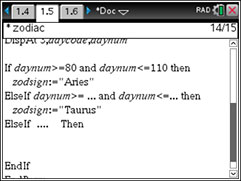
Step 7
Each ‘Then action’ stores the corresponding sign into the variable zodsign. This provides another opportunity to take advantage of Copy and Paste. However, be careful with Capricorn.
Test your program thoroughly referring to the Zodiac chart above. At the end of all the If statements, simply display zodsign.
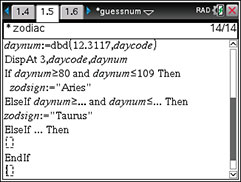
Step 8
If the dbd( ) function receives invalid information (such as month 13 or day 45) then an error occurs and the program terminates.
In order to be sure valid information is entered, we will need to build a loop to repeatedly ask for the data until good numbers are entered. We’ll discuss loops in the next unit.
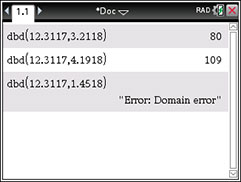
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 4: Loops
Skill Builder 1 For Loops
Download Teacher/Student DocsIn this lesson, you will learn about the loop concept in programming and examine the For…EndFor loop
Objectives:
- Describe the concept of looping in programming
- Write programs and functions using the For…EndFor structure
- Control the amount of output space (scrolling) taken using DispAt
- Using getKey(1) as a 'pause' command
Step 1
About Loops
The TI-Basic programming language has the ability to process a set of statements over and over. This repetition of statements is called looping.
The three loop structures you will learn in this Unit are each accessed by selecting menu > Control from the Program Editor menu. (See For, While, and Loop to the right.)
The While . . . and Loop . . . structures will be explored in later lessons in this Unit. For more information about additional Control structures in this menu, see the Reference Guide at the TI Codes website.
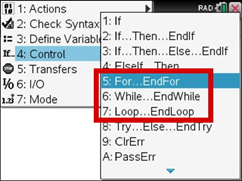
Step 2
For…EndFor
The For…EndFor loop is used to process an arithmetic sequence of values. This process is known as ‘iteration’.
Selecting the For…EndFor statement from the control menu gives you the necessary components for building the rest of the structure:
For , ,
EndFor
The commas after the word For indicate that you need to provide at least three components. An additional comma and a fourth component can be added for the increment value. (Note: If the increment value is 1, the fourth component can be omitted.)
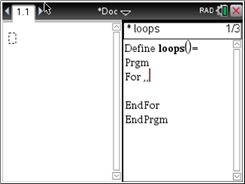
Step 3
Here's an example:
For i, 1, n, 1
Disp i, i²
EndFor
- i is the loop control variable: The first item must be a variable.
- 1 is the starting value: Each time the loop is processed it will take on values, or count, from the specified start value (1).
- n is the ending value: Each time the loop is processed it will take on values, or count, to the specified end value (n).
- 1 is the increment value: Each time the loop is processed it will take on values, or count, from the start to the end value by the specified increment value (1).
You can also read the For statement as "For i going from 1 to n by 1s"
Any or all of the last three components can be numbers, variables, or expressions.
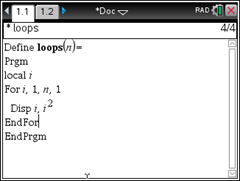
Step 4
Write the Program
Enter the code into a program using an argument (n), and run it.
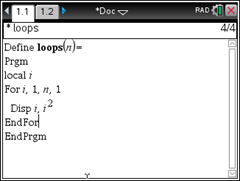
Step 5
Running the Program
Running the program produces the results shown to the right (in a split screen to see the code and the result).
- The value for n (5) is an argument to the program.
- The loop control variable is a Local variable; it does not affect the rest of the problem.
- The loop begins with i=1 and Displays the values of i and i2.
- After the Disp statement, the EndFor statement passes control back to the For statement where the increment (1) is added to i.
- If the resulting value is less than or equal to the end value, the loop is processed again with the new value for i.
- This process is repeated until the end value is surpassed.
After the loop ends, i will be larger than the end value. Add another Disp i statement after the EndFor statement to see for yourself.
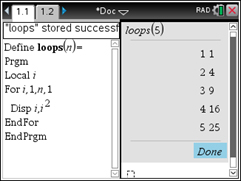
Step 6
Large Output and DispAt
When you use a ‘large’ argument in this program, such as loops(10) or loops(100), the output is a long list and is challenging to inspect by scrolling.
You can use DispAt 1, i, i2 to place all output information on the same line of the screen. Try it.
DispAt is located in menu > I/O.
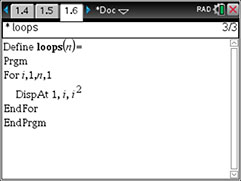
Step 7
The output is displayed too fast to observe each pair of values. There are two ways to fix this problem.
One Fix for DispAt
There are two ways to control the speed of the output. The first is:
Wait <seconds>
Wait is located in menu > Control. (Since Wait is the last option, use the up arrow.)
Adding Wait 3 after DispAt tells the program to wait or pause for three seconds after each new set of values is displayed.
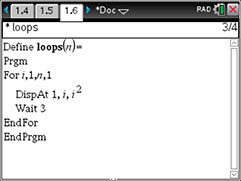
Step 8
Another Fix for DispAt
The second method for controlling the speed of the output is:
getKey(1)
getKey( ) is located in menu > I/O.
Adding getKey(1) (you have to type the number 1) after DispAt pauses the program until a key is pressed.
Consider using a message such as DispAt 2, “Press any key to continue” before the getKey(1) function. This gives the user greater control than Wait.
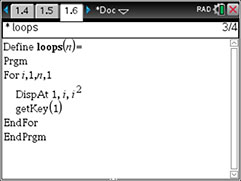
Skill Builder 2 While
Download Teacher/Student DocsIn this lesson, you will learn about the most versatile of the loops, While…EndWhile loop.
Objectives:
- Write a simple While loop
- Use the While loop to ensure valid data entry
Step 1
The While…EndWhile loop (menu > Control) continues to loop as long as its <condition> is True. The structure looks like this:
<initialize the condition>
While <condition>
<loop body>
EndWhile
- Initialize refers to setting up one or more variables so that the While statement can properly evaluate the condition the first time. The initialization establishes a value of True or False for the variable(s). If the initial condition is False, the loop is skipped. If the condition is True, then the loop body is processed.
- The <condition> is a logical expression such as X>0.
Step 2
- The <loop body> is any set of statements, including other loops and If structures. The <loop body> is processed whenever the <condition> is True.
- Somewhere in the <loop body>, there must be a statement or statements that alters the <condition>. Without a chance in the condition, you will create an infinite loop - a loop that never ends.
- The keyword EndWhile is used to indicate the bottom of the <loop body>. At the EndWhile statement, the program loops back to the While statement and tests the <condition> again. If the condition is False, the loop is finished. If the condition is True, the loop body is processed again.
Step 3
This split-screen to the right illustrates a While loop and its output.
The assignment statement k:=1 at the top of the program sets the initial condition to a known False value so that the loop will be processed. Without this initialization, the variable k could be undefined or contain any stored value, introducing an unknown value into the program.
Somewhere inside the <loop body>, there should be a statement that will have an effect on the <condition> so that the loop will eventually end and the statements after the loop can be processed. Usually this statement is near the bottom of the <loop body>. k:=k+1 ensures that eventually k will be greater than n.
