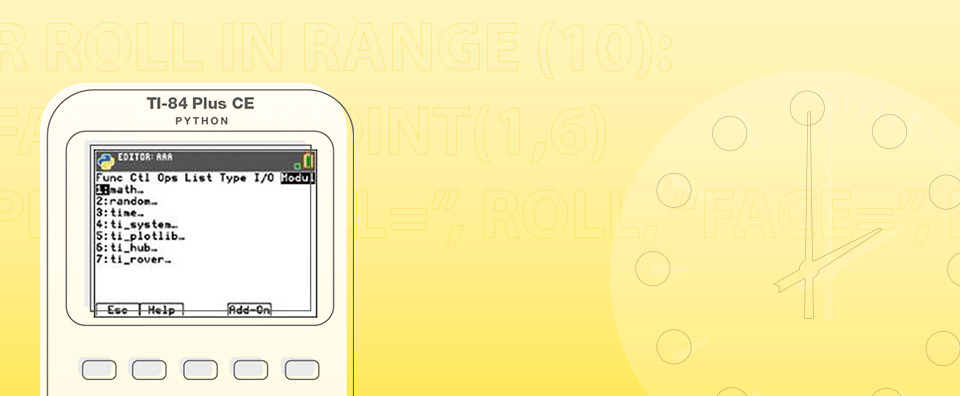
Python modules for TI-84 Plus CE Python graphing calculator
These short activities are designed to introduce
the TI modules, and demonstrate various ways in
which they can be used to extend the capabilities
of the TI-84 Plus CE Python graphing calculator.
Note: These lessons require the use of the TI-84 Plus CE Python graphing calculator.
Overview
The TI Modules
The Python programming environments developed for the TI-84 Plus CE Python include some special modules developed by Texas Instruments. Some of these modules are accessible on the main menus (like ti_system) and others are found in special sub-menus on the TI-84 Plus CE Python see <Fns..> Modul <Add-On>.
The core Python programming language is small and fast and was designed to be ‘modular’ with separate, external modules, packages, and libraries. The TI modules tap the unique features of the Texas Instruments family of handheld computers, allowing graphics programming and access to external devices such as the TI Innovator Hub, Rover, micro:bit and other devices.
Some of these modules are introduced through the core Units of the TI Codes materials. This section of TI Codes provides some activities that introduce the functionality of additional modules. As new modules are developed, this area will grow, so be sure to check back on occasion.
Turtle Graphics
Mini project 1: Getting started - Polygons
Download documentsTurtle Graphics is a TI-developed Python module ideal for folks new to graphics programming. Create simple or complex patterns, shapes, geometric forms and more in an entertaining, active, pesudo-physical learning experience.
Background: “Turtle Graphics” is a concept originally introduced into the programming language Logo created by Feurzeig, Solomon, and Papert in the mid-1960’s. The ‘turtle’ is a graphical object that responds to programming commands like forward(distance) and right(angle). The turtle module is object-oriented and the basic turtle functions are included on the turtle menus.
Intro 2
In the image to the right, the blue and yellow triangle represents the ‘turtle’. The turtle carries a ‘pen’ that can leave a trail behind as the turtle moves. The black segments are the path that the turtle followed.
To use turtle graphics on your TI-84 Plus CE Python, you must have the turtle module (TURTLE.8xv) on your calculator. Use TI-Connect CE to install the file to your calculator. The free turtle module and documentation can be found here.
This lesson assumes that you are using OS version 5.7 (or later) which includes a special ‘soft key’ to access some special TI-developed<Add-On> modules.
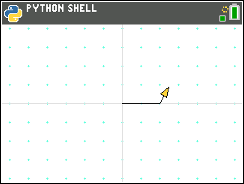
Step 1
Begin a new Python program. Ours is named TA. (Turtle program A)
Select <Fns…> Modul. At the bottom of the screen there is an
<Add-On> soft key. Select <Add-On> and select
from turtle import *
You also get the statement t = Turtle( ) in your code which creates a Turtle object variable named t.
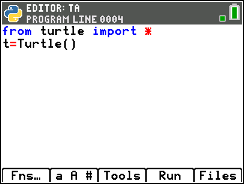
Step 2
All turtle statements (methods) by pressing [math] turtle…
Note that there are five sub-menus at the top of the screen:
Move Draw Pen Settings State
Each containing specific turtle functions. All turtle functions begin with ‘t.’ which is the default turtle name. You can change the turtle name (and even have multiple turtles in the same program) but you are then responsible for editing the turtle name. The Move menu is shown here. Check out the items on the other menus.
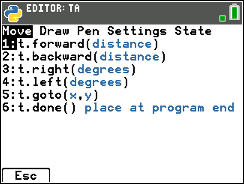
Step 3
To get the turtle to make a square, write a for loop that contains the two statements
t.forward(50) # pixels
t.left(90) # degrees
After the for loop add the statement
t.done()
which keeps the graphics on the screen until you press the [clear] key.
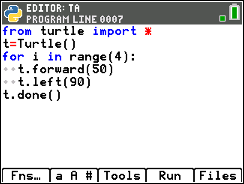
Step 4
After entering the code, <Run> the program to see the turtle make a square.
The turtle starts at the origin facing to the right. ‘50’ is 50 pixels on the screen. The grid dots are 25 pixels apart.. ‘90’ represents 90 degrees.
At the end of the program, the turtle is back at the origin facing to the right. The graphics remain on the screen until you press the [clear] key.
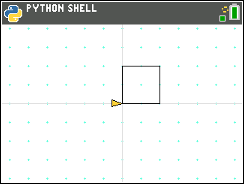
Step 5
Modify the square program to let the user enter the number of sides so that the turtle can draw a regular polygon. Use an input statement:
n=int(input(“num of sides? “))and alter the loop code.
The tricky part is to figure out what the turning angle should be when you know the number of sides. The user entered 7 sides to produce this screen showing a septagon.
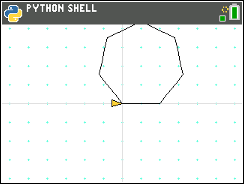
Step 6
Change the speed of the drawing using
t.speed(0) (fastest)
found on [math] turtle… Settings
The complete polygon code is shown here.
Note that the input statement precedes the t = Turtle() statement because the constructor puts the screen into the graphics mode.
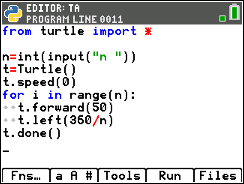
Step 7
Adding Color
There are two color settings on the [math] turtle… menus:
t.pencolor(r, g, b) (on the Pen submenu)
t.fillcolor(r, g, b) (on the Draw submenu)
You can change pencolor( ) on the fly, so each side of the polygon can be a different color (not shown). But fillcolor( ) must be set before drawing a shape. See the red hexagon example in the next step…
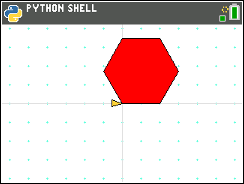
Step 8
To make the red hexagon in the previous step:
Set the fillcolor( , , ) before making the polygon and issue the t.begin_fill() command.
During the drawing process the system keeps a list of vertices visited.
After the polygon is drawn use the t.end_fill() method to fill the polygon.
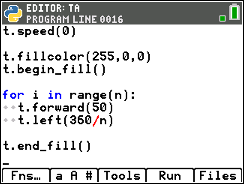
Step 9
Now modify the program to make randomly colored polygons at random places on the screen until the [clear] key is pressed.
- Use while not escape(): for the main loop.
- Use t.hideturtle() and t.hidegrid() to improve the appearance.
- Use t.penup(); t.goto( , ); t.setheading() and t.pendown() to place the turtle at a random starting point on the screen facing in a random direction.
The turtle screen ranges from -159 to 159 (pixels) horizontally and -106 to 106 vertically and it’s OK if the turtle goes off the screen.
The heading can be any angle (degrees).
Use the for loop to make each ploygon.
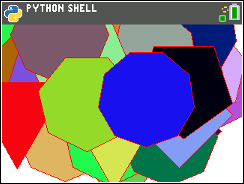
Mini project 2: Stars and Fireworks
Download documentsAfter learning about the basics of ‘Turtle Graphics’ in the previous lesson, let’s try to make a more complex display of stars and fireworks!
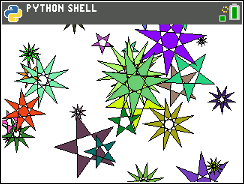
Step 1
Part 1: Drawing Stars
When making polygons using the turtle, you use the Geometric property that the sum of the exterior angles of a polygon is 360 degrees. When making a regular polygon the turning angle is therefore 360/n since all the angles are equal.
In the first part of this activity, you will use a similar property to produce star polygons like the one shown to the right.
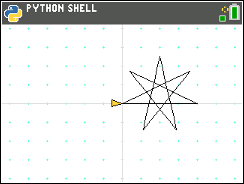
Step 2
First let’s take a look at the basic regular polygon program from the previous activity. The program shown here makes a regular septagon.
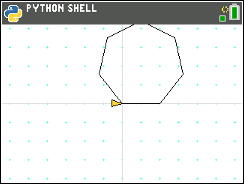
Step 3
Change the code to make a star…
In the turn function, instead of 360 use an integer multiple of 360.
To get this image we used 2*360.
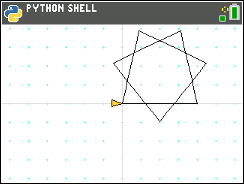
Step 4
You might try a higher multiple of 360 This star uses 3*360 instead of 2*360.
The multiple represents the number of revolutions that the turtle makes to complete the star. This is a (7/3) star polygon since it has seven vertices and takes the turtle three revolutions to complete.
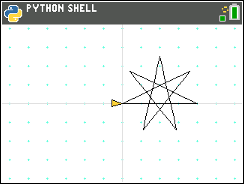
Step 5
When you add polygon filling to a star polygon, the results can be surprising.
Add t.fillcolor( , , ), t.begin_fill( ) and t.end_fill( ) to your program in the appropriate places.
The entire star is not filled. There are places that the fill algorithm skips because it is looking for ‘borders’.
Step 6
Part 2: Fireworks!
In this section of the activity you will use lots of random values to generate these awesome fireworks on the screen.
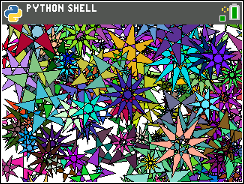
Step 7
In the star-making loop shown here, n is the number of vertices and r is the number of revolutions. What values for n and r make for pleasing stars?
We will limit n to be random odd numbers: 5,7,9,11…
For ‘pointy’ stars we will make r = int(n/2)
We will also use a random position, heading, color, and side length for each star in this project.
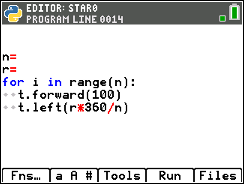
Step 8
First test these star-making options:
n = 2 * randint(2, 10) + 1 # odd numbers
r = int(n / 2 # or r = n // 2
Running this program will make a pointy star with an odd random number of vertices.
Step 9a
Now add code to the program to:
- Hide the turtle
- Hide the grid
- Make the turtle move fast: t.speed( 0 )
- Put the star-making code in a while not escape(): loop
- Assign a random odd value to n
- Calculate r
- Draw the star using the for loop
- Clear the screen to see the different stars. This is temporary.
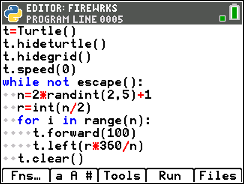
Step 9b
Test your program several times to see various stars displayed
like this one.
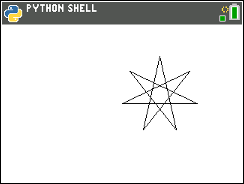
Step 10
We’re ready for the fireworks:
- each star will be drawn in a different position on the screen using a different heading with different colors and sizes.
- assign random values to appropriately-named variables to achieve the fireworks effect as seen here.
- #comment the t.clear( ) statement since we want to fill the screen with stars.
Run the program and enjoy the show!
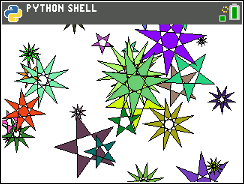
Mini project 3: Turtle Races
Download documentsThis activity extends your turtle skills and concepts and demonstrates the use of multiple turtles competing in a ‘race’ across the screen. Two turtles are in a race to the finish line! Let’s write a program that simulates this race.
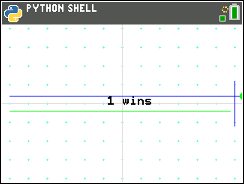
Step 1
Start a new Python program (TD) and add the turtle module to the code by using <Fns> Modul <Add-Ons>:
from turtle import *
Recall that selecting this statement from the menu actually pastes two statements into your Editor (separated by the semi-colon) on the same line as seen here.
But this program requires two turtles. The second line only creates one turtle object, t.
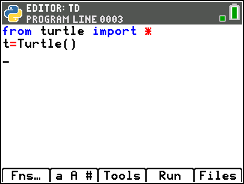
Step 2
Change the variable name of the turtle from t to t1. Copy and paste this line and name the second turtle t2.
t1 = Turtle()
t2 = Turtle()
When you select any turtle function from the menu you now have to modify the names of the turtles to suit this program.
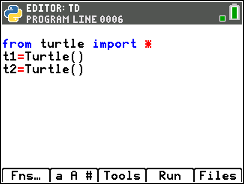
Step 3
Since the turtle window domain is -159 to 159, set the startline and finishline to be near the left and right edge of the screen:
startline = -150
finishline = 150
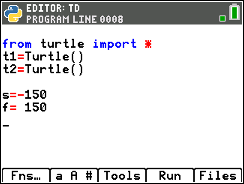
Step 4
Now do some turtle housekeeping: set the speed of each turtle to the same value (you can adjust these later) and hide both turtles.
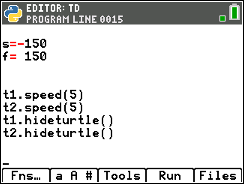
Step 5
Use one of the turtles to make the finish line:
t1.penup()
t1.goto(f,-30)
t1.pendown()
t1.goto(f,30)
t1.penup()
Remember that throughout this program, after selecting a turtle function from the menus, you must edit the turtle name by adding a 1 or 2 to the turtle name t. as necessary.
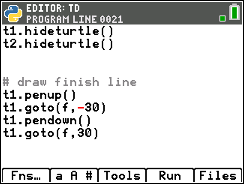
Step 6
You can test the program now to see that the finish line is showing on the right side of the screen as seen here.
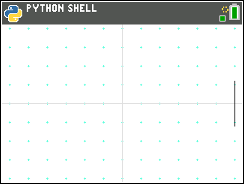
Step 7
Place the two turtles at the starting line and get them ready to race. Place one turtle above the x-axis and one below the x-axis Our two ‘lanes’ are y = 10 and y = -10. You can choose other lanes.
Make sure both turtle pens are up before moving them.
Use t.goto() to place the turtles at the start line.
t1.goto(s, 10)
t2.goto(s, –10)
t.goto is on the turtle… Move menu.
After the turtles have been moved, put the pens down again.
You can also set the pen color of each turtle. t.pencolor() is on Turtle Graphics > Pen Control
Think: in what direction are the (hidden) turtles now facing?
t.goto() does not affect the heading.
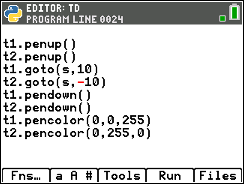
Step 8
We are ready to start the race…
while t1.xcor() < f and t2.xcor() < f:This while loop (the race) ends when one of the turtles crosses the finish line (f). The function t.xcor() gives a turtle’s x-coordinate and is found on the turtle… > State menu:
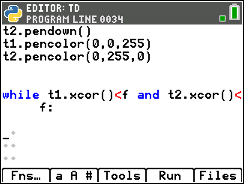
Step 9
Generate two random* values: d1 is the distance turtle 1 will move and d2 is the distance turtle 2 will move (in pixels). But they cannot both move at the same time! We let turtle 1 move first. (more about this later). Make a small dot at the turtle’s new position.
while t1.xcor() < f and t2.xcor() < f:
d1=randint(10, 30)
d2=randint(10, 30)
t1.forward(d1)
t1.dot(3)
*Remember to add from random import randint at the top of your program.
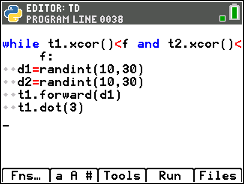
Step 10
Down the track, we make sure that turtle 1 has not crossed the finish line before letting turtle 2 make a move.
if t1.xcor() < f:t2.forward(d2)
t2.dot(3)
This is the end of the while loop. Test the program again to see the turtles race across the screen.
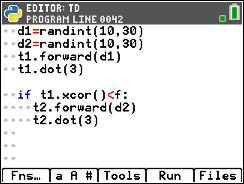
Step 11
After the whle loop ends, report the winner using an if…else structure:
if t1.xcor() >= f:
t1.penup()
t1.goto(-20,10)
t1.write('1 wins')
else:
t2.penup()
t2.goto(-20,3)
t2.write('2 wins')
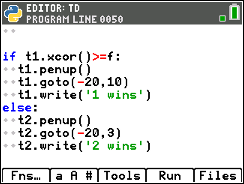
Step 12
Test your program now to see that both turtles can win and that the code is working properly.
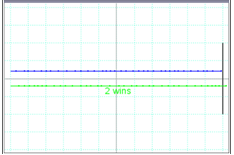
Step 13
Challenges:
- Is it ‘fair’ that turtle 1 always goes first? Build a routine that chooses a turtle to go first at random. Does it make a difference in the outcome? Should it be just the first move or every move that is random?
- Analyze the program: Embed the setup functions and the race in another
while get_key() != ”esc”:
loop, change the turtle speeds to 0, keep track of the number of wins for each turtle and display the them on the screen. Let the program run for awhile to see if the race is ‘fair’.
- Tortoise v. Hare
Tortoise and Hare are having a race. The slow but steady Tortoise takes small steps and the lazy Hare takes giant leaps. For every ten small (random) steps Tortoise takes, Hare makes one giant (random) leap. Create a race program between Tortoise and Hare in which each wins some of the races. Analyze your code as above to see how ‘fair’ your code is.
- MP 1
- MP 2
- MP 3
TI Draw
Mini project 1: Getting started - Circles
Download documentsComputer graphics is the most fun of all programming projects. Here’s an introduction the ti_draw module by making a collection of random colorful circles on the screen.
The <Add On> ti_draw module contains functions that let you produce colorful graphics like this on the screen.
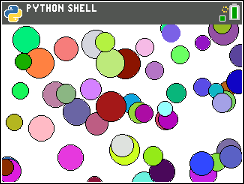
Step 1
In a new program add the three import statements:
from random import *
from ti_draw import *
from ti_system import *
To get ti_draw, select <Fns> Modul <Add Ons>. ti_draw appears along with all other ‘add-on’ modules you may have installed.
If you are not seeing TI Draw, make sure you have updated to latest OS. For more details go here.
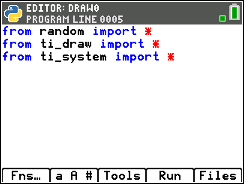
Step 2
When the statement from ti_draw import * is in the Editor, a new item, ti_draw…, appears on the Modul menu.
Use the [math] key as a shortcut to the Modul menu as seen here (but the <Add Ons> soft key does not appear).
This ti_draw… menu is where you find all the Shape drawing functions and Control tools on two sub-menus: Shape and Control.
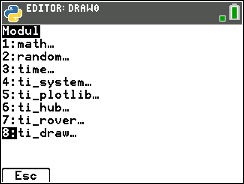
Step 3
The Shape menu:
Each of the Drawing functions on the Shape menu requires a certain number of arguments depending on the shape. The menu shows blue placeholders for the arguments, but they are not included in the function when inserted in the program.
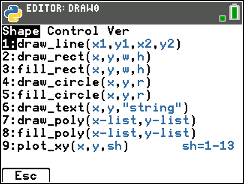
Step 4
The Control menu contains functions that affect the screen and drawing state (clear the screen or a region of the screen, color, pen size, and window coordinates).
The function show_draw( ) is used at the end of a program (or to pause a program) to keep the image on the screen until the [clear] key is pressed.
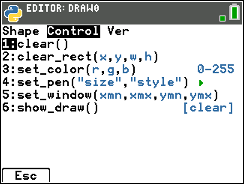
Step 5
Select clear( ) from the Control menu. This will clear the Shell screen to prepare it for drawing shapes on a blank ‘canvas’.
Get while not escape(): from [math] ti_system….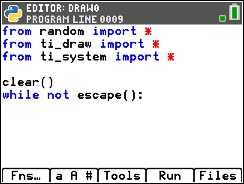
Step 6
To make random circles you need a bunch of random values for the positions, radii and colors of the circles. Use randint( , ) (from [math] random…) to assign appropriate values to the variables.
The screen is 318x212 pixels and the color values must be integers in the domain [0..255]. The pixel (0, 0) is in the upper left corner of the screen. It’s OK to plot graphics that are off-screen, too).
x and y are the coordinates of the center of the circle.
r is the radius of the circle.
a, b, and c are the three color values, red, green and blue, required for set_color( , , ) coming up next…
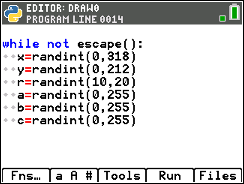
Step 7
Get set_color( , , ) from the [math] ti_draw… Control menu and use the variables a, b, and c for the three arguments controlling the red, green and blue channels of the pixels.
Note that you get the commas inside the parentheses, but not the blue argument placeholders seen in the menu.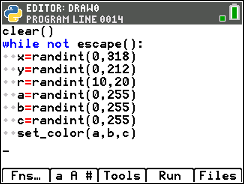
Step 8
Get fill_circle( ) from [math] ti_draw… Shape menu.
(x, y) is the center of the circle and r is the radius of the circle.
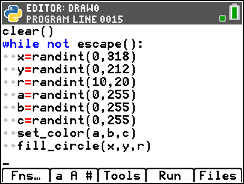
Step 9
<Run> the program. Press [clear] to stop the program and add one more feature to the project…
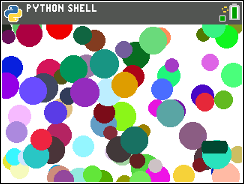
Step 10
Add a black border to each circle to help them stand out.
