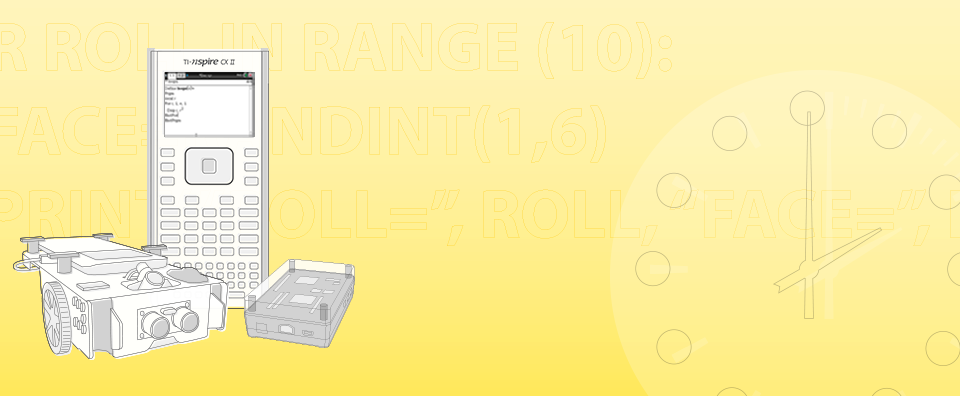
10 Minutes of Code: Python and TI-Innovator™ Technology
These short, easy-to-teach activities are designed to be completed in order, using TI-Nspire™ CX II and TI-Innovator™ Technology.
Note: These lessons require the use of TI-Nspire™ CX II Technology with OS 5.2 and above.
Unit 1: Getting Started With Python and the TI-Innovator™ Hub
Skill Builder 1: Light It Up
Download Teacher/Student DocsIn this lesson, you will learn the basics of writing and running a Python program and using the "light" (the red LED) on the TI-Innovator™ Hub.
Objectives:
- Create and run a Python program
- Control the light on the TI-Innovator™ Hub
Step 1
Welcome to the world of TI-Innovator™ Hub programming using Python on your TI-Nspire™ CX II graphing calculator! Your first program will operate the red LED on the TI-Innovator™ Hub circuit board. It is hard to see on the board, but when you turn it on you will know it.
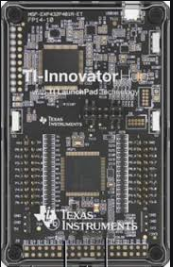
Step 2
-
Open a new TI-Nspire™ Document. The available applications are listed.
Select Add Python and then select New….
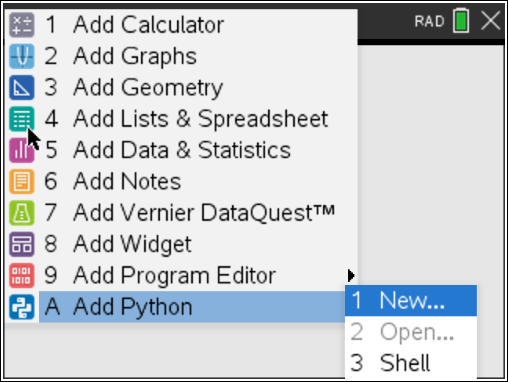
Step 3
-
Type a name for the Python program (we use u1sb1) and press enter.
We will look at the "Type:" menu later.
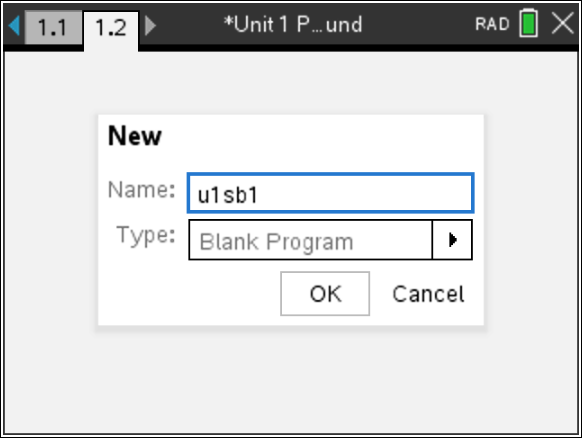
Step 4
You are now in the Python Editor. Press the menu key. Each of these menu items contains related Python programming tools. Our main interest for now is the TI Hub menu. Select the top item from that menu:
from ti_hub import *
This Python command gives you the tools (commands) needed to operate the devices on (or connected to) the TI-Innovator™ Hub.
Also, this statement will check to see if a TI-Innovator™ Hub is connected. If not, then the program will not run.
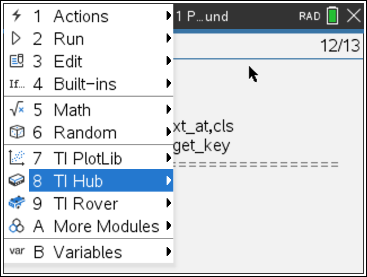
Step 5
The next statement you will use is:
light.on()
Can you guess what it does?
This statement is found on
menu > TI Hub > Hub Built-in Devices > Light Output > on().
All TI-Innovator™ Hub-related features are on the TI Hub menu.
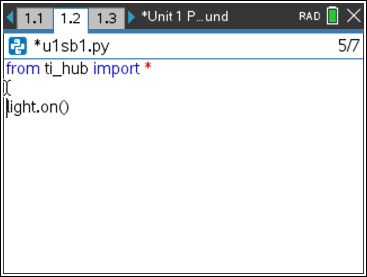
Step 6
-
You are now ready to run this amazing program. You could use
menu > Run > Run (Ctrl+R)
or simply press ctrl+R on your keypad. Your TI-Nspire™ CX II graphing calculator screen will look like this one. Pressing ctrl+R added a page to your document and placed a Python Shell app on it. You are now using the Python Shell. The Shell is similar to the calculator application on the TI-Nspire™ family graphing calculator. This is the place where Python programs are executed (actually, the only place).
The >>> symbol is the Python command prompt. It is waiting for the next command. But if you look at the TI-Innovator™ Hub you will see a red LED lit up. That’s the result of the light.on() statement in your program.
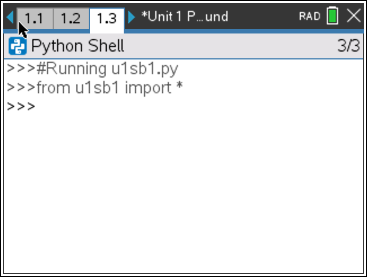
Step 7
-
Your Python program is now on the page before this Shell page. To go back to editing your program, press ctrl+left arrow. Notice on the TI-Innovator™ Hub that the red LED is still on.
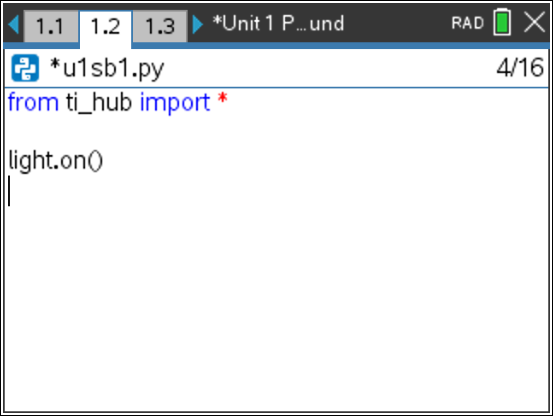
Step 8
-
Can you guess what command turns the light off? You can find it on:
menu > TI Hub > Hub Built-in Devices > Light Output > …
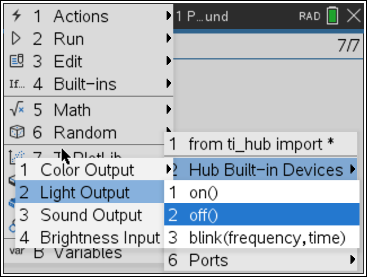
Step 9
-
Add the light.off() command after the light.on() command. It is okay to skip lines in the Python Editor. They have no effect on the execution and they make the code easier to read. Run the program again. Do you see the LED blink quickly? Too fast?
In the next few steps, we add a feature that lets you control just how long the LED stays lit.
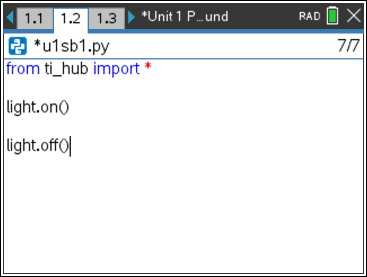
Step 10
-
Place your cursor on the line below from ti_hub import *.
Press menu > More Modules > Time and select:
from time import *
Between the light.on() and light.off() statements add the statement
sleep(seconds)
found on the Time menu as well.
The word seconds is a placeholder.
Replace it with a number, like 2 or 3.
The sleep() function tells the computer to wait or pause for that many seconds before going to the next statement in the program.
When you run the program now (press ctrl+R), the LED will stay lit for your chosen number of seconds before turning off.
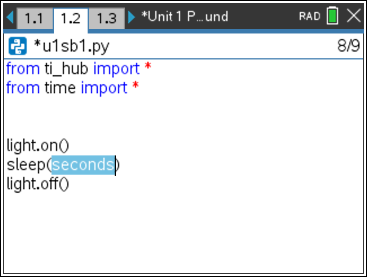
Step 11
-
To make the light blink, you could repeat the sequence of statements in the program or …
on the Light Output menu there is also a blink() function that causes the LED to blink. light.blink() has two parameters: frequency and time. Replace both with numbers and figure out the pattern. Pay attention to the pop-up tool tip!
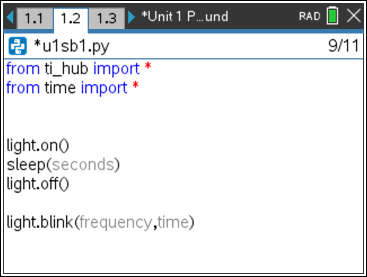
Skill Builder 2: Color and the Hub Project
Download Teacher/Student DocsIn this lesson, you will learn about controlling the color LED on the TI-Innovator™ Hub using a Python program template.
Objectives:
- Use a Python project template
- Control the color LED on the TI-Innovator™ Hub
Step 1
The color LED has three color channels: red, green, and blue. This is often referred to as an “RGB LED.” Computer screens, phone screens and TV screens all use many of these LEDs to create images.
To get a unique color, mix the correct amounts of red, green and blue. Many colors are possible with the right mix of these three primary colors of light.
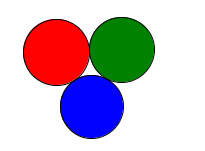
Step 2
-
Start a new TI-Nspire™ Document using home > New or insert a new page in a document using ctrl+doc (+ page).
Select Add Python > New….
Type a name for the Python program (we used U1SB2). Do not press enter yet! From the Type: dropdown list (click the arrow at the right of the field), select Hub Project. Press enter.
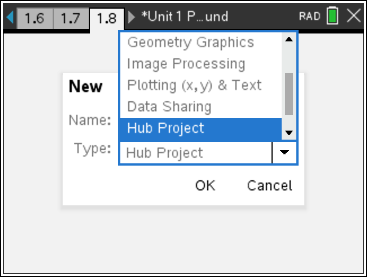
Step 3
- The "Hub Project" template provides several import statements at the top of the Python Editor. There are also some #comment statements. The # sign (number, hash, pound, octothorpe) indicates the start of a comment and these comments are ignored when running the program. Comments are used to add personal notes to a program.
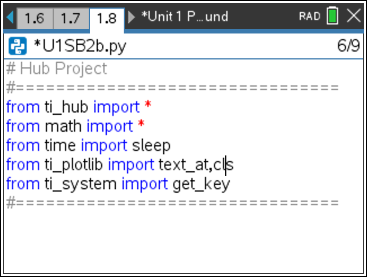
Step 4
- The import statements provide several tools that will come in handy when working with the TI-Innovator™ Hub. We will not address all these tools in this lesson, but they will be used in later lessons. In the first lesson you used the ti_hub module and the time module (for the sleep() function) by selecting those import statements from different menus. The template gathers several useful tools together for you. There are several other templates available for various types of Python programming projects on the TI-Nspire™ CX II graphing calculator.
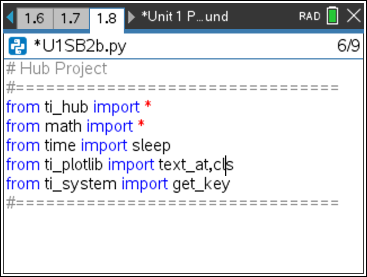
Step 5
-
Place your cursor at the bottom of the program (below the bottom most #===== comment line).
Press menu > TI Hub > Hub Built-in Devices > Color Output. Select rgb(red,green,blue) which places the statement
color.rgb(red, green, blue)
into your program. The three inline prompts (red, green and blue) must be replaced with numbers, each between 0 and 255 as the hovering tool tip indicates. Choose three numbers, pressing tab or the right arrow to go from field to field.
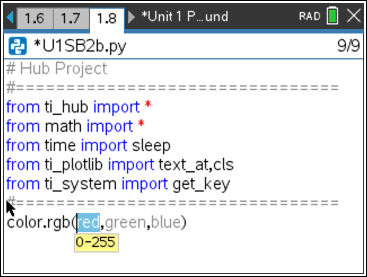
Step 6
- Run your program. The Shell app appears on the screen and the color LED will light up on the TI-Innovator™ Hub. Now go back one page (ctrl+left arrow) in the document to the Python Editor and try other numbers for the three color channels.
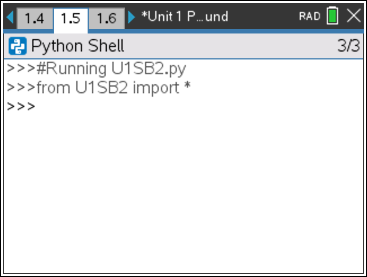
Step 7
-
Two other functions on the Color Output menu are
blink(frequency, time)
and
off().
Can you guess what they do? Try adding them to the program to test your guess.
Remember to save your work.
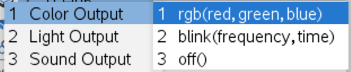
Skill Builder 3: Input and Sound
Download Teacher/Student DocsIn this lesson, you will learn about two ways to make sound on the TI-Innovator™ Hub and use variables to store and retrieve values.
Objectives:
- Produce a sound on the TI-Innovator™ Hub
- Use variables to store entered values
- Use the input() function and the int() function
- Play musical notes
Step 1
The TI-Innovator™ Hub houses a small speaker, called SOUND. The speaker is on the bottom of the hub. There is no amplifier, so the volume is very low. It is designed that way so that your classroom does not get too noisy.
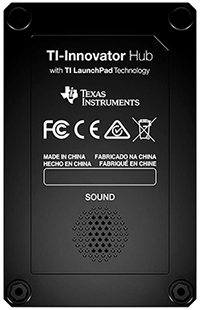
Step 2
-
Start a new Python project using the "Hub Project" template.
You can either start a new document (home > New) or add a page to your current document (ctrl+doc). Select Add Python > New…. Be sure to select the Hub Project template.
Note: Python is case sensitive — x and X represent two different things. All keywords are lowercase. Using a capital letter in a keyword like ‘If’ results in an error.
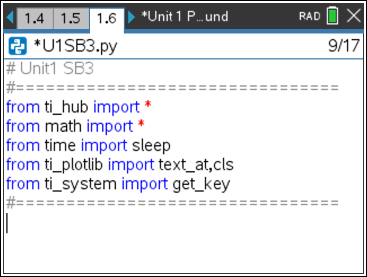
Step 3
-
You will write two input statements to get values to create a sound. These values will be stored in two variables, f and t. You will write two statements like this:
f = input(“Frequency? “)
t = input(“Time? “)We will do this step-by-step.
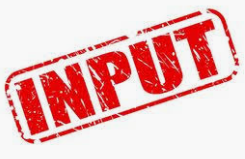
Step 4
-
Type the letter f and the = sign on a blank line below the bottom comment line #=============.
f =
Leave your cursor to the right of the = sign for the next step.
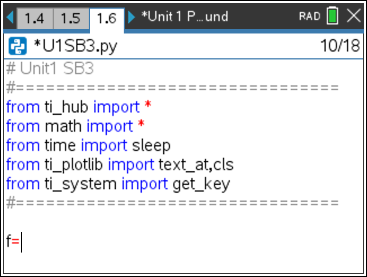
Step 5
- Now use the input() function from menu > Built-ins > I/O.
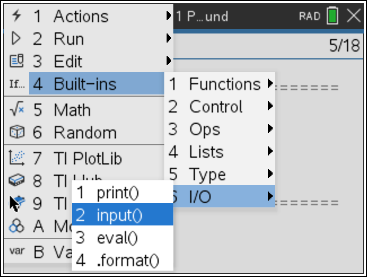
Step 6
-
Selecting input() from the menu pastes the word input() into your program after the = sign. Inside the parentheses, type the “prompt” for this input statement.
On the handheld, the quotation mark is ctrl+x (the multiplication key). For a capital letter use the shift key. Locate the question mark (?) on the punctuation key next to the letter "g" on the keypad. Be sure to use the closing quote, as well.
On the next line, build another input statement for the variable t.
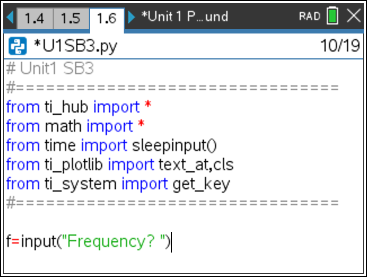
Step 7
-
Below the two input statements, get the sound.tone() function from
menu > TI Hub > Hub Built-in Devices > Sound Output
You will see the statement
sound.tone(frequency, time)
added to your program.
In place of frequency, type the variable f. In place of time, type the variable t.
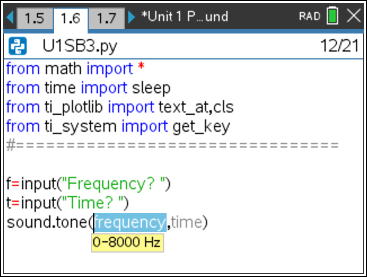
Step 8
-
Press ctrl+R to run the program. At the prompts, enter 440 for Frequency and 2 for Time.
And then … oops! A "runtime error!" This error occurs because the input function gives a value that the sound function cannot use; it is a string (“440” and “2”) rather than the numeric values 440 and 2.
You need to convert each string value to a numeric value using either float() (for a decimal number) or int() (for an integer).
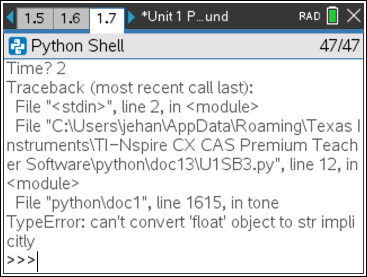
Step 9
-
Back in your program, add the int() function in front of each input function. You can type it in or find the int() function on
Menu > Built-ins > Type.
f = int( input( “Frequency? “) )
t = int( input( “Time? “) )Notice the special placement of all the parentheses! You will have to move one parenthesis around. Notice that there are two right parentheses at the end of each statement.
Run the program again and enter 440 and 2, you will hear a tone of 440 Hz for two seconds from the speaker on the bottom of the TI-Innovator™ Hub.. Press ctrl+R to rerun the program in the Shell to try other frequencies (between 0 and 8000 Hz). Can you hear them all?
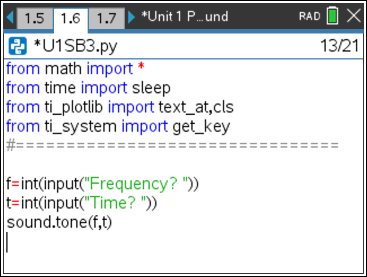
Step 10
-
Play Notes
The sound device can also play musical notes using their names:
sound.note( “A4”,2)
plays the note A in the fourth octave.
Change the first input function to request a note rather than a frequency. Since the note is in quotes (a string), you do not need the int() function.
Change the sound command from tone to note by retyping it or by using the menu. When you run the program, enter a note like A4 (without quotes).
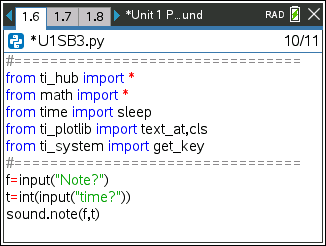
Application: The Traffic Signals
Download Teacher/Student DocsIn this application you will write a program to simulate a traffic signal: both lights and sounds (for the blind).
Objectives:
- Control the timing of the red, yellow and green states of a traffic signal
- Combine light, color and sound into one project
- Use a while loop to repeat a procedure until esc is pressed
Step 1
A traffic light has three separate bulbs in red, yellow and green so that colorblind people can tell the state of the light just by the position of the lit bulb.
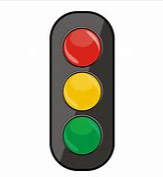
Step 2
On the side of the road there is also an audio signal to let blind pedestrians know when it is safe to cross the street. You will make the sound as part of your traffic signal system.

Step 3
-
Write a program to input the number of seconds the traffic light is red, yellow and green. When the traffic light is red, both the color LED and the red LED should be on. When the traffic light is yellow or green, only the color LED should be on. When the traffic light is green, there should also be an audio signal indicating that it is safe to go.
Start with a new Python TI-Innovator™ Hub project. Ours is called u1app.
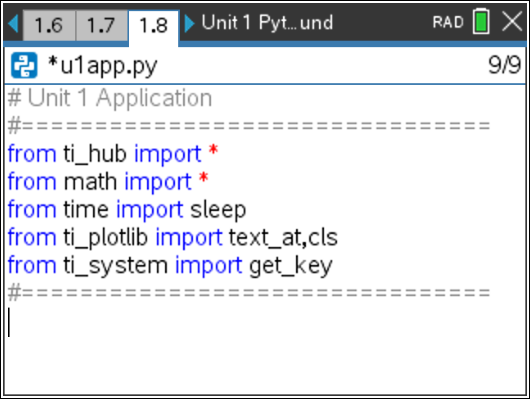
Step 4
-
Add three input() statements for the times (in seconds) that the light is red, yellow and green. For simplicity, we use only whole numbers so remember to use the int() function around the input() function to convert the entered string into a number.
The screenshot only shows one sample statement.
Note the capital "O" in redOn. Python is case-sensitive, so all references to this variable must be written the exact same way.
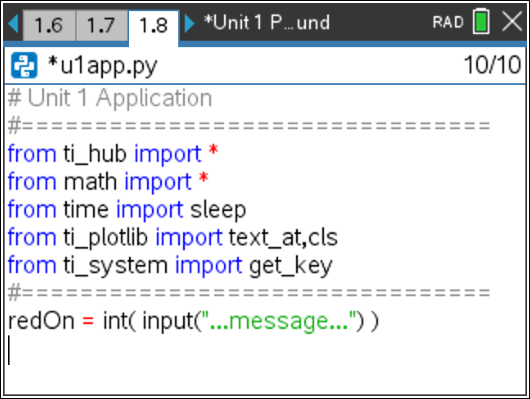
Step 5
-
Start with the red light. Turn both the color LED red and turn the light (red LED) on, too.
Use the sleep() function to pause the program for the redOn number of seconds using the statement:
sleep(redOn)
Be sure to turn the light off when the color changes to yellow.