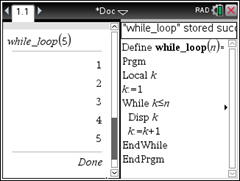
Step 4
The program demonstrates the While loop equivalent of the following For loop:
For k,1, n Disp k EndFor |
k:=1 While k ≤ n Disp k k:= k + 1 EndWhile |
This illustrates the power and convenience of using a For loop which is more compact.
Step 5
Checking for Valid Input with While…EndWhile
Write a section of a program (a ‘code segment’) that makes sure that the user enters a positive number, tells the user when an entry is invalid, and prompts the user to enter another value in its place.
The output of the code segment is shown to the right with some non-positive numbers entered to see the effect.
Try to write the statements for While…EndWhile structure without peeking at the next page!
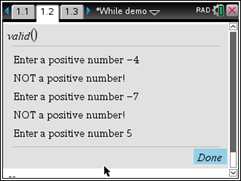
Step 6
- We begin by starting a new program. The name used here is valid.
- Create a Local variable n, and use the Request statement to get a value from the user. Note that the prompt asks for a positive number.
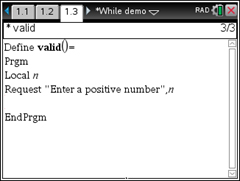
Step 7
- Insert the While structure from the menu > Control menu. Both the While and EndWhile commands are pasted into the code, and the cursor appears after the word While so that the condition can be entered.
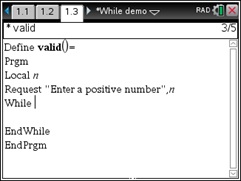
Step 8
- Type the condition n≤0.
The ≤ can be accessed by selecting.
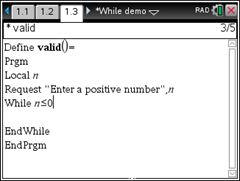
Step 9
-
Finally, complete the loop body by providing an error message. Use the Text statement (menu > I/O) to display "Not a positive number!"
The Text statement pauses the program and displays the promptString in a dialog box.
- Enter another Request statement (menu > I/O) to let the user enter a value for n again.
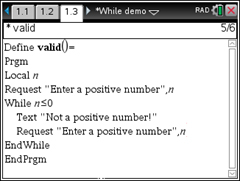
Step 10
The final program valid( ) to the right produced the interaction seen earlier in this lesson.
Notice the two identical Request statements.
- The first is used to initialize the condition (n≤0). If a positive number is entered at this point, then the loop will not be processed at all.
- However, if 0 or a negative number is entered, then the loop body displays the error message (Text) and Requests another value.
- The Request statements do not have to be identical but they both do have to get a value for the same variable. Can you combine the Text and Request statements in the loop body into one statement?
The loop will continue as long as a non-positive value is entered.
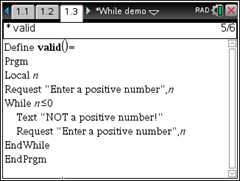
Skill Builder 3 The Loop loop
Download Teacher/Student DocsIn this lesson, you will learn about the all-purpose Loop…EndLoop structure.
Objectives:
- Use the Loop…EndLoop structure
- Use the Exit statement to get out of a loop
Step 1
What is this thing called Loop…EndLoop?
The Loop…EndLoop structure creates a more flexible (but possibly hard-to-follow) loop. It repeatedly executes the statements in the loop body. Note that this loop will be executed endlessly, unless an Exit instruction is executed somewhere inside the loop body.
If no Exit statement is encountered, then the loop will be an ‘infinite’ loop. If you accidentally run into an infinite loop on the handheld, press and hold until the program ‘breaks’.
The Loop…EndLoop structure is processed at least once since there’s no condition to be met for its entry.
Exit forces program flow to process the statement immediately after EndLoop.
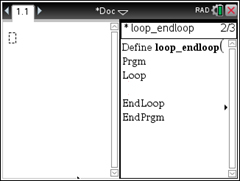
Step 2
Example: How many random numbers from 1 to 6 can be generated before two consecutive values are the same?
In the program to the right*, observe the use of Loop…EndLoop with a conditional Exit statement. When the random integer generator randInt(1,6) produces two consecutive identical values, then the loop Exits to process the Disp statement at the bottom of the program.
The reserved word Exit is located on menu > Transfers.
The same effect can be accomplished with a While loop. Think about how you could do this.
* An enlongated image was used here to show the entire program which would not fit in one screen.
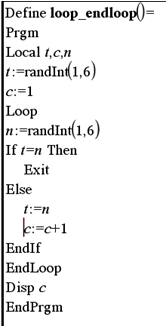
Step 3
Program: Guess My Number
To demonstrate the use of Loop…EndLoop, we’ll develop a two-player game to guess a random number from 1 to 10. When a player guesses the number, then the program ends with a ‘winner’ message. Sample output of such a program is shown to the right.
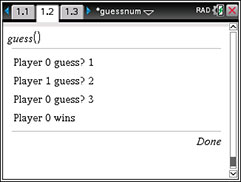
Step 4
- Start a new program called guess.
- Start by setting up (initializing) the game. Identify three variables, the player number (player), the computer’s or handheld's number (number), and the player’s guess (guessnum).
- The computer picks a random number from 1 to 10. We start with the player numbered 0. This makes it easy to change the player number as we’ll soon see.
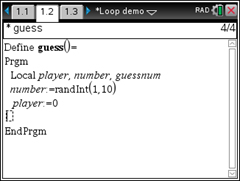
Step 5
-
Next build the loop where we first ask the player to enter a guess. The complete statement is:
Request "Player " & string(player) & " guess?", guessnum
The ‘&’ symbol is for concatenation of strings. The prompt portion of a Request statement must be a string so the player variable (a number) is converted to a string with the string( ) function found in the Catalog.
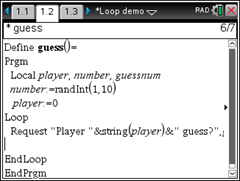
Step 6
-
Next build the Exit condition. When a player guesses the number, then the loop will Exit. Use the primitive If statement here:
If guessnum=number
Exit
Recall that If without Then processes the next statement when the condition is true; otherwise, the next statement is skipped.
These two statements can be placed on the same line by using a colon (:) to separate the statements: If guessnum = number : Exit
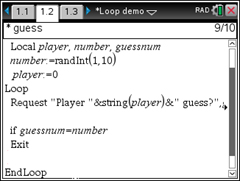
Step 7
-
To switch players, we use the clever statement:
player:= 1 - player
This unique statement switches the value of player between 0 and 1. That is, when player is 0 the statement changes it to 1 and when player is 1 the statement changes it to 0. Try it!
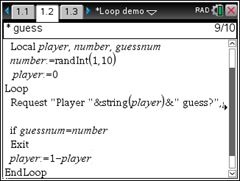
Step 8
-
Finally, add in a Text statement after the loop to congratulate the winner:
Text “Player” & string(player) & “wins!!”
Tip: If you don’t like seeing ‘player 0’ and ‘player 1’, then just add 1 to the player variable in this statement and in the Request statement. The user sees a 1 or a 2 even though the computer uses 0 and 1 for the players.
- Before running the program, save the document in case the program becomes stuck in an infinite loop. Once the program begins, you must play until there’s a winner. There is no escaping the Request statement.
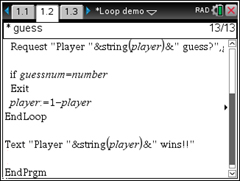
Application Bank Notices
Download Teacher/Student DocsThis application, "Bank Notices," makes use of loops to enter as many values as needed and, as an extension, checks for valid values entered and uses If statements to display an appropriate message.