Use set_color(0, 0, 0) and draw_circle(x, y, r) at the bottom of the loop (but still inside the loop).
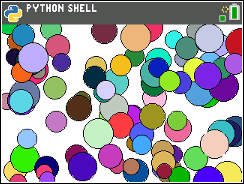
Step 11
set_pen(“size”, “style”) has two arguments that you choose from special menus that determine the appearance of the drawing pen. This function is placed before the draw_circle( , , ) statement like the set_color( , , ) function and sets the pen state until another set_pen( , ) ciommand is issued.
In this image we have chosen “medium” for the size and “solid” for the pen style. We also changed the random radii of the circles.
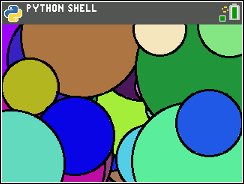
Step 12
Here’s most of the final code.
Add show_draw() at the end of the program to keep the graphics on the screen until you press the [clear] key again.
The numbers used in the program are arbitrary. Feel free to change them to see the effects. Try some other Shapes found on the ti_draw… menu.
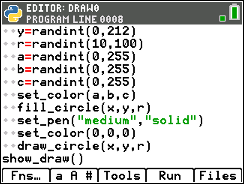
Mini project 2: Animation
Download documentsAfter completing the ‘Getting Started…’ activity let’s make an object move on the screen. Animation is big business and computers have changed the landscape.
This project produces a ‘bouncing ball’ animation on the graphics ‘canvas’.
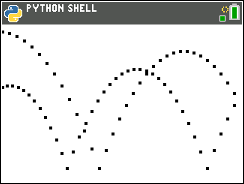
Step 1
Begin with this short program that causes a point to move across the screen.
The point starts at the middle of the left edge of the screen: (0, 106).
plot_xy( , , ) is found in the ti_draw… module and simply plots the point (x, y).
In plot_xy(x, y, 1) the 1 represents the point style which can be a value from 1 to 13. Try the other styles.
The if statement at the end causes the point to ‘wrap around’ on the screen. Your first modification will cause the point to move back and forth between the sides of the screen.
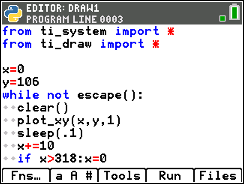
Step 2
Introduce another variable, dx = 10, and use it to make the point move to the right in place of the constant 10 used in the previous step.
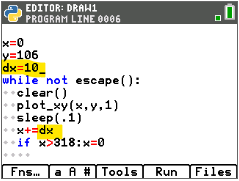
Step 3
Change the if statement to reverse direction by changing the value of dx.
if x > 318 : dx = -dx
Add another bit of code to cause the point to change direction at the left side of the screen, too. Try it yourself first.
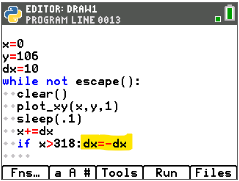
Step 4
Did you add an or… clause to the condition?
if x > 318 or x<0: dx = -dx
Test the program now to see that the point moves right and left on the screen until you press [clear].
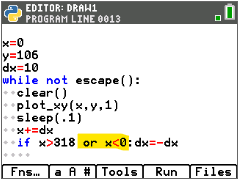
Step 5
Modify the program to introduce vertical motion as well, but this time instead of moving at a fixed rate, make the point accelerate like a falling object: seemingly under the effect of gravity!
Introduce two new variables: dy = 0 for the change in the y-position
and g = 2 to represent ‘gravity’.
The value of g is arbitrary and can be edited later to see the effect.
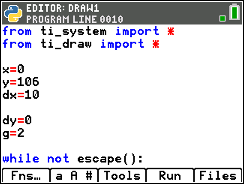
Step 6
When x changes value by adding dx, so do y and dy:
dy increases by the amount g andy increases by the amount dy.
Note: (x, y) is position. dx and dy represent velocity (changes in position). g represents acceleration (change in velocity).
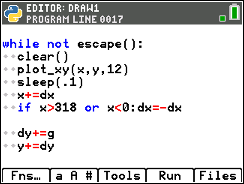
Step 7
When the point reaches the bottom of the screen (200 is close to the bottom), make the point ‘bounce’ upward using dy = -dy + g (change direction). The extra +g here is to decrease the energy in the bouncing ball. Without it the ball will return to its original height and that is unrealistic.
We also make the point slow down (lose energy) horizontally:
dx *= 0.9.
Eventually the point will stop moving.
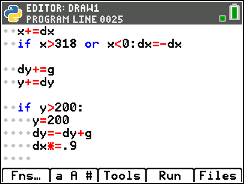
Step 8a
Change the starting position of the point from (0, 106) to (0,10) to give the point more vertical room to fall.
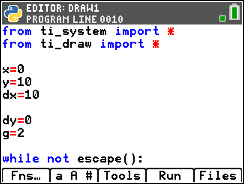
Step 8b
<Run> the program and experiment with the numbers used in the code to see the effect of each variable.
Change the plot_xy(x, y, style) and color (use set_color( , , ) ) of the point.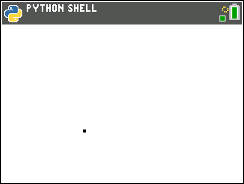
Step 9
Move the clear( ) function before the while not escape() statement so that each position of the point remains on the screen, thus showing the complete path of the point. This is point style 3. What patterns do you see in this path?
Challenge: connect the dots!
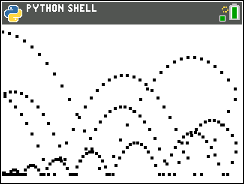
Mini project 3: Interactive art
Download documentsThe most versatile tool in the TI-84 Plus CE Python library is the wait_key( ) function. In this activity you will make use of this function to make an interactive ‘Etch-A-Sketch’® style drawing program.
Using the wait_key( ) function found in the ti_system module it is possible to make dynamic, interactive graphical applications (and games!). In this activity you will build a simple drawing program that begins by using the four arrow keys and can be extended to make use of other keys for changing color, point style, making ‘stamps’ and other features.
“Etch-A-Sketch” is a registered trademark of SPIN MASTER LTD.
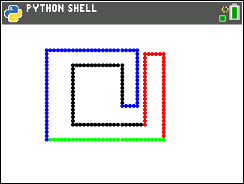
Step 1
Begin a new program and add
from ti_draw import *
found in <Fns…> Modul <Add-Ons>
Also import the ti_system module to use the wait_key() function.
The first statement sets up a comfortable canvas coordinate system with (0,0) in the lower left corner and each pixel representing one unit:
set_window(0, 317, 0, 211)
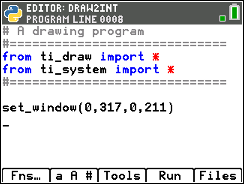
Step 2
Start in the center of the screen. Assign 159 to the variable x and 105 to the variable y. This can be done in a single line:
x, y = 159, 105
We usually use the statement while not escape(): to end a program, but in this project we need to test the value of wait_key() to see which key is pressed then act accordingly, so we will assign wait_key() to a variable named k. First assign 0 to the variable k:
k = 0
Before the while loop, use the clear() function found on [math] ti_draw… Control to clear the screen:
clear()
Then write the while loop that terminates when k is 9.
while k != 9:
9 is the value that wait_key() returns when pressing the [clear] key.
Step 3
In the loop body, plot the point (x, y) using the ti_draw function
plot_xy(x, y, 1)
Note: he third argument is the point ‘style’ which can be a value from 1 to 13. You can try other styles.
Now assign the variable k the result of the wait_key( ) function:
k = wait_key( )
wait_key() returns a unique number for each key on the keypad.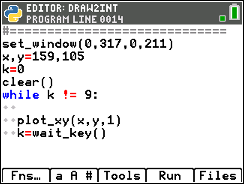
Step 4
You can <Run> the program now to see a dot in the center of the screen, but it does not move yet.
Press [clear] to end the program then return to the <Editor>.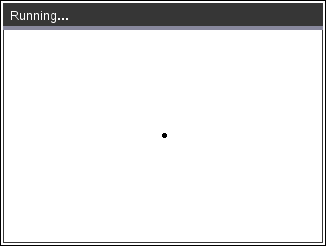
Step 5
Check the value of k and cause the point to be repositioned at a new location depending on the key pressed. The four arrow keys on the keypad are numbered 1: “right”, 2: “left”, 3: “up”, and 4: “down” and each one will impact either the variable x or the variable y.
The first of four if statements is:
if k == 1:
x += 5
If the ‘right arrow’ key is pressed add 5 to the value of x.
You could also write x = x + 5 (or some other number).
Write the other three if statements now.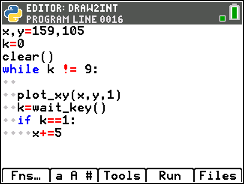
Step 6
Did you write these three?
if k == 2:
x -= 5
if k == 3:
y += 5
if k == 4:
y -= 5
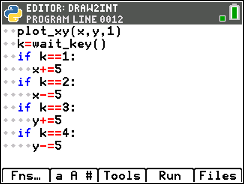
Step 7
Test your program now. When you see the dot, use the four arrow keys to draw.
Again, press [clear] to end the program.
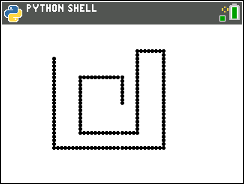
Step 8
Side note: Almost all keys on the keypad return a value like the arrow keys. All values are numbers. To determine these numbers, you could write this short program:
[2nd] and [alpha] do not give values because they are modifier keys that change the behaviors of some of the other keys. Make notes about which keys produce which values.
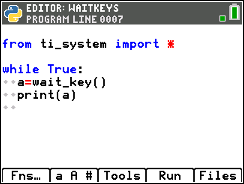
Step 9
You can use other keys to add features to your program. Many other features are possible. Just add an if statement to your program to incorporate the feature. Some possibilities:
- use three other keys and the set_color( , , ) function to change the drawing color to red, green, or blue.
- Use another key to clear( ) the screen (but not the [clear] key – that’s already taken)
- Use another key to switch to erase mode (set the color to white, the background color). But you will need some other key to return to black
- Add a key to make a stamp (“s”?). The stamp can be something like a polygon or a circle.
- Change the increment (we originally used 5 in this activity) values in your code to make the point move more or less for each keypress as seen here where 1 was used to make it look like solid lines.
Step 10
Your drawing point might go off the screen (see the green section of this path). How should you handle that? There are two common options:
- Stop at the edge of the screen (like a wall)
- Wrap around to the opposite side of the screen (called ‘toral’ mode).
(an increment of 10 and point style 12 was used for this image)
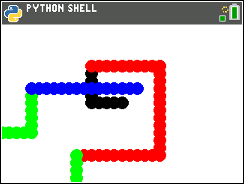
- MP 1
- MP 2
- MP 3
TI Image
Mini project 1: Getting started – Image Processing
Download documents"Image Processing" refers to the science of modifying some or all of the pixels in a digital image. An image is a rectangular arrangement of pixels in rows and columns. Each pixel is a color ‘tuple’ consisting of certain amounts of red, green, and blue, each value in the range 0..255. This first activity introduces the functions in the ti_image module and converts a color image to varying shades of gray.
Overview of Image Processing
Changing a color image to shades of gray, flipping or rotating an image, resizing and making other ‘adjustments’ to the image are all possible through Python programming with the right tools contained in ti_image.
The<Add-On> ti_image module for the TI-84 Plus CE Python allows you to address the pixels in an image. Use TI Connect CE or TI-SmartView Explorer (teacher software only) workspace to import an image from your computer to the handheld or emulator.
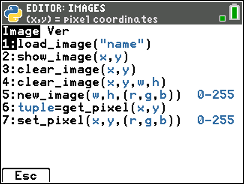
Step 1
We’ve chosen this picture of a snake (a python to be exact!) for our image processing projects. The image is 100x100 pixels. You can download this picture of a python from SNAKE.8xv. This special AppVar contains an image and can be transferred to your TI-84 Plus CE Python calculator using your TI-Connect CE software (this free software is available here).
Step 2
A closer look at the image shows the individual pixels (colored squares) that make up the image.
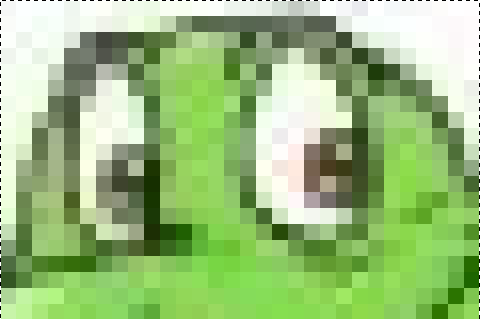
Step 3
When imported into the calculator, the image file appears as an AppVar in the Memory Management system:
[mem] > Memory Management > Appvars
The image file is called ‘SNAKE’ (note: all uppercase) in the AppVar list shown here. Notice that there is not a PY designation. It is NOT a Python program. It is a picture of a python.
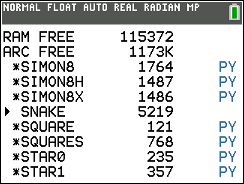
Step 4
Start the Python app, create a new program (ours is called GRAYSCAL) and select <Fns> Modul <Add Ons>. If the ti_image module is present then the import statement appears on this menu (along with all other Add-on modules that you have).
Select
from ti_image import *
If you are not seeing TI Image, make sure you have updated to latest OS. For more details go here.
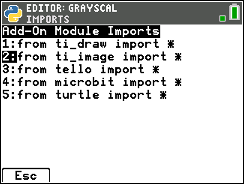
Step 5
When the import statement is in your Editor, the Modul ([math]) menu now contains an additional sub-menu: ti_image…
If there are additional <Add-On> import statements in your code they will appear here as well and the order that they appear on this menu can vary.
Remember to use the [math] key as a shortcut for <Fns> Modul but note that the <Add On> menu does not appear.
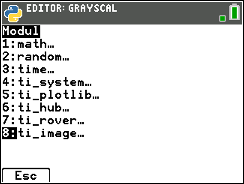
Step 6
All the Image Processing statements are found on one menu shown here. To load the SNAKE image into your program select
load_image(“name”) and type the filename SNAKE into the argument:
load_image(“SNAKE”)
Be sure to use UPPERCASE characters.
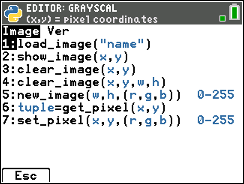
Step 7
Add the function show_image( , ) from the same [math] ti_image… menu. Use the position (50, 50) on the screen for the upper-left corner of the image.
These numbers (50, 50) as well as the image size (100x100) are important to the rest of the program.
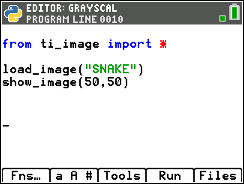
Step 8
Run the program now to see that the image appears on the screen. Notice that the screen is not cleared before the image appears. We will fix this in the next step.
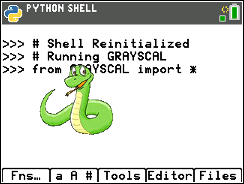
Step 9
Near the top of your program, before the .show_image() function, add the clear_image() function found on [math] ti_image…
The Image menu is shown here. Note the numbers to the right of clear_image: (319, 209). The screen is 320x240 pixels but this includes the top gray status bar that is always visible, so there are only 210 (0..209) rows of pixels available to the programmer.
The other clear_image() functions let you clear only a rectangular region of the screen or clear a region in a color of your choice.
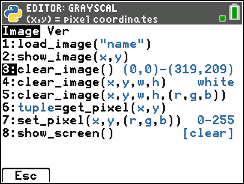
Step 10a
Move the image to the upper left corner of the screen by changing:
show_image(0, 0)
Also create four variables that store the Width and Height of the screen and the width and height of the image. Remember that Python is case-sensitive.
These variables can come in handy for positioning the image on the screen.
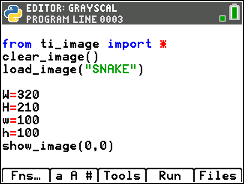
Step 10b
show_image() quickly ‘paints’ the image onto the drawing ‘canvas’, in this instance starting at (0,0), the upper left corner of the screen.
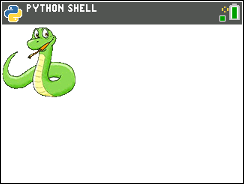
Step 11a
Now for the ‘processing’ part: To convert this image to grayscale we will:
- get the three color values of every pixel of the image
- compute the average color of each pixel
- put the new (gray) pixel somewhere else on the screen.
Use a nested pair of for loops (one inside the other):
the outer ( j ) loop processes one row of the image at a time
the inner ( i ) loop handles one pixel at a time in the current row
Note the twp range( ) arguments h and w which were assigned the width and height of the image earlier.
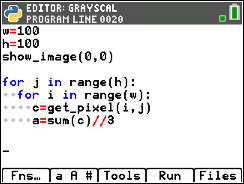
Step 11b
The statement
c = get_pixel( i, j )
found on [math] ti_image… assigns the variable c all three color values of the screen pixel at column i, row j. If printed, c would look like this: c = (r, g, b) where r, g and b are numbers. c is called a ‘tuple’ in Python.
The statement
a = sum(c) // 3
calculates the average of the three values in c as an integer (//) the function sum( ) is found on <Fns…> List
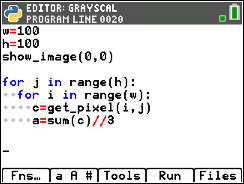
Step 12
The last two statements needed in the program are:
set_pixel(190 + i, j, (a, a, a) )
show_screen()
set_pixel( ) is part of the inner for loop so is properly indented.
The coordinates used, (190 + i, j), allow both the original image and the grayscale image to appear on the screen at the same time.
(a, a, a) is the tuple used to paint the pixel in some color. Since all three values are the same, the color is a shade of gray.
show_screen( ) is used at the very end of the program to keep the canvas on the screen until you press the [clear] key.
Both functions are found on [math] ti_image…
Step 13
<Run> the program. Your new gray snake will gradually appear on the right side of the screen. In a few minutes your complete image will be drawn, pixel-by-pixel. The white region surrounding the python remains white because the average of (255, 255, 255) is 255. Only the snake is converted to various shades of gray.
Press [clear] to exit the graphics screen and return to the Shell prompt.
Try editing the program to move the two images to different positions on the screen. Can they be placed in the same position? How would you ‘center’ an image on the screen knowing the four dimension values, W, H, w, and h?
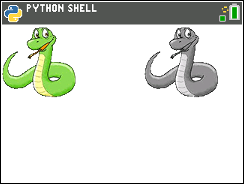
Mini project 2: Transformations
Download documentsThis activity builds on the grayscale activity from Getting Started with Image Processing. You will perform image transformations like flipping, mirroring and rotating an image.
First make a copy of your grayscale activity that you made in the “Getting Started…” activity. To copy a program, select <Files>, point to the desired program, select <Manage> Replicate program and give the new program a name. We named it IMGTRANS.
If you did not complete that activity, this screen shows most of the code that was created. Enter this code into a python program.
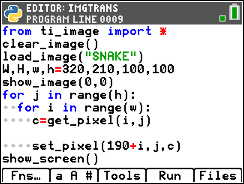
Step 1
This image of a snake (python) is used in this activity again. Recall that the image is 100x100 pixels. You can download this picture of a python from SNAKE.8xv. This special AppVar contains an image and can be transferred to your TI-84 Plus CE Python calculator using your TI-Connect CE software (this free software is available here).
Step 2
Here’s the code again. We will keep almost all the GRAYSCAL code but notice that the average a= … statement has been removed and set_pixel( ) uses the tuple variable c for the color.
Our goal in this part of the activity is to move the pixels, not change their color. We will flip the image vertically, so that the snake is upside down and the tongue is still pointing to the left.
Just modify the set_pixel( ) statement… Try it yourself!
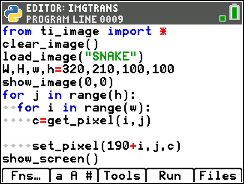
Step 3
The pixels in the top row move to the bottom row. As the row numbers increase in the original image, they will decrease in the flipped image:
Row j ⇒ Row (h-1) – j
Why (h – 1)? Remember that since the top row is row # 0 then the bottom row is row # 99.
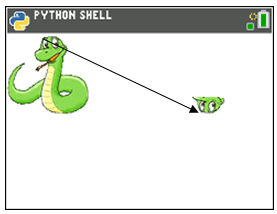
Step 4
Change the set_pixel( ) statement:
set_pixel(190 + i, (h - 1) – j, c)
190 + i makes the image appear on the right side of the screen.
c is the color tuple from the original image.
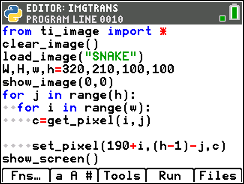
Step 5
<Run> the program. Soon your flipped image is complete!
Other transformations include mirroring (flipping left to right) and rotating (90, 180, and 270 degrees).
The next part of this activity demonstrates mirroring…
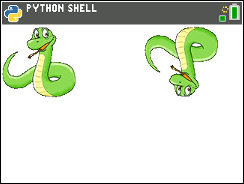
Step 6
Mirroring the image is similar to flipping. We just need to determine the proper position of each new pixel, so the only change to the code will be in the set_pixel( ) coordinate expressions.
Try it yourself now and remember to keep the new image on the top right side of the screen.
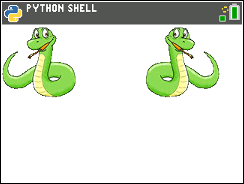
Step 7
Leave 190+ in the x-coordinate but change the i.
Row j in the original goes to row j in the copy.
In each row, pixel i from the left moves to pixel i from the right
and the right edge is (w - 1), since the left edge is 0.
Try again!