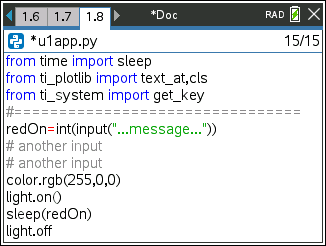
Step 6
-
Turn the color LED yellow for the yellow signal and then green for the green signal.
While the green light is lit, also make a sound (use either .tone or .note) using the speaker. The sound should stay on as long as the green light is lit and then turn off.
Run your program to test it. Watch the lights on the TI-Innovator™ Hub.
Step 7
-
If your program worked properly, it ended after one cycle through the three colors. To allow the program to cycle though the three colors repeatedly, you will add a loop to your code.
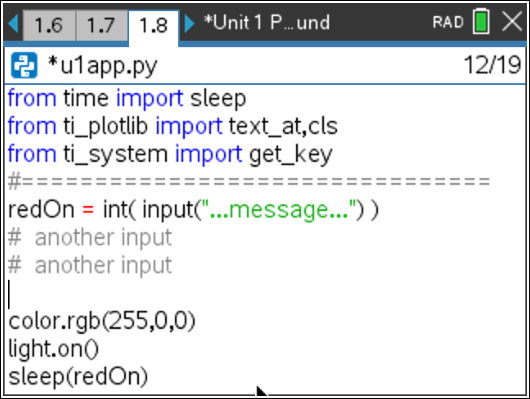
Step 8
-
A loop is a programming control structure that processes a block of code over and over. One type of Python loop is the while <condition>: loop. The <condition> is a Boolean expression, one that evaluates to either True or False.
An example: while x<10:
block(the colon (:) is required)
(block is intentionally indented)As long as x is less than 10 the statements in the block will be executed repeatedly. When x is greater than or equal to 10, the loop will end, and processing passes to the first statement below the block.
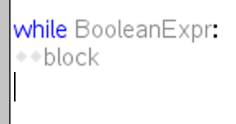
Step 9
-
Insert a blank line in your program below the three input statements.
Then get the statement
while get_key() != ”esc”:
from menu > TI Hub > Commands.
Notice that the light gray word block is indented two spaces and that these spaces have light gray diamond placeholders (they really are just spaces).
!= is the Python symbol for "does not equal."
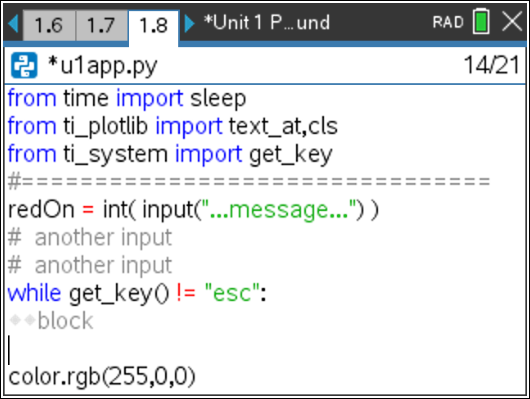
Step 10
-
The while loop executes all the statements in its block over and over until the esc key is pressed. The block is defined by the indent (two spaces).
Delete the word block (an inline prompt) and then indent the rest of your program two spaces. You could type two spaces at the beginning of each line, press tab at the beginning of each line, or take this shortcut:
Select all the statements below the while statement (using shift+down arrow) and then press the tab key. This indents each selected line.
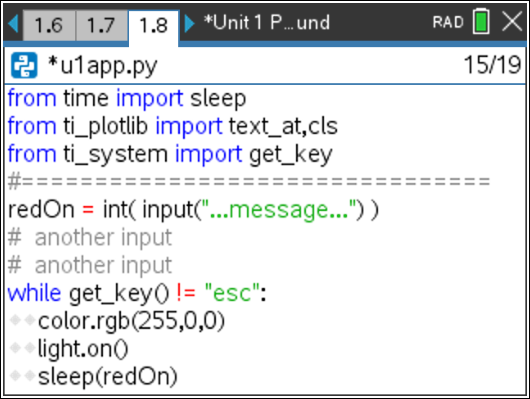
Step 11
-
Check to make sure that all your color, light and sound statements are indented the same number of spaces (two). This makes all your light code become the while block.
Caution: Improper indenting can lead to errors.
Run your program again. Press esc when you are ready to stop the program. There may be a delay in stopping the program because it must go through all the block code and stops the loop only after the green light finishes.
When the program ends, what is the state of the LEDs on the TI-Innovator™ Hub? How would you make sure that they are both turned off?
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 2: for loops With the TI-Innovator™ Hub
Skill Builder 1: Looping the Light
Download Teacher/Student DocsIn this lesson, you will be introduced to the concept of a for loop in the context of the TI-Innovator™ Hub.
Objectives:
- Make a for loop using the range() function
- Input decimal values using float()
- Create a custom blinking light
Step 1
To loop through a set of code a specified number of times, we can use a for loop with a range() function.
The range() function returns a sequence of numbers, starting from 0 by default, and increments by 1 (by default), and ends at a specified number.
The function light.blink() does not give you enough control over the blink cycle. How long is the light on and how long is it off between blinks? Can you have it blink three times in 10 seconds?
This lesson will develop a program that gives you this control and also direct control over the total number of blinks.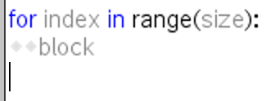
Step 2
-
Start with a New Python Hub Project template.
Write three input statements:
- One for the total number of blinks
- One for the onTime (the time that the LED is on during a blink)
- One for the offTime (the time between blinks)
Caution: You may want to have blink times that are not whole numbers. Rather than using int() around the input() function, you can use float(). This allows you to enter numbers with decimals.
float() and int() are found on menu > Built-ins > Type (along with others).
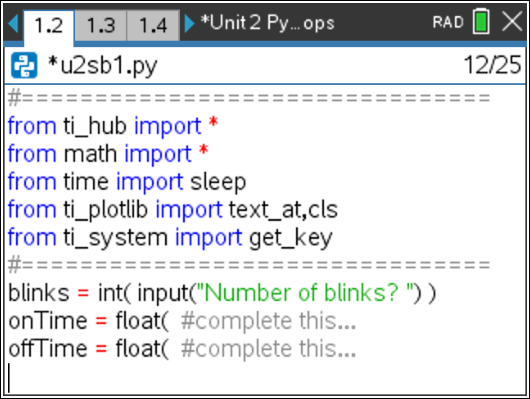
Step 3
-
Insert the statement
for index in range(size):
blockselected from the menu > Built-ins > Control.
The inline prompts "index", "size", and "block" must be replaced next.
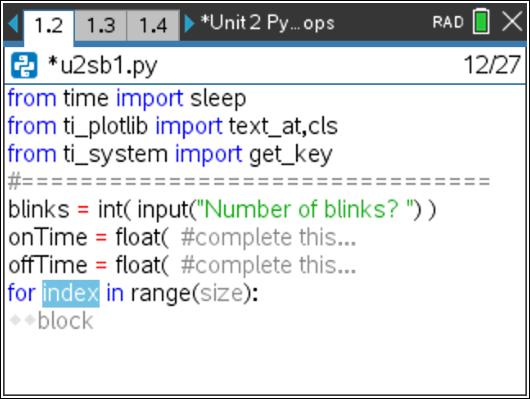
Step 4
-
Replace "index" with any variable (i is commonly used). Press the tab key to move to the next field.
Replace "size" with the variable blinks that was used in the first input statement. Press tab again to highlight "block."
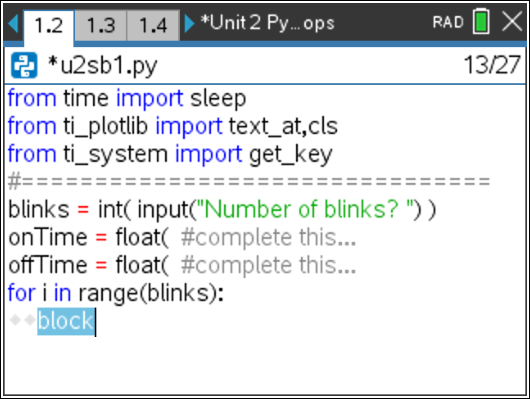
Step 5
-
The for loop "block" is the set of statements that operate the light (the red LED on the TI-Innovator™ Hub). Use light.on(), light.off() and sleep() statements. Try it yourself now. Remember to use the variables for timing that you used in the input statements.
You can use decimal values for all the input statements, but the number of blinks will be converted to an integer. You cannot make half of a blink!
The next step shows the completed program.
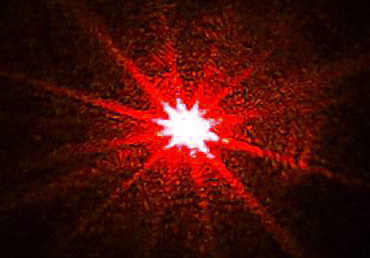
Step 6
-
Your program should resemble this:
blinks = int( input("Number of blinks? ") )
onTime = float( #complete this...
offTime = float( #complete this...
for i in range(blinks):
light.on()
sleep(onTime)
light.off()
sleep(offTime)
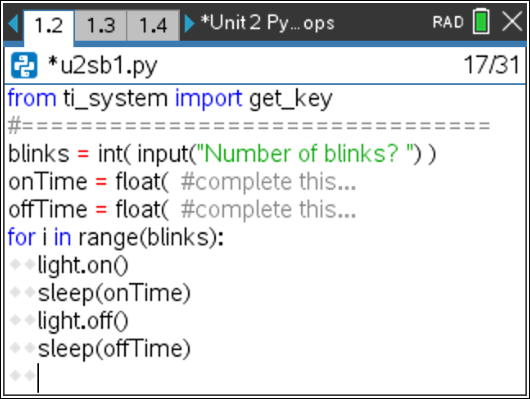
Step 7
- When you run the program, enter your values, and then watch the LED. When the program ends, is the light on or off?
The LED blinks but nothing happens on the TI-Nspire™ CX II graphing calculator screen. Add a print statement inside the for loop to display the current blink number:
print(i)
print() is found on menu > Built-ins > I/O.
Running the program now shows the value of the index variable i on the Shell screen as the program makes the LED blink to your specifications. Do you notice anything unusual about the numbers on the screen?
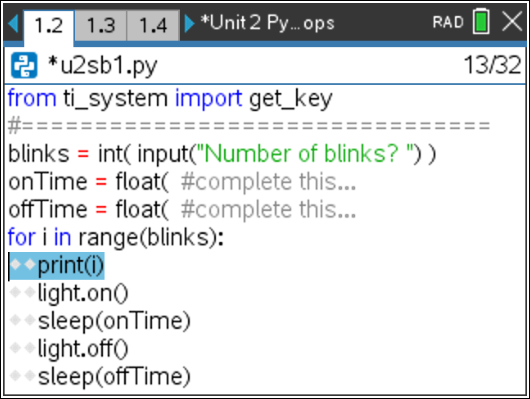
Step 8
-
Instead of the standard Python print() function, you could use a special Texas Instruments developed text_at() function that the Hub Project template imports. See the import statements at the top of your program.
From menu > TI Hub > Commands, select text_at(…).
text_at( row , "text" , "align" )
Use row 6.
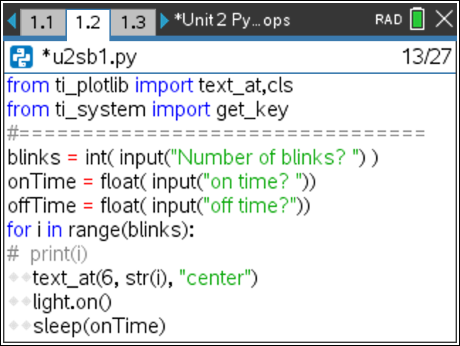
Step 9
-
In place of "text," use the expression str(i) which is found on
Menu > Built-ins > Type and converts the integer value of i into a string.From "align," select one of the three pop-up choices: left, center or right.
On the print(i) statement, press ctrl+t to turn it into a comment.
Your code should resemble this:
# print(i)
text_at(6, str(i), “center”)
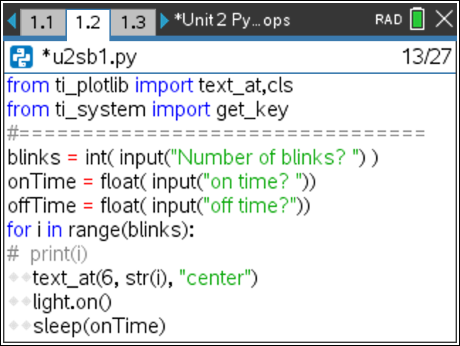
Step 10
Run the program again. What values do you need to enter to get the light to blink three times in 10 seconds? Try this:
blinks = 3
onTime = 2
offTime = 2
The third blink ends at the start of second 10 but the program does not end for another two seconds. Are there other options?
Remember to save your work.
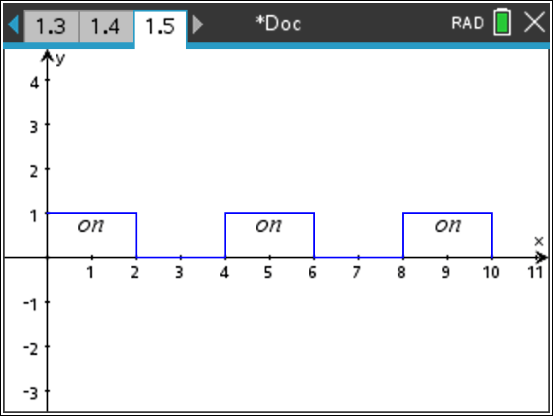
Skill Builder 2: Loop Through Color
Download Teacher/Student DocsIn this lesson, you will learn about color mixing to make a variety of colors on the color LED using several for loops.
Objectives:
- Use the for loop with the range() function
- Control the many colors possible on the color LED
- Use copy/paste/edit for simpler coding of longer programs
Step 1
All the colors of the rainbow (and more) are possible using the color LED on the TI-Innovator™ Hub. This lesson builds a program that will change the color LED to many different colors using for loops. You will get some practice with the different loop options.
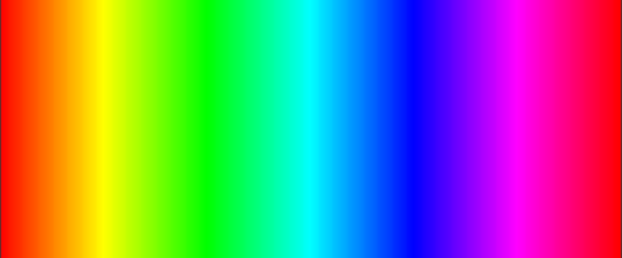
Step 2
-
Start a new Python Hub Project.
This program will gradually increase and decrease the LED’s color channels, one at a time, to mix some colors.
Write two input statements to enter a step value and a delay value. The step value can be an int() but the delay should be a float() since it may need to be between 0 and 1 (a decimal value).
step is the space between color values and delay is the time delay for each color step.
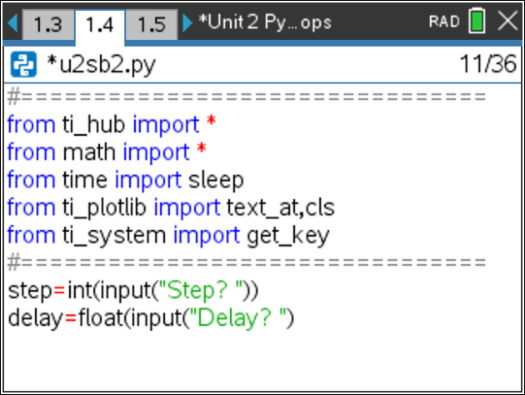
Step 3
Press menu > Built-ins > Control and notice the for index in… section of the menu (shown at the right).
All these statements use the range() function but have different arguments.
The most versatile of the three is:
for index in range(start, stop, step):
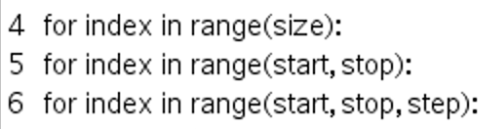
Step 4
-
Select the statement
for index in range(start, stop, step):
and change index to the variable i. Enter 0 for start, 256 for stop, and step for step. (You must type the word "step" since this is the variable used in the input statement.)
This makes the loop go from 0 up to at most 255 by adding step in each step of the loop. For example, if step is 10 the values of i will be
0, 10, 20, 30, … up to?
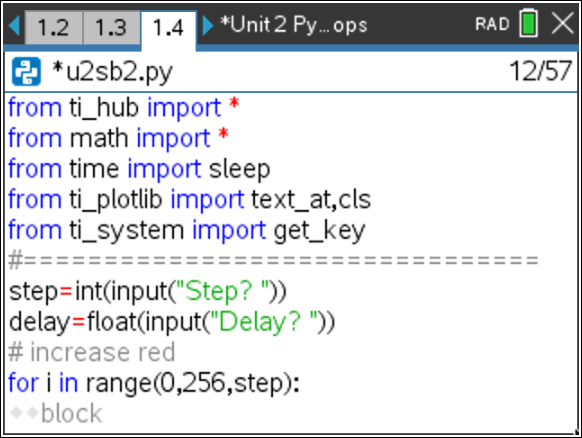
Step 5
-
For the loop block use
color.rgb(i, 0, 0) (red only)
found on menu > TI Hub > Hub Built in Devices > Color Output.
Press ctrl+R to run the program. Notice that the LED lights up immediately. It is supposed to light up slowly. You need to slow the process down a bit using your delay variable. Try it yourself before going to the next step.
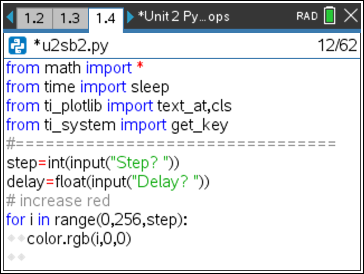
Step 6
Did you add a sleep(delay) statement?
Now the LED gradually adds red. Next write another for loop to gradually add green to the red LED.
You can copy the red for loop and paste it below the loop and then edit the text to control only the green channel.
Be careful about the indenting.
Make sure the red channel stays fully lit the whole time.
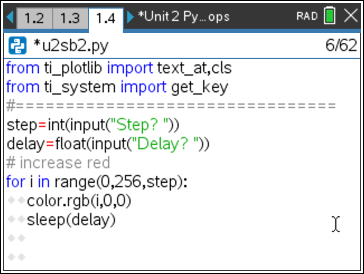
Step 7
Here’s the green loop:
for i in range(0, 256, step):
color.rgb(255, i, 0)
sleep(delay)Note that the red channel is set to 255 and the loop variable is now in the green position.
Run the program again. What color do you see at the end of the program now?
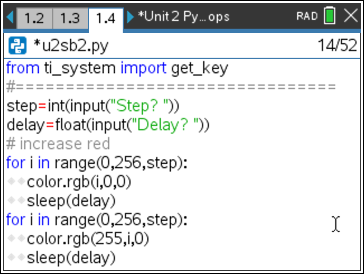
Step 8
-
Now for the tricky part … gradually remove the redness by changing the "direction" of the for loop:
for i in range(255, 0, -step):
This loop starts at i=255 and the loop index i decreases by the step value.
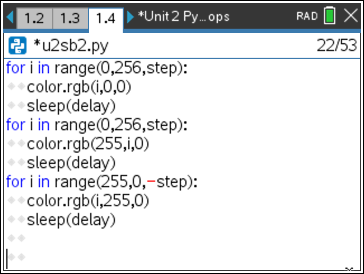
Step 9
-
It might help to keep things organized by adding # comments before each for statement to explain what it does more clearly. Comments begin with the # symbol (press ctrl+T) and are ignored when the program is run.
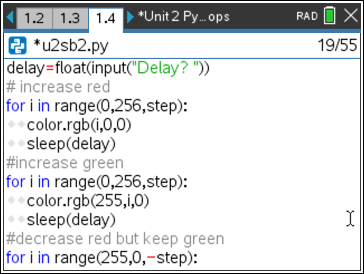
Step 10
-
To complete this project, add loops to:
- Increase blue
- Decrease green but keep blue
- Increase red (again)
- Decrease blue but keep red
- Decrease red to turn it off slowly
This process mixes all three pairs of colors: red-green, green-blue and blue-red. In the process you should also see yellow, cyan and magenta appear.
At the end of the program, the LED should be off.
Remember to save your work.
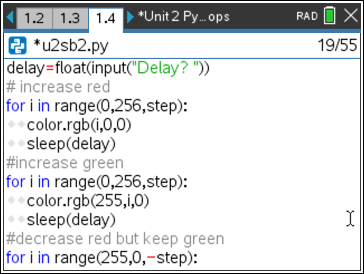
Skill Builder 3: Looping with Sound
Download Teacher/Student DocsIn this lesson, you will learn to play a song on the TI-Innovator™ Hub.
Objectives:
- Use a for loop with specific frequencies
- Use a loop with a list of musical notes
- Write a program to play a song
Step 1
A Little Music Theory
Musical notes are determined by the frequency of a vibrating object such as a speaker, drumhead, or string as in a guitar or piano. The notes of the musical scale have a special mathematical relationship. There are 12 steps in an octave. If a note has frequency F, then the very next note has frequency F × 12√2.
Multiplying a note’s frequency by 12√2 or 21/12 (the twelfth root of 2) twelve times results in a doubling of the original frequency, so the last note in the octave (or the first note in the next octave) has frequency of F ×(21/12)12 = 2 × F. For example, if a note has a frequency of 440 Hz, the same note an octave above it has a frequency of 880 Hz, and the same note an octave below it has a frequency of 220 Hz.
Step 2
The human ear tends to hear two notes that are an octave apart as being essentially "the same", due to closely related harmonics. For this reason, notes that are an octave apart are given the same name in the Western system of music notation - the name of the note one octave above C is also C. The 12 intervals between these notes are called ‘semitones’.
In this project we’ll take advantage of the 21/12 principle to generate the 12 notes in an octave (both naturals and sharps) plus the first note in the next octave.
Middle C (the lowest note in the staff pictured above) has a frequency of 261.64 Hz. An octave above Middle C, also known as Treble C, has a frequency 2 × 261.64 Hz or 523.28 Hz. There are 12 steps (semitones) between these two notes, and each step is 21/12 times the note before it.
Step 3
-
In the image to the right, enter 261.64 into a Calculator app. Then, in the next line, first press the multiplication key. The handheld supplies Ans at the beginning (not shown) because the multiplication symbol requires something in front of it.
We multiply Ans by 2^(1/12) and press enter. Then, press enter repeatedly to produce the sequence of answers shown.
We will incorporate this repetitive principle into our program. If you continue pressing enter, the twelfth answer will be 523.28, exactly two times the starting value, because F × (21/12)12 = 2 × F.