Objectives:
- Use Counter and Accumulator statements
- Use a loop in a program to get an undetermined amount of data
- Use a ‘flag’ value to terminate a loop
Step 1
An Example of Nested Structures
- Nesting is the programming technique of placing one control structure inside another. The term is derived from the idea of placing one cardboard box inside another in order to save space.
- A programmer places loops within loops, such as Ifs inside loops and loops inside Ifs, to accomplish more complex tasks as the program requires.
- It’s important to put one complete structure completely inside a block of another in order to avoid errors.
- The program listing at the right shows a While loop and an If structure inside another While loop.
- Notice the multiple uses of the EndWhile statement; the program 'knows' which EndWhile belongs with which While.
- The indenting is for visual confirmation and helps to clarify the logic.
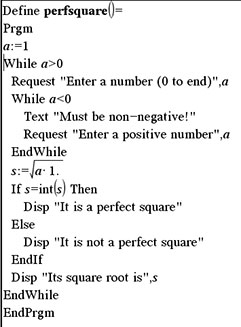
Step 2
The program first sets up a loop to continue until the entered value is zero. Then it tests to see if a<0. If it is, the program displays “Must be non-negative!” and asks for another value for a. But when a is not negative then the square root calculation and an If statement is processed and then the Disp statement is executed. When 0 is entered the program ends.
Step 3
Summary of the Three TI-Nspire™ CX Loops:
For(var, start, end) | While <condition> | Loop If <condition> : Exit |
EndFor | EndWhile | EndLoop |
For( is used when ‘counting’ or processing an arithmetic sequence of values (iteration).
While is used when you might be able to skip the loop body completely.
Loop is used when you are certain that you want the loop body to run at least once and it must contain an Exit statement, usually as part of an If statement.
Step 4
Unit 4 Application: Program “Bank Notices”
A bank customer can have several bank accounts at a certain bank. The bank requires a minimum average balance of $1000 in all the accounts to avoid paying service charges.
If the average is between $1000 and $1250, then the bank sends the customer a notice with a warning of the potential of a service charge.
When the average moves above $1250, the bank sends the customer a thank you message for maintaining a good average balance.
Let’s write a program that gives the user information about their accounts. The user will enter account balances. The program will determine the average balance amounts, and display the number of accounts, the average of the balances, and an appropriate message to the user. The messages can be: “SERVICE FEE CHARGED”, “IN DANGER OF A SERVICE FEE”, and “THANK YOU FOR YOUR BUSINESS!”.
Step 5
We can use two methods for entering an unknown number of values:
- Method 1: Ask for the total number of accounts first and use a For…EndFor loop to enter the values.
- Method 2: Ask for amounts, but use a ‘flag’ value such as -999 to indicate that there are no more accounts. This method will use a While…EndWhile loop.
In both methods, we will have to keep a running total of the values entered.
In Method 2, we also have to count the number of account balances so that we can divide the total by that count.
Your program should display 1) the number of account balances entered, 2) the average of the account balances, and 3) the appropriate message based on the balance average.
If the average is below 1000: “SERVICE FEE CHARGED”
If the average is 1000 to 1250: “IN DANGER…”
If the average is above 1250: “THANK YOU…”
Step 6
Counters and Accumulators
A statement such as c:=c+1 is called a ‘counter’ because it adds 1 to the variable c each time it is executed.
A statement such as t:=t+n is called an ‘accumulator’ because it keeps a running total of the values of the variable n. The value of n is added to the variable t and then that sum is stored back into the variable t. At the end of a loop, t will contain the total of the n values encountered.
Here’s an example that uses a counter, an accumulator (total), and a ‘flag’ value (-999) to keep track of the number of amounts entered in a program:
Step 7
Prgm | Notes | Sample Output |
Local counter,amount,total total:=0 counter:=0 Request "Amount?",amount While amount≠−999 counter:=counter+1 total:=total+amount Request "Amount?",amount EndWhile Disp "Count=",counter Disp "Total=",total endPrgm |
Initialize variables get first amount as long as it is not -999 add 1 to the counter add the amount to the total ask for another amount |
![]() |
The While…EndWhile loop above continues counting and accumulating the amounts as long as -999 is not entered. When -999 is entered, the loop stops, and the results are displayed.
Step 8
Extension
As part of your input routine, check to make sure that the value entered is a legitimate amount (greater than 0), and take appropriate action when the entered value is not legitimate.
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 5: Lists, Graphs, and Dynamic Programs
Skill Builder 1 Programming with Lists
Download Teacher/Student DocsIn this lesson, you will learn about using Lists in programs to produce interesting, interactive data graphs.
Objectives:
- Describe the basics of lists in programs
- Write programs that use lists to create scatter plots
Step 1
TI-Basic does not provide direct graphical statements. A program can define functions for graphing and can create lists to produce scatterplots, but cannot plot points directly.
In this unit, we will develop three big ideas:
- working with lists in a program;
- setting up a Graphs app to display a scatter plot of those lists; and
- using 'dynamic' programs that can run 'on demand'.
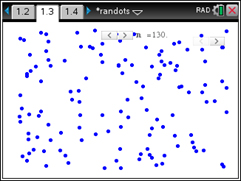
Step 2
Defining Lists
Lists are defined using the curly braces, { }. To create an empty list, use a statement like mylist:={ } with nothing between the braces.
The list elements (values between the braces) are addressed using square braces after the list name, such as in mylist[3], which refers to the third element in mylist.
Add elements to a list by storing a value in the position immediately after the last value of the list. For example, if a list contains three elements such as {12, 7, 2} then you can add an element to this list by storing a value in list element number [4]. Entering mylist[4]:=17, results in mylist = {12, 7, 2, 17}. Knowing how many elements are in a list is very helpful. dim(listname) tells how many elements are in the list (the dimension of the list). This command is often used to add an element to the end of a list. For example:
mylist[Dim(mylist)+1]:=<some value>.
Step 3
Programming Random Dots
We’ll begin by writing a program that will create a random dot pattern in a Graphs app.
1. Create a new program (we called it randots), and use the argument n.
- n represents the number of dots the program will create.
- The program will create (or revise) two ‘global’ lists of random numbers for use in a scatter plot in a Graphs app.
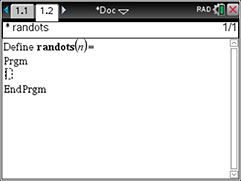
Step 4
Random Number Generators
There are several random number functions in the TI-Nspire™. The two most common are rand() and randInt().
- rand() creates a random decimal between 0 and 1.
- randInt(a,b) creates a random integer between a and b inclusive. Example: randInt(1,6) creates a random number from 1 to 6, inclusive. Try this command in a Calculator app.
Step 5
Our first try at a list of random numbers:
xs := randInt(-10, 10, n)
ys := randInt(-6, 6, n)
- The third argument n in the two variable assignments above cause the randInt( ) function to create a list of n random integers in the specified interval rather than a single random number.
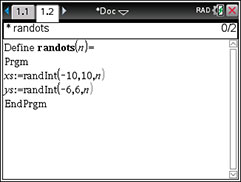
Step 6
- Select ctrl+R to store and prepare to run the program. In the Calculator app, run the program with a small argument such as 5, and observe the values of xs and ys. Your values will probably differ from the ones to the right.
Note: To get a split screen with the Calculator app on the right from the Program Editor, select doc > Page Layout > Select Layout, and choose the vertical split icon (Layout 2). Add the Calculator app to the new window on the right.
