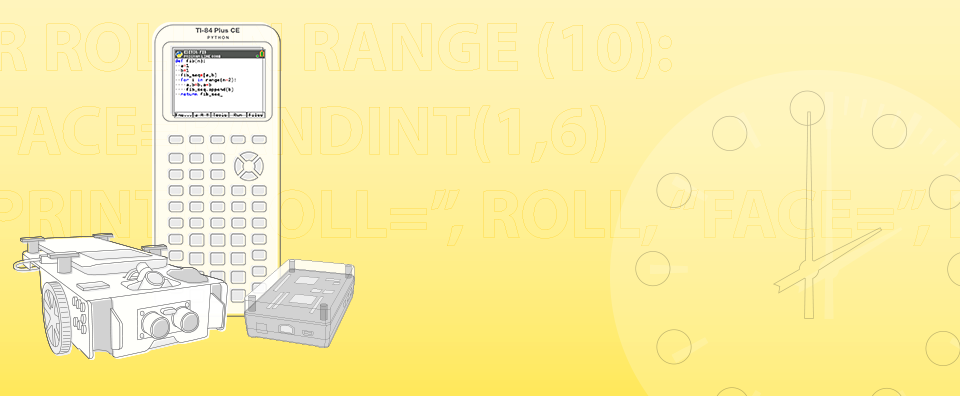
10 Minutes of Code: Python and TI-Innovator™ Technology
These short, easy-to-teach activities are designed to
be completed in order, using TI-84 Plus CE Python graphing calculator and TI-Innovator™ Technology.
Note: These lessons require the use of the TI-84 Plus CE Python graphing calculator.
Unit 1: Getting Started With Python and the TI-Innovator™ Hub
Skill Builder 1: Light It Up
Download Teacher/Student DocsIn this lesson, you will learn the basics of writing and running a Python program and using the "light" (the red LED) on the TI-Innovator™ Hub.
Objectives:
- Create and run a Python program
- Control the light on the TI-Innovator™ Hub
Intro 2
Welcome to the world of TI-Innovator Hub programming using Python on your TI-84 Plus CE Python. Your calculator must be labeled ‘PYTHON’ above the screen.
Your first program will operate the red LED on the TI-Innovator Hub circuit board. It is hard to see on the board, but when you turn it on you will know it.
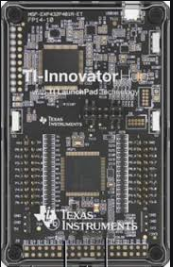
Step 1
To get started with Python programming, press the [prgm] key on your calculator and select the Python App. The app loads available Python files into the File Manager screen shown to the right. There are three demo files included from the factory but the list can include other files that may have been created. As you make more files, they will be added to this list alphabetically. On the bottom of the screen are five ‘soft keys’: <Run>, <Edit>, <New>, <Shell> and <Manage>. Select a ‘soft key’ using one of the five graphing keys at the top of the keypad. These ‘soft keys’ will change depending on the current selection.
Note: the top of your calculator will have the word ‘PYTHON’ below TI-84 Plus CE. The image shown here comes from the emulator software (TI SmartView™ CE).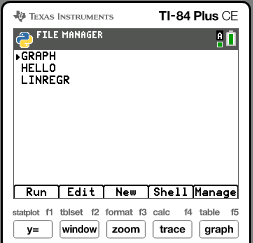
Step 2
Start a new program by selecting the <New> soft key(press the [zoom] key). Since your first program will control the red LED on the TI-Innovator hub, name the program LITE. Your keypad is set to UPPERCASE alpha as the blinking cursor indicates, so just type the letters L-I-T-E and then…
Note that this screen image (and the rest of the screen images in this course) does not include the top row of keys but only the on-screen ‘soft keys’.
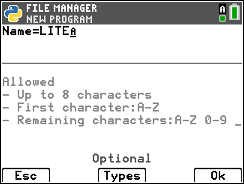
Step 3
… select the <Ok> soft key or press the [enter] key. You are now using the Python Editor as indicated on the top of the screen.
The five soft keys now contain tools for editing your program:
- <Fns…> contains many programming commands in lots and lots of sub-menus.
- <a A #> is an on-screen ‘Character Map’ and one-line editor with some useful programming symbols.
- <Tools> contains some useful editing tools, especially ‘Undo Clear’.
- <Run> will run your program in the Python Shell. Select <Editor> from the Shell screen to return to this Editor.
- <Files> takes you back to the File Manager. In the File Manager first point to your file and then select <Edit>.
You can try any of these keys. They contain either an <Esc> option or an <Edit> or <Editor> option.
Step 4
To control the TI-Innovator Hub LED you must start your program with a special TI-developed module called ‘light’:
Select <Fns…>. On the next screen shown to the right, select the Modul menu by pressing the right-arrow key 5 times or the left-arrow key just once. Take a moment to explore the other five sub-menus.
From the Modul menu select the ti_hub… menu option as shown using the arrow keys and the [enter] key (or press [6]).
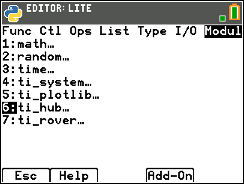
Step 5
On the next screen, select Hub Built-in devices… (since the red LED is built into the TI-Innovator Hub) by pressing [enter] or [1].
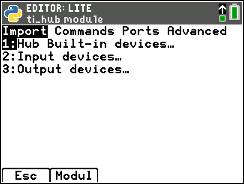
Step 6
Finally, select Light from the Hub Built-in devices menu. Here you can either use the <Import> soft key or just press [enter] or [2].
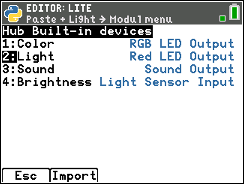
Step 7
All that menu navigation results in the statement
import light
pasted as the first line of code in your program. The word ‘import’ is blue because it is a Python ‘reserved’ word. The Editor uses color to highlight keywords, symbols, and literal strings as you will soon see.
Note our cursor is on PROGRAM LINE 0002 of the Editor now as indicated in the status line at the top of the screen.
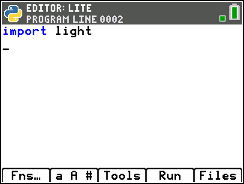
Step 8
For the next statement, press the [math] key on the keypad which now directly brings up the Modul screen as shown here. But note that there is now a new menu item at the bottom of the list: Light…
Select the Light… menu item and see…
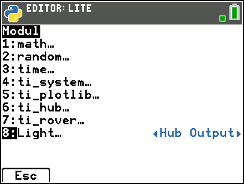
Step 9
… the three Light functions: on( ), off( ) and blink(freq, time).
Select on( ).
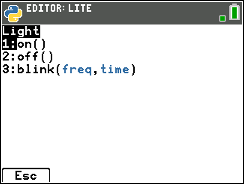
Step 10
Your program now contains two statements.
With your TI-Innovator attached to your calculator, select the <Run> soft key to execute the program. Did a red light come on in the Hub?
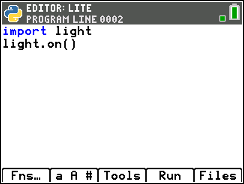
Step 11
If your program runs successfully, you will see the screen to the right and the red LED lights up on the TI-Innovator hub. Congratulations! You have written your first TI-Innovator program using Python.
The screen now displays the Python Shell as indicated at the top of the screen. The messages on the screen indicate the last actions performed. The two lines beginning with >>> # (the octothorpe) are comments. The line >>> from LITE import * is the command that executes the code in your LITE program. The last line is the Shell prompt >>> and the cursor | on this line is waiting for you to enter a Shell command because your program is done.
But note that the red LED on the hub is still lit. How can you turn it off?
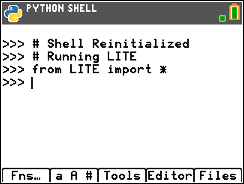
Step 12
Return to your program code by selecting <Editor>. Your cursor should be blinking at the end of the light.on( ) line 0002. Press [enter] to move the cursor to the next line. Add a statement to turn the light off. Try it yourself before going to the next step.
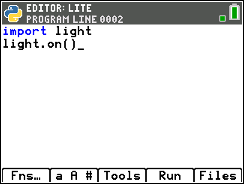
Step 13
Did you use the light.off( ) function? It’s found on [math] Light… just below light.on( ).
<Run> the program again and watch the TI-Innovator hub carefully. You should see the red LED quickly flash. To repeatedly run the program simply press the <Editor> <Run> soft key since it toggles between the Editor and the Run operation (Shell).
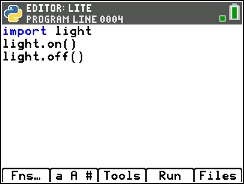
Step 14
To control the timing of the LED (how long it stays on before turning off) you will add a sleep( ) function …
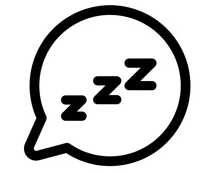
Step 15
Back in the <Editor> add two blank lines to your code by pressing [enter] at the beginning of each light statement. Now, with your cursor on the line below the import light statement…
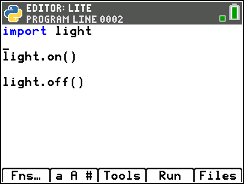
Step 16
Add the statement
from time import *
found on [math] time…
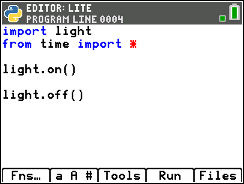
Step 17
Now, between light.on and light.off, add the sleep( ) function also found on [math] time. (Simply use the arrows on keypad to navigate up and down lines in your code.)
Inside the sleep( ), type a number of seconds inside the parenthesis. This statement will thus pause processing for that many seconds before proceeding to the next statement so that the LED stays on for some seconds before turning off.
Run the program now and watch the LED.
After the light.off() statement, give the light.blink( ) command a try (note that it takes two arguments separated by a comma (provided for you).
See if you can figure out what the two numbers are used for! Experiment a little, changing the numbers, and watching what happens.
Skill Builder 2: Color and the Hub Project
Download Teacher/Student DocsIn this lesson, you will learn about controlling the color LED on the TI-Innovator™ Hub using a Python program template.
Objectives:
- Use a Python project template
- Control the color LED on the TI-Innovator™ Hub
Intro 2
The color LED has three color channels: red, green, and blue. This is often referred to as an “RGB LED.” Computer screens, phone screens and TV screens all use many of these LEDs to create images.
To get a unique color, mix the correct amounts of red, green and blue. Many colors are possible with the right mix of these three primary colors of light.
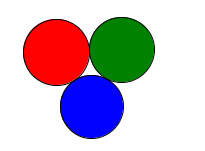
Step 1
Begin this lesson with a new Python file. If you are not using the Python App, press [prgm] and select Python App.
If you are using the Python App and are using the Editor or Shell, go to the File Manager by selecting the <Files> soft key.
Select <New> and first select the <Types> soft key. Select Hub Project from the list and name the program COLR (‘COLOR’ is already being used). Notice that the screen to the right now says ‘Hub Project’ right above the <Types> soft key. Press <enter> to begin the Editor.
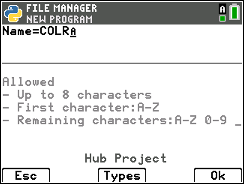
Step 2
The Hub Project template provides two import statements. These modules provide some functions like sleep( ) that will come in handy later. The top line of code is a #comment since it starts with # (number sign, hash, pound, or octothorpe). The entire line is colored light gray. #comments are ignored when the program is run and are a handy way to document your program and to help in debugging (removing errors). Press [2nd] [3] for the # symbol or find it on the <a A #> Character Map.
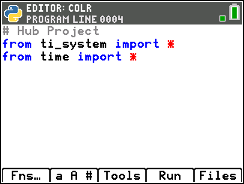
Step 3
We need one more import statement. Place your cursor below the last import statement and press
[math] > ti_hub… > Hub Built-in devices… and select Color.
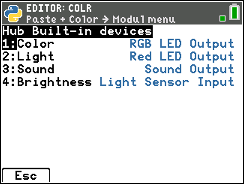
Step 4
This adds the statement import color to your code.
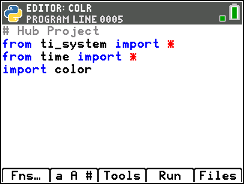
Step 5
With your cursor on a new line below import color, press [math] and select the new menu item at the bottom: Color…
There are three functions on the Color menu. The first one,
rgb(r, g, b)
establishes the color of the LED using the three channels: red, green, and blue. Each of these three arguments must be an integer in the range 0 to 255 as indicated on this screen. Values outside this range will produce an error when the program is run.
blink( , ) and off( ) work like the corresponding light functions from the previous Skill Builder.
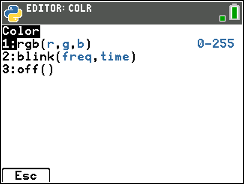
Step 6
Enter three numbers, each between 0 and 255 in the parentheses. The commas are provided for you. We chose 255,100, 0.
<Run> the program.
Watch the TI-Innovator Hub and see your custom color appear.
If you encounter an error, make sure that the Hub is properly attached to your calculator (has a green power light) and that all the code is exactly correct. A misplaced comma, missing parenthesis, or misspelling can cause an error. To return to the Editor select <Editor> in the Shell.
If all goes well, the color LED will remain lit and you can return to the Editor and try different r, g, and b values. Select <Run> again after editing.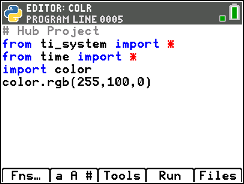
Step 7
Two other functions on the [math] Color menu are
blink(freq, time)
and
off( )
Can you guess what they do? Try adding them to the program to test your guess. You can also incorporate the sleep( ) function (that’s why the time module is imported) into your code as you did in Skill Builder 1.
Challenge: Try making a program to display various colors like the rainbow or your school colors.
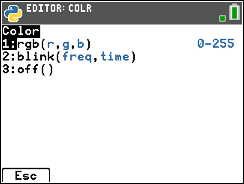
Skill Builder 3: Input and Sound
Download Teacher/Student DocsIn this lesson, you will learn about two ways to make sound on the TI-Innovator™ Hub and use variables to store and retrieve values.
Objectives:
- Produce a sound on the TI-Innovator™ Hub
- Use variables to store entered values
- Use the input() function and the int() function
- Play musical notes
Intro 2
The TI-Innovator™ Hub houses a small speaker, called SOUND. The speaker is on the bottom of the hub. There is no amplifier, so the volume is very low. It is designed that way so that your classroom does not get too noisy.
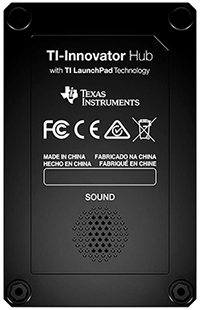
Step 1
Start a new Python file using the Hub Project template.
Ours is named SOUNDA since SOUND is already taken.
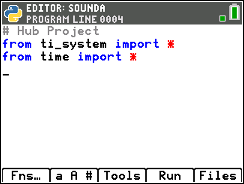
Step 2
You will write two input statements to get values to create a sound. These values will be stored in two variables, f and t. Write two statements like this:
f = input(“Frequency? “)
t = input(“Time? “)
We will do this step-by-step next…
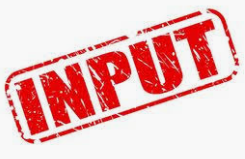
Step 3
Type the lowercase letter f (press [alpha] [F]) and the = sign on a blank line below the bottom import statement. Use the [sto>] key for the = sign.
f =
Leave your cursor to the right of the = sign for the next step.
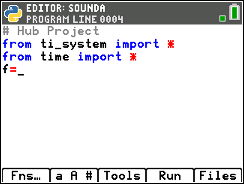
Step 4
Now get the input( ) function by selecting it from <Fns…> I/O
f = input( )
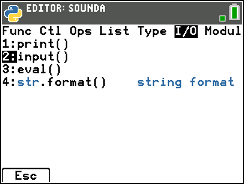
Step 5
Selecting input() from the menu pastes the word input( ) into your program after the = sign.
Inside the parentheses, type the “prompt” for this input statement in quotation marks.
To type the prompt “frequency? “, turn on alpha-lock by pressing [2nd ] [alpha].
The quotation mark is on the [+] key. The question mark (?) is on the
[ (-) ] (negation) key next to the [enter] key. The space character is on the [0] key. Be sure to use the closing quote as well, the same as the opening quote.
On the next line make another, similar input statement for the variable t which stands for time.
Step 6
Below the two input statements, add another import statement to access the sound functions on the TI-Innovator™ Hub.
To paste the import statement:
Press [math] and select ti_hub… Hub Built-in devices… Sound…
To get the sound.tone( , ) function:
Press [math] again and select the new Sound… menu item at the bottom of the list to get the tone(freq, time) function.
Tip: you can press [up-arrow] to quickly get to the bottom of any menu immediately.
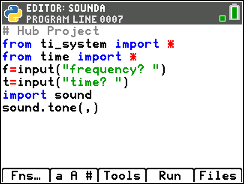
Step 7
The two arguments for the tone function are the two variables, f and t which have been assigned values using the input functions.
Since these are just single letters you can press [alpha] and the proper letter key for each. Be sure f is before the comma and t is after the comma inside the parentheses as shown.
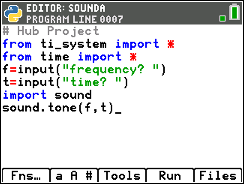
Step 8
<Run> the program. At the prompts, enter a frequency of 440 and enter 2 for the time.
And then… oops! A ‘runtime error’! This error occurs because the input functions return values that the sound function cannot use: they are strings, “440” and “2”, rather than numeric values, 440 and 2. You need to convert each string value to a numeric value using either float( ) (for a decimal number) or int( ) (for an integer). We will correct this error next. But first…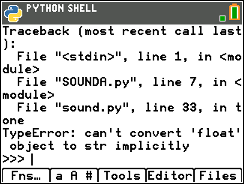
Step 9
Programming errors come in three flavors:
Syntax errors – errors in typing (spelling/grammar/structure).
Runtime errors – errors during program execution, such as division by zero, incorrect data types, or Hub not present.Programmer errors – errors in logic or expressions that cause the program to produce incorrect results. These are the hardest errors to correct because they happen in the programmer’s head.
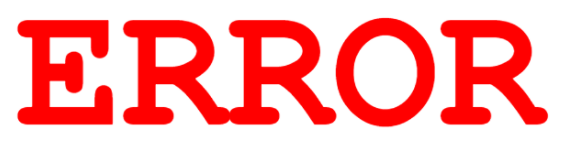
Step 10
To convert the values of f and t to integers use the int( ) function found on the <Fns…) Type menu. Insert the two statements:
f = int( f )
t = int( t )
As shown in the image, these two statements belong after the input statements and before the sound.tone statement. Order matters!
You can also use float( ) in place of int( ) if you expect to enter decimal values.
Note that the import sound statement has been moved up to be with the other import statements. This is more common in Python coding.
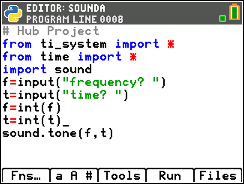
Step 11
<Run> the program again and enter 440 for frequency and 2 for time again. You will hear a tone of 440Hz for 2 seconds from the speaker on the bottom of the TI-Innovator Hub. The Shell prompt is displayed on the calculator screen since the program is done. Select <Tools> and press [enter] to Rerun Last Program to try other frequencies (between 0 and 8000 Hz) and times. Can you hear all the frequencies?
Try a ‘low’ frequency value like 3 or 6. What do you hear?
Note: If you do not see the shell prompt >>> after a few seconds, then press the [on] key to ‘break’ the program.
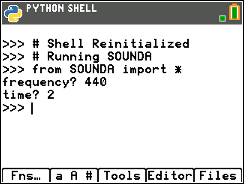
Step 12
Playing Notes…
sound can also play musical notes using their names:
sound.note( “A4”,2)
plays the note A in the 4th octave.
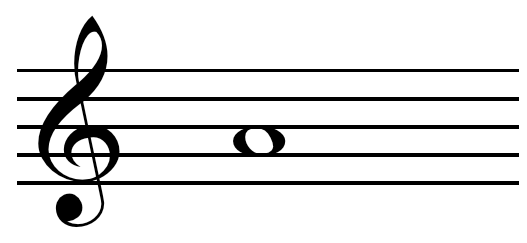
Step 13
To copy the SOUNDA program, from the Editor or the Shell…
- select <Files>, and, in the File Manager screen,
- point to the SOUNDA filename,
- select <Manage> Replicate Program…
- -name the copy SOUNDB and press [enter] or select <Ok>.
You are taken immediately into the Editor with the code for SOUNDB showing.
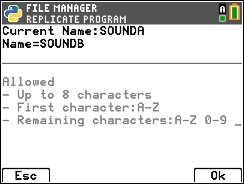
Step 14
Make three changes in the SOUNDB program as shown:
- Change “frequency? “ to “note? ” in the f = input() statement.
- #comment the f=int(f) statement by placing the cursor at the beginning of that line and typing [2nd ] [3] for the # sign because the note function needs its first argument to be a string, not an integer.
- Change sound.tone to sound.note by deleting ‘tone’ and typing ‘note’ in its place (without quotes).
Note: You can delete the line f=int(f), too, but it’s good practice to use a comment in case you may need the statement in the future.
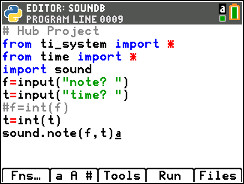
Step 15
After making all three changes, <Run> the program. At the note? prompt type a4 without quotes as shown. Enter 2 for the time again.
Do you hear the same sound as before?
A4 is the note A in the 4th octave (A above middle C) and has a frequency of 440Hz. Try other notes (letters A,B,C,D,E,F,G) (uppercase or lowercase) in octaves (1,2,3,4,5,6,7) Remember, just Select <Tools> and press [enter] to Rerun Last Program to try other frequencies.
There are two other functions on the Sound… menu that include a ‘tempo’ argument. Give them a try and figure out what ‘tempo’ means.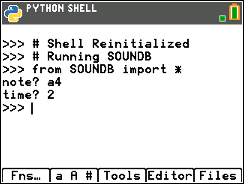
Application: Traffic Light
Download Teacher/Student DocsIn this application you will write a program to simulate a traffic signal: both lights and sounds (for the blind).
Objectives:
- Control the timing of the red, yellow and green states of a traffic signal
- Combine light, color and sound into one project
- Use a while loop to repeat a procedure until esc is pressed
Intro 2
A traffic light has three separate bulbs in red, yellow and green so that colorblind people can tell the state of the light just by the position of the lit bulb.
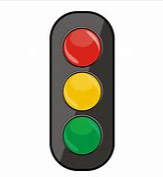
Intro 3
On the side of the road there is also an audio signal to let blind pedestrians know when it is safe to cross the street. You will make the sound as part of your traffic signal system.

Step 1
Write a program to input the numbers of seconds the traffic light is red, yellow, and green. When the traffic light is red, both the color LED and the red LED should be on. When the traffic light is yellow or green, only the color LED should be on. When the traffic light is green, there should also be an audio signal indicating that it is safe to go.
Start with a new Python Hub Project. Ours is called TRAFFIC.
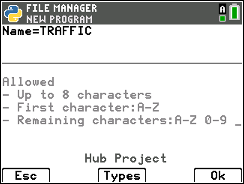
Step 2
Since we know that we will be using light, color and sound, add all three import statements to your code using the [math] menu.
The order of these three statements is not important.
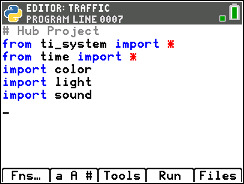
Step 3
Write three input statements that accept and store the times that each color light will be on. For long text writing (to use more meaningful variable names) try using the <a A #> Character Map. In this editor lowercase alpha-lock is automatically on and you can simply type your letters. For a capital letter select the < a◄►A> toggle. Some special symbols are also on this screen so use the arrow keys and either <Select> or [enter] to add them to your line at the top of the screen.
When you are done, select <Paste> to paste the text into the Python Editor. You can write partial lines of code and complete them in the Editor.
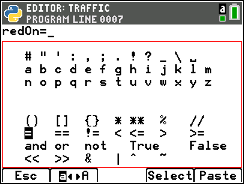
Step 4
After typing redOn= in the Character Map, select <Paste> to return to the Editor. Then get the input() function from <Fns…> I/O.
Turn on alpha-lock ([2nd ] [alpha] and type the prompt inside the parentheses. Recall that the quotation mark (") is on the [+] key.
Note: you can use shorter variable names to reduce the typing. Meaningful variable names help make your code easier to read.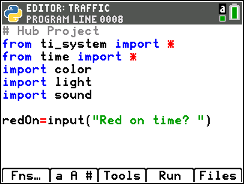
Step 5
Repeat for the other two colors of a traffic light.
Or use the <Tools> Copy line and <Tools> Paste line below then edit the new statement.
Note that we are using a capital ‘O’ in the variable names. This capital letter must be used whenever the code refers to one of these three variables. The capital letter makes reading the variable name easier.
Does your code look like this this so far?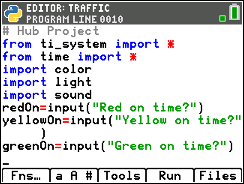
Step 6
Convert each string to an integer (or a float for decimals).
You can combine the int( ) function with the input( ) function to reduce typing.
Example: r = int( input(“Red on time?”) )
(not shown and using the variable name r instead of redOn) Be careful about the positions of the parentheses.
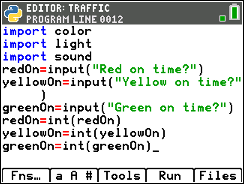
Step 7
Since we want our traffic light to operate continuously we introduce a special while loop:
while not escape():
♦ ♦
found on both [math] ti_system… and [math] ti_hub… Commands
A programming loop is a section (block) of code that is
executed repeatedly until some condition occurs.
This loop executes the (indented) block of code as long as the ‘escape’ key is not pressed. Two indentation spaces are provided in the beginning of the next line and all subsequent lines in the block must also be indented this same number of spaces. The Editor provides these spaces for you until you delete them using the [del] key.
Step 8
In the block (the indented section of code), write the code to control the lights, colors, and sounds. Use sleep( ) functions (found on [math] time…) to control the timing of the lights and synchronize the sound with the green light.
- When the light is red we want both the red LED (light) and the color LED (in red) to be on.
- Use the redOn variable in sleep( ) function to keep the lights on for the right amount of time.
- For the green and yellow lights, turn the red LED off (light.off())and just use the color LED.
When the light is green make a beeping sound (use the ‘tempo’ option in the .tone( ) or .note( ) function.
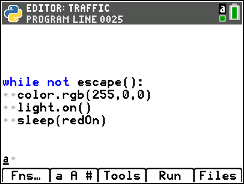
Step 9
All of the statements are inside the while not escape() loop (indented two spaces) and are not displayed in this image.
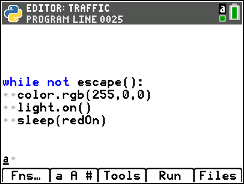
Step 10
When you press the [clear] key to end the loop, turn all the lights and sound off. Dedent (unindent) your cursor to the left side of the screen using the [del] key or use <Tools> Dedent.
Write the statements to turn both lights off.
Do you need to turn the sound off? No. It will stop by itself. How could you turn the sound off at this point?
Note: the code for the while block is missing. The two .off( ) functions are not part of that loop since they are not indented.
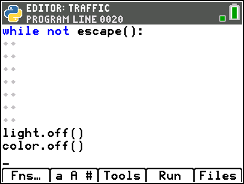
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 2: for loops with the TI-Innovator™ Hub
Skill Builder 1: Looping the Light
Download Teacher/Student DocsIn this lesson, you will be introduced to the concept of a for loop in the context of the TI-Innovator™ Hub.
Objectives:
- Make a for loop using the range() function
- Input decimal values using float()
- Create a custom blinking light
Intro 2
To loop through a set of code a specified number of times, we can use a for loop with a range() function.
The range() function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and ends at a specified number.
The function light.blink() does not give you enough control over the blink cycle. How long is the light on and how long is it off between blinks? Can you have it blink three times in 10 seconds?
This lesson will develop a program that gives you this control and also direct control over the total number of blinks.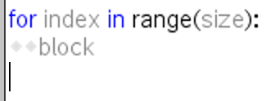
Step 1
Start with a <New> Hub Project template. We named it BLINKER.
Import the light module using [math] ti_hub… Hub Built-in devices…
Write three input statements:
- One for the total number of blinks
- One for the onTime (the time that the LED is on during a blink)
- One for the offTime (the time between blinks)
Step 1B
You may want to have blink times that are not whole numbers. Rather than using the int() function, you can use float() for the onTime and offTime. This allows you to enter numbers with decimals. Rather than have separate statements for the input and the type conversion, the two actions can be combined into one statement:
blinks = int(input(“how many blinks?”))
First get the float( ) function (<Fns…> Type) and then, inside the parentheses, paste the input( ) function (<Fns…> I/O). Note the two right parentheses at the end of this statement.
Tip: Since the three statements are similar, this is a good place to try <Tools> Copy Line and <Tools> Paste Line Below. Then edit each line to suit.
Step 2
Insert this for statement:
for i in range(size):
♦ ♦
found on <Fns…> Ctl. There are several different for loop options. Choose the first one.
The placeholder size is shown on the menu but is not pasted into your code.
Notice the two indentation spaces (the gray diamond symbols). The for loop ‘block’ is the set of statements that are all indented these two spaces (like the while loop in the previous lesson).
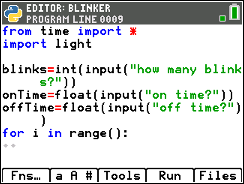
Step 3
Type the variable blinks in the parentheses of the range() function:
for i in range( blinks ):
Move your cursor to the next line using the [downarrow] key. Do not press [enter]. Be sure that the for statement still contains the colon (:) at the end of the line.
Important Tip: if your program gets stuck in a long or ‘infinite’ loop, you can ‘break’ the program by pressing the [on] key.
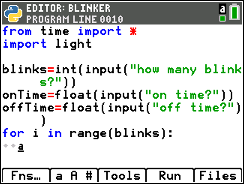
Step 4
The for loop ‘block’ is the set of statements that operate the light (the red LED on the TI-Innovator Hub). Use light.on(), light.off() and sleep() statements. Try it yourself now. Remember to use the variables for timing that you used in the input statements. Be sure that each statement is indented two spaces (provided for you by the Editor).
You can use decimal values for all three input statements, but the number of blinks will be converted to an integer. There’s no such thing as ½ of a blink!
The next step shows the completed program.
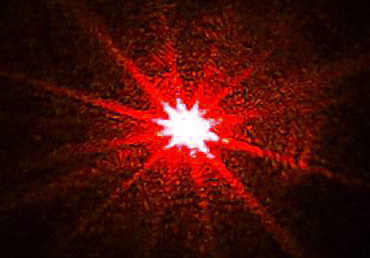
Step 5
Your program should resemble this:
blinks = int( input("how many blinks? ") )
onTime = float(…
offTime = float(…
for i in range(blinks):
♦ ♦ light.on()
♦ ♦ sleep(onTime)
♦ ♦ light.off()
♦ ♦ sleep(offTime)
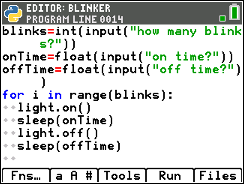
Step 6
<Run> the program and enter your values and then watch the red LED on the TI-Innovator Hub. When the program ends, is the light on or off?
The LED blinks but nothing happens on the calculator screen. Add a print statement inside the for loop to display the current blink number:
print(i)
print() is found on <Fns…> I/O. Type the variable i inside the parentheses.
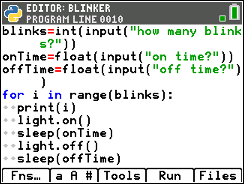
Step 7
Instead of the standard Python print( ) function you could use a special Texas Instruments developed disp_at( ) function that the Hub Project template imports.
From [math] > ti_hub… > Commands, select
disp_at( row , "text" , "align" )
You will be prompted for the align value (“left”, “center”, or “right”). Choose “center”. You can always change it later.
Use row 9.
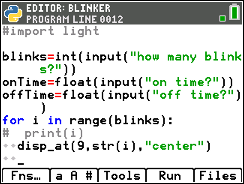
Step 7B
In place of “text” (the second argument), use the expression str(i) without quotes which is found on <Fns… > Type and converts the integer value of i into a string. You must supply the variable i inside the parentheses.
Turn the print(i) statement into a comment by typing # in front of it using [2nd] [3].
Your code should resemble this:
# print(i)
disp_at(9, str(i), “center”)
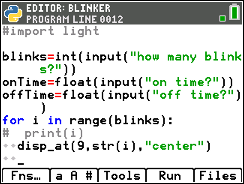
Step 8
<Run> the program again. You should now see the numbers displayed near the bottom center of the screen, all in the same position. Other disp_ functions are available on the ti_hub > Commands menu to make your screen prettier. Use disp_clr() to clear the screen before and after the loop.
What values do you need to enter to get the light to blink 3 times in 10 seconds? This challenge is left as an exercise.
Tip: There is another version of disp_at:
disp_at( row, column, “text”)
There are 11 rows numbered 1..11 and 32 columns numbered 1..32.
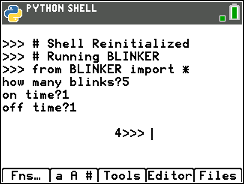
Skill Builder 2: Loop Through Color
Download Teacher/Student DocsIn this lesson, you will learn about color mixing to make a variety of colors on the color LED using just several if statements in a loop.
Objectives:
- Use if statements to change the color of the LED
- Use copy line/paste line and edit for simpler coding of longer programs
Intro 2
Many colors are possible (over 16 million!) using the color LED on the TI-Innovator Hub. This lesson develops a program that gradually changes the color LED from red to blue by mixing changing amounts of each color.
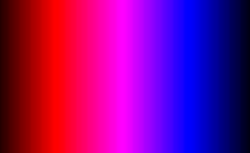
Step 1
Start a new Python Hub Project (ours is called COLORS) and add import color from [math] ti_hub….
Tip: you can also easily type this statement (and any others) manually using the <a A #> Character Map.
This program will gradually increase and decrease two of the LED’s three color channels to mix some colors.
Start by assigning values to two variables: step is the space (increment) between color values and delay is the time delay for each color step. Begin with a step of 10 and a delay of 0.1 at first. Type the words step and delay or use simpler variables like s and d.
Assign 0 to two variables, r and b, representing the starting values for the red and blue channels.
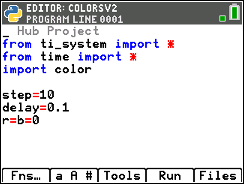
Step 2
Add the loop
while not escape( ):
♦ ♦
from [math] ti_system…
so that when you run the program you can press the [clear] key to exit at any time.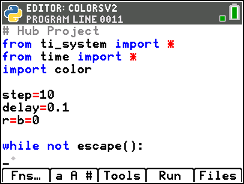
Step 3
Begin the loop block by turning the LED on using the current values of r and b for the red and blue channels. We will not use the green channel at all. These variables will change value further down in the loop.
♦ ♦ color.rgb(r, 0, b)
Then use the delay variable in the sleep( ) function found on [math] time…
♦ ♦ sleep(delay)
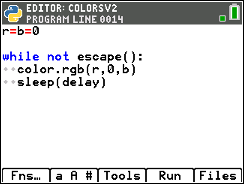
Step 4
We will write four if structures to change the values of r and b. Each will gradually change one color channel.
The first if structure increases the value of r when b is 0.
r += step means the same as r = r + step
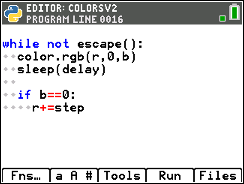
Step 5
Next check to make sure r does not exceed 255. If it does, then limit r to 255 and start to increase b. This gradually adds blue to the bright red LED.
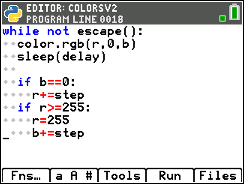
Step 6
Do the same with the value of b: when b exceeds 255 we limit it to 255 and then decrease the value of r. This removes red gradually from the mixed colors (bright magenta).
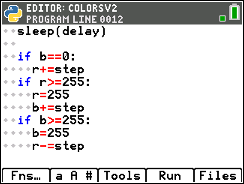
Step 7
Finally, when r reaches (or passes, depending on step) 0, limit it to 0 and begin decreasing the value of b. This gradually darkens the blue LED
We now have the four if structures shown to the right. Again, think carefully about the effect of each.
<Run> the program and watch the color LED brighten to red, then change to magenta (purple) and get pretty bright, then gradually change to blue and then…
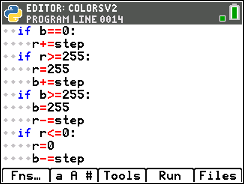
Step 8
When the blue value decreases, it eventually takes on a value less than 0 and then the program tries to use that value in the color.rgb( ) function which creates the error shown here.
When decreasing b from 255 by 10’s (our step value) we encounter -5 which causes the error. There are several ways to correct this runtime error. Try it yourself before seeing two suggestions in the next step.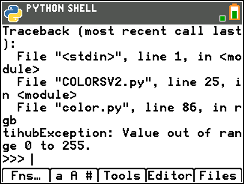
Step 9
One fix is:
- ·only allow a non-negative value for b when executing the color.rgb() function:
if b >= 0:
♦ ♦ color.rgb(r, 0, b)
Tip: to indent a statement use the <Tools> menu. - and modify the first if statement to include negative values for b in the condition:
♦ ♦ if b <= 0: - and assign 0 to b inside this block:
♦ ♦ b = 0
Now all four if structures look pretty much the same!
Another possible fix is to just add if b<0: b=0 right before the color.rgb( ) function (not shown)
Step 10
Try various values for step and delay. A large step and a small delay make the program run faster.
Challenge: can you incorporate the green channel, too? You can see all the colors of the rainbow if you mix the colors properly.
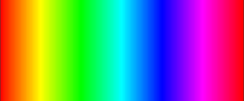
Skill Builder 3: Looping with Sound
Download Teacher/Student DocsIn this lesson, you will learn to play a song on the TI-Innovator™ Hub.
Objectives:
- Use a for loop with specific frequencies
- Use a loop with a list of musical notes
- Write a program to play a song
Intro 2
A Little Music Theory
Musical notes are determined by the frequency of a vibrating object such as a speaker, drumhead, or string as in a guitar or piano. The notes of the musical scale have a special mathematical relationship. There are 12 steps in an octave. If a note has frequency F, then the very next note has frequency F × 12√2.
Multiplying a note’s frequency by 12√2 or 21/12 (the twelfth root of 2) twelve times results in a doubling of the original frequency, so the last note in the octave (or the first note in the next octave) has frequency of F ×(21/12)12 = 2 × F. For example, if a note has a frequency of 440 Hz, the same note an octave above it has a frequency of 880 Hz, and the same note an octave below it has a frequency of 220 Hz.
Intro 3
The human ear tends to hear two notes that are an octave apart as being essentially "the same", due to closely related harmonics. For this reason, notes that are an octave apart are given the same name in the Western system of music notation - the name of the note one octave above C is also C. The 12 intervals between these notes are called ‘semitones’.
In this project we’ll take advantage of the 21/12 principle to generate the 12 notes in an octave (both naturals and sharps) plus the first note in the next octave.
Middle C (the lowest note in the staff pictured above) has a frequency of 261.64 Hz. An octave above Middle C, also known as Treble C, has a frequency 2 × 261.64 Hz or 523.28 Hz. There are 12 steps (semitones) between these two notes, and each step is 21/12 times the note before it.
Step 1
On the calculator’s home screen (if you are using Python, quit the app by pressing [quit] ([2nd] [mode]) and press [enter] or <ok>).
Type the number 261.64 and press [enter]. Then, on the next line, first press the multiplication key [x]. The handheld supplies the variable Ans at the beginning because the multiplication symbol requires something in front of it.
We multiply Ans by 2^(1/12) (you do not need the parentheses) and press [enter]. Then, press [enter] repeatedly to produce the sequence of answers shown.
We will incorporate this repetitive principle into our program. If you continue pressing [enter], the twelfth answer will be 523.28, exactly two times the starting value, because F × (21/12)12 = F × 2.
Step 2
In the Python App, start a <New> Python Hub Project and import sound from [math] ti_hub…. Begin by assigning the number 261.64 to the variable freq.
freq = 261.64
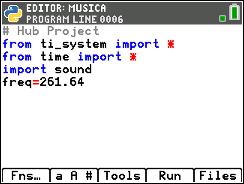
Step 3
Now write a for loop to do something 12 times:
for i in range(12):
♦ ♦
Recall that the for statements are found on <Fns…> Ctl
You must supply the number 12.
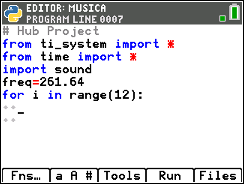
Step 4
In the for loop block do two things:
Play the frequency value on the speaker for one second:
♦ ♦ sound.tone(freq, 1)
Then increase the frequency by a factor of 2**(1/12) (the twelfth root of 2).
♦ ♦ freq = freq * 2 ** (1/12)
** is the Python ‘raise to the power’ or ‘exponent’ operator. You can use the [ ^ ] key or use two [ × ] (multiplication) symbols to produce this special operator.
Note: are the parentheses around (1/12) necessary here? YES! Although there is no computer error generated, your notes would be wrong. Omitting these parentheses is a programmer error, not syntax or runtime error. Try it!Step 5
<Run> the program. If there are no errors in your code you will hear a fast 12-note scale. The program runs too fast to hear all the individual notes. The one second delay inside in the sound function takes place in the TI-Innovator Hub, not in the handheld. The program sends the next sound too quickly and replaces the current sound.
Tell the program to ‘wait’ while each note is playing by adding a sleep(1) function to the loop after the sound is played. You might also try a value slightly larger than 1.
You may also want to add a print(freq) statement to see the frequency values being played while they are playing.
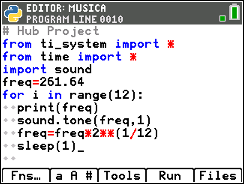
Step 6
There is another way to play musical notes on the TI-Innovator Hub. Add the following code to your program:
sleep(2)
for note in ["c4","d4","e4","f4","g4","a4","b4","c5"]:
♦ ♦ sound.note(note,1)
♦ ♦ sleep(1)
Note: the note names can be UPPERCASE or lowercase (“C4” or “c4”).
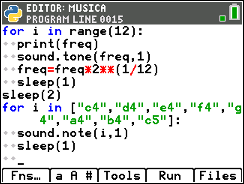
Step 6B
Find the square brackets [ and ]
- on the keypad by pressing [2nd ] [ x ] (multiply) and [2nd ] [ - ] (subtract)
- on the [list] key, [2nd ] [stat]
- on the <Fns…> List menu
- on the <a A #> Character Map
Type the list contents using [alpha] for the quotes and letters but not the commas.
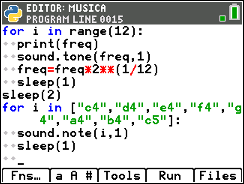
Step 6C
Explanation:
sleep(2) is just a 2-second pause between the first part of the program and this second part.
for note in is the start of a typical for loop statement. You can get this form of the for statement from menu > Built-ins > Control > for index in list:.
["c4","d4","e4","f4","g4","a4","b4","c5"] is a list of musical notes, each in quotes (a string). “c4” is note C in the 4th octave (‘middle c’, which has frequency 261.64 Hz).sound.note(noteName ,time) This loop plays the familiar ‘do-re-mi-fa-sol-la-ti-do’ scale. It leaves out the sharps in the octave.
Can you modify the list to play a song?
Application: Computer Music
Download Teacher/Student DocsObjectives:
- Use the for loop to control the number of notes
- Use the random number generator to create random musical notes
Intro 2
In this unit you used for loops to control light, colors, and sounds. In this application, you will create a program to play computer-generated random sound or music. This challenge can take three approaches:
- play purely random tones (frequencies),
- play random notes using their special frequencies, or
- play random notes using their names (in a list).
You will also use random durations (timings) for each tone/note. And, for the icing on the cake, each note can create a different color using the color LED.
Step 1
Make a new Python Hub Project (this one is named MUSICB) and import color and sound from the [math] ti_hub… menu.
You will also need a function that can produce ‘random’ numbers. These functions are part of the standard Python commands but are found in a separate module that the Hub Project template does not import.
Press [math] random… and add the statement
from random import *
to your collection of import statements at the top of your code.
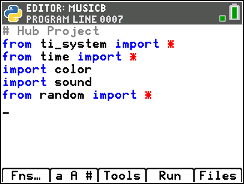
Step 2
Write an input statement to enter the number of sounds to play (n).
Convert the value of n to an integer.
Use n in a for loop to play the random sounds.
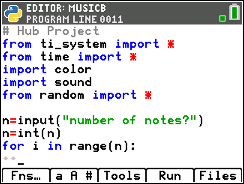
Step 3
Use the randint() function found in [math]random…
r = randint( , )
The variable r is assigned a random integer from min to max for later use. min and max will be replaced with numbers. But, before you enter those numbers, consider the next step…
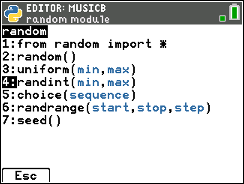
Step 4
r represents a sound frequency. Not all frequencies are ‘audible’. Very small frequencies and very large frequencies should be avoided because they are outside our hearing range.
Recall that when working with music in the previous lesson you used frequencies in the hundreds, so when choosing min and max keep that in mind.
Add another random variable t (for time) and use the uniform() random number generator also found on <math> random… . This returns a random decimal number between min and max so some notes will play for part of a second. Your choice of min and max here will depend on how long you want each note to last.
Step 5
Next make the sound using sound.tone() and enter the variables representing frequency and time, the variables r and t respectively.
Add the sleep() function to pause the program while the tone is playing. For how long should the program wait?
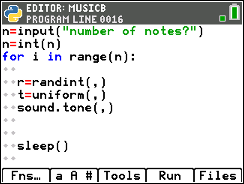
Step 6
How about using the color LED? Remember that there are three color channels, red, green, and blue, that are limited to the values 0 to 255. You can have the LED light up in purely random colors using the randint( , ) function for each channel. For example:
red = randint( 0 , 255 )
Or you can make the colors depend on the frequency r or the time t or both. But be careful about going ‘out of range’ beyond 255.
The screen to the right is not the complete program! You need to fill in the proper elements in each function.
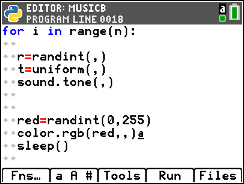
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 3: Brightness, if and while With the TI-Innovator™ Hub
Skill Builder 1: Measuring Light
Download Teacher/Student DocsIn this lesson, you will take control of the brightness sensor on the TI-Innovator™ Hub and learn how to make use of its information.
Objectives:
- Read the brightness sensor
- Set the range of the brightness sensor
- Monitor the brightness sensor
- Control a light with the brightness sensor
Intro 2
Unlike the light, color, and sound features of the TI-Innovator™ Hub, the brightness sensor is an Input device rather than an Output device. A program can obtain information from the brightness sensor and take actions based on that numeric value. You can either work with the default brightness values or you can set the range of values with a special brightness.range() function.
The brightness sensor is clearly labeled on one end of the TI-Innovator™ Hub.
Step 1
Begin a new Python Hub Project, import the brightns module from [math] ti_hub… Built-in devices… and start with these three statements found on [math] ti_hub… Commands
disp_clr()
disp_at(11, “Press clear to end”, ”center”)
while not escape():
♦ ♦
disp_clr( ) clears the calculator screen.
disp_at( ) displays the message at the bottom center of the screen.
“center” is chosen from a special sub-menu.
“Press clear to end” is typed in by hand.
The [clear] key acts as an ‘escape’ key.
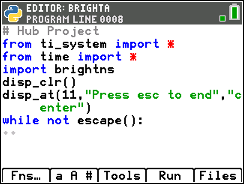
Step 2
In the while block use two statements: one to read the brightness and one to display the resulting value.
Assign the brightness measurement to the variable b:
♦ ♦b = brightns.measurement()
[math] Brightness….
Then:
♦ ♦disp_at(6, "brightness = " + str(b), "left")
found on [math] ti_hub… Commands
disp_at(6… is the middle line on the screen.
See the next step for an explanation of + str(b) …
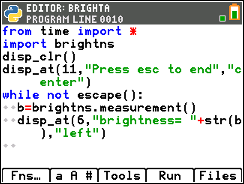
Step 3
About "brightness = " + str(b):
str(b) (found on <Fns… > Type) converts the numeric value of b into a string because disp_at() can only display text (characters), not values of numeric variables.
The + (addition) sign combines the string “brightness = “ with the string value of b. This ‘addition of strings’ is called concatenation.
Alignment “left” is better than “center” in this case. If you prefer to use “center”, then new data might not completely erase old data since the line will vary in length. You can move the text closer to the center by adding spaces before “ brightness = ” (inside the quotes). You can change the alignment by replacing “left” with “center” or “right”.
You can also suppress (hide) the cursor by using disp_cursor(0) before the while loop (not shown).
<Run> the program to see the output screen shown to the right.Step 4
Slow the display down a bit by adding a sleep() statement right after the disp_at() statement. Be sure it is indented to match the other statements in the while block. Enter a delay value (number of seconds) for the argument.
<Run> the program again and determine the lowest and highest values that the brightness sensor delivers by changing the light intensity hitting the sensor.
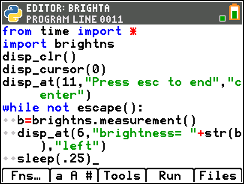
Step 5
You can set the range of values that the brightness sensor delivers using the statement
brightns.range(min, max)
found on [math]> Brightness…
Place this statement before the while loop. Use any values for min and max but be sure that min < max.
Change the two range values (we use 0, 255) and run the program again to observe the values produced. You now have a custom digital light meter.
Why is this important? We’ll see in the next few lessons…
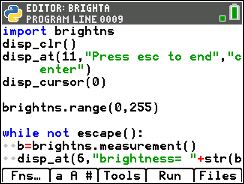
Skill Builder 2: Make a Light Switch
Download Teacher/Student DocsIn this lesson, you will learn to use the brightness sensor to control the light (the red LED) automatically.
Objectives:
- Set up and monitor the brightness sensor
- Introduce the if statements
- Control the TI-Innovator™ Hub light (the red LED) using the brightness sensor
Intro 2
Now that you can monitor the light coming into the TI-Innovator™ Hub, use that information to cause the onboard light (the red LED) to switch on/off when the brightness value changes.
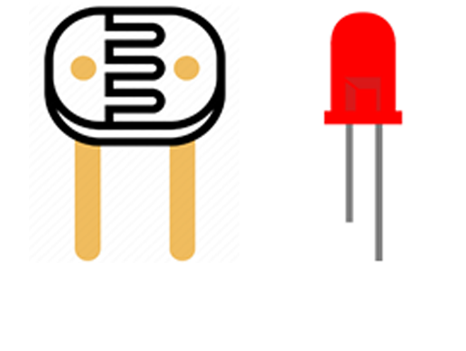
Step 1
Make a copy of the program you used in Skill Builder 1 of this Unit.
To copy a program (from the Editor or Shell), select <Files> to go to the File Manager, use the arrow keys to point the selection arrow on the screen to your BRIGHTA program (shown) and select <Manage>. Choose Replicate Program and type the new program’s name, BRIGHTB [enter].
Your new program appears in the Editor and will appear in the <Files> list later.
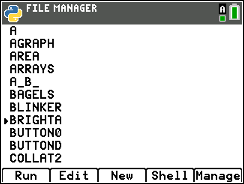
Step 2
The if statements:
On the <Files> Ctl menu there are three if… statements shown at the right. Each version is used in different programming situations and all of them depend on one or more logical conditions (expressions that are either True or False):
if <condition>:
if <condition>:
if <condition1>:
block
block
block
else:
elif <condition2>:
block
block
else:
block
elif is Python’s version of ‘else if’.
Note that if : and elif : require a condition between the word and the colon. else: does not.
Step 3
Immediately after reading the brightness in your program, insert the if..else structure from <Files> Ctl.
The <condition> placed after the word if will depend on the brightness variable b.
♦ ♦if b > 25:
♦ ♦ ♦ ♦ TRUE block
♦ ♦else:
♦ ♦ ♦ ♦ FALSE block
The ‘greater than’ operator (>) is found on the [test] menu ([2nd ] [math]) along with all the other relational and logical operators. Be sure to leave the colon (:) at the end of the if statement.
The value 25 is only a sample value. You will change it to adapt to your lighting situation.
Note: if and else are indented two spaces because they are inside the while loop. But the if : and else: blocks are indented another two spaces. Indentation is the Python way of indicating these special blocks of code. See the <Tools> menu for Indent and Dedent (un-indent) options or just place spaces (or delete spaces) at the beginning of a line to control indentation. The gray diamonds are this Editor’s method of indicating the indentation. Improper indentation can result in either a syntax error or a programmer error.
Step 4
In order to control the light on the TI-Innovator Hub you must import light. Place this statement at the top of your code along with the other import statements. Find import light on <math> ti_hub… Built-in devices.
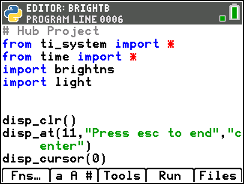
Step 5
Complete the two missing (if and else) blocks: One will turn the light (the red LED) on and the other will turn it off. Study the logic of the structure to decide which action goes in which block. In the screen to the right, we leave the answer up to you. There are ??? in the screen image for you to replace with the proper functions.
After entering the proper statements, <Run> the program. Change the brightness reaching the sensor. You may have to adjust the threshold value of 25 in the if statement to suit your lighting situation and your brightns.range( ) setting.
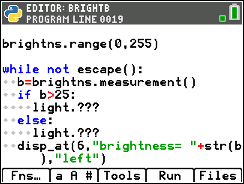
Skill Builder 3: Brightness and Color
Download Teacher/Student DocsIn this lesson, you will use the brightness sensor to control the color LED.
Objectives:
- Use brightness.range() to change the brightness scale
- Use the brightness value to light up the color LED
- Investigate numeric transformations
Intro 2
Unlike the TI-Innovator™ Hub light (the red LED), the color LED can vary in brightness. You will now use the brightness sensor to control that LED.
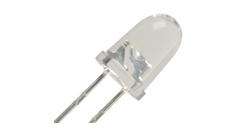
Step 1
Make another copy of your brightness metering program We copied BRIGHTA to BRIGHTC. Add import color at the top of your code found on [math] ti_hub….
Since the three color channels of the color LED only permit values from 0 to 255, set the brightness range from 0 to 255.
brightness.range(0, 255)
The brightness value b can now be used as values for the three color channels.
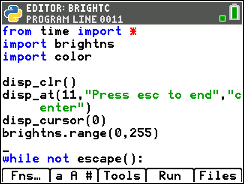
Step 2
Add a statement after the brightness.measurement() statement to light up the color LED using the variable b for all three channels:
color.rgb(b,b,b)
from [math] Color…
Use the variable b for all three color channels.
<Run> your program.
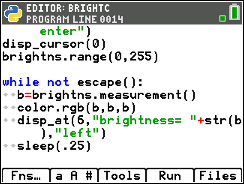
Step 3
Notice that the LED gets brighter as the light level increases. This is backwards! The darker the room, the brighter the light should be. Change the variable b after the brightns.measurement() to make the LED bright for low values and dim for large values. (See b=??? in the screen to the right.)
Try it yourself before proceeding to the next step.
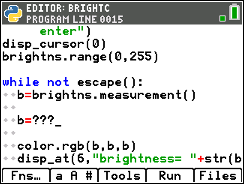
Step 4
Here’s an expression that works:
b = 255 - b
When b is 0, the expression 255-b is 255; when b is 255, the expression 255-b is 0. The effect of the statement is to ‘reverse’ the values of b.
You may have to move (cut/paste) your disp_at() statement in the program to display the original value of b and not the transformed value or have two disp_at() statements to show both values.
Can you modify the program to produce other colors besides white?
When the program ends, the color LED may remain on. Add a statement at the end of the loop (not indented) to turn the color LED off.
Application: Lite Music
Download Teacher/Student DocsIn this application, you will control sounds using the brightness sensor. There are three parts to this project:
- Light tones (frequencies)
- Notes using tones (frequencies of notes)
- Notes using a list of note “names”
Objectives:
- Set the brightness.range() so that the value is suitable for making sounds
- Play sounds or musical notes by varying the brightness
Intro 2
In an earlier lesson, you learned about sound.tone() and sound.note() using the TI-Innovator Hub. In this lesson, you will use the brightness sensor to create ‘noise’ and ‘music’ (sometimes it is hard to tell the difference!). There are three different options when working with the Sound module and this activity demonstrates all three approaches.
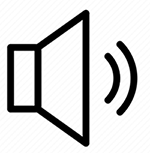
Step 1
Part 1: Light Tones
Again, use the original ‘brightness meter’ program from the first lesson in this unit. Make another copy of the program using <Files> <Manage> Replicate Program (ours is BRIGHTD) and add import sound near the top using [math] ti_hub… Hub Built-in devices.
In the next step you must decide what brightns.range() would be appropriate to use to make sounds that you can hear.
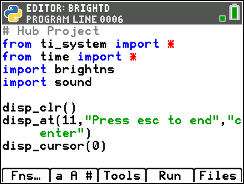
Step 2
For tones we can use any frequency between 0 and 8000 Hz, but many of these frequencies are too high or too low for humans to hear. Start with a range of (100, 1000) and adjust it to your liking later.
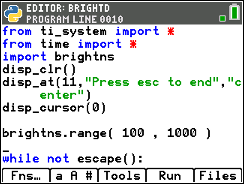
Step 3
Add the sound.tone( ) statement below the brightns.measurement() statement and use the variable b for the frequency argument. Set the sound’s time to your preference and use the same value in the sleep() statement so that the TI-Innovator Hub and the handheld are in sync.
Try making the sleep() value a little larger than the tone time value. This puts a little silent gap between sounds.
<Run> your program now and then adjust the numbers you used.
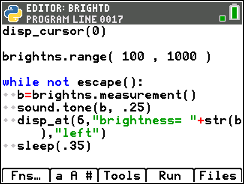
Step 4
In the five octaves pictured, there are a total of 60 notes (12 per octave).
Note A1 (A in the first octave) has frequency 55 Hz.
Subsequent notes have frequency 55 * 2 ** (k/12), where k is the note number after A1. A1 is note number zero since when k=0, 2**(0/12) = 1.
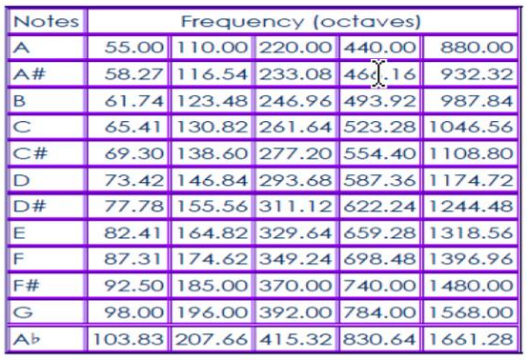
Step 5
To play ‘notes,’ modify your program:
- Change the brightns.range() to be 0…59.
- brightns.measurement() produces a decimal value but we only want integers so
- convert b to an integer using b = int(b).
int( ) is found on <Fns...> Type.
Calculate a note’s frequency using f = 55*2**(b/12).
Use the variable f in the sound.tone( , ) statement for frequency.
Try the program again. Some notes might be too high or too low. What can you do to limit the range of the notes?
Step 6
Part 3: Notes Using a List
Recall that the sound object can also use .note “names”.
At the top of your program (before the while loop), make a list of note “names” as you did in an earlier lesson:
notes = [“C5”, ”D5”, ”E5”,… ]
Set the brightness.range( , ) to be (0, # of notes in your list -1).
Convert the variable b to an integer.
Use the variable b as the index of the notes list:
; sound.note(notes[b], .25)
Run the program now.
This program plays the notes in the list with low brightness using the beginning notes and high brightness using the notes from the end of the list.
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 4: Rover’s Driving Features
Skill Builder 1: Make It Move!
Download Teacher/Student DocsIn this lesson, you will begin to operate the TI-Innovator™ Rover robotic vehicle by making Rover move and turn.
Objectives:
- Make Rover move
- Make Rover drive in a pattern
Intro 2
Python’s TI-Innovator™ Rover commands all begin with rv. This syntax is a result of the way that the ti_rover module is imported into your programs (import ti_rover as rv). The same is true of the ti_plotlib module. This technique is called ‘aliasing’ the module name (replacing it with a shorter name).
When you write a program that operates Rover, you are controlling the vehicle through easy-to-use commands but there’s a lot going on ‘behind the scenes’ just like other TI-Innovator™ Hub commands.
Be sure your TI-84 Plus CE Python is connected to Rover and that Rover’s power is turned on.
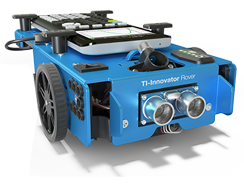
Step 1
Begin a <New> Python program and select the Rover template from the <Type> menu when entering the name of the program. Ours is ROVER1. (turn [alpha-lock] off to type the digit 1 in the program name).
Note the last import statement: import ti_rover as rv. This syntax means that all functions selected from this module will begin with the prefix ‘rv.’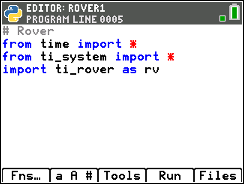
Step 2
To make Rover go forward use <math> ti_rover… to get this statement from the Drive menu shown:
rv.forward(distance)
Notice that the menu does not include rv. in front of forward but rv. is pasted into your program. This is done to save space on the menu.
The distance argument is measured in ‘grid units’ and you will be able to determine what that means in a moment. Use the number 1 for the argument and run the program. If Rover moves… hooray!
But… just how far did Rover move? Measure the distance that Rover moved.
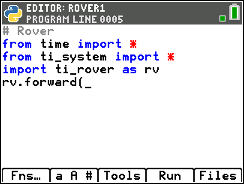
Step 3
Try the four basic drive commands: forward, backward, left, and right.
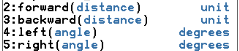
Step 4
To drive a square:
Erase the drive commands you entered (or make them comments using [2nd] [3] for the # symbol as shown).
Use a for loop to make Rover drive in a square pattern.
Begin with:
for i in range(size):
♦ ♦
which is found on <Fns…> Ctl
Use an appropriate size (the number of sides of a square) to make a square and add just two statements in the (indented) loop block. Try it yourself and run the program.
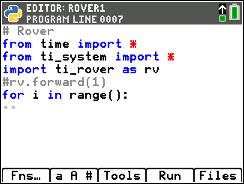
Step 5
Did you write something like this?
♦ ♦rv.forward(1)
♦ ♦rv.right(90)
If Rover moves in a square pattern… Congratulations!
Can you make a larger square? A different shape? Try adding a marker to Rover to draw the patterns.
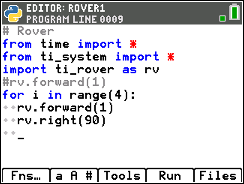
Skill Builder 2: Light at the Corners
Download Teacher/Student DocsIn this lesson, you will learn about two interesting features of Rover: the color LED and waiting for Rover to be ready for the next task.
Objectives:
- Control Rover’s color LED
- Wait for Rover to finish the current task before starting a new task
- Lighting up the LED at the proper time
Intro 2
On top of Rover near the battery strength meter (the 4 green lights) there is a color LED just like on the TI-Innovator™ Hub. But you cannot see the TI-Innovator Hub’s LEDs. This LED works like the TI-Innovator Hub LED. It requires values in the form (red, green, blue).
In a new Rover Coding template program, look at the color… command found on [math] ti_rover… I/O Outputs which is pasted into your program as
rv.color_rgb( , , ).
Provide values for the three color channels, red, green, and blue (0 to 255 each) then <Run> the program to see the LED light up in your chosen color.
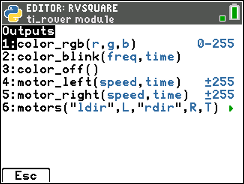
Step 1
In the previous lesson, you made Rover drive in a square pattern using a for loop. In this lesson, you will have the color LED light up in red at the corners only.
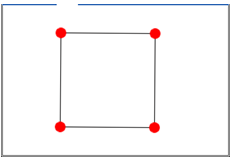
Step 2
Make a copy of your square program from Unit 4 Skill Builder 1.
<Files> (point to program) <Manage> Replicate Program
Just before the turn statement (left or right) in your program, turn the LED red. After the turn statement, turn the LED off:
rv.color_rgb(255,0,0)
rv.left(90)
rv.color_off()
Both color statements are on the same menu:
[math] ti_rover… I/O Outputs.
<Run> the program. Does it do what you expected?
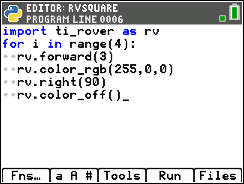
Step 3
This attempt does not work correctly because the TI-84 is working faster than the Rover. The calculator sends all the instructions to the TI-Innovator Hub as fast as it can. The TI-Innovator Hub then stores the driving instructions and processes them as a driver following a route on a mapping app, one at a time. The drive commands are stored in a ‘queue’ (a list) and are processed one at a time because each instruction takes some time to finish.
But the rv.color_rgb( ) function is not a driving command. When the TI-Innovator Hub receives this instruction, it is processed immediately (independent of the driving commands). So, the LED blinks rapidly four times right at the start of the driving.
Step 4
Fortunately, there is a statement that you can use to tell the program to wait until Rover is ‘ready’ to turn. After the rv.forward() statement add the statement
rv.wait_until_done( )found on [math] ti_rover… Commands
This instruction is telling the calculator to wait until it receives a signal from Rover that the Rover is finished with the rv.forward( ) command. The red LED then comes on and Rover turns.
Test your program now.
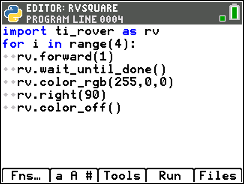
Step 5
Notice that the LED blinks quickly right at the beginning of the turn. The turning also takes some time to complete, so you must tell the calculator to wait again while the turning is taking place before turning the LED off.
Add another rv.wait_until_done() statement after the turn statement and before the LED is turned off so that the LED stays on throughout the entire turn.
Try your program again.
Can you have the LED light up in another color along the sides of the square? Hint: It only takes one more statement.
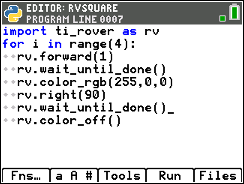
Skill Builder 3: Custom Turns and a Polygon
Download Teacher/Student DocsIn this lesson, you will use custom turning angles and have Rover drive along a pentagonal path, lighting up in two different colors: one along the sides and another around the corners.
Objectives:
- Controlling the amount of turning
- Use left() and right() with arguments
- Use colors around the pentagon
Intro 2
Your project in this lesson will make Rover drive a pentagonal path. The square was easy because you knew to turn 90 degrees. But for a pentagon, you must tell Rover how many degrees to turn at each vertex.
From your geometry experience, can you determine how many degrees Rover needs to turn at each vertex? See the hint in the picture to the right.
In addition to the driving, you will also have Rover put on a light show. Use the color LED on Rover to display intensities of one color along the sides of the pentagon and another color at the vertices.
And, for extra fun, you can insert a marker in the marker holder and draw the pentagon — on paper, not on the table or the floor!
Step 1
Begin by making a copy of the square-driving-with-lights program from the last lesson. Ours is named RVPENTA and we will change it from driving a square to driving a pentagon. The RVPENTA program is shown here before making changes to it.
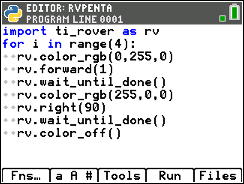
Step 2
Make two changes to convert from a square to a pentagon: there are 5 sides, not 4, and the angle to turn is 72 degrees (why?):
for i in range(5)
♦ ♦rv.right(72)
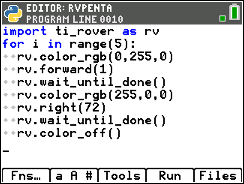
Step 3
Next, as Rover drives around the pentagon, the LED should start out with a dim color and gradually brighten. Use a variable or expression that makes the LED burn brighter at each side and each vertex. Your mathematics will come in handy.
Be careful to make sure that the color values stay between 0 and 255. You can use the variable i which varies from 0 to 4 to calculate a color value.
One possible expression: 50 + 50 * i which produces the values 50, 100, 150, 200, and 250 when the value of i is 0,1,2,3, and 4.
Note that the last statement, rv.color_off() is no longer indented. This takes the statement out of the loop so that the LED is not turned off at each vertex, but only once at the end of the program. To turn the LED off use either rv.color_rgb(0, 0, 0) or rv.color_off(). They do the same thing.
Application: Custom Polygons
Download Teacher/Student DocsIn this lesson, you will earn your Rover driver’s license by designing a "regular polygon" maker.
Objectives:
- Use input() statements to enter data for the number of vertices and the length of each side of a regular polygon
- Display lights along the sides and corners.
Intro 2
Now that you have successfully driven a square and a pentagonal path, you are ready to take your Rover driving test.
Create a program in which you enter the number of vertices and the length of each side of a regular polygon, drive that route, and light up the sky with a dazzling array of colors along the way. In addition to simply moving Rover, your program will seek input from the user, drive the proper distance for each side, and calculate the proper angle to turn at each vertex.
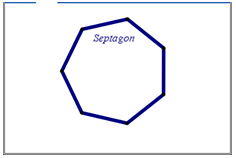
Step 1
Make a copy of your pentagon program from the last lesson. We name it RVPOLY. Your code should be similar to the image to the right. You will make some additions and changes to this code.
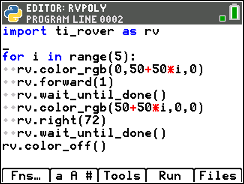
Step 2
Before the for loop, write two input statements to enter the number of vertices (n) and the length of each side (s).
n = ….(an input statement)
s = ….(another input statement)
Remember to use int(input(…)).
It is good to use more informative variable names like vertices and length. Just be careful not to use Python reserved words.
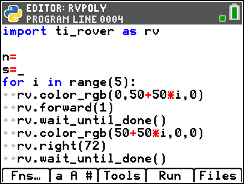
Step 3
There are three values in the loop statements that need to change:
range( ? ), forward( ? ), and right( ? ))
After editing those three arguments, <Run> your program, enter values for the input statements, and test your program.
Watch Rover carefully or insert a marker in the marker holder and draw the polygon on paper.
If you used an expression for the brightness of the LED along each side, then you will also have to adjust those LED statements to account for the change in the number of vertices. It would be better to use a variety of colors, not just red and green. Consider random colors. To ensure that the color values remain in the range (0..255) and do not cause an error, you can add (… ) % 255 to your color values expressions.
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 5: Rover’s Sensors
Skill Builder 1: Introducing Ranger
Download Teacher/Student DocsIn this lesson, you will learn to read the distance from the front of the TI-Innovator™ Rover to an obstacle. This lesson monitors that distance and displays information in two different ways. Another sensor is on the bottom of Rover and it can "see" colors.
Objectives:
- Read Rover’s Ranger measurement
- Display it on the screen using print()
- Display it on the screen using text_at()
- Determine the unit of measure
Intro 2
The two small cylinders on the front of Rover are not headlights. They are an Ultrasonic Ranger. A silent (to us) tone is sent out by one of the two sensors and the other one "hears" the echo when the sound bounces off an obstacle. The internal software then calculates the distance to the obstacle using the speed of the sound and the time that it takes for the echo to return to the sensor: D = V * T
All that happens in the blink of an eye!
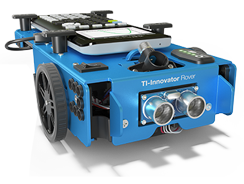
Step 1
Begin a new Python Rover Coding project. For this lesson, use the convenient ‘press [clear] to exit’ loop:
while not escape( ):
block
You will find this command on several menus, but since you are using Rover commands, look in [math] ti_rover… Commands.
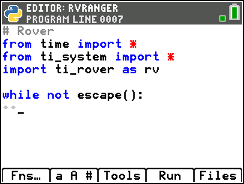
Step 2
Add three statements in the while block to
- read the distance in front of Rover:
d = rv.ranger_measurement()
find this on [math] ti_rover… I/O Inputs… - print it on the screen:
("distance= ",d) - wait before reading the next distance:
sleep(.5)
Run the program and move your hand toward and away from the front of Rover. Point Rover at a wall, the ceiling, and the floor. Watch the values that are displayed. Note: Rover does not move…yet.
Can you determine what distance unit (feet, meters, etc.) is being used?
Step 3
Rather than have the printed numbers scroll down the screen, improve the display of the distance values by using the disp_at() function. If you worked through the previous TI-Innovator™ Hub lessons (Units 1, 2, and 3), you have used that command before.
Add disp_clr() before the while loop and disp_at() in place of print() in the loop. Both functions are found on [math] ti_rover… Commands
Recall that disp_at( ) has three arguments: line number, string, and alignment. Use str(d) to convert the value of variable d to a string.
str( ) is found on <Fns…> Type.
Remember to use [2nd ] [3] for the # sign to comment a line.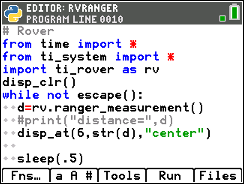
Step 4
You now have a digital tape measure. In the next lesson you will use this information to keep Rover from crashing into something.
Skill Builder 2: Off the Wall
Download Teacher/Student DocsIn this lesson, you will use the TI-Innovator™ Rover’s Ultrasonic Ranger to avoid an obstacle.
Objectives:
- Read the distance to an object in front of Rover
- If the distance is "too small," turn around and
- Continue driving
Intro 2
As Rover is driving, your program can use the Ultrasonic Ranger to detect an obstacle ahead and interrupt Rover to take another route. In this project, the Rover will drive back and forth between two opposite walls.
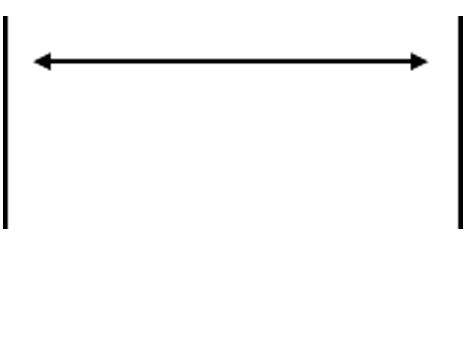
Step 1
Begin a new Python program using the Rover Coding template.
Get Rover moving using:
rv.forward (100)
Yes, that’s 10 meters, or about 39 feet, but there will be an obstacle in the way.
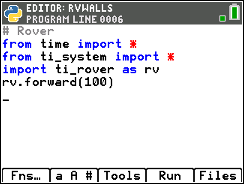
Step 2
Read the distance in front of Rover:
d = rv.ranger_measurement()
As long as there’s room to proceed, keep going. But if Rover is too close to an obstacle, turn around. How close is ‘too close’? That is for you to determine.
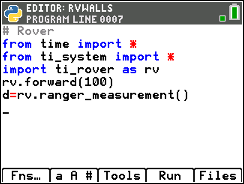
Step 3
Add a while loop to continue monitoring the distance as long as the obstacle is ‘far away’:
while d > ??? :
(Replace the question marks (???) with a ‘small’ number.)
♦ ♦d = rv.ranger_measurement()
For now, just stop Rover when the loop ends.
rv.stop()
Notes: while is on <Fns…> Ctl
> is on [test] ([2nd ] [math])
rv.stop() is on [math] ti_rover… Drive
Note: rv.stop( ) executes as a ‘pause’ and then rv.resume( ) continues with the Rover movements in the queue. But, if another movement command is executed after rv.stop( ), then the movement queue is cleared.
Step 3B
Test your program now by pointing Rover towards a ‘wall’ (less than 40 feet away) and <Run> the program. Rover should stop before hitting the ‘wall’.
Step 4
When Rover encounters the obstacle, the Rover should turn around. After Rover stops, have the Rover turn around using:
rv.left(180) or rv.right(180)
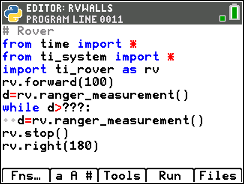
Step 5
After turning around, repeat the entire process again by making the whole program so far part of a while not escape( ) loop. Remember that Rover is moving, so it might be tricky to press the [clear] key.
Place the statement
while not escape( ):
at the beginning of your code (after the imports).
Indent all the statements below this while statement to make them the while block. On each line select <Tools> Indent. Note that one line is already indented because it’s part of another while loop. When one loop is embedded inside another the structures are ‘nested’.
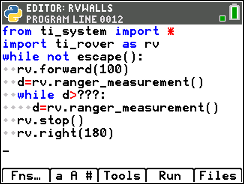
Step 6
Before running your program, add one more statement to the main loop. After the turn around statement (ours is rv.right(180)) add
rv.wait_until_done().
This is necessary because the turn command is part of the drive queue so the program will immediately proceed to read the ranger measurement which is not in the drive queue. Since the wall is still there, Rover will turn around again right away. The program must wait until Rover turns all the way around before driving forward again.
<Run> the program with Rover between two ‘walls’ or use your hand as a wall. Rover needs about 20cm to turn around since the stern is longer than the bow. while d > 0.2 gives Rover room to turn around. Try the program with and without the last wait… statement.
Skill Builder 3: Spot the Color
Download Teacher/Student DocsIn this lesson, you will learn to use Rover’s color sensor to change direction when a color is detected.
**Colored paper or large colored shapes are needed for this lesson.
Objectives:
- Use the color sensor to detect and react to a color
Intro 2
Rover has a color sensor on the bottom. You can see a light shining on the floor under the color sensor. The light helps Rover to see the color beneath it. First write a ‘test’ program to see what kind of values the color sensor produces and then you will be able to write a program to react to different colors. You will need some colored paper like construction paper or just print out some colored shapes like the rectangles at the right. They should be large enough for Rover to ‘see’.
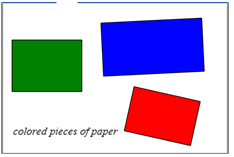
Intro 3
On the menu > TI-Rover > Inputs, there are five different color measurements available. The function color_measurement() returns a value from 1 to 9 where:
1=red, 2=green, 3=blue, 4=cyan, 5=magenta, 6=yellow, 7=black, 8=white, 9=gray
The other four measurements return the amount of the indicated color in the range 0…255, as shown on the menu.
Tip: do not confuse rv.color_measurement() (an input command that reads the color sensor on the bottom of Rover) with rv.color() (an output command that controls the color LED on top of Rover.
Step 1
Here is a short ‘test’ program using the Rover Coding template to determine the values that the color measurement functions produce.
while not escape( ):♦ ♦ c = rv.color_measurement()
♦ ♦ disp_at(7, str(c), "left")
Try all five color measurements (color_, red_, green_, blue_, and gray_) on various colored surfaces and observe the values displayed.
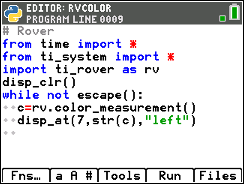
Step 2
Depending on your floor color (preferably white), make some colored pieces of paper (like ‘sticky notes’ or colorful construction paper) to place in front of Rover so that Rover can ‘see’ the change in color from the floor. Test these patches first to see what colors Rover ‘sees’.
Write a program to get Rover to react to the different colors. In the sample page to the right, the blue border should keep Rover on the page:
When Rover ‘sees’ blue, turn around.
When Rover ‘sees’ yellow, turn right or left.
You can also add statements to the program to control the color LED on Rover and to display the color value seen.
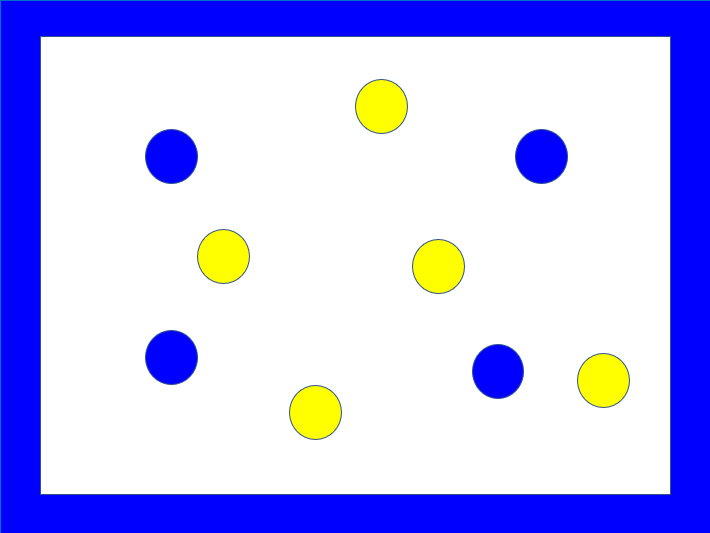
Step 3
Copy the color ‘test’ program you started. Add a statement before the loop to start Rover moving forward:
rv.forward(10)
Next, inside the loop, monitor the color below Rover…
♦ ♦ c = rv.color_measurement()
When color is blue, make Rover turn 180 degrees and, when the color is yellow, make Rover turn 90 degrees. This requires two if statements.
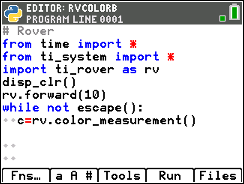
Step 4
Add two if statements and use the values you got from testing your color samples in place of the ?s:
if c == ?:
♦ ♦
if c == ?:
♦ ♦
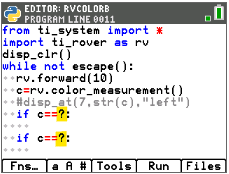
Step 5
The two if blocks are similar. Just remember blue — 180 degrees and yellow — 90 degrees.
Each block will:
- Stop Rover
- Turn (90 or 180)
- Go forward a little bit to move away from the colored spot
- Tell the TI-84 to wait until Rover is done with these three tasks
Try it yourself before proceeding…
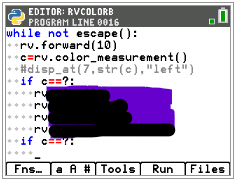
Step 6
Do your if blocks resemble this?
♦ ♦if c == 3:(Blue; use another number if your color is not blue.)
♦ ♦ ♦ ♦rv.stop()
♦ ♦ ♦ ♦rv.right(180)
♦ ♦ ♦ ♦rv.forward(1) # to move off the blue spot
♦ ♦ ♦ ♦rv.wait_until_done()
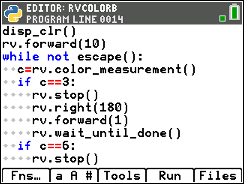
Step 7
When Rover ‘sees’ blue (when c==3) it turns around and goes forward a bit to get away from the red spot.
When Rover ‘sees’ yellow (when c==6) it turns 90 degrees and goes forward a bit.
At the bottom of the while loop get Rover moving again.
♦ ♦rv.forward(10)
Try using other color measurement functions and other driving actions to see what works best for your environment.
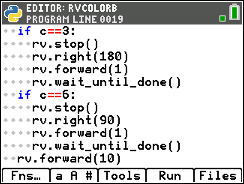
Application: The Winding Road
Download Teacher/Student DocsIn this application, you will use Rover’s color sensor to follow a curved path on paper. (A curved path on paper like the one shown on the next screen is needed for this application.)
Objectives:
- Use the color sensor to detect and follow a curved path on paper
Intro 2
Write a program to keep Rover ‘on track’ to follow a curved path like the one shown to the right.
Rover will start at the left edge of this BLUE and YELLOW page and travel to the right following the curved path across the paper.
When Rover ‘sees’ BLUE, it will turn to the left a little and move forward a little. When Rover ‘sees’ YELLOW, it will turn to the right a little and move forward a little.
Experiment with the turning angle and the moving distance to see how the Rover reacts to the two different colors.
If your page is blue and yellow as in this image, you can use blue_measurement() to see what values are given by each side of the paper. If you use different colors such as black/white, red/green, you can use gray_measurement() (or red_, green_, or blue_).
Step 1
Here is the original short ‘test’ program from the last lesson to determine the values that the color measurement functions produce.
Choose the color measurement type (color_, red_, green_, blue_, or gray_) that gives the greatest variation in values for your two different colors and the greatest consistency in values when looking at each of the colors separately.
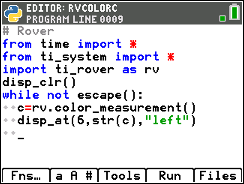
Step 2
For your path-following program:
- Start Rover on the left or right edge of this paper near the curved path
- Check the color
- If the color is BLUE, then turn a bit toward the YELLOW side
- Otherwise, turn a bit toward the BLUE side
- Move forward a short distance
- Repeat from step b until you reach the end of the path
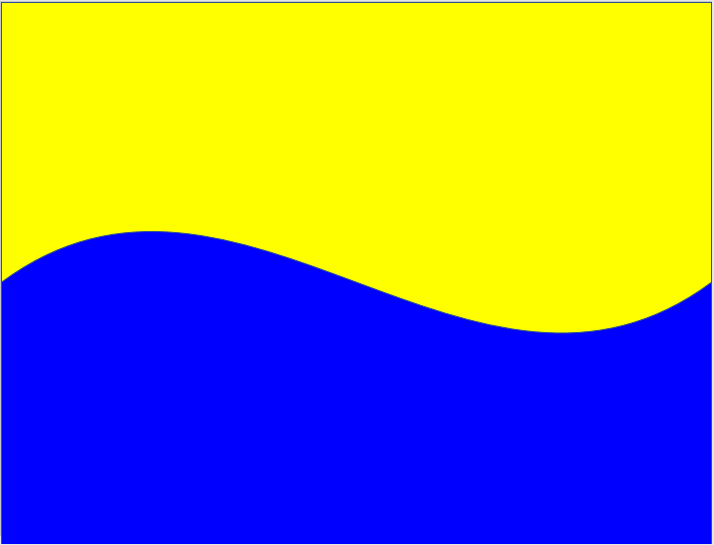
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 6: Rover’s Coordinates
Skill Builder 1: Drive and Plot
Download Teacher/Student DocsIn this lesson, you will learn about the Rover’s coordinate system and how to drive to a particular point represented by an ordered pair of numbers.
Objectives:
- Understand the Rover’s coordinate system and initial position and heading
- Make the Rover move to a certain point on the coordinate plane
- Plot Rover’s points on the TI-84 Plus CE Python screen
Intro 1
The Rover has a ‘built-in’ coordinate system just like a Cartesian graphing system. When you import ti_rover as rv, the Rover’s position on the coordinate grid is set to (0,0) and its heading is 0 degrees (pointing toward the positive x-axis, or ‘east’ on a map).
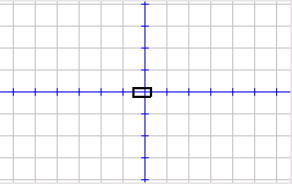
Intro 2
In addition to Rover’s coordinate grid, we can use the on-screen coordinate system that is available from the ti_plotlib module which is also imported into the Rover Coding template for just this reason. If you use these statements from menu [math]> ti_plotLib, you will get the screen to the right when you run:
import ti_plotlib as plt
plt.cls()
plt.grid(1,1,"dashed")
plt.window(-10,10,-7,7)
plt.axes("on")
plt.show_plot()
As we tell Rover to move around to points on the floor, we will also be plotting those points on the screen.
Step 1
Start a new Python program using the Rover template and add the statement:
import ti_plotlib as plt
found on [math] ti_plotlib…
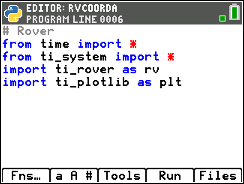
Step 2
Add the setup statements described earlier:
plt.cls()
plt.grid(1,1,"dashed")
plt.window(-10,10,-7,7)
plt.axes("on")
and add the statement to plot Rover’s starting position at the origin:
plt.plot(0,0,”o”)
found on [math] ti_plotlib Draw. You will select the dot type from an additional sub-menu.
Be sure to select the plot statement 5: plot(x, y, “mark”) and not 6: plot(xlist, ylist, “mark”).
Step 3
Depending on the space that you have, make Rover drive to a point in each of the four quadrants using rv.to_xy() found on menu > TI Rover > Drive.
rv.to_xy(1,1)
rv.to_xy(-1,1)
rv.to_xy(-1,-1)
rv.to_xy(1,-1)
You do not have to use 1s and you don’t even have to use the same numbers. But you do have to make sure that Rover visits all four quadrants.
Try your program now. Notice that Rover turns directly toward the position of the next point before moving there.
Step 4
Add plt.plot(x,y,”mark”) statements to your program to plot the points on the screen right after Rover reaches them. Try it now.
Did the program perform as you expected?
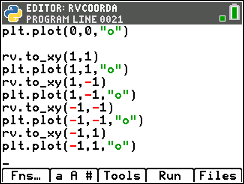
Step 5
Answer: No!
The points on the screen are plotted almost immediately when the program runs, and it takes Rover some time to drive to all four points.
How do you sync the plotting with the driving?
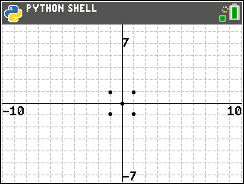
Step 6
Use the Rover function rv.wait_until_done() found on [math] ti_rover > Commands to pause the program while Rover is moving. You will need one of these functions for each driving point and order of the statements in the program does matter.
Try it now. Where will you place those wait functions?
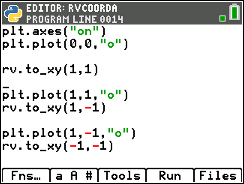
Step 7
At the end of your program, send Rover to the ‘home’ position, (0, 0). Also use the statement:
rv.to_angle(0, “degrees”)
found on [math] ti_over… to have Rover point in its original direction (‘east’). It’s nice to put the toys away, right?
And finally, to keep the graphic display on the screen add the statement:
plt.show_plot()
which waits for the [clear] key to be pressed before returning to the Python Shell prompt.
Skill Builder 2: The Distance Formula
Download Teacher/Student Docs**You will need a meter stick or metric tape measure for this lesson.
Objectives:
- Move to two different points
- Use a marker to draw the segment
- Use a function to compute the distance between two points and display the distance
- Measure the distance between the points
- Compute the error in the measurement versus the computation
Intro 2
d = sqrt((6 - 2)**2 + (4 - 1)**2)
This evaluates to: d = 5
Can you find a 3-4-5 right triangle in the image?
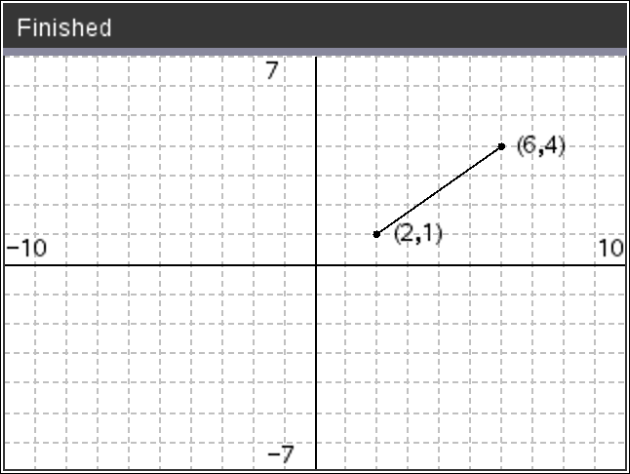
Step 1
Start a new Python Rover Coding project.
Define a function called dist which takes four arguments (two pairs of coordinates) and will return the distance between the two points.
The def function() template is found on menu > Built-ins > Functions.
The body of the function consists of one calculation:
and the return statement: return d
return is found on menu > Built-ins > Functions
Make sure these two statements are indented the same amount.
Note: there are two options for evaluating a square root:
( )**0.5
sqrt( )(must use from math import * for this function)
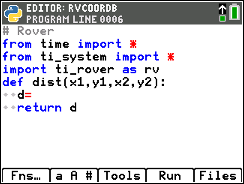
Step 2
Below the function (after the return statement), begin the main program. Be sure your code is no longer indented. Write four input() statements (using copy and paste) to enter the coordinates of the two points.
Create simple prompts for the inputs and use the float() function to convert the input result from a string to a decimal value. Only one of these four statements is shown to the right. We are using the variable a to store the first x-coordinate. Use b, c, and d for the other three coordinates.
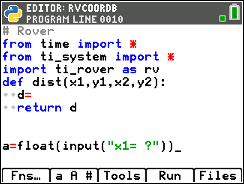
Step 3
After the four input() statements, make Rover drive to the first point:
rv.to_xy(a,b)
Pause there while you insert a marker in the Rover’s marker holder to draw a line segment. Then continue driving to the second point. A good pause technique is:
print(“insert marker”)
disp_wait( )
When you run the program and see ‘insert marker’ wait for Rover to stop and insert a marker into Rover and then press the [clear] key on the calculator.
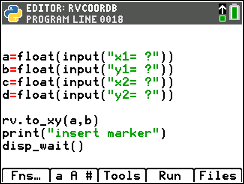
Step 4
Have Rover drive to the second point (making a line segment) and then use the dist function to determine the calculated distance between the two points:
cd = dist(a, b, c, d)
we use the variable cd for ‘calculated distance’
Notice that the letter ‘d’ is used as a variable in two different ways: in the main program, it represents the second point’s y-coordinate but in the dist( ) function it is used to store and return the value of the calculated distance. These two variables do not conflict with each other because they have a different ‘scope’: the part of the program where the variable ‘lives’ (or: is valid).
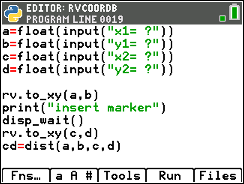
Step 5
Use a ruler or tape measure to determine the length of the segment that Rover made.
Add an input( ) statement to your program so that you can enter the measured distance, md.
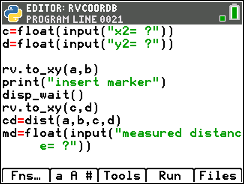
Step 6
Add two print() statements to display the two distance values.
How does the measured distance compare to the calculated distance?
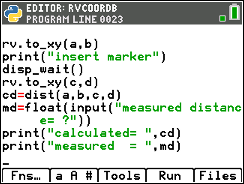
Step 7
Calculate the percent error using the formula
(measured - calculated) / calculated * 100
and print the error.
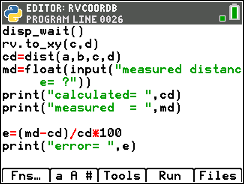
Skill Builder 3: Make the Shape
Download Teacher/Student DocsIn this lesson, you will write a program to make a pre-designed two-dimensional shape. You will program with lists and use a loop to get Rover to draw (or just drive) the shape on paper.
Objectives:
- Create lists in the calculator’s Stat Editor
- Use recall_list in Python
- Use a for loop to process elements in the lists
- Use a ‘pause’ statement to control processing
Intro 2
This project requires you to make two lists that represent the x- and y-coordinates of the vertices of a shape of your own design. In this lesson, we will use the design of the block letter T (for Texas!) shown to the right. Your goal is to have the Rover make this design using a marker (or just follow the route if no marker is available).
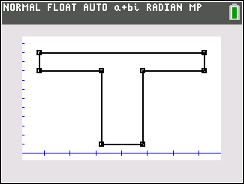
Step 1
Before writing the program, enter your shape’s coordinates into two lists in the TI-84 Stat Editor: Quit Python, press [2nd] [stat] and select Edit… from the menu. All the coordinates of the ‘T’ shape are given in the image to the right. Note that the names of the two lists are L1 and L2. These names are important when you write your Python program.
You can test your values by setting up a Scatter Plot ([2nd] [y=]) and an appropriate viewing window ([zoom] ZoomStat. You should see the plot shown in the previous step.
Why do we start and end with the same coordinate pair (4, 1)? What would it draw without the last 4,1?
Step 2
Now begin a new Python Rover Coding project.
Your first two new statements will get the lists from the TI-84 variables and store them in two Python variables.
From [math] ti_system… menu select the statement:
var=recall_list(“name”)
Reminder: the blue text is not included in your code.
You need two of these statements, so just copy and paste the statement using the <Tools> menu (or get the statement from the menu again).
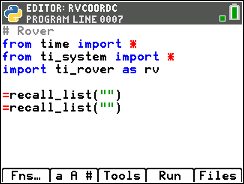
Step 3
To complete the statements, insert variables to the left of the = signs and, inside the quotes, type 1 and 2 for the lists L1 and L2 in the calculator.
We are using the variables xs and ys for the two Python lists.
This special TI-developed function copies data from the calculator side of the TI-84 into the Python environment. Just the numbers of the lists are needed.
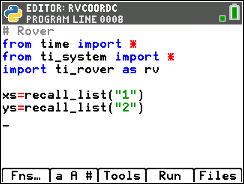
Step 4
You are now ready to program the Rover’s route. Remember that Rover begins at the point (0,0). Your first point might not be the origin, so have Rover move to the first point before pausing to insert the marker. The first point is ( xs[0], ys[0] ), so use the statement:
rv.to_xy(xs[0], ys[0])
- On the keypad (see [2nd] [x] (multiply) and [2nd] [-] (subtract).
- On the [list] menu ([2nd ] [stat])
- On the Python menu <Fns…> List
- On the <a A #> Character Map
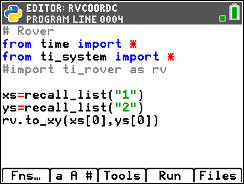
Step 5
Add a statement that pauses processing while you insert a marker (if you have one) after Rover reached the starting point:
disp_wait()
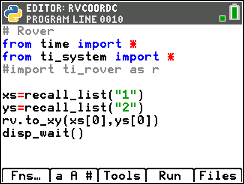
Step 6
Make a for loop to drive to the rest of the points:
for i in range(1, len(xs)):
♦ ♦
len(xs) is the length (size) of the list xs. If the length is 12, then the loop ends with the value i = 11, the last element of the list.
Notes: use the for i in range(start, stop) structure on <Fns…> Ctl
len( ) is found on <Fns…> List
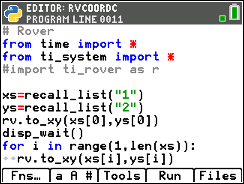
Step 7
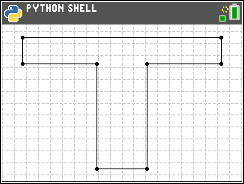
Application: A Random Walk
Download Teacher/Student DocsIn this application, you will see how often Rover can travel on the grid (making random turns north and east only) from the origin (0, 0) to the point (2, 2). You will also investigate the experimental and theoretical probabilities of reaching that point at random.
Objectives:
- Use a random number to decide in which direction to go next
- Determine success or failure of reaching the goal point (2,2)
- Plot coordinates on the screen
- Use the color LED to report success or failure
- Repeat the experiment multiple times and determine how often Rover reaches the goal
- Examine the theoretical probability of reaching the goal
Intro 2
Rover starts at the origin (O). At each grid point, Rover can move only east (to the right) or north (up) at random, one unit at a time. There are several different routes Rover can take to reach the goal (2, 2). One route is highlighted in red/bold in the image to the right. In how many different ways can Rover get to the goal? If each move is random it is possible that Rover will miss the goal.
What is the probability that Rover makes it to the goal?
Write a simulation of this problem to keep track of the number of times Rover makes it to the goal and determine the percentage of the trials that are successful.
Think about the failures: How do you know that Rover fails to make it to the goal?
Step 1
Begin a new Python Rover Coding project and add
import ti_plotlib as plt
Since this is a ‘random’ walk also add the random module:
from random import *
Set up a plt screen to display Rover’s positions along the route using the ti_plotlib Setup menu:
plt.cls()
plt.window(-1, 4, -1, 4)
plt.grid(1, 1, "dash")
plt.axes("on")
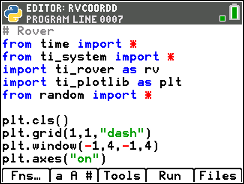
Step 2
Use a variable for the number of trials: tr = 10
Use a variable to count the successes: su = 0
Use two variables for the goal point: px, py = 2, 2 (Yes, this is valid!)
Use a for loop to perform the trials: for i in range(tr):
Now begin a trial:
In the loop block, use two different variables for Rover’s position:
♦ ♦rx, ry = 0, 0
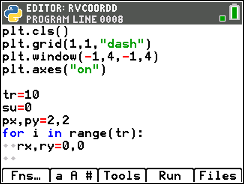
Step 3
Plot this initial point on the graphing screen:
♦ ♦plt.plot(rx, ry, ”o”)
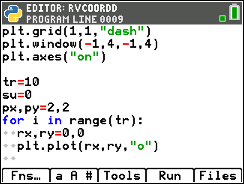
Step 4
Make a while loop that continues as long as Rover is not at the goal point and Rover has not failed. Think about what condition determines a failure.
This additional condition is left as an exercise.
Find != (‘does not equal’) and and on the [test] key ([2nd] [math])
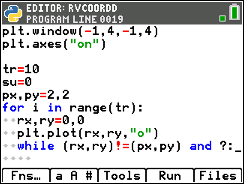
Step 5
In the while block, use randint(0, 1) to decide whether to go east (0 degrees) or north (90 degrees). This is accomplished with the statement:
♦ ♦ ♦ ♦dir = randint(0, 1) * 90
Recall that randint( , ) is found in the random… module.
Notice the extra indentation. This statement is in the while block which is in the for block.
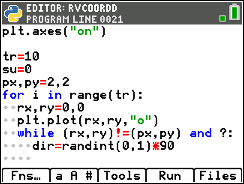
Step 6
Get Rover to turn to the correct angle (dir) and move forward 1 unit.
(The statements in the image are incomplete)
Add an rv.wait_until_done() statement to control the plotting speed.
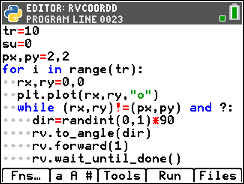
Step 7
Update Rover’s position variables, rx and ry.
If Rover moved east (dir == 0),
add 1 to rx.
Otherwise,
add 1 to ry.
Plot Rover’s position in the screen using plt.plot(rx,ry,”o”) using proper indentation!
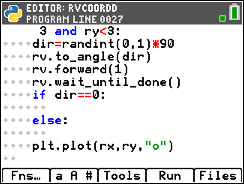
Step 8
After the while loop ends, determine if Rover was successful
if (rx, ry) == (px, py):
else:
and count the success by adding 1 to the variable successes.
Print “SUCCESS!” or “FAIL”.
If Rover is successful, light the color LED in GREEN, otherwise light the color LED in RED or use the colors of your choice.
- Two statements for Rover to return to (0,0) and face east (to angle 0 degrees)
- A statement to turn the LED off
- Statements to redraw the plotting screen starting with plt.cls(), and then use the three Setup statements again
Step 9
After the for loop ends (when all the trials are finished), print the results of the experiments:
- The total number of successes
- The percentage of the successes (successes / trials *100)
A sample run of 10 trials is shown in the image to the right. The percentage is the experimental probability of success.
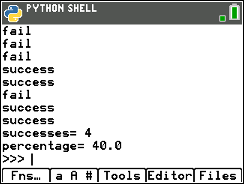
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 7: The TI-RGB Array
Skill Builder 1: Light Them Up
Download Teacher/Student DocsIn this lesson, you will learn to control the 16 LEDs on the TI-RGB Array both collectively (all at once) and individually (one at a time).
Objectives:
- Light up ALL LEDs and make them blink in unison
- Use another loop to light up and turn off the LEDs one at a time
Note: The simulation that is used in this unit to replicate the lights on the TI-RGB Array is used for demonstration purposes only. This tool is not a TI product and is not available for purchase or distribution by TI.
Caution: Rapidly flashing lights may be disturbing for some students, so it is wise to use sleep() statements to slow things down a bit.
Intro 2
TI-RGB Array | back | breadboard ports |
The TI-RGB Array is a circuit board with 16 color LEDs and a controller chip and comes with a short 4-wire cable. It connects to the TI-Innovator™ Hub using the breadboard (BB) ports on the TI-Innovator™ Hub. Follow the wiring instructions on the back of the circuit board to connect it to the TI-Innovator™ Hub; connect the TI-Innovator Hub to your TI-84 Plus CE Python.
Step 1
Start a new Python program using the Hub Project template.
Press [math] ti_hub… Output devices… and select the TI-RGB Array.
This pastes from rgb_arr import * into your code.
Again, press [math] and select the new TI-RGB Array… menu item at the bottom of the list. Select the first option var = rgb_array().
Then, in front of the = sign, type any variable name. We use r.
This is Object-Oriented Programming at work: The variable (r) is an instance (object) of the rgb_array class. You will use methods of the class in the rest of these lessons.
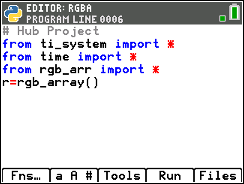
Step 2
To get the lights to light up all at once, on the next line of your program type your variable name followed by a period or decimal point.
r
Press [math] TI-RGB Array… and select var.set_all(r, g, b) so that the method is appended to the variable as shown.
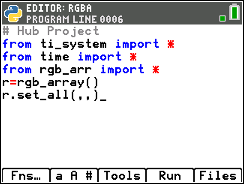
Step 3
Enter the three arguments, each one a value from 0 to 255, to control the color of the LEDs on the RGB Array.
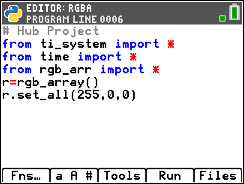
Step 4
<Run> your program to see all the LEDs light up in your selected color. Notice that the LEDs remain lit even after the program ends
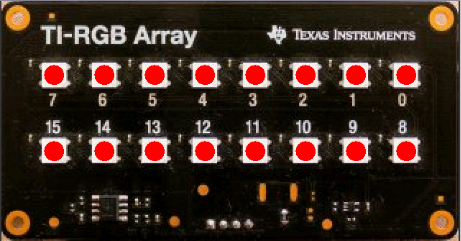
Step 5
r.all_off( )
found on the [math] TI-RGB Array menu.
Add a sleep( ) statement to keep the lights on for a few seconds otherwise you won’t see anything happen.
When you <Run> the program now you should see the LED come on and then turn off.
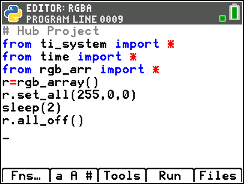
Step 6
Put your LED control statements into a for loop to make them blink on and off several times. Add another sleep() after they are turned off. You may want to adjust the sleep times to speed things up a bit. Be sure to indent all statements in the loop block using <Tools> Indent ► on each line.
Note: Do not include the constructor statement r = rgb_array() in the loop block. It only needs to be defined once!
<Run> your program to test it before you continue.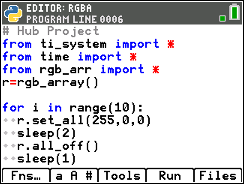
Step 7
If your program blinks all LEDs at once, then you are successful. Now let’s control the LEDs one at a time with an inner loop.
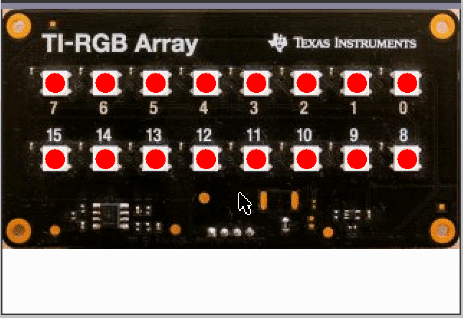
Step 8
Below the for i… loop, add an inner loop: for j in range(16):
Be sure to change the loop variable from i to j.
Indent all four loop block statements so that they now apply to the inner loop.
Change the statement r.set_all( r, g, b ) to:
r.set( j, r, g, b )
You can either erase the .set_all() statement and select the .set() statement from the menu or just edit the statement. r.set( , , , ) takes four arguments: the LED position and the three color values. Use the inner loop variable j as the led_position and enter your three color values.
Change the sleep values and (optionally) the outer loop range( ) argument to speed things up a bit.
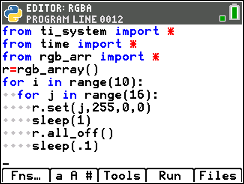
Step 9
<Run> your program. Now your 16 LEDs light up one at a time several times.
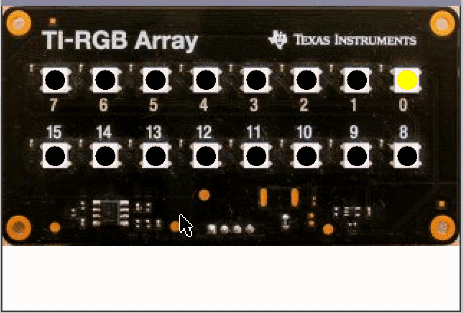
Skill Builder 2: A Rainbow of Color
Download Teacher/Student DocsIn this lesson, you will light up the LEDs in random colors.
Objectives:
- Import another module
- Use randint() to generate random LEDs in random colors
- Use esc to end the program
Step 1
Begin a new Python Hub Project. Add the TI-RGB Array module
[math] ti_hub… Output devices… TI-RGB Array…
Make a variable using the constructor =rgb_arr( ) by selecting it from:
[math] TI-RGB Array…
We use the variable r.
Add the while not escape() loop from
[math] ti_system…
so that your program will loop until you press the [clear] key.
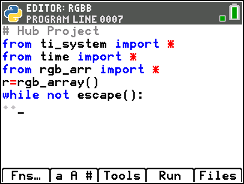
Step 2
Write four statements using randint() to assign values to variables representing the LED number (0…15) and the colors red, green, and blue.
randint() is found in the random module which is not included in the import section at the top of your program. You will have to add the module yourself. Find that import statement on [math] random…
Try it yourself before looking at the next step.
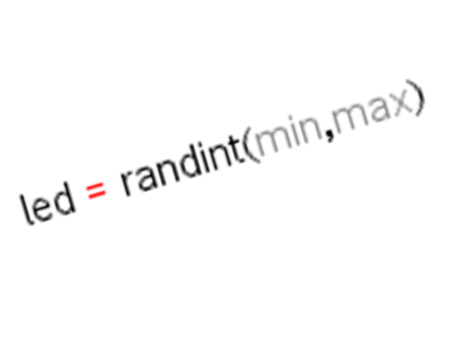
Step 3
Have the TI-RGB Array light up the random LED in the random color using:
r.set(led, red, g, b)
Try your program now. If the lights are flashing too fast, add a sleep() statement after the r.set( ) statement.
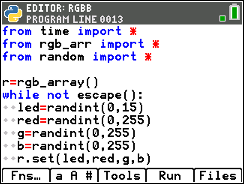
Step 4
At the end of your program, turn all the LEDs off:
r.all_off()
Notice that this statement is un-indented.
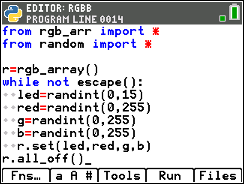
Step 5
Did your program work like this?
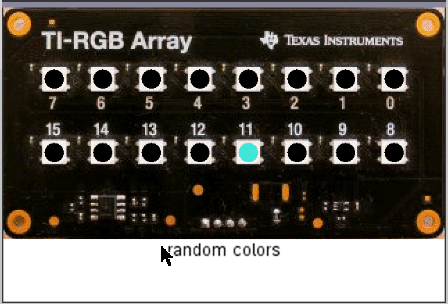
Skill Builder 3: Sequencing
Download Teacher/Student DocsIn this lesson, you will learn to control two LEDs at once in a loop to create a "marquee" effect.
Objectives:
- Use a for loop to light up a single varying LED
- Use a mathematics expression to control another LED at the same time
Intro 2
The overhead sign outside of some movie theatres has a border of lights flashing in sequence like ants marching. You can create a similar effect on the TI-RGB Array by turning on and off lights in sequence.
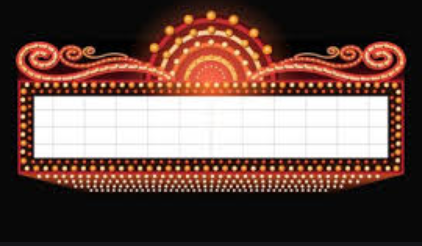
Intro 3
Your program in this lesson will light up two LEDs at a time, one on the top row going from right-to-left (0 to 7) and one on the bottom row going from left-to right (15 to 8). What is the relationship between the top row sequence and the bottom row sequence?
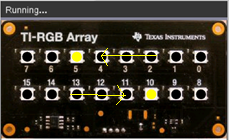
Step 1
Begin a new Python Hub Project and import the TI-RGB Array module.
Make a variable using the var=rgb_array( ) constructor. We use the variable r again, but you are free to choose your own variable.
Add the statement:
while not escape( ):
♦ ♦
found at [math] ti_system…
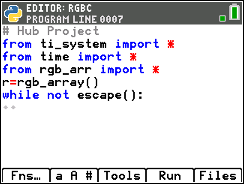
Step 2
Use a for loop to light up the top row in sequence from right to left (0 to 7).
♦ ♦ for t in range (8):
♦ ♦ ♦ ♦ r.set(t, 255,255,0) (This is yellow.)
Use the variable t because this controls the top row.
Remember that range(8) processes the numbers from 0 to 7.
Test your program now.
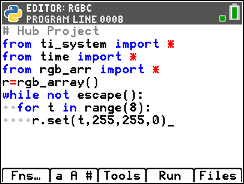
Step 3
All 8 LEDs light up very quickly and, at the end of the program, all the top row LEDs are on.
Next deal with the bottom row. The bottom row must go from 15 to 8.
What is the relationship between bottom (b) and top (t)?
♦ ♦ ♦ ♦ b = ? ? ?
♦ ♦ ♦ ♦ r.set(b, 255, 255, 0)
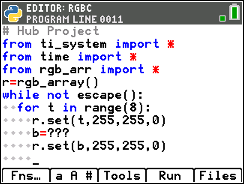
Step 4
Did you write b = 15 – t?
<Run> the program now. All 16 LEDs light up very quickly.
Add two statements: a sleep( ) statement to slow things down and a statement to turn all LEDs off at the bottom of the loop block.
♦ ♦ ♦ ♦ sleep(.25)
♦ ♦ ♦ ♦ r.all_off()
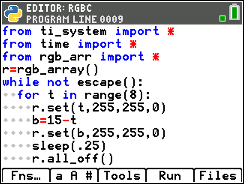
Step 5
Try your program again. Adjust the sleep() value and perhaps add another sleep() after all_off().
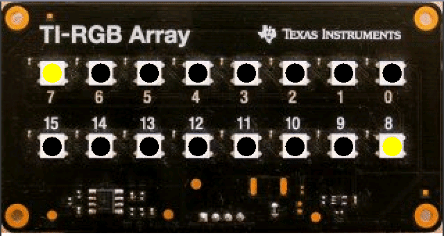
Application: Smart Lights
Download Teacher/Student DocsIn this application, you will control the number of LEDs lit on the TI-RGB Array using the TI-Innovator™ Hub’s brightness sensor.
Objectives:
- Use the brightness sensor to control the TI-RGB Array
- Adjust the brightness range to suit the TI-RGB Array
- Make sure that all 16 LEDs are impacted by the brightness
Intro 2
Smart Lights
As the room darkens, the lights in the room get brighter. Imagine a "smart home" with no light switches! Write a program that monitors the brightness and turns on more or less LEDs, as necessary.
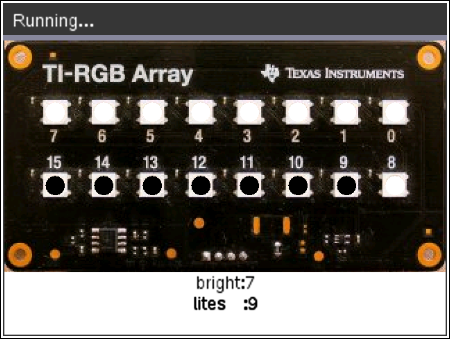
Step 1
As in the previous lessons, begin this Python Hub Project by importing the TI-RGB Array module and use the r = rgb_array() constructor and the while loop to terminate the program with [clear].
r = rgb_array()
while not escape():
♦ ♦
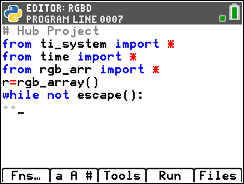
Step 2
To use the brightness sensor, you must first import the Brightness module. Press [math] ti_hub… Hub Built-in devicesBrightness
import brightns
Then, before the while loop, set the brightness.range() to match the number of LEDs on the TI-RGB Array board that could be lit: 0 to 16.
brightness.range(0,16)
by pressing [math] Brightness…
Use the range (0, 16) because this is the range of the number of LEDs to light up on the board.
The maximum value the sensor will produce is 16. Is the minimum 0?
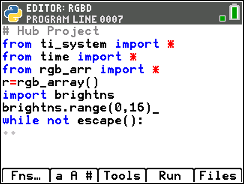
Step 3
In the while block, start by reading the brightness.measurement() and store the value in a variable (b).
♦ ♦ b = brightness.measurement()
The function produces a floating-point number (float, decimal). Convert it to an integer value using:
♦ ♦ b = int(bright)
or combine the two statements into one operation:
♦ ♦ b = int(brightness.measurement())
Recall that int( ) is found on <Fns…> Type
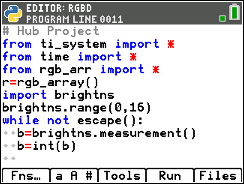
Step 4
To test your program: add disp_at() statement found on
[math] ti_hub Commands:
♦ ♦ disp_at(6, str(b), “left”)
Recall that you need str(b) because the disp_at( ) function requires a string to display. You can either type str( ) or get it from <Fns…> Type.
Run the program to ensure that all seventeen values (0…16) do appear. If not, then adjust the range() so that they do. Try using an artificial light source such as a flashlight or the ‘flashlight’ feature on a smartphone.
You may want to add disp_clr() before the loop (not shown).
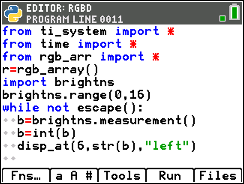
Step 5
Since 0 is the darkest value and 16 is the brightest, we want the number of LEDs lit to be the opposite: when bright = 0, there should be 16 LEDs lit and when bright is 16, there should be 0 LEDs lit.
Write an expression for the number of lights (l) in terms of brightness (b).
l = ? ? ?
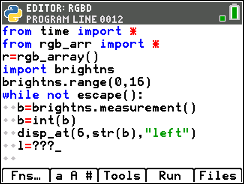
Step 6
It’s possible that all LEDs should be off:
♦ ♦ if l == 0:
♦ ♦ ♦ ♦ r.all_off()
♦ ♦ else:
♦ ♦ ♦ ♦
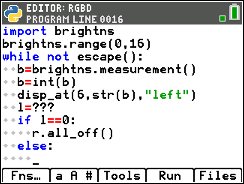
Step 7
We want all the LEDs to be affected by the brightness so we will use a for loop to control the state of every LED every time. The variable l is a deciding factor when turning an LED on or off:
for i in range(1,17):
(Remember that the value 17 is not processed by the loop. The variable i takes on the values from 1 to 16 thus representing the 16 LEDs.)
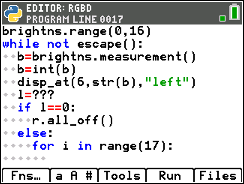
Step 8
Complete the program by adding an if…else… statement to tell the TI-Innovator Hub which LEDs are on and which ones are off.
Hint: If l is 1, then you want to turn on LED 0. When l is 16, you want to turn on all LEDs (#0 to #15). Use the color (255,255,255) to get a bright white light.
Remember to turn all the LEDs off just before the end of the program.
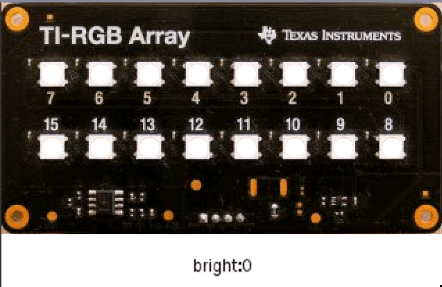
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application