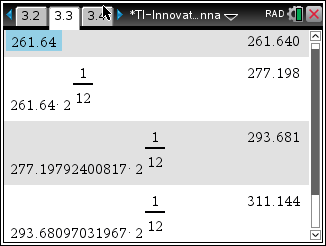
Step 4
-
Start a New Python Hub Project. Begin by assigning the number 261.64 to the variable freq.
freq = 261.64
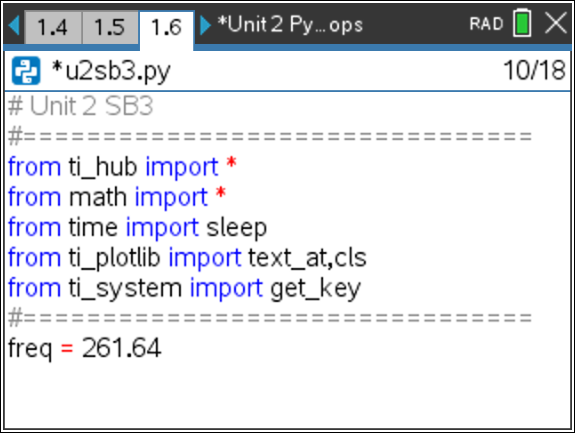
Step 5
-
Now write a for loop to do something 12 times:
for i in range(12):
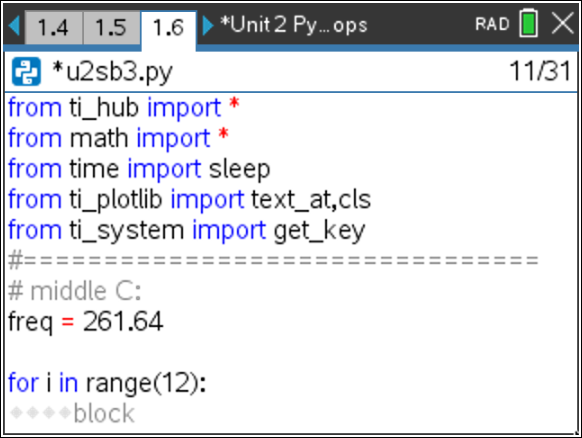
Step 6
In the for loop block do two things:
Play the frequency value on the speaker for one second:
sound.tone(freq,1)
Then, increase the frequency by a factor of 2**(1/12) (the twelfth root of 2).
freq = freq * 2 ** (1/12)
** is the python ‘raise to the power’ or ‘exponent’ operator.
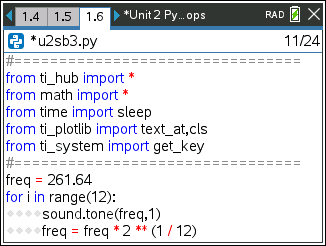
Step 7
-
If you run the program now (assuming there are no errors in your code), you will hear only the last tone. The program runs too fast to hear all the notes. The one second delay in the sound function takes place in the TI-Innovator™ Hub, not in the handheld. The program sends the next sound too quickly. Tell the program to ‘wait’ while each note is playing by adding a sleep(1) function to the loop after the sound is played.
You may also want to add a print() statement to see the frequencies being played at the same time they are playing.
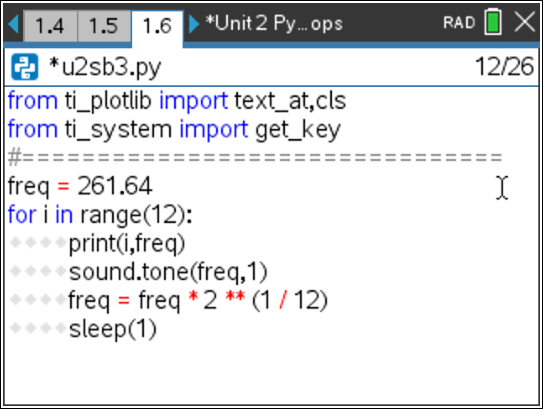
Step 8
There is another way to play musical notes on the TI-Innovator™ Hub. Add the following code to your program:
sleep(2)
for note in ["c4","d4","e4","f4","g4","a4","b4","c5"]:
sound.note(note,1)
sleep(1)Find the square brackets [ ] on the keypad by pressing ctrl+( to the left of the 0 key.
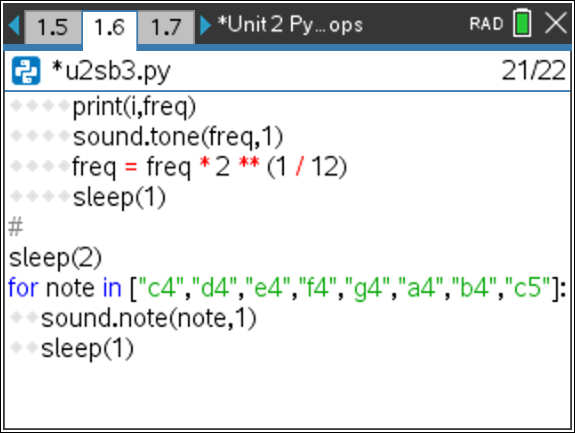
Step 9
Explanation:
sleep(2) is just a 2-second pause between the first part of the program and this second part.
for note in is the start of a typical for loop statement. You can get this form of the for statement from menu > Built-ins > Control > for index in list:.
["c4","d4","e4","f4","g4","a4","b4","c5"] is a list of musical notes, each in quotes (a string). “c4” is note C in the 4th octave (‘middle c’, which has frequency 261.64 Hz).sound.note(noteName ,time) This loop plays the familiar ‘do-re-mi-fa-sol-la-ti-do’ scale. It leaves out the sharps in the octave.
Can you modify the list to play a song?
Application: Computer Music
Download Teacher/Student DocsIn this application, you will learn to generate random computer music.
Objectives:
- Use the for loop to control the number of notes
- Use the random number generator to create random musical notes
Step 1
In this unit, you used for loops to control light, colors and sounds. In this application, you will create a program to play computer-generated random sound or music. This challenge can take three approaches: a) play purely random tones (frequencies); b) play random notes using their special frequencies; or c) play random notes using their names (in a list). You will also use random durations (timings) for each tone/note. And, for the icing on the cake, each note can create a different color using the color LED.
Step 2
-
Make a new Python Hub Project.
You will need a function that can deliver random numbers. This tool is part of the standard Python commands, but it is found in a separate module that the Hub Project template does not import.
Press menu > Random and add the statement
from random import *
to your collection of import statements at the top of your code.
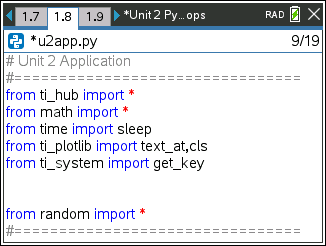
Step 3
-
Use the esc key to terminate the program.
Press menu > TI Hub > Commands and select the statement
while get_key() != “esc”:
Be sure to indent the rest of the statements.
When you run this program press the esc key to end the program.
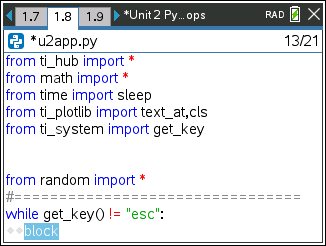
Step 4
Use the randint() function found in menu > Random:
r = randint(min, max)The variable r stores a random integer for later use. min and max will be replaced with numbers. But, before you enter those numbers, consider the next step.
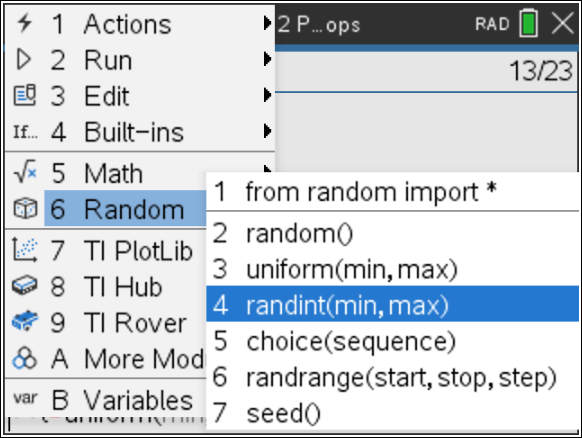
Step 5
-
r represents a sound frequency. Not all frequencies are "audible." Very small frequencies and very large frequencies should be avoided because they are outside our hearing range.
Recall that, when working with music in the previous lesson, you used frequencies in the hundreds, so when choosing min and max keep that in mind.
Add another random variable t (for time) and use the uniform() random number generator found on menu > Random > uniform(min, max). This returns a random decimal number between min and max so some notes will play for a part of a second. Your choice of min and max here will depend on how long you want each note to last.
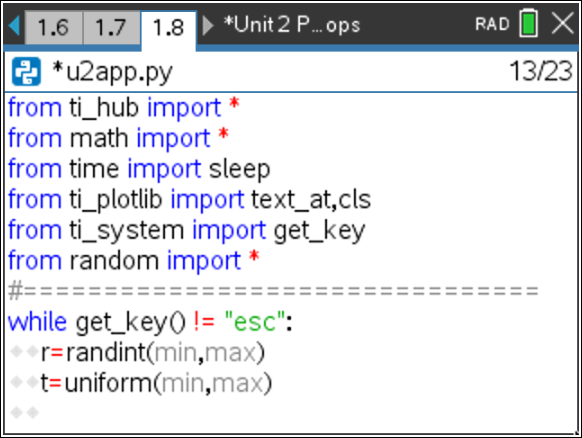
Step 6
-
Next, create the sound using sound.tone(), and replace the prompts frequency and time with your variables r and t, respectively.
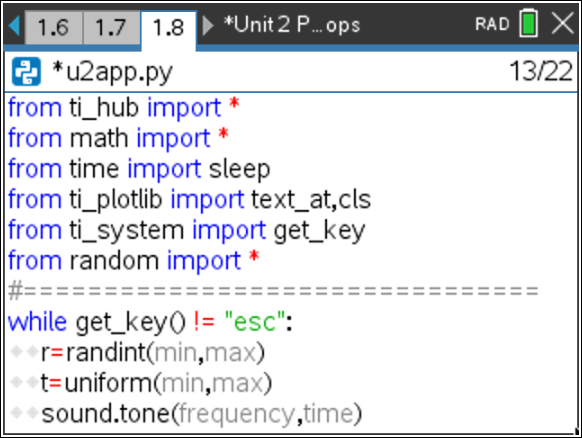
Step 7
-
Remember to add a sleep() function to pause the computer while the tone is playing. For how long should the program wait?
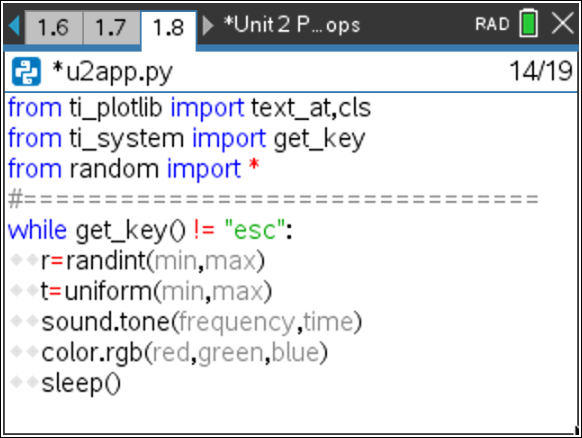
Step 8
-
How about the color LED? Remember that there are three color channels — red, green and blue — , that are limited to the values 0 to 255. You can have the LED light up in purely random colors using the randint(0,255) function for each channel
red = randint(0,255)
or you can make the color depend on the frequency r or the time t or both. Be careful about going "out of range" beyond 255.
The screen to the right is not the complete program!
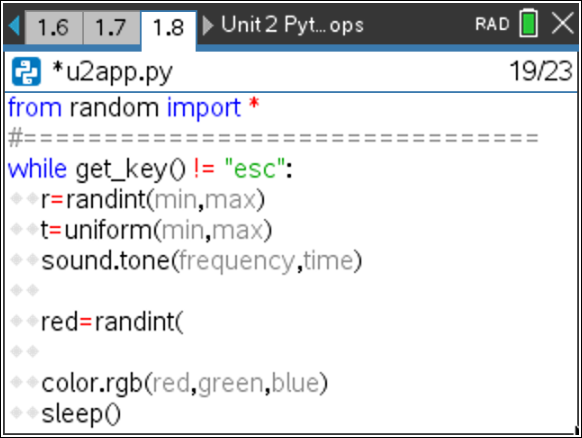
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 3: Brightness, if and while With the TI-Innovator™ Hub
Skill Builder 1: Measuring Light
Download Teacher/Student DocsIn this lesson, you will take control of the brightness sensor on the TI-Innovator™ Hub and learn how to make use of its information.
Objectives:
- Read the brightness sensor
- Set the range of the brightness sensor
- Monitor the brightness sensor
- Control a light with the brightness sensor
Step 1
Unlike the light, color, and sound features of the TI-Innovator™ Hub, the brightness sensor is an Input device rather than an Output device. A program can obtain information from the brightness sensor and take actions based on that numeric value. You can either work with the default brightness values or you can set the range of values with a special brightness.range() function.
The brightness sensor is clearly labeled on one end of the TI-Innovator™ Hub.
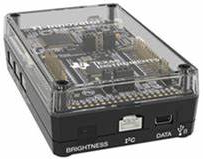
Step 2
-
Begin a new Python Hub Project, and start with the three statements
cls()
text_at(13,”Press [esc] to end”,”center”)
while get_key() != “esc”:
blockall found at menu > TI Hub > Commands.
cls() clears the screen.
text_at(13, …) prints the message at the bottom center of the screen.
When running the program, press esc to end.
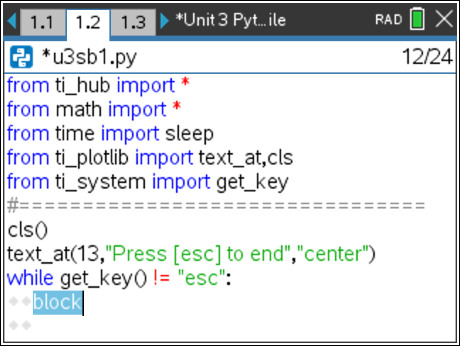
Step 3
-
In the while block, use two statements: one to read the brightness and one to display the value.
Assign b to read the brightness measurement
b = brightness.measurement()
found on menu > TI Hub > Hub Built-in Devices > Brightness Input
Add:
text_at(7, "brightness = " + str(b), "left")
text_at(7… is the vertical middle of the screen.
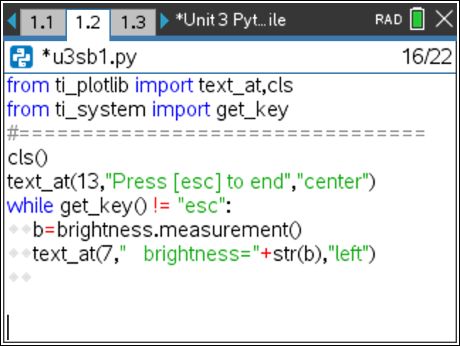
Step 4
-
About "brightness = " + str(b):
str(b) (found on menu > Built-ins > Type) converts the numeric value of b into a string because text_at() can only display text (characters), not values of numeric variables.
The + sign combines the word "brightness = " with the string value of b. This type of "addition of strings" is called concatenation.
Alignment “left” is better than “center” in this case. If you prefer to use “center,” then new data might not completely erase old data since the line will vary in length. You can move the text closer to the center by adding spaces before “ brightness = ” (inside the quotes).
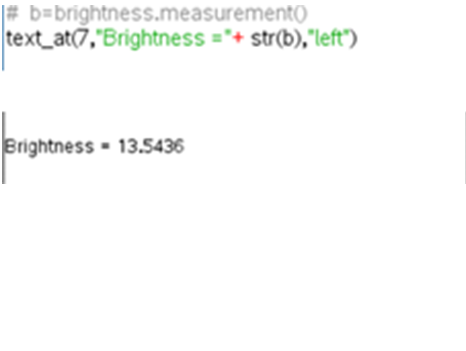
Step 5
-
Run the program now to see the effect on the screen. You should see something like this, and your brightness value will be changing.
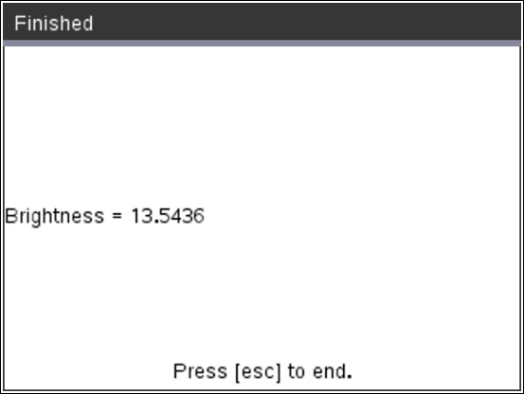
Step 6
-
Slow the display down a bit by adding a sleep() statement right after the text_at() statement. Be sure it is indented to match the other statements in the while block.
Run the program again and determine the lowest and highest values that the sensor currently delivers by changing the light intensity hitting the sensor.
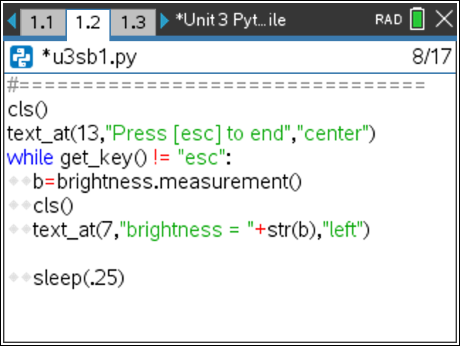
Step 7
-
You can set the range of values that the brightness sensor delivers using the statement
brightness.range(min, max)
found on menu > TI Hub > Hub Built-in Devices > Brightness Input.
Be sure to place this statement before the while loop. Use any values for min and max but be sure that min < max.
Change the range and run the program again to observe the values produced. You now have a custom digital light meter.
Why is this important? Check out the next few lessons.
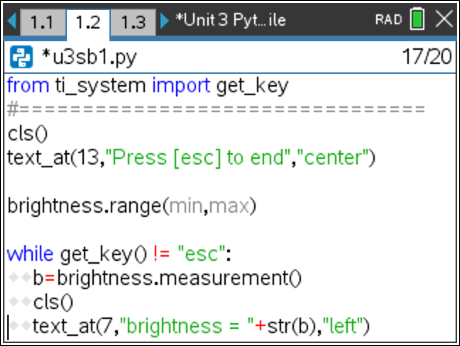
Skill Builder 2: Make a Light Switch
Download Teacher/Student DocsIn this lesson, you will learn to use the brightness sensor to control the light (the red LED) automatically.
Objectives:
- Set up and monitor the brightness sensor
- Introduce the if statements
- Control the TI-Innovator™ Hub light (the red LED) using the brightness sensor
Step 1
Now that you can monitor the light coming into the TI-Innovator™ Hub, use that information to cause the onboard light (the red LED) to switch on/off when the brightness value changes.
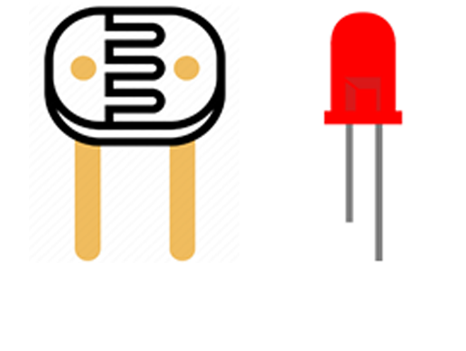
Step 2
-
Make a copy of the program you used in Skill Builder 1 of this unit.
To copy a program from the Editor, press menu > Actions > Create Copy….
If "Create Copy…" is not available, press ctrl+B in the Editor to store the program, and then try again. There should not be an asterisk (*) in front of the filename at the top.
The dialog box has a 1 added to your Python filename. If this is sufficient, press enter. Otherwise, change the name and press enter.
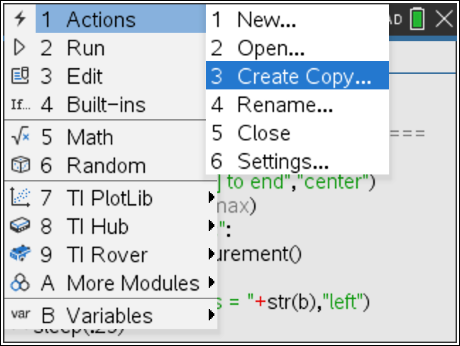
Step 3
-
Your TI-Nspire™ Document now has another Python Editor app with the copied program loaded on the page after the original program. Browse through the pages to confirm this, and make sure you return to the page with the copy of the program because we will make changes to this program.
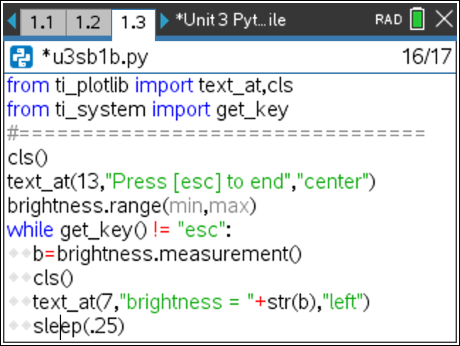
Step 4
-
The if statements:
On menu > Built-ins > Control, there are three if… statements shown at the right. Each version is used in different situations in programs and all of them depend on one or more logical conditions (expressions that are either True or False):
if <condition>: if <condition>: if <condition>:
block block block
else: elif <condition>:
block
else:
block
elif is Python’s version of "else if."
Pasting one of these structures into your program will display BooleanExpr into the <condition> field. It means the same thing.
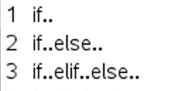
Step 5
-
Immediately after reading the brightness in your program, insert the
if..else structure from menu > Built-ins > Control.
The <condition> or BooleanExpr will depend on the variable b
if b > 25:
block
else:
blockBe sure to leave the colon (:) at the end of the if statement.
The value 25 is only a sample value. Change it depending on your lighting situation.
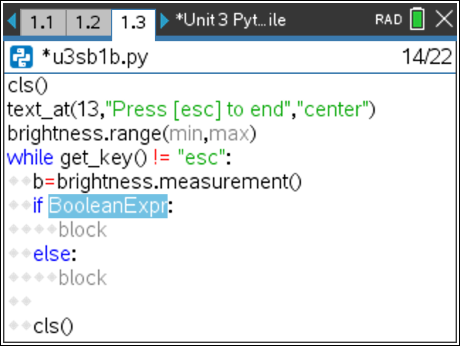
Step 6
-
Replace the two blocks: One will turn the light on and the other will turn it off. Study the logic of the structure to decide which action goes where. In the screen to the right, we leave the answer up to you. There are ??? in the screen image for you to replace.
Recall that you can find the light. statements on menu > TI Hub > Hub Built-in Devices > Light Output.
Run the program. Save your work!
You may have to adjust the threshold value of 25 in the if statement to suit your lighting situation and your brightness.range() setting.
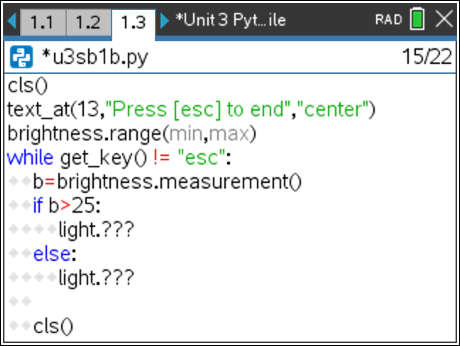
Skill Builder 3: Brightness and Color
Download Teacher/Student DocsIn this lesson, you will use the brightness sensor to control the color LED.
Objectives:
- Use brightness.range() to change the brightness scale
- Use the brightness value to light up the color LED
- Investigate numeric transformations
Step 1
Unlike the TI-Innovator™ Hub light (the red LED), the color LED can vary in brightness. You will now use the brightness sensor to control that LED.