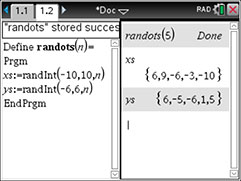
Step 7
- Add a Graphs app to this problem, and set up a scatter plot of (xs,ys) by selecting menu > Graph Entry/Edit > Scatterplot.
- Enter xs for the x← field, use the down arrow to move to the y← field, and enter ys.
- Press enter to plot the points.
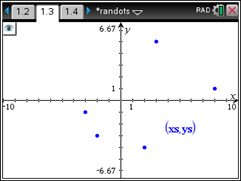
Step 8
Do you see why we chose those intervals for the random numbers functions? Study the Standard Window settings using the menu > Window/Zoom > Window Settings.
- Now that we’ve tested the program, go back to the Calculator app, and rerun the program with a larger (but not too large) value for n.
- Return to the graph to see the results.
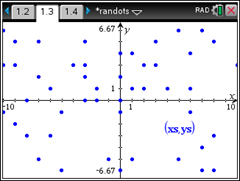
Skill Builder 2 Lists and Math Boxes
Download Teacher/Student DocsIn this lesson, you will learn about controlling individual elements in lists and discover the method of running a program dynamically from a Notes app Math Box.
Objectives:
- Generate random decimal values on a desired interval
- Use the 'interactive' property of Math Boxes in a Notes app to make a program run dynamically.
Step 1
In the previous lesson, we wrote a program that generates two lists of random integers and displays those lists in the form of a scatterplot in a Graphs app. Recall the output of the randots(n) program. A sample is shown to the right.
The randots( ) program was limited to generating only integral values for the x- and y-coordinates of the scatterplot so all the points are located on the grid points of the graph. In this activity, we'll extend the program to include non-integer values so that the plot will be more densely packed.
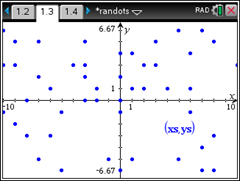
Step 2
From Integers to Decimals
Rather than use randInt(-10, 10, n) we will use the rand( ) function to generate a decimal (between 0 and 1) and then ‘scale’ the decimal so that the points fill the screen (using the Standard window).
First, let's examine the rand() and rand(n) function outputs in a Calculator app. Notice that without an argument, rand() generates a single random decimal between 0 and 1. With an argument of 3, however, it gives a list of three decimals between 0 and 1. This random list can then be used as part of an expression to change those values to go beyond the range of 0 and 1. For example, 5*rand(3) produces a list of three random decimals between 0 and 5.
We need to use this knowledge to generate a random decimal between -10 and 10. That’s a range of 20 units. We’ll start the expression with -10, and add a random value from 0 to 20 using 20*rand( ).
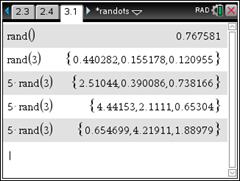
Step 3
Modifying the randots(n) Program
- In our randots(n) program, change the randInt functions to an expression using rand( )
xs:= -10 + 20*rand(n)
ys:= -6 + 12*rand(n)
These expressions generate n decimal values of xs between -10 and 10 and n values of ys between -6 and 6.
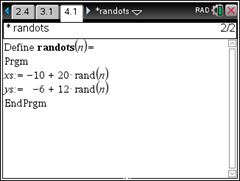
Step 4
- Run the program in a Calculator app, and observe the values of xs and ys.
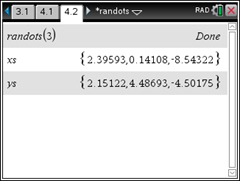
Step 5
- Finally, observe a scatterplot of (xs,ys). We used randots(3) for the image to the right.
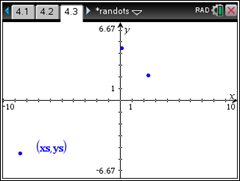
Step 6
- Run the program again with a larger value of the argument. randots(50) was used for the image to the right.
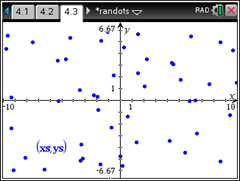
Step 7
Dynamic Programs
- To have the program run dynamically, add a Notes app to the problem.
- Insert a Math Box into the Notes app by selecting menu > Insert > Math Box.
- Type the program name (or use the var key) with a variable (we used k) as the argument into the Math Box, and press enter.
- An error message is displayed because the argument is an undefined variable. This will be resolved shortly. Press enter or click OK to dismiss the dialog box.
- The actual parameter you use does not have to be the same as the argument that you used in the Program Editor. It represents the value that is ‘passed’ to the program’s argument.
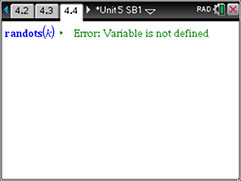
Step 8
- In the Graphs app containing the scatter plot, insert a slider (menu > Actions > Insert Slider).
- Important! In the Slider Settings box, make the variable name for the slider the same as the argument you used in the Math Box. Recall that we used the variable k.
- Set the value to 1, the Minimum to 1, and Maximum to 100, and the Step size to 1 as in the image to the right.
- Press enter or click OK to place the slider on the Graphs app.
- Move the slider to a convenient location, and press enter or click again to place it.
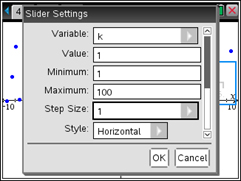
Step 9
You will see that all the dots disappear except for one. This is a result of the program responding to the new value for k (1 in our demo) causing the program to respond from the Math Box in the Notes app.
If you look at the Notes app, you’ll see that the error message has been replaced by the word ‘Done’. The program is running properly now that the argument k is defined.
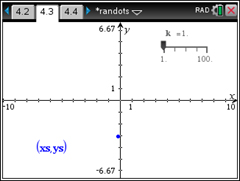
Step 10
- In the Graphs app, operate the slider for k. Each time the value of k changes it causes the program to rerun, thus generating a whole new set of points for the scatterplot. This 'dynamic' nature of programs residing in 'interactive' Math Boxes is a very powerful feature of the TI-Nspire™
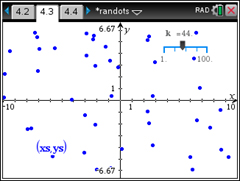
Skill Builder 3 Simulations
Download Teacher/Student DocsIn this lesson, you will learn about using dynamic programs to produce other types of graphs using the Data & Statistics app.
Objectives:
- Write a program that dynamically updates a graphical display
- Write a program that simulates a real-world occurrence (tossing a pair of dice) and plot the results in a meaningful graph
Step 1
Dynamic programs can produce much more than random (or not-so-random) scatter plots.
In this lesson, we’ll develop a demonstration of the distribution of the sum of two fair 6-sided dice when they are rolled a large number of times.
When you toss a pair of 6-sided dice, the sum of the values on the two dice can range from a low of 2 (by rolling two ones) to a high of 12 (by rolling two sixes). But, are the eleven totals all equally likely, or do some totals occur more often than others? Let’s develop a TI-Nspire™ CX activity that allows us to simulate the experiment using a program and a graph.
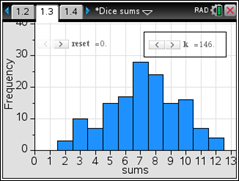
Step 2
- Begin a new Problem either by starting a new document or select doc > Insert > Problem.
- Select Add Program Editor > New… from the menu, and name the program.
- The program contains just one statement and no argument:
sums:=randInt(1, 6, k) + randInt(1, 6, k)
Two global variables will be established when the program runs:
k represents the number of tosses of the pair of dice (trials).
sums is a list holding each of the k sums of the tosses (outcomes).