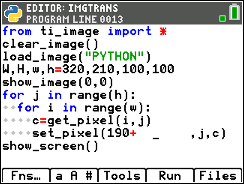
Step 8
The statement is:
set_pixel(190+ (w – 1 ) - i ), j, c)
190+ is used to place the image on the right side of the screen
(w – 1) is the right edge of the new image
i is i pixels from the left edge of the original image
j is the row number from the original image
c is the color tuple from the original image
so, 190+ (w – 1) – i is the ith pixel from the right edge of the new image!
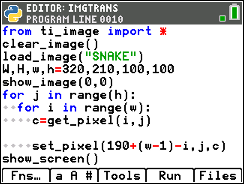
Step 9
<Run> the program to see the mirrored image eventually appear!
Please be patient.
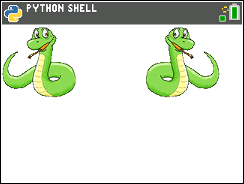
Step 10
Challenge 1:
You can get an idea that your algorithm is working properly more quickly by plotting fewer pixels. Modify your program to plot every second, third or fourth pixel in the image instead of all of them.
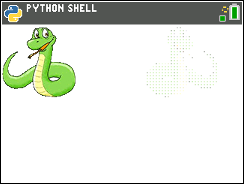
Step 11
Challenge 2: Rotate
Use the same image processing technique to rotate the image.Hint: row i from the top of the original image moves to column i (from the left) in the new image working from bottom to top. rowcolrowcol
Pixel( i, j ) ⇒ ( j, (h – 1) - i ).
This will rotate the image 90 degrees counter-clockwise. You can also rotate 180 degrees (that’s not the same as flipping!) or 90 degrees clockwise.
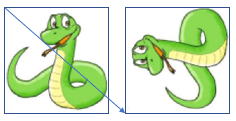
Mini project 3: Extracting colors
Download documentsAs you well know by now, a digital image is made up of a mixture of three ‘primary’ colors: red, green, and blue. In this activity we will ‘extract’ the colors in an image and then see the various ways in which they can then be combined to alter the image.
A color pixel is a very tiny light LED: Light Emitting Diode) with three separate parts. When a lot of these LEDs are arranged very close together with varying amounts of each color showing we see a ‘full color’ image. This program will do the opposite: take a ‘full color’ image and separate the three primary colors into three separate images.
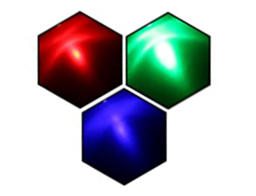
Step 1
Make another copy of the original GRAYSCAL program or the IMGTRANS program. This one is called IMGCOLRS and uses the same SNAKE image as the other two programs. Inside the for loops, delete all but the c = get_pixel( ) function.
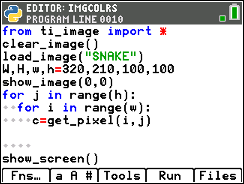
Step 2
Replace the variable c in the get_pixel( ) statement with three variables:
r, g, b = get_pixel(x, y)
This statement gives each variable one of the three values of the tuple returned by get_pixel( ).
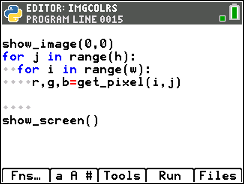
Step 3
Now build three new images on the screen. One will be red only, one blue only, and one green only. A sample statement is:
set_pixel(x, y, (r, 0, 0) )
which makes a pixel using the only the red value from the original color image. You have to decide where on the screen the three new images should appear: Replace x and y in the function above with different expressions for each color.
Try it now.
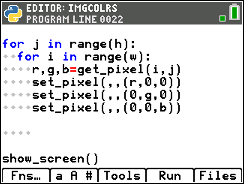
Step 4
We have placed the three separated color images here. See the next step for the code used for this arrangement and the final images.
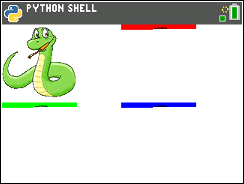
Step 5
The code:
set_pixel(159 + i, j, (r, 0, 0) )
set_pixel(i, 104 + j, (0, g, 0) )
set_pixel(159 + i, 104 + j, (0, 0, b) )
Notice that the white area of the image is separated into red, green, and blue too! White is the combination (sum) of all three colors of light (255, 255, 255). Black is the absence of light (0, 0, 0).
The snake gets darker in the red and blue images because the green has been removed.
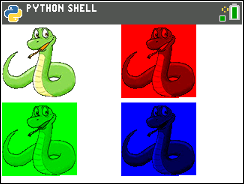
Step 6
You can also mix the colors together to make other colors:
set_pixel(x, y, (r, g, 0) )
mixes red and green together to get…. yellow!
Try mixing other colors.
You can also mix portions of the colors:
set_pixel(x, y, (.5 * r, 2 * g, .8 * b) )
just be careful that no color value exceeds 255.
You can use min(2*g, 255) to limit a value that gets larger.
How would you adjust the brightness of the image?
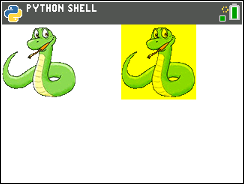
- MP 1
- MP 2
- MP 3
TI PlotLib
Mini project 1: Getting started – Coin Toss
Download documentsThis activity introduces the ti_plotLib module that is used for plotting points and graphing data sets (scatter plots).
Included in the TI-84Plus CE Python system, the ti_plotLib module contains statements and functions used for plotting data sets (pairs of lists), individual points, lines, and drawing text on a graph screen. This activity introduces the module through a coin-tossing (percent heads) simulation.
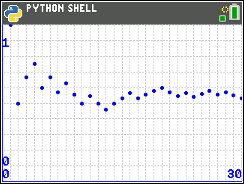
Step 1
The ti_plotlib Setup menu contains functions that prepare the graph screen:
cls( ) – clear the screen<br>
grid( ) – set the grid scale values – style chosen from a sub-menu
window( ), auto-window() – set the viewing window
axes( ) – style of axes (or off) mode chosen from a sub-menu
labels( ) – label the axes
title( ) – place a title at the top of the graph
show_plot( ) – pause while showing the plot. Press [clear] to exit
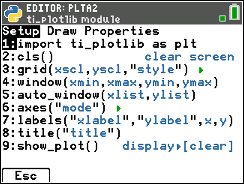
Step 2
The ti_plotLib Draw menu (many have sub-menus):
color( ) – set the plot color
scatter( ) – a scatterplot of the lists with connecting segments
plot( ) – a scatterplot of either two lists (dots only) or plot a point
line( ) – draw a line between two points
lin_reg( ) – show a least squares regression line for the two lists
pen( ) – set the pen size and style for lines
text_at( ) – draw “text” at row number
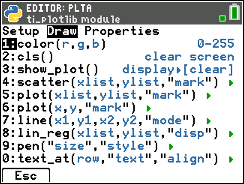
Step 3
Coin Tossing: When tossing a fair coin, approximately half of the tosses will be heads. If you toss the coin just a few times the likelihood that there will be an equal number of heads and tails is small. But, as the number of tosses grows, this ratio improves towards our expectation.
This activity creates an interactive simulation of coin tosses and displays a growing scatter plot of the percentage of the outcomes that are heads.
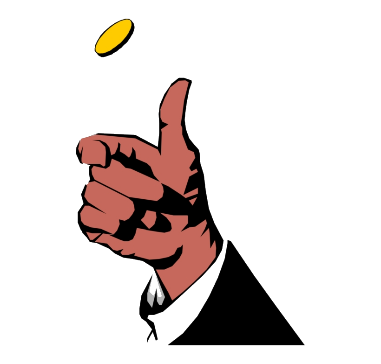
Step 4
Begin a new Python program (we called it ‘PLTA’) and select the <Type> Plotting (x,y) & Text template from the dropdown list when naming the program. This template provides the unique import statement:
import ti_plotlib as plt
along with an incomplete ‘demo’ program. The lists x and y do not contain any data. But all the plt. functons you will need are already in the code!
This type of import statement requires that all ti_plotlib functions be preceded by the alternate name plt. In Python-ese this is called ‘aliasing’ the module (give it a different, usually shorter name). When selecting ti_plotlib functions from the menus they will include this name at the beginning of the function as seen in the statements shown here.
Note: the Setup statements, if used, should be listed in this order since each one paints the canvas (screen) over the previous one: clear the screen, set the window, draw the grid, then draw the axes.
Step 5
The main program consists of a loop that ends when [clear] is pressed. Usually this is done with the statement: while not escape( ):
but this program needs to also pause until a key is pressed to toss more coins when you are ready. Near the top of the program, below the two list assignments, assign the variable k the result of wait_key( ):
k = wait_key( )
and write the while loop that ends when k equals 9 by writing:
while k != 9:
because the wait_key( ) function returns the value 9 when the [clear] key is pressed.
Indent all the plt. statements except the last one to become part of the loop body.
Add from random import * at the top of your program since we’ll be ‘tossing a coin’ using the randint( ) function. (not shown)
Step 6
The plot will show the toss number along the x-axis and the percentage of heads tossed (as a decimal) on the y-axis. The percentage will be a number between 0 and 1.
Initialize two counting variables:
t = h = 0
before the while loop.
t counts the number of tosses
h counts the number of heads
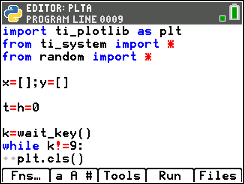
Step 7
In the while loop body, turn your attention to coin tossing and recording data. At each keypress, toss the coin 10 times using a for loop:
for i in range(10):t += 1# count the toss
x += [t]# append the count to the list x
h += randint(0,1)# toss coin and add 1 if head
y += [h / t]# append the ratio to the list y
Note: x += [t] (shorthand for x = x + [t]) is the same as x.append(t).
Find the square brackets on [2nd] [stat] or on <a A #>
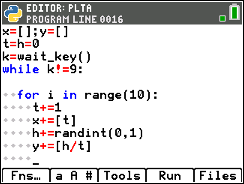
Step 8
It’s time to address the plot functions:
#comment the functions .auto_window(), .labels(), and .grid().
press [2nd] [3] for the #comment symbol (#)
Add a custom window setting:
plt.window(0, t, 0, 1)
that changes the window to suit the number of tosses t
Find plt.window(, , , ) on [math] ti_plotlib… Setup
Note that all the plt. functions are still part of the while loop (indented_ except for the last one, plt.show_plot( ), which simply pauses the program until the [clear] key is pressed.
The last statement in the while loop is the wait_key() function to pause and wait for another keypress:
k = wait_key( )
Step 9
<Run> the program and press a key. You will see the first 10 dots on the graph. Each represents the percentage of the number of heads after each toss. The x-axis ranges from 0 to 10 and the y-axis ranges from 0 to 1.
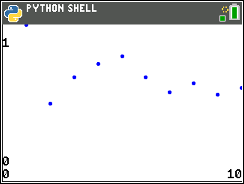
Step 10
Press a key several more times. The while loop adds 10 tosses for each keypress and updates the plot to show more tosses. The number in the lower right corner (150 in this image) is the total number of tosses thus far.
Notice the pattern in the plot: The dots added on the right get closer to the vertical center of the screen which represents the value y=0.5. This is what you expect from tossing a coin a large number of times: the number of heads is approximately 50% (0.5 or ½) of the tosses.
To end the program, press the [clear] key twice: once to end the while loop and once to end the plt.show_plot( ) function at the bottom of the program.
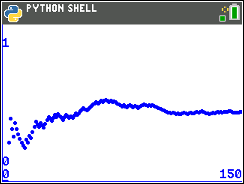
Step 11
If you continue to press a key to toss more coins, eventually you will encounter the MemoryError indicated here. The Python App runs out of memory because the lists get too large.
Challenge:
You can overcome the memory limit either by:
- Plot fewer data points (say, every 10 tosses instead of every one). You will still run out of memory but at a larger (10x)total.
- Only plot the last 100 data points. You won’t run out of memory. But you will have to adjust the viewing window so that the plot still fills the screen.
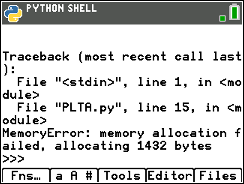
Mini project 2: Sequences
Download documentsThis activity demonstrates plotting mathematical sequences using the ti_plotLib module in two different ways: by plotting individual points and by plotting lists.
Part 1:
Begin a Python program using the ‘Plotting (x,y) & Text’ template from the ‘Type:’ dropdown list. Our program is called PLTB.
Let’s start by graphing both an arithmetic (linear) and a geometric (exponential) sequence in one graph.
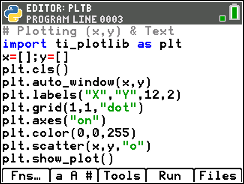
Step 1
As explained in the Getting Started activity and worth repeating here, this template provides the unique import statement:
import ti_plotlib as plt
along with a nearly complete set of programming functions.
This type of import statement requires that all ti_plotlib functions be preceded by the alternate module name plt. This is called ‘aliasing’ the module (give it a different, shorter name). When selecting ti_plotlib function from the menus, they will include this name at the beginning of the function as seen here in the first three functions provided.
Note: the statements are placed in a particular order. First set the window, label the axes, draw the grid, draw the axes. The plot the data.
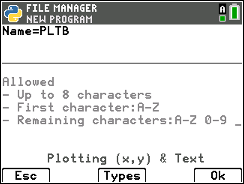
Step 2
We will not use the auto_window() function. Instead, use four ‘window variables’ to set the plt.window() because you might need these values elsewhere in your code.
x0, x1, y0, y1 = -5, 30, -5, 100
We’re placing the origin in the lower left corner of the screen because we will be plotting points in the first quadrant.
Having the four window variables assigned on a single line makes them easier to locate & edit and saves vertical space in your program.
The plt.grid((1, 1, “dotted”) values can be edited as needed. Change the y-scale value from 1 to to 10. There are limitations on the window and these grid values may interfere with the run of your program.
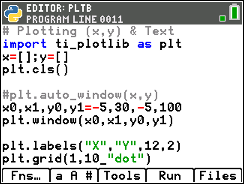
Step 3
Plot both an arithmetic and a geometric sequence in the same graph to compare them. Use a for loop that makes use of the window variable:
for n in range(x1):
Note: change the loop variable from i to n.
In the loop body, first assign two variables the value of an arithmetic/linear term like 3n and a geometric/exponential term like 2n
a = 3*n# arithmetic
b = 2**n# geometric
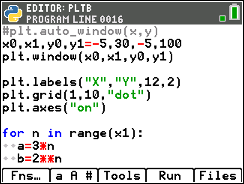
Step 4
Plot each term using a different plot style (“mark”):
plt.plot(n, a, "+")
plt.plot(n, b, "o")
Get plt.plot(x, y, “mark”) from [math] ti_plotLib… > Draw
Note: There are two plt.plot( ) functions on the menu. One is for plotting lists and the other is for plotting points.
The plot “mark” is chosen from a sub-menu and can be changed (by hand) and is limited to “.”, “+”, “x”, or “o”.
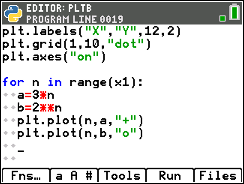
Step 5
<Run> the program and you should see the two plots. One is the graph of points on a line and the other quickly disappears off the top of the screen. Which is which?
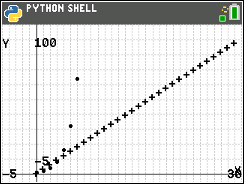
Step 6
Try changing the window settings to see different views of these plots. Depending on your window values you may encounter the error seen here: We changed xmax (x1) to 100 and got this ‘invalid grid scale’ error because the grid lines would be too close together.
Either:
- change the plt.grid( ) x-scale value to a larger number (like 10) or
- #comment the plt.grid( ) function to hide the grid.
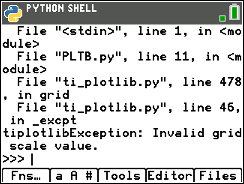
Step 7
After fixing the grid issue, running the program now should produce a graph like the one shown here. Notice how the geometric sequence grows so quickly compared to the arithmetic sequence. How high is each sequence at the right edge of the screen?
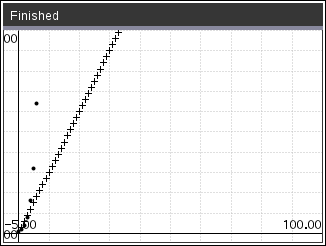
Step 8
In the next section you will use most of this program to plot a different sequence, make a copy of it: Select <Files>, point to this file and select <Manage> Replicate Program… and provide a name for the new program. We use PLTB2
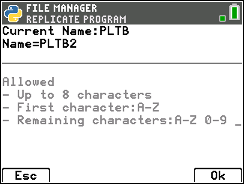
Step 9
Part 2: The Collatz Conjecture
The final sequence in this activity is based on the Collatz Conjecture which is also discussed in the TI Codes Python materials.
The Collatz Conjecture goes like this:
- Start with any counting number (we will use input( ) ).
- If it is even, then divide it by 2
- Otherwise, multiply it by 3 and add 1
Repeat steps 2 and 3 with the new number.
The Conjecture (guess) is that all numbers eventually will become 1 but this has not been proven… yet.
Starting with the number 37, we get the sequence shown to the right in the Python Shell. Let’s plot some Collatz sequences like this one.Step 10
This program will differ greatly from the previous one as we will save the plotting till the end using two lists.
Press [enter] a few times after the import statement to make room for some new code to generate some data.
Write the first two statements shown:
print("Collatz Conjecture")
n = int(input("Number? ") )
Remember that int() is on the <Fns…> Type
and input() is on <Fns… > I/O
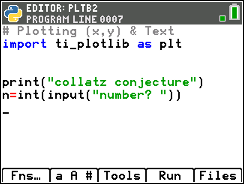
Step 11
Initialize a counter variable c to be 0.
c = 0
Create two lists:
xs is the list of counters, starting with 0.
ys is the list of terms in the Collatz sequence for n, starting with n.
xs = [c]
ys = [n]
We’re going to make a big assumption here: eventually the sequence will reach 1. After all, it has never not happened, right?
while n > 1:
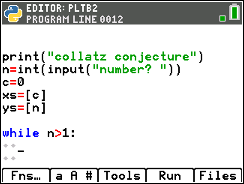
Step 12
Write the if… else structure that processes the Collatz algorithm:
while n>1:
if n % 2 == 0:# % is ‘mod’
n //= 2# integer division
else:
n = 3 * n + 1
Note: use integer division (//) to ensure all values are integers and there are no rounding issues.
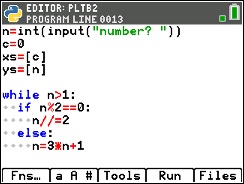
Step 13
Still in the loop: increment the counter and add (append) the counter c and the number n to their associated lists. Note the use of ‘addition’ here to append a list to another list:
c += 1xs += [c]# same as xs.append(c)
ys += [n]
Note that these statements are part of the while loop but not part of the else: block and are indented accordingly.
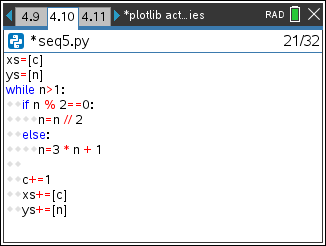
Step 14
When the while loop ends the sequence is complete so we’re ready to plot the data.
Much of the code for plotting is already in the program but needs some editing to suit our project.
We can use the plt.auto_window() function but use the arguments (xs, ys) that we created above. #comment the other window variables and function.
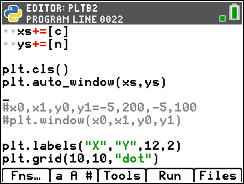
Step 15
The next three statements control the labels, grid and axes.
Plot the lists using eitherplt.plot(xs, ys, “o” ) will plot the lists with
segments connecting the dots.
or :
plt.scatter(xs, ys, “o”) to plot just the points
without the connections.
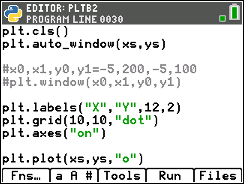
Step 16
Run the program. At the input prompt enter a positive integer. The plot displays the unique progression of the Collatz sequence of numbers for your entered number and the xmax value on the screen is a little more than the number of steps it took to reach 1. Your starting value is the point on the y-axis (it was 37 for this image).
If your graph does not remain on the screen, use plt.show_plot() after plotting.
Note: For some numbers (like 125) you might have a grid scale issue, so just comment the .grid( ) statement or find a scale that works.
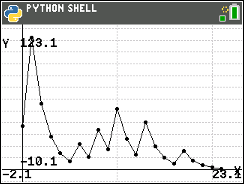
Step 17a
To display the initial value on the graph use:
plt.text_at(row, "text", "align")
found on [menu] TI PlotLib > Draw
row is a number from 1 to 13 that you enter.
“text” is your text (or a string variable) to display
“align” can be “left”, “center”, or “right” selected from a pop-up menu.
Since n is changed in the Collatz sequence generation, to display the starting number for your sequence add a statement right after the input statement that stores the value of n as a string:
t = str(n)
str() is found on [menu] Built-Ins > Type and use that t variable in the statement
plt.text_at(3, t, “center”)
Step 17b
99’ at the top center of this screen is the result of the plt.text_at() function.
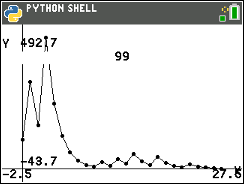
Step 18
Each starting value has a unique sequence. Some take quite a while to reach 1. Can you find a number that does not reach 1? If so, you will be famous!
Challenge: for each Natural number (up to an entered number) plot the length (number of terms) of its Collatz sequence.
For many other interesting integer sequences see https://oeis.org/, the Online Encyclopedia of Integer Sequences.
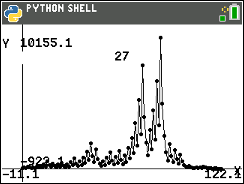
Mini project 3: Graphing Functions
Download documentsThe TI PlotLib module is also well-suited for graphing smooth, continuous functions: plotting points one-by-one requires extra work to ‘connect the dots’ but using lists works as we did in the previous activity, Sequences, can work very well with the right setup functions.
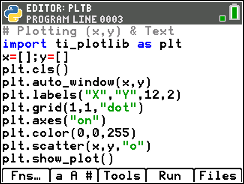
Step 1
Begin a Python program using the ‘Plotting (x,y) & Text’ template from the ‘Type:’ dropdown list. Our program is called PLTC.