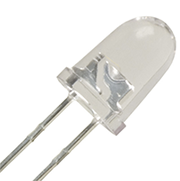
Step 2
-
Make another copy of your brightness metering program (or your brightness and light program from the last lesson — they are almost the same).
Since the three color channels of the color LED only permit values from 0 to 255, set the brightness range from 0 to 255.
brightness.range(0, 255)
The brightness value b can now be used as values for the three color channels … perhaps.
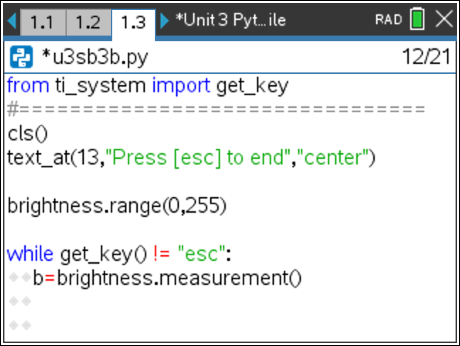
Step 3
Add a statement after the brightness.measurement() statement to light up the color LED using the variable b for all three channels:
color.rgb(b,b,b)
Run your program.
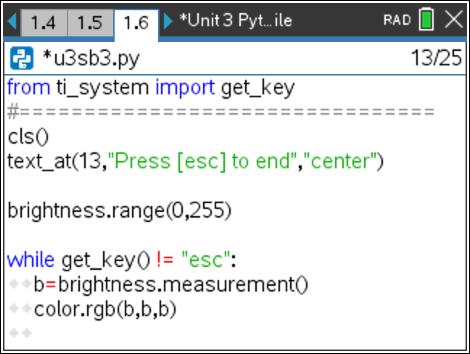
Step 4
-
Notice that the LED gets brighter as the light level increases. This is backwards! The darker the room, the brighter the light should be. Change the variable b (between the brightness.measurement() and the color.rgb()) to make the LED bright for low values and dim for large values. (See b=??? in the screen to the right.)
Try it yourself before proceeding to the next step.
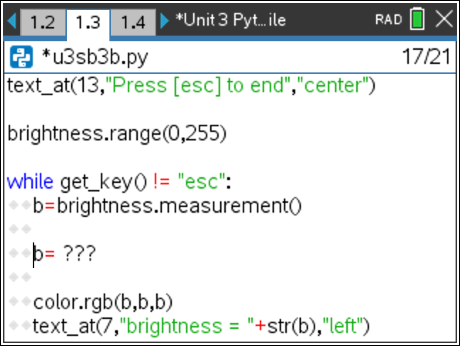
Step 5
Here’s one that works:
b=255 - b
When b is 0, the expression 255-b changes the value of b to 255; when b is 255, the expression 255-b changes the value of b to 0.
You may have to move your text_at() statement in the program to display the original value of b and not the transformed value.
Can you modify the program to produce other colors besides white?
When the program ends, the color LED may remain on. Add a statement at the end of the loop (not indented) to turn the color LED off.
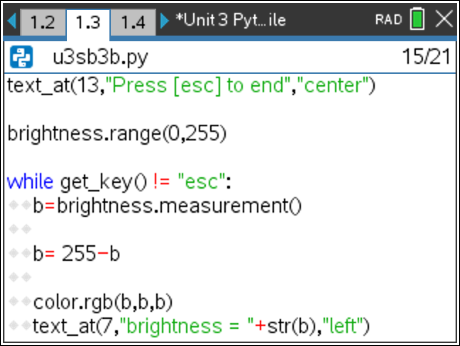
Application: Lite Music
Download Teacher/Student DocsIn this application, you will control sounds using the brightness sensor.
Objectives:
- Set the brightness.range() so that the value is suitable for making sounds
- Play sounds or musical notes by varying the brightness
Step 1
In an earlier lesson, you learned about sound.tone() and sound.note() using the TI-Innovator™ Hub. In this lesson, you will use the brightness sensor to create "noise" and "music" (sometimes it is hard to tell the difference).
There are three parts to this project:
- Light tones (frequencies)
- Notes using tones (frequencies of notes)
- Notes using a list of note “names”
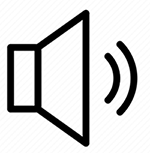
Step 2
Part 1: Light Tones
-
Again, use the original "brightness meter" program from the first lesson in this unit. Make another copy of the program using menu > Actions > Create Copy….
Next, you must decide what brightness.range() would be appropriate to use for sounds.
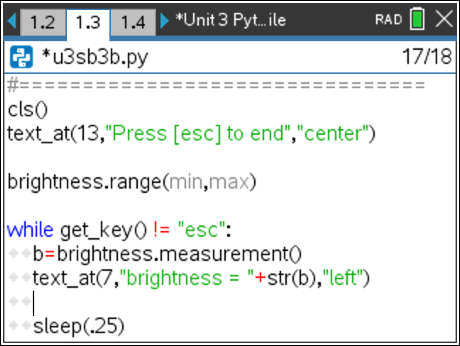
Step 3
-
For tones we can use any frequency between 0 and 8000 Hz, but many of those frequencies are too high or too low for humans to hear. Start with a range of (100,1000) and adjust to your liking.
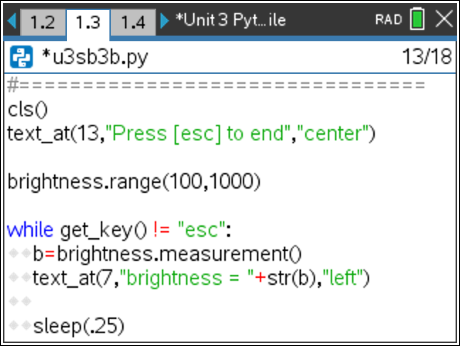
Step 4
-
Add the sound.tone() statement below the brightness.measurement statement and use the variable b for the frequency argument. Set the sound’s time to your preference and use the same value in the sleep() statement so that the TI-Innovator™ Hub and the handheld are in sync.
If you like, try making the sleep() value a little larger than the tone time value. This puts a little silent gap between sounds.
Test your program now and then adjust the numbers you used.
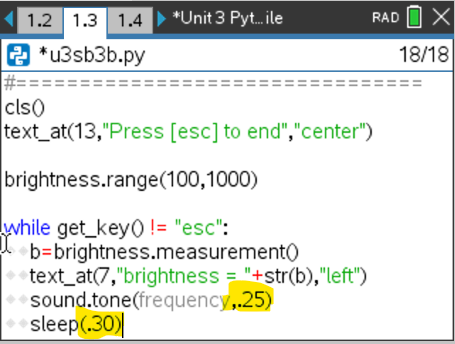
Step 5
Part 2: Musical Notes Using Frequencies
-
In the five octaves pictured, there are a total of 60 notes (12 per octave).
Note A1 (A in the first octave) has frequency 55 Hz.
Subsequent notes have frequency 55 * 2 ** (k/12), where k is the note number after A1. A1 is note number zero: k=0 → 2**(0/12) = 1.
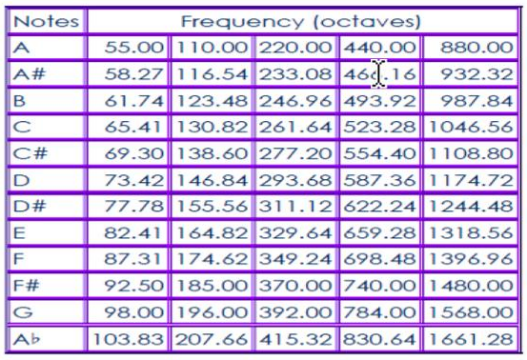
Step 6
-
To play "notes," modify your program.
Change the brightness.range() to be 0…59.
brightness.measurement() produces a decimal but we only want integers so convert b to an integer using b = int(b).
int() is found on menu > Built-ins > Type.
Calculate a note’s frequency using f = 55*2**(b/12).
Use the variable f in the sound.tone() statement for frequency.
Try the program again. Some notes might be too high or too low. What can you do to limit the range of the notes?
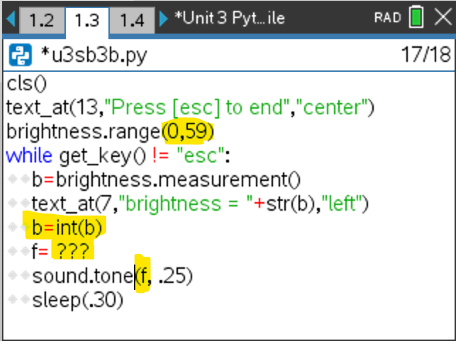
Step 7
Part 3: Notes Using a List
-
Recall that the sound object can also use note “names”.
At the top of your program (before the while loop), make a list of note “names” as you did in an earlier lesson:
notes = [“C5”, ”D5”, ”E5”, … ]
Note names are any of the letters ABCDEFG followed by a number from 12345.
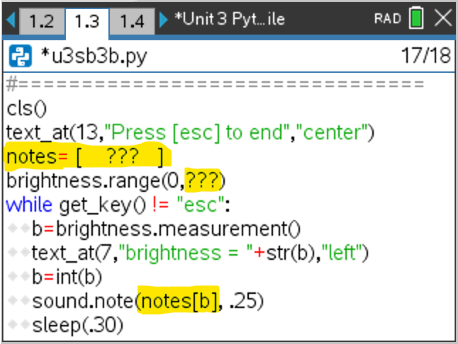
Step 8
-
Set the brightness.range() to be (0, # of notes in your list -1).
Convert the variable b to an integer.
Use the variable b as the index of the notes list:
sound.note(notes[b], .25)
Run the program now.
This program plays the notes in the list with low brightness using the beginning notes and high brightness using the notes from the end of the list.
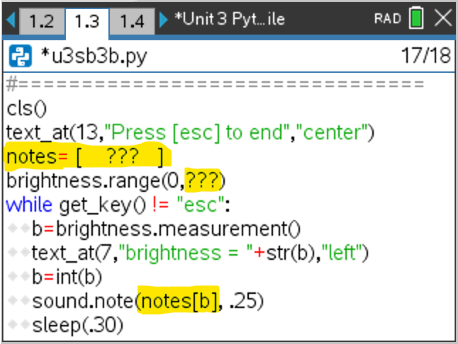
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 4: Rover’s Driving Features
Skill Builder 1: Make Rover Move!
Download Teacher/Student DocsIn this lesson, you will begin to operate the TI-Innovator™ Rover robotic vehicle by making Rover move and turn.
Objectives:
- Make Rover move
- Make Rover drive in a pattern
Step 1
Python’s TI-Innovator™ Rover commands all begin with rv. This syntax is a result of the way that the ti_rover module is imported into your programs (import ti_rover as rv). The same is true of the ti_plotlib module. This technique is called "aliasing" the module name (replacing it with a shorter name).
When you write a program that operates Rover, you are controlling the vehicle through easy-to-use commands but there’s a lot going on behind the scenes just like other TI-Innovator™ Hub commands.
Be sure your TI-Nspire™ CX II graphing calculator is attached and connected to Rover and that Rover’s power is turned on.
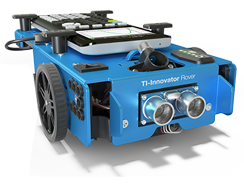
Step 2
-
Begin a new Python program and select the Rover Coding template from the Type: dropdown after entering the name of the program.
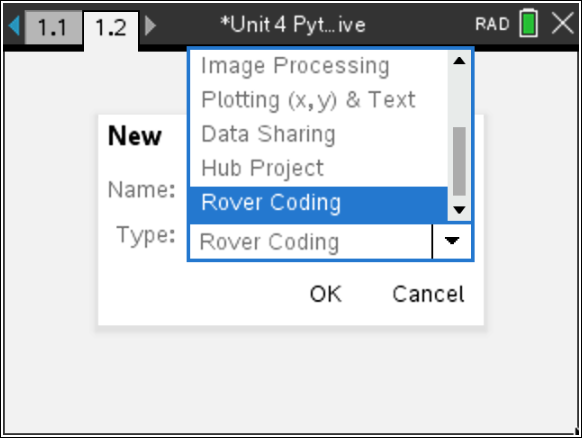
Step 3
-
The template contains many import statements to give easy access to several useful functions.
The most important one right now is the first statement:
import ti_rover as rv
This form of the import statement indicates that you must use rv. when using any of the methods in this module. When you choose the Rover functions from the menu, the rv. is automatically included.
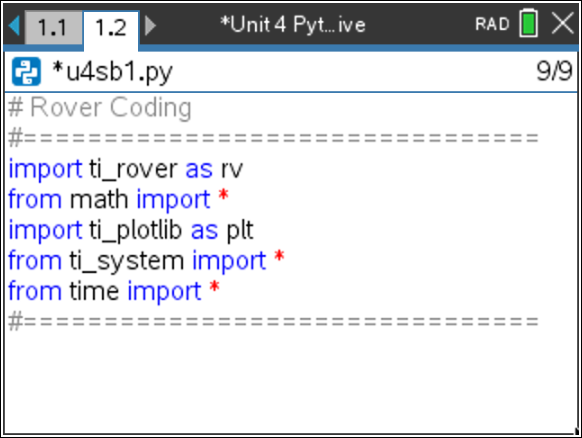
Step 4
-
To make Rover go forward use menu > TI Rover > Drive to get this statement:
rv.forward(distance)
Notice that the menu does not include rv. in front of forward but rv. is pasted into your program. This is done to save space on the menu.
The distance prompt is measured in "grid units" and you will be able to determine what that means in a moment. Use the number 1 as the distance and run the program. If Rover moves, hooray!
But … how far did Rover move?
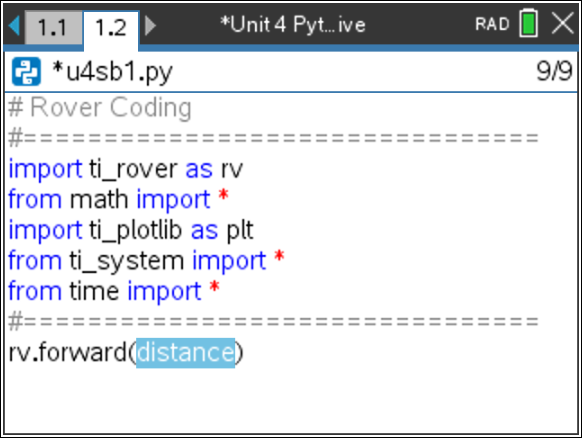
Step 5
-
Try the basic drive commands: forward, backward, left and right.
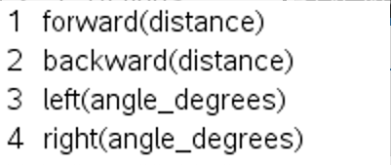
Step 6
-
To drive a square:
Erase the drive commands you entered (or make them comments).
Use a for loop to make Rover drive in a square pattern.
Begin with:
for index in range(size):
Use an appropriate index and size to make a square and add just two statements in the loop block. Try it yourself and run the program.
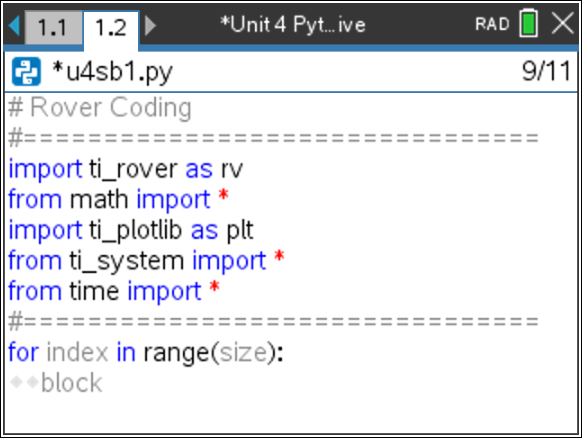
Step 7
-
Did you write something like this?
Congratulations! Remember to save your work.
What other patterns can you make?
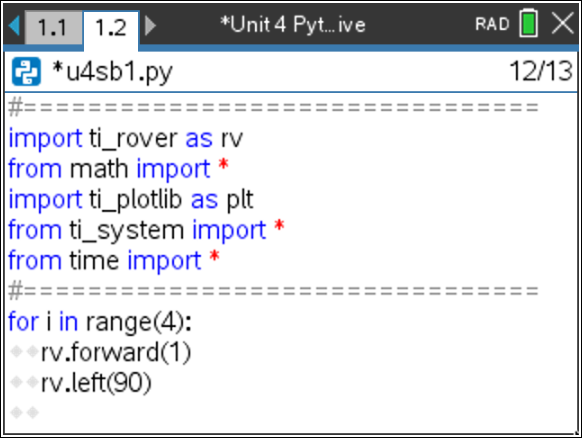
Skill Builder 2: Light at the Corners
Download Teacher/Student DocsIn this lesson, you will learn about two interesting features of Rover: the color LED and waiting for Rover to be ready for the next task.
Objectives:
- Control Rover’s color LED
- Wait for Rover to finish the current task before starting a new task
- Lighting up the LED at the proper time
Step 1
On top of Rover, near the battery strength meter (the 4 green lights), there is a color LED just like on the TI-Innovator™ Hub. But you cannot see the TI-Innovator™ Hub’s LEDs. This LED works like the TI-Innovator™ Hub LED. It requires values in the form (red, green, blue).
In a new Rover Coding template program, try the command found on
menu > TI Rover > Outputs > color_rgb()
which is pasted into your program as
rv.color_rgb(red, green, blue)
Provide values for the three color channels (0 to 255 each) and run the program to see the LED light up in your color.
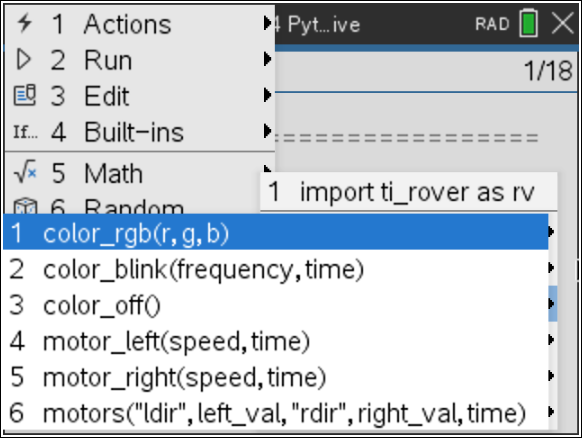
Step 2
-
In the previous lesson, you made Rover drive in a square pattern using a for loop. In this lesson, you will have the color LED light up in red at the corners only.
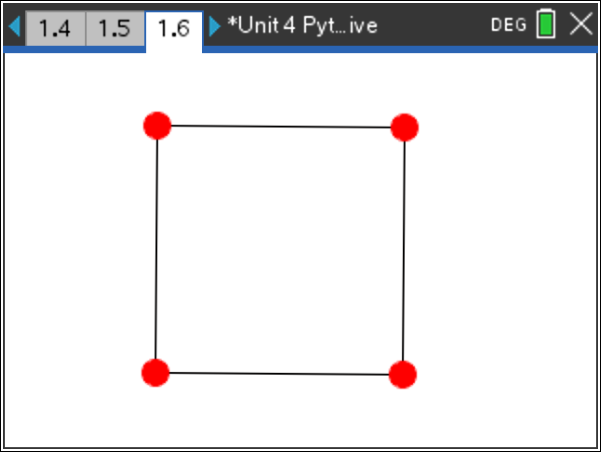
Step 3
-
Make a copy of your square program from Unit 4 Skill Builder 1.
menu > Actions > Create copy…
Just before the turn statement (left or right) in your program, turn the LED red. After the turn statement, turn the LED off:
rv.color_rgb(255,0,0)
rv.left(90)
rv.color_off()Both color statements are on menu > TI Rover > Outputs.
Run the program. Does it do what you expected?
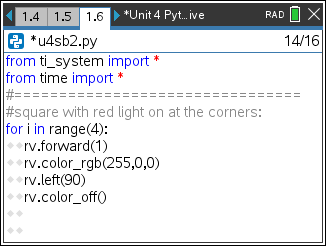
Step 4
-
This attempt does not work correctly because the TI-Nspire™ CX II graphing calculator is working faster than the Rover. The TI-Nspire™ CX II graphing calculator sends all the instructions to the TI-Innovator™ Hub as fast as it can. The TI-Innovator™ Hub then stores the driving instructions and processes them as a driver following a route on a mapping app, one at a time. The drive commands are stored in a queue (a list) and are processed one at a time because each instruction takes some time to finish.
But the rv.color command is not a driving command. When the TI-Innovator™ Hub receives this instruction, it is processed immediately (independent of the driving commands). So, the LED blinks rapidly four times right at the start of the driving.
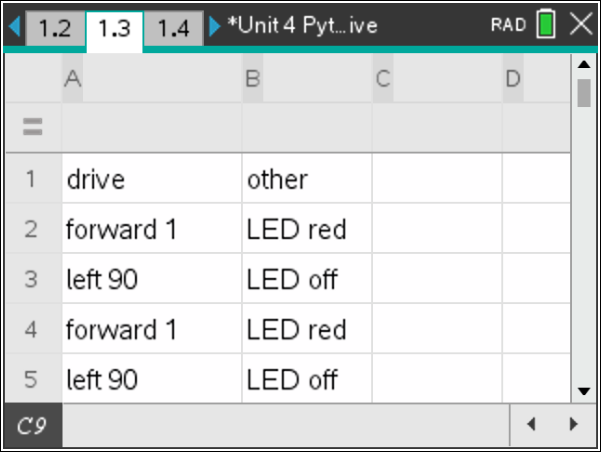
Step 5
-
Fortunately, there is a statement that you can use to tell the program to wait until Rover is "ready" to turn. After the rv.forward() statement add the statement
rv.wait_until_done()
found on menu > TI Rover > Commands.
This instruction is telling the TI-Nspire™ CX II graphing calculator to wait until it receives a signal from Rover that the Rover is finished with the rv.forward () command. The red LED then comes on and Rover turns.
Test your program now.
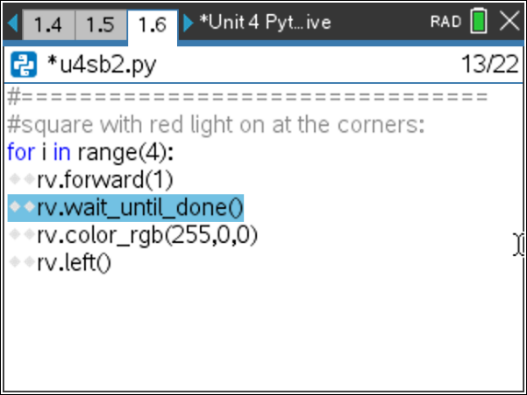
Step 6
-
Notice that the LED flashes quickly at the beginning of the turn. The turning also takes some time to complete, so you must tell the TI-Nspire™ CX II graphing calculator to wait again while the turn is taking place before turning the LED off.
Add another rv.wait_until_done() statement after the rv.left() statement and before the LED is turned off so that the LED stays on throughout the entire turn.
Try your program again.
Can you have the LED light up in another color along the sides of the square? Hint: It only takes one more statement.