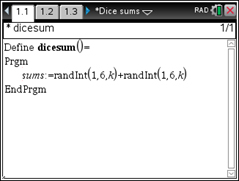
Step 3
- Press ctrl+B to store the program. Do not run it yet.
- Insert a Notes app by selecting doc > Insert > Notes or by selecting ctrl+doc or ctrl+I and then selecting Add Notes.
- Insert a Math Box by selecting menu > Insert > Math Box.
- Type the name of the program and a set of parentheses, and press enter.
Don’t be surprised to see an error message since the program is being run and the global variable k in the program has no value yet.
- Press enter, or click OK.
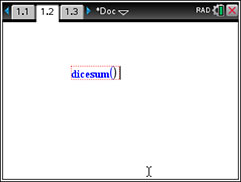
Step 4
- Insert a Data & Statistics app by selecting doc > Insert > Data & Statistics or by selecting ctrl+doc or ctrl+I and then selecting Add Data & Statistics.
- Insert a slider (menu > Actions > Insert Slider) for the variable k with a value of 1, a minimum of 1, a maximum of 500, and a step size of 1.
The variable k represents the number of times the dice are tossed (trials). - Click OK, and then place the slider near the top of the page.
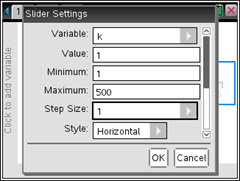
Step 5
Now that k is defined, the program runs from within the Notes app. You will see ‘Caption: sums’ at the top of the app and a dot representing the sum of a pair of dice.
Don’t use the slider yet!
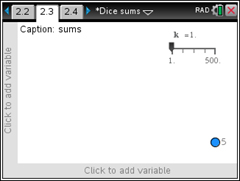
Step 6
- Click the text 'Click to add variable' at the bottom of the app, and select sums for the horizontal axis.
- The result is a vertical 'dot plot' of the sums list.
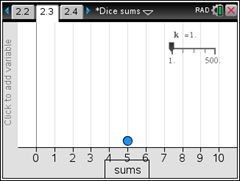
Step 7
- Change the plot type to a histogram by selecting menu > Plot Type > Histogram.
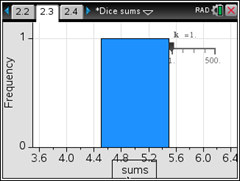
Step 8
- Now activate the slider to generate more sums.
- As k increases, you will have to adjust the viewing window by selecting menu > Window/Zoom > Zoom Data.
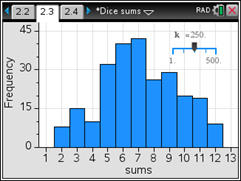
Application The Sierpinski Gasket
Download Teacher/Student DocsIn this Application, we will develop a project to play the ‘Chaos Game’ that generates the Sierpinski Gasket, a famous fractal.
Objectives:
- Write a program that generates an interesting image (the Sierpinski Gasket)
- Control the permitted actions allowed on a slider control
Step 1
The Sierpinski Gasket
Step 2
Another way of generating the Gasket that we will use in this project is called the Chaos Game. The rules are:
- Select three (3) points in a plane to form three vertices. For the program described below, the selected points are (0,0), (2,0), and (1,1). (We understand that this is not an equilateral triangle.)
- Start by randomly selecting any point (preferably inside the triangle but this does not matter), and consider that point to be your ‘current’ position.
- Randomly select any one of the three vertex points.
- Move half the distance from your current position to the selected vertex (that is, calculate the midpoint of your current position and that vertex).
- Plot this new current position.
- Repeat from step 3.
We will use a growing scatter plot to visually represent this game. Along the way, we’ll learn how to reset a scatter plot and how to prevent a slider from going backwards (disabling the left arrow or down arrow part of a minimized slider).
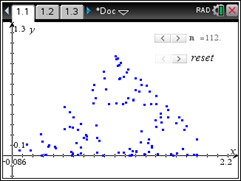
Step 3
Set Up the Graphs Page
- Start a new document, and add a Graphs app.
- Move the origin to the lower left corner of the page by “grabbing” the graph at an empty location on the screen. Change the x- and y-axis values so that they are similar to those shown to the right. (Remember: The triangle’s vertices will be (0,0), (2,0), and (1,1).) The axes end values are subjective and can be adjusted later.
It's a good idea to save your document now and to save often using ctrl+S throughout this project in case something goes awry along the way.
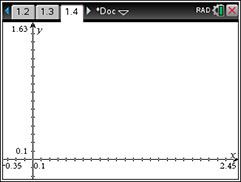
Step 4
- Set up a scatter plot of xs and ys (menu > Graph Entry/Edit > Scatter Plot).
These variables are not yet defined so no graph will appear when you press enter. Also, there will be a message related to creating sliders for xs and ys, but at this point, click on cancel.
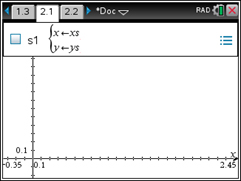
Step 5
- Insert two sliders (menu > Actions > Insert Slider):
- n – value 0, minimum 0, maximum 2000, step size 1, horizontal, and minimized
- reset – value 0, minimum 0, maximum 1, step size 1, horizontal, and minimized
- Move and place these sliders in the top right corner of the screen so that they do not interfere with the triangle.
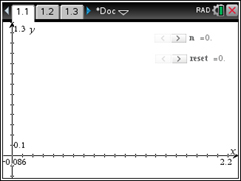
Step 6
Writing the Program
- Insert a page (ctrl+doc), and select Add Program Editor from the menu. Select New….
- Name the program sierpinski.
- Do not add any arguments.
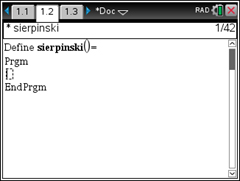
Step 7
- Include the Local statement here in case any auxiliary variables are needed later in the program. Use the variable xxx as a placeholder for now.
- Enter the If…Then…EndIf structure as shown:
If n=0 or reset=1 Then
xs:={ }
ys:={ }
reset:=0
n:=0
EndIF
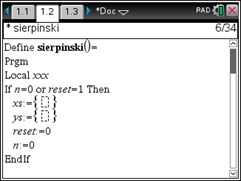
Step 8
- The ‘reset’ actions should occur when the program first runs (n = 0), so we include this condition as part of the first If statement.
- When the reset slider is used, all the data in lists xs and ys are erased (assigned an empty set), and the variables reset and n are both set back to 0.
- Setting reset:=0 in this part of the program might seem like an unusual choice, but the result is that the value of reset on the screen will always appear to be 0.
- Clicking the reset button causes reset to become 1, forcing the program to run. The program immediately sets the value of reset back to 0 (along with the other commands in this part of the program).
Step 9
We want the value of n (the number of points plotted) to increase and not decrease. Do this by keeping track of the last (previous) value of n and compare it to the new (current) value of n. If the new value is less than the last value, it will be ignored by setting n to be the last value.
- Use the variable lastn to hold the previous value of n. This should be entered before EndPrgm:
lastn:= n
EndPrgm
Step 10
- Also, initialize lastn in the first If structure:
lastn:= 0
The next portion of code checks to see if n is less than the previous n (when the left arrow of the n slider is clicked).
- After the initializing section, add:
If n < lastn Then
n:= lastn
Else
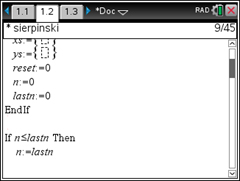
Step 11
- Next, after the Else statement, build the lists for the scatter plot.
By the rules of the Chaos Game (rule 2), there first needs to be a random number selected. The first data point can be any point. So, if n is 1, we then assign a random number to xs[1] and ys[1]:
If n = 1 Then
xs[1]:= rand()
ys[1]:= rand()
Else
Recall that the rand() function generates a random decimal from 0 to 1. The x- and y-coordinates will both have a value between 0 and 1.
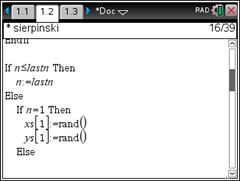
Step 12
We’re now ready to tackle the heart of the algorithm mentioned at the start. We’ll repeat it here to refresh our memory of these previously stated rules from the Chaos Game:
- Randomly select any one of the three vertex points.
- Move half the distance from your current position to the selected vertex (that is, calculate the midpoint of your current position and that vertex).
- Plot this new current position. (Note that this new position is added to lists xs and ys.)
- Repeat from Step 3.
Step 13
- According to rule 3 of the Chaos Game, now randomly select one of the vertices of the original triangle.
Use v:= randint(1,3) to select a random integer which will help distinguish among the three vertices.
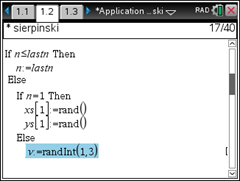
Step 14
Build a set of If statements based on v to compute the next midpoint. Recall that the three vertices we’ve chosen to use are (0, 0), (2, 0), and (1, 1).
- If v=1, arbitrarily use the point (0, 0) and the last point in the lists to calculate a midpoint.
Recall from Geometry that the coordinates of the midpoint between two points (x1, x2) and (y1, y2) are (x1+x2)/2 and (y1+y2)/2.
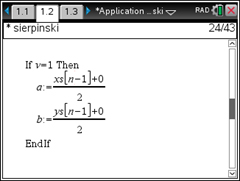
Step 15
At this location in the program, the last values of xs and ys are xs[n-1] and ys[n-1]; we got here by increasing n but have not yet added the coordinates of another point to the lists.
This analysis gives rise to the code seen to the right. v, a, and b are temporary local variables.
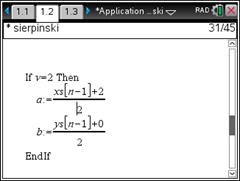
Step 16
- At the top of the program, edit the Local statement to become:
Local v, a, b - We can then easily duplicate this If structure to handle the other two vertices, (2,0) and (1,1).
Note: A more efficient structure here would be the If…Then…ElseIf…Else…EndIf structure. See if you can implement this section of the program using that structure instead.
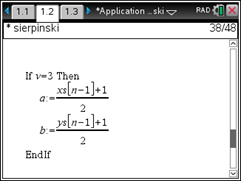
Step 17
- Add the values of a and b to the end of our two lists.
The nth position is actually one spot past the end of the lists, and this is always permitted.
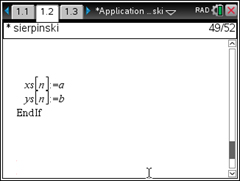
Step 18
If you’ve been selecting the If structures from the Control menu and inserting the blocks in the correct places, then you’ll have another EndIf at the bottom of the program just before lastn:=n.
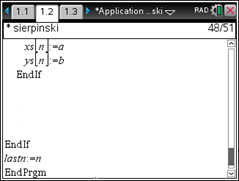
Step 19
Running the Program
- Press ctrl+B to ‘Check Syntax & Store.’
If there are any errors, you must check your code carefully. A complete program listing is provided in the Teacher's Notes document. - Add a Notes app, and insert a Math Box (ctrl+M). Type the name of the program, a left parenthesis and press enter.
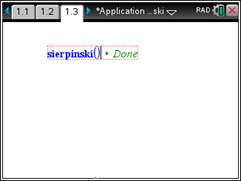
Step 20
- Switch to the Graphs app, and hide the (xs,ys) label. Try the two sliders.
- n adds points to the scatter plot. reset will erase all points and set n back to 0.
- The left arrow button on the n slider should not work, and the left arrow button on the reset slider should always be disabled.
- reset should always appear to be 0 because the program detects its change to 1 and immediately changes it back to 0.
- The value of n is limited by the maximum value set in the slider’s Settings.
- The more points plotted, the closer the image appears to represent the Sierpinski Gasket.
Congratulations! Be sure to save and share your accomplishments!
Now back to the Local statement. Which variables in this program can be Local? Which variables are needed to produce the picture?
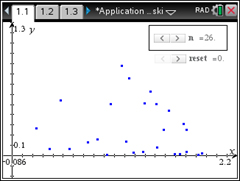
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 6: Drawing (TI-Nspire™ CX II Required)
Skill Builder 1 Basic Shapes
Download Teacher/Student DocsIn this lesson, you will learn about using the Draw features in the programming world of TI-Nspire™ CX II.
Note: All of the following lessons in Unit 6 require the TI-Nspire™ CX II with OS 5.0 or higher.
Objectives:
- Draw some shapes
- Handling the drawing screen
- Running and ending a program using Draw commands
- Changing the drawing color
Step 1
Draw a Line
- Start a new document and add a Program Editor. Name the program shapes.
- Press [menu] and select Draw >Shapes and observe the list of shapes available.
- Select DrawLine.
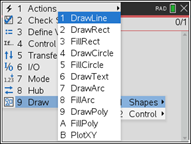
Step 2
- To draw a line, specify the endpoints, (x1, y1) and (x2, y2), of the line.
- The format of the command is:
DrawLine x1, y1, x2, y2 - To get started, use these values:
DrawLine 10, 20, 100, 120
Note: Draw statements do not use parentheses. Using parentheses causes an error.
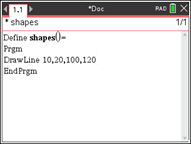
Step 3
- Press ctrl-R to prepare to run the program and press [enter] to execute the program on the Calculator app.
You now see a screen like the one on the right. You are seeing a special graphics drawing ‘window’ for displaying the Draw commands. (10,20) is the upper left endpoint of the line and (100,120) is the lower right endpoint. The title bar on the screen displays ‘Finished’ indicating that the program has ended but the handheld still displays the drawing. Press any key to close the drawing screen and return to the Calculator app.
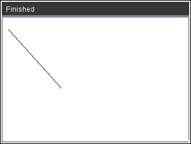
Step 4
Draw a Rectangle
- Return to the Program Editor and, after the DrawLine statement, add a line of code to draw a rectangle: choose DrawRect from [menu] > Draw > Shapes
DrawRect 10,20,100,120
Use the same four values that you used for the DrawLine statement.
Can you predict what will happen?
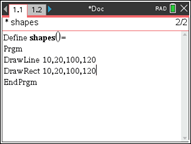
Step 5
- Press ctrl-R and run the program again. Why is the lower right corner off a bit?
The DrawRect command uses the form:
DrawRect x, y, width, height
What should the width and height of the rectangle be so that the line is a diagonal of the rectangle?
Bet you’re wondering what the screen dimensions are. Before advancing, see if you can draw a line that is a diagonal of the screen.
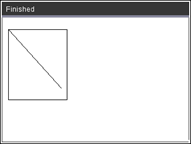
Step 6
Screen Size
- The drawing screen is 318 x 212.
- The upper left corner of the screen is (0,0).
- The lower right corner of the screen is (317, 211)
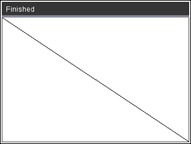
Step 7
- The statement DrawLine 0, 0, 317, 211 draws a diagonal on the screen.
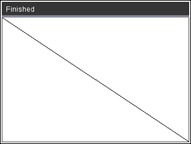
Step 8
- Write the statement that draws the other diagonal of the screen.
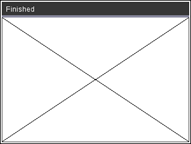
Step 9
Other Shapes
DrawCircle x, y, r where (x,y) is the center and r is the radius
Example:
- DrawCircle 159, 106, 50
FillRect and FillCircle are similar but they fill the shapes with the current color.
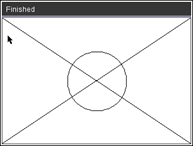
Step 10
Changing Drawing Color
- In the Program Editor, on a blank line choose the SetColor statement from
[menu] > Draw > Control > SetColor
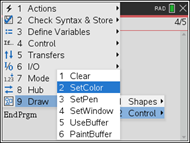
Step 11
R, G, B
Color is determined by three values: the amount of Red, Green and Blue to mix together. Each of these three values must be in the range 0..255.
SetColor red_value, green_value, blue_value
Example:
SetColor 128, 191, 30
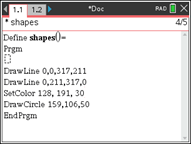
Step 12
- Use the SetColor statement before drawing something.
- Try writing a program that draws 100 random filled circles in random colors. Use a For loop.
If you want a circle and its interior to be two different colors use two different pairs of statements:
Setcolor 255, 0, 0 | red |
FillCircle 100, 100, 50 | interior of circle |
SetColor 0, 0, 0 | black |
DrawCircle 100, 100, 50 | the circle itself |
… draws a black circle with a red interior. But if you draw the circle first, then the filled circle, you will not see the black circle because the red covers it. Try it.
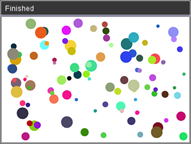
Skill Builder 2 Settings
Download Teacher/Student DocsIn this lesson, you will change the viewing window and investigate its impact on the Draw commands. You will also learn about SetPen and DrawArc.
(Note: This lesson requires the TI-Nspire™ CX II with OS 5.0 or higher)
Objectives:
- Change the Drawing window settings
- Change the drawing pen
- Draw some arcs and ellipses and see the relationship between arcs and rectangles
Step 1
The default drawing screen is odd compared to graphing:
(0,0) is in the upper left corner and the y-values (the vertical coordinates) increase as you go down the screen.
It’s like looking at a first quadrant window, but it’s upside down. Most graphical programming environments are like this. The SetWindow statement allows us to work with more familiar screen coordinates, but it does have an impact on the use of the drawing shapes.
Step 2
Window Settings
- Start a new program and name it ‘window’.
- Draw a diagonal on the screen for reference purposes:
DrawLine 0, 0, 317, 211 - Run the program (press ctrl-R, then [enter]) to confirm that you did this right. You should see a line from the upper left to lower right corner of the screen.
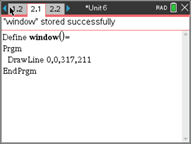
Step 3
- After the DrawLine statement, add a SetWindow statement. This statement is found on [menu] > Draw > Control > SetWindow
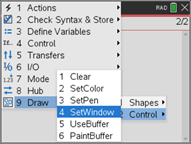
Step 4
- To set up a drawing screen that is similar to the Graphs app’s standard window, use
SetWindow -10, 10, -6.667, 6.667
If you run the program now you will not notice a change in the drawing screen. You still see a diagonal from upper left to lower right.
The syntax for SetWindow is
SetWindow xmin, xmax, ymin, ymax
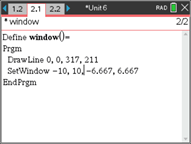
Step 5
Effect on Drawing
- After the SetWindow statement, draw a rectangle from (0,0) with width 3 and height 2 using:
DrawRect 0, 0, 3, 2
Run the program again. Do you see the rectangle near the center of the screen? The origin (0,0) is now at the center of the screen and the x- and y- coordinate system is just like a Graphs app standard window. The width of the rectangle is measured to the right. Its height is measured upward. The unit length is similar to the Graphs unit. And the original diagonal line remains on the screen. The SetWindow statements did not affect the diagonal that was drawn first.
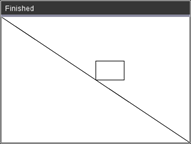
Step 6
Change the Drawing Pen
- The SetPen statement lets you change the thickness and style of the lines being drawn by the Draw statements.
The syntax is
SetPen thickness, style
Example: before the DrawRect statement use
SetPen 3, 3
Both thickness and style can be from 1 to 3.
Try them all!
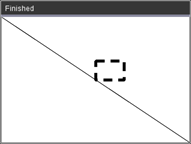
Step 7
Draw an Arc
- DrawArc is similar to DrawRect. This makes it more useful as we’ll see.
- In a new program, make the window -10, 10, -7, 7 and make a rectangle from -2, -2 with both width and height equal to 4:
SetWindow -10, 10, -7, 7
DrawRect -2, -2, 4, 4
When you run the program you should see a square in the center of the screen.
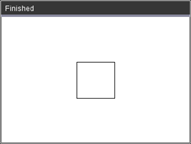
Step 8
- Change the drawing color to red using SetColor 255,0,0
- Select DrawArc from [menu] > Draw > Shapes. Use the same values as in DrawRect and add two more parameters: the startangle and arcangle for the arc:
DrawArc -2, -2, 4, 4, 0, 90 - Run the program. This draws a circular arc counterclockwise 90 degrees.
The arc is inscribed in the rectangle and goes from 0 degrees (‘east’) and turns to 90 degrees (‘north’). Now remove or hide the rectangle (make it a comment ©). Change the shape of the arc by changing the width, height, start angle and arc angle. If you see any error messages just edit your numbers accordingly.
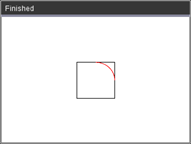
Step 9
Draw a Face
In the previous example you used equal width and height values to make an arc of a circle. But the width and height of the arc can be different. This allows for elliptical arcs, too.
- Try this code that produces the ‘smile’ on the right. Can you add two eyes and a nose?
SetWindow −10, 10, −7, 7
© DrawRect −2,−2,4,4 ← this line is a comment and is ignored.
SetColor 255, 0, 0
SetPen 3, 1
DrawArc −2, −2, 4, 2, −180, 180
To turn a line into a comment, place the text cursor at the beginning of the line and use [menu] > Actions > Insert Comment. The © symbol is inserted and the line is ignored during the program’s execution. This helps to preserve the code without processing it in case you need it later.
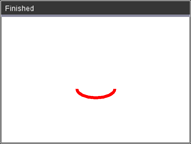
Skill Builder 3 Plotting a Point
Download Teacher/Student DocsIn this lesson, you will write a program to plot a point, automatically move it, and display its coordinates as it moves. Use [esc] to end the program.
(Note: This lesson requires the TI-Nspire™ CX II with OS 5.0 or higher)
Objectives:
- Plot a point
- Move it automatically
- Display its coordinates
- Use getKey(0) to look for a keypress
Step 1
Plotting Points
So far you have experienced drawing shapes (line, rectangle, circle and arc). There’s another tool available: PlotXY lets you plot a point using its coordinates (x, y).
The syntax is: PlotXY x, y, style
x, y represent the coordinates of the point based on the current window setting.
style is a value from 1 to 13 and affects the size/shape of the point. It is required.
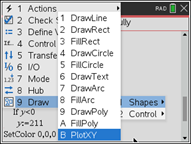
Step 2
Plot a Point
- Start a new program named movepoint
- Set up a nice window: SetWindow -15, 15, -10, 10
- Begin by plotting the point at the origin:
x:=0
y:=0
PlotXY x, y, 1
PlotXY (found on [menu] >Draw > Shapes) plots the point (x,y) in style number 1. We use the variables x and y because they will change value in the program to make the point move. Try some of the other styles (1 to 13 for the third parameter).
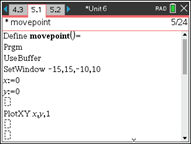
Step 3
The Main Loop
We will use a getkey(0) statement ([menu] > I/O) to look for the ‘esc’ key to end the program:
- After the PlotXY statement add:
k:=” ”(nothing between the quotes)
While k≠”esc”
(loop body)
k:=getKey(0)
EndWhile
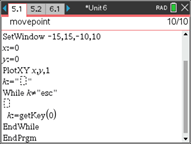
Step 4
Move the Point
The loop will make the point appear to move by changing the x-coordinate. But first, the point needs to be erased from its current position. We will ‘erase’ it by plotting it in the background color (white)…
- In the loop body between While and getKey(0) add:
SetColor 255,255,255 white PlotXY x, y, 1 erase current point x:=x+1 increase x SetColor 0, 0, 0 black PlotXY x, y, 1 plot new point Wait 0.5 wait ½ second
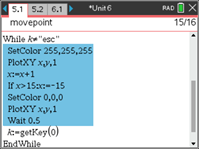
Step 5
Wait is on [menu] > Control and tells the calculator to pause processing for that number of seconds.
Run the program to see the point move to the right. Notice that it goes off the screen?
Press [esc] to end the program and modify the program to keep the point with in the screen bounds:
Step 6
- After x := x + 1 add the statement:
if x > 15 at the right edge of screen? x := -15 go to the left edge of the screen Challenge: Can you make the point ‘turn around’ and move to the left when it reaches the right edge of the screen and move to the right when it reaches the left edge?
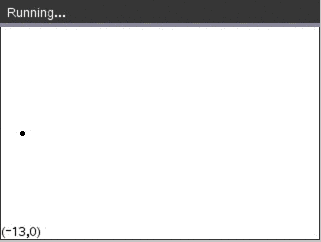
Step 7
Show the Coordinates
While the drawing screen is showing, I/O statements like Disp and Request are disabled. To get text information to appear on the drawing screen we have to use DrawText (found on [menu] > Draw > DrawText).
Syntax: DrawText thing1, thing2, thing3, …
things are: string literals like “Hello, world!”
numbers
variables
expressions
Example (without programming): on a Calculator app, type
drawtext 30, 50, “The value of x is “, x
(your value of x may differ!)
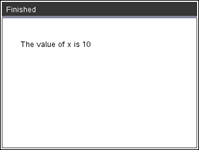
Step 8
- Now, back to our program. Add some statements to display the coordinates of the point as it moves. Just before the Wait statement add the statements:
SetColor 0, 0, 0 | black |
DrawText -15, -10, “(“, x,” ,”, y, ”)” | display (x,y) |
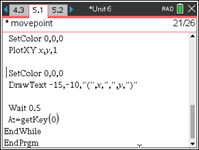
Application Manual Animation
Download Teacher/Student DocsWrite a program to move an object on the screen using the arrow keys (an interactive animation). This forms the basis of many video games.
(Note: This lesson requires the TI-Nspire™ CX II with OS 5.0 or higher)
Objectives:
- Use getKey(1) to read the keypad
- Make changes to coordinates depending on keypresses
- Erase and redraw a shape
Step 1
In the previous lesson you made a point move across the screen by itself and used getKey(0) to end the program. Now you will write a program that lets the user move the point (or any shape) using the arrow keys. In this project you will use getKey(1) which pauses processing and waits for a keypress. Only the arrow keys (“up”, “down”, “left”, right”) and the “esc” key are important to this project.
Step 2
Getting Started: Draw the Point
- Start a new program.
- DO NOT USE SetWindow.
The default window is (0..317) x (0..211). - The point will start near the center (159,106) of the screen:
x := 159
y := 106
PlotXY x, y, 1
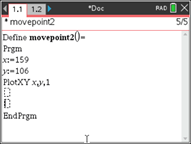
Step 3
The Main Loop
- The main program loop continues until the “esc” key is pressed.
k := getKey(1) wait for a keypress to start While k ≠ “esc” as long as it’s not [esc] loop body k := getKey(1) wait for a keypress to continue EndWhile EndPrgm
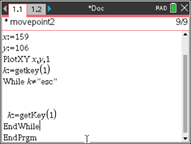
Step 4
The Arrow Keys
- The four arrow keys are “up”, “down”, “left” and “right”. Check k for each of these values and change either x or y accordingly:
If k = “left”
x := x – 1 (1 is arbitrary; it could be larger)
Recall that this is the simplest form of the If statements (no Then or Else).
Compete the three other If statements for the other arrow keys.
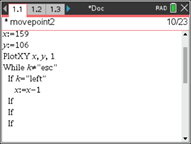
Step 5
Re-draw the Point
- After the four If statements, plot the point again using its new coordinates.
MPlotXY x, y, 1
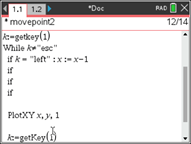
Step 6
Test the Program
Use the arrow keys. Press [esc] to end the program. If all goes well the point moves… and leaves a trail behind.
What happens when the point gets to the edge of the screen? Currently, it goes off the screen. There are some choices to make here: you can make it simply stop at the edge and not go any further or you can make it go elsewhere, typically to the opposite edge of the screen. This last option is called wrap-around or toral mode.
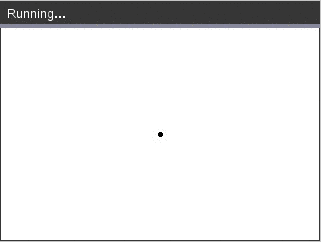
Step 7
Complete the Project
In Skill Builder 3 you learned about ‘erasing a point’ Add that feature to this project so that the point only appears to move.
To handle the edges of the screen, add four If statements to the code:
If x > 317
x:= ?
Step 8
Can you move an entire set of shapes?
This project only deals with manually moving a point. Consider replacing the point with some shapes. An efficient method for doing this would be to write a separate program (a ‘subroutine’). Within the same problem, write a new program called drawshape(x, y) and, in the movepoint2 program, use the statement drawshape(x, y) instead of PlotXY x,y,1. See an example to the right.
In the drawshape(x, y) program, draw some shapes using x and y as a reference point. Your object can then consist of several different pieces and use several colors.
To erase the shapes consider using the Clear statement ([menu] > Draw > Clear). Clear all by itself clears the screen. To erase or clear just a rectangular portion of the screen use:
Clear x, y, width, height
(similar to DrawRect)
Have fun!
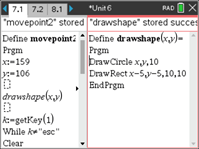
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
End of Course Projects
Extensions and More Practice
Download Student DocReady for more? Now that you have completed the course, you are ready to try your hand at some more challenges! Make sure you have completed all the Units prior to starting this section. The projects here will have you apply what you have already learned. Download the document here for challenges to choose from.
If you get stuck, refer back to the previous Units in the course. For an extra challenge, design your own program from scratch. If it’s really cool, post your work to social media and tag it @TICalculators. We would love to see your creativity! Good Luck and Get Coding!