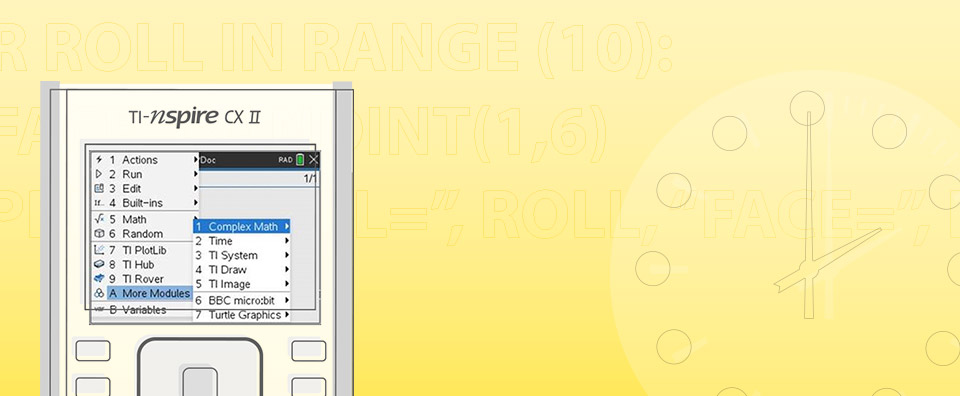
Python modules for TI-Nspire™ CX II graphing calculators
These short activities are designed to introduce the TI modules, and demonstrate various ways in which they can be used to extend the capabilities of TI-Nspire™ CX II technology.
Note: These lessons require the use of TI-Nspire™ CX II technology with OS 5.2 and above.
Overview
The TI Modules
The Python programming environments developed for the TI-Nspire™ CX II include some special modules developed by Texas Instruments. Some of these modules are accessible on the main menus (like ti_system) and others are found in special sub-menus: on the TI-Nspire™ CX II see [menu] More Modules.
The core Python programming language is small and fast and was designed to be ‘modular’ with separate, external modules, packages, and libraries. The TI modules tap the unique features of the Texas Instruments family of handheld computers, allowing graphics programming and access to external devices such as the TI Innovator Hub, Rover, micro:bit and other devices.
Some of these modules are introduced through the core Units of the TI Codes materials. This section of TI Codes provides some activities that introduce the functionality of these additional modules. As new modules are developed, this area will grow, so be sure to check back on occasion.
Turtle Graphics
Mini project 1: Getting started - Polygons
Download documentsTurtle Graphics is a TI-developed Python module ideal for folks new to graphics programming. Create simple or complex patterns, shapes, geometric forms and more in an entertaining, active, pseudo-physical learning experience. The current turtle module is v3.2.1. Future modules may have additional features.
Background: “Turtle Graphics” is a concept originally introduced into the programming language Logo created by Feurzeig, Solomon, and Papert in the mid-1960’s. The ‘turtle’ is a graphical object that responds to programming commands like forward(distance) and right(angle). The turtle module is object-oriented and the basic turtle functions are included on the turtle menus.Intro 2
In the image to the right, the small blue and yellow triangle represents the turtle object. The turtle carries a ‘pen’ that can leave a trail behind as the turtle moves. The black segments are the path that the turtle followed.
To use Turtle Graphics on your TI-Nspire CX II, you must have the turtle module turtle.tns in your PyLib folder on your device. The free turtle module and documentation, including installation instructions, are available here.
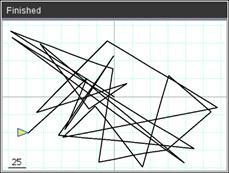
Step 1
Begin a new, blank Python program. Ours is named turtle1.
Press [menu] > More Modules > Turtle Graphics and get the statement
from turtle import *
You will also get the statement t = Turtle( ) in your code which creates a Turtle object named t.
If Turtle Graphics does not appear on the More Modules list, then the turtle module is not installed or there are too many items (> 20) for the menu to display. Go back a step, and review the directions.
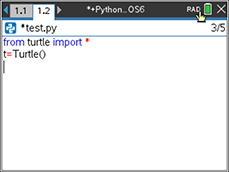
Step 2
See [menu] More Modules > Turtle Graphics again.
There are eight sub-menus (2..9):
Each contains specific turtle functions (and more). All turtle functions begin with object variable t. which is the default turtle name. You can change the turtle name (and even have multiple turtles in a program) but you are then responsible for editing the turtle name. The Move menu is shown here. Check out the items on the other menus.
Step 3
To get the turtle to make a square, write a for loop that contains just two statements:
for i in range(4):◆ ◆ t.forward(50)# pixels
◆ ◆ t.left(90 # degrees
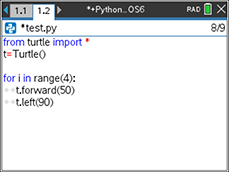
Step 4
After entering the code, run the program to see the turtle make a square.
The turtle starts at the origin facing to the right. ‘50’ is 50 pixels on the screen. The grid lines are 25 pixels apart as indicated by the legend in the lower left corner of the screen. ‘90’ represents 90 degrees.
At the end of the program, the turtle is back at the origin facing to the right. The graphics remain on the screen until you press a key.
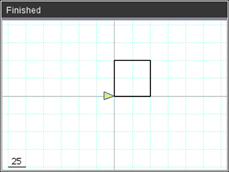
Step 5
Modify the square program to let the user enter the number of sides so that the turtle can draw a regular polygon. Use an input statement:
n=int(input(“num of sides? “))
and change the loop code.
The tricky part is to figure out what the turning angle should be when you know the number of sides. The user entered 7 sides to produce this screen showing a septagon.
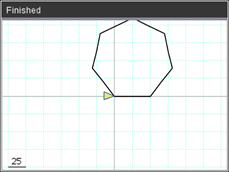
Step 6
t.speed(0) (fastest)
found on [menu] > More Modules > Turtle Graphics > Settings
The complete polygon code is shown to the right.
Note that the input statement must come before the t=Turtle() constructor because the constructor creates the graphics screen.
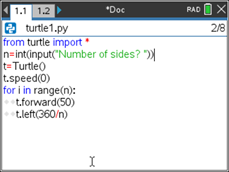
Step 7
Adding color:
There are two color methods on the Turtle Graphics menus:t.pencolor(r, g, b) (on the Pen control submenu)
t.fillcolor(r, g, b) (on the Filling submenu)
You can change pencolor( ) on the fly, so each side of the polygon can even be a different color (not shown).
But fillcolor( ) must be set before drawing a shape. See this red pentagon example in the next step…
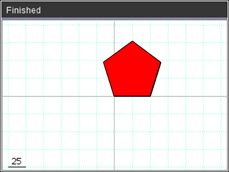
Step 7b
When selecting t.pencolor() from the Pen Control menu you are given a pop-up list of color names from which to choose. You may select one of these colors or… ignore the list (press [esc]), remove the quotes, and enter three integer values (red, green, blue) to establish a color:
Use either form of this statement:
t.pencolor(“red”)
t.pencolor(255, 0, 0)
Only the color “names” on the pop-up list are allowed, but any numeric values from 0 to 255 are permitted.
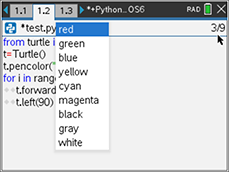
Step 8
Before making the polygon:
set the
fillcolor( ) (from the turtle Filling menu, with the same options
as pencolor)
and issue the
t.begin_fill() command also found on the Filling menu..
During the drawing loop, the system keeps a list of vertices visited.
after the polygon is drawn use the
t.end_fill() method to fill the polygon.
What value was entered for n to make the pentagon?
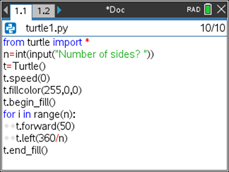
Step 9
Now modify the program to make randomly colored polygons at random places on the screen until the [esc] key is pressed.
- Use t.hideturtle() and t.hidegrid() to improve the appearance and speed.
- Use while get_key()!=”esc”: for the main loop.
- Use t.penup(), t.goto( , ), t.setheading(), and t.pendown() to place the turtle at a random starting point on the screen facing in a random direction with a random side length.
The turtle screen ranges from -159 to 159 (pixels) horizontally and -106 to 106 vertically and it’s OK if the turtle goes off the screen. The heading can be any angle (degrees). - Use the for loop to make each ploygon with a random number of sides.
Mini project 2: Stars and Fireworks
Download documentsAfter learning about the basics of ‘Turtle Graphics’ in the previous lesson, let’s try to make a more complex display of stars and fireworks!
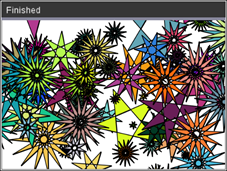
Step 1
Part I: Drawing Stars
When making polygons using the turtle, you use the Geometric property that the sum of the exterior angles of a polygon is 360 degrees. When making a regular polygon the turning angle is therefore 360/n since all the angles are equal.
In this first part of the activity, you will use a similar property to produce star polygons like the one shown at the right.
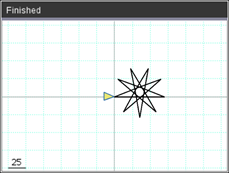
Step 2
First let’s take a look at the basic regular polygon program from the previous activity.
The program shown here makes a regular septagon.
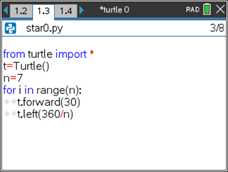
Step 2b
Run this program to make the regular septagon.
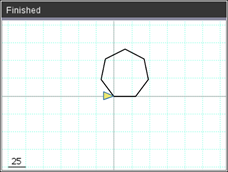
Step 3
Change the code to make a star…
In the turn function, instead of 360, use an integer multiple of 360.
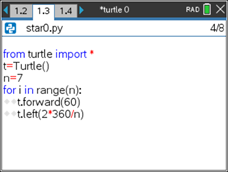
Step 4
You might try a higher multiple of 360 This star uses 3*360 instead of 2*360.
The multiple represents the number of revolutions that the turtle makes to complete the star. This is called a (7/3) star polygon since it has seven vertices and it takes three revolutions to complete.
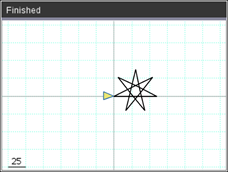
Step 5
When you add polygon filling to a star polygon, the results can be surprising.
Add t.fillcolor( , , ), t.begin_fill( ) and t.end_fill( ) to your program.
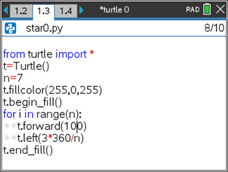
Step 5b
The entire star is not filled. There are places that the fill algorithm skips because it is looking for ‘borders’.
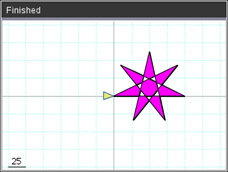
Step 6
Part II: The Fireworks!
In this section of the activity you will use lots of random values to generate these awesome fireworks on the screen.
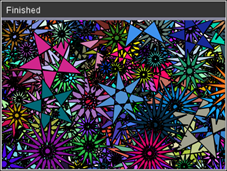
Step 7
In the star-making loop shown here n is the number of vertices and r is the number of revolutions. What values for n and r make for pleasing stars?
We will limit n to be random odd numbers: 5,7,9,11…For ‘pointy’ stars we will make r = int(n/2)
We will also use a random position, heading, color, and side length for each star in this project.
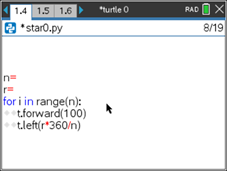
Step 8
First test these star-making options:
n = 2 * randint(2, 10) + 1 # odd numbers
r = int(n / 2) # or r = n // 2
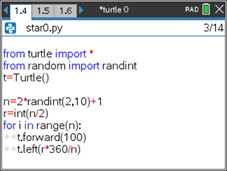
Step 8b
Running this program will make a pointy star with an odd random number of vertices.
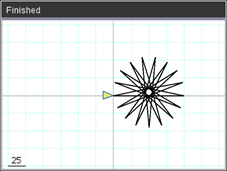
Step 9
Now add code to the program to:
- Hide the turtle
- Hide the grid
- Make the turtle move fast: t.speed( 10 ) (or 0)
- Put the star-making code in a while not escape(): loop
- Assign a random odd value to n
- Calculate r
- Draw the star using the for loop
- Clear the screen to see the different stars. This is temporary.
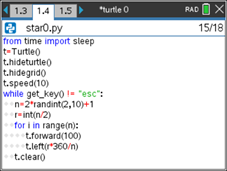
Step 9b
Test your program to see various stars displayed like this one.
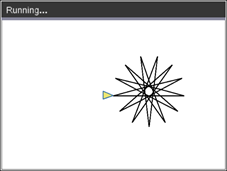
Step 10
We’re ready for the fireworks:
- each star will be drawn in a different position on the screen using a different heading with different colors and sizes.
- assign random values to appropriately-named variables to achieve the fireworks effect as seen here.
- #comment the t.clear( ) statement since we want to fill the screen with stars.
- Run the program and enjoy the show!
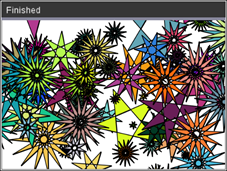
Mini project 3: Turtle races
Download documentsThis activity extends your turtle skills and concepts and demonstrates the use of multiple turtles competing in a ‘race’ across the screen.
Introduction: Two turtles are in a race to the finish line! Let’s write a program that simulates this race.
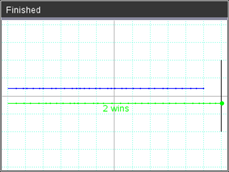
Step 1
Start a new Python program (turt_race.py) and add the turtle module to the code by using [menu] > More Modules > Turtle Graphics:
from turtle import *
Recall that selecting this statement from the menu actually pastes two statements into your Editor as seen here.
But this program requires two turtles. The line only creates one turtle object, t.
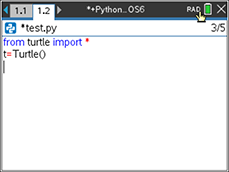
Step 2
Change the variable name of the turtle from t to t1. Copy and paste this line and name the second turtle t2.
t1 = Turtle()
t2 = Turtle()
When you select any turtle function from the menu you now have to modify the names of the turtles to suit this program.
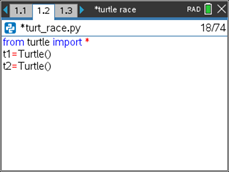
Step 3
Since the turtle window domain is -159 to 159, set the startline and finishline to be near the left and right edge of the screen:
startline = -150
finishline = 150
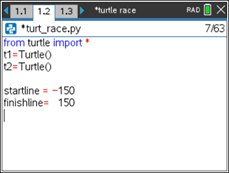
Step 4
Now do some turtle housekeeping: hide both turtles and set the speed of each turtle to the same value. Optionally, hide the scale in the lower left corner of the screen. Only one turtle needs to hide the scale.
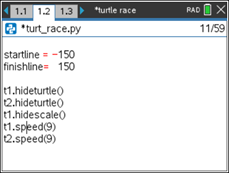
Step 5
Use one of the turtles to make the finish line:
# draw the finish line
t1.penup()
t1.goto(finishline,-50)
t1.pendown()
t1.goto(finishline,50)
t1.penup()
Remember that throughout this program, after selecting a turtle function from the menus, you must edit the turtle name by adding a 1 or 2 to the turtle name t. as necessary.
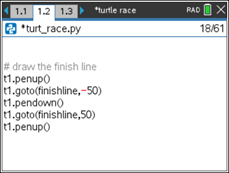
Step 6
You can test the program now to see that the finish line is showing on the right side of the screen as seen here.
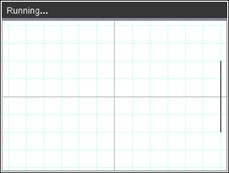
Step 7
Place the two turtles at the starting line and get them ready to race. Place one turtle above the x-axis and one below the x-axis Our two ‘lanes’ are y = 10 and y = -10. You can choose other lanes.
Make sure both turtle pens are up.
You can also set the pen color of each turtle. t.pencolor() is on Turtle Graphics > Pen Control
After the turtles have been moved, put the pens down again.
Think: in what direction are the (hidden) turtles facing? t.goto() does not affect the heading.
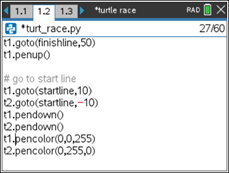
Step 8
We are ready to start the race…
while t1.xcor() < finishline and t2.xcor() < finishline:
This while loop ends when one of the turtles crosses the finish line. The function t.xcor() gives a turtle’s x-coordinate and is found on the Turtle Graphics > Tell Turtle’s state menu.
Remember to add the digits to the turtle names.
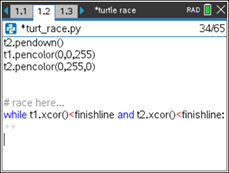
Step 9
Generate two random* values: d1 is the distance turtle 1 will move and d2 is the distance turtle 2 will move (in pixels). But they cannot both move at the same time! We let turtle 1 move first. (more about this later). Make a small dot at the turtle’s new position.
while t1.xcor() < finishline and t2.xcor() < finishline:
d1=randint(5, 12)
d2=randint(5, 12)
t1.forward(d1)
t1.dot(1)
*Remember to add from random import randint at the top of your program.
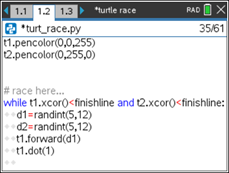
Step 10
Down the track, we make sure that turtle 1 has not crossed the finish line before letting turtle 2 make a move.
if t1.xcor() < finishline:
t2.forward(d2)
t2.dot(1)
This is the end of the while loop. Test the program again to see the turtles race across the screen.
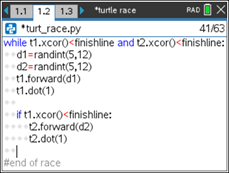
Step 11
After the whle loop ends, report the winner using an if…else structure:
if t1.xcor() >= finishline:
t1.penup()
t1.goto(-20,20)
t1.write('1 wins')
else:
t2.penup()
t2.goto(-20,-30)
t2.write('2 wins')
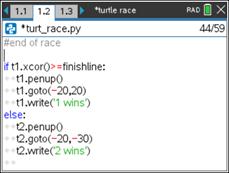
Step 12
Test your program now to see that both turtles can win and that the code is working properly.
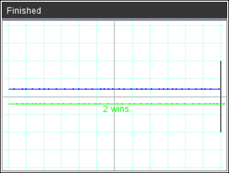
Step 13
Challenges:
- Is it ‘fair’ that turtle 1 always goes first? Build a routine that chooses a turtle to go first at random. Does it make a difference in the outcome? Should it be just the first move or every move that is random?
- Embed the setup functions and the race in another
while get_key() != ”esc”:
loop, change the turtle speeds to 0, keep track of the number of wins for each turtle and display the them on the screen. Let the program run for awhile to see if the race is ‘fair’. - Tortoise v. Hare
Tortoise takes small steps and the lazy Hare takes giant leaps. For every ten small (random) steps Tortoise takes, Hare makes one giant (random) leap. Create a race program between Tortoise and Hare in which each wins some of the races. Analyze your code as above to see how ‘fair’ your code is.
- MP 1
- MP 2
- MP 3
TI Draw
Mini project 1: Getting started - The Shield
Download documentsComputer graphics is the most fun of all programming projects. We introduce the ti_draw module by making the CapTIan’s Shield using some of the tools in the module. Be warned that this project uses some trigonometry, but can easily be revised to avoid the trig functions.
Our superhero, the capTIan, has the powerful shield seen here. Lets’ make the shield using Python and the ti_draw module.
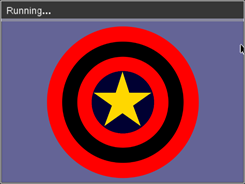
Step 1
Start a new program using the Type: Geometry Graphics template.
This template provides the import statement:
from ti_draw import *
which gives access to many drawing functions such as circles, segments, and rectangles.
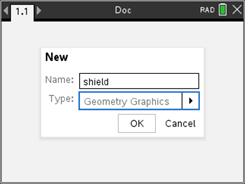
Step 2
The ti_draw module contains functions that let you create graphical images on your TI-Nspire CX II screen. The graphics ‘canvas’ is a separate display from the Python Shell and is activated when any of the ti_draw functions are processed. The canvas disappears at the end of the program.
Use [menu] More Modules > TI Draw to access all these graphics tools on two submenus: Shape and Control. Visit them now.
As long as the drawing ‘canvas’ is showing (even if the program is ‘Finished’), the TI-Nspire is locked. When the program displays ‘Finished’ at the top of the screen, press any key to close the canvas.
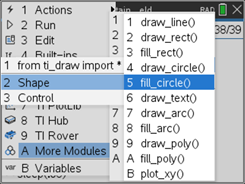
Step 3
The first two functions to use are set_window() and set_color(). Both are found on [menu] More Modules > TI Draw > Control
Add the arguments shown here:
set_window( -159, 159, -106, 106)
Since the screen is 318x212 pixels, this window places the origin near the center of the screen and makes each pixel represent one graphical ‘unit’.
set_color(100, 100, 100)
This function establishes the drawing color using three values: red, green, and blue, each between 0 and 255. (100,100,100) is a light gray.
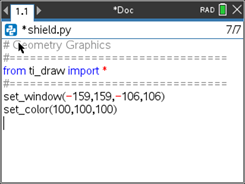
Step 4
To make the gray background, use the function:
fill_rect(-159, - 106, 318, 212)
found on [menu] More Modules > TI Draw > Shape
(-159, -106) is the lower left corner of the screen (remember our window setting). 318 is the width of the screen and 212 is the height of the screen. When using a window setting, width is measured left-to-right and height is measured bottom-to-top.
Run the program now to see the gray screen. Press any key to close the canvas and return to the Shell. Then press ctrl_leftarrow to return to your Editor.
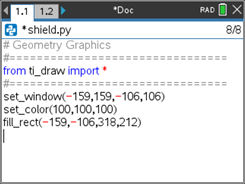
Step 5
Make the red and black circles next, from outermost to innermost so that the smaller ones cover part of the interior of the larger ones.
The centers of the circle are all (0,0) and the largest radius that will keep all the circles on the screen is 106 (the distance from the origin to the top and bottom of the screen. Our radii will be 100, 80, 60 and 40 for the ‘bulls-eye’ in the center.
First use set_color(r, g, b) to establish the color of a circle then use fill_circle(x, y, r) to make the circle.
set_color(255,0,0)# red
fill_circle(0,0,100)# largest circle
results in the red circle shown here.
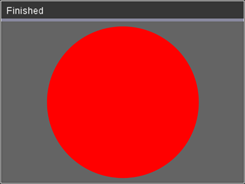
Step 6
Make two more circles: a black one and another red one, using radii of 80 and 60. Use set_color( ), too.
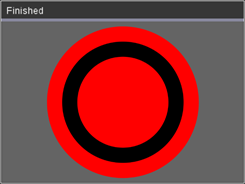
Step 7
Make a dark blue center circle with a radius of 40:
set_color(0, 0, 50)# dark bluefill_circle(0, 0, 40)# bulls-eye
The innermost circle here may appear black.
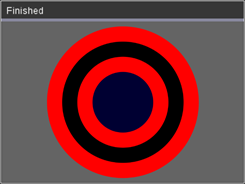
Step 9
Now comes the gold star, where Trigonometry comes into play!
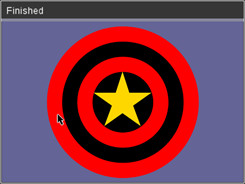
Step 10
The 5-pointed star shown here is a regular decagon (10 vertices, 10 sides). Five of the vertices are on the last circle you made. The other five vertices are all on a smaller circle shown here in gray. To make the star we will use fill_polygon() which takes two lists as arguments: a list of x-coordinates and a list of y-coordinates of the vertices of the polygon.
So, the next task is to make those lists…
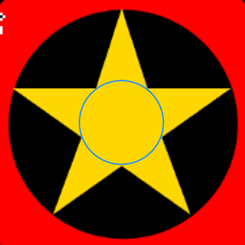
Step 11
Start with two empty lists called x and y:
x=[ ]
y=[ ]
The brackets are found on the ctrl-left_parenthesis key. We will add values to these lists next using a for loop:
for i in range(10):found on [menu] Built-Ins > Control
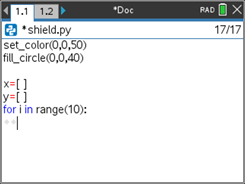
Step 12
Here is the complete polygon code.
I = 0 # angle measure
for t in range(10):
if t % 2 == 0:
x.append(40 * cos(i * pi / 180))
y.append(40 * sin(i * pi / 180))
else:
x.append(15 * cos(i * pi / 180))
y.append(15 * sin(i * pi / 180))
i += 36
fill_poly(x,y)
see the next step for the explanation…
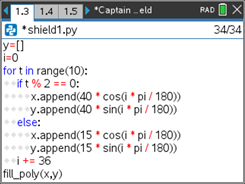
Step 13
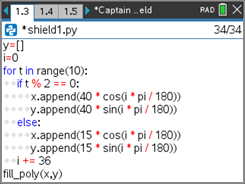
if t % 2 ==0:
x.append(40 * cos(i * pi / 180))
if t % 2 == 0: accounts for every other vertex being on a different circle.
x.append( adds a value to the end of the x-list.