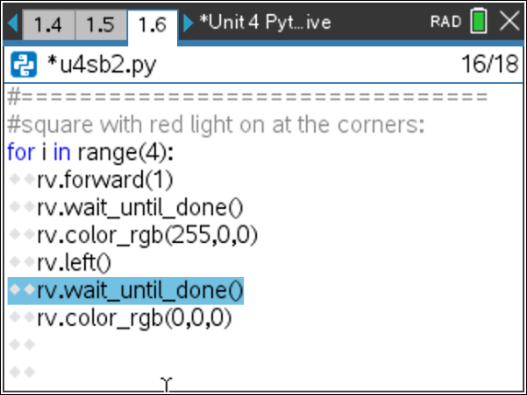
Skill Builder 3: Custom Turns and a Polygon
Download Teacher/Student DocsIn this lesson, you will use custom turning angles and have Rover drive along a pentagonal path, lighting up in two different colors: one along the sides and another around the corners.
Objectives:
- Controlling the amount of turning
- Use left() and right() with arguments
- Use colors around the pentagon
Step 1
Your project in this lesson will make Rover drive a pentagonal path. The square was easy because you knew to turn 90 degrees. But for a pentagon, you must tell Rover how many degrees to turn at each vertex.
From your geometry experience, can you determine how many degrees Rover needs to turn at each vertex? See the hint in the picture to the right.
In addition to the driving, you will also have Rover put on a light show. Use the color LED on Rover to display intensities of one color along the sides of the pentagon and another color at the vertices.
And, for extra fun, you can insert a marker in the marker holder and draw the pentagon — on paper, not on the table or the floor!
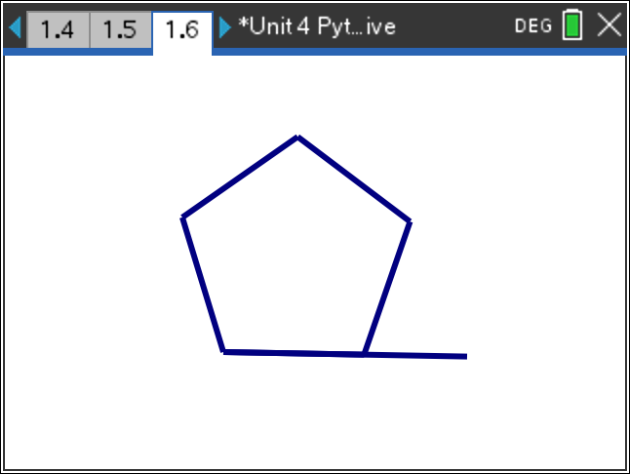
Step 2
-
Begin by making a copy of the square-driving program from the last lesson.
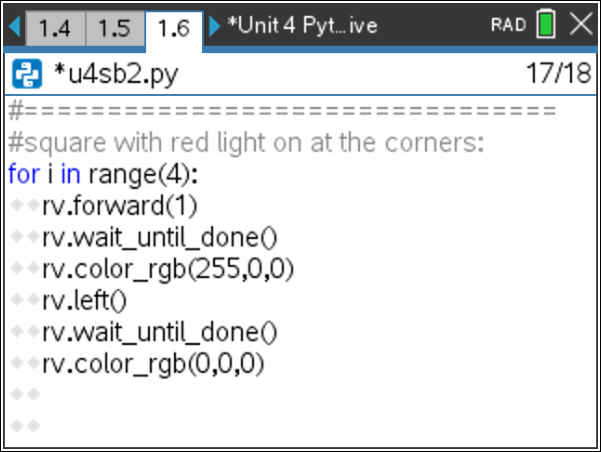
Step 3
-
Make two changes to convert from a square to a pentagon: There are five sides, not four, and the angle to turn is 72 degrees:
for i in range(5)
rv.left(72)
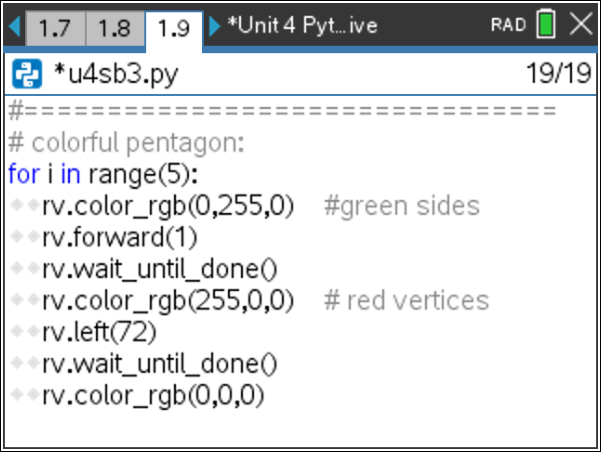
Step 4
-
Next, as Rover drives around the pentagon, the LED should start out with a dim color and gradually brighten. Use a variable or expression that makes the LED burn brighter at each side and each vertex. Your mathematics will come in handy.
Be careful to make sure that the color values stay between 0 and 255. You can use the variable i which varies from 0 to 4 to calculate a color value.
One possible expression: 50 + 50 * i
Note that the last statement, rv.color_rgb(0,0,0), is not indented (dedented). This takes the statement out of the loop so that the LED is not turned off at each vertex, but only once at the end of the program. To turn the LED off, use either rv.color_rgb(0,0,0) or rv.color_off(). They do the same thing.
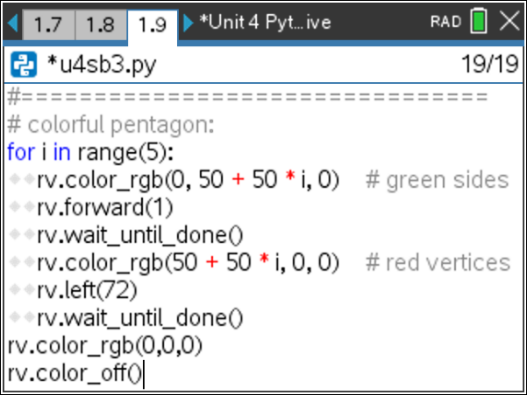
Application: Custom Polygons
Download Teacher/Student DocsIn this lesson, you will earn your Rover driver’s license by designing a "regular polygon" maker.
Objectives:
- Use input() statements to enter data for the number of vertices and the length of each side of a regular polygon
- Display lights along the sides and corners.
Step 1
Now that you have successfully driven a square and a pentagonal path, you are ready to take your Rover driving test.
Create a program in which you enter the number of vertices and the length of each side of a regular polygon, drive that route, and light up the sky with a dazzling array of colors along the way. In addition to simply moving Rover, your program will seek input from the user, drive the proper distance for each side, and calculate the proper angle to turn at each vertex.
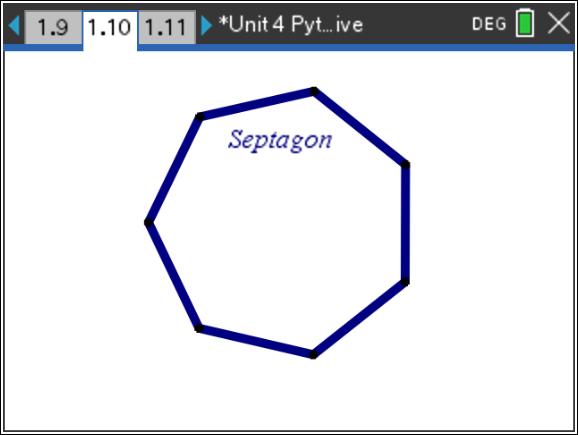
Step 2
-
Make a copy of your pentagon program from the last lesson. Your code should be similar to the image to the right. You will make some additions and changes to this code.
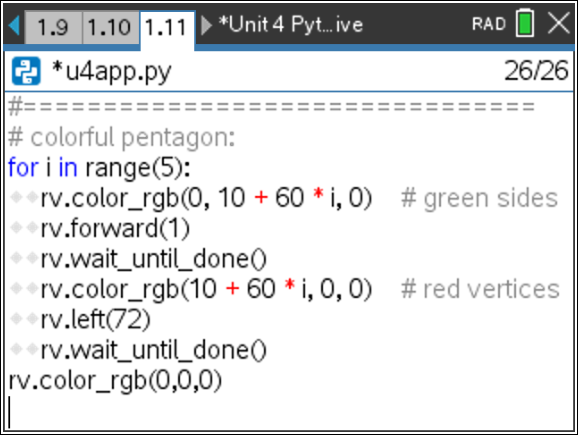
Step 3
-
Before the for loop, write two input statements to enter the number of vertices (n) and the length of each side (s).
n = …. (an input statement)
s = …. (another input statement)It is good to use more informative variable names like vertices and length but be careful not to use Python reserved words.
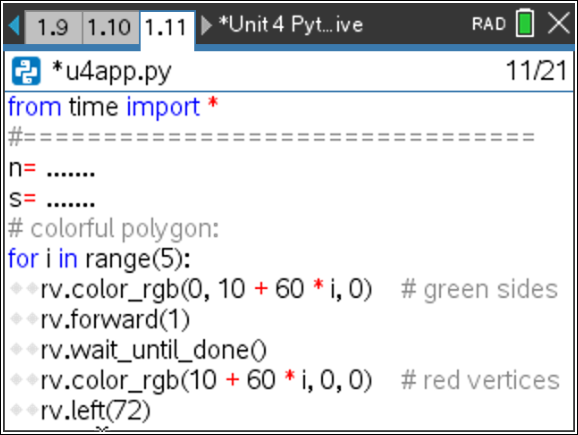
Step 4
-
There are three values in the loop statements that need to change:
range( ? ), forward( ? ), and left( ? )
After editing those three arguments, run your program, enter values for the input statements, and test your program.
Watch Rover carefully or insert a marker in the marker holder and draw the polygon on paper.
If you used an expression for the brightness of the LED along each side, then you will also have to adjust those LED statements to account for the change in the number of vertices. It would be better to use a variety of colors, not just red and green. Try random colors!
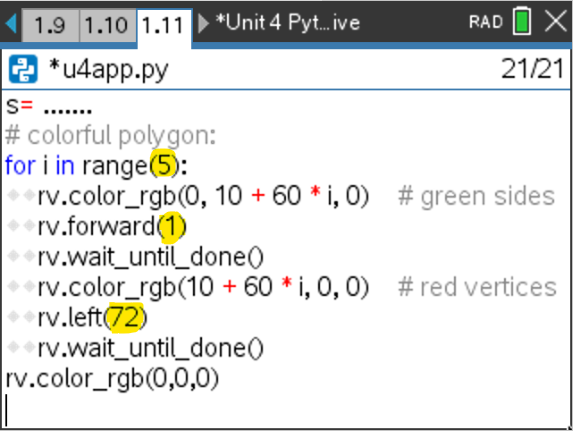
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 5: Rover’s Sensors
Skill Builder 1: Introducing Ranger
Download Teacher/Student DocsIn this lesson, you will learn to read the distance from the front of the TI-Innovator™ Rover to an obstacle. This lesson monitors that distance and displays information in two different ways. Another sensor is on the bottom of Rover and it can "see" colors.
Objectives:
- Read Rover’s Ranger measurement
- Display it on the screen using print()
- Display it on the screen using text_at()
- Determine the unit of measure
Step 1
The two small cylinders on the front of Rover are not headlights. They are an Ultrasonic Ranger. A silent (to us) tone is sent out by one of the two sensors and the other one "hears" the echo when the sound bounces off an obstacle. The internal software then calculates the distance to the obstacle using the speed of the sound and the time that it takes for the echo to return to the sensor: D = V * T
All that happens in the blink of an eye!
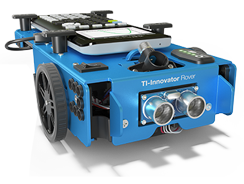
Step 2
-
Begin a new Python Rover Coding project. For this lesson, use the convenient "press esc to exit" loop:
while get_key() != “esc”:
blockYou will find this command on several menus, but since you are using Rover commands, look in menu > TI Rover > Commands.
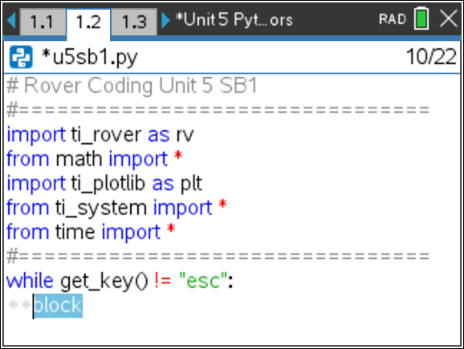
Step 3
-
Add three statements in the while block to
- read the distance in front of Rover:
dist = rv.ranger_measurement()
(Find this on menu > TI Rover > Inputs.) - print it on the screen:
print("Distance= ",dist)
- wait before reading the next distance:
sleep(.5)
Run the program and move your hand toward and away from the front of Rover. Point Rover at a wall, the ceiling and the floor. Watch the values that are displayed. Note: Rover does not move … yet.
Can you determine what distance unit (feet, meters, etc.) is being used?
- read the distance in front of Rover:
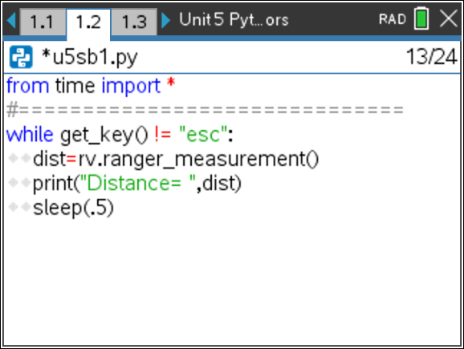
Step 4
-
Rather than have the printed numbers scroll down the screen, improve the display of the distance values by using the text_at() function found in the ti_plotlib module. If you worked through the previous TI-Innovator™ Hub lessons (Units 1, 2 and 3), you have used that command before. But here you must provide the (temporary) name of the module due to the way it is imported in the Rover template:
import ti_plotlib as plt
This requires that any functions that reside in the ti_plotlib module must be preceded by the "alias" name plt. You will also use the cls() function in the module which clears the drawing screen.
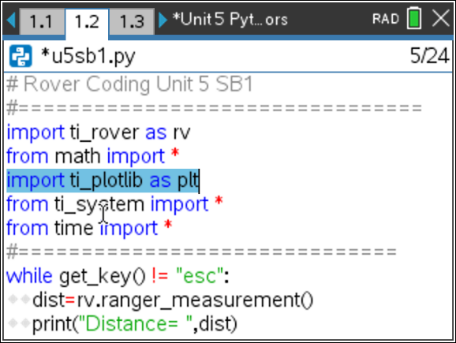
Step 5
-
See the functions
plt.cls()
plt.text_at(…)found on menu > TI PlotLib > Draw.
Other functions found in this module are used for graphing and will be useful when you start using Rover’s Coordinate System in the next unit.
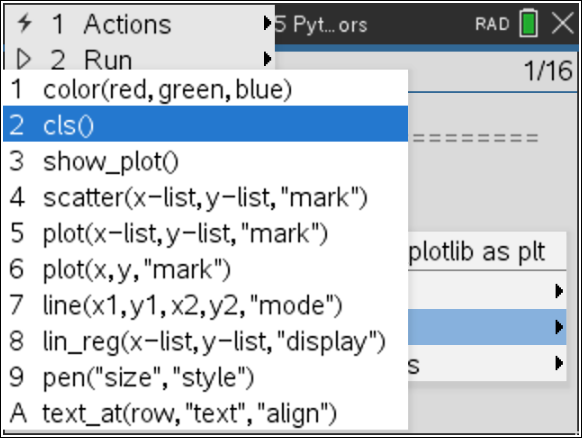
Step 6
-
Turn the print() statement into a #comment by pressing ctrl+T on the print() statement.
Add the two plt functions
plt.cls()
plt.text_at(7, str(dist), ”left”)found on menu > TI PlotLib > Draw.
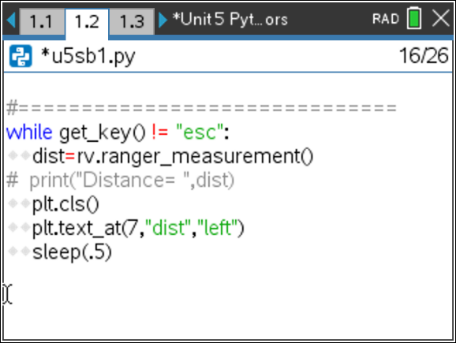
Step 7
-
About str(dist) again: The plt.text_at() function can only display “text” which is either a literal string “in quotes” or a variable containing a string. dist is a variable containing a number, so it needs to be converted to a string using the str() function found on:
menu > Built Ins > Type
Row 7 is the vertical center line of the 13-line layout of this screen.
You can sample the distance faster by using a smaller sleep() value.
You have just created a digital tape measure!
In the next lesson, you will use this information to keep Rover from crashing into something.
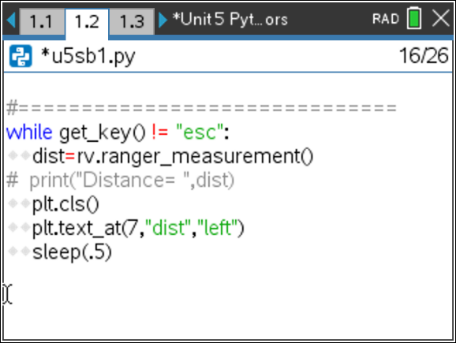
Skill Builder 2: Off the Wall
Download Teacher/Student DocsIn this lesson, you will use the TI-Innovator™ Rover’s Ultrasonic Ranger to avoid an obstacle.
Objectives:
- Read the distance to an object in front of Rover
- If the distance is "too small," turn around and continue driving
Step 1
As Rover is driving, your program can use the Ultrasonic Ranger to detect an obstacle ahead and interrupt Rover to take another route. In this project, the Rover will drive back and forth between two opposite walls.
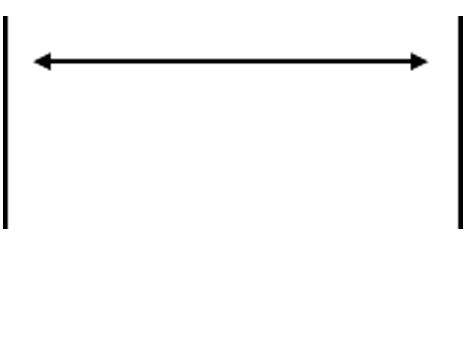
Step 2
-
Begin a new Python program using the Rover Coding template.
Get Rover moving using:
rv.forward (100)
Yes, that’s 10 meters, or about 39 feet, but there will be an obstacle in the way.
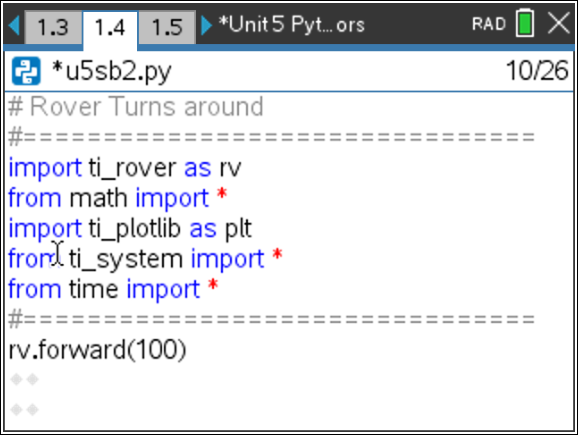
Step 3
-
Read the distance in front of Rover:
dist = rv.ranger_measurement()
As long as there’s room to proceed, keep going. But if Rover is too close to an obstacle, turn around. How close is too close? That is for you to determine.
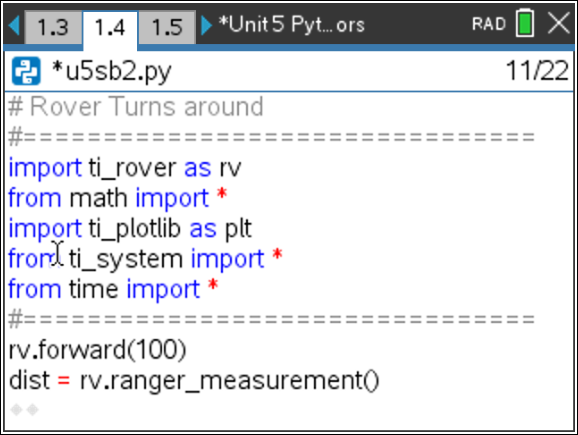
Step 4
-
Add a while loop to continue monitoring the distance as long as the obstacle is "far away":
while dist > ? :
(Replace the question mark (?) with a "small" number.)
dist = rv.ranger_measurement()For now, just stop Rover when the loop ends.
rv.stop()
rv.stop() is found on menu > TI Rover > Drive.
Test your program by pointing Rover towards a "wall" (less than 40 feet away) and run the program. Rover should stop before hitting the "wall".
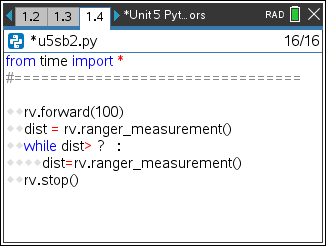
Step 5
-
When Rover encounters the obstacle, the Rover should turn around. After Rover stops, have the Rover turn around using:
rv.left(180) or rv.right(180)
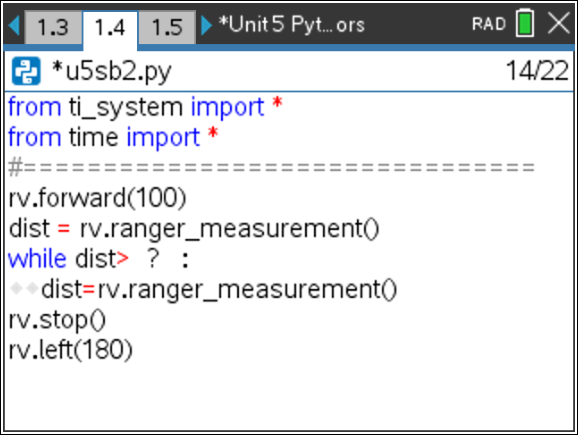
Step 6
-
After turning around, go in the opposite direction (forward) until a key is pressed. Remember that Rover is moving, so it might be tricky to press the esc key.
Place the statement
while get_key() != “esc”:
at the beginning of your code.
Edit the statement so that any key can be used to stop the program:
while get_key() == “”:
(== stands for “equals”, “” stands for "nothing")
Indent all the statements below this while statement to make them the while block. The easiest way to indent a group of statements is to select all those statements with shift+down arrow, and then press the tab key.
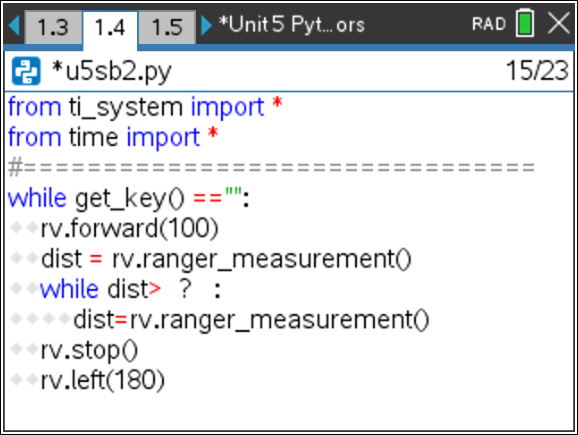
Step 7
-
Run the program now. Rover approaches the wall, stops, and turns around (if there’s enough room). Wait — something is wrong. Rover is stuck!
Turning around takes Rover a second or two, but your program proceeds back to the top of the loop immediately, tells Rover to go forward, then detects the wall again and stops. Rover turns, goes forward, and stops. Rover is overwhelmed because the commands are all coming in almost at once. You will hear the motors churning, but Rover does not move. Everything is happening too fast and Rover will have a nervous breakdown if something is not done … and soon!
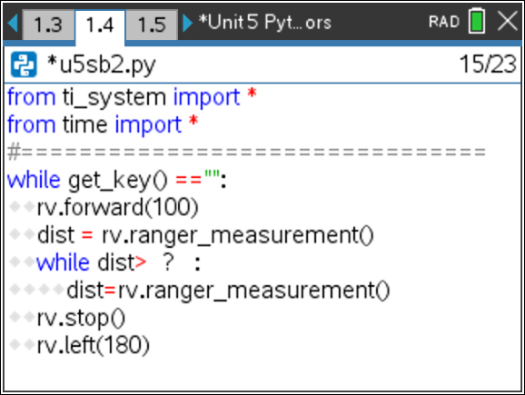
Step 8
-
Press any key to terminate the program.
Rover might still churn for a while. If so, just unplug Rover from the handheld, turn the power switch off, and then reconnect and turn on again.
To fix the issue in your program, make the TI-Nspire™ CX II graphing calculator wait until Rover has finished turning around by adding the statement:
rv.wait_until_done()
Place this statement just below the rv.left(180) statement.
Run the program again and Rover will happily travel between two opposite walls.
Rover needs about 20 cm to turn around. The Rover’s aft is longer than the bow. while dist > 0.2: handles this well.
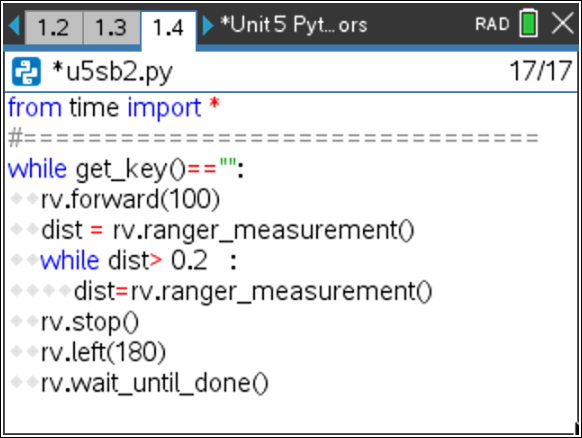
Skill Builder 3: Spot the Color
Download Teacher/Student DocsIn this lesson, you will learn to use Rover’s color sensor to change direction when a color is detected. Colored paper or large colored shapes are needed for this lesson.
Objectives:
- Use the color sensor to detect and react to a color
Step 1
Rover has a color sensor on the bottom. You can see a light shining on the floor under the color sensor. The light helps Rover to see the color beneath it. First write a "test" program to see what kind of values the color sensor produces and then you will be able to write a program to react to different colors. You will need some colored paper like construction paper or just print out some colored shapes like the rectangles at the right. They should be large enough for Rover to "see."
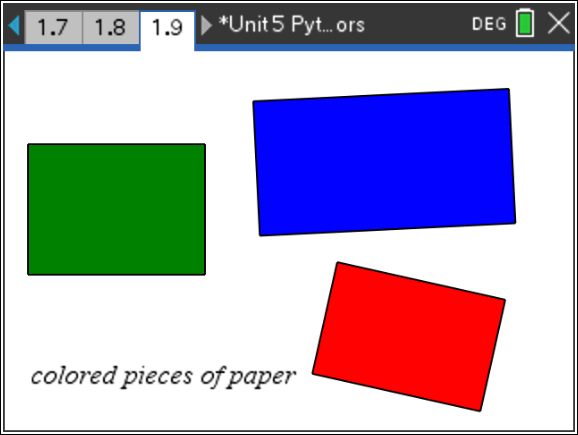
Step 2
On the menu > TI-Rover > Inputs, there are five different color measurements available. The function color_measurement() returns a value from 1 to 9 where:
1=red, 2=green, 3=blue, 4=cyan, 5=magenta, 6=yellow, 7=black, 8=white, 9=gray
The other four measurements return the amount of the indicated color in the range 0…255, as shown on the menu.
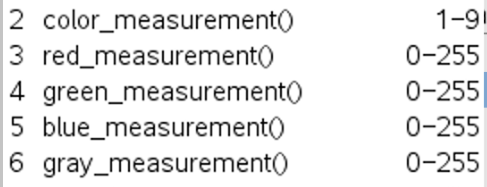
Step 3
-
Here is a short "test" program using the Rover Coding template to determine the values that the color measurement functions produce.
while get_key() != "esc":
c = rv.color_measurement()
plt.text_at(7, str(c), "left")Try all five color measurements (color_, red_, green_, blue_ and gray_) on various colored surfaces and observe the values displayed.
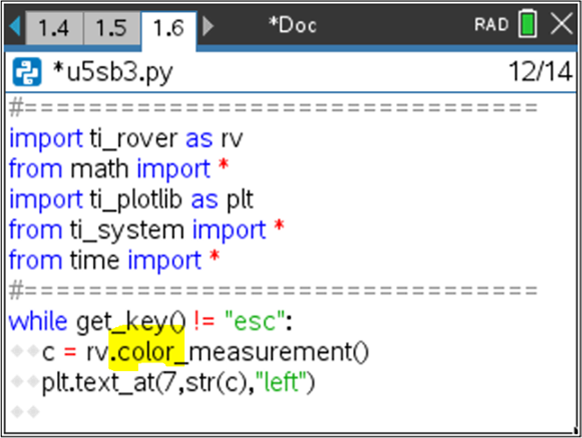
Step 4
-
Depending on your floor color, make some colored pieces of paper (like "sticky notes" or colorful construction paper) to place in front of Rover so that Rover can "see" the change in color from the floor. Test these patches first to see what Rover "sees".
Write a program to get Rover to "react" to the difference in colors. In the sample page to the right, the red border should keep Rover on the page:
When Rover "sees" red, turn around.
When Rover "sees" green, turn right or left.
You can also add statements to the program to control the light or color LED.
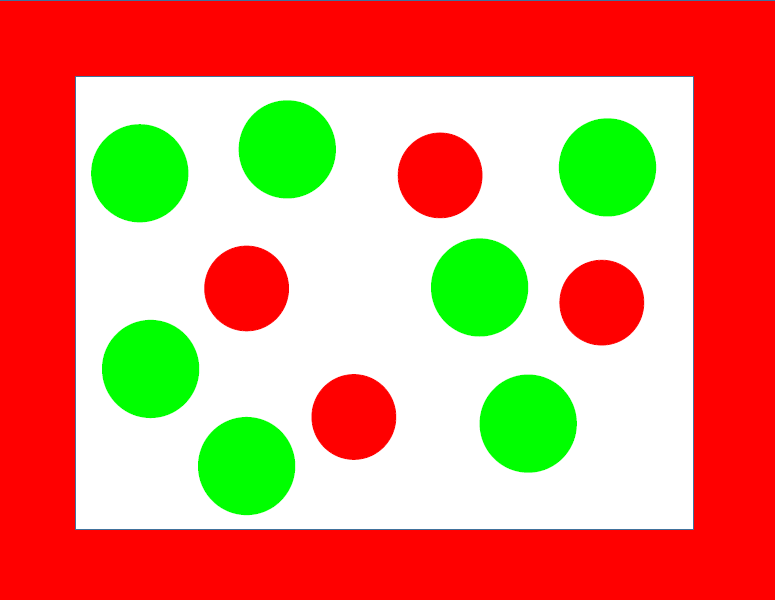
Step 5
-
Edit the color "test" program you started. Add a statement at the beginning of the loop to start Rover moving forward:
rv.forward(10)
Next, monitor the color below Rover. Changing the variable c to color makes the program more readable:
color = rv.color_measurement()
When color is red, make Rover turn 180 degrees and, when the color is green, make Rover turn 90 degrees. This requires two if statements.
Delete plt.text_at(7, str(c), "left").
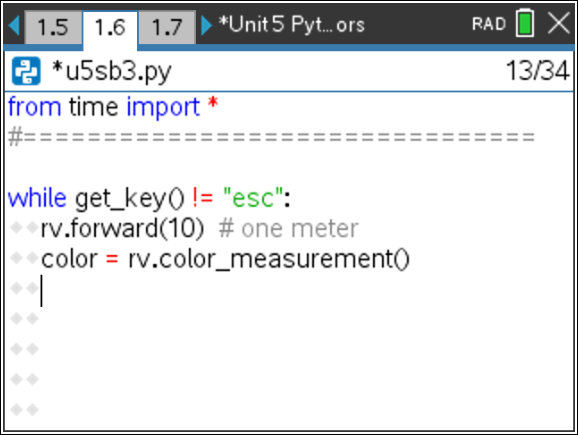
Step 6
-
Add two if statements and use the values you got from testing your color samples in place of the ?s:
if color == ?:
block
if color == ?:
block(You will not see the placeholder "block" appear for the if statement and the words "red" and "green" in the image are just placeholders.)
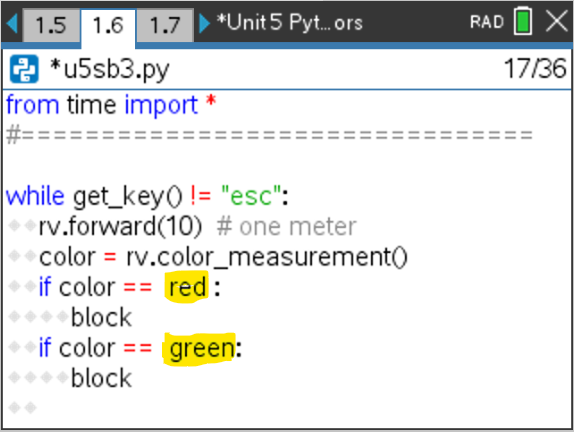
Step 7
-
The two if blocks are similar. Just remember red-right and green-left.
Each block will:
- Stop Rover
- Turn 180 degrees
- Go forward a little bit to move away from the colored spot
- Tell the TI-Nspire™ CX II graphing calculator to wait until Rover has finished these three tasks
Try it yourself before proceeding.
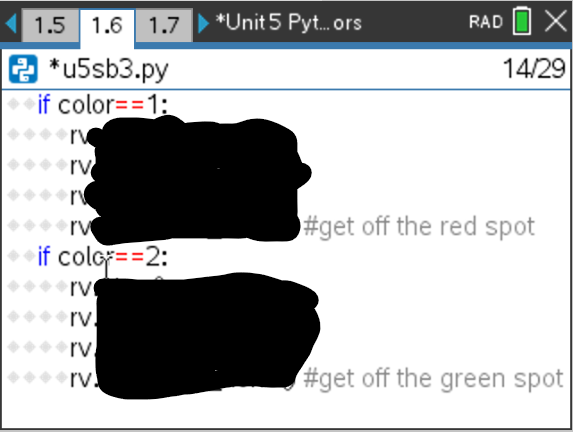
Step 8
Do your if blocks resemble this?
if color == 1: (Red; use another number if your color is not red.)
rv.stop()
rv.right(180)
rv.forward(1)
rv.wait_until_done()Tip: If you assign the variable red = 1 at the top of your program, then you can write if color == red: in your if statement. This makes the intent clear.
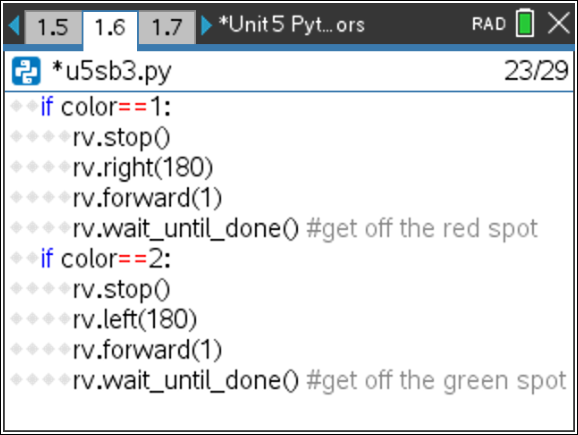
Application: The Winding Road
Download Teacher/Student DocsIn this application, you will use Rover’s color sensor to follow a curved path on paper. (A curved path on paper like the one shown in Step 1 is needed for this application.)
Objectives:
- Use the color sensor to detect and follow a curved path on paper
Step 1
Write a program to keep Rover "on track" to follow a curved path similar to this one:
→ |
Rover will start at the left edge of the page and travel to the right following the curved path across the paper.
When Rover "sees" RED, it will turn to the left a little and move forward a little. When Rover "sees" WHITE, it will turn to the right a little and move forward a little.
Experiment with the turning angle and the moving distance to see how the Rover reacts to the different colors.
If your page is red and white as in the image above, you can use red_measurement() to see what values are given by each side of the paper. If you use a different color such as black, you can use gray_measurement() (or green_ or blue_).
Step 2
-
Here is the original short "test" program from the last lesson to determine the values that the color measurement functions produce.
Choose the color measurement type (color_, red_, green_, blue_ or gray_) that gives the greatest variation in values for your two different colors and the greatest consistency in values when looking at each of the colors separately.
You can also use a more descriptive variable such as color instead of c as you did in the previous lesson:
color= rv.color_measurement()
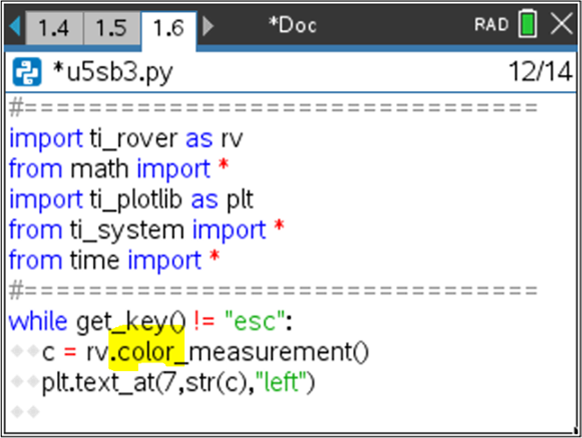
Step 3
-
For your path-following program:
- Start Rover on the left or right edge of this paper near the curved path
- Check the color
- If the color is red, then turn a bit toward the white side
- Otherwise, turn a bit toward the red side
- Move forward a short distance
- Repeat from step b until you reach the end of the path
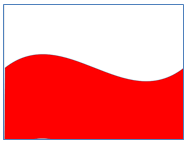
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 6: Coordinates With Rover
Skill Builder 1: Drive and Plot
Download Teacher/Student DocsIn this lesson, you will learn about the Rover’s coordinate system and how to drive to a particular point represented by an ordered pair of numbers.
Objectives:
- Understand the Rover’s coordinate system and initial position and heading
- Make the Rover move to a certain point on the coordinate plane
- Plot Rover’s points on the TI-Nspire™ CX II graphing calculator’s screen
Step 1
The Rover has a built-in coordinate system just like a Cartesian graphing system. When you import ti_rover as rv, the Rover’s position on the coordinate grid is set to (0,0) and its heading is 0 degrees (pointing toward the positive x-axis, or "east" on a map).
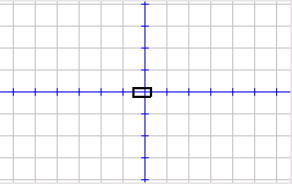
Step 2
In addition to Rover’s coordinate grid, we can use the on-screen coordinate system that is available from the ti_plotlib module which is also imported into the Rover Coding template for just this reason. If you use just these three statements from menu > TI PlotLib > Setup, you will get the screen to the right when you run:
plt.window(-10,10,-7,7)
plt.grid(1,1,"dashed")
plt.axes("on")
As we tell Rover to move around to points on the floor, we will also be plotting those points on the screen.
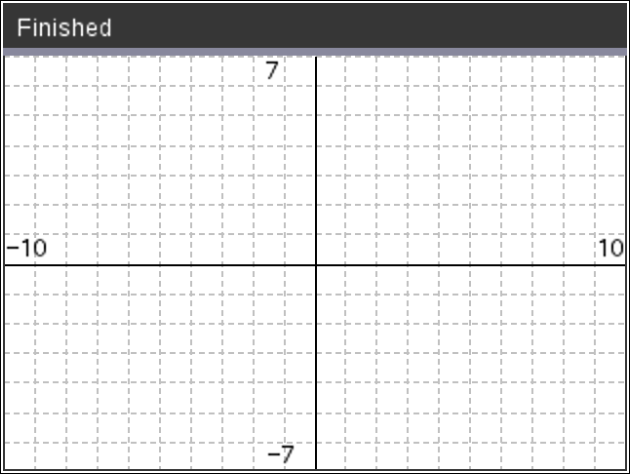
Step 3
-
The first point to plot is Rover’s home position: (0, 0) using the statement
plt.plot(x,y,”mark”)
found on menu > TI PlotLib > Draw.
Change the point (x, y) to (0, 0) and then select a “mark” to represent Rover from the provided list. If you run the program now, you will see a mark (we chose the dot "o") at the origin in the center of the screen.
Now, whenever Rover drives to a point on the floor, also plot the point on the screen.
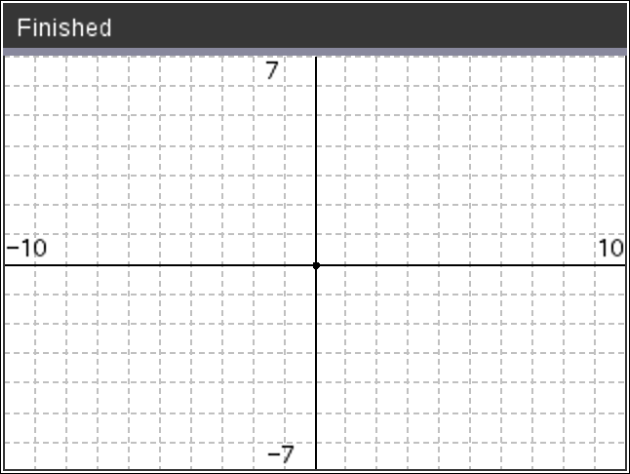
Step 4
-
Depending on the space that you have, make Rover drive to a point in each of the four quadrants using rv.to_xy() found on menu > TI Rover > Drive.
rv.to_xy(1,1)
rv.to_xy(-1,1)
rv.to_xy(-1,-1)
rv.to_xy(1,-1)You do not have to use 1s and you don’t even have to use the same numbers. But you do have to make sure that Rover visits all four quadrants.
Try your program now. Notice that Rover turns directly toward the position of the next point before moving there.
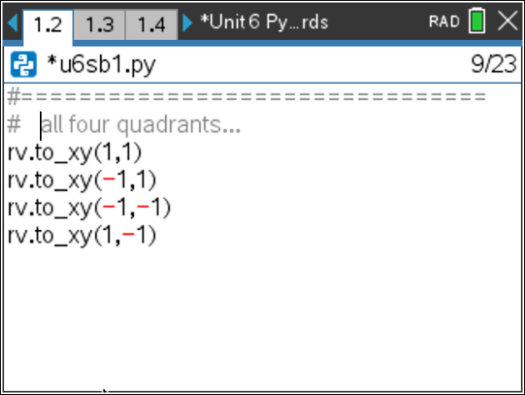
Step 5
-
Add plt.plot(x,y,”mark”) statements to your program to plot the points on the screen after Rover reaches them. Try it now. Did the program perform as you expected?
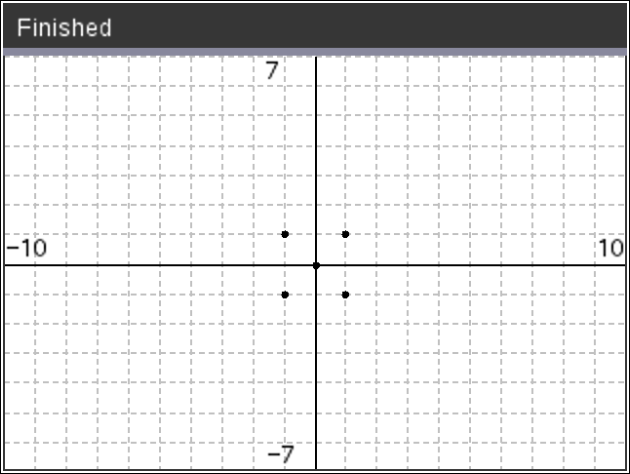
Step 6
-
Answer: No! The points on the screen are plotted almost immediately when the program runs, and it takes Rover some time to drive to all four points. How do you sync the plotting with the driving?
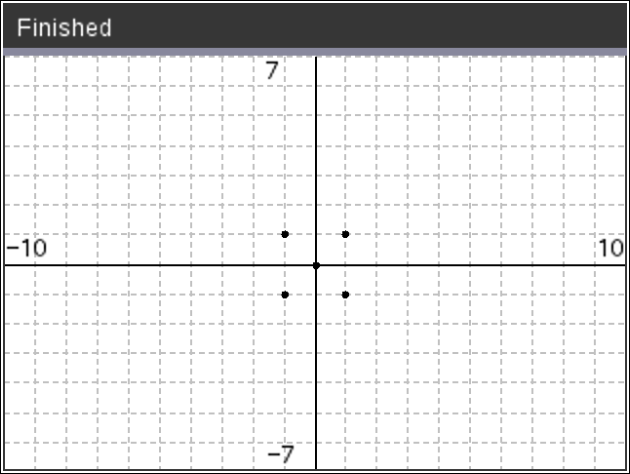
Step 7
-
Use the Rover function rv.wait_until_done() found on menu > TI Rover > Commands to pause the program while Rover is moving. You will need one of these functions for each driving point and order does matter. Try it now. Where will you place those wait functions?
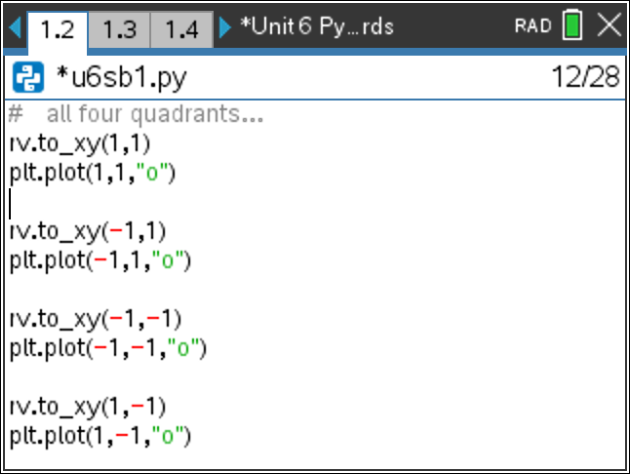
Step 8
-
At the end of your program, send Rover to the "home" position, (0, 0). Use the statement
rv.to_angle(0, “degrees”)
found on menu > TI Rover > Drive to have Rover point in the original direction ("east"). It’s nice to put the toys away, right?
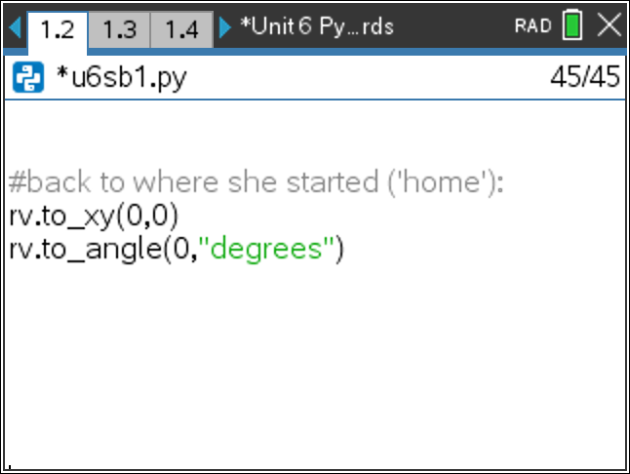
Skill Builder 2: The Distance Formula
Download Teacher/Student DocsIn this lesson, you will use the distance formula from coordinate Geometry to calculate the distance between two points and compare the Rover’s measured distance against the calculated distance. (You will need a meter stick or metric tape measure for this lesson.)