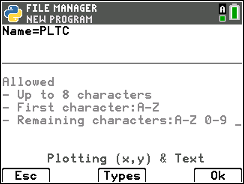
Step 2
This template provides the import statement:
import ti_plotlib as plt
along with an incomplete demo program. The lists x and y do not contain any data.
Since most of the code is already designed for plotting these lists, let’s give it some data to plot.
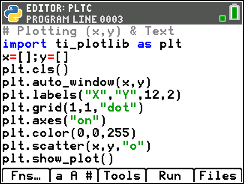
Step 3
Change the names of the two list variables to xs and ys. Make room below these assignment statements for more code.
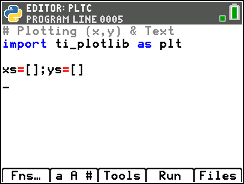
Step 4
Use four ‘window variables’ to establish a viewing window. We will use these values in plt.window( ) rather than rely on the plt.auto_window provided.
x0, x1, y0, y1 = -10, 10, -7, 7
These values are close to a standard viewing window in the Graphs app. The origin is near the center of the screen.
Having the four window variables assigned on a single line makes them easier to locate and edit and saves vertical space in your program.
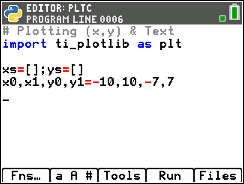
Step 5
It is useful have the Width and Height of the screen in pixels stored in two Uppercase variables:
W, H = 318, 212
(For a capital letter press [alpha] twice, then the letter)
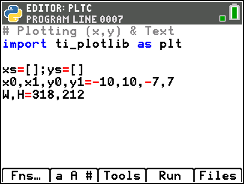
Step 6
We will plot determine a value of the function for every pixel on the screen, but we need to know the ‘distance’ between pixels based on our chosen window settings:
d = (x1 – x0) / W
d is the ‘change in x’ as we move from one pixel to the next in our chosen viewing window.
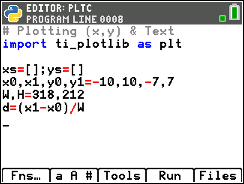
Step 7
We’re ready to generate the coordinate values using a loop. A for loop is tempting, but the Python for loop can only process integer values, not decimals.
Use a while loop instead. Start at x0 and have the loop end when x reaches x1 by adding d to x in each step of the loop:x = x0
while x <= x1:
# some code goes here
x += d
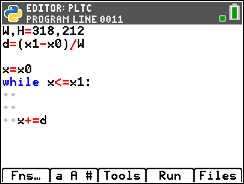
Step 8
In the loop body, evaluate your function and add the point coordinates to the lists x and y.
We’ve chosen to plot the function
y = x**2 - 5
(You can also define a function and use it here!)
xs.append(x)
ys.append(y)
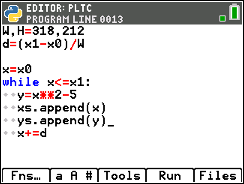
Step 9
We’re done with the while loop that creates the lists. Turn your attention to the plot functions below the loop. The most important change is the names of the two lists: xs and ys. These are used in both the auto_window(x, y) and the scatter(x, y) functions.
But… we will replace auto_window() with plt.window() and make use of our custom window settings.
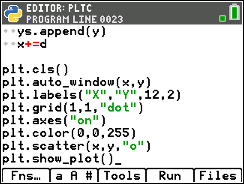
Step 10
Make the changes highlighted here:
#comment plt.auto_window() statement ([2nd] [3] produces #)
Add plt.window(, , , ) selected from [math] ti_plotlib… Setup and use your window variables x0, x1, y0, y1.
Change plt.scatter() to plt.plot(xs, ys, “.”) selected from
[math] ti_plotlib… Draw.
Note that the “mark” is the small dot.
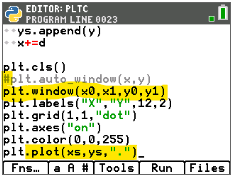
Step 11
<Run> your program after maing the changes. If your graph does not appear and you see just the Shell prompt then there is an error. But it’s probably not your fault! At the Shell prompt press [2nd] [uparrow] several times to get the error message to appear…
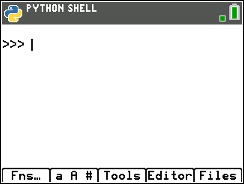
Step 12
We got the MemoryError shown here. There’s not enough memory for all the elements we are generating in the two lists (318*2).
To fix the error we need to generate fewer elements in the lists.
Return to the <Editor> …
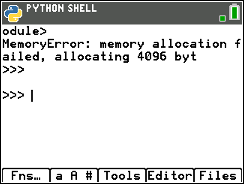
Step 13
In the statement where d is calculated, change the calculation:
d = 2 * (x1 - x0) / W
This forces the program to calculate a point at every other pixel thus creating half as many data points in the plot.
After making the change… <Run> the program again
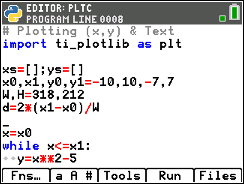
Step 14
<Run> the program again and you should see the graph shown here.
Challenge 1: plot two functions at the same time in different colors. Be careful about memory usage.
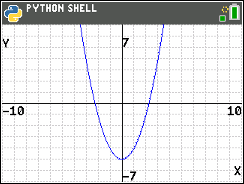
- MP 1
- MP 2
- MP 3
Micro:bit
Mini project 1: Getting started with Micro:Bit
Download documentsIn this lesson, you will write your first python programs to control the micro:bit display in different ways. This lesson has two parts:
- Part 1: Alien Encounter
- Part 2: Displaying images
Objectives:
- Control the display on the micro:bit board using .show( ), .scroll( ) and .show(image)
- Control the speed of the display using sleep(ms) and delay=
- Writing a loop structure
Step 1
Before you begin, be sure that:
- You are using a TI-84 Plus CE Python with OS 5.7
- You are comfortable programming in python and/or you have already completed Units 1 through 5.
- Your micro:bit is connected to your calculator and lit.
- You have followed the set-up directions and file transfers in the micro:bit Getting Started Guide:
https://education.ti.com/microbit
and thus have the TI runtime file on the micro:bit and the latest microbit modules in your calculator
This setup process should only have to be done once but keep informed periodically about updates/upgrades.
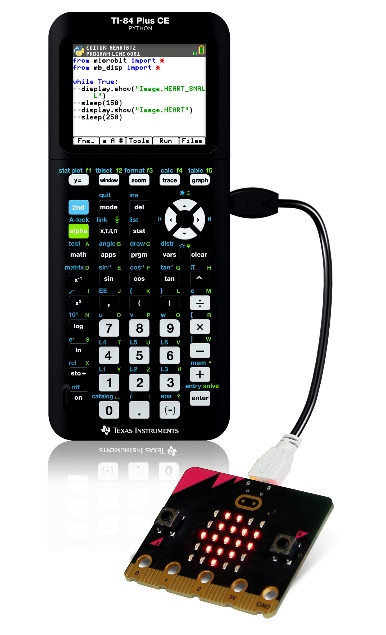
Step 2
From the home screen (Quit the Python App) you can check your calculator to be sure that the appropriate files are installed: press [2nd] [+] for [mem], select Mem Management > AppVars and scroll to the ‘M’ section to find MICROBIT and the MB_* support files shown.
Note: there are more than those displayed in this image: a total of 11 (version 1) or 13 (version 2) micro:bit AppVars.
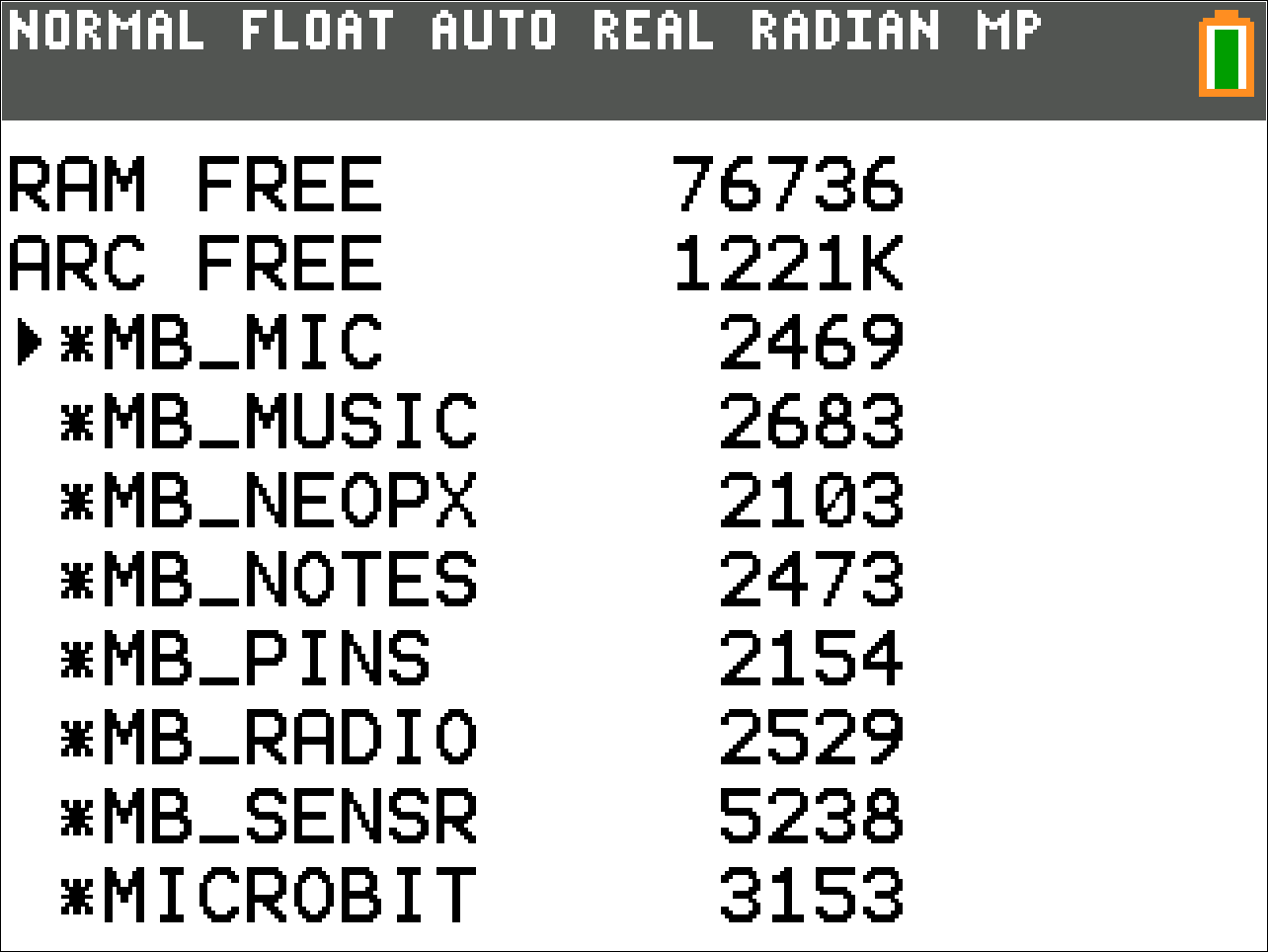
Step 3
If all is good and the setup has been done correctly, your micro:bit should look like this when it has power from the calculator:
The display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
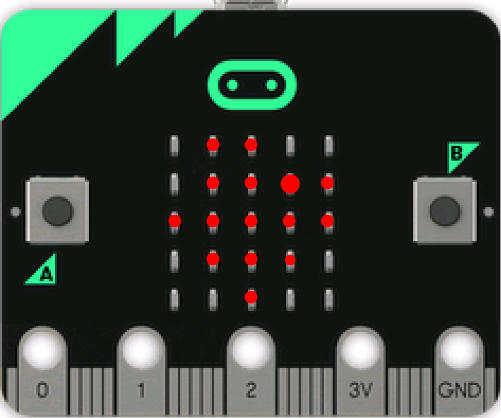
Step 4
Part 1: Alien Encounter
Of course, as with every other first programming experience, you will start with displaying a message on the micro:bit display. In the Python Editor start a <New> program (we name it GREETING).
In OS version 5.7, when selecting <Fns…> Modul, a soft key appears called <Add-On> which gives access to additional TI-developed modules. (if you don’t see this, you need to update to OS 5.7)
Tip: If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit and plug it in again (reset).
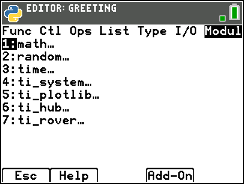
Step 5
Select <Add-On> and see some additional modules that are available, some of them shown here. Your list may differ but should include microbit. Select from microbit import * to paste that code into your program.
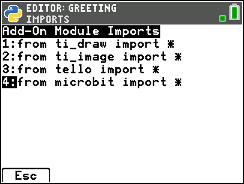
Step 6
In the Editor, the presence of this statement adds a new option to the bottom of both the <Fns…> Modul menu and the [math] menu: Micro:bit…
As you add features from Micro:bit this menu will grow!
Note: [math] is a keypad shortcut for <Fns…> Modul but does not display the <Add-On> soft key.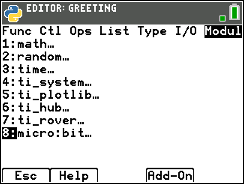
Step 7
The Micro:bit… sub-menu contains all the tools necessary for programming the micro:bit in separate sub-modules.
Each of these menu items will paste its own import statement since the tools are all in separate Python modules (AppVars). If you are using a micro:bit version 1 there are fewer items on this screen.
Select the Display menu…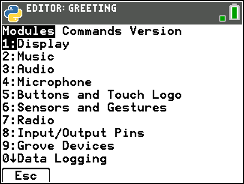
Step 8
After selecting Display, you see a new import statement in your program:
from mb_disp import *
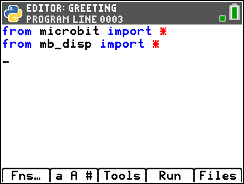
Step 9
Look at the [math] menu again: There is now a display… menu item added to the bottom of the list. Select display…
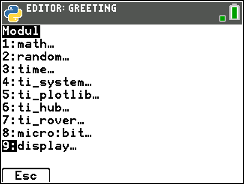
Step 10
The display… menu (shown) contains the functions that control the display on the micro:bit, the 5x5 grid of red LEDs in the center of the board and more.
Select .show(val)
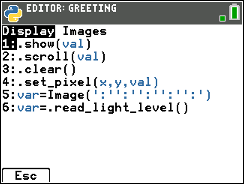
Step 11
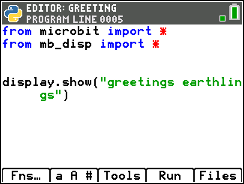
display.show(“greetings earthlings”)
Press [2nd ] [alpha] to turn alpha lock on and press [+] for a quotation mark. All letters typed will be lowercase. For uppercase, press [alpha].
Note: There are two optional arguments that you can add:
display.show( , delay = 400, wait = True)
delay=(time in milliseconds) controls the speed of the display.
wait= (True or False) tells the micro:bit/calculator to finish displaying before moving on to do something else.
Each parameter can and should be edited to see their impact and each may be specified using their keyword. This is recommended, but not required.
<Run> the program and watch the display on the micro:bit.
You will see the letters of your message appear, one letter at a time, on the display. The lowercase letters ‘e’ do appear twice but you cannot distinguish two of them.
Step 12
A better method for displaying long messages is:
display.scroll(“greetings earthlings”)
which is also found on the [math] display… menu.
Make the previous .show() statement a #comment. Place the cursor at the beginning of that line and press [2nd] [3] to insert a ‘#’ and then run the program again.
Yes, you can also simply change .show to .scroll by typing.Note: .scroll() also supports the optional delay= and wait= arguments.
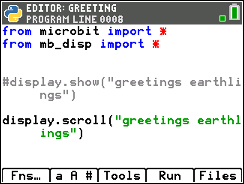
Step 13
The display.scroll( ) function causes the message to scroll smoothly from right to left like a banner. delay controls the speed of the scrolling. wait tells the calculator and micro:bit to wait until the scroll is done before moving on to another instruction. Try other delay values to see the effect on the scrolling.
display.scroll( , delay = 100, wait = True)
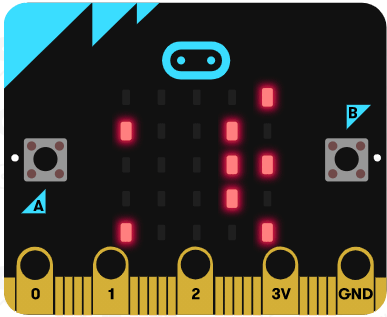
Step 14
Part 2: Displaying images- Be.Still.My.Beating.Heart
This section shows you how to display images on the micro:bit.
Select <Files> and make a new program called HEART.
In the Editor, add the two import statements as before:
from microbit import *
from mb_disp import *
First, get the microbit module from <Fns…> <Add-On>
Then press [math] Micro:bit… for display…
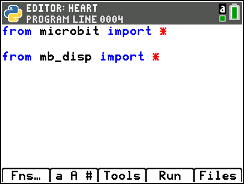
Step 15
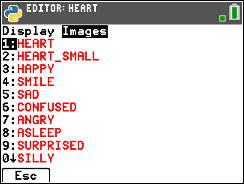
display.show( … )
Again, this statement is found on:
[math] display…
HEART
from the [math] display… Images sub-menu.
Note: there are 36 Images to choose from and you can design your own using var=Image( … ) found on the Display sub-menu. See micro:bit documentation online for information on this feature.
In case you have not noticed…to cut down on keypresses, the menu system allows for up-arrow and left-arrow ‘wraparound’, so, for example:
Select [math] then up-arrow to display…
Step 16
The string “Image.Heart” is inserted into your code as shown in this screen.
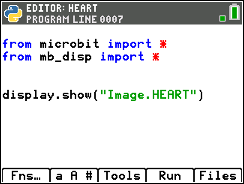
Step 17
<Run> the program. Do you see the heart ❤?
This display remains on the micro:bit until something takes its place, even after the program is done. To restore the original TI icon, reset the micro:bit. There is a ‘reset’ button on the back of the micro:bit or you can unplug and re-plug from the calculator. Or just leave it alone.
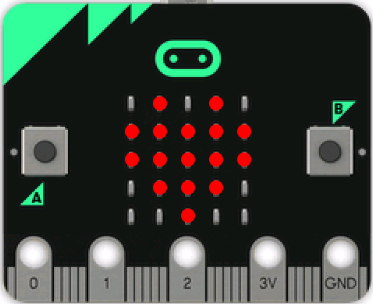
Step 18
Go back to the Editor and add another display.show( ) statement to show the small heart:
display.show(“Image.HEART_SMALL”, …)
Be sure to get the display.show( ) statement first, then paste the “Image.HEART_SMALL” string. You can find this ‘small heart’ string on the same Image sub-menu right below HEART.
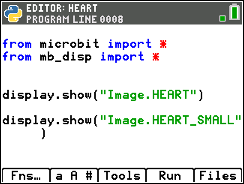
Step 19
<Run> the program again. It quickly displays the large heart and then displays the small heart that looks like this.
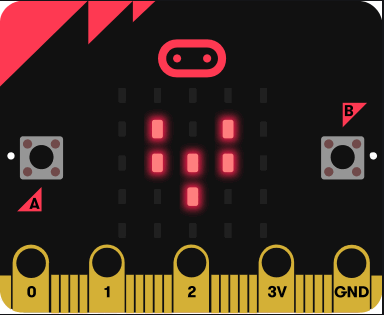
Step 20
Make a loop: To get the two hearts to blink repeatedly (‘beat’), embed the two display statements in a loop. Before the two display statements insert:
while not escape( ):
♦ ♦
found on [math] ti_system…
and indent the two display statements so that they form the loop body.
You also need to import ti_system in order to use this special while statement. Place that import statement at the top of your code.
Important Tip: new to Python? Indentation is critical in Python programs. This is how python interprets loop blocks and if blocks. If the two display statements are not indented the same number of spaces then you will see a syntax error. Use the [space] key ([alpha] [0]) or select <Tools> Indent►to indent both lines the same amount. Indentation spaces are indicated in this Editor as light gray diamond symbols (♦ ♦) to help with proper indentation.
Step 21
<Run> your program again and watch the Beating Heart! Press the [clear] key to end the program.
Tip: if you ever think your program is stuck in an infinite loop, press and hold the [on] key on your calculator to ‘break’ the program. This could happen if you use while True:. These lessons avoid that type of structure by using the while not escape(): loop.
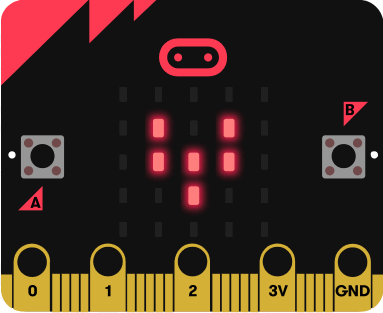
Step 22
To control the beating heartrate, add the delay= and, optionally wait=, argument and adjust the values in these two display commands as shown here. Remember that the delay value is in milliseconds.
Note: some blank lines have been removed from the program in this screen to show the entire program.
Change both wait= parameters to False to see how fast the heart blinks. False is found on <a A #>. wait = True tells the micro:bit to complete the task before going to the next task (like ‘pause’ or ‘sleep’). When False it goes immediately to the next task and ignores the delay= value.
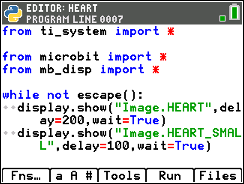
Mini project 2: Using the Buttons
Download documentsThere are three parts to this lesson:
- Part 1: Investigate the button functions
- Part 2: Use a button to generate some data
- Part 3: store data in a list using buttons and transfer the list to the TI-84 Plus CE.
Objectives:
- Read and process the A and B buttons on the micro:bit
- Observe the difference between .was and .is
- Investigate collected data from the micro:bit
- Transfer data from python to TI-84 Plus CE
Intro 2
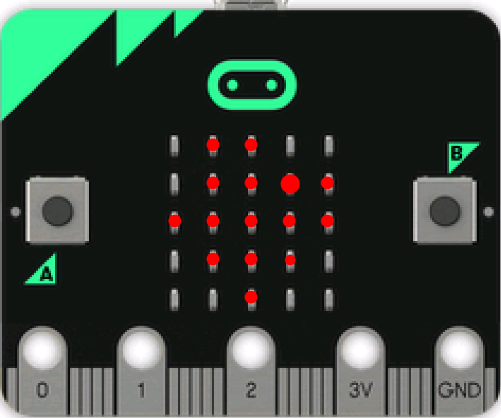
Step 1
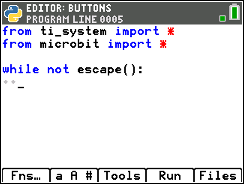
Add the ti_system module to your code using [math] ti_system:
from ti_system import * From <Fns…> Modul select <Add-On> microbit to get the statement:
from microbit import *
Then start a loop:
while not escape():
♦ ♦
The special while loop can be found under [math] ti_system… or <Fns…> Modul ti_system…
Important: The microbit import must come after the ti_system import statement!
Handy Tip: Replicate this file as MBSTART and use it as a template for all other micro:bit programs. Just replicate the file using the name of a new program!
Step 2
To use the micro:bit buttons A and B, you must first import the buttons module.
Place your cursor below all existing import statements at the top of your code (on a blank line).
Press [math] and select Micro:bit…. Choose Buttons and Touch Logo. This inserts the import statement from mb_butns import *
It also adds a new item to the <Fns…> Modul (or [math]) menu below Micro:bit….
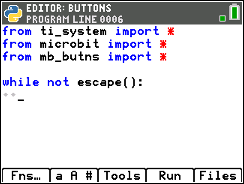
Step 3
To test button A, in the while loop body add the following if: structure:
♦ ♦if button_a.was_pressed():
♦ ♦ ♦ ♦print("button A")
if is found on <Fns…> Ctl and automatically adds leading spaces below it for further indentation of the block.
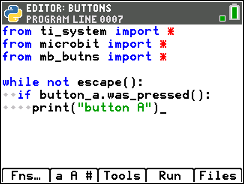
Step 3b
The condition button_a.was_pressed() is found on the menu item:
[math] buttons and touch…
Select .was_pressed() under the button A menu.
Remember to leave the colon at the end of the if statement:
print( ) is on <Fns…> I/O
Type the text “button A” inside the print( ) function.
Note: .is_pressed() will also be discussed later.
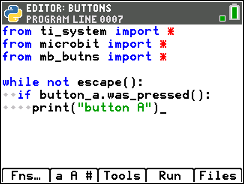
Step 4
<Run> the program. It looks like nothing is happening. Press and release button A on the micro:bit. You will see ‘Button A’ appear on the calculator screen. Each time you press and release the button the text will appear as in this image.
Reminder: if you think your program is stuck in an infinite loop press and hold the [on] key on your calculator to ‘break’ the program.
Press [clear] to end the while loop (and the program) and then return to the Python <Editor>.
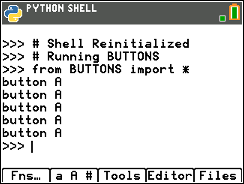
Step 5
Add another if statement to check button B using the condition button_b.is_pressed(). You will see how .is_ and .was_ differ soon.
♦ ♦if button_b.is_pressed():
♦ ♦ ♦ ♦print("Button B")
Tip: again, pay attention to the indentations!
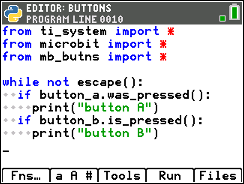
Step 6
<Run> the program again. Try both buttons A and B.
Tap each button and press-n-hold each button.
You will see ‘Button B’ repeatedly displayed as long as button B is held down, but not ‘Button A’. There is a difference between .was_pressed() (which needs a release of the button to be reset) and .is_pressed() which just checks to see if the button is down at the very moment that the statement is processed.
Note: if you tap button B quickly, the program may not display Button B since the button is not down at the very moment the if statement is being processed.
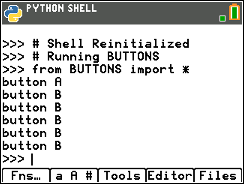
Step 7
Part 2: Use a button to generate some data
Let’s use a button to toss a die (a cube numbered 1..6 on each face). When button A is pressed, assign a random integer from 1 to 6 to a variable. You can use ‘button A was pressed’ or ‘button a is pressed’. Try both to see the difference.
Display the value of the die on the micro:bit only. Try it yourself before looking at the next step. We will use the current program and add code to simulate the die toss.
Can you determine what number is on the bottom of the pictured die?
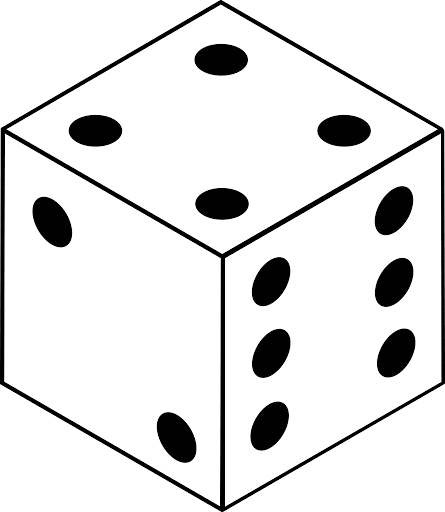
Step 8
Add the two statements highlighted as shown in the image:
from random import *and randint( , ) are both found on
[math] random…
The variable die and the arguments ♦ ♦ ♦ ♦die = randint(1, 6) are typed in manually. Notice that the statement is indented to be part of the if button_a… block.
Again, be careful about the indentation.
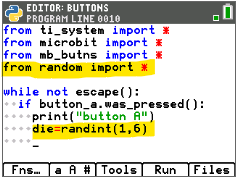
Step 9
Now note these two new highlighted statements in the image again.
After the value of the die has been established, we would like to display it on the micro:bit.
First, in order to use the micro:bit display features, you must import the display module (the top statement). Get it from [math] Micro:bit….
Then add the statement:
♦ ♦ ♦ ♦display.show(die)
below the die= statement to show the value of the die on the micro:bit.
Micro:bit display commands are found under [math] display…
<Run> the program again. When you press button A you see ‘Button A’ on the handheld screen and the die value on the micro:bit display changes… but not every time! Sometimes the random number selected is the same as the last number and… that’s OK. The presses are ‘independent events’.
Step 10
Part 3: Collecting data
Tossing all those dice with just a button press is nice, but for further study it would be helpful to store all those values so that you can interpret the data: which number occurs most often? What is the average number? and so on.
In the next step, you will add statements to the program to:
- Create an empty list
- Add (.append) the die value to the list
- Store the list from python to the TI-84 Plus CE system for analysis
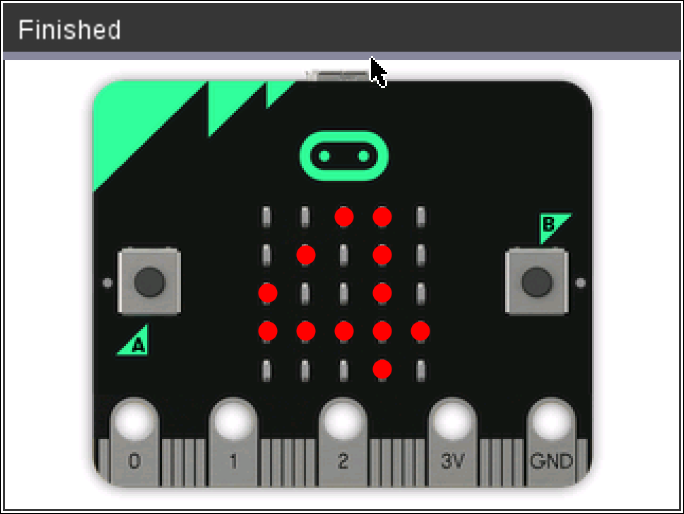
Step 11
Begin with an empty list. The empty list assignment belongs at the start of the program, before the while loop:
tosses = [ ]
The variable name, tosses, is typed in. The square brackets are found on the keypad, on <a A #>, on [list] ([2nd] [stat]) and on <Fns…> List.
After the die value is determined, it is added to the list with the statement:
tosses.append(die)
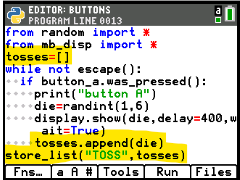
Step 11b
At the end of the program, after the while loop ends, the final list is stored to a TI-84 list:
store_list(“TOSS”, tosses)
the store_list function is on [math] ti_system…
Note that this statement is not indented at all so that it is not inside the while loop but is only executed once at the end of the program once [clear] is pressed. This function stores the Python list tosses over to the CE list TOSS. The CE list “TOSS” must be UPPERCASE and less than 6 characters.
**Again, pay attention to the indentations, especially store_list() which is not indented at all so that it is not part of the while loop.
Note: the display.show( ) statement has been modified to include the optional delay= and wait= arguments as explained in the previous lesson.
Note: You may need to add a sleep(100) statement to the while loop (just before the if statement) if button A does not respond.
Step 12
Button B is not used (yet). Can you use button B for something special here?
When you run the program now:
- if you used .was_pressed, press and release button A many times (You should see ‘button A’ on the calculator screen and numbers on the micro:bit display).
- if you use .is_pressed, hold button A down.
Press [clear] to end the while loop. Your python program had a list named ‘tosses’ and now your TI-84 CE also has a list named ‘LTOSS’. These are two separate lists in two separate environments.
Quit Python and set up a [stat plot] histogram of TOSS. You can also perform a 1-var Stats analysis. Do you notice a pattern? Try more tosses*!
*Note: due to memory constraints, the store_list( ) function is limited to lists of 100 elements maximum.
Mini project 3: The Light Sensor
Download documentsIn this lesson, you will monitor the light sensor on the micro:bit and store the data in a TI-84 Plus CE list for further analysis.
Objectives:
- Read and display the brightness sensor on the micro:bit
- Transfer data from python to TI-84 Plus CE
- Investigate collected data from the micro:bit
Step 1
The micro:bit can read the ambient light level using the display LEDs. Yes, the display LEDs can also be used as an input device!
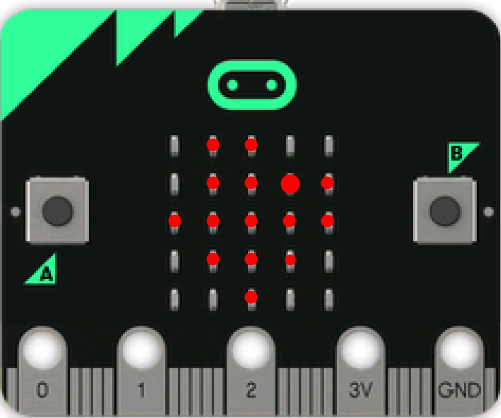
Step 2
In a new program (LITE) add the usual imports and the while loop.
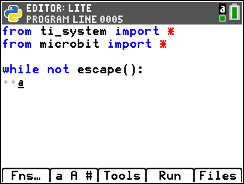
Step 3
Add an import statement at the top to access the micro:bit display menu:
from mb_disp import *
In the while loop body, write the assignment statement:
♦ ♦b = display.read_light_level()
Type b and get = .read_light_level() from [math] Display…
Add a print statement to see what the function produces:
♦ ♦print(“b = “, b)
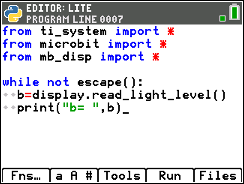
Step 4
<Run> the program and point the display side of the micro:bit at a light source. It does not matter what is showing on the display. Move the micro:bit toward and away from the light and observe the changing values on the TI-84 screen. You should see values varying between 0 and 255.
Note: if you do not see changing values as in this image, add a sleep(100) statement to the loop to slow things down.
As you probably expect, the further from the light source, the lower the light level value. Now you will add code to the program to collect the light level data. Then you can create a scatter plot of light vs. time.
Press [clear] to end the program and go back to the <Editor>.
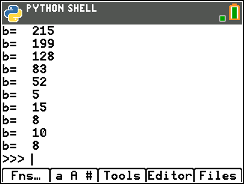
Step 5
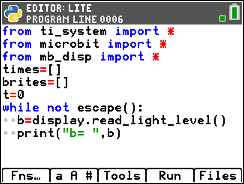
times = [ ]
brites = [ ]
Find the square brackets on the keypad, on <a A #>, on <Fns…> List or on [list]. You can use shorter variable names to save on typing (like ts and bs).
Also before the while loop, add a statement to start a ‘time’ counter variable (t) at 0:
t = 0
Avoid using the word ‘time’ as a variable because there is a time module. It is a good practice to pluralize list names because they contain many values.
Step 6
In the loop body, after the print statement, add a statement to increase the timer variable t. We will use a one second time interval between light readings, so use:
♦ ♦t = t + 1
Note: in Python you can also write this statement as: t+=1
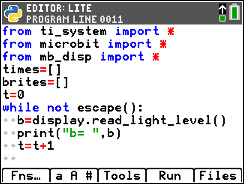
Step 7
Add the values of t and b to their respective lists using the statements:
♦ ♦times.append(t)
♦ ♦brites.append(b)
.append( ) is found on <Fns…> List and is pasted after typing the variable name.
These statements add the current b (brightness) value and t (time) value to the end of the lists.
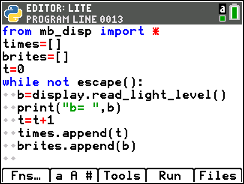
Step 8
To control the timing of the sampling, add:
♦ ♦sleep(1000)
after the two .append statements. This pauses data collection for one second between each sample.
sleep( ) is included in the microbit module and is modified to use milliseconds.
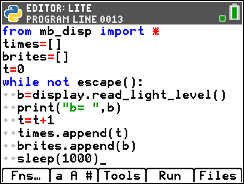
Step 9
After the while loop ends, store the two Python lists into TI-84 Plus CE lists using store_list( ) found on [math]ti_system….
The TI-84 list names (the arguments in “QUOTES”) must be 5 UPPERcase letters or less. We use BRITS and TIMES for those lists. The second argument of store_list( , ) is the Python list variable to store.
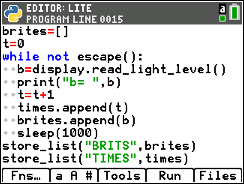
Step 10
<Run> the program. Start with the micro:bit close to your light source. An exposed light bulb or a smartphone flashlight work well. Slowly but steadily move the micro:bit away from the light at a constant rate until the brightness reading is less than 10.
Press [clear] to end the program.
Repeat the process until you feel that you may have ‘good’ data. Displaying the data on the calculator screen using print( ) or disp_at( ) may be helpful. Sample data in this image of the TI-84 Plus CE Stat Editor shows some collected data in the lists TIMES and BRITS.
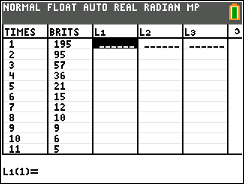
Mini project 4: Pair Of Dice
Download documentsIn this activity you will write a program to collect data using the micro:bit and run the program while observing a dot plot grow on a graphics screen using ti_plotlib.
- Write a micro:bit data collection program
- Create a dynamic plot of the collected data as the program is running
Step 1
This activity is a compilation of the micro:bit skills you learned in the previous activities:
- write a program that uses a button to collect some data,
- display the data on the micro:bit,
- show a growing dot plot of the collected data and then store the lists as TI-84 Plus CE lists for further study and analysis.
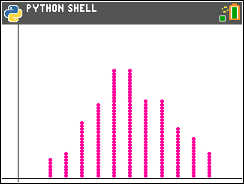
Step 2
The micro:bit can act as a 2-dice roller and display two values (sequentially). The calculator can display a growing dot plot of the sums recorded at the same time.
Write a program that conducts the dice tossing experiment and collects the data. At the end of the program store the data into CE lists for further study and analysis on the calculator. Let’s get started…
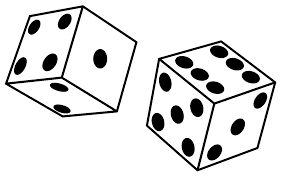
Step 3
Start a new program called DICEPAIR.
You will need a bunch of Python tools for this program so there are quite a few imports that will take place.
- ti_system for escape( )
- random for randint( , )
- ti_plotlib for the Python graphics
- microbit for the micro:bit
- mb_disp for the micro:bit display
- mb_butns for the micro:bit buttons
Tip: as you develop a program from scratch you will find that you need more import statements than you originally thought. It’s fine to go back and add them as needed.
Step 4
Use button A to toss the dice and button B to ‘reset’ the data collection and start over. It will be convenient then to have a ‘setup’ subroutine that can be used in different parts of the program to set up the plot screen in the calculator.
Start your code with a def setup( ): function.
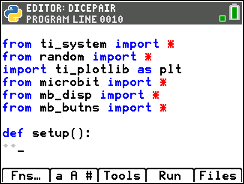
Step 5
This function performs the setup commands for plotting as indicated by the.plt prefix.
Find these commands on [math] ti_plotlib.
All these statements are indented to form the function body.
Remember that the order of these setup commands is important and they should be used in the order that they appear on the menu.
The color statement is on the Draw menu. We chose black axes and purple dots.
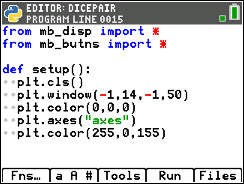
Step 6
Begin the main program using a #comment as an indicator.
Create two lists:
ttls=[0,1,2,3,4,5,6,7,8,10,11,12]
sums=[0]*13
ttls[0] and ttls[1] are not possible sums but act as ‘placeholders’ so that the indexes match the element contents. When the sum of the dice is 2 we will increment element sums[2].
Note: To produce a scatter plot we need two lists. ttls is the first list and sums is the second list.
Now ‘call’ the setup( ) function you defined above to prepare the calculator screen for plotting.
Step 7
Begin with the usual…
while not escape():
♦ ♦ if button_a.is_pressed():
Why did we choose .is_ instead of .was_ ? Think about the difference, or just wait and see…
Remember, pressing button A:
- tosses the dice
- displays them on the micro:bit
- calculates the total
- increments (adds 1 to) the proper element of sums
- produces a scatter plot.
Try writing the code for button A now.
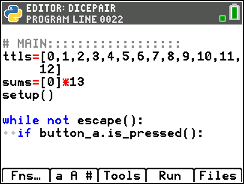
Step 8
Here is most of the code for button A. One feature is missing: displaying the two dice on the micro:bit. That is left as a project for you.
Hint: The command
display.show(<variable>, delay=400, wait=True)
displays the value of the <variable> on the micro:bit. You will need to display two values (each die).
Good luck!
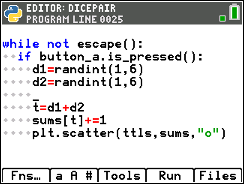
Step 9
You can test your program as written so far (even without the display. statements). It should show a scatter plot on the calculator screen as you hold down button A (fast). That’s why we chose .is_.
If we used .was_ then we would have to click and release button A to make each toss.
But wait… there’s more!
Press [clear] to end the program.
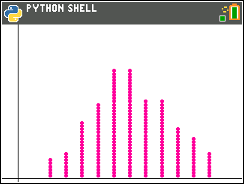
Step 10
There are two more tasks:
(in addition to making the micro:bit display the values of the dice under button A)
- Button B should act as a ‘reset’ button: clearing the screen by calling setup( ) and set the sums list to all 0’s again. That’s only two statements in the if button_b… block as shown.
Note the use of the setup() function again. - The final two lists should be exported to the CE for further study. You need two store_list( , ) statements (incomplete as shown), one for each list in the program.
Pay attention to the indentation.
Note: we used .was_ instead of .is_ for button B. Can you feel the difference?
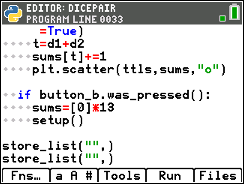
Step 11
After you have stored the two lists by pressing [clear] to end the loop and the program, quit the Python app ([2nd ] [quit]) and set up a scatter plot of TTLS, SUMS - the CE lists are all CAPS - as shown in this image.
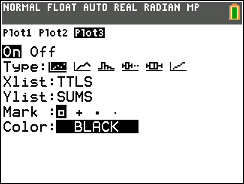
- MP 1
- MP 2
- MP 3
- MP 4
Micro:bit with Expansion Board
Mini project 1: The Light Switch
Download documentsThe micro:bit has a row of gold connections along the bottom edge for connecting electronic accessories. To make these connections easier there are many ‘expansion boards’ available that allow us to ‘plug in’ those accessories using standard cables. Two of these expansion boards are the Grove shield and the Bitmaker expansion board. The projects presented here will work with these and many other expansion boards. In addition to the expansion board, you will use some Grove accessories: an external LED (any one color), a light sensor, an ultrasonic ranger, and an external speaker.
These activities assume you have completed the first set of micro:bit activities.
Step 1
Before you begin, be sure that:
- your micro:bit is connected to your TI-84 Plus CE Python
- your micro:bit is inserted in the expansion board.
(Grove shield shown here) - for this activity, you must have a Grove LED accessory and 4-wire connecting cable as shown here connected to pin0.
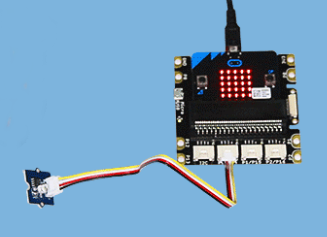
Step 2
Your micro:bit should look like this when it has power from the
TI-84 Plus CE Python graphing calculator:
Recall that the display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
This activity has two parts: an on/off switch and a temporary-on switch.
There’s also a ‘Challenge’ at the end.
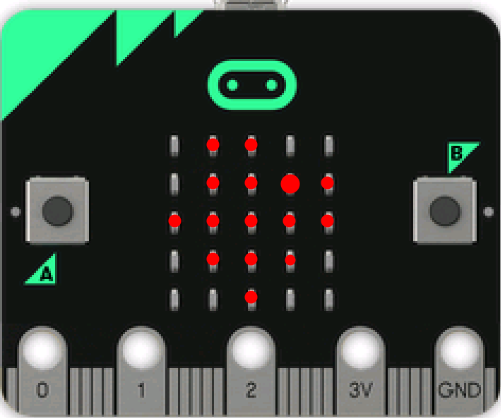
Step 3
The Grove LED is a small circuit board with a white (or colored) LED on it. Turn the yellow dial (a potentiometer) all the way to the right to get the maximum brightness. Connect this LED board to the port labeled P0 (pin0) on the expansion board using the cable included.
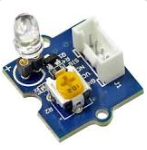
Step 4
Caution! The LED can be removed from its socket on the circuit board. Be careful when re-inserting the wires properly. There is a positive (anode) wire and a negative (cathode) wire and the circuit board is labeled with ‘+’ and ‘-‘ symbols. The negative wire on the LED is indicated by a ‘flat spot’ on the base of the LED as shown here.
Inserting the LED incorrectly will cause it to not work but will not damage it.
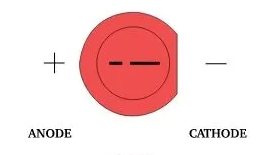
Step 5
Push-button light switches like the one seen here were common in the first half of the 20th century (1900-1950’s) when they were replaced with toggle switches. One button turned the circuit on while the other turned it off (and popped the other button out). These two projects will make similar switches using the micro:bit buttons.
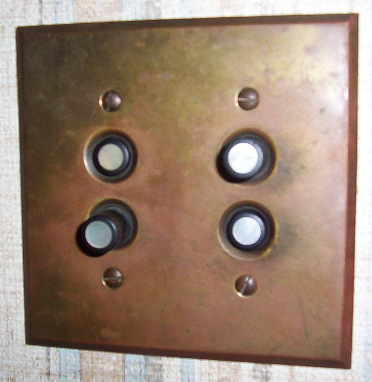
Step 6
Part 1: The 2-button on-off switch
Start a new Python program (SWITCH1) and add:
from microbit import *
Remember to get the statement from <Fns…> Modul <Add-On>
Tip: This statement tests to see if the micro:bit is present. If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit from the calculator and plug it in again (reset).
After the microbit import statement is in the Editor, press [math] to see the micro:bit… menu item. Select it to also add the buttons module and then the I/O pins module to your program.
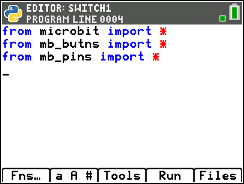
Step 7
Make a while not escape loop using the command found on
[math] > micro:bit… > Commands
while not escape():
♦ ♦
We also added a comment and a print( ) statement to explain the program.
Note that while not escape(): is included in the micro:bit Commands sub-menu. There’s no need to import the ti_system module.
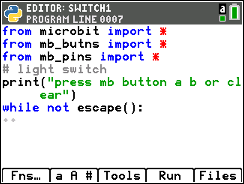
Step 8
In the loop body, add two if statements, one for each micro:bit button using the two _was_pressed() functions:
♦ ♦ if button_a.was_pressed():
♦ ♦ ♦ ♦
♦ ♦ if button_b.was_pressed():
♦ ♦ ♦ ♦
if . . is found on <Fns…> Ctl.
The two button functions are found on
[math] buttons and touch…
under the Button_a and Button_b sub-menus.
One button will turn the LED on and the other will turn it off.
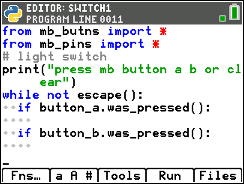
Step 9
The LED lights up when it gets power from the micro:bit. We will use digital values for turning it on and off.
To turn the LED on using button A, use the function:
pin0.write_digital( 1 )
(if your LED is connected to the pin0 port of the expansion board) in the first if block.
pin0 is a special micro:bit variable found on
[math] I/O pins under the Pins sub-menu
.write_digital( ) is on the [math] I/O pins Digital menu.
Add the argument 1 to the function.
Can you figure out how to turn the LED off? See the next step…
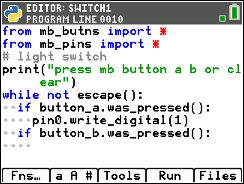
Step 10
In a similar manner, turn the LED off when button B was pressed. Set the digital value to 0 as shown here.
Test your program now to be sure the LED is working properly.
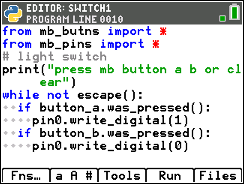
Step 11
Make one enhancement: display the letter of each button on the micro:bit display: Add display.show(“A”) when button A is pressed and a similar statement for button B. Remember that you will have to import the micro:bit display sub-module to your program to use the display.show( ) function.
After the while loop ends (by pressing [clear]), make sure that the LED is off and that the display is cleared (or display the special TI logo image using display.show(ti) to return the micro:bit display to its original state).
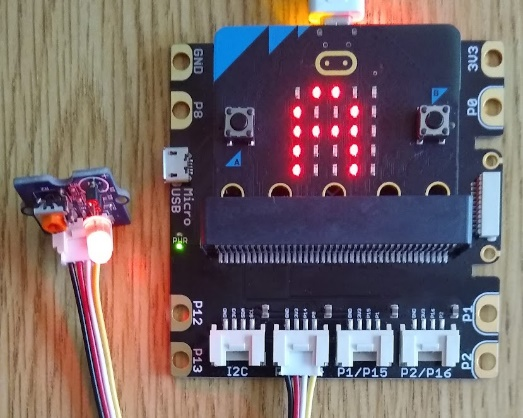
Step 12
Part 2: The ON button
This program will light the LED when button A is held down and it will be off when the button is released. Make a copy of your program from Part 1 of this activity. Use [Files] > Manage (point to switch1) and select Replicate Program
Give the new program a name (ours is SWITCH2).
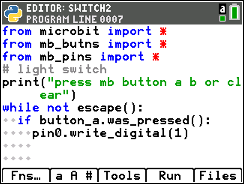
Step 13
In the new program, modify the button A if statement: change was to is.
if button_a.is_pressed():
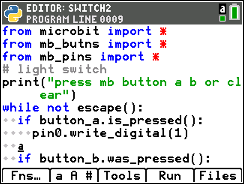
Step 14
We will not need the button B condition, but we will need to turn the LED off when button A is not pressed, so replace the if button_b… statement with an else: clause for if button_a… and turn off the LED in the else: block.
if button_a.is_pressed():
pin0.write_digital(1) # on
else:
pin0.write_digital(0) # off
Note that if button_b… is not needed and is now commented and else: lines up with if!
If you displayed the letter “B” in the first project, change it to a space “ “ here so that nothing is displayed when no button is pressed.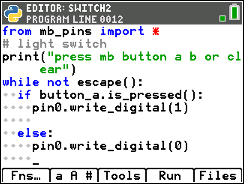
Step 15
Challenge: Make a single-button on-off switch. Can you modify the program again to turn the LED on when button A is pressed once and turn it off when button A is pressed again? That is, button A toggles the LED on and off by itself and does not need to be held down. Button B is not used at all. If you have micro:bit version 2, try using the pin_logo (the gold oval above the 5x5 display in the image).
The display can show a ‘1’ when the LED is on and ‘0’ when it is off.
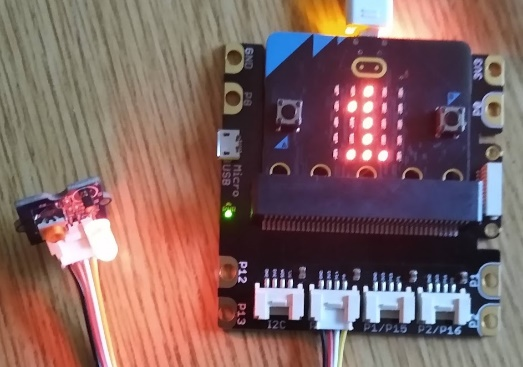
Mini project 2: Weather Face
Download documentsThe micro:bit has an internal temperature sensor in its CPU. In this activity you will use an external temperature sensor to monitor the temperature away from the micro:bit itself to get a more accurate reading. The activity is divided into two parts:
Part 1: The Weather Face
Part 2: Tracking Temperature
These activities assume you have completed the first set of micro:bit activities and will be using an external Grove temperature sensor.
Step 1
Before you begin, be sure that:
- your micro:bit is connected to your TI-84 Plus CE Python
- your micro:bit is inserted in the expansion board.
(Grove shield shown here) - the Grove temperature sensor is connected to pin0
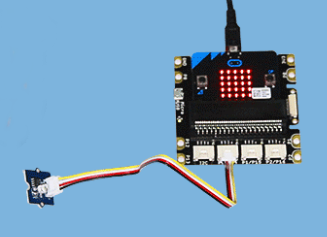
Step 2
Your micro:bit should look like this when it has power from the
TI-84 Plus CE Python graphing calculator:
Recall that the display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
This activity has two parts: a temperature monitoring application and a temperature tracking program.
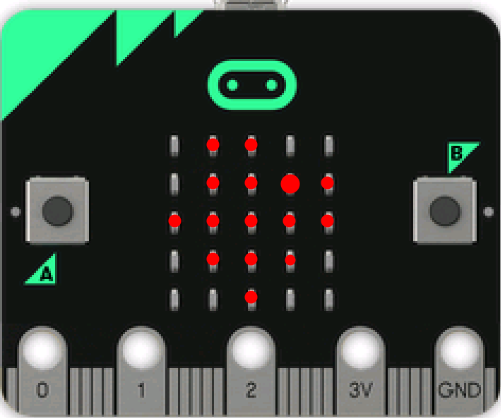
Step 3
This is the Grove temperature sensor: Connect the sensor board to the port labeled P0/P14 (pin0) on the expansion board using the included cable. You can use a different port if you also change the pin variable in the code to match.
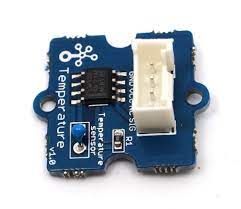
Step 4
Part 1: Weather Face
Some indoor/outdoor thermometers have an image of a person indicating the current weather conditions. This program will display an appropriate ‘face’ on the micro:bit display that will depend on the current temperature.
Start a new Python program. Ours is called WEATHER1.
In the Python Editor use <Fns…> Modul <Add-On> micro:bit to select the import statement:
from microbit import *
Then, with the micro:bit module in the editor, press [math] micro:bit… and select Grove devices… from the menu to paste the two new import statements into your program.
Tip: If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit and plug it in again (reset).
Step 5
Write our favorite loop:
while not escape():
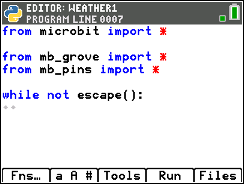
Step 6
In the loop body, read the Grove temperature sensor using the function found on
[math] > grove...
= grove.read_temperature( )
There are two placeholders for you to fill in.
Use any variable on the left of the = sign for your temperature variable.
For the ( ) argument use pin0 (or whatever socket your sensor is plugged into on the expansion board).
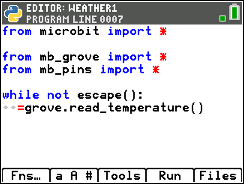
Step 7
In the image to the right, we used the variable t to hold the temperature and pin0 for the argument:
Type the letter t in front of the = sign.
Get the variable pin0 from the [math] I/O Pins… Pins sub-menu
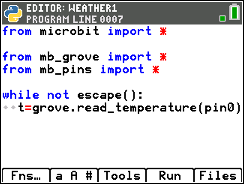
Step 8
Add a print statement to display the temperature to be sure that everything is working:
print(“temperature = “, t)
Run the program now to see temperature values displayed on the
TI-84 Plus CE Python. If you think the numbers displayed are strange, keep in mind that the temperature is displayed in the Celsius scale. If you prefer to work with the Fahrenheit scale, then you will have to do the conversion yourself in the program.
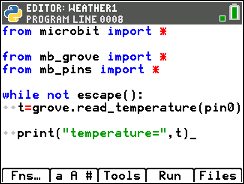
Step 9
Our program will display three different faces depending on the temperature (cold, mild, or hot). This is an ideal place for an
if…elif…else structure. Get this structure from
<Fns…> Ctl
All three components are inserted into your code, and you must complete the structure with conditions (after if and elif) and actions (the three blank lines).
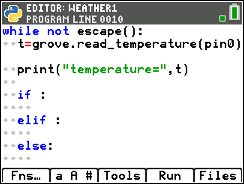
Step 10
To make use of the micro:bit display we need to import the display module at the top of our program, too:
from mb_disp import *
found on [math] micro:bit…
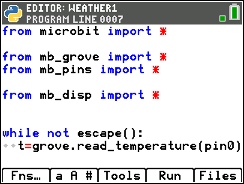
Step 11
The If… block will handle the ‘cold’ temperature. When it’s cold, we’ll display the ANGRY face:
if t < 15:
display.show(Image.ANGRY)
Find both display.show( ) and Image.ANGRY on the [math] Display… menus.
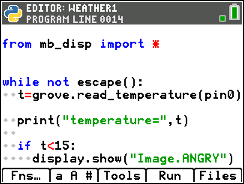
Step 12
The elf : block also requires a condition since it stands for ‘else if…’. This time we check for ‘comfortable’ temperatures between 15 and 27 degrees Celsius. But the first condition took care of the lower bound so we only need to check the upper bound:
elif temp < 27 :
and we can display the HAPPY face:
display.show(Image.HAPPY)
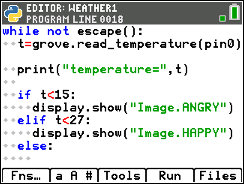
Step 13
What face would you like to show when it’s ‘hot’? Add the statement to display another face in the else: block.
To test your program, you will have to subject the temperature sensor to a range of temperatures. You can place it in a refrigerator for a cool temperature but be careful when looking for a warm temperature. A hot day outside is OK but do not place the sensor in an oven or microwave and do not get it wet. Try rubbing your hands together (feel the heat?) and then place the sensor between your hands.
Can you see all three faces on the micro:bit?
Now try this…
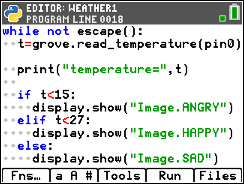
Step 14
Part 2: Tracking Temperature
Let’s monitor the temperature for a while. Use two lists to collect ‘time’ and temperature data and transfer the lists to the TI-84 Plus CE Python for further study.
Make a copy of your WEATHER1 program (or whatever you called it) using the File Manager.
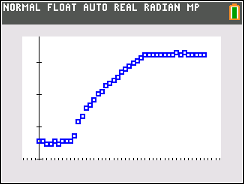
Step 15
In your new program, before the while loop, create two empty lists
times = [ ]
temps = [ ]
and a timer variable. Call it tim so that the variable does not interfere with the time module:
tim = 0
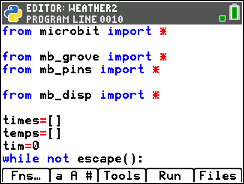
Step 16
Below the print statement, add (append) the current values of time tim and temperature t to their respective lists:
times.append(tim)
temps.append(t)
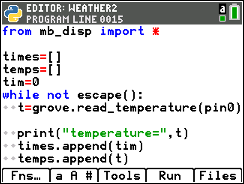
Step 17
At the bottom of the while loop (after the else: block) add a statement to increment the tim counter:
tim += 1increment the tim variable representing one sample.
This statement is indented to be part of the while loop block but not part of the else: block.
Note: the tim counter does not accurately measure seconds. It’s measuring samples. About timing: since it takes time to read the temperature sensor, to print the temperature, and to display the face image, the time between samples is probably more than one second. If you really want the tim variable to actually represent seconds, there are more accurate methods.
Step 18
When the while loop ends, transfer the two lists to two TI-Nspire lists using the function store_list( ). This function is included in the micro:bit module and can be found on the [math] micro:bit… Commands menu.
We need two store_list( , ) functions:
store_list(“TIMES”,times)
store_list(“TEMPS”, temps)
We can use the same list names as the python variable names but the TI-84 names must be all CAPS in quotes. You could also use the built-in lists L1..L6 by using only a number from 1 to 6 in the quotes.
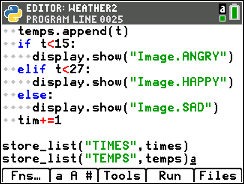
Step 19
After running your program for a while, when pressing [clear] the two lists are stored in the TI-84 Plus CE Python.
Set up a scatter plot of the two lists and display in in an appropriate viewing window. The plot shown here was created by warming the temperature in my hand for a while.
How do you explain this behavior?
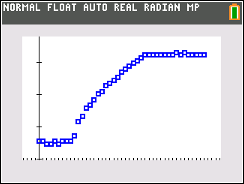
Step 20
The plot seen here was created by placing the sensor in a freezer for a while.
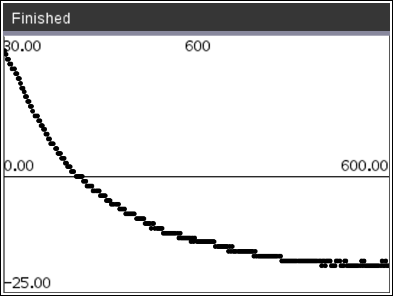
Mini project 3: Intruder alert
Download documentsThe Ultrasonic Ranger can be used to detect a change in distance. This concept can be used to build an alarm-type system that triggers lights and sounds when the distance is not within an allowed range. This activity develops the basic tools for an alarm system and the idea can be adapted to lots of different applications.
This alarm will trigger when a small box containing the ranger is opened.
Step 1
Before you begin, be sure that:
- your micro:bit is connected to your TI-84 Plus CE Python
- your micro:bit is inserted in the expansion board.
(Grove shield shown here) - the Grove accessories you will need are coming up…
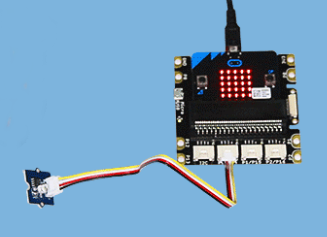
Step 2
Your micro:bit should look like this when it has power from the
TI-84 Plus CE Python graphing calculator:
Recall that the display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
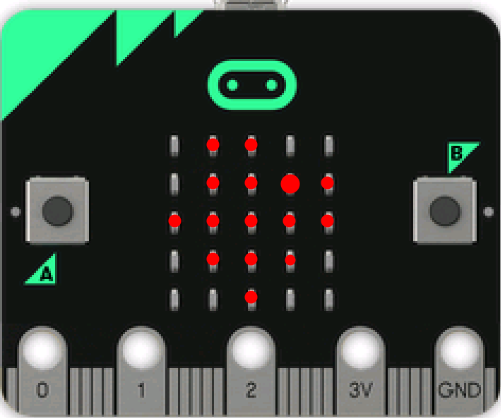
Step 3
This activity uses three external devices: the ultrasonic ranger, a speaker, and the LED that was used in the Light Switch activity. On the LED board, remember to turn the yellow dial (a potentiometer) all the way to the right to get the maximum brightness. Make the following micro:bit connections:
pin0: speaker
pin1: ranger
pin2: LED
Note micro:bit version 2 has an on-board speaker. An external speaker can also be used. We will also use the micro:bit display board to flash lights in a custom pattern.
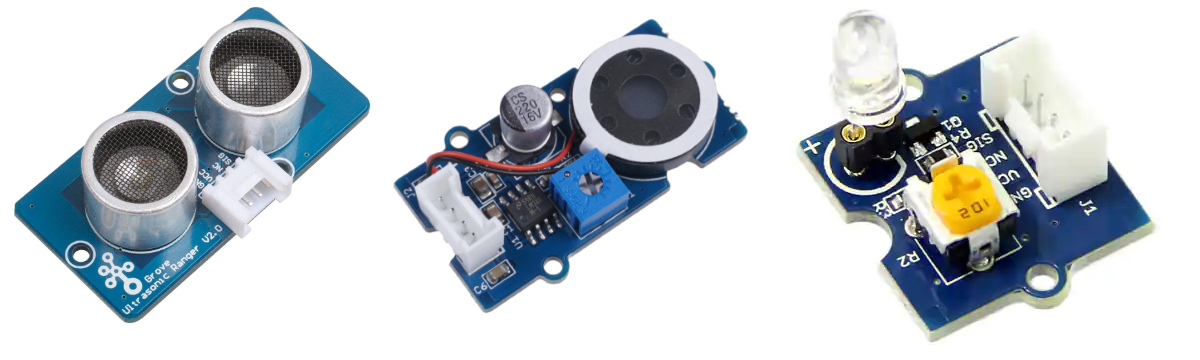
Step 4
Start a new Python program. We named it INTRUDR1. Add the micro:bit module to your code using <fns…> Modul <Add-On> micro:bit:
from microbit import *
Tip: If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit and plug it in again (reset).
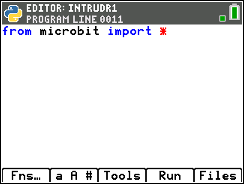
Step 5
With the micro:bit module in the editor, add some additional components using the [math] micro:bit… menus.
Grove Devices will add both the mb_grove and mb_pins modules.
Music adds both mb_music and mb_notes modules.
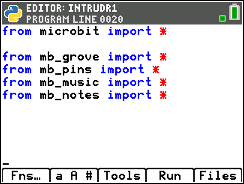
Step 6
The program is divided into two parts:
- a loop that ‘watches’ the distance, and
- another loop that produces the alarm effects: lights and sounds.
There are two while loops in the program…
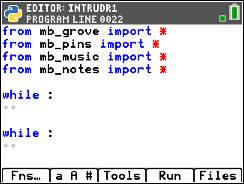
Step 7
We’ve added a statement to clear the micro:bit display and to show that the alarm is set on the calculator screen.
The first loop simply monitors the distance from the ranger that is connected to pin1 on the expansion board:
dist = grove.read_ranger_cm(pin1)
while dist < 10:
dist = grove.read_ranger_cm(pin1)
Get grove.read_ranger_cm() from [math] grove… Complete the statement by adding the variable name and the pin variable.
Recall that the ranger will be inside a small (<10cm high) closed box with a lid. The ranger (not the micro:bit) is placed on the inside bottom of the box facing upward to detect when the box cover is opened. If the lid is closed this loop will continue. When the lid is opened this loop ends.
Step 8
Sounding the alarm:
The second loop creates the alarm effects. If you have an external speaker and LED you can use those to produce lights and sound. You can also use the on-board display and speaker (speaker is on micro:bit version 2 only).
We will use the [clear] key to end the program:
while not escape():
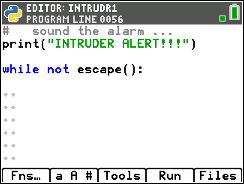
Step 9
To make the LED turn on and an alarm to sound, use the functions
grove.power(pin2, 100)
music.pitch(440, 100)
grove.power( ) is found on [math] grove… Output menu
pin2 is the connection for the LED
100 is full intensity (brightness)
music.pitch( ) is found on [math] music…
440 is 440Hz which is middle A on the music scale
100 is 0.1 seconds (100 milliseconds)
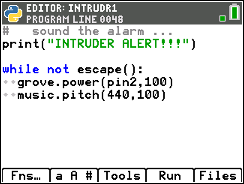
Step 10
To make the LED blink and the alarm to sound ‘alarming’ add two more statements to turn the LED off and change the musical note:
grove.power(pin2, 0)
music.pitch(220, 100)
You can choose other frequencies for the musical tones.
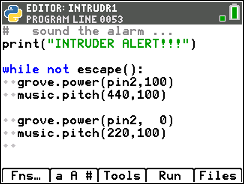
Step 11
Let’s add a flashing display effect on the micro:bit using
display.show( ).
Remember to first import mb_disp for the display functions.
The display can show any of the images in the built-in collection or you can make your own custom images using the Image( ) function (shown here) found on [math] display….
These image definitions can go at the top of your program (below the import section and before any loops).
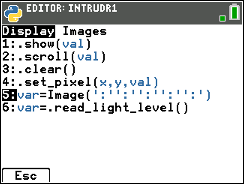
Step 12a
Here are two sample custom images:
The variable names are img_alarm1 and img_alarm2
The argument is a “string” of 25 digits (each from 0 to 9 indicating brightness) for each of the 25 pixels on the display. Each row of 5 values is separate by a colon (:).
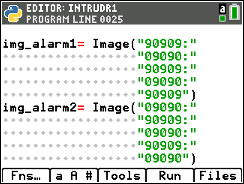
Step 12b
It is convenient to write the string on five separate lines to arrange the digits as they will appear on the display (in a 5x5 grid):
Image("90909:"
"09090:"
"90909:"
"09090:"
"90909")
But it is also possible to write the string in one long line:
Image("90909:09090:90909:09090:90909")
or with extra quotes:
Image("90909:””09090:””90909:””09090:””90909")
Python will concatenate the separate strings for you!
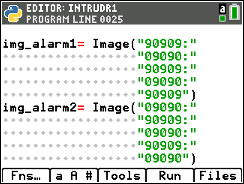
Step 13
img_alarm1 lights up every other pixel on the display in a ‘checkerboard’ pattern.
img_alarm2 does the opposite pattern.
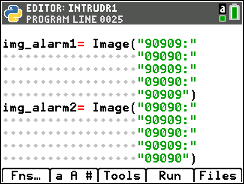
Step 14
Finally, to get the display to flash when the alarm goes on, add two statements to your alarm code to display the two images (at the right moments)
display.show( ) is on [math] micro:bit… display…and just type the names of your custom image variables as the arguments.
This image also shows the two optional arguments for display.show(): delay is in ms, wait=True tells the system to wait until the image is displayed for the proper time before proceeding to the next statement.
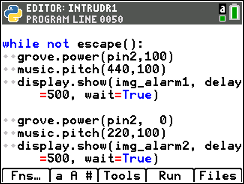
- MP 1
- MP 2
- MP 3
Tello Drone
Mini project 1: Hello Tello
Download documentsThese mini-projects are designed to help you learn to operate the Tello flying drone using your TI-84 Plus CE with Python programming.
They assume you have a working knowledge of Python programming on the TI-84 Plus CE Python. While the hardware setup requires a micro:bit, expansion board, and wi-fi adapter, the Tello functions and methods do not require coding skills involving these intermediate devices.
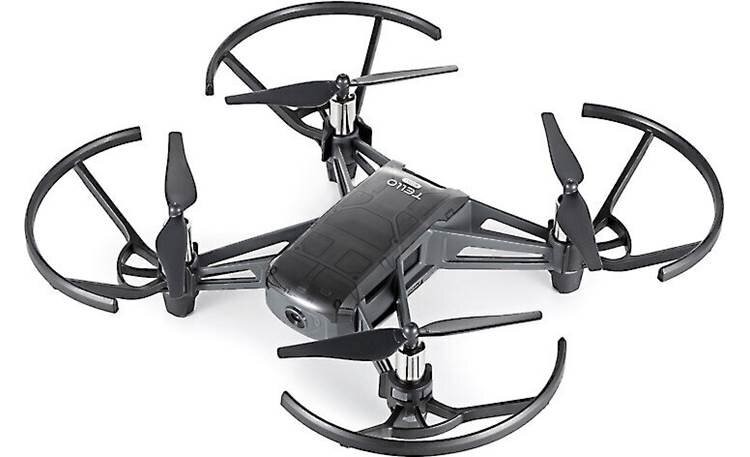
Intro 2
The TI-84 Plus CE communicates with the Tello using:
- the Tello Python module
- a BBC micro:bit v2 board with the appropriate TI runtime
- a micro:bit expansion board,
- a small wi-fi board,
- an external power supply.
The setup seen here involves connecting the hardware, installing the Tello Python module to your TI-84 Plus CE graphing calculator, installing the special TI-developed micro:bit .hex file, and then configuring the system (pairing the Tello drone with the micro:bit).
You can watch the YouTube videos for an overview of this process or read the setup instructions included in the download package. The necessary files (tello.8xv, tellocfg.8xv, and ti_runtime*.hex) along with detailed step-by-step instructions are available here.
This first mini-project just gets the Tello drone off the ground…
Intro 3
Tello needs to ‘see’ the ground and a colorful, patterned surface helps Tello maintain its position in the air. Tello has a camera on the bottom and a ‘Vision Positioning System’ to help it stay in one place while hovering. If the surface below Tello is a plain, solid color (that is, no pattern) then Tello switches to an ‘Attitude Positioning System’ which is not as reliable as its Vision System so be careful. The blinking LED on the front of Tello indicates which system is in use (green=Vision, red= Attitude). This is explained in more detail in the Tello User Manual.
Proceed to the next step to program your TI-84 Plus CE Python graphing calculator to control Tello when your hardware and software are ready.
Step 1
From the TI-84 Python File Manager, start a new Python program (ours is called telfirst.py). Import the tello module using [<Fns...> Modul <Add-On> and select
from tello import *
Note: if you do not see Tello on the Add-On Module Import screen then the module is not properly installed in the calculator.
Note: you will also see the tellocfg module on the <Add-On> menu. This module is used to configure the Tello/micro:bit system when necessary.
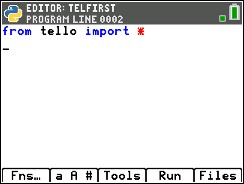
Step 2
This is the tello module menu system. Note that there are five submenus: Fly, Data, Maneuver, Set, and Commands that contain all the available Tello methods. You will investigate many (but not all) of these methods in these mini-projects.
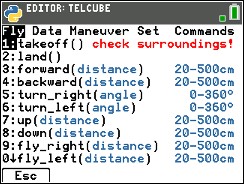
Step 3
Make sure the Tello is turned on. There will be a rapidly blinking yellow LED on the front of Tello indicating that Tello is ready to be connected to wi-fi. Check your connections now by running the program.
You should see:
Connecting to micro:bit...
Micro:bit is connected
Connecting to Tello 1...
Tello 1 is connected✓
>>>
If there is a connection problem, an error message will appear instead. Your Tello may have a different ‘name’ that was assigned during the setup process. The Tello used here is named ‘1'.
Step 4
A good practice is to check the Tello battery charge at the beginning of your programs.
Get the battery( ) function from
[math] > tello drone…> Data > var=battery()
And insert a variable name in front of the equals sign: b is a common choice, for ‘battery’.
Run the program again.
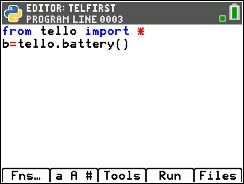
Step 5
The battery() function reports the battery charge level as a percentage. If the charge level is too low (<10%), Tello will not take off.
Note: there’s no need to print(b). The battery() function, as well as most other Tello methods, displays information for you.
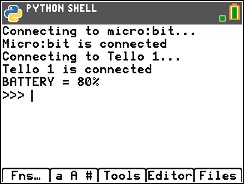
Step 6
If the connections are still successful and there’s enough battery charge, then prepare to fly!
Using the takeoff() command causes the Tello to fly upwards about 70 - 80cm (about 27-32 inches). Be sure there is room above the Tello for this maneuver.
Also, what goes up must come down. We must have full control of the drone at all times, so we must make the Tello land() as well.
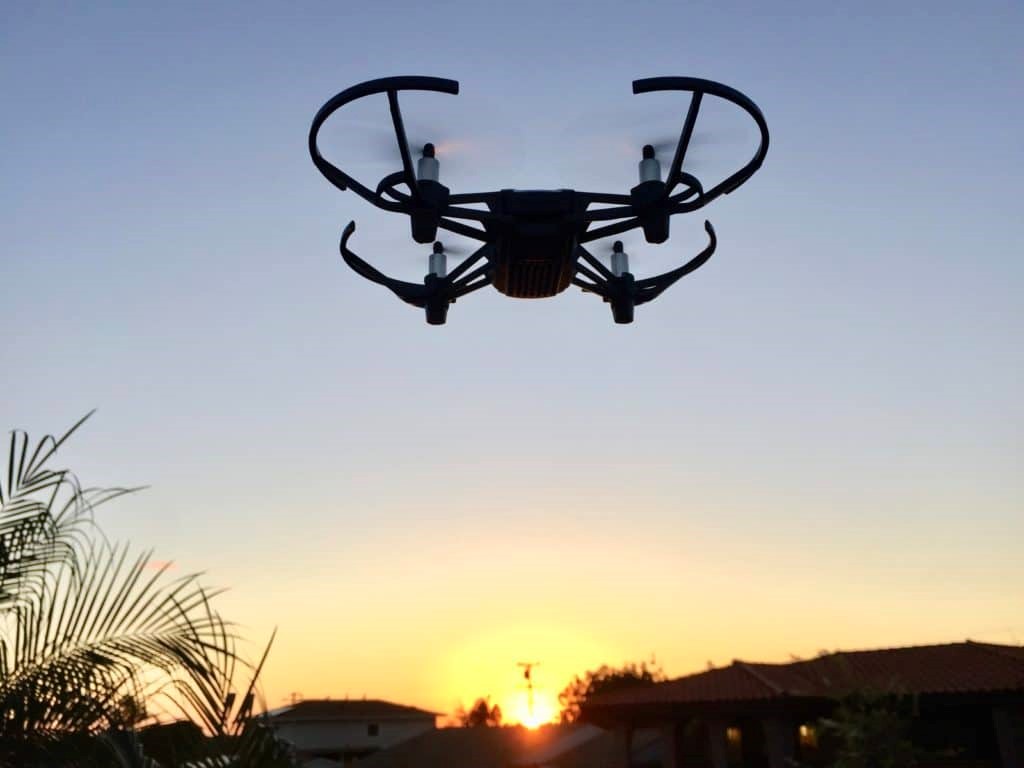
Step 7
[math] > tello drone… > Tello > Fly
tello.takeoff()
tello.land()
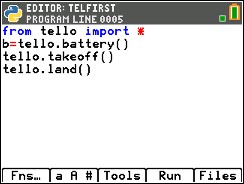
Step 8
Again, make sure all hardware is on, including Tello, and run the program. Your Shell screen will display the previous ‘Connecting…’ messages and then show messages for each command that is sent to the Tello. The Tello will go up, hover for a few seconds, and then land.
Tello should land very close to its starting location. If it drifts a little bit that’s OK, but if it wanders too far, then Tello is having trouble ‘seeing’ the ground. Be sure the surface has a colorful pattern and that the area is well-lit.
Tello communicates with the micro:bit and the TI-84 to confirm that the tasks are properly Completed .
After Tello lands, the program is finished.
Step 9
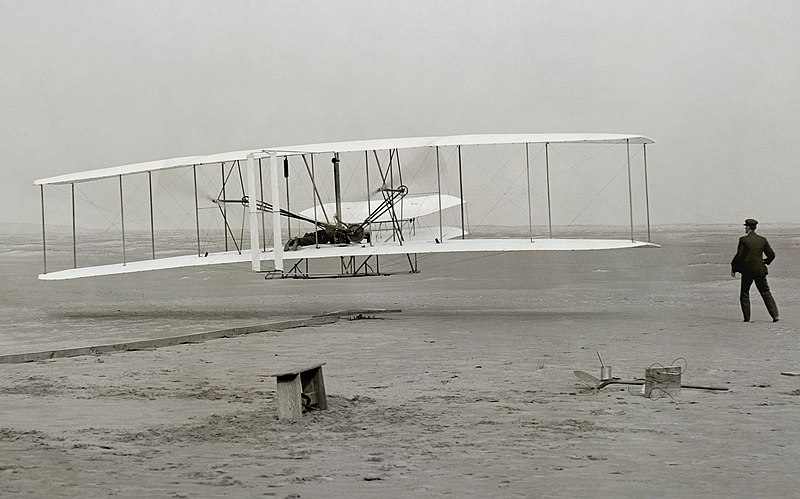
* John T. Daniels, Public domain, via Wikimedia Commons
https://commons.wikimedia.org/wiki/File:First_flight2.jpg
Congratulations on your first successful flight! The Wright Brothers would be proud of you!
Let’s try another maneuver…
Step 10
The [math] > tello drone… > Fly menu contains lots of possible maneuvers.
Two simple but elegant maneuvers that are relatively safe are the turn_right( ) and turn_left( ) methods. These keep the Tello in one position and rotate it the given number of degrees.
Trytello.turn_right(360)
tello.turn_left(360)
while Tello is in the air. Insert these functions between the takeoff() and land() methods in your program and enter the number of degrees to turn as each argument.
See the next step for the code…
Step 11
Your program should look like the one shown here.
Again, re-check your hardware, including Tello itself and then run the program. Tello turns itself off after a few minutes of inactivity to save the battery.
Tello will take off, spin to the right a full turn, then spin to the left a full turn, then land – hopefully to the same place that it took off!
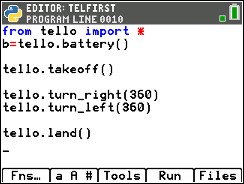
Step 12
While the program is running, your TI-84 Plus CE Python screen will report the progress of each maneuver.
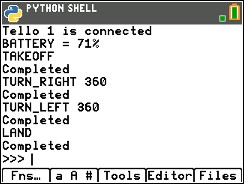
Step 13
Another pair of maneuvers to use are tello.up( ) and tello.down( ) which are also found on the Tello > Fly menu.
The arguments, distance, are measured in centimeters. Be sure that when flying upward that there is room above to make the maneuver. Tello does not have a camera or sensor on top so it may crash into the ceiling!
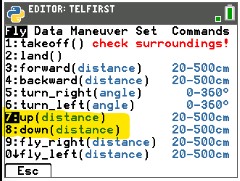
Step 14
Fail: When using tello.down( ), Tello cannot go below about 30cm (about 12 inches) from the surface. Tello does have a sensor on the bottom to measure its height above the ‘ground’. In the screen to the right, after takeoff, Tello attempted to go down 100cm as the code requested, but only went down about 50cm, where it reached the 30cm height limit. It hovered there for a while then reported “Fail” and landed the drone. The program’s land( ) function also failed because the drone had already landed.
There are other situations where an operation can ‘Fail’.
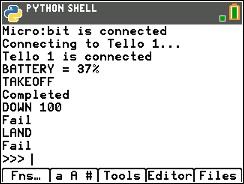
Mini project 2: The One Where Tello Tours the Room
Download documentsAfter successfully completing mini-project 1 your TI-84 Plus CE-to-Tello system is properly set up and ready to fly. In this mini project, your Tello will depart its landing zone and take a tour of your room based on your measurements.
Remember the caution from project 1: the surface that Tello flies over needs to be a varied pattern. If the ‘floor’ is a smooth, uniform color then Tello will have difficulty maintaining its ‘Vision Positioning System’ and could possibly wander off course. And be sure the flight area (the entire room) is well-lit so that Tello can ‘see’ the ground. Keep a watchful eye on Tello as it flies around the room and be prepared to ‘pick up the pieces’. Tello does not look forward.
Also be aware of the surroundings. You do not want to crash into something or hit someone.
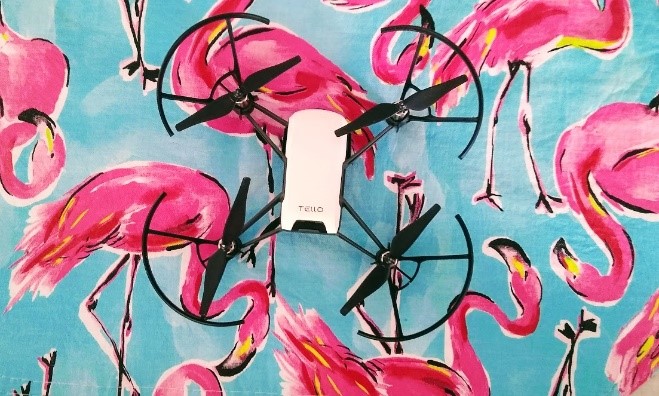
Intro 2
The room you are working in is likely in the shape of a rectangle. Let’s start the Tello in the center of the room, fly toward one wall, then fly along the four walls and return to the center of the room. See the red route pictured here.
In order to perform this task, you need to measure the dimensions of the room in feet. Use a regular carpenter's tape measure to measure the room. Write down your measurements to use in the program.
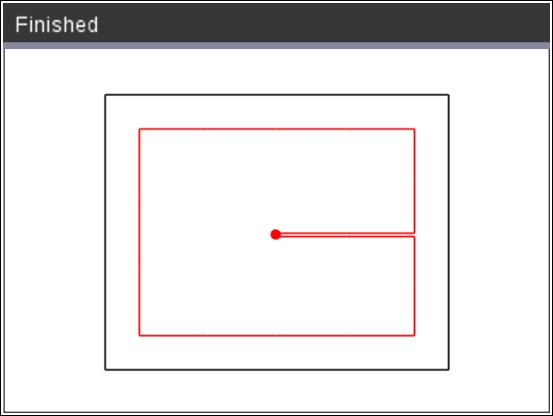
Step 1
Begin a new Python program (ours is called teltour.py). Import the Tello module from <Fns….> Modul <Add On> from tello import *
from tello import *
and perform a pre-flight check of the battery level before flying:
b = tello.battery( )
found on [menu] More Modules > Tello > Flight data.
Use a variable in place of the var placeholder. If the battery is too low (10% or less) Tello will refuse to take off. If you think there is not enough charge to complete your mission, you can stop the program by pressing [on] on the keypad.
Tello will eventually land.
Step 2
Since you measured the room in feet and Tello only accepts measurements in centimeters, define a function to convert feet to centimeters. Get
def ( ) :
from <Fns…> menu
Also, get the return statement from the same menu and be sure it is indented to be the last statement of the function. We fill in the details next…
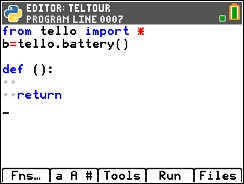
Step 3
The function name can be any identifier, such as f. We prefer to use a meaningful name, so we’ll use ftcm and use the argument ft to represent the incoming value, a distance measured in feet.
The block of the function is the formula that converts feet to centimeters:
cm = ft * 12 * 2.54
and the return statement uses the variable cm to send its value back to the program. The completed function is:
cm = ft * 12 * 2.54
return cm
You will use this function in the Tello Fly methods that require a distance. Now to code the main flight plan.
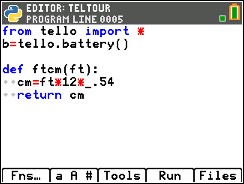
Step 4
For simplicity in running the program many times to work out the kinks in the flight, assign the dimensions of the room to two variables: w for width and le for length. The room we are using is 14 feet wide and 20 feet long. Your room will likely be different, but the algorithm should apply to any room size.
Notes:
- We use the identifier le because l (lowercase ‘L’) can be confused with the letter ‘I’ and the number 1. ln is the natural logarithm and len is a built-in length function.
- To make a more user-friendly program you can use two input functions to enter these values, but who wants to type in two numbers every time you test the program?
- Tello's maximum distance for a leg is 500cm, or about 16.4 feet. If your room is larger, then limit the dimensions so that Tello does not exceed this travel limit in any one leg of the journey.
Step 5
Recall that our flight plan has Tello start in the center of the room facing one wall (w in our code). The front of Tello has the tiny status LED. The back holds the battery.
The Tello function tello.forward(cm) causes Tello to fly forward some distance. How far is it to the wall?answer: length/2
but we don’t want Tello to crash into the wall, so we stop short of the wall, say leaving 2 feet of space to the wall: le / 2 – 2 Note: The drawing to the right was made with Turtle graphics
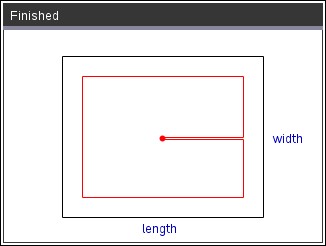
Step 6
In your program, issue the takeoff function, then use the forward function, both found on [math] > tello drone > Fly
The forward(distance) argument is a value in centimeters, but our distance to fly, length / 2 – 2, is in feet so we use our conversion function as part of the argument:
tello.forward( ftcm(le / 2 – 2) )
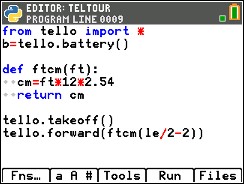
Step 7
For testing purposes, temporarily use the tello.backward( ) function to return Tello to its starting location to make sure that Tello behaves properly. Use the same argument as in the tello.forward( ) function.
Then use the tello.land( ) function.
Remember that all tello.fly methods are on
[math] > tello drone > FlyDouble-check your code, stand back, make sure the flight path is clear, then <Run> the program.
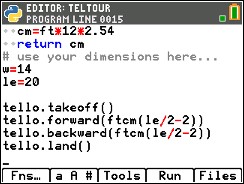
Step 8
If Tello returns to (approximately) its starting position, then you can complete the program to fly around the room. The next portion of the path travels along the wall (the bold red line seen here).
What is the length of this segment?
Answer: width / 2 - 2
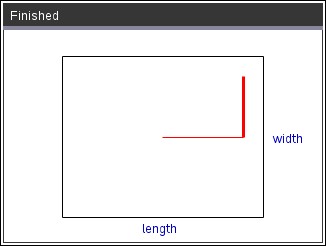
Step 9
At this point you, have two options: use tello.turn_left(90) and use tello.forward( ) or just use tello.fly_left( ).
tello.fly_left( distance ) makes Tello fly to its left without turning.
If it is safe to land in the corner, then use the tello.land( ) function and test your flight now. And try both methods mentioned above as shown in the image as a #comment.
For the rest of the tour, you should stick with the method you use here: either (turning and forward) or just (flying in different directions).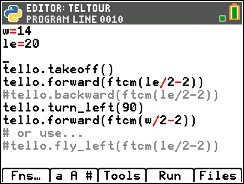
Step 10
The third leg of the trip is along the wall. How long is this segment?
Answer: length – 4
Remember that we must stay 2 feet from all walls.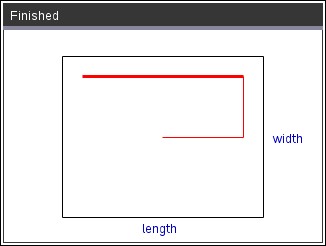
Step 11
Again, use either
tello.turn_left(90)
tello.forward( distance ) assuming Tello is always flying forward
or use
tello.backward( distance ) assuming Tello is not turning.
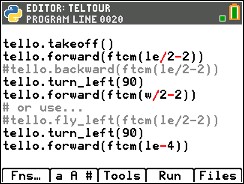
Step 12
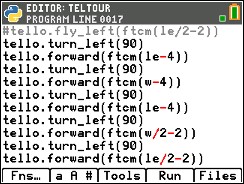
tello.forward(ft_to_cm(length / 2 - 2))
#tello.backward(ft_to_cm(length / 2 - 2))
tello.turn_left( 90 )
tello.forward( ft_to_cm(width / 2 - 2) )
tello.turn_left( 90 )
tello.forward( ft_to_cm(length - 4) ) tello.turn_left( 90 )
tello.forward( ft_to_cm(width - 4) )
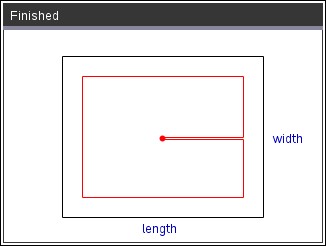
tello.forward( ft_to_cm(length - 4) )
tello.turn_left( 90 )
tello.forward( ft_to_cm(width / 2 - 2) )
tello.turn_left( 90 )
tello.forward(ft_to_cm(length / 2 - 2))
tello.land()
If Tello’s ‘Vision Positioning System’ works properly, Tello should have no trouble making this Grand Tour of your room!
Step 13
To travel the same route without turning, use the Tello flying methods .forward( ), .fly_left( ), .backward( ), and .fly_right( ) in the proper sequence using the proper distances. The final program will be much shorter because there is no need to turn.
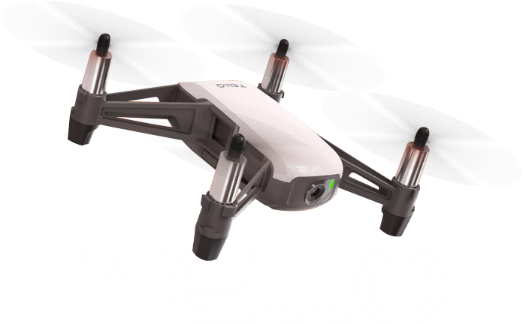
Mini project 3: Tello Search and Rescue
Download documentsOn Thanksgiving (USA) 2022, a cruise passenger fell overboard in the Gulf of Mexico. The US Coast Guard set out on a rescue mission to locate the passenger. Their search area was over 7,000 square miles. They located and rescued the passenger after he spent 20 hours treading water in the middle of the Gulf. How did they do that?
In December 2022, two men started sailing a boat from New Jersey to Florida. The Coast Guard started a search after reports the men were overdue in their journey. Eventually, the agency searched a combined 21,164 square miles from northern Florida to New Jersey. The men were rescued after 10 days at sea. How did they do that?
See “Using drones in Search and Rescue”Intro 2
In this mini-project, you will write a Python program to conduct a coordinated ‘search’ of an area using Tello. There are several search patterns (routes) that professionals use to conduct a systematic search of a region depending on the situation.
Get your TI-84 Plus CE Python and Tello system ready to fly…
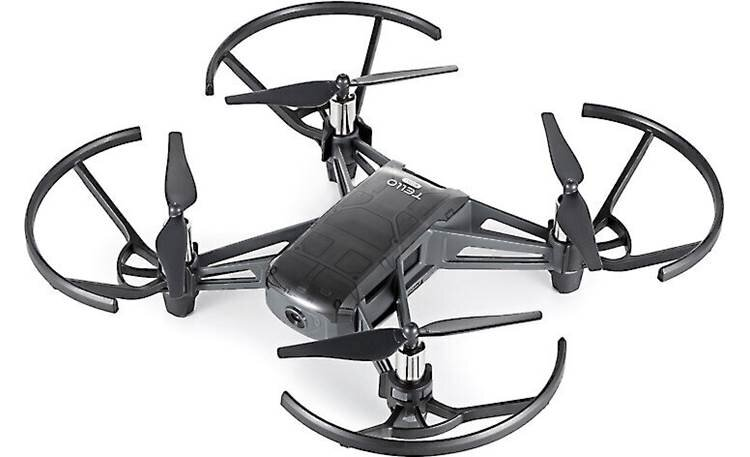
Step 1
Here is your first pattern, called the ‘parallel track search’. A drone starts in the lower left corner and flies the indicated path. At the end of the search, the drone can either land or return to its starting position.
Inputs to the program will be the length and width of the rectangular search area plus one other important measurement.
The program will calculate the lengths of each of the flight segments and the number of steps needed to complete the search and then use a loop to conduct the flight.
Note: screenshot made using turtle graphics..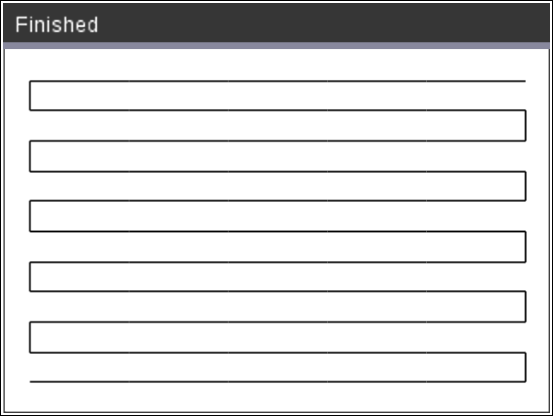
Step 2
For the purpose of this project, length is the horizontal distance (the parallel tracks) and width is the vertical distance in the image shown here.
The other important measurement in this search pattern is called the “sweep width” the distance between the (horizontal) flight segments.Using length, width and sweep_width you can create an algorithm for Tello to complete this mission.
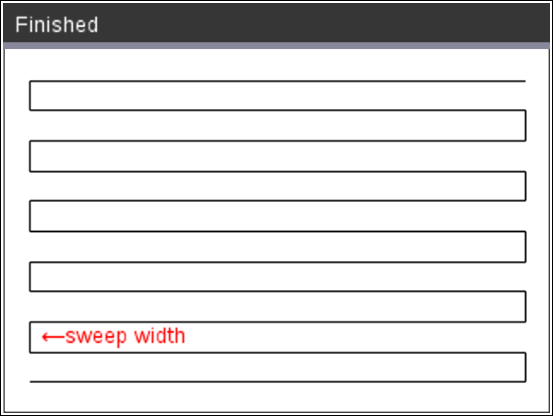
Step 3
Begin a new Python program (ours is named telsrch0.py). Import the Tello module and perform a battery check.
Use [math] > tello drone... for all the Tello functions.
Create three variables to define the search pattern:
w = 80
lnth = 100
sw = 20 # minimum distance*
These three values are all that is needed to conduct the search.
* Note: Tello flight distances are limited to be between 20 and 500 centimeters.
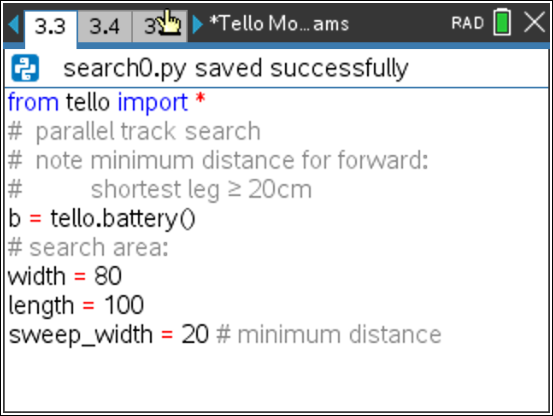
Step 4
One ‘step’ of the search consists of two horizontal segments and two short sweep_width segments as seen here. At the end of this path, Tello will be pointing in the same direction as it started (facing to the right in the image) so that this pattern can be repeated to complete the entire search.
We need to calculate the number of these steps needed to complete the search. See the next step…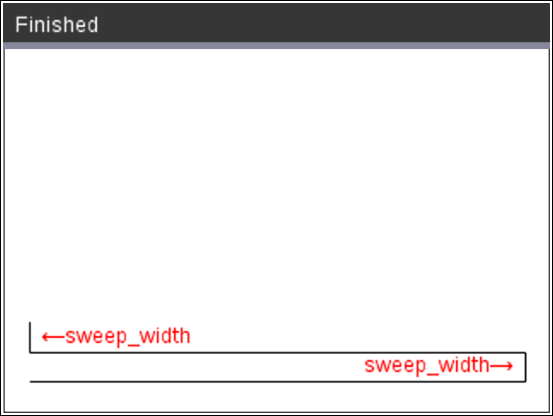
Step 5
In the image shown here, each flight segment is shown in a different color. The last (top) segment is added separately. How many steps are needed? steps depends only on the sweep_width and the width of the search area. Notice that there are two sweep_widths in each segment, so the number of steps needed is:
s = w // (2 * sw)
We use integer division (//) because this value will be used in a for loop.
Parentheses are required to control the order of operations.
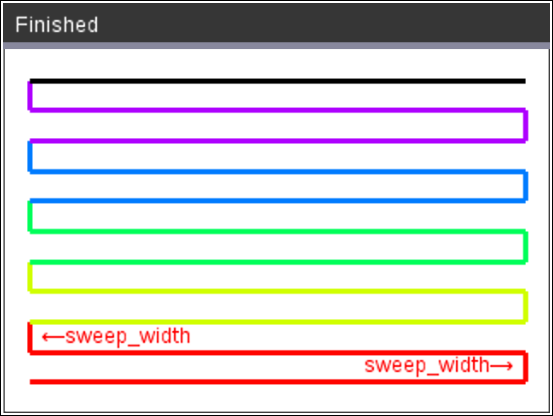
Step 6
In your program, calculate the number of steps, launch Tello, and start a for loop to conduct the search:
s = w // (2 * sw)
tello.takeoff( )
for i in range( s ):
♦ ♦
Note that steps must be an integer.
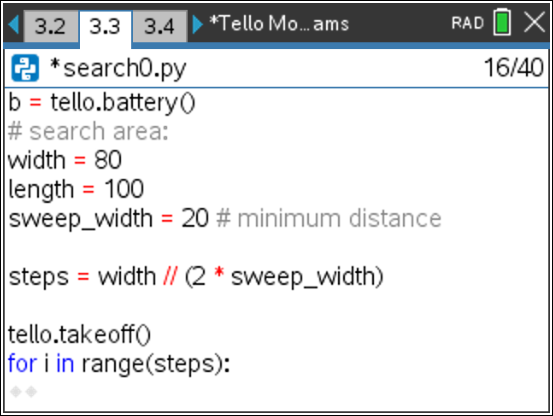
Step 7
The loop body (indented) will contain two long flights (the length of the area) and two short ones, the sweep_width.
Recall from the last mini-project (Tour the Room) that there are two methods you can use: turn at each corner or just fly in the proper direction. Since we used the turn method in the last project, let’s use only the proper fly routines here.
Start by flying forward the length of the area:
♦ ♦tello.forward(lnth)
Try completing the loop yourself before looking at the code in the next step…
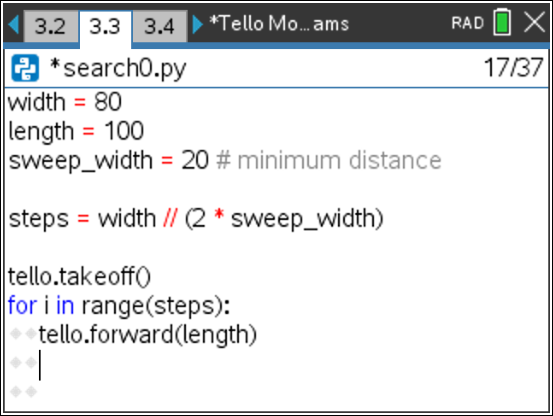
Step 8
The complete loop is:
for i in range(s):
♦ ♦tello.forward(lnth)
♦ ♦tello.fly_left(sw)
♦ ♦tello.backward(lnth)
♦ ♦tello.fly_left(sw)
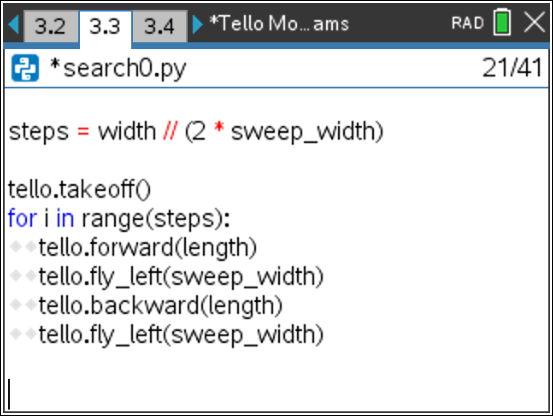
Step 9
This loop (repeated s times) will leave Tello in the upper left corner. You have two options: quit searching here or complete the top segment to go to the upper right corner (the bold black segment pictured here). In either case, you must make sure Tello returns to the landing zone where it started because it might not be safe to land anywhere else.
We’ll take on the challenge of completing the entire pattern and then returning home…
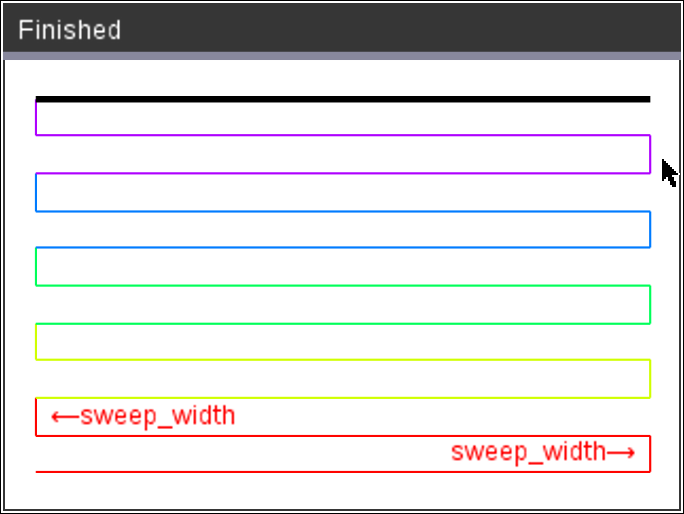
Step 10
tello.forward(lnth)
to complete the pattern.
This statement (and the ones that will follow) is not indented because it is not part of the for loop.
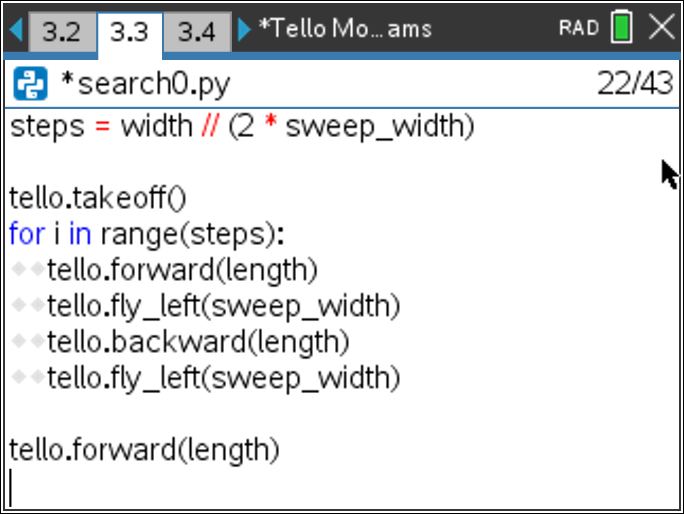
Step 11
tello.fly_right(w)
tello.backward(lnth)
tello.land()
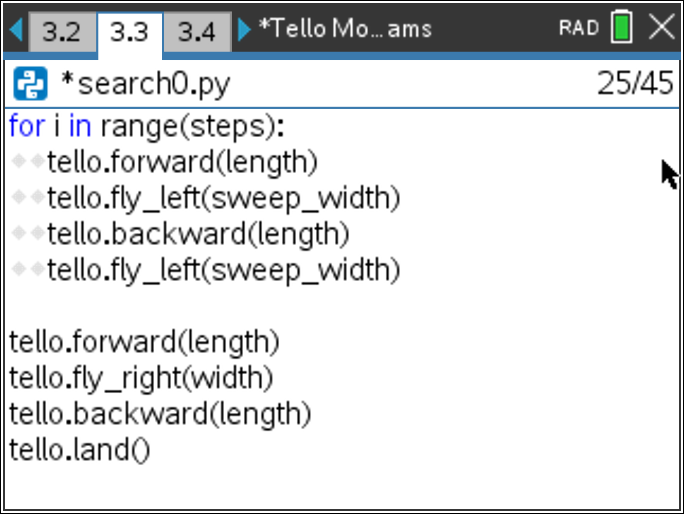
Step 12
This flight can be tricky. Start with a small search area. Since the sweep width must be at least 20cm, try lngh = 50, w = 60, sw = 30 to test your code. Use a large, safe, open area (a square meter or more ) for testing.
Start Tello in one corner of the region facing in the proper direction, and give it a try. You should get the pattern seen here and Tello should return to its takeoff site.
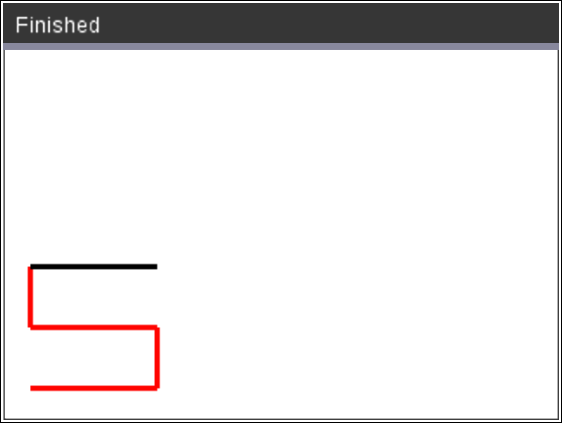
Step 13
There are other search and rescue patterns that can be found online.
Another interesting one is the “expanding box” pattern pictured (created using Turtle Graphics). Can you make Tello fly this ‘square spiral” pattern? How about other spirals?
Special thanks to the United States Coast Guard for their courage, talent, and inspiration.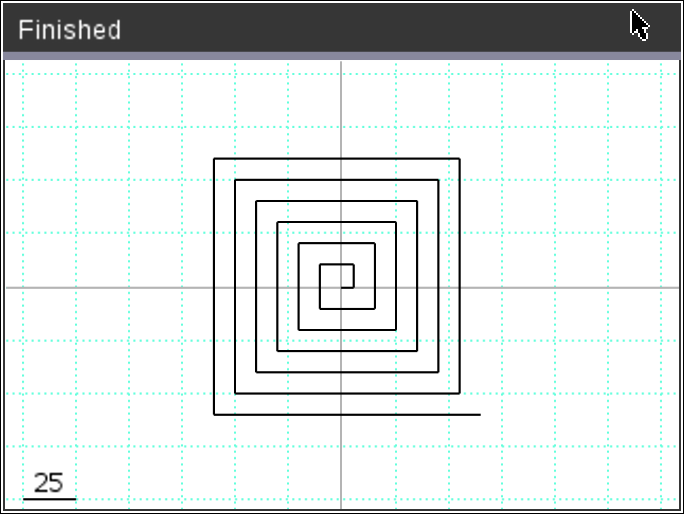
Step 14
Extensions (optional)
For additional activities to use Tello, check out the downloads here:
- Tello’s Data: Tello contains sensors that can detect some information about its surroundings and position. This mini-project introduces some of these sensors and explores patterns among the data collected.
- Fly the Cube: Tello flies forward, backward, leftward, rightward, upward, & downward. It can fly in THREE DIMENSIONS (3D, also known as space). Using a special Tello “Mission Pad”, which assigns Tello a 3D coordinate system so that you can tell Tello to directly .goto( ) a particular point in space by giving the point’s three coordinates as a location. Note: The Tello EDU model is required.
- MP 1
- MP 2
- MP 3