40 is the radius of the outer vertices.
The variable i represents the angle, measured counter-clockwise starting from ‘east’. cos( i * pi / 180) and sin( i * pi / 180) calculate the x- and y-coordinates of the point on the unit circle that is i degrees around the circle. It is multiplied by pi / 180 to convert degrees to radians which is the domain of the Python trig functions.
Step 13b
Add from math import * at the top of your program to gain access to sin, cos and pi. At the bottom of the loop, the variable i is increased by 36 degrees (1/10 of 360). The else: block calculates the inner vertices that will have a radius of 15 instead of 40. Why 15? Try other values here to see the impact.
The for loop calculates the coordinates of all 10 vertices of the star and places them into the two lists x and y.
After the loop, fill_poly(x, y) draws the polygon. But… add a set_color() function just before the fill_poly() function.
We used gold:
set_color(255,215,0)
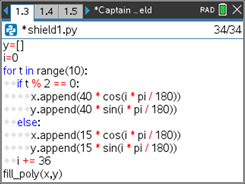
Step 14
When you run the program you will see the gold star in the center, but it is ‘tilted’ because the starting point is facing to the right. To get the star to point upward, change the initial value of i before the for loop begins to:
i = 90
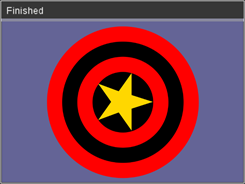
Step 15
Your star now points upward.
In the next section you will make the star spin!
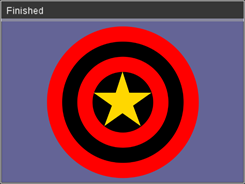
Step 16
Part 2: Spin the star!
Animation is produced in the CX graphics canvas by redrawing a section of the screen. Since the star is in the smallest circle it is only necessary to redraw that circle with the star in a different position.
We can accomplish this animation using the loopwhile get_key() != ’esc’:
found in the ti_system module so you will need to add
from ti_system import *
to the top of your program.
The while statement goes above the code for the innermost (blue) circle.
Indent the entire polygon routine to become the body of the while loop. Move the initial value of i=90 before this while statement.
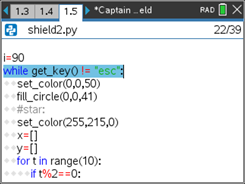
Step 17
After the polygon is drawn, add a small amount to the value of i. We use
i += 4
This causes the next polygon to start 4 degrees counterclockwise from the last one. You should try other values, too.
Perhaps add a sleep( ) function in the loop body to slow the rotation. If you do, remember to add from time import * to your program.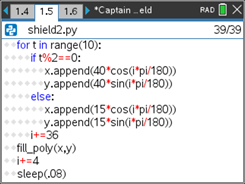
Step 18
If all is well, then the star spins counter-clockwise. Press [esc] to end the program.
Go back into the code and edit some of the numbers to see the effect each has on the capTIan’s shield.
Challenge:
- Can you make the star spin clockwise?
- Change the star to a six-point star.
- How about an n-point star?
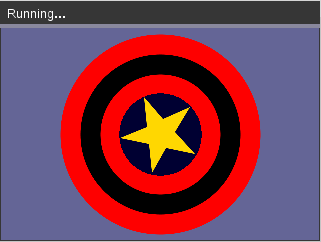
Mini project 2: Flags
Download documentsIn the first activity using the ti_draw module, you were introduced to the rectangle, circle and polygon tools. Here you will apply your drawing skills to make an image of a flag. Our demonstration makes the Texas flag, of course, for the home of Texas Instruments, Inc.
Most flags have a nice geometric design. Some flags are a little more complex. In this activity you will make the flag of the state of Texas, USA, since this is Texas Instruments, Inc. There are only three colors and the challenge is to make the Lone Star in the proper position. Your experience with the capTIan’s Shield will come in handy!
You can find most flag proportions and design specifications online.
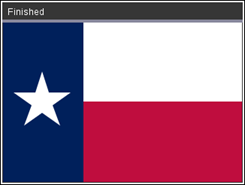
Step 1
Begin a new Python program using the Geometry Graphics template.
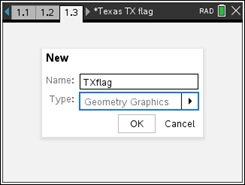
Step 2
We will use the default window which has the origin (0,0) in the upper left corner. All values here are pixels. The top right corner is (317, 0) and the bottom left corner is (0,211). When drawing a rectangle, the width is measured from left to right and the height is measured from top-to-bottom.
The rectangle shown here is made with:
draw_rect(50, 50, 100, 75)
(50,50) is the upper left corner. The width is 100 (to the right) and the height is 75 (down).
Remember that the screen is 318x212 pixels which means the aspect ratio is 3:2 (318 / 212). Many flags use this aspect ratio.
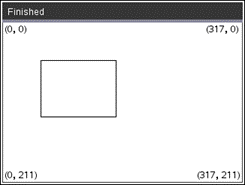
Step 3
Since the screen is white, begin the flag by drawing a red rectangle in the lower half of the screen:
set_color(255,0,0)
fill_rect(0,106,318,106)
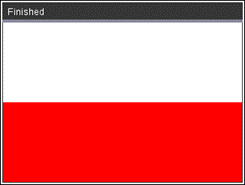
Step 4
For the blue field, try drawing a filled rectangle in the left one-third of the screen. Try it yourself before looking at the next step.
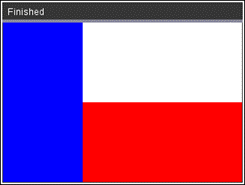
Step 5
We used:
set_color(0, 0, 255)
fill_rect(0, 0, 318/3, 212)
Note: Yes, you can use expressions in the function arguments.
It’s ok if you draw ‘off the screen’!
The proportions look right, but the colors are ‘off’.
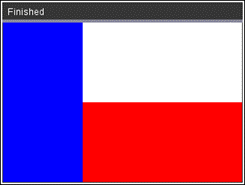
Step 6
Now for the fun part: the star!
If you completed the previous capTIan’s shield activity you can use the star-making code here. You will have to adjust the numbers used in the algorithm to place the star in the proper position with the proper dimensions.
Note that we have altered the colors here. You can do that at any time. You can find most flag design details online including dimensions, proportions, and colors.
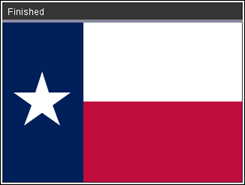
Step 7
Here is the complete star code from the capTIan’s shield activity:
x=[ ]
y=[ ]
i=0
for t in range(10):
if t % 2 == 0:
x.append(40 * cos(i * pi / 180))
y.append(40 * sin(i * pi / 180))
else:
x.append(15 * cos(i * pi / 180))
y.append(15 * sin(i * pi / 180))
i += 36
fill_poly(x,y)
Step 8
Adding the star code above to the Texas flag produces the image seen here. You can see a piece of the star in the upper left corner. It needs some adjusting to be placed in the proper position.
Translate the star to the middle of the blue rectangle by adding values to the x- and y-coordinates.
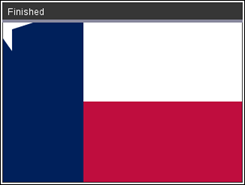
Step 9
We added 53 (half the width of the blue rectangle) to the x-coordinates and 106 (one half the height of the flag) to the y-coordinates:
if t % 2 == 0:
x.append(53+ 40 * cos(i * pi / 180))
y.append(106+ 40 * sin(i * pi / 180))
else:
x.append(53+ 15 * cos(i * pi / 180))
y.append(106+ 15 * sin(i * pi / 180))
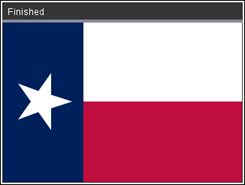
Step 10
To rotate the star so that it points straight up, change the initial value of the variable i as you did for the capTIan’s shield. Try it now.
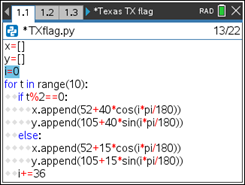
Step 11
i = -90
since the screen is coordinated ‘upside down’! The y-values increase from top to bottom in this ‘default’ window.
You task is to find (online) the appropriate shades of red and blue for this Texas (‘The Lone Star State”) flag.
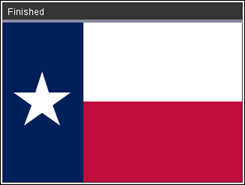
Step 12
Challenge: select a state, province, or country flag to design using your TI-Nspire CX II and your Python drawing tools.
For the flag of Canada, you will have to determine the coordinates of the vertices of the maple leaf polygon and then use fill_poly(x, y) to make the leaf.
The lists of the x- and y-coordinates are hidden in this image.
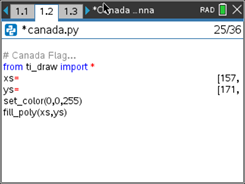
Mini project 3: Interactive art
Download documentsThe most versatile tool in the TI-Nspire™ CX II Python library is the get_key( ) function. In this activity you will make use of this function to make an interactive ‘Etch-A-Sketch’® style drawing program.
Using the get_key( ) function found in the ti_system module it is possible to make dynamic, interactive graphical applications (and games!). In this activity, you will build a simple drawing program that begins by using the four arrow keys and can be extended to make use of other keys for changing color, points style, making ‘stamps’ or other features.
“Etch-A-Sketch” is a registered trademark of SPIN MASTER LTD.
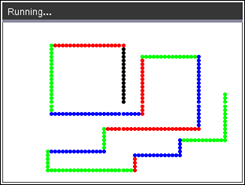
Step 1
Begin a new program using the Geometry Graphics template and import the ti_system module to use the get_key() function.
The first statement sets up a comfortable canvas coordinate system with (0,0) in the lower left corner and each pixel is one unit.
set_window(0, 317, 0, 211)
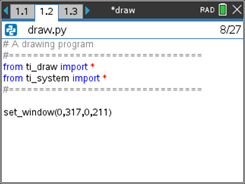
Step 2
Start in the center of the screen. Assign 159 to the variable x and 105 to the variable y. This can be done in a single line:
x, y = 159, 105
We usually use the statement while get_key() != ”esc”: but in this project we need to test the value of get_key() to see which key is pressed and act accordingly, so we will assign get_key() to a variable named key. First assign an empty string to the variable key:
key = “”# nothing in the quotes
Then write the while loop that terminates when key is “esc”.
while key != “esc”:
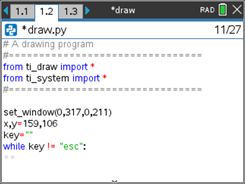
Step 3
In the loop body, plot the point (x, y) using the ti_draw function
plot_xy(x, y, 1)
key = get_key( )
Note that get_key( ) can also be written get_key(0).
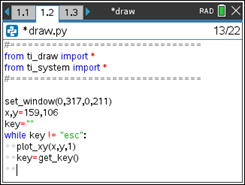
Step 4
You can run the program now to see a dot in the center of the screen. Press [esc] to end the program.
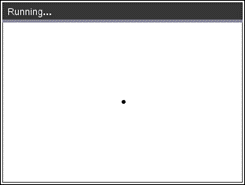
Step 5
Check the value of key and cause the point to be repositioned at a new location depending on the key pressed. The four arrow keys on the keypad are called “up”, “down”, “left”, and “right” and each one will impact either the variable x or the variable y.
The first of four if statements is:
if key == “right”:x += 1
You could also write x = x + 1 (or another number)
Write the other three if statements.
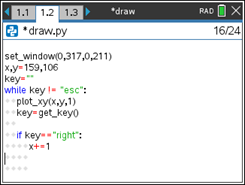
Step 6
Did you write these?
if key == "left":x -= 1
if key=="up":
y += 1
if key=="down":
y -= 1
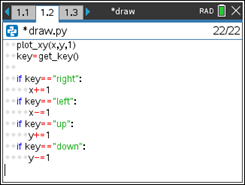
Step 7
Test your program now.
When you see the dot, use the four arrow keys to draw.
Again, press [esc] to end the program.
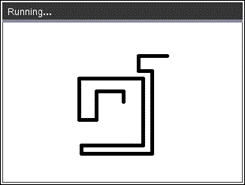
Step 8
Almost all keys on the keypad return a value like the arrow keys. All values are “strings”. For example, the [tab] key gives the string “tab”; and the [3] key becomes “3” in your code. The letter keys give lowercase letters. [shift] and [ctrl] do not give values because they are modifier keys that change the behaviors of some of the other keys.
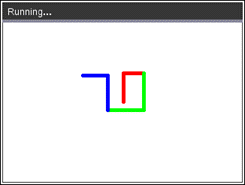
Step 9
You can use other keys to add features to your program. Many other features are possible. Just add an if statement to your program to incorporate the feature. Some possibilities:
- use “r”, “g”, and “b” and the set_color( , , ) function to change the drawing color to red, green, or blue.
- Use the “c” key to clear( ) the screen
- Use the “e” key to switch to erase mode (set the color to white).
- But you will need some other key to return to black. Your choice
- Add a key to make a stamp (“s”?). the stamp can be a polygon or a circle.
- Add a key to perform some random behavior (computer art?).
Add a Notes page in front of your program to explain all the keystrokes that your program uses.
Change the increment values in your code to make the point move further in each keypress as seen here.
- MP 1
- MP 2
- MP 3
TI Image
Mini project 1: Getting started - Image processing
Download documents“Image Processing” refers to the science of modifying some or all of the pixels in a digital image. An image is a rectangular arrangement of pixels in rows and columns. Each pixel is a color ‘tuple’ consisting of certain amounts of red, green, and blue, each value in the range 0..255. This first activity introduces the functions in the ti_image module and converts a color image to varying shades of gray.
Note: the program that you develop in this activity will be copied and modified in the second and third activities in this series, so it is important to complete this activity first.
Introduction: Image Processing
Changing a color image to shades of gray, flipping or rotating an image, resizing and making other ‘adjustments’ (brightness, contrast, etc.) to an image are all possible through Python programming with the right tools contained in ti_image and the right programming algorithms.
Note: The head of this python is gray. The rest is beige and green.
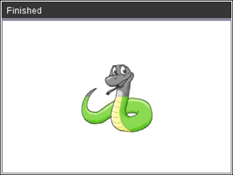
Step 1
The ti_image module included in the TI-Nspire™ CX II Python system allows you to perform many image processing functions. But there are more image processing functions than the ones found on [menu] Mode Modules > TI Image… shown here. They are accessed in a unique way as you will soon see.
This activity introduces these and the other ‘hidden’ image processing functions.
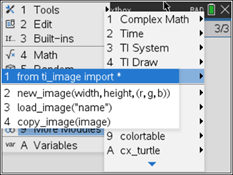
Step 2
We’ve chosen this picture of a python for our image processing projects.
Download the Image_Processing_Activities.tns file that contains this image and a ‘startup’ program for you to copy and modify for all the activities in the TI Image block. This file is used in all three of these TI Image activities.
Use any TI-Nspire CX II Computer Software to transfer the file to your handheld or use the free online TI-Nspire CX II Connect utility here.
When using this file for these Image Processing activities make a copy of the Python program. In the pythonimage.py Editor, press [menu] > Actions > Create Copy… and give the copy a new name.
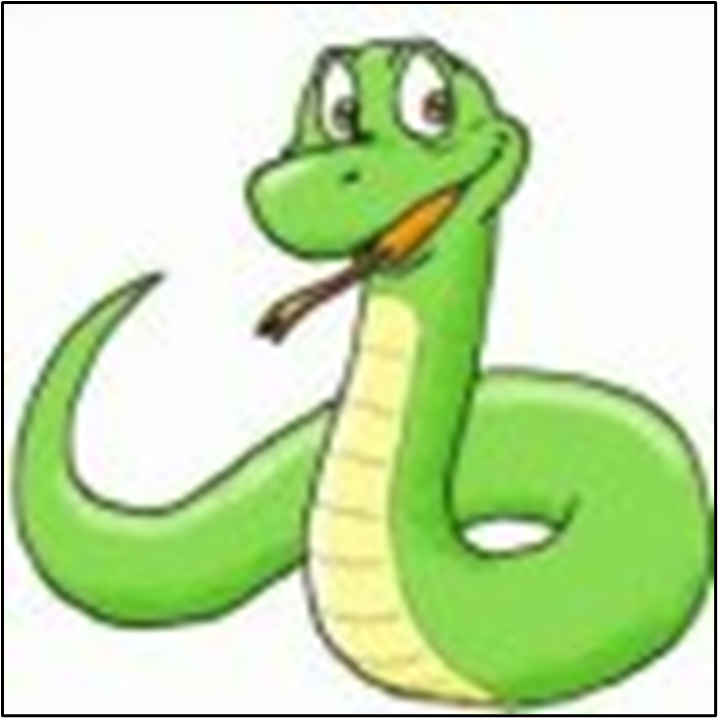
Step 3
A closer (zoomed in) look at the image shows the individual pixels (colored squares) that make up the image
The image must reside in a Notes app and have a name…
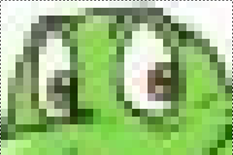
Step 4
To name an image, [right-click] (press [ctrl]-[menu] on the handheld) on the image in the Notes App and select ‘Name Image’. We named the image in the sample file ‘python0’.
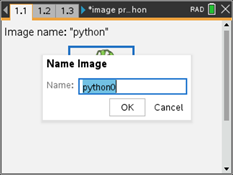
Step 5
You are now ready to write a program that manipulates the python0 image. Start a new Python program (ours is named ‘grayscale0’) and for the Type: select Image Processing from the list of templates.
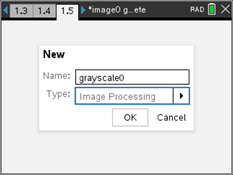
Step 6
The template imports the ti_image module and the function get_screen_dim() from the ti_draw module.
First get the width W and height H of the screen using:
W, H = get_screen_dim()These two values will come in handy when positioning the image on the graphics canvas.
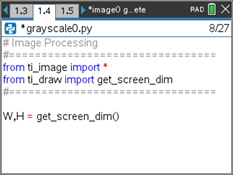
Step 7
Now you are ready to work with the image. Press [menu]> More Modules > TI Image and select load_image(“name”). Type a variable name in place of ‘var’ (we use img) and move the cursor onto the “name” place-holder and select your image from the pop-up menu. (The pop-up list shows all images in the document.)
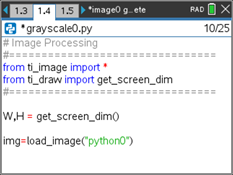
Step 8
Here’s some Editor ‘magic’: now that there is an image variable in the code, type the name of the variable (ours is img) followed by a period (.). A pop-up menu appears (shown here) with all the additional functions that are available for image processing.
w and h are the width and height of the image in pixels. These are very useful values for several reasons. Store them in two more program variables:
w = img.w
h = img.h
Note: now there are four distinct variables: W, H – the screen dimensions and w, h – the image dimensions. Remember that Python is case-sensitive.
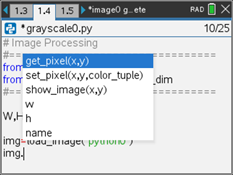
Step 9
Print the width and height of the image and run the program.
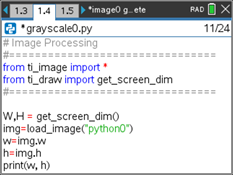
Step 10
Our python0 image is 100x100 pixels. That’s a total of 10,000 pixels! Image processing will take some time. Larger images will take longer to process.
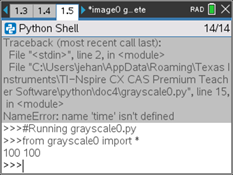
Step 11
Back to the program…
To display the image on the screen use img.show_image(x, y).
x and y are the position of the upper left corner of the image.
To center the image on the screen (regardless of the size of the screen and the image) use your defined variables:
img.show_image((W-w)/2, (H-h)/2)
To write this statement type img followed by a period (.). Select show_image( ) from the menu that pops up.
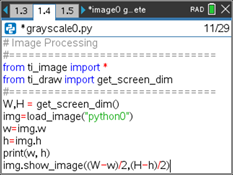
Step 11b
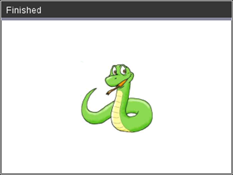
Step 12
Make a new image variable (img2) the same size as the original:
[menu]> More Modules > TI Image > new_image…
Use w and h for the width and height and (0,0,0) (black) for the color of each pixel.
img2 = new_image(w, h, (0, 0, 0) )
This variable will contain our grayscale image.
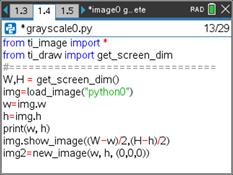
Step 13
Use two (nested) for loops to get the color of each pixel in the image.
The outer loop processes each row of pixels in the image. The inner loop processes each i pixel (column i) in row j.
The value returned by .get_pixel() is a tuple of three values (red, green, blue). By assigning them all to one variable we are creating a Python ‘tuple’ (similar to a list, but not quite the same thing). The three color elements are addressed by using c[0] (red), c[1] (green), and c[2] (blue).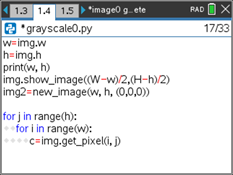
Step 14
To convert the image to grayscale, one option is to simply average the three color values and set all three of the new pixel’s colors to that average.
Try it yourself before seeing the next step.
Note: due to the human eye’s perception of colors, there are other options (‘weighted averaging’) for computing the value of the grays in a color image.
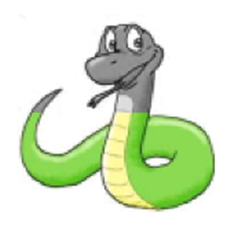
Step 15
Calculate the average of the three colors in the pixel:
avg = (c[0] + c[1] + c[2]) / 3Type img2. and select
img2.set_pixel( , , )Complete the three arguments using:
img2.set_pixel(i, j, (avg, avg, avg))Tip: you could also use avg=sum(c)/3.
sum( ) is found on [menu] Built-ins> Lists
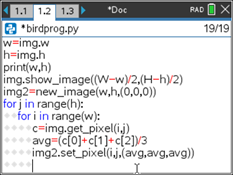
Step 16
After the two loops end, display the new image:
img2.show_image((W-w)/2, (H-h)/2)
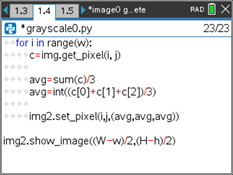
Step 17
Run the program. For a large image it can take quite a while to process the entire image… be patient.
Since all three color channels of each pixel are the same value, the image is displayed in various shades of gray: That’s grayscale!
If you move img2.show_image( ) inside the outer for loop you will see the transition from color to grayscale but this also slows the processing a bit.
Challenge: Can you show the color image and the grayscale image on the same screen (side-by-side)?
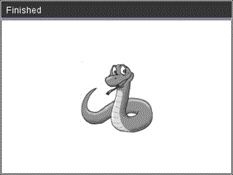
Mini project 2: Image transformations
Download documentsIn the “Getting Started…” activity you downloaded a working TI-Nspire document found at Image_Processing_Activities.tns. Make another copy of the startup code (pythonimage.py) in the document to work on this project, too.
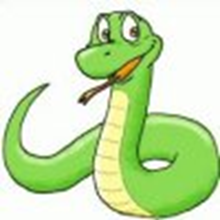
Step 1
To work with your own image, after inserting an image into your TI-Nspire document (on a Notes app), it will appear like this.
Name the image by right-clicking (press [ctrl]-[menu] on the handheld) the image and selecting ‘Name Image’. Use that name in your Python programs.
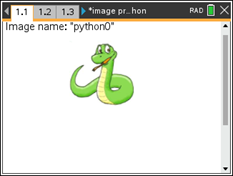
Step 2
To flip an image (vertically) or mirror an image (horizontally), get the value of each pixel and place it in a new (opposite) position in a new image. Let’s flip an image first.
Use the ‘generic’ image processing code provided in the document to load and show the image.
Use the same nested for loops for the rows and columns.
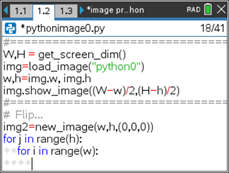
Step 3
‘Flip’ means to transfer pixels between top and bottom. The top row is row 0 and the bottom row is row (h-1). Python counting always starts with 0.
In general, row j from the top of the old image moves to row (h-1) - j from the bottom in the new image.
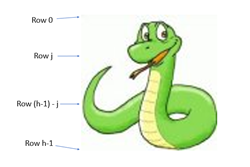
Step 4
We can perform this transformation in just one or two statements: get the value of pixel (i, j) from the original image and place it in position
(i, (h-1) – j) in the new image:
c =img.get_pixel(i, j)
img2.set_pixel(i, (h - 1) - j, c)
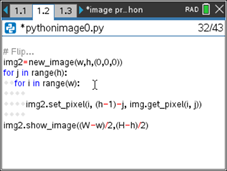
Step 4b
These two statements can be combined into just one statement:
img2.set_pixel(i, (h-1)-j, img.get_pixel(i, j))
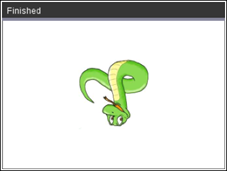
Step 5
To mirror the original image (left <-> right), perform a similar operation on the columns instead of the rows.
To show both images at once just change the positions in
img.show_image( , )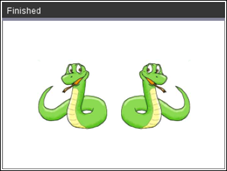
Step 6
Challenge 1: Efficiency
Perform the transformation (flip or mirror) using only one image variable, not two. Note that this does require modifying the original image, but only within the program, not the actual image in the Notes app of the document. Would the program perform faster?
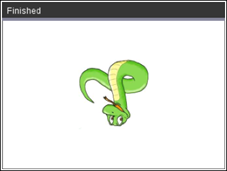
Step 7
Challenge 2: Rotate
Use the same image processing technique to rotate the image.
Hint: row i from the top of the original image moves to column i (from the left) in the new image working from bottom to top.rowcolrowcol
Pixel( i, j ) ⇒ ( j, (h – 1) - i ).
This will rotate the image 90 degrees counter-clockwise. You can also rotate 180 degrees (that’s not the same as flipping!) or 90 degrees clockwise.
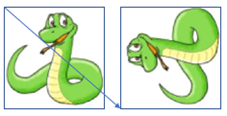
Mini project 3: Colors and slides
Download documentsIn this third activity, you will ‘extract’ the three colors from a color image and produce a slideshow of the four distinct images.
All computer images consist of just three ‘primary’ colors: red, green, and blue. Since each of these color values can vary from 0 to 255, there are over 16 million colors that can be produced (256**3). One of the weird results of mixing light wavelengths together is that the color (0, 0, 0) is ‘black’ (the absence of light) and (255, 255, 255) is ‘white’ (the presence of all three channels at their fullest). So merging the red, green, and blue images (shown here) makes the original image of the python in the upper left. This activity does the reverse: ‘extract’ the three colors channels and display this screen. Part 2 of the activity will demonstrate the ‘slideshow’ capabilities of image processing.
Step 1
In the “Getting Started…” activity you downloaded a working TI-Nspire document found at Image_Processing_Activities.tns. Make another copy of the startup code (pythonimage.py) in the document to work on this project, too.
But show the original image in the upper left corner of the screen using: img.show_image(0,0) and….
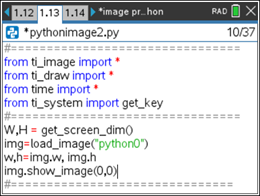
Step 2
Create three new images, one for each color channel:
img1 = new_image(w, h, (0, 0, 0))
img2 = new_image(w, h, (0, 0, 0))
img3 = new_image(w, h, (0, 0, 0))
Use the same nested for loops as in the other activities, but use three distinct variables to retrieve the three color values of each pixel separately:
r, g, b = img.get_pixel(i, j)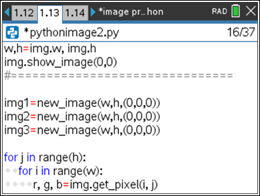
Step 3
Place each of the three colors into the separate images:
img1.set_pixel(i, j, (r, 0, 0))
img2.set_pixel(i, j, (0, g, 0))
img3.set_pixel(i, j, (0, 0, b))
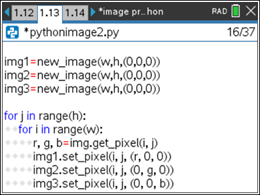
Step 4
Add the show_image() functions in the outer for loop to see the three color images being produced on the screen in three different positions:
img1.show_image(159, 0)
img2.show_image(0, 106)
img3.show_image(159, 106)
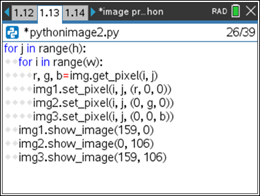
Step 5
Run the program to see the three separate color images produced like this. Are you surprised that the white area of the original image turns red, green, and blue in the separated images? Think about that.
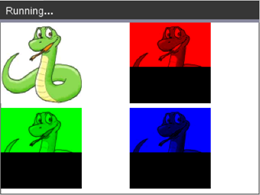
Step 6
Part 2: The Slideshow
Now that your program has four images stored, you can create a ‘slideshow’ or ‘movie’ by displaying the images in the same position, one after the other in a continuous loop.
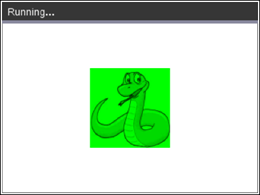
Step 7
Separate the first part of the program from the second using a message and the function get_key(1) found in the ti_system module. get_key(1) acts as a pause statement that responds to ‘Press any key’.
# Part 2:
draw_text(20, 50, "Press a key...")
get_key(1)
# Slideshow...
These statements are not part of any previous loops so they are not indented.
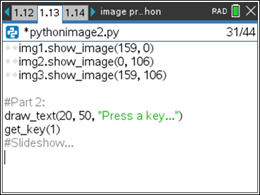
Step 8
To clear the screen, use the clear( ) function from the ti_draw module. For the import statement you can write
from ti_draw import clear
to import only the clear() function.
Make a list of the images in your slideshow:
slides = [img, img1, img2, img3]
i = 0
And use the
while get_key() != “esc”:
loop found in ti_system.
Remember to import get_key from the ti_system module.
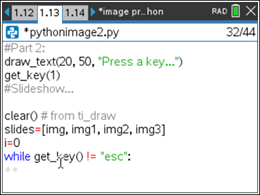
Step 9
Display image I from the list of slides
slides[i].show_image((W-w)/2, (H-h)/2)
Recall the expressions for centering the images on the screen.
Pause processing for a moment using sleep(n)
sleep(1) # edit the sleep time for speed.
Increment the slide counter and use % 4 to just use the values 0, 1, 2, and 3.
i = (i + 1 ) % 4
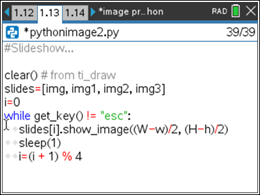
Step 10
Run the program. The first part generates the three color-separated images. The second part displays the four images in a slideshow.
Challenge: Make a copy of the program and, in the second program, mix two of the three primary colors together: (red and green), (green and blue), and (red and blue) and see the color effects produced in the three images (not shown).
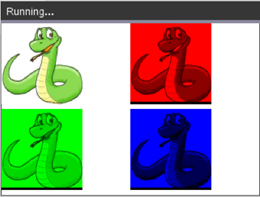
- MP 1
- MP 2
- MP 3
TI PlotLib
Mini project 1: Getting started - Coin toss
Download documentsThis activity introduces the TI PlotLib module that is used for plotting points and graphing data sets (scatter plots).
Introduction: Included in the TI-Nspire CX II Python system, the TI PlotLib module contains statements and functions used for the graphical plotting of data sets (pairs of lists), individual points, lines, and text. This activity introduces the module through a coin-tossing (percent heads) simulation.
The TI PlotLib Draw menu is shown here. There is also a Setup menu that prepares the screen for graphing.
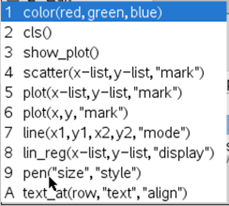
Step 1
Coin Tossing: When tossing a fair coin, approximately half of the tosses will be heads. If you toss the coin just a few times the likelihood that there will be an equal number of heads and tails is small. But as the number of tosses grows this ratio improves towards our expectation.
This activity creates an interactive simulation of coin tosses and displays a growing scatter plot of the percentage of the outcomes that are heads.
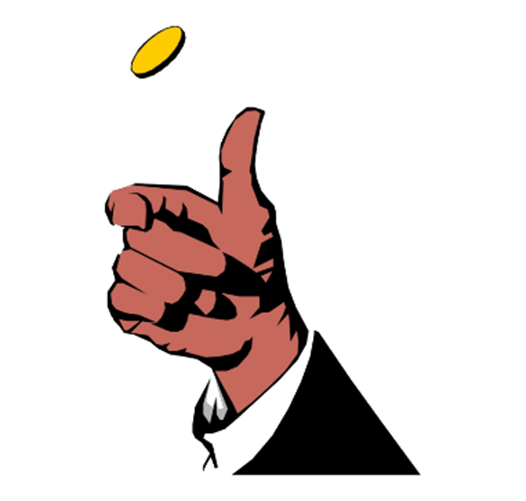
Step 2
Begin a new Python program (we called it ‘coins’) and select the Type: Plotting (x,y) & Text template from the dropdown list. This template provides the unique import statement:
import ti_plotlib as plt
along with several ‘Setup’ functions.
This type of import statement requires that all ti_plotlib functions be preceded by the alternate module name plt. In Python-ese this is called ‘aliasing’ the module (give it a different, usually shorter name). When selecting ti_plotlib functions from the menus, they will include this name at the beginning of the function as seen in the first three statements provided.
Note: these three statements, if used, must be listed in this order since each one paints the canvas (screen) over the previous one: first set the window, then draw the grid, then draw the axes on top of the grid.
Step 3
Add two more import statements at the top of the program:
from random import *
from ti_system import *
random contains the randint(,) function to simulate tossing a coin.
ti_system contains the get_key() function for monitoring keypresses.
coins is the number of coins to toss at each keypress.
10 can be changed later.
tosses is the total number of tosses.
heads is the number of heads tossed.
xs and ys are two empty lists that will store the toss numbers
and the percentage of heads each that toss.
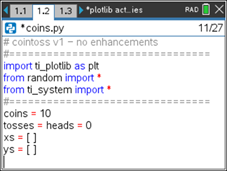
Step 4
The main program consists of a loop that ends when [esc] is pressed. Usually this is done with the statement: while get_key() !=” esc”:
found on [menu] More Modules > TI System, but…
We want this program to pause and wait for a keypress before tossing the next batch of coins.
Use the number 1 as the argument of get_key( 1 )
Note: get_key() and get_key(0) do not pause the program but rather check the keyboard buffer to see if a key was pressed since the last execution of the function.
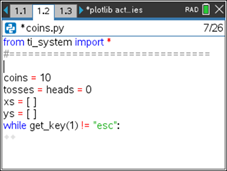
Step 5
Toss the coins using a for loop:
In the loop body, begin by counting the toss:
tosses += 1
Flip the coin using randint(0,1) we add this value to the heads variable so that heads is incremented when the result is 1.
heads += randint(0,1)
This is much more efficient than an if statement!
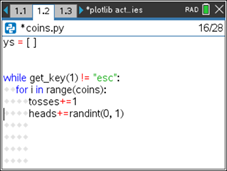
Step 6
frac = heads / tosses
The result will be a decimal between 0 and 1.
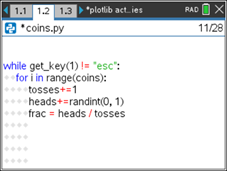
Step 7
Add the values of tosses and frac to the two lists:
xs.append(tosses)
ys.append(frac)
.append( ) is in [menu] Built-ins > Lists
This completes the coin-tossing loop. Move on to the plotting functions you started with…
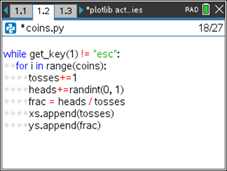
Step 8
Set the window:
plt.window(0, tosses, 0, 1)
Set the grid:
plt.grid(10, 0.25, ”dotted”)
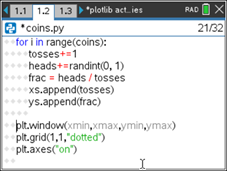
Step 9
Here are the modified setup functions.
Now add the actual plot function:
plt.scatter(xs, ys, ”o”)
for x-list use your list xs
for y-list use your list ys
for the “mark” select the “o” (large) dot.
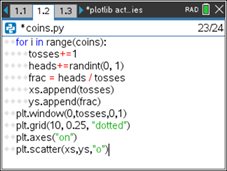
Step 10
We’re done! Run the program.
At the Shell, press a key. You will see a plot of the first 10 coin tosses. Remember that the x-axis represents ‘toss number’ and the y-values are the percentage (in decimal form) of the number of heads tossed. The first one will be either at the top or bottom of the screen (either a head or a tail). There may be more than one.
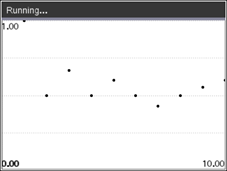
Step 11
Press the key again… and again… and again. As more coin tosses are added to the simulation the plot gets longer, but the screen is ‘squeezed’. The number in the lower right corner is the number of tosses. Also, notice that the vertical grid lines are getting closer together: this will cause a problem soon…
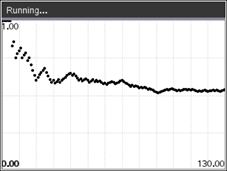
Step 12
When the number of tosses gets ‘large enough’ you may encounter the ‘invalid grid scale’ error shown here.
We got this error quickly by changing coins to 500 at the top of the program.
Prevent this error by either:
- Comment the #plt.grid() statement in the program
- Edit the grid so that the grid lines do not get too close together:
plt.grid(tosses / 10, 0.25, “dotted”)
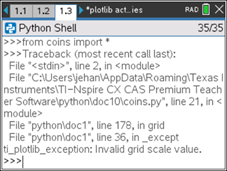
Mini project 2: Sequences
Download documentsThis activity demonstrates plotting mathematical sequences using the TI PlotLib module in two different ways: by plotting individual points and by plotting lists.
Part 1 - Start with an arithmetic and a geometric sequence in a single program. Begin a Python program using the ‘Plotting (x,y) & Text’ template from the ‘Type:’ dropdown list. Our program is called seq1.
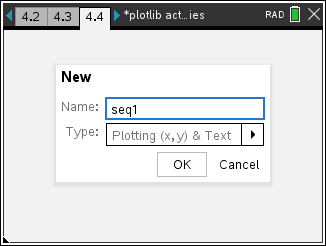
Step 1
As explained in the Getting Started activity and worth repeating here, this template provides the unique import statement:
import ti_plotlib as pltalong with several ‘Setup’ functions.
This type of import statement requires that all ti_plotlib functions be preceded by the alternate module name plt. This is called ‘aliasing’ the module (give it a different, shorter name). When selecting ti_plotlib function from the menus, they will include this name at the beginning of the function as seen here in the first three functions provided.
Note: these three statements, if used, must be listed in this order since each paints the canvas (screen) over the previous screen. First set the window, then draw the grid, then draw the axes on top of the grid.
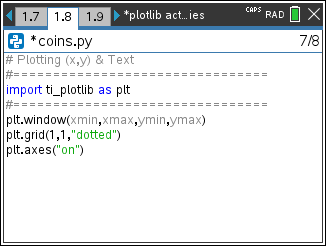
Step 2
Use four ‘window variables’ to set the plt.window because you might need these values elsewhere in your code.
xmin, xmax, ymin, ymax = -5, 30, -5, 100
We’re placing the origin in the lower left corner of the screen because we will be plotting points in the first quadrant.
Having the four window variables assigned on a single line makes them easier to locate & edit and saves vertical space in your program.
The plt.grid((1, 1, “dotted”) values can be edited as needed. Change the y-scale value from 1 to to 10. There are limitations on the window and these grid values may interfere with the run of your program.
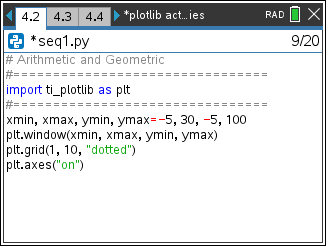
Step 3
Plot both an arithmetic and a geometric sequence in the same graph to compare them. Use a for loop that makes use of the window variable:
for n in range(xmax):
Note: change the loop variable from i to n.
In the loop body, first assign two variables the value of an arithmetic/linear term like 3n and a geometric/exponential term like 2n
a = 3*n# arithmetic
b = 2**n# geometric
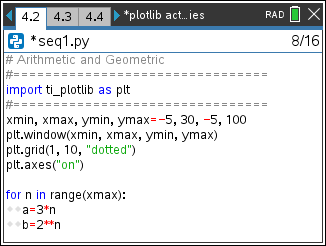
Step 4
Plot each term using a different plot style (“mark”):
plt.plot(n, a, "+")plt.plot(n, b, "o")
Get plt.plot(x, y, “mark”) from [menu] TI PlotLib > Draw
Note: There are two plt.plot( ) functions on the menu. One is for plotting lists and the other is for plotting points.
The plot “mark” can be changed (by hand) and is limited to “.”, “+”, “x”, or “o”.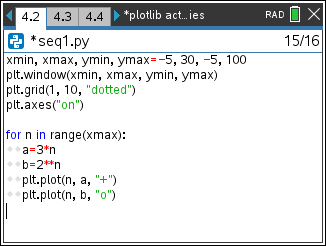
Step 5
Run the program and you should see the two plots. One is the graph of points on a line and the other quickly disappears off the top of the screen. Which is which?
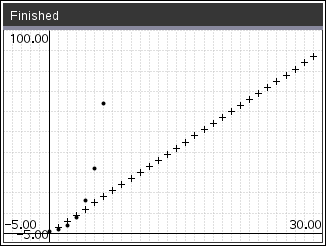
Step 6
Try changing the window settings to see different views of these plots. Depending on your window values you may encounter the error seen here: We changed xmax to 100 and got the ‘invalid grid scale’ error because the grid lines would be too close together.
Either:
- change the plt.grid( ) x-scale value to a larger number (like 10) or
- #comment the plt.grid( ) function to hide the grid.
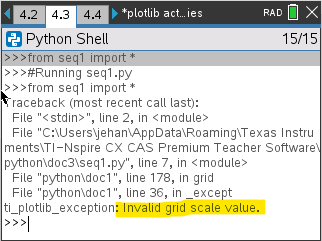
Step 7
But even using plt.grid(10, 10, ”dotted”) still produces another error! Plotting limits values to be between -2,147,483,648 and 2,147,483,647 (that’s 2**31 - 1).
This is a special constraint built into the ti_plotlib module.
The geometric/exponential sequence b = 2**n gets too large to plot. Can you fix it? See the next step…
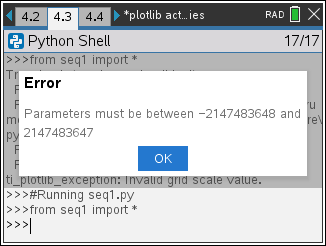
Step 8
We can fix this ‘overflow’ plotting issue by adding a condition:
if b < ymax:
plt.plot(n, b, ”o”)
since there’s no need to plot a point that’s not on the screen. You could also place the same restriction on the arithmetic sequence plot statement.
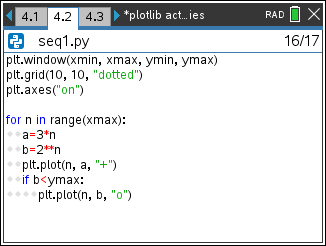
Step 9
Running the program now should produce a graph like the one shown here. Notice how the geometric sequence grows so quickly compared to the arithmetic sequence. Both sequences go off the screen, but the arithmetic sequence does not exceed the ti_plotlib upper limit.
In the next section you will use most of this program to plot a different sequence, so we will make a copy of it…
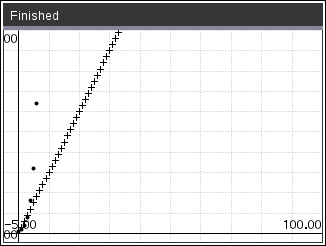
Step 10
Part 2: The Collatz Conjecture
The final sequence in this activity is based on the Collatz Conjecture which is also discussed in the TI Codes Python materials.
The Collatz Conjecture goes like this:
- Start with any counting number (we will use input( ) ).
- If it is even, then divide it by 2
- Otherwise, multiply it by 3 and add 1
Repeat steps 2 and 3 with the new number.
The Conjecture (guess) is that all numbers eventually will become 1 but this has not been proven… yet.
Starting with the number 37, we get the sequence shown to the right in the Python Shell. Let’s plot some Collatz sequences.
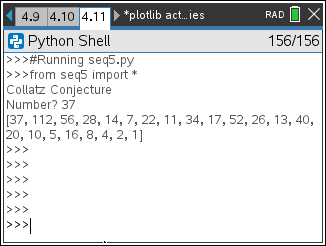
Step 11
This program will differ greatly from the previous one, but we start with the usual plt. setup statements so make a copy of your last program (Harmonic sequence). Ours is named seq5.
Press [enter] a few times after the import statement to make room for some new code: the plan is to make two lists and then use plt.scatter( ) or plt.plot( ) to plot the lists rather than one point at a time as was done in Part 1.
Write the first two statements shown:
print("Collatz Conjecture")
n = int(input("Number? ") )
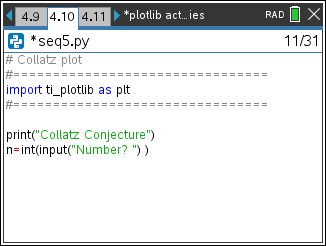
Step 12
Initialize a counter variable c to be 0.
c = 0 Create two lists:xs is the list of counters, starting with 0.
ys is the list of terms in the Collatz sequence for n, starting with n.
xs = [c]
ys = [n]
We’re going to make a big assumption here: eventually the sequence will reach 1. After all, it has never not happened, right?
while n > 1:
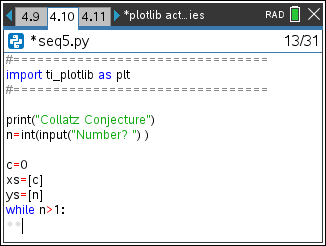
Step 13
Write the if… else structure that processes the Collatz algorithm:
while n>1:
if n % 2 == 0:# % is ‘mod’
n = n // 2# integer division
else:
n = 3 * n + 1
Note: use integer division (//) to ensure all values are integers and there are no rounding issues.
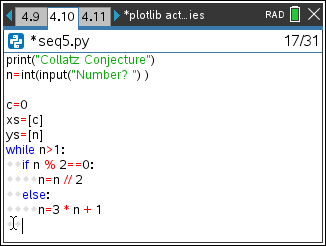
Step 14
c += 1
xs += [c]# same as xs.append(c)
ys += [n]
Note that these statements are part of the while loop but not part of the else: block and are indented accordingly.
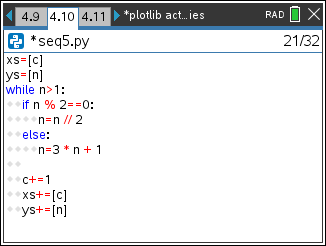
Step 15
When the while loop ends the sequence is complete so we’re ready to plot the data.
Use a special window that depends on the data itself. We write each window variable assignment on a separate line for readability:
xmin = -1
xmax = c # the final counter value
ymin = -10
ymax = 1.1 * max(ys) # so all fit on screen
# 1.1* is 10 percent more than the largest
# number in the list ys
plt.window(xmin,xmax,ymin,ymax)
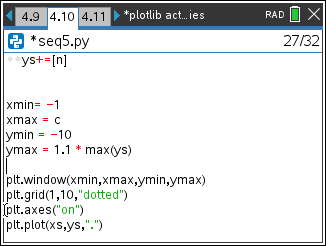
Step 16
plt.plot(xs, ys, “o” ) will plot the lists with
segments connecting the dots.
or use:
plt.scatter(xs, ys, “o”) to plot just the points
without the connections.
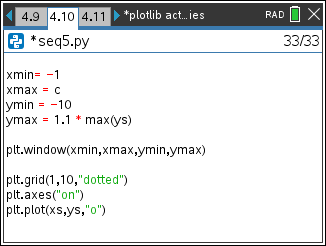
Step 17
Run the program. At the input prompt enter a positive integer. The plot displays the unique progression of the Collatz sequence of numbers for your entered number and the xmax value on the screen is the number of steps it took to reach 1. Your starting value is the point on the y-axis (it was 37 for this image; ymax is 120, not 20).
Note: For some numbers (like 125) you might have a grid scale issue, so just comment the .grid( ) statement or find a scale that works.
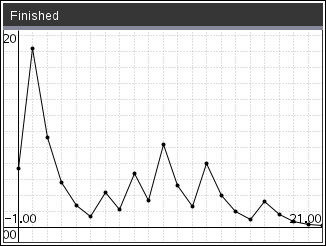
Step 18
To display the initial value on the graph use:
plt.text_at(row, "text", "align")
found on [menu] TI PlotLib > Draw
row is a number from 1 to 13 that you enter.
“text” is your text (or a string variable) to display
“align” can be “left”, “center”, or “right” selected from a pop-up menu.
To display the starting number for your sequence, add a statement right after the input statement that stores the value of n as a string:
txt = str(n)
str() is found on [menu] Built-Ins > Type and use that txt variable in the plt.text_at( ) statement.
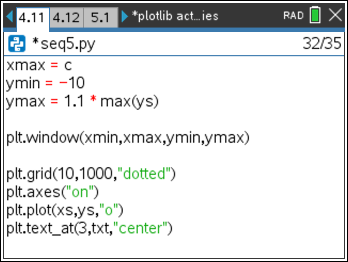
Step 18b
The ‘37’ at the top center of this screen is the result of the plt.text_at() function:
plt.text_at(3, txt, “center”)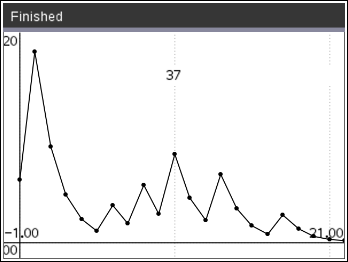
Step 19
For more precise position of text you can use the alternate form:
plt.text_at( row, col, “text”)(which is not found on the menus).
Use a column value from 1 to 30+.
For this screen, plt.text_at(3, 5, txt) was used.
For other interesting integer sequences see https://oeis.org/, the
Online Encyclopedia of Integer Sequences.
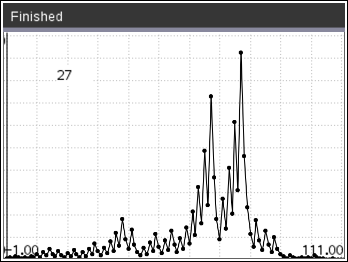
Mini project 3: Graphing functions
Download documentsThe TI PlotLib module is well-suited for graphing functions in two different ways: plotting points one-by-one or using lists as we did in the previous activity, Sequences.
Part 1: Graphing by plotting points.
Begin a Python program using the ‘Plotting (x,y) & Text’ template from the ‘Type:’ dropdown list. Our program is called func1.
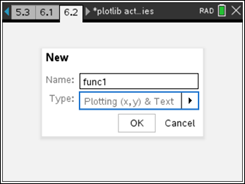
Step 1
This template provides the import statement:
import ti_plotlib as plt
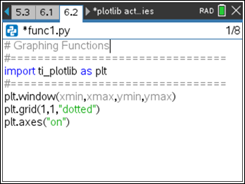
Step 2
Use four ‘window variables’ to set the plt.window( ) because you may need these values elsewhere in your code.
xmin, xmax, ymin, ymax = -10, 10, -7, 7
Having the four window variables assigned on a single line makes them easier to locate and edit and saves vertical space in your program.
The plt.grid((1, 1, “dotted”) values can be edited as needed but here are limitations on the window and these grid values may interfere with the run of your program if the grid lines are too close together.
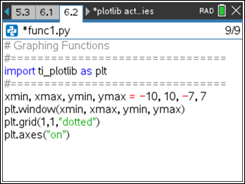
Step 3
It will be useful to know the Width and Height of the screen in pixels. There is a function, get_screen_dim( ), in the TI Draw module that gives these two values using one function:
W, H = get_screen_dim( )
(yes, we use capital letters here)
Find this function on [menu] More Modules > TI Draw > Control
Remember to add another import statement to be able to use this function:from ti_draw import get_screen_dim
imports just that function from the ti_draw module (no parentheses).
Note that the function returns two values at once!
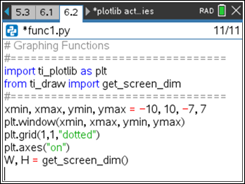
Step 4
We can plot a point of the function for every pixel on the screen, but we need to know the ‘distance’ between pixels based on our current window settings:
dx = (xmax – xmin) / W dx is the ‘change in x’ as we move from one pixel to the next in our window.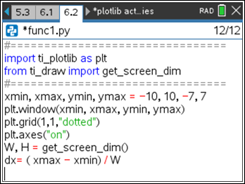
Step 5
We’re ready to plot the points using a loop. A for loop is tempting, but the Python for loop can only process integer values, not decimals.
Use a while loop instead. Start at xmin and have the loop end when x reaches xmax by adding dx in each step of the loop:
x = xmin
while x <= xmax:
# some code here
x += dx
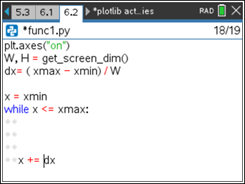
Step 6
In the loop body, evaluate your function and plot the point.
We’ve chosen to plot the function
y = x**2 - 5
(You can also define a function and use it here!)
plt.plot(x, y, “o”)
and select the “o” mark from the pop-up menu. You must change the gray x and y to the variables x and y.
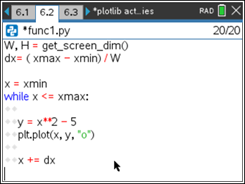
Step 7
Run the program to see the parabola shown here. Note that only dots are displayed, not a smooth, connected curve.
Change the color of the function using plt.color(r, g, b) before the while loop begins.
You can change the grid settings or turn off the grid by #commenting the plt.grid( ) function.
You can hide the window settings by changing
plt.axes(“on”) toplt.axes(“axes”)(window values “on”)(“axes” only)
If you try other functions you may need to edit the window settings.
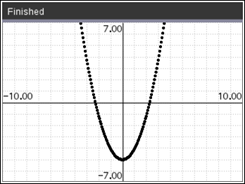
Step 8
Part 2: Smooth, connected curves using lists.
Another way to graph a function uses two lists: one for the x’s and one for the y’s. Go back to the Editor…
Before the while loop, initialize two variables with empty lists:
xs = [ ]
ys = [ ]
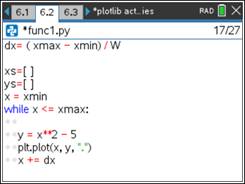
Step 9
In the loop body, after calculating y, .append the values of x and y to their respective lists:
xs.append(x)
ys.append(y)
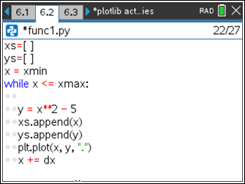
Step 10
plt.plot(xs, ys, “.”)
You must move the plt.plot( ) function to the bottom of the program outside the while loop (dedented) because the plot will cause an error if there is only one element in the list.
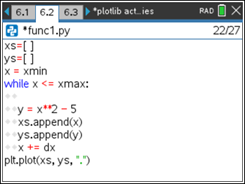
- MP 1
- MP 2
- MP 3
Micro:bit
Mini project 1: Getting Started with Micro:Bit
Download documentsIn this lesson, you will write your first Python programs to control the micro:bit display in different ways. This lesson has two parts:
- Part 1 — Alien encounter
- Part 2 — Displaying images
*These projects use micro:bit v1 and work on v2 as well. For best experience, be sure your equipment is up-to-date. The instructions here are written for TI-Nspire OS v.6.0, microbit.tns module (v3.3.8 for micro:bit v2; v2.34 for micro:bit v1), and the micro:bit .hex file (there are separate files for v1 and v2:3.2.0).
Step 1
Before you begin, be sure that:
- Your TI-Nspire™ CX II graphing calculator has OS 6.0 or higher
- You are comfortable programming in Python and/or you have completed Units 1 through 5.
- Your micro:bit is connected to your TI-Nspire™ CX II graphing calculator
- You have followed the setup directions and file transfers in the micro:bit Getting Started Guide
This setup process should only have to be done once, but keep informed periodically about updates/upgrades.
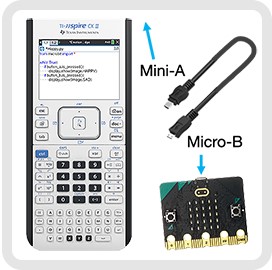
Step 2
If all is good, and the setup has been done correctly, your micro:bit should look like this when it has power from the TI-Nspire™ CX II graphing calculator:
The display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
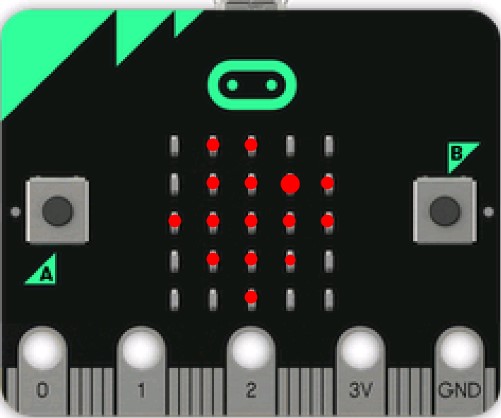
Step 3
And finally …
The micro:bit module is installed to your Python Library. In a Python Editor, press [menu] > More Modules and see that BBC micro:bit is listed beneath the TI modules.
Note: In OS 5.3 and above, Python modules that are stored in your Pylib folder on your device are shown on this menu in addition to the ti_ modules. Your list may differ from the one shown here. The modules are listed alphabetically by filename, so BBC micro:bit is listed among the M’s in the list, not the B’s.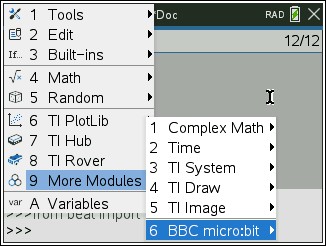
Step 4
Part 1: alien encounter:
Of course, as with every other first programming experience, you will start with displaying a message on the micro:bit display.
Begin a new blank program named 'greetings'. In the Python Editor use [menu] > More Modules > BBC micro:bit to select the import statement at the top of the menu items:
from microbit import *
Tip: If the message “micro:bit not connected” ever appears, just unplug the micro:bit and plug it in again (reset).
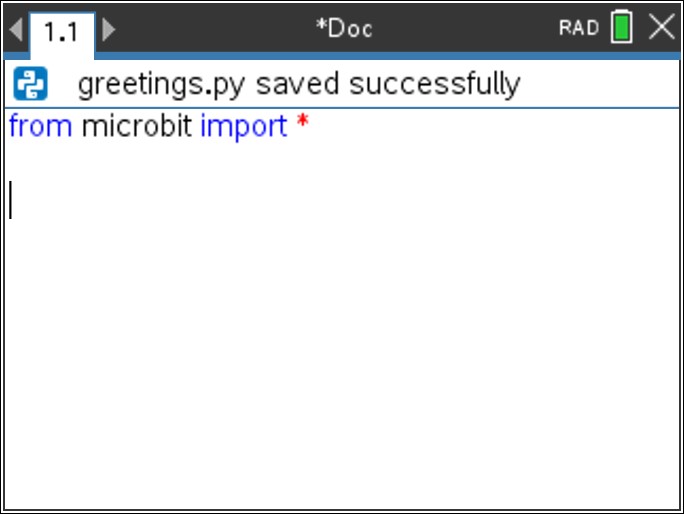
Step 5
To display a text message on the micro:bit display, use the statement:
display.show(text)
This statement is found on:
[menu] > More Modules > BBC micro:bit >
Display
The statement is inserted as display.show("text"), but (text) is just a placeholder that must be replaced with something. Inside the quotes, replace text by typing your message:
“greetings, earthlings”
When you run this program by pressing [ctrl] [R], you will see the letters of your message appear, one letter at a time, on the display. The lowercase letters e do appear twice, but you cannot distinguish between the two of them.
If you make a mistake, go back to page 1.1 to edit your program, and then run the program again. Did you forget to add quotes around the text you wanted to display?
Step 6
display.scroll(“greetings, earthlings”)
which is also found on
[menu] > More Modules > BBC micro:bit > Display
To complete the .scroll( ) statement you can copy/paste the string from the .show statement.
Make the previous .show() statement a #comment (place the cursor on that line and press [ctrl] [T]) to disable it, and then run the program again.
Yes, you can also simply change .show to.scroll by typing.
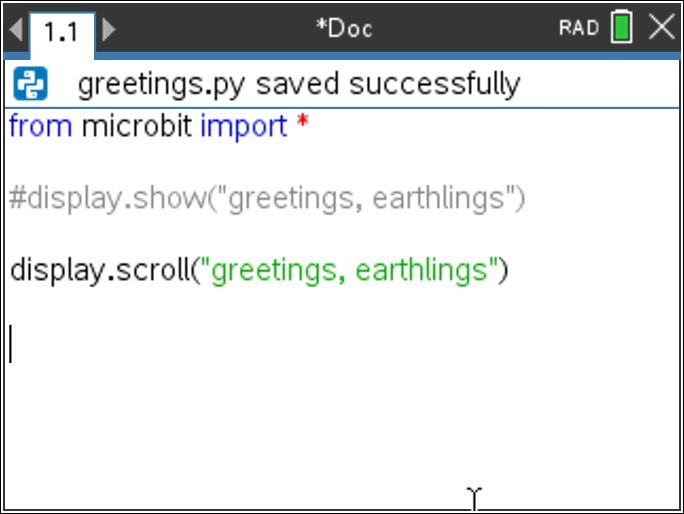
Step 7
The .scroll() method causes the message to scroll (move) from right to left like a banner across the display making it easier to read.
You can control the speed of the scrolling by adding the delay= parameter:
display.scroll(“greetings, earthlings”, delay = 200)
which uses a 200 millisecond (0.2 second) delay in the scrolling. Try other delay values, too.
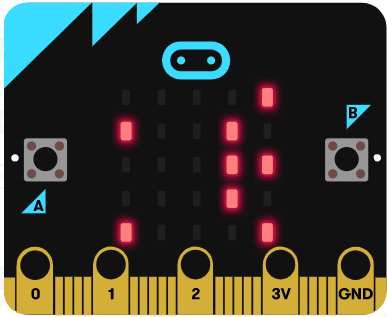
Step 8
Part 2: Be.Still.My.Beating.Heart
Press [ctrl] [doc] to insert a page and select Add Python > New to add a new Python program to your document (ours is named “beat”).
In the Python Editor, use [menu] > More Modules > BBC micro:bit and select the import statement at the top of the list:
from microbit import *
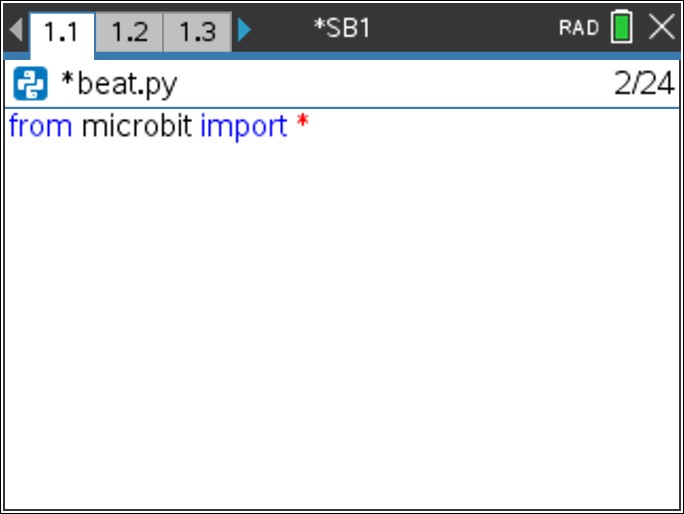
Step 9
display.show( image )
This statement is found on: [menu] > More Modules > BBC micro:bit > Display
Inside the parentheses, replace the prompt by selecting:
HEART
from the pop-up menu
Note: the list of available images is not on the menus and only appears when selecting display.show(image) from the menu.
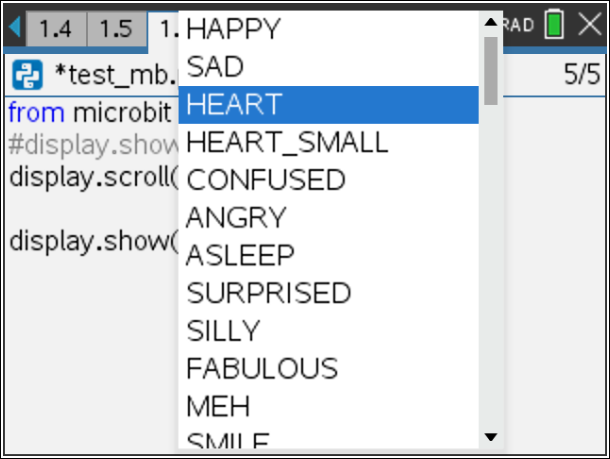
Step 10
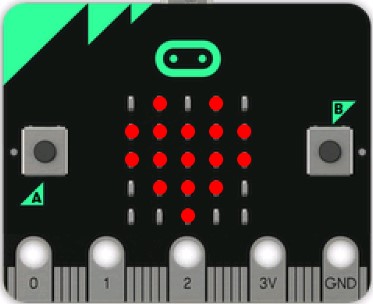
Step 11
Go back to the Program Editor on the previous page, and add another display statement to show the small heart:
display.show(Image.HEART_SMALL)
You can find this statement on the menu:
[menu] > More Modules > BBC micro:bit > Display
Tip: You can also copy/paste the first display statement and edit (type the _SMALL). It must have the underscore _ and be uppercase.
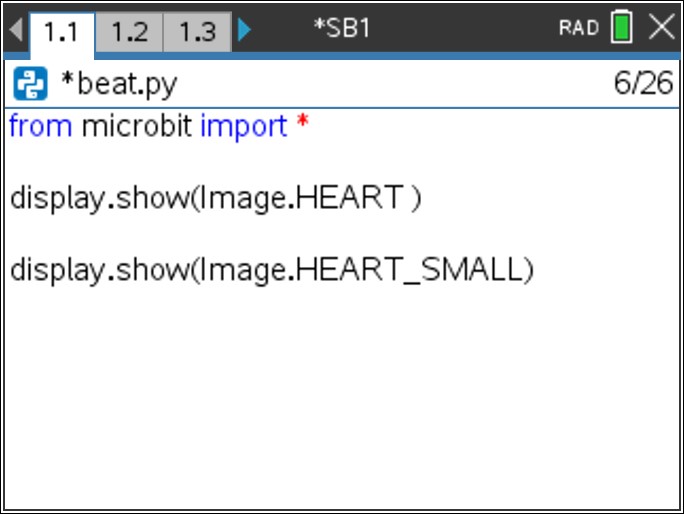
Step 12
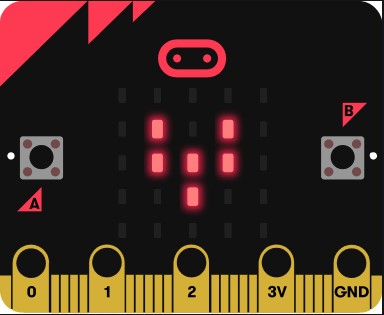
Step 13
while get_key() != ”esc”:
found on [menu] > More Modules > BBC micro:bit > Commands
and indent the two display statements so that they form the loop body.
Important tip: Indentation is critical in Python programs. This is how Python interprets loop blocks and if blocks. If the two display statements are not indented the same number of spaces then you will see a syntax error. Use the [space] key or the [tab] key to indent the two lines the same amount. Indentation spaces are indicated in this Editor as light gray diamond symbols (◆◆) to help with proper indentation.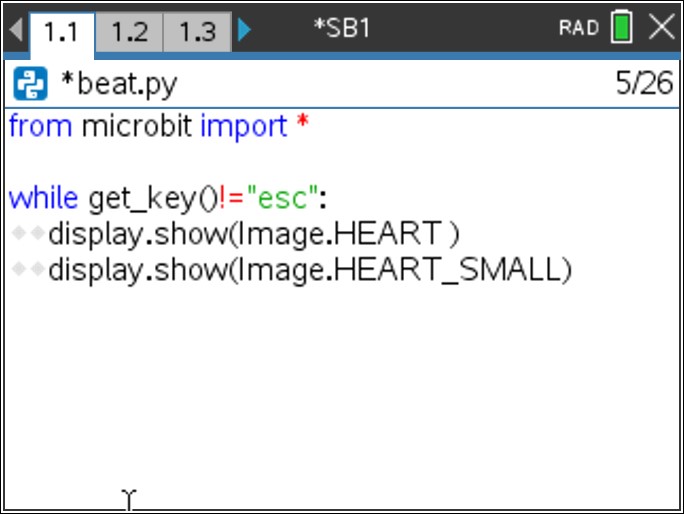
Step 14
Run your program again and watch the Beating Heart. Press the [esc] key to end the program.
Tip: If you ever think your program is stuck in an infinite loop, press and hold the [home/on] key on your TI-Nspire™ CX II graphing calculator to “break” the program. This could happen if you use while True: from the Commands menu improperly. These lessons avoid that type of structure.
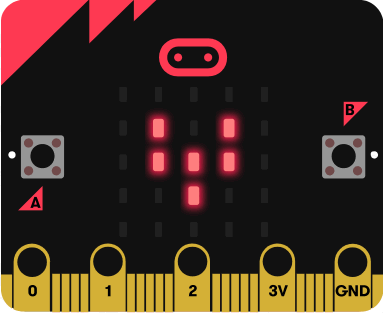
Step 15
The micro:bit Commands menu contains some useful python commands that are also found on other menus. The micro:bit module imports these python commands for you. Use the sleep( ) function to control your beating heart rate in the next step…
You can use these and any other Python commands from other menus. You are not limited to just using the BBC micro:bit menu, but you may need to provide the proper import commands.
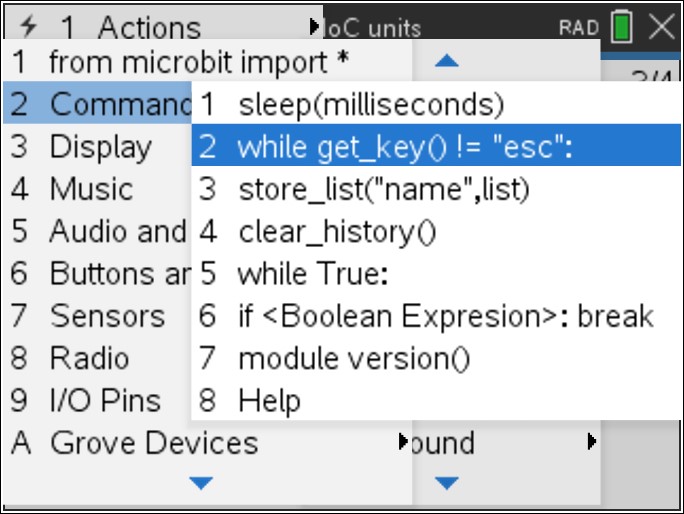
Step 16
sleep(1000) means 1000 milliseconds or a 1 second delay.
and is found on [menu] > More Modules > BBC micro:bit > CommandsTip: Watch the indentation!
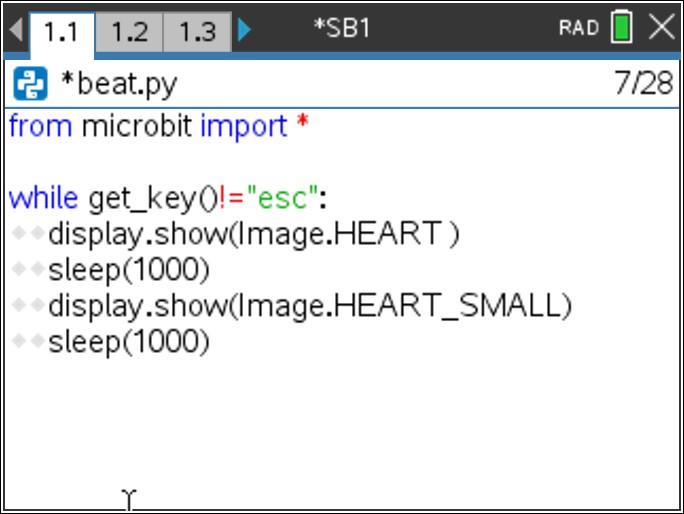
Mini project 2: Buttons and Gestures
Download documentsIn this lesson you will learn about using the micro:bit buttons and gestures and then write a program to toss a die and collect the values in a list to be transferred to a data plot.
There are two parts to this lesson:
- Part 1 — Investigating buttons and gestures
- Part 2 — Using a button or gesture to generate some data
Step 1
The micro:bit has two buttons, labeled A and B, on each side of the display. The Python micro:bit module has two similar methods for reading the buttons and then performing tasks based on those buttons. First you will test the methods and then write a program that lets you collect data and analyze it elsewhere in the TI-Nspire™ CX II graphing calculator.
There is also a 3-axis accelerometer/compass chip on the back of the micro:bit and methods for interpreting micro:bit movement and orientation.
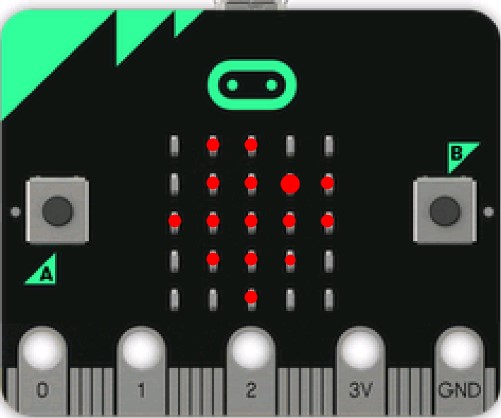
Step 2
Part 1: Investigating buttons and gestures
Start a new blank Python program in a document.
We named the program buttons_gestures.
From [menu] > More Modules > BBC micro:bit select the import statement at the top of the list:
from microbit import *
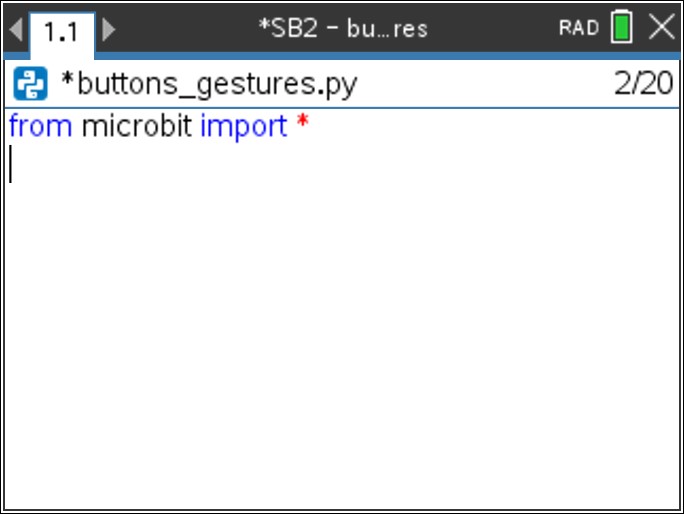
Step 3
while get_key()! = ’esc’:
from[menu] > More Modules > BBC micro:bit > Commands
Almost all your micro:bit programs will be designed this way
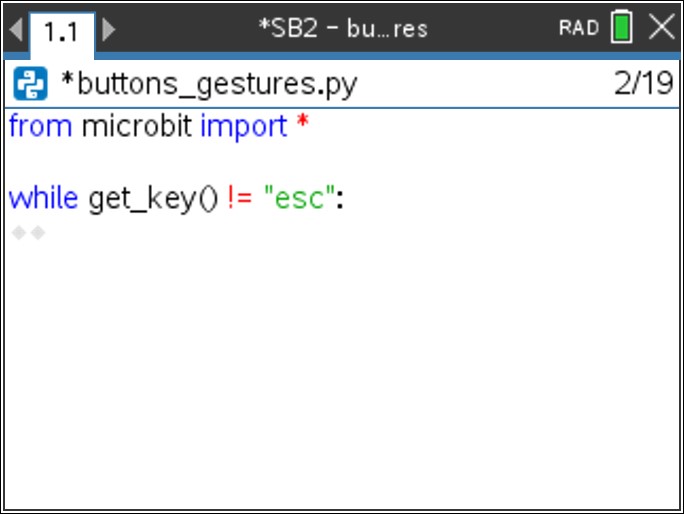
Step 4
To test button A, add the if: structure:
♦ ♦ if button_a.was_pressed():
♦ ♦ ♦ print("Button A")
if is indented to be part of the while loop and print( ) is indented even more to be part of the if block. Remember that proper indentation is very important in Python. The wrong indentation can cause syntax errors or improper execution of your code. Note the light gray diamond symbols (♦ ♦) that indicate the indentation spacing.
if is found on [menu] > Built-ins > Control.
The condition button_a.was_pressed() is found on
[menu] > More Modules> BBC micro:bit > Buttons and Logo Touch
print( ) is on [menu] > Built ins > I/O
Type the text “Button A” inside the print( ) function.
Note: is_pressed() will also be discussed later.
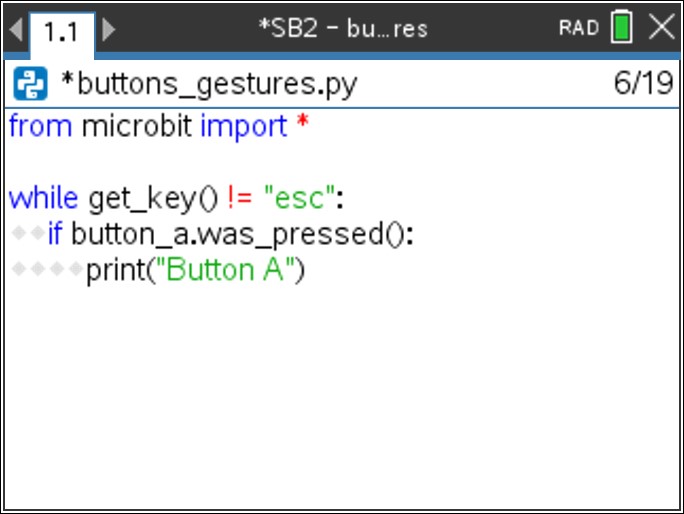
Step 5
Press [esc] and return to the Python Editor.
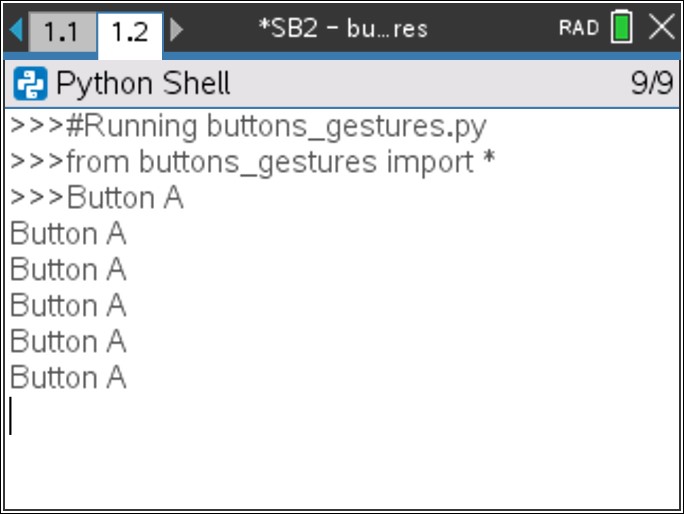
Step 6
button_b.is pressed(). Note that “IS” is different than “WAS.”
You will see how they differ soon.
◆◆if button_b.is_pressed():
◆◆◆◆print("Button B")
Tip: Again, pay attention to the indentations!
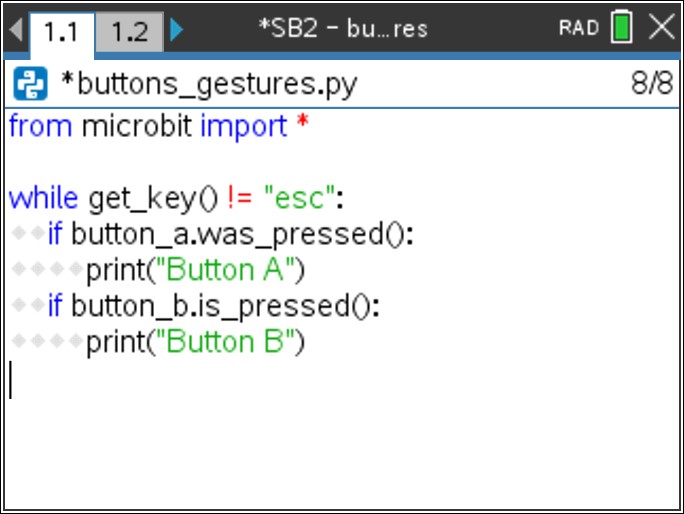
Step 7
Run the program again (press [ctrl] [R]). Try both buttons A and B.
Tap each button and press-n-hold each button.
You will see “Button B” repeatedly displayed as long as button B is held down, but not “Button A.” There is a difference between .was_pressed() (which needs a release of the button to be reset) and .is_pressed() which just checks to see if the button is down at the very moment that the statement is processed.
Note: If you tap button B quickly, the program may not display “Button B” since the button is not down at the very moment the if statement is being processed.
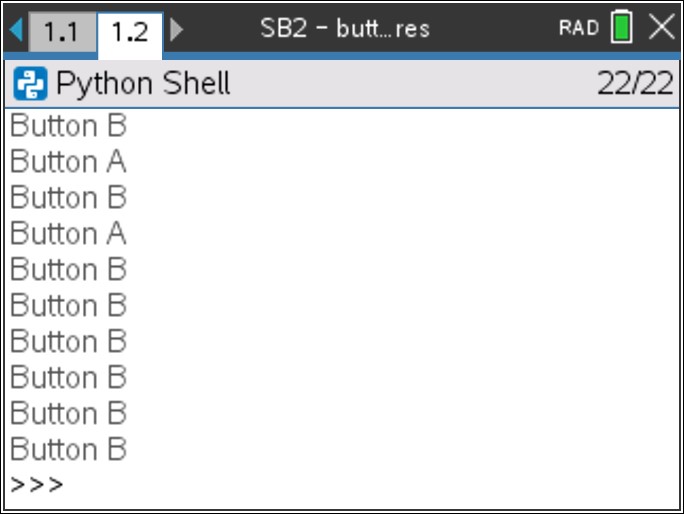
Step 8
This lesson explores these gestures and demonstrates how to use them in programming your TI-Nspire™ CX II graphing calculator with micro:bit.
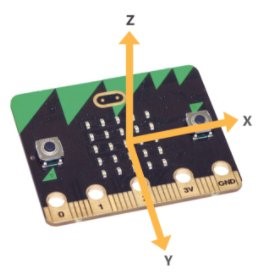
Step 9
Get the gesture value from micro:bit, and store it in the variable g:
♦ ♦ g = accelerometer.current_gesture()
Tip: Again, pay attention to the indentations!
Type ♦ ♦g = and then
Find accelerometer.current_gesture() on
[menu] > More Modules > BBC micro:bit > Sensors > Gestures >
Then print the value of g:♦ ♦ print(g)
Reminder: print( ) is on [menu] > Built ins > I/ONote that these two statements are indented one stop to be part of the while loop but not part of the if statement above it. Remember that the indentation of each line determines the meaning, so be careful!
Step 10
Some of the available gestures are: face up, face down, up, down, left, right, shake. These are returned as string values. Can you see all these gestures on your screen?
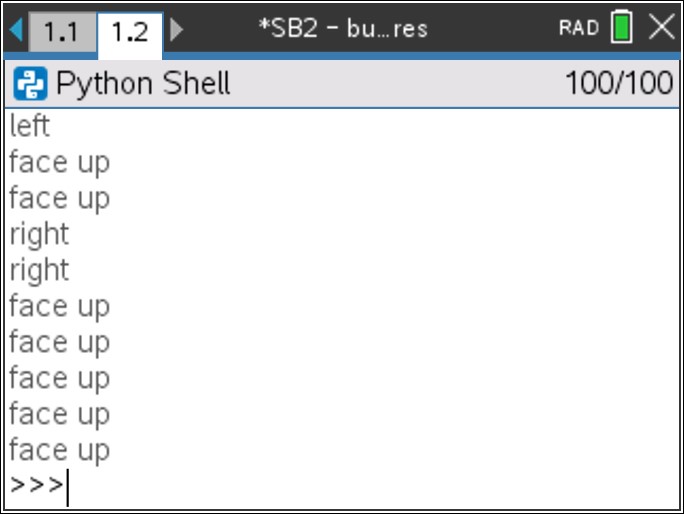
Step 11
◆◆if accelerometer.was_gesture(“face down”):
◆◆◆◆print(“face down”)
The gesture “strings” are all on a pop-up menu.
Yes, you can simply type the gesture string but in the .was_gesture() method it must match the menu item exactly.
When you run this program, you will notice the change in the output:
.current_gesture() constantly prints the gesture.
.was_gesture() only prints when the gesture changes.
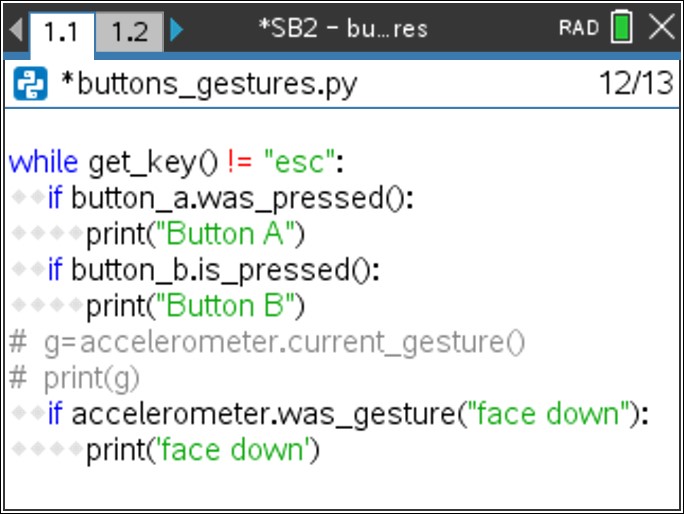
Step 12
The next part of this lesson builds an activity that produces some data using the micro:bit for further investigation on the TI-Nspire™ CX II graphing calculator.
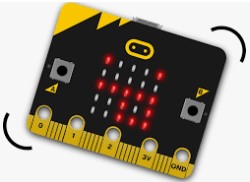
Step 13
Display the value on the micro:bit only. Try it yourself before looking at the next step. We will use the current program and add code to simulate the die toss.
Can you determine what number is on the bottom of the pictured die?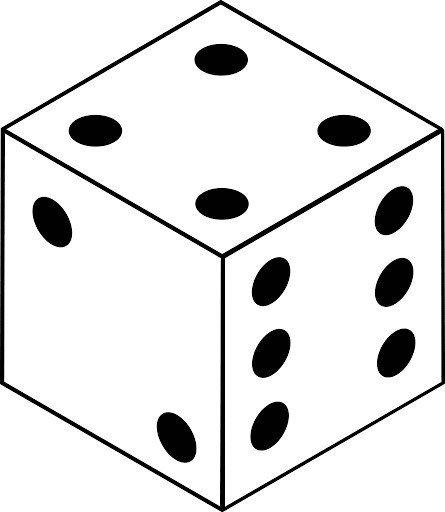
Step 14
from random import * and randint() are both found on
[menu] > Random
The rest of the statement ◆◆◆◆die = randint(1, 6) is typed in manually and is carefully indented because it is a part of the if button_a… block.
Again, be careful about the indentation.
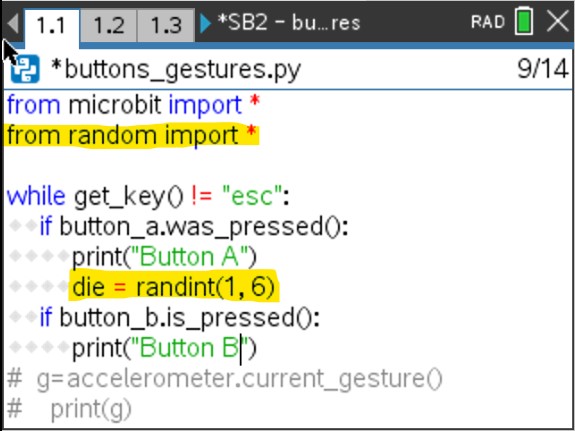
Step 15
display.show(die)
to show the value of the die on the micro:bit display.
Run the program again. When you press button A you see “Button A” on the handheld screen and the number on the micro:bit display changes … but not every time! Sometimes the random number selected is the same as the last number and that’s OK. The presses are “independent events.”
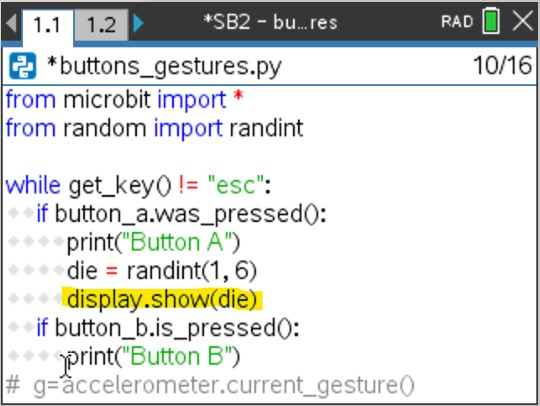
Step 16
Add statements to the program to:
- Create an empty list
- Add (.append) the die value to the list
- Store the list from Python to the TI-Nspire™ CX II graphing calculator for analysis
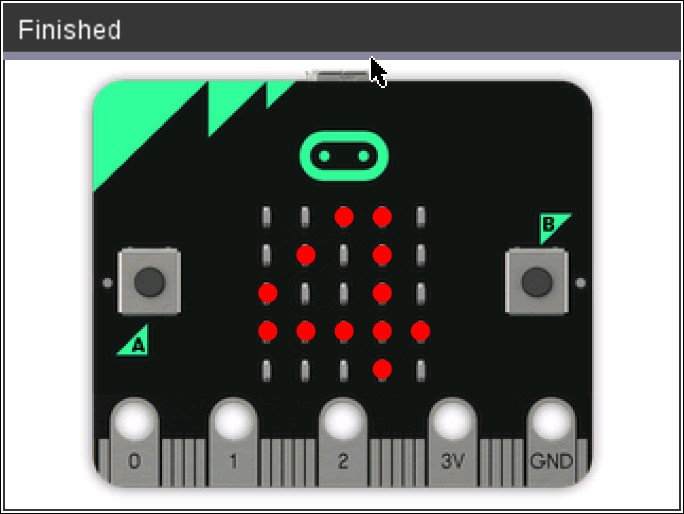
Step 17
The empty list assignment belongs at the start of the program:
tosses = [ ]
The brackets are on the keypad and on [menu] > Built-ins > Lists
After the die is determined, it is added to the list with:
tosses.append(die)
.append() is found on [menu] > Built-ins > Lists
At the end of the program the final list is stored to a TI-Nspire™ list:
store_list(“tosses”, tosses)
the store_list function is on [menu] >BBC micro:bit >Commands
Note: The two commented lines were removed so that all the code fits on the screen. You can leave those comments in your program.
Step 18
When you run the program now, press button A many times, then press [esc] to end the program. Your Python program has a list named “tosses” and now your TI-Nspire™ CX II graphing calculator also has a list named “tosses.” These are two separate lists.
Press [ctrl]-[doc] or [ctrl]-[I] to insert a page and add a Data & Statistics app. A bunch of dots will be scattered around the graph. Click the message “Click to add variable” at the bottom of the new app and select the list tosses. Do you see the screen to the right? Each dot represents one of your die tosses. What information can you gather from this graph? Can you change the graph to a Histogram? Hint: Check the app’s [menu].
You can modify this program in several ways. For example: Show (print) how many times you toss the die while you are doing it.
Note: Button B and your gesture have no effect on the die. Try modifying your code to toss a die only when you “shake” the micro:bit.
Remember to save your document!
Step 19
- Indent the store_list() method so that the list is stored to the TI-Nspire™ CX II graphing calculator at every button_a press
- print(“done.”) at the end of the program so that you know that the program has finished
- #comment the if buttonB… code and the if accelerometer… code
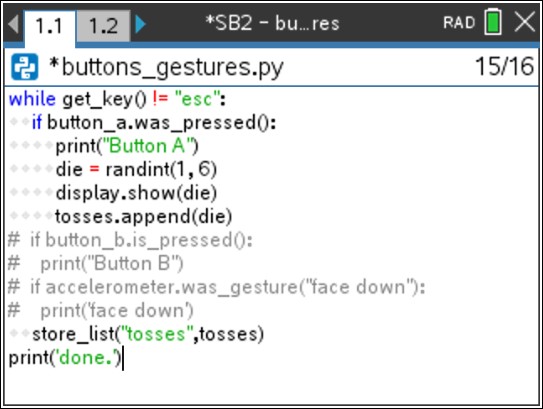
Step 20
Run the program by pressing [ctrl] [R] on the Shell. Press button A on the micro:bit to begin tossing the die and watch the dot plot grow!
The plot will display “No numeric data” at first because the tosses list has been emptied by the program but fear not, pressing button A will begin plotting the data.
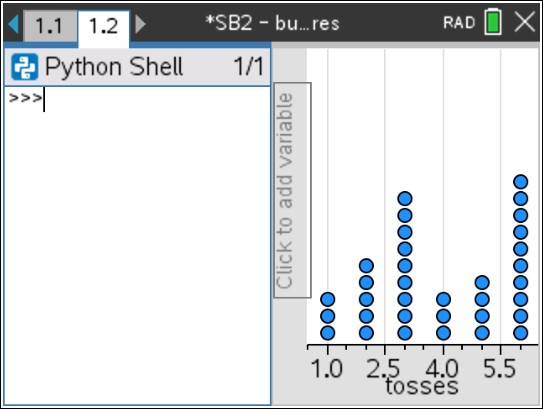
Mini project 3: The Light Sensor
Download documentsIn this lesson, you will monitor the light sensor on the micro:bit and store the data in a TI-Nspire™ list for further analysis.
Step 1
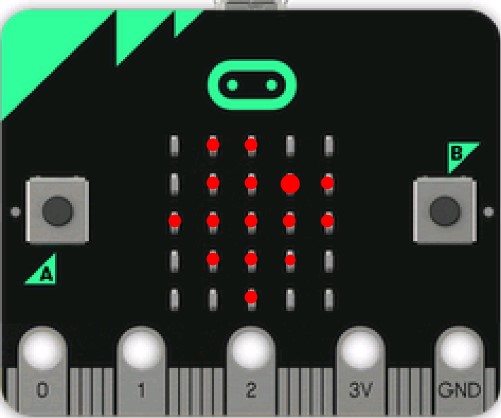
Step 2
Start a new blank python program.
We named the program bright.
To check the values that the light sensor can detect, write the following short program using the BBC micro:bit menus:
from microbit import *
while get_key() != "esc":
♦ ♦bright = display.read_light_level()
♦ ♦print("brightness = ",bright)
Find var = read_light_level() on [menu] > BBC micro:bit >Sensors
bright will be the variable name that you type.
Reminder: [menu] > More Modules > BBC micro:bit > Commands> while get_key() != "esc":
Remember to indent the last two statements so that they are the while loop body.
Step 3
Run the program and point the display of the micro:bit at a light source. It does not matter what is showing on the display. Move the micro:bit toward and away from the light and observe the changing values on the TI-Nspire™ CX II graphing calculator screen. You should see values varying between 0 and 255.
As you probably expect, the further from the light source, the lower the light level value. Now you will add code to the program to collect the light level data to create a scatter plot of light versus time.
Press [esc] to end the program and go back to the Editor.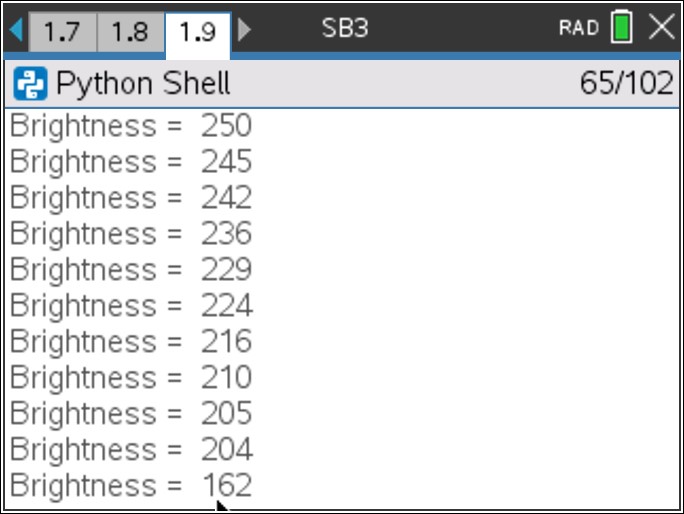
Step 4
times = [ ]
brights = [ ]
Find the square brackets on the keypad [ctrl] [left parenthesis] or on
[menu] > Built-ins > Lists
Also before the while loop, add a statement to start a time counter variable (t) at 0:
t = 0
Avoid using the word “time” as a variable because there is a time module. Also, it is a good practice to pluralize list names because they contain many values.
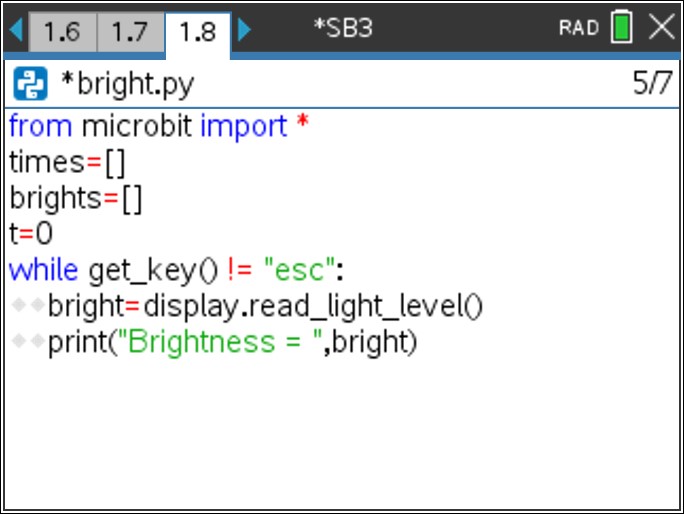
Step 5
◆◆t = t + 1
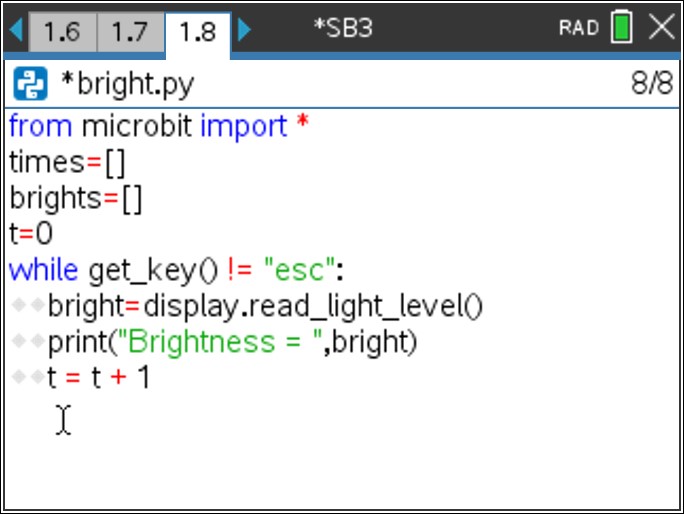
Step 6
◆◆times.append(t)
◆◆brights.append(bright)
.append( ) is found on [menu] > Built-ins > Lists
These statements add the current bright value and t (time) value to the lists.
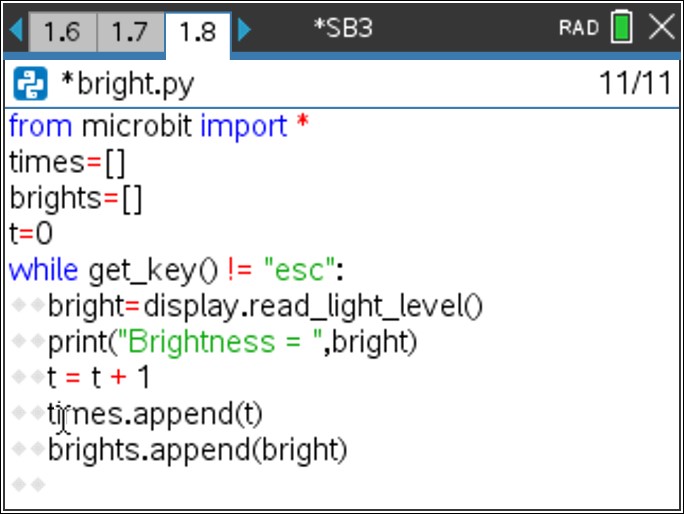
Step 7
◆◆sleep(1000)
after the two .append statements. This pauses data collection for one second between samples. sleep( ) is found on:
[menu] > BBC micro:bit > Commands
Recall that when using micro:bit, sleep( ) uses milliseconds for its argument. This sampling occurs once a second.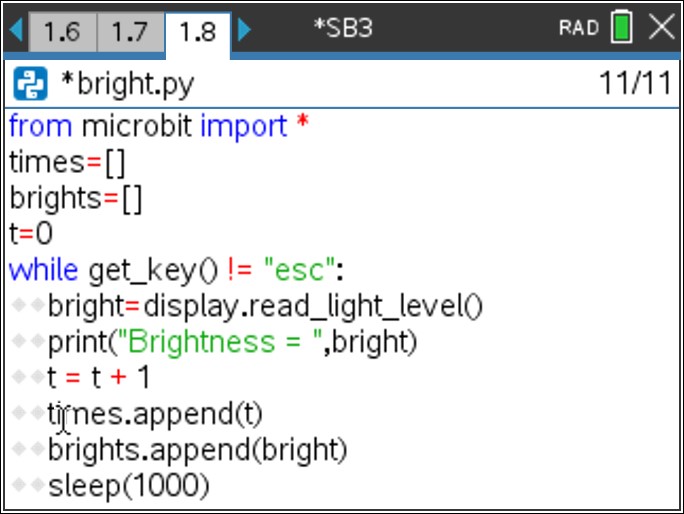
Step 8
Start at the beginning of a new line (no indent spaces!). Find store_list() on [menu] > BBC micro:bit > Commands. It takes two arguments: the “TI-Nspire™ list name” in quotes and the Python list name without quotes. Follow the tool-tip prompts.
In this program, the Python lists are only stored to TI-Nspire™ lists just before the program ends when you press [esc] to exit the loop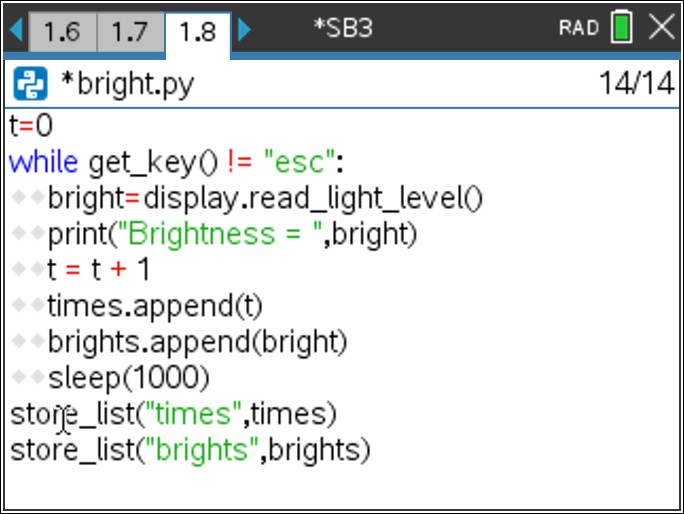
Step 9
Press [esc] to end the program.
Repeat the process until you feel you have “good” data.
Tip: A smartphone flashlight works great.
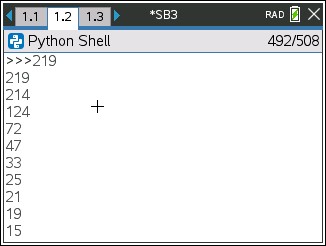
Step 10
When the program ends, add a page to your document by pressing [ctrl] [doc]. Select “Add Data & Statistics.”
You will see a page similar to this image. One of your lists appears with the points scattered around the screen. You now have to organize the plot.
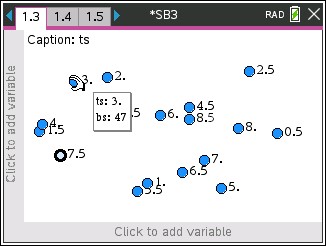
Step 11
Click the prompt “Click to add variable” on the left side of the screen, and select the list brights. This is the dependent variable. This organizes your scatter plot like the image shown here.
You may have to run the program several times to get a good, smooth plot like the one shown here. You may also have to adjust the viewing window when using different data.
You may also adjust the time interval between samples, but be sure to edit both the sleep(1000 ) argument and the counter value (t = t + 1).
What do you notice?
- What pattern do you see? What mathematical model fits this physical phenomenon?
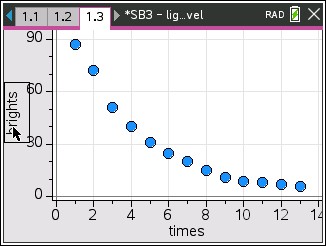
Mini project 4: Pair Of Dice
Download documentsIn this activity, you will write a program to collect data using the micro:bit, and run the program while observing a dot plot grow on a split page of the TI-Nspire™ CX II graphing calculator.
Objectives:
- Write a micro:bit data-collection program
- Create a dynamic Data & Statistics plot of the collected data
Step 1
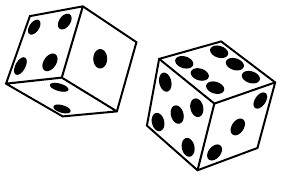
Step 2
- Runs the Python program on one side of the screen (the Python Shell) and
- Displays a dot plot (or histogram) of the collected data as you are running the program (using a Data & Statistics app)
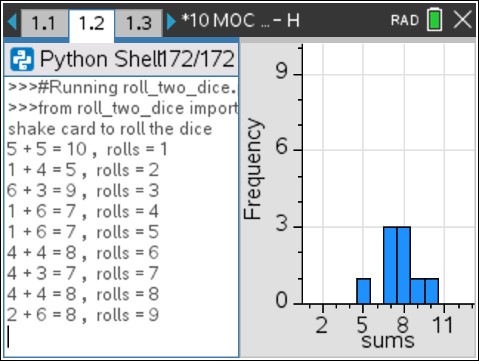
Step 3
sums = [ ]
Immediately store this list to a TI-Nspire™ variable (using the same name)store_list(“sums”, sums)
so that the TI-Nspire™ list is cleared as well.print( ) some instructions to the user before the loop begins. We are going to use the “shake” gesture to roll the dice.
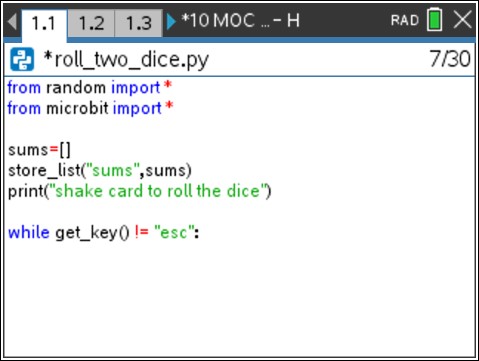
Step 4
- Toss two dice (generate two random integers)
- Add them together
- Append the sum to the sums list
- Print the two dice values, their sum and the roll number on the TI-Nspire™ CX II graphing calculator screen [Hint: len(sums) is the roll number]
- Display both die values on the micro:bit
- Store the list to a TI-Nspire™ variable
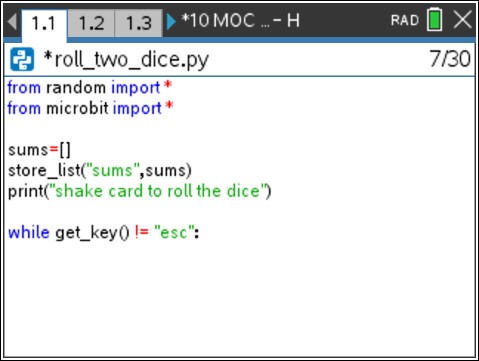
Step 5
◆◆if accelerometer.was_gesture("shake"):
◆◆◆◆display.clear()
◆◆◆◆r1 = randint(1,6)
◆◆◆◆r2 = randint(1,6)
Again, note the indentations.
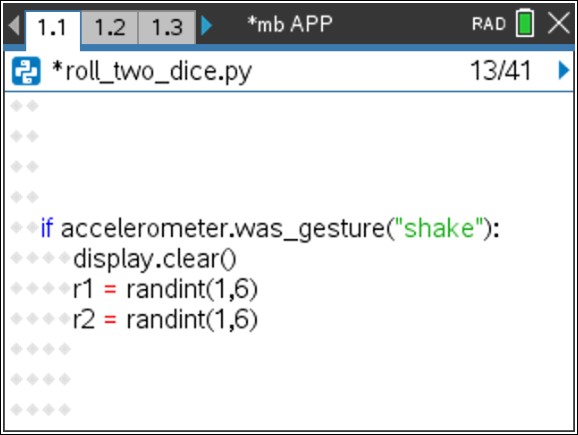
Step 6
sum = r1 + r2
sums.append(sum)
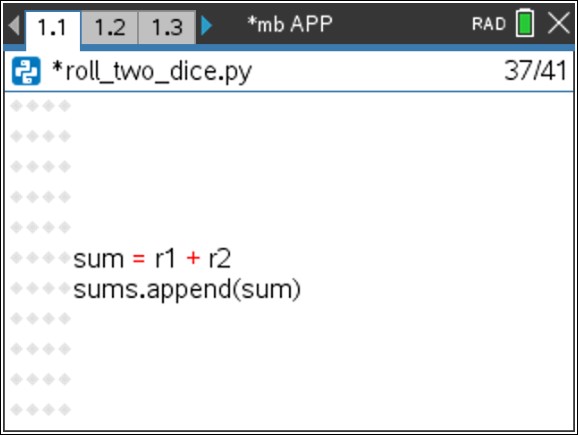
Step 7
Display the two dice on the micro:bit display. Remember that the two dice might have the same value, so we want to make sure that both will actually appear:
display.clear()
display.show(r1)
sleep(250)
display.clear()
display.show(r2)
sleep(250)
You might prefer a longer delay in the sleep( ) commands.
If you have entered the code properly and in the right sequence, try running the program now and shake the micro:bit. You should see two numbers displayed on the micro:bit.
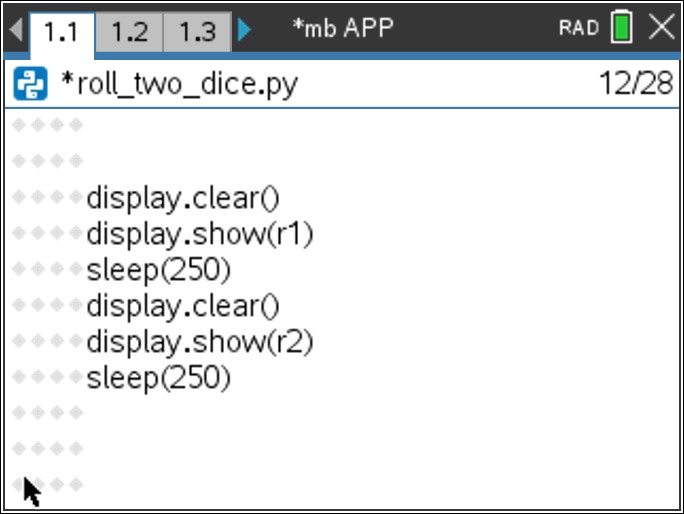
Step 8
◆◆◆◆print (r1, "+", r2,"=",sum,", ","rolls =", len(sums))
which results in the lines shown in this image.
Be careful about the punctuation!
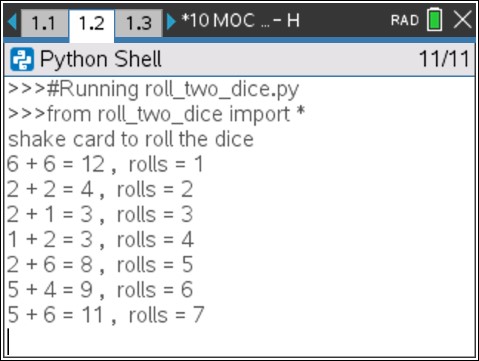
Step 9
◆◆◆◆store_list(“sums”, sums)
This store_list( ) statement is deep inside the while and if blocks so that the TI-Nspire™ list is updated every time a new pair of dice is generated.
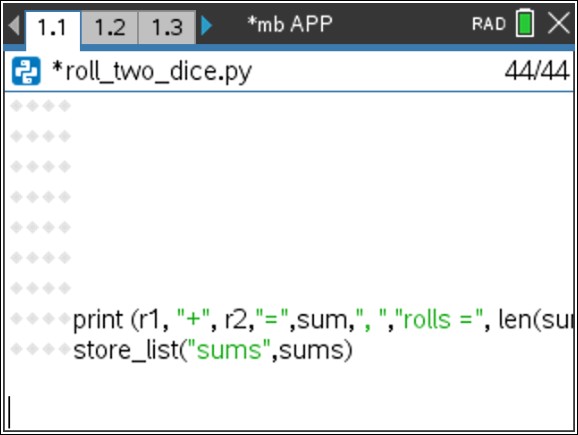
Step 10
In the Python Shell (at the command prompt >>>) press [ctrl] [doc] or [ctrl] [I] to insert a page. Select the Data & Statistics app. You should see a screen similar to the one on the right. Your sums data is scattered around the screen.
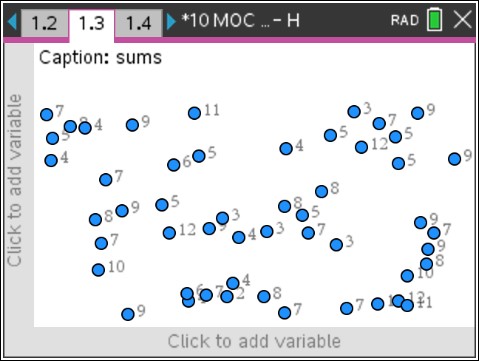
Step 11
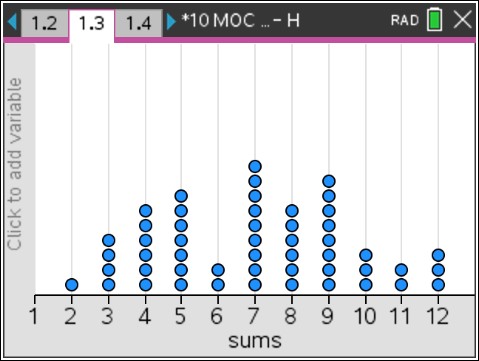
Step 12
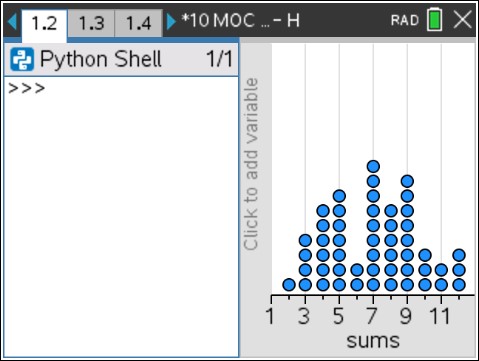
Step 13
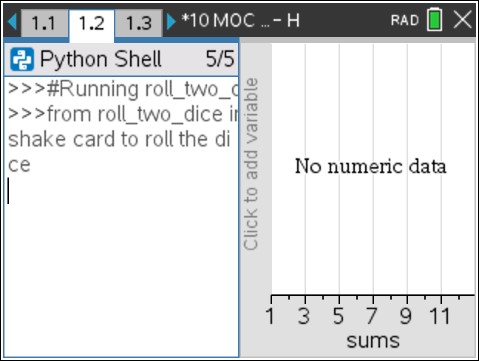
The Shell has been “re-initialized,” so pressing [ctrl] [R] will not re-run the program. Go back to the Python Editor and press [ctrl] [R] to run the program. It runs in the half-screen Shell as shown here. You see “No numeric data” on the right because the program stores an empty list right away.
As you collect the data (shake the micro:bit to roll the dice) your sums values appear as dots in the Data & Statistics app on the right.
Pressing [esc] will end the program and you can do a lot of other 1-variable data analysis in the TI-Nspire™ CX II graphing calculator environment.
Pressing [ctrl] [R] again now (in the Python Shell) does re-run the program.
Tip: To clear the Shell at the start of each run add the statement to the beginning of your program:
clear_history()
found on [menu] > More Modules > BBC micro:bit > Commands
Enjoy, and remember to save your document.
- MP 1
- MP 2
- MP 3
- MP 4
Micro:bit with Expansion Board
Mini project 1: The Light Switch
Download documentsThe micro:bit has a row of gold connections along the bottom edge for connecting electronic accessories. To make these connections easier there are many ‘expansion boards’ available that allow us to ‘plug in’ those accessories using standard cables. Two of these expansion boards are the Grove shield and the Bitmaker expansion board. The projects presented here will work with these and many other expansion boards. In addition to the expansion board, you will use some Grove accessories: an external LED (any one color), a light sensor, an ultrasonic ranger, and an external speaker.
These activities assume you have completed the first set of micro:bit activities.
Step 1
Before you begin, be sure that:
- your micro:bit is connected to your TI-Nspire™ CX II
- your micro:bit is inserted in the expansion board.
(Grove shield is shown here) - for this activity, you must have a Grove LED accessory and 4-wire connecting cable as shown here.
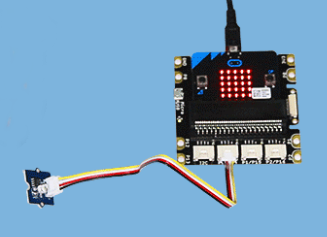
Step 2
Your micro:bit should look like this when it has power from the
TI-Nspire™ graphing calculator:
Recall that the display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
This activity has two parts: an on/off switch and a temporary-on switch.
There’s also a ‘Challenge’ at the end.
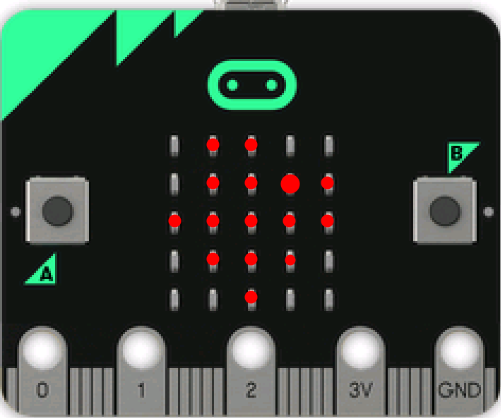
Step 3
The Grove LED is a small circuit board with a white (or colored) LED on it. Turn the yellow dial (a potentiometer) all the way to the right to get the maximum brightness. Connect this LED board to the port labeled P0 (pin0) on the expansion board using the cable included.
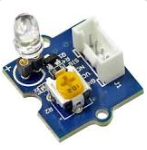
Step 4
Caution! The LED can be removed from its socket on the circuit board. Be careful when re-inserting the wires properly. There is a positive (anode) wire and a negative (cathode) wire and the circuit board is labeled with ‘+’ and ‘-‘ symbols. The negative wire on the LED is indicated by a ‘flat spot’ on the base of the LED as shown here.
Inserting the LED incorrectly will cause it to not work but will not damage it.
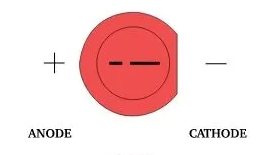
Step 5
Push-button light switches like the one seen here were common in the first half of the 20th century (1900-1950’s) when they were replaced with toggle switches. One button turned the circuit on while the other turned it off (and popped the other button out). These two projects will make similar switches using the micro:bit buttons.
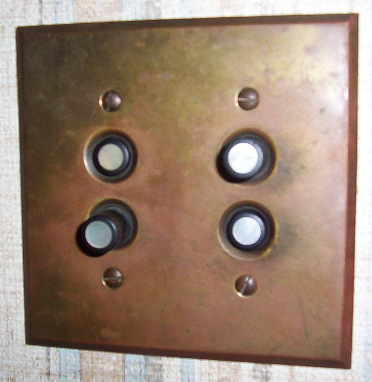
Step 6
Part 1: The 2-button on-off switch
Start a new TI-Nspire™ document and select Add Python > New to begin a new program (blank program), named ‘switch1’. In the Python Editor use [menu] > More Modules > BBC micro:bit to select the import statement at the top of the menu items:
from microbit import *
Tip: This statement tests to see if the micro:bit is present. If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit from the calculator and plug it in again (reset).
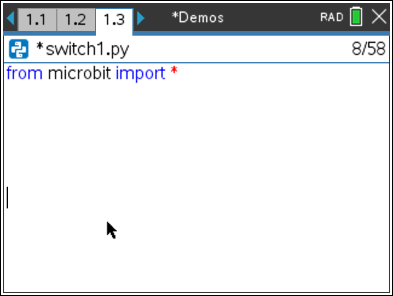
Step 7
Make a while not escape loop using the command found on
[menu] > More Modules > BBC micro:bit > Commands>
while get_key() != “esc”:
♦ ♦
We also added a comment and a print( ) statement to explain the program.
Note that get_key( ) is included in the micro:bit module. There’s no need to import the ti_system module.
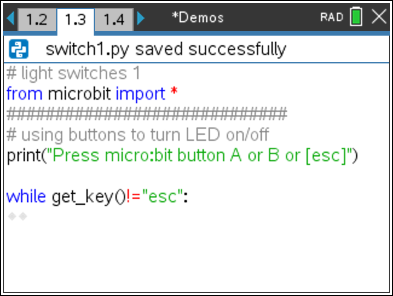
Step 8
In the loop body, add two if statements, one for each micro:bit button using the two _was_pressed() functions:
♦ ♦ if button_a.was_pressed():♦ ♦ ♦ ♦
♦ ♦ if button_b.was_pressed():
♦ ♦ ♦ ♦
These functions are found on
[menu] > More Modules > BBC micro:bit > Buttons under button_a and button_b.
One button will turn the LED on and the other will turn it off.
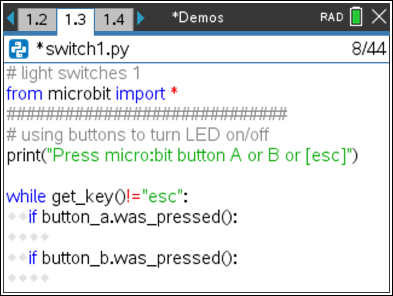
Step 9
The LED lights up when it gets power from the micro:bit. It responds to power levels in the (decimal) range from 0 to 100. So…
To turn the LED on brightly, use the function:
grove.set_power(pin0, 100)
found under the BBC micro:bit > Grove Devices > Output menu.
pin0 is a special micro:bit variable found on
> BBC micro:bit > I/O Pins
With your cursor on the first argument of the function, select pin0 from the list (or you can simply type it in manually).
The second argument of the grove.set_power( , ) function is the power level to send.
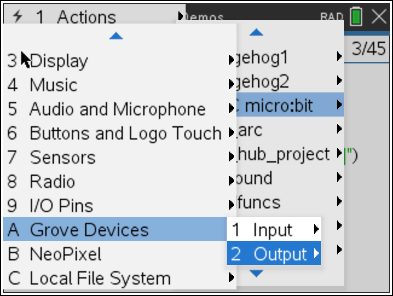
Step 10
In a similar manner, turn the LED off when button B was pressed. Set the digital value to 0 as shown here.
Test your program now to be sure the LED is working properly. Try other power levels for each button.
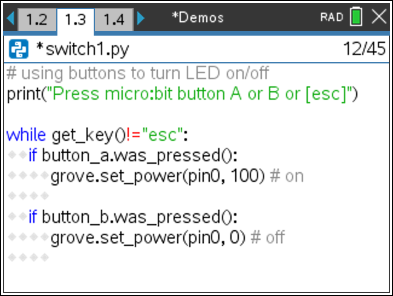
Step 11
Make one enhancement: display the letter of each button on the micro:bit display: Add display.show(“A”) when button A is pressed and a similar statement for button B.
After the while loop ends (by pressing [esc]), turn the LED off and clear the display (or display the special TI logo image using display.show(ti) to return the micro:bit display to its original state).
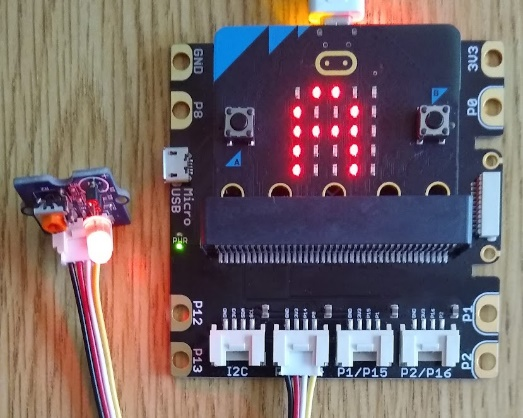
Step 12
Part 2: The ON button
This program will light the LED when button A is held down and it will be off when the button is released. Make a copy of your program from Part 1 of this activity. Use [menu] > Actions > Create Copy…
Give the new program a name (ours is switch2.py).
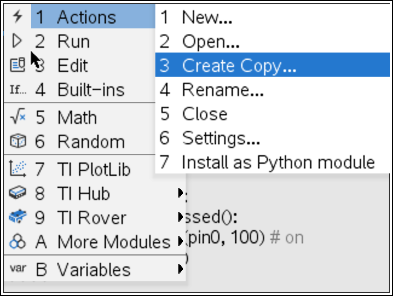
Step 13
In the new program, modify the button A if statement: change was to is.
if button_a.is_pressed():
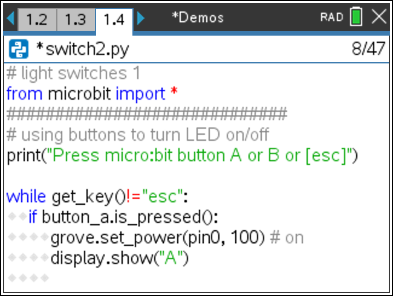
Step 14
We will not need the button B condition, but we will need to turn the LED off when button A is not pressed, so replace the if button_b… statement with an else: clause for if button_a… and turn off the LED in the else: block.
if button_a.is_pressed():
grove.set_power(pin0, 100) # on
display.show("A")
else:
grove.set_power(pin0, 0) # off
# if button_b.was_pressed():
Note that if button_b… is now commented and else: lines up with if!
If you displayed the letter “B” in the first project, change it to a space “ “ here so that nothing is displayed when no button is pressed.
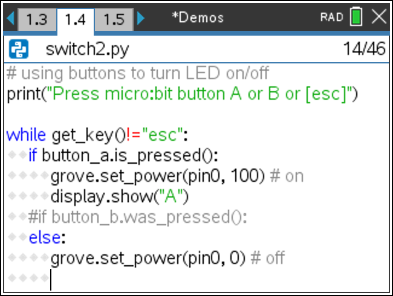
Step 15
Challenge: Make a single-button on-off switch. Can you modify the program again to turn the LED on when button A is pressed once and turn it off when button A is pressed again? That is, button A toggles the LED on and off by itself and does not need to be held down. Button B is not used at all. If you have micro:bit version 2, try using the pin_logo (the gold oval above the 5x5 display in the image).
The display can show a ‘1’ when the LED is on and ‘0’ when it is off.
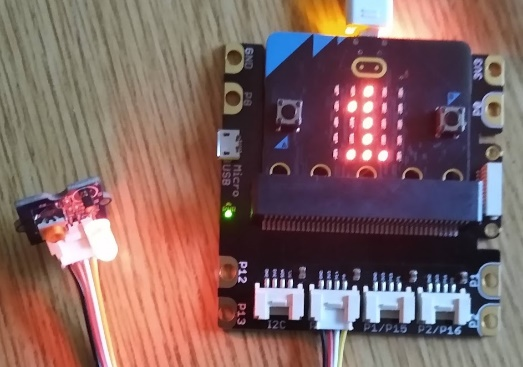
Mini project 2: Weather Face
Download documentsThe micro:bit has an internal temperature sensor in its CPU. In this activity you will use an external temperature sensor to monitor the temperature away from the micro:bit itself to get a more accurate reading. The activity is divided into two parts:
Part 1: The Weather Face
Part 2: Tracking Temperature
These activities assume you have completed the first set of micro:bit activities and will be using an external Grove temperature sensor.
Step 1
Before you begin, be sure that:
- your micro:bit is connected to your TI-Nspire™ CX II
- your micro:bit is inserted in the expansion board.
(Grove shield shown here) - the Grove temperature sensor is attached to pin0
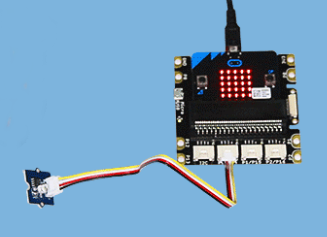
Step 2
Your micro:bit should look like this when it has power from the
TI-Nspire™ graphing calculator:
Recall that the display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
This activity has two parts: a temperature monitoring application and a temperature tracking program.
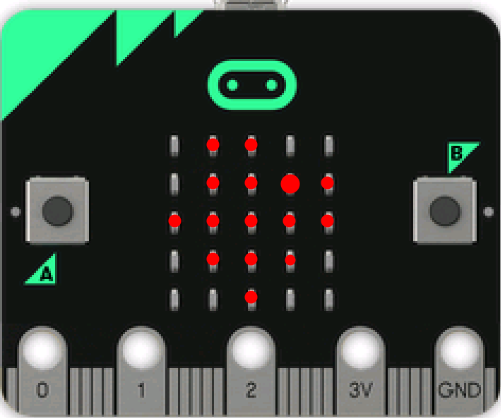
Step 3
This is the Grove temperature sensor: Connect the sensor board to the port labeled P0/P14 (pin0) on the expansion board using the included cable. You can use a different port if you also change the pin variable in the code to match.
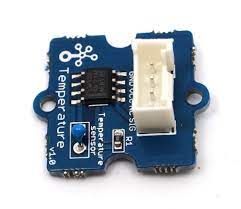
Step 4
Part 1: Weather Face
Some indoor/outdoor thermometers have an image of a person indicating the current weather conditions. This program will display an appropriate ‘face’ on the micro:bit display that will depend on the current temperature.
In the Python Editor use [menu] > More Modules > BBC micro:bit to select the import statement at the top of the menu items:
from microbit import *
Tip: If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit and plug it in again (reset).
Step 5
Write our favorite loop:
while get_key() != “esc”:
It’s found in
[menu] > More Modules > BBC micro:bit > Commands >
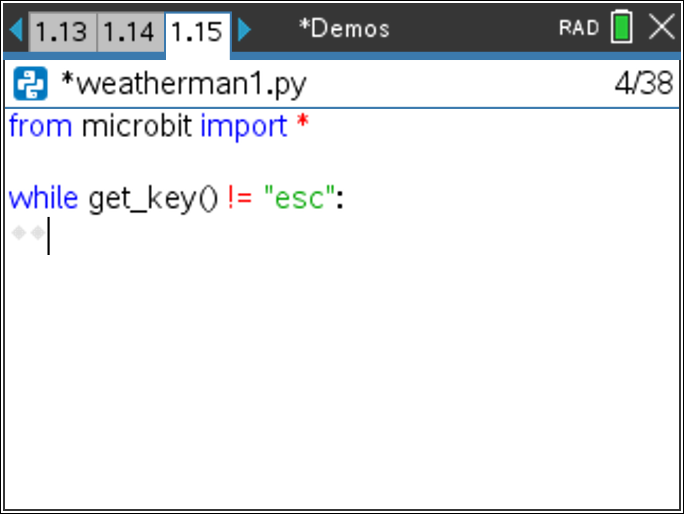
Step 6
In the loop body, read the Grove temperature sensor using the function found on
[menu] > More Modules > BBC micro:bit > Grove devices > Input
var = grove.read_temperature(pin)
Use any variable on the left of the = sign for your temperature variable.
For the (pin) argument, select pin0 from the pop-up list (or whatever socket your sensor is plugged into on the expansion board).
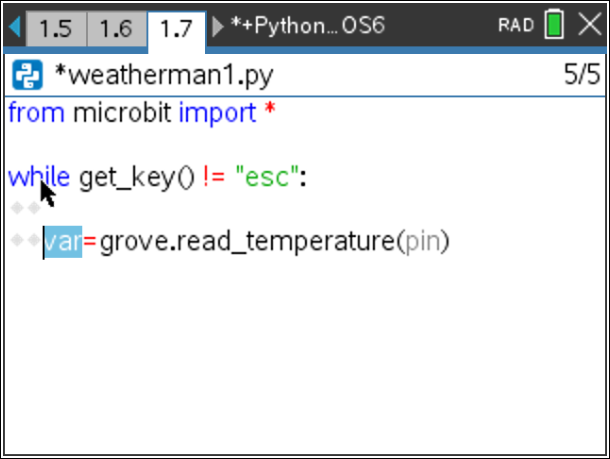
Step 7
In the image to the right, we used the variable temp to hold the temperature and pin0 for the argument.
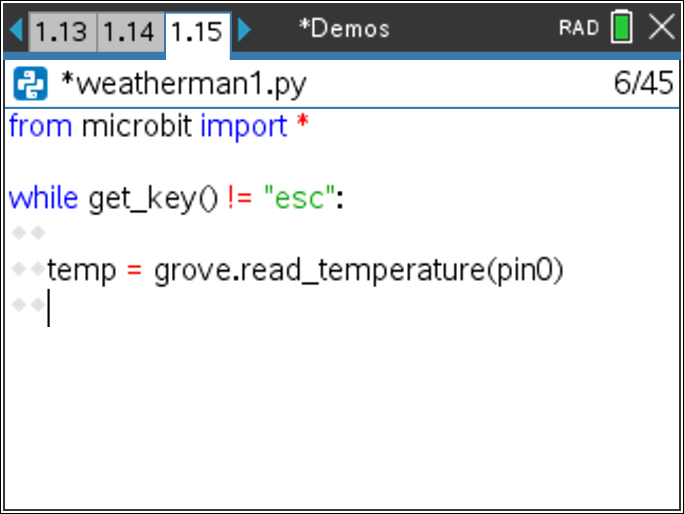
Step 8
Add a print statement to display the temperature to be sure that everything is working:
print(“temperature = “,temp)
Run the program now to see temperature values displayed on the
TI-Nspire™.
If you think the numbers displayed are strange, keep in mind that the temperature is displayed in the Celsius scale. If you prefer to work with the Fahrenheit scale, then you will have to do the conversion yourself in the program.
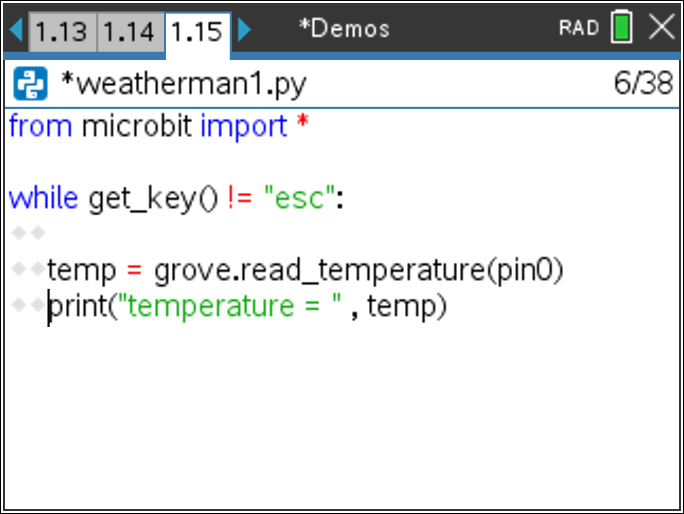
Step 9
Our program will display three different faces depending on the temperature (cold, mild, or hot). This is an ideal place for an
if…elif…else structure. Get this structure from
[menu] > Built-ins > Control
All three components are inserted into your code, and you must replace the placeholders with conditions (BooleanExp) and actions (block).
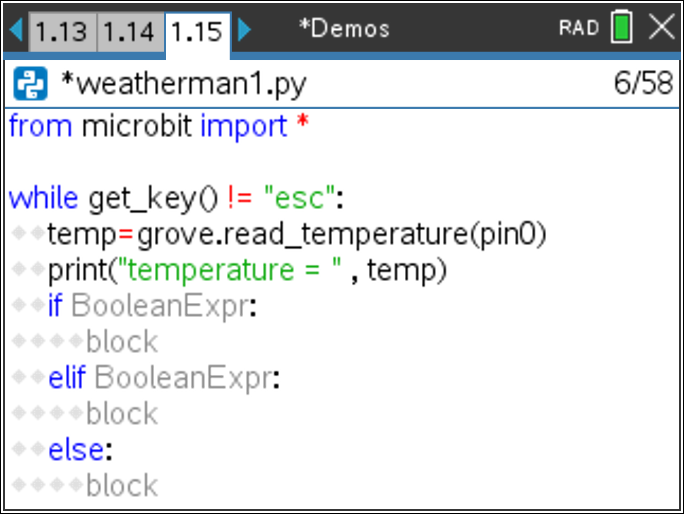
Step 10
The If… block will handle the ‘cold’ temperature. When it’s cold, we’ll display the ANGRY face:
if t < 15:
display.show(Image.ANGRY)
Find display.show( ) on the BBC microbit > Display menu and select ANGRY from the pop-up list of images.
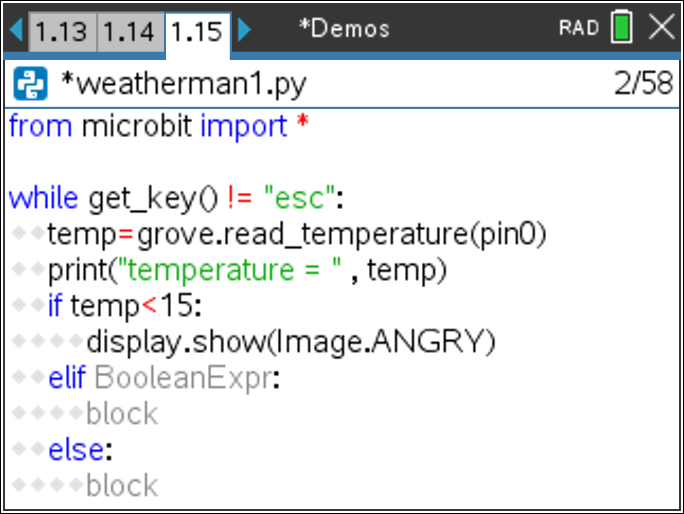
Step 11
The elf : block also requires a condition since it stands for ‘else if…’. This time we check for ‘comfortable’ temperatures between 15 and 27 degrees Celsius. But the first condition took care of the lower bound so we only need to check the upper bound:
elif temp < 27 :
and we can display the HAPPY face:
display.show(Image.HAPPY)
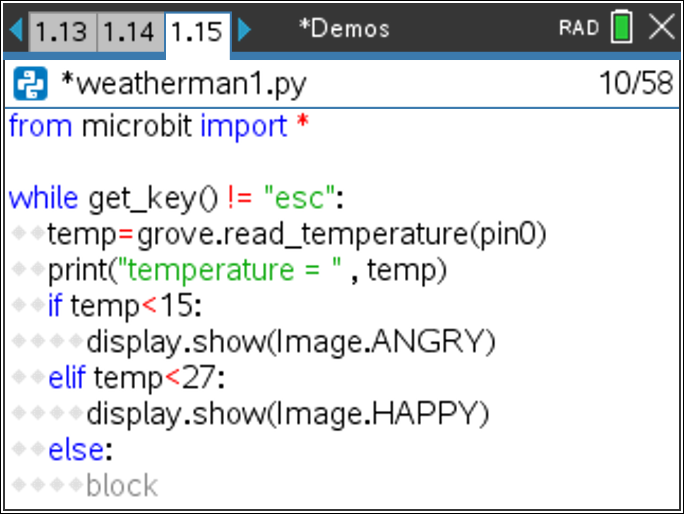
Step 12
What face would you like to show when it’s ‘hot’? Add the statement to display another face in the else: block.
To test your program, you will have to subject the temperature sensor to a range of temperatures. You can place it in a refrigerator for a cool temperature but be careful when looking for a warm temperature. A hot day outside is OK but do not place the sensor in an oven or microwave and do not get it wet. Try rubbing your hands together (feel the heat?) and then place the sensor between your hands.
Can you see all three faces on the micro:bit?
Now try this…
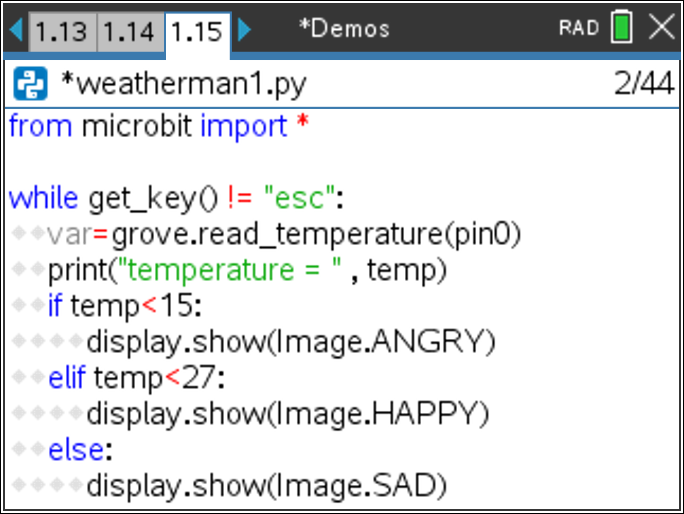
Step 13
Part 2: Tracking Temperature
Let’s monitor the temperature for a while. Use two lists to collect ‘time’ and temperature data and transfer the lists to the TI-Nspire™ CX for further study.
Make a copy of your weatheman1.py program (or whatever you called it).
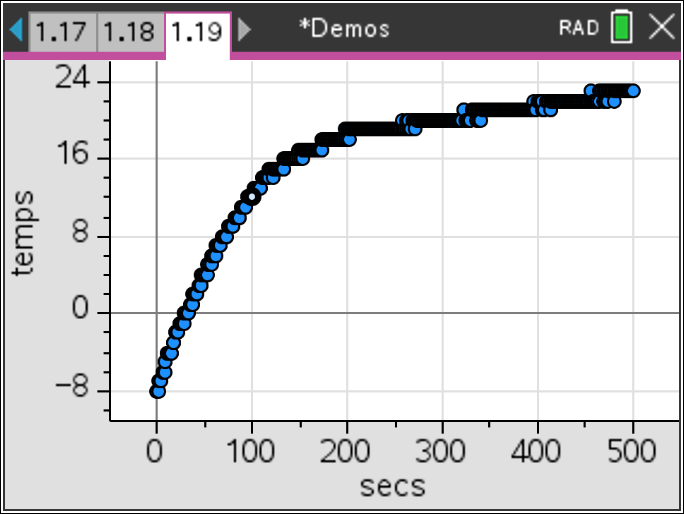
Step 14
In your new program, before the while loop, create two empty lists
times = [ ]
temps = [ ]
and a timer variable. Call it tim so that the variable does not interfere with the time module:
tim = 0
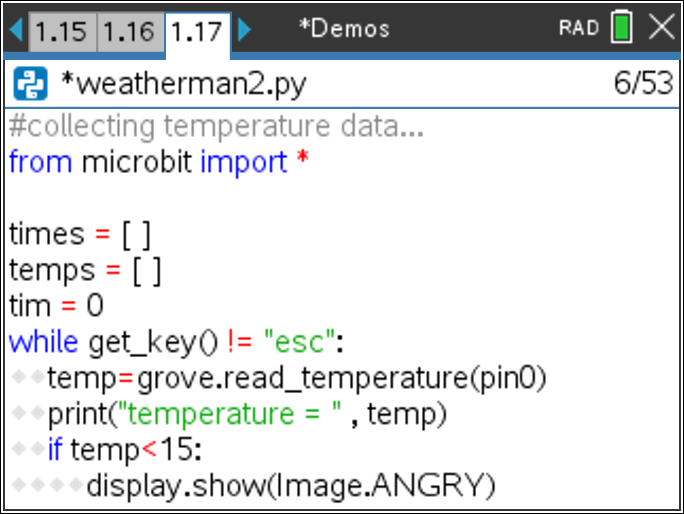
Step 15
Below the print statement, add (append) the current values of time tim and temp to their respective lists:
times.append(tim)
temps.append(t)
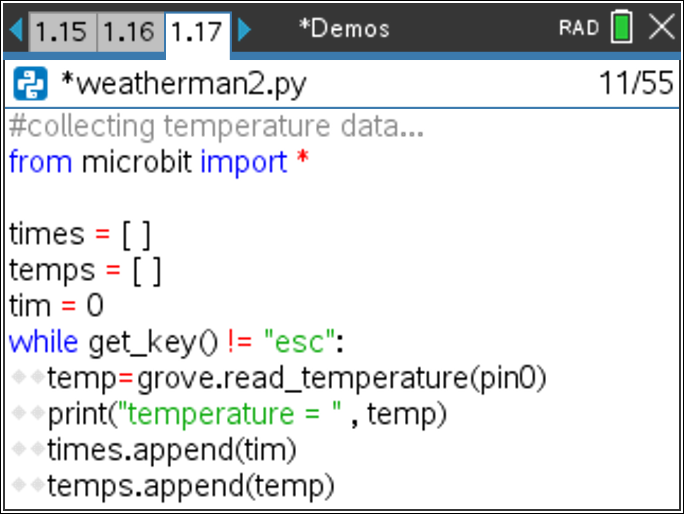
Step 16
sleep(1000)optional!
tim += 1increment the tim variable representing one sample.
These two statements are indented to be part of the while loop block.
Note about timing: since it takes time to read the temperature sensor, to print the temperature, and to display the face image, the sleep(1000) pause is too large. So the tim variable is really just a sample counter. If you want the tim variable to actually represent seconds there are more accurate methods using the internal clock (time functions).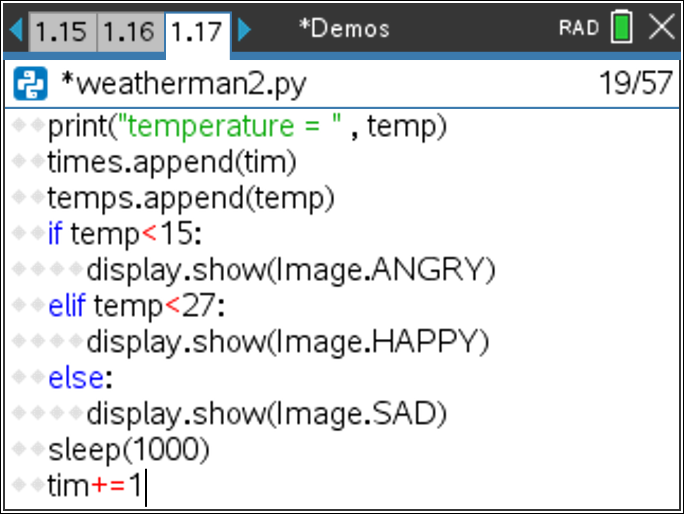
Step 17
When the while loop ends, transfer the two lists to two TI-Nspire™ lists using the function store_list( ). This function is included in the BBC micro:bit module and can be found on the > BBC micro:bit > Commands menu.
We need two store_list( , ) functions:
store_list(“TIMES”,times)
store_list(“TEMPS”, temps)
We can use the same list names as the python variable names but the TI-Nspire™ names must be in quotes.
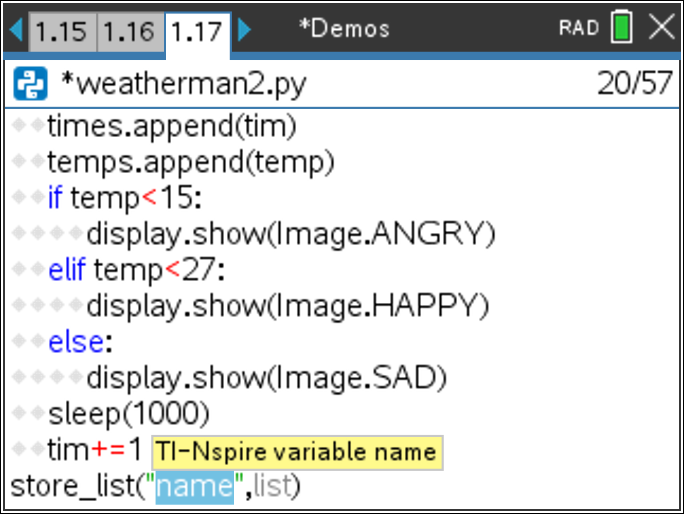
Step 18
After running your program for a while, when pressing [esc], the two lists are stored in the TI-Nspire™. Add a Data & Statistics app to your document and set up a scatter plot of the two lists by clicking the prompts on the bottom and left side of the screen. You will also have to adjust the viewing window, too.
The plot seen here was created by putting the temperature sensor in a freezer for a few minutes to get it really cold (-8C) then, taking the sensor out of the freezer, running the program to watch It warm up to room temperature (24C).
How do you explain this behavior?
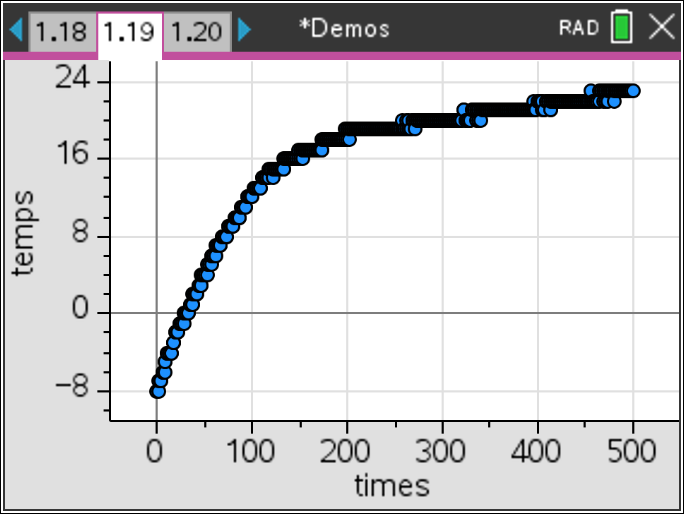
Step 19
Challenge: you can also use ti_plotlib in your program to plot the data as it is being collected. If you have not yet used ti_plotlib, see the ti_plotlib activities in the [+] Python Modules section of this site.
The plot seen here was created by placing the sensor in a freezer for a while.
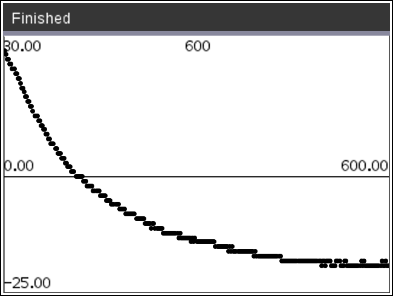
Mini project 3: Intruder alert
Download documentsThe Ultrasonic Ranger can be used to detect a change in distance. This concept can be used to build an alarm-type system that triggers lights and sounds when the distance is not within an allowed range. This activity develops the basic tools for an alarm system and the idea can be adapted to lots of different applications.
This alarm will trigger when a small box containing the ranger is opened.
Step 1
Before you begin, be sure that:
- your micro:bit is connected to your TI-Nspire™ CX II
- your micro:bit is inserted in the expansion board.
(Grove shield shown here) - the Grove accessories you will need are coming up…
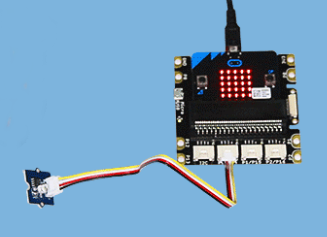
Step 2
Your micro:bit should look like this when it has power from the TI-Nspire™ graphing calculator:
Recall that the display on the micro:bit is showing the TI logo, an icon of the state of Texas with a bright spot near Dallas, the home of Texas Instruments, Inc.
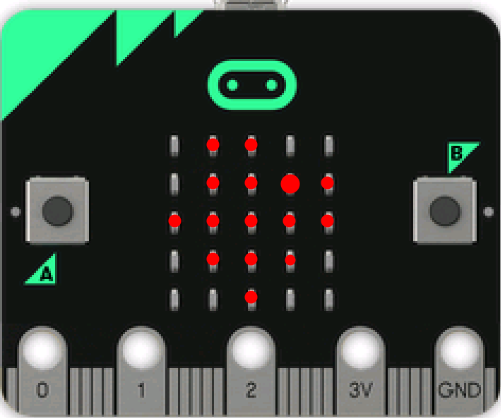
Step 3
This activity uses three external devices: the ultrasonic ranger, a speaker, and the LED that was used in the Light Switch activity. On the LED board, remember to turn the yellow dial (a potentiometer) all the way to the right to get the maximum brightness. Make the following micro:bit connections:
pin0: speaker
pin1: ranger
pin2: LED
Note micro:bit version 2 has an on-board speaker. An external speaker can also be used. We will also use the micro:bit display board to flash lights in a custom pattern.
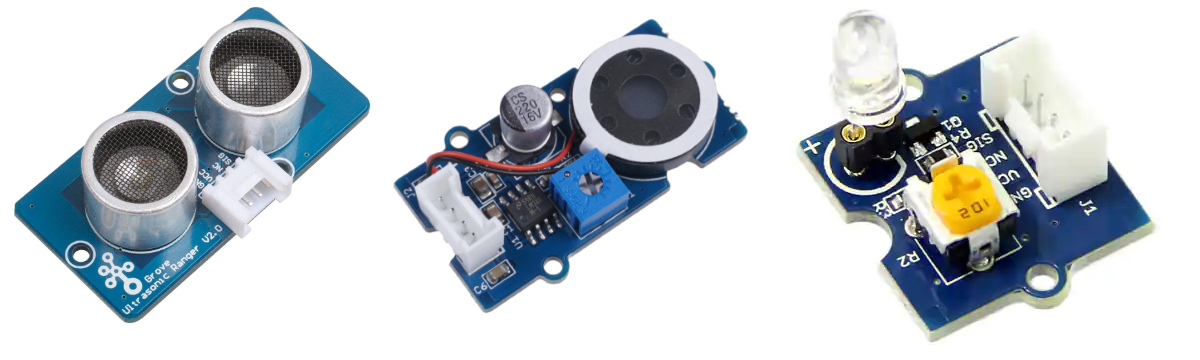
Step 4
Start a new TI-Nspire™ document and select Add Python > New to begin a new program (blank program), named ‘intruder1’. In the Python Editor use [menu] > More Modules > BBC micro:bit to select the import statement at the top of the menu items:
from microbit import *
Tip: If the message ‘micro:bit not connected’ ever appears, just unplug the micro:bit and plug it in again (reset).
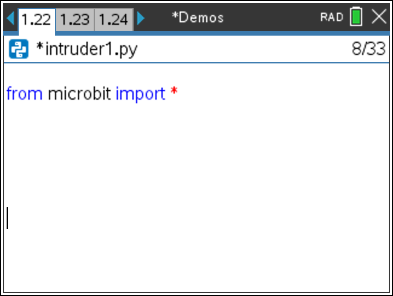
Step 5
The program is divided into two parts:
- a loop that ‘watches’ the distance, and
- another loop that produces the alarm effects: lights and sounds.
There are two while loops in the program…
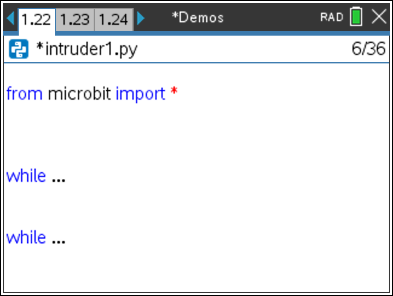
Step 6
The first loop simply monitors the distance from the ranger that is connected to pin1 on the expansion board:
dist = grove.read_ranger_cm(pin1)
while dist < 10:
dist = grove.read_ranger_cm(pin1)
sleep(250)
Recall that the ranger will be inside a small (<10cm high) closed box with a lid. The ranger (not the micro:bit) is placed on the inside bottom of the box facing upward to detect when the box cover is opened. If the lid is closed this loop will continue. When the lid is opened this loop ends.
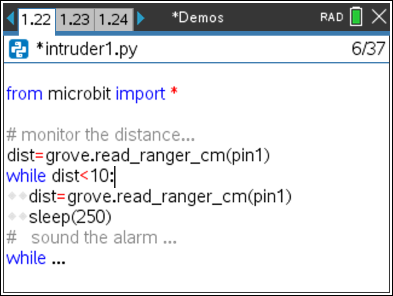
Step 7
Sounding the alarm:
The second loop creates the alarm effects. If you have an external speaker and LED you can use those to produce lights and sound. You can also use the on-board display and speaker (speaker is on micro:bit version 2 only).
This second loop ends when a ‘special key’ is pressed. We will use the [esc] key as usual but in practice you should use a special ‘password’ key to stop the alarm:
while get_key() != “esc”:
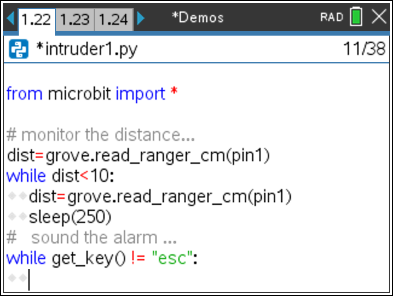
Step 8
To make the LED turn on and an alarm to sound, use the functions
grove.set_power(pin2, 100)
music.pitch(440, 100)
pin2 is the connection for the LED
100 is full intensity (brightness)
music.pitch( ) is found on > micro:bit > music
440 is 440Hz which is middle A on the music scale
100 is 0.1 seconds (100 milliseconds)
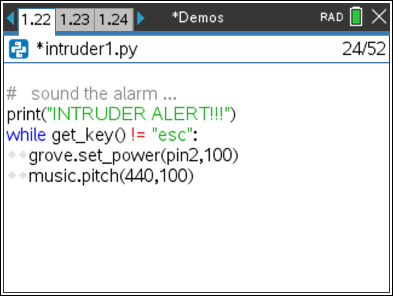
Step 9
To make the LED blink and the alarm to sound ‘alarming’ add two more statements to turn the LED off and change the musical note:
grove.power(pin2, 0)
music.pitch(220, 100)
You can choose other frequencies for the musical tones.
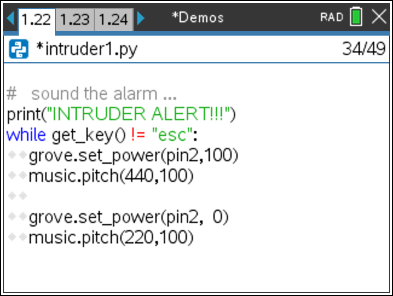
Step 10
Let’s add a flashing display effect on the micro:bit using
display.show( ).
The display can show any of the images in the built-in collection or you can make your own custom images using the Image( ) function found on the > micro:bit > Display menu shown here.
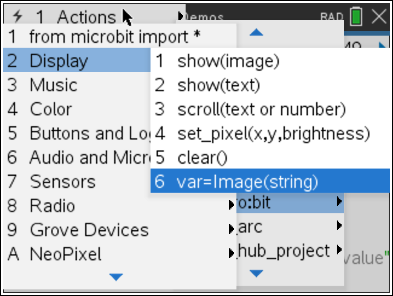
Step 11a
Here is a sample custom image:
The variable name is img_alarm1
The argument is a “string” of 25 digits (each from 0 to 9 indicating brightness) for each of the 25 pixels on the display. Each row of 5 values is separate by a colon (:).
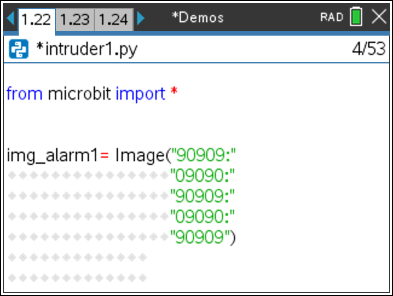
Step 11b
It is convenient to write the string on five separate lines to arrange the digits as they will appear on the display (in a 5x5 grid):
Image("90909:"
"09090:"
"90909:"
"09090:"
"90909")
But it is also possible to write the string in one long line:
Image("90909:09090:90909:09090:90909")
or with extra quotes:
Image("90909:””09090:””90909:””09090:””90909")
Python will concatenate the separate strings for you!
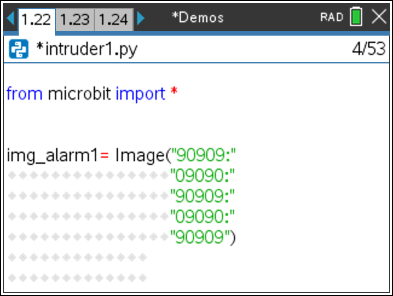
Step 12
img_alarm1 lights up every other pixel on the display in a ‘checkerboard’ pattern.
Make another image which does the opposite pattern:
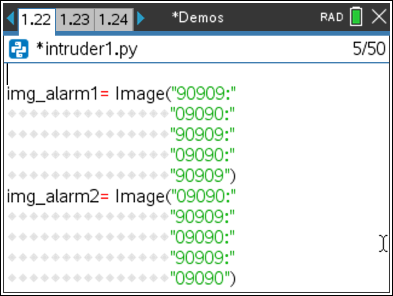
Step 13
Finally, to get the display to flash when the alarm goes on, add two statements to your alarm code to display the two images (at the right moments!)
display.show( ) is on >micro:bit > Display
Type the names of your custom image variables as the arguments.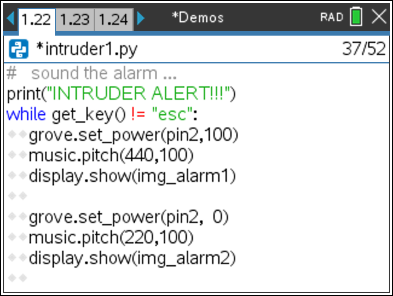
- MP 1
- MP 2
- MP 3
Tello Drone
Mini project 1: Hello Tello
Download documents
These mini-projects are designed to help you learn to operate the Tello flying drone using your TI-Nspire™ CX II with Python programming.
They assume you have a working knowledge of Python programming on the TI-Nspire™ CX II. While the hardware setup requires a micro:bit, expansion board, and wi-fi adapter, the Tello functions and methods do not require coding skills involving these intermediate devices.
Note: These activities are compatible with the Tello (White), Tello EDU (Black), and Tello TT.
Intro 2
The TI-Nspire™ CX II communicates with the Tello using:
- the Tello Python module
- mini-OTG USB to micro-USB cable
- a BBC micro:bit V2 with the TI runtime hex file
- a Grove BitMaker V2 expansion board
- a Grove Wi-Fi V2 module
- an external battery (either USB or micro:bit AAA battery pack)
The setup seen here involves connecting the hardware, installing the Tello Python module to your TI-Nspire™ CX II, installing the special TI-developed micro:bit .hex file, and then configuring the system (pairing the Tello drone with the micro:bit).
You can watch the YouTube videos for an overview of this process or read the setup instructions included in the download package. The necessary files (tello.tns and ti_runtime.hex) along with detailed step-by-step instructions are available here.
This first mini-project just gets the Tello drone off the ground…
Intro 3
Tello needs to ‘see’ the ground and a colorful, patterned surface helps Tello maintain its position in the air. Tello has a camera on the bottom and a ‘Vision Positioning System’ to help it stay in one place while hovering. If the surface below Tello is a plain, solid color (that is, no pattern) then Tello switches to an ‘Attitude Positioning System’ which is not as reliable as its Vision System so be careful. The blinking LED on the front of Tello indicates which system is in use (green=Vision, red= Attitude). This is explained in more detail in the Tello User Manual.
Proceed to the next step to program your TI-Nspire™ to control Tello when your hardware and software are ready.
Step 1
In a TI-Nspire document, start a new Python program (ours is called first_tello.py). Import the tello module using [menu] > More Modules > Tello and select
from tello import *
Note: if you do not see Tello on the More Modules menu, review the installing module section in the video or Getting Started guide.
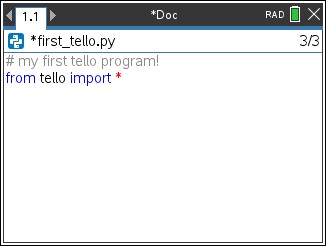
Step 2
Before proceeding, ensure you’ve completed the one-time activation and pairing of your Tello.
Step 3
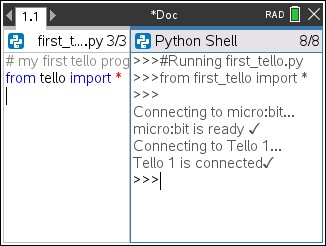
Note: the split-screen in the image is just
the program. Your Shell will appear on a
page after the program page.
Make sure the Tello is turned on. Wait until the LED on the front of the Tello is rapidly blinking yellow. This indicates Tello is ready. Check your connections now by running the program. You should see:
Connecting to micro:bit...
micro:bit is ready ✓
Connecting to Tello 1...
Tello 1 is connected✓
>>>
You may have assigned a different name to your Tello during pairing. The Tello used here is named ‘Tello 1’.
If you experience a connection problem, reference the troubleshooting section in the Getting Started guide.Step 4
A good practice is to check the Tello battery charge at the beginning of your programs.
Get the battery( ) function from
[menu] > More Modules > Tello > Flight Data
And replace var with any variable name: b is a common choice, for ‘battery’. When running, this function reports the battery charge level in a percent. If the charge is too low(<10%), Tello will not fly.
Note: there’s no need to print(b). The battery() function, as well as most other Tello methods, displays information for you.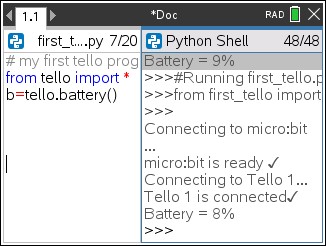
Step 5
If the connections are successful and there's enough battery charge, then prepare to fly!
The takeoff() command causes the Tello to fly upwards about 70 - 80cm (about 27-32 inches). Be sure there is room above the Tello for this maneuver.
Also, what goes up must come down. We must have full control of the drone at all times, so we must make the Tello land() as well.
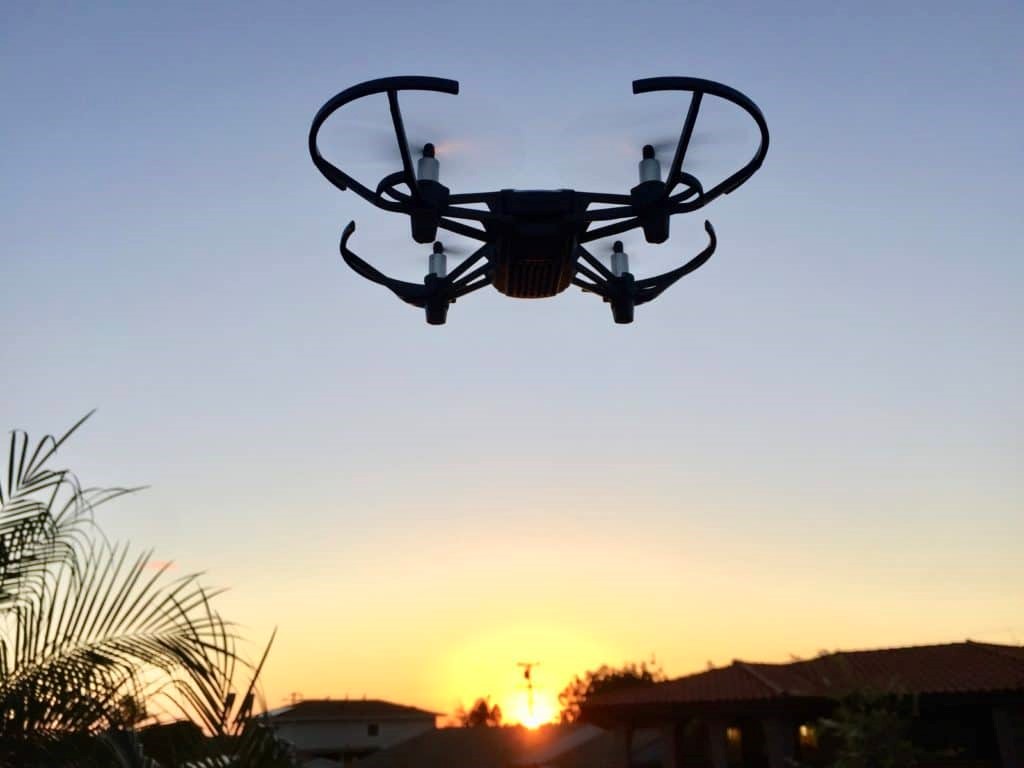
Step 6
In your program, add these two statements, both found on
[menu] > More Modules > Tello > Fly
tello.takeoff()
tello.land()
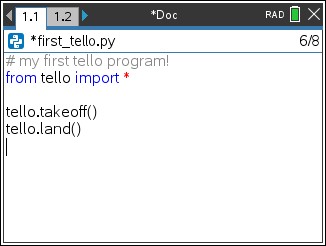
Step 7
Again, make sure all hardware is on, including Tello, and run the program. Your Shell screen will display the previous ‘Connecting…’ messages and then show messages for each command that is sent to the Tello. The Tello will go up, hover for a few seconds, and then land.
Tello should land very close to its starting location. If it drifts a little bit that’s OK, but if it wanders too far, then Tello is having trouble ‘seeing’ the ground. Be sure the surface has a colorful pattern and that the area is well-lit.
Tello communicates with the micro:bit and the TI-Nspire™ to confirm that the tasks are properly Completed ✓.
After Tello lands, the program is finished.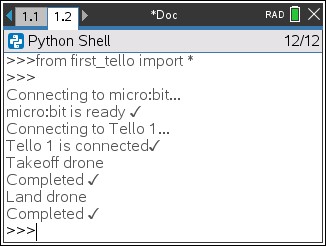
Step 8
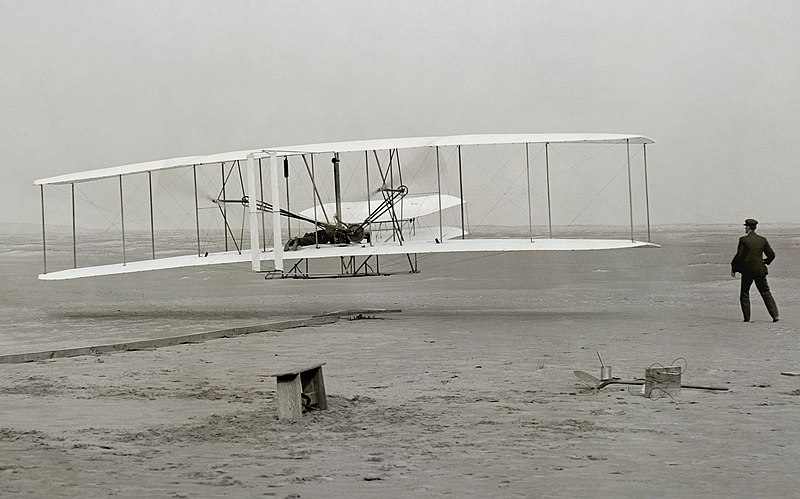
* John T. Daniels, Public domain, via Wikimedia Commons
https://commons.wikimedia.org/wiki/File:First_flight2.jpg
Congratulations on your first successful flight! The Wright Brothers would be proud of you!
Let’s try another maneuver…
Step 9
The Tello > Fly menu contains lots of possible maneuvers.
Two simple but elegant maneuvers are the turn_right( ) and turn_left( ) methods. These keep the Tello in one position and rotate it the given number of degrees.
Try
tello.turn_right(360)
tello.turn_left(360)
Insert these functions between the takeoff() and land() methods in your program and enter the number of degrees to turn as each argument.
See the next step for the code…
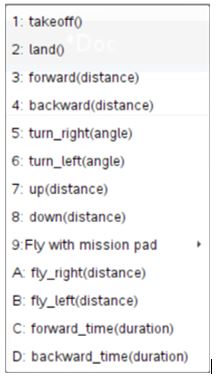
Step 10
Your program should look like the one shown here.
Again, re-check your hardware, including Tello itself and then run the program. Tello turns itself off after a few minutes of inactivity to save the battery.
Tello will take off, spin to the right a full turn, then spin to the left a full turn, and then land.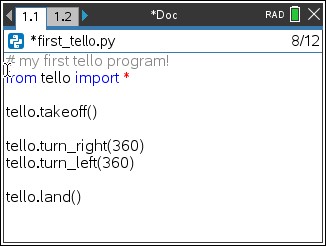
Step 11
While the program is running, your TI-Nspire screen will report the progress of each maneuver.
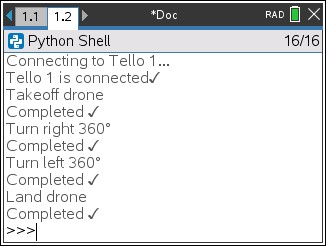
Step 12
Another pair of maneuvers to use are tello.up( ) and tello.down( ) which are also found on the Tello > Fly menu.
The distance argument is measured in centimeters. Be sure that when flying upward, there is room above to make the maneuver. Tello does not have a camera or sensor on top so it may crash into the ceiling!
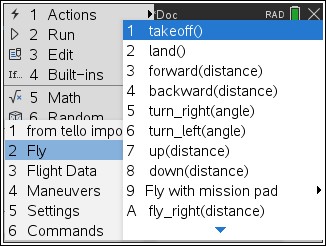
Step 13
Fail: When using tello.down( ), Tello cannot go below about 30cm (12 inches) from the floor. Tello does have a sensor on the bottom to measure its height above the ground. In the screen to the right, after takeoff, Tello attempted to go down 100cm as the code requested, but only went down about 50cm, where it reached the 30cm limit. It hovered there for a while then reported "Fail" and landed the drone.
The program's land( ) function also failed because the drone had already landed.
There are other situations where an operation can ‘Fail’.