Objectives:
- Move to two different points
- Use a marker to draw the segment
- Use a function to compute the distance between two points and display the distance
- Measure the distance between the points
- Compute the error in the measurement versus the computation
Step 1
Recall the “Distance Formula”, which is based on the Pythagorean Theorem:
Based on the image to the right, this becomes the Python statement:
d = sqrt((6 - 2)**2 + (4 - 1)**2)
This evaluates to: d = 5
Can you find a 3-4-5 right triangle in the image?
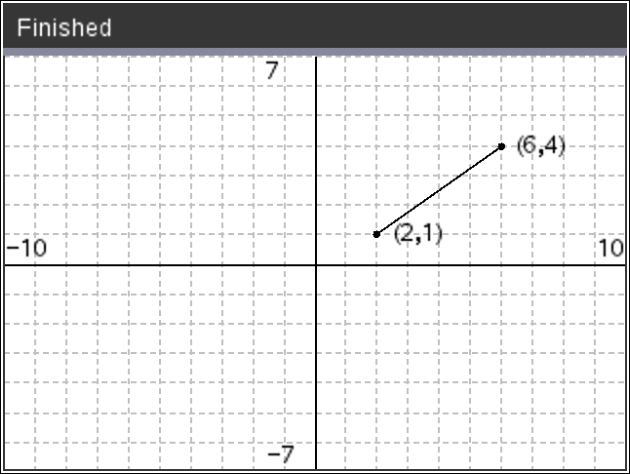
Step 2
-
Start a new Python Rover Coding project.
Define a function called dist which takes four arguments (two pairs of coordinates) and will return the distance between the two points.
The def function() template is found on menu > Built-ins > Functions.
The body of the function consists of one calculation:
and the return statement: return d
return is found on menu > Built-ins > Functions
Make sure these two statements are indented the same amount.
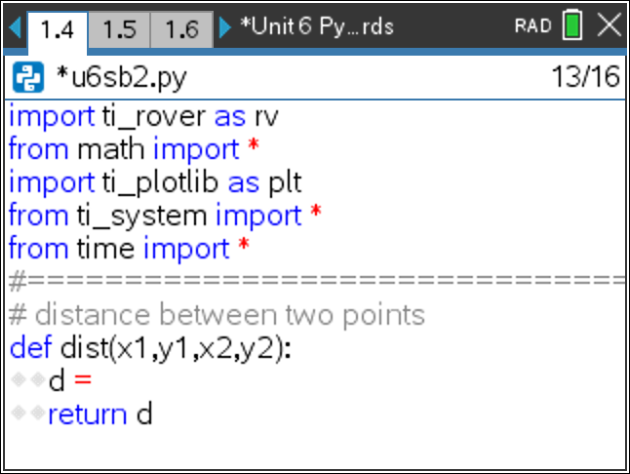
Step 3
-
Below the function (after the return statement), begin the main program. Be sure your code is no longer indented. Write four input() statements (using copy and paste) to enter the coordinates of the two points. Create simple prompts for the inputs and use the float() function to convert the input result from a string to a decimal value. Only one of these four statements is shown to the right. We are using the variable a to store the first x-coordinate. Use b, c and d for the other three coordinates.
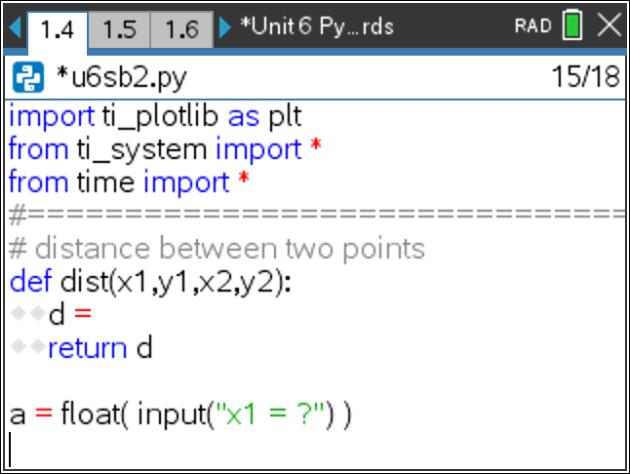
Step 4
-
After the four input() statements, make Rover drive to the first point. Pause there while you insert a marker in the Rover’s marker holder to draw a line segment. Then continue driving to the second point. A good pause statement is:
input( “press [enter] to continue.”)
The result of this input function does not assign any value to a variable because nothing is entered.
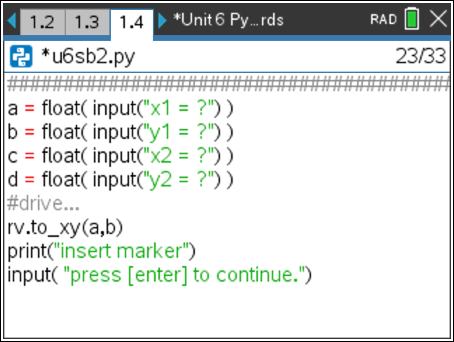
Step 5
-
Now have the program evaluate the distance function using the coordinates of your two points:
calculated_distance = dist(a, b, c, d)
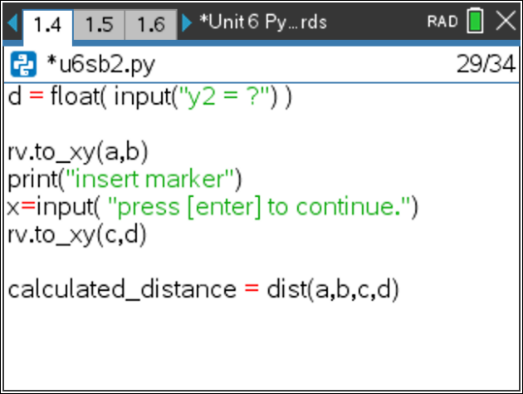
Step 6
-
Use a ruler or tape measure to determine the length of the segment that Rover made.
Add an input() statement to your program so that you can enter the measured_distance.
Add print() statements to display the two distance variables.
How does the measured distance compare to the calculated distance?
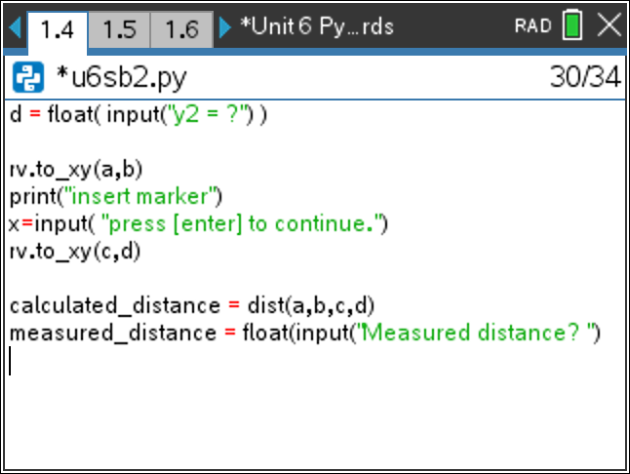
Step 7
-
Calculate the percent error using the formula
(measured - calculated) / calculated * 100and print the error.
Skill Builder 3: Make the Shape
Download Teacher/Student DocsIn this lesson, you will write a program to make a pre-designed two-dimensional shape. You will program with lists and use a loop to get Rover to draw (or just drive) the shape on paper.
Objectives:
- Create lists in a Lists & Spreadsheet app
- Use recall_list in Python
- Use a for loop to process elements in a list
- Use a pause statement to control processing
Step 1
This project requires you to make two lists that represent the x- and y-coordinates of the vertices of a shape of your own design. In this lesson, we will use the design of the block letter T shown to the right. Your goal is to have the Rover make this design using a marker (or just follow the route if no marker is available).
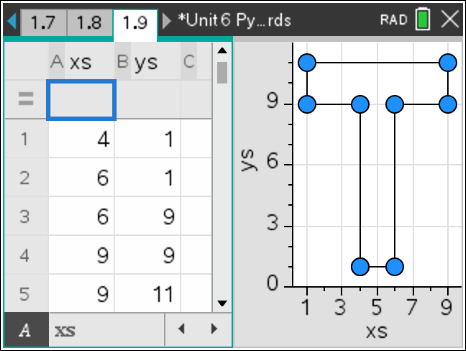
Step 2
-
Before writing the program, enter your shape’s coordinates into two lists in a TI-Nspire™ CX II graphing calculator’s Lists & Spreadsheet app. All the coordinates of the T shape are given in the image to the right. Note that the names of the two lists are xs and ys. These names are important for your program.
You can test your values by setting up a Scatter Plot on a Graphs app or use the Data & Statistics app (Quick Graph from the Lists & Spreadsheet app).
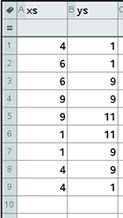
Step 3
-
Begin a new Python Rover Coding project.
Your first two new statements will get the lists from the TI-Nspire™ CX II graphing calculator’s variables and store them in two Python variables. It’s okay to use the same variable names, but they are really not the same variables.
From menu > More modules > TI System, use the statement:
recall_list(“name”)
You need two of these statements, so just select, copy, and paste the statement (or get it from the menu again).
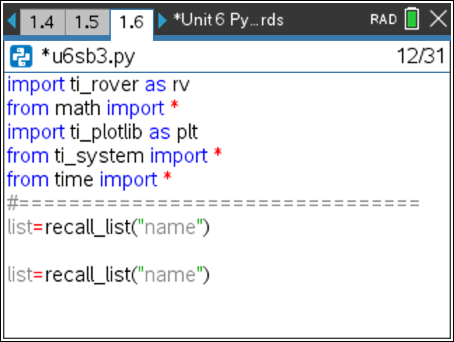
Step 4
-
In one of these statements use xs for list and name and in the other use ys.
These two statements get the lists “xs” and “ys” from the TI-Nspire™ CX II graphing calculator and store them in the Python variables xs and ys, respectively (to the left of the = sign).
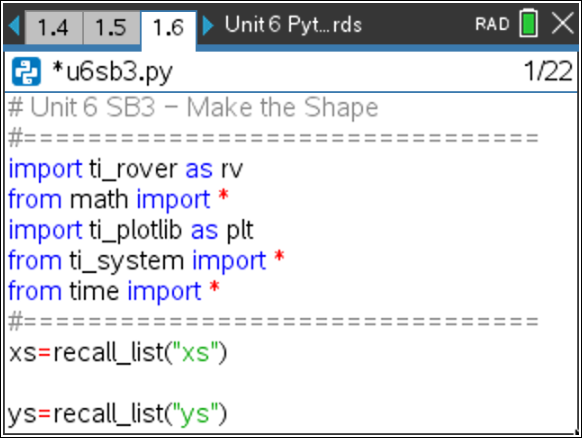
Step 5
-
You are now ready to program the Rover’s route. Remember that Rover begins at the point (0,0). Your first point might not be the origin, so have Rover move to the first point before pausing to insert the marker. The first point is ( xs[0], ys[0] ), so use the statement:
rv.to_xy(xs[0], ys[0])
[ ] are found on the left parenthesis key to the left of the 0 key.
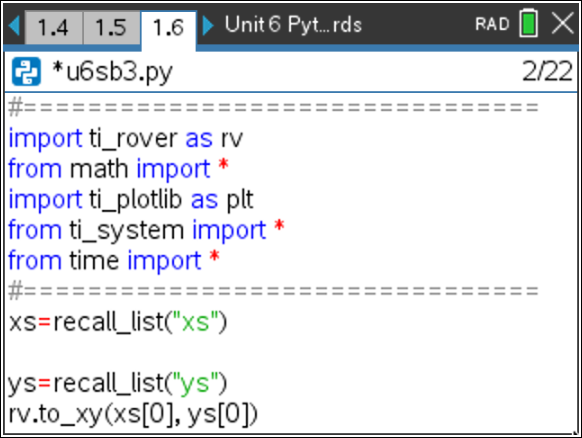
Step 6
-
Add a statement that pauses processing while you insert a marker (if you have one).
input(“Press enter to continue.”)
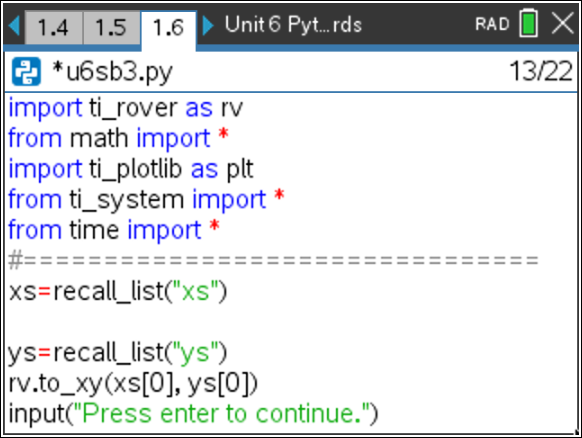
Step 7
-
Make a for loop to drive to the rest of the points:
for i in range(1, len(xs)):
blocklen(xs) is the length (size) of the list xs. If the length is 12,
then the loop ends with the value i = 11, the last element of the list.
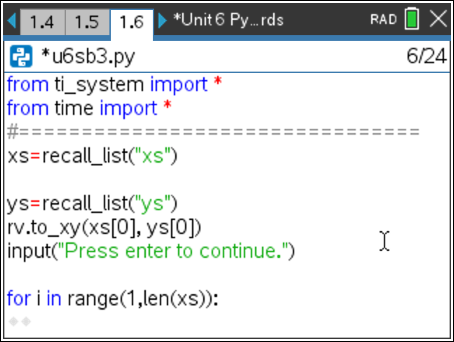
Step 8
-
Complete the block of the loop using the statement:
rv.to_xy(xs[i], ys[i])
After the loop (unindent), pause again to remove the marker, and then send Rover back to the origin (0,0) facing east.
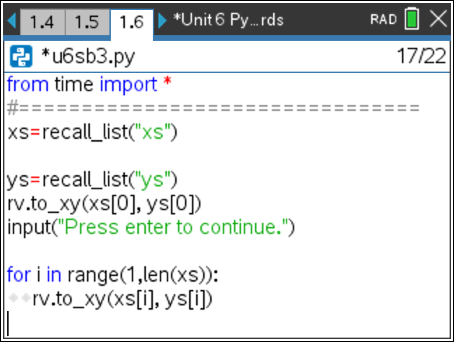
Step 9
-
Challenge: Use the ti_plotlib tools to also plot the shape’s coordinates on the screen in sync with Rover. Use plt.auto_window(xs,ys) to set up the window for your points. At the end, you can use the function plt.plot(xs,ys,”mark”) to draw a connected scatter plot.
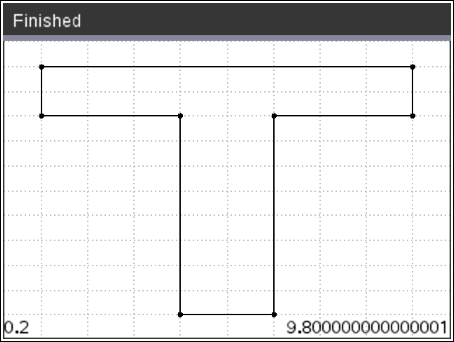
Application: A Random Walk
Download Teacher/Student DocsIn this application, you will see how often Rover can travel on the grid (making random turns north and east only) from the origin (0, 0) to the point (2, 2). You will also investigate the experimental and theoretical probabilities of reaching that point at random.
Objectives:
- Use a random number to decide in which direction to go next
- Determine success or failure of reaching the goal point (2,2)
- Plot coordinates on the screen
- Use the color LED to report success or failure
- Repeat the experiment multiple times and determine how often Rover reaches the goal
- Examine the theoretical probability of reaching the goal
Step 1
Rover starts at the origin (O). At each grid point, Rover can move only east (right) or north (up) at random, one unit at a time. There are several different routes Rover can take to reach the goal (2, 2). One is highlighted in red/bold in the image to the right. In how many different ways can Rover get to the goal? If each move is random it is possible that Rover will miss the goal?
What is the probability that Rover makes it to the goal?
Write a simulation of this problem to keep track of the number of times Rover makes it to the goal and determine the percentage of the trials that are successful.
Think about the failures: How do you know that Rover fails to make it to the goal?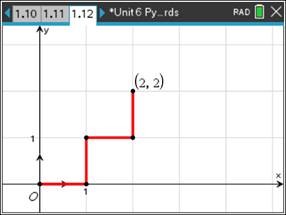
Step 2
-
Begin a new Python Rover Coding project.
Set up a plt screen to display Rover’s positions along the route using the TI PlotLib > Setup menu:
plt.window(-1, 4, -1, 4)
plt.grid(1, 1, "dashed")
plt.axes("on")
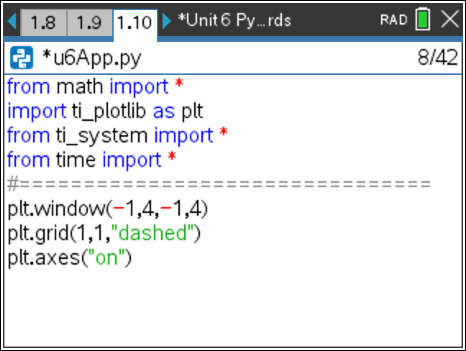
Step 3
-
Use a variable for the number of trials: trials = 10
Use a variable to count the successes: successes = 0
Use two variables for the goal point: px, py = 2, 2 (Yes, this is valid!)
Use a for loop to perform the trials: for i in range(trials):
Now begin a trial:
In the loop block, use two different variables for Rover’s position: rx, ry = 0, 0
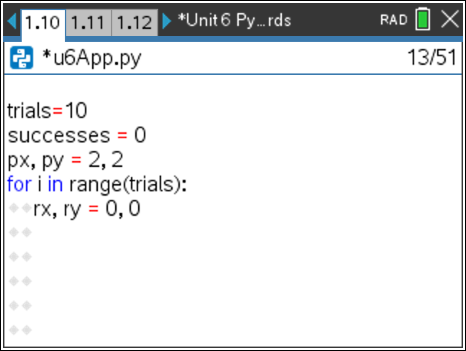
Step 4
-
Plot that point in the graphing screen: plt.plot(rx,ry,”o”)
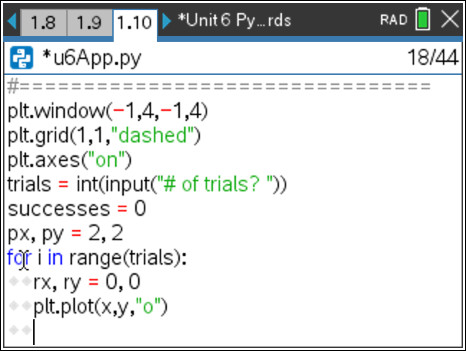
Step 5
- Make a while loop that continues as long as Rover is not at the goal point and Rover has not failed. Think about what condition determines a failure.
This additional condition is left as an exercise.
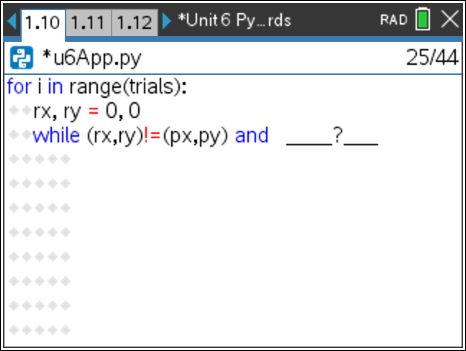
Step 6
-
In the while block, use randint(0, 1) to decide whether to go east (0 degrees) or north (90 degrees). This is accomplished with the statement:
dir = randint(0, 1) * 90
You will need to add from random import* to your import section to use the randint() statement. It is not included in the Rover Coding template.
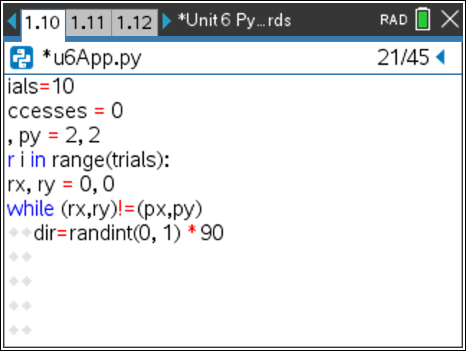
Step 7
-
Get Rover to turn to the correct angle (dir) and move forward 1 unit. (The statements in the image are incomplete.)
Add an rv.wait_until_done() statement to control the plotting speed.
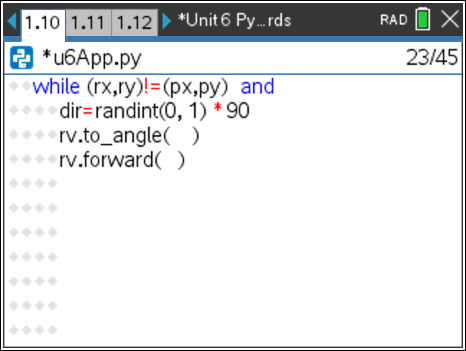
Step 8
-
Update Rover’s position variables, rx and ry.
If Rover moved east (dir == 0),
add 1 to rx.
Otherwise,
add 1 to ry.
Plot Rover’s position in the screen using plt.plot(rx,ry,”o”) (not shown).
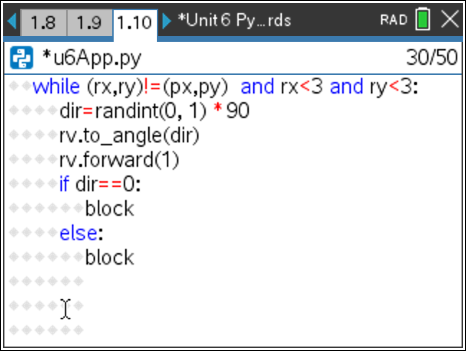
Step 9
-
After the while loop ends, determine if Rover was successful
if (rx, ry) == (px, py):
and count the success by adding 1 to the variable successes.
Print “SUCCESS!” or “FAIL”.
If Rover is successful, light the color LED in GREEN, otherwise light the color LED in RED.
Then enter (not shown):
- two statements for Rover to return to (0,0) and face east (to angle 0 degrees)
- a statement to turn the LED off
- statements to redraw the plotting screen starting with plt.cls(), and then use the three Setup statements again
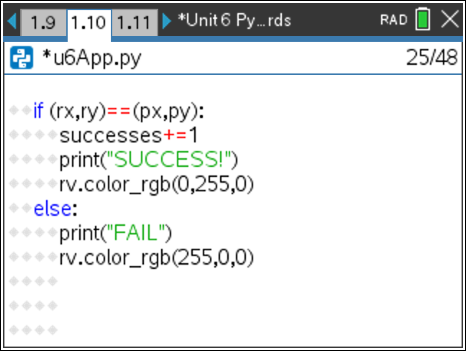
Step 10
After the for loop ends (when all the trials are finished), print the results of the experiments:
- the total number of successes
- the percentage of the successes (successes / trials *100)
A sample run of 10 trials is shown in the image to the right. The percentage is the experimental probability of success.
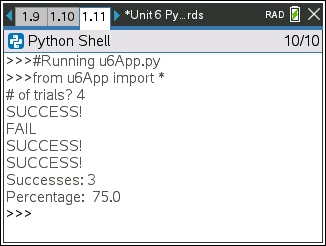
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 7: The TI-RGB Array
Skill Builder 1: Light Them Up
Download Teacher/Student DocsIn this first lesson of Unit 7, you will learn to control the 16 color LEDs on the TI-RGB Array as a group and individually.
Objectives:
- Light up ALL LEDs and make them blink in unison
- Use another loop to light up and turn off the LEDs one at a time
Note: The “TI RGB Array Sim” that is shown to replicate the lights on the circuit board, has been created, and used for demonstration purposes. This tool is not available for purchase or distribution by TI.
Caution: rapidly flashing lights may be disturbing for some students, so it is wise to use Wait statements to slow things down a bit.
Step 1
TI-RGB Array | back | breadboard ports |
The TI-RGB Array is a circuit board with 16 color LEDs and a controller chip and comes with a short four-wire cable. It connects to the TI-Innovator™ Hub using the breadboard (BB) ports on the TI-Innovator™ Hub. Follow the wiring instructions on the back of the circuit board to connect it to the TI-Innovator™ Hub; connect the TI-Innovator™ Hub to your TI-Nspire™ CX II graphing calculator.
Step 2
-
Start a new Python program using the Hub Project template.
Press menu > TI Hub > Add Output Device and select the TI-RGB Array.
In place of the var placeholder, type any variable name. We used cb (for "circuit board").
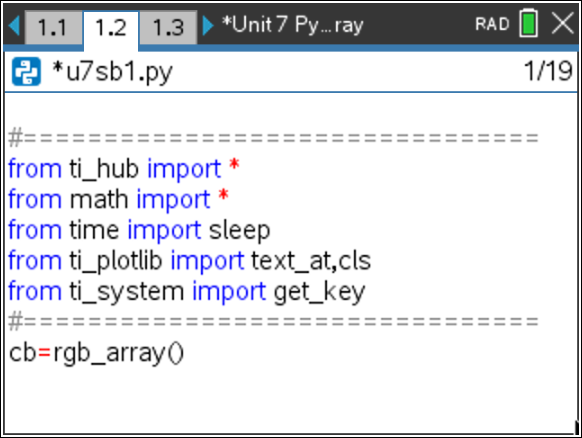
Step 3
-
To get the lights to light up all at once, on the next line of your program type your variable name followed by a period or decimal point.
cb.
A dialog box pops up in the editor showing all the methods (functions) that are available to the rgb_array() class. Your variable (cb) is an instance of that class (an object) and can use any of these class methods.
Select set_all(red, green, blue), as shown.
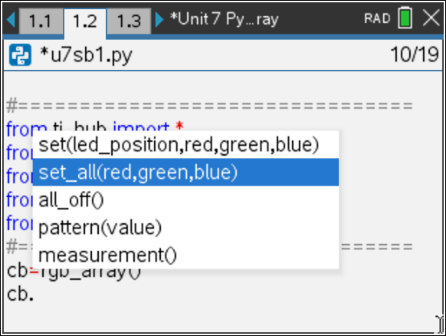
Step 4
-
Your statement consists of your variable name, a period and the function you selected from the pop-up list.
As with a lot of other commands in the Python menus, this one contains three inline prompts (red, green and blue) with a tool tip on each indicating the allowed values (0—255).
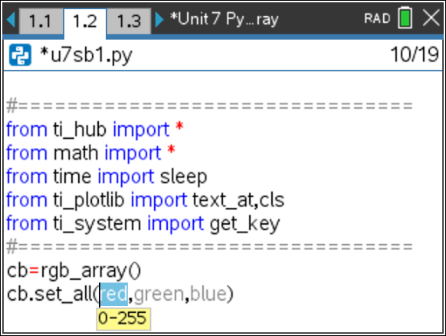
Step 5
-
Choose and enter values for the three colors.
Run your program and see that all 16 LEDs light up in your color.
Notice that the LEDs remain lit even after the program ends.
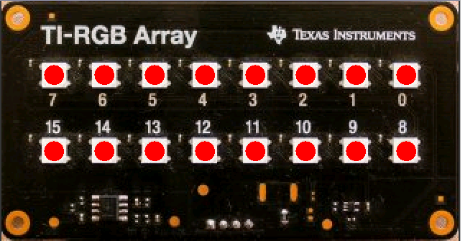
Step 6
-
To turn the LEDs off, use the statement:
cb.all_off()
Type your variable name and a period again and select all_off() from the list.
And, add a sleep(2) (seconds) between the lighting up and the shutting off; otherwise, you won’t see anything!
When you run the program now, the LEDs stay lit for two seconds.
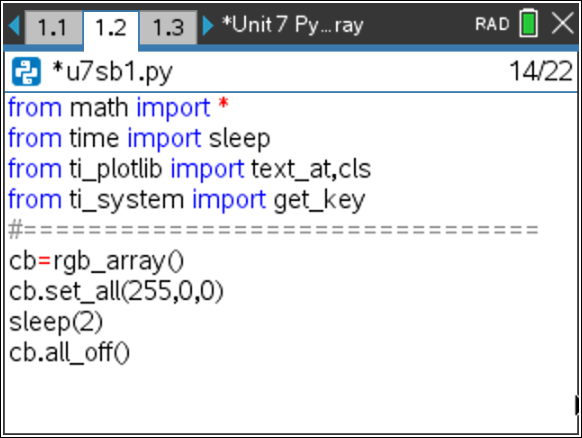
Step 7
-
Put your LED control statements into a for loop to make them blink on and off several times. Add another sleep() after they are turned off. You may want to adjust the sleep times to speed things up a bit. Be sure to indent all statements in the loop block.
Note: Do not include the constructor statement cb = rgb_array() in the loop block. It only needs to be defined once!
Run your program to test it before you continue.
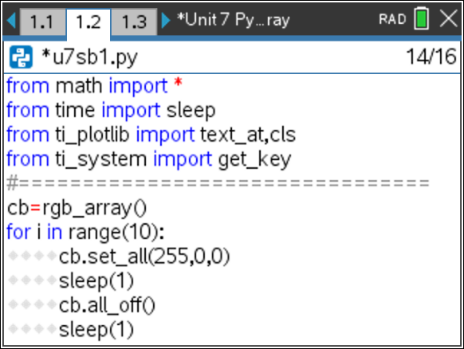
Step 8
-
If your program blinks all LEDs at once, then you are successful. Now let’s control the LEDs one at a time with an inner loop.
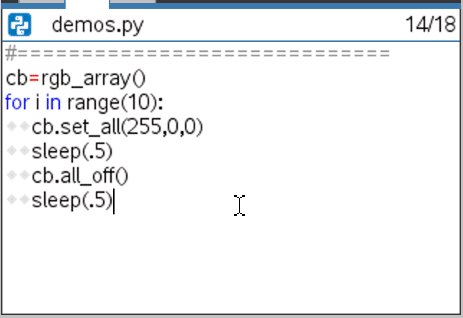
Step 9
-
Below the for i… loop, add an inner loop: for j in range(16):
Indent all four loop block statements so that they now apply to the inner loop.
Delete the statement cb.set_all(…), but leave the blank line. In its place, type
cb.
and select set(led_position, red, green, blue).
Use the inner loop variable j as the led_position and enter your color values.
Change the sleep values and the outer loop range() to speed things up a bit.
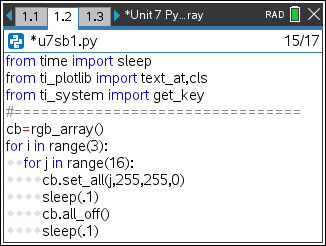
Step 10
-
Run your program. Now your 16 LEDs light up one at a time for three times.
Remember to save your document.
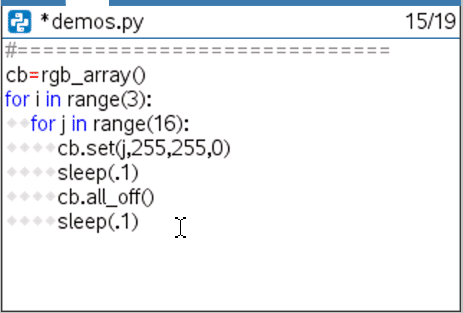
Skill Builder 2: A Rainbow of Color
Download Teacher/Student DocsIn this lesson, you will light up the LEDs in random colors.
Objectives:
- Import another module
- Use randint() to generate random LEDs in random colors
- Use esc to end the program
Step 1
-
Begin a new Python Hub Project.
Make a variable using the rgb_array() constructor by selecting it from:
menu > TI Hub > Add Output Device
Use the variable cb (for "circuit board") again.
Add the usual esc keypress loop from
menu > TI Hub > Commands
so that your program will loop until you press esc.
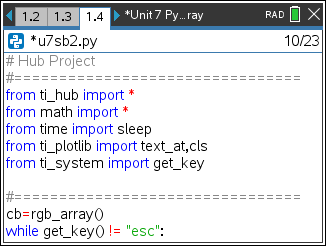
Step 2
-
Write four statements using randint() to assign values to variables representing the LED number (0…15) and the colors red, green and blue.
randint() is found in the random module which is not included in the import section at the top of your program. You will have to add it yourself. Find that import statement on menu > Random.
Try it yourself before looking at the next step.
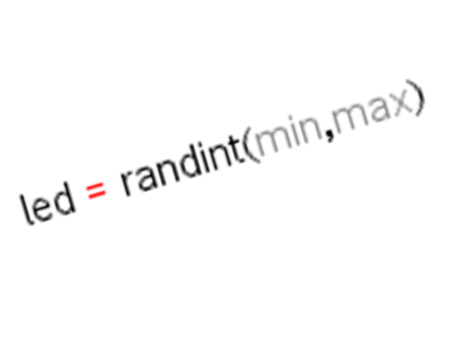
Step 3
-
Have the TI-RGB Array light up the random LED in the random color using:
cb.set(led, r, g, b)
Try your program now. If the lights are flashing too fast, add a sleep() statement after the cb.set() statement.
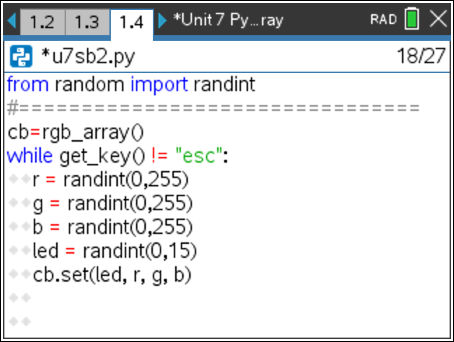
Step 4
-
At the end of your program, turn all the LEDs off:
cb.all_off()
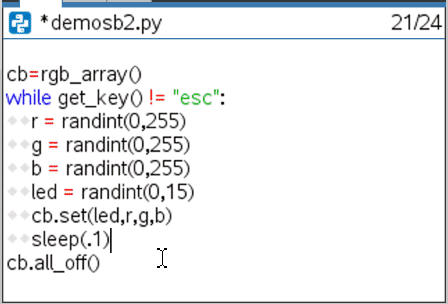
Skill Builder 3: Sequencing
Download Teacher/Student DocsIn this lesson, you will learn to control two LEDs at once in a loop to create a "marquee" effect.
Objectives:
- Use a for loop to light up a single varying LED
- Use a mathematics expression to control another LED at the same time
Step 1
The overhead sign outside of some movie theatres has a border of lights flashing in sequence like ants marching. You can create a similar effect on the TI-RGB Array by turning on and off lights in sequence.
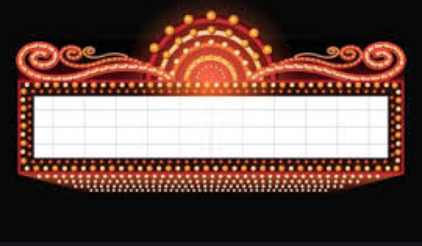
Step 2
Your program in this lesson will light up two LEDs at a time, one on the top row going from right to left (0 to 7) and one on the bottom row going from left to right (15 to 8). What is the relationship between the top row sequence and the bottom row sequence?
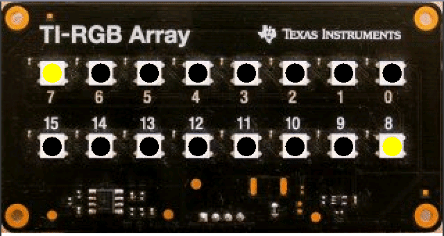
Step 3
-
Begin a new Python Hub Project.
Make a variable using the rgb_array() constructor and use the esc keypress loop as in the last lesson.
We use the variable cb again, but you are free to choose your own variable.
Add the statement
while get_key() != “esc”:
blockfound at menu > TI Hub > Commands.
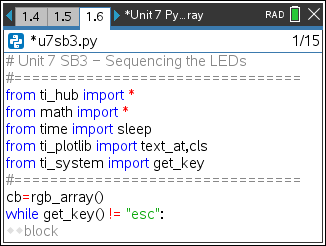
Step 4
-
Use a for loop to light up the top row in sequence from right to left (0 to 7).
for top in range (8):
cb.set(top, 255,255,0) (This is yellow.)Use the variable top because this controls the top row.
Remember that range(8) processes the numbers from 0 to 7.
Test your program now.
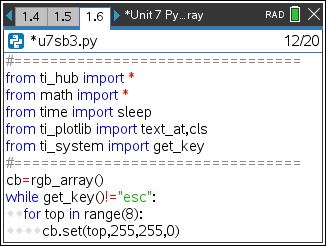
Step 5
-
All eight LEDs light up very quickly and, at the end of the program, all the top row LEDs are on.
Next deal with the bottom row. The bottom row must go from 15 to eight. What is the relationship between bottom and top?
bottom = ? ? ?
cb.set(bottom, 255, 255, 0)
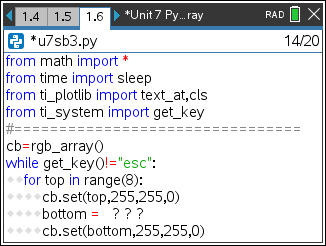
Step 6
-
Run the program now. All 16 LEDs light up very quickly. Add two statements: a sleep() statement to slow things down and a statement to turn all LEDs off at the bottom of the loop block.
sleep(.25)
cb.all_off()
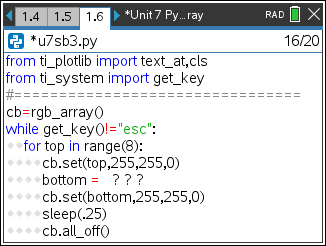
Step 7
-
Try your program again. Adjust the sleep() value and perhaps add another sleep() after all_off().
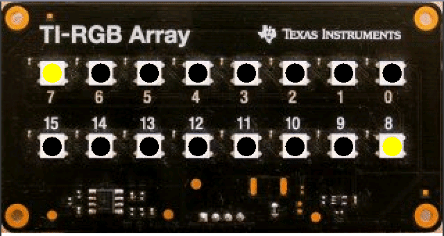
Application: Smart Lights
Download Teacher/Student DocsIn this application, you will control the number of LEDs lit on the TI-RGB Array using the TI-Innovator™ Hub’s brightness sensor.
Objectives:
- Use the brightness sensor to control the TI-RGB Array
- Adjust the brightness range to suit the TI-RGB Array
- Make sure that all 16 LEDs are impacted by the brightness
Step 1
Smart Lights
As the room darkens, the lights in the room get brighter. Imagine a "smart home" with no light switches! Write a program that monitors the brightness and turns on more or less LEDs, as necessary.
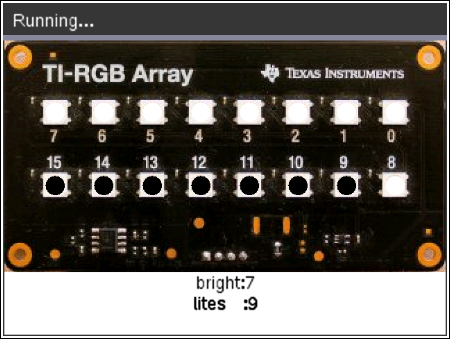
Step 2
-
As usual, begin this Python Hub Project using the rgb_array() constructor and the while loop to terminate the program with esc.
cb = rgb_array()
while get_key() != ”esc”:
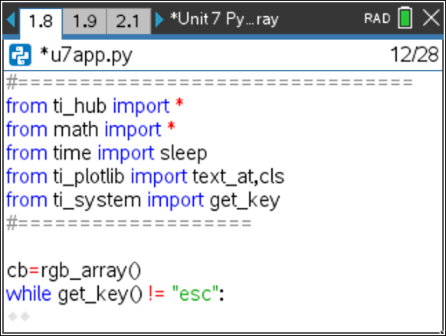
Step 3
-
Before the while loop, set the brightness.range() to match the number of LEDs on the TI-RGB Array board that could be lit: 0 to 16.
Press menu > TI Hub > Hub Built-in Devices > Brightness Input > range(min,max) for the statement:
brightness.range(0,16)
Use 0,16 because this is the range of the number of LEDs to light up on the board.
The maximum value the sensor will produce is 16. Is the minimum 0?
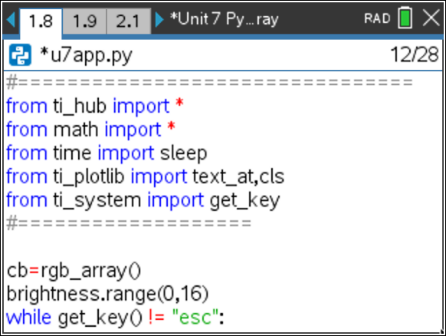
Step 4
-
In the while block, start by reading the brightness.measurement() and store the value in a variable (bright).
bright = brightness.measurement()
The function produces a floating-point number (float, decimal). Convert it to an integer value using:
bright = int(bright)
Or, combine the two statements into one operation:
bright = int(brightness.measurement())
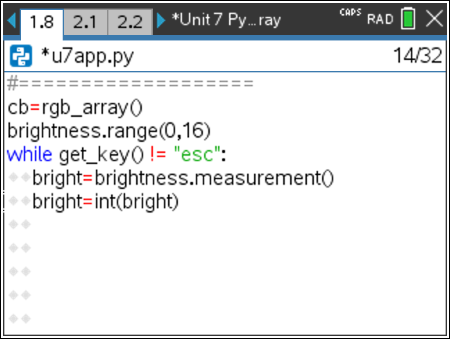
Step 5
-
To test your program: add text_at() statement found on menu > TI Hub > Commands:
text_at(7, str(bright), “left”)
Recall that you need str(bright) because the text_at() function requires a string to display. You can either type str() or get it from menu > Built-ins > Type.
Run the program to ensure that all seventeen values (0…16) do appear. If not, then adjust the range() so that they do. Try using an artificial light source such as a flashlight or the "flashlight" feature on a smartphone.
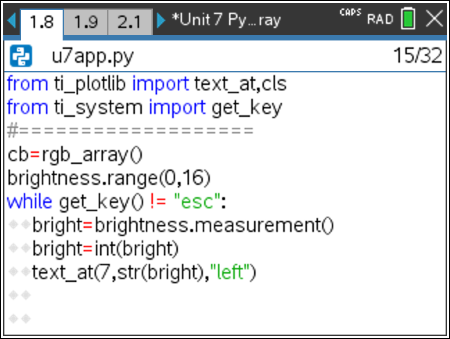
Step 6
-
Since 0 is the darkest value and 16 is the brightest, we want the number of LEDs lit to be the opposite: when bright = 0, there should be 16 LEDs lit and when bright is 16, there should be 0 LEDs lit.
Write an expression for lites in terms of bright.
lites = ? ? ?
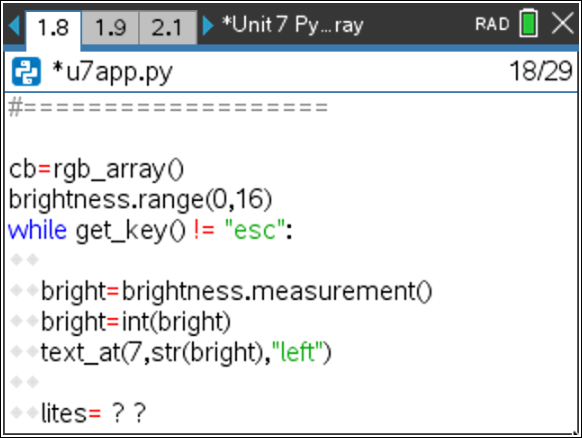
Step 7
-
It’s possible that all LEDs should be off:
if lites == 0:
cb.all_off()
else:
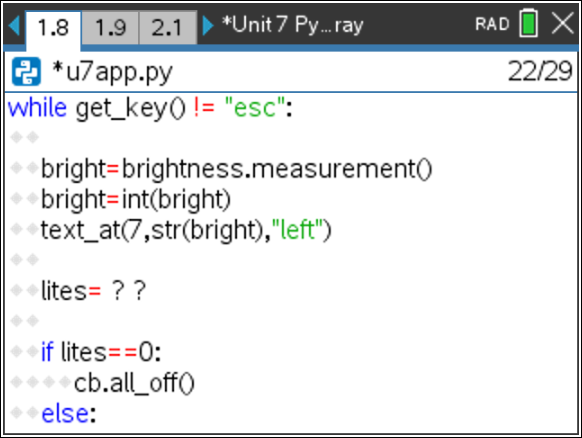
Step 8
-
We want all the LEDs to be affected by the brightness so we will use a for loop to control the state of every LED every time. The lites variable is a deciding factor when turning a LED on or off:
for i in range(1,17):
(Remember that the value 17 is not processed by the loop so i takes on the values from 1 to 16 representing the 16 LEDs.)
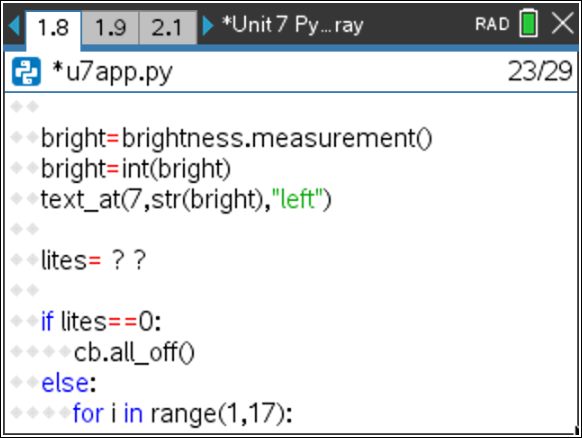
Step 9
-
Complete the program by adding an if…else… statement to tell the TI-Innovator™ Hub which LEDs are on and which ones are off.
Hint: If lites is 1, then you want to turn on LED 0. When lites is 16, you want to turn on all LEDs (#0 to #15). Use the color (255,255,255) to get a bright white light.
Remember to turn all the LEDs off at the end of the program.
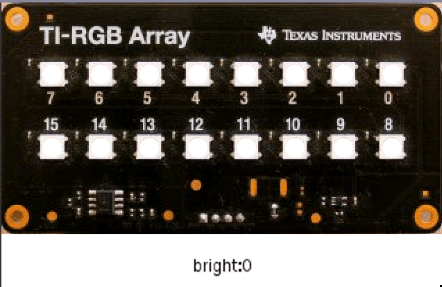
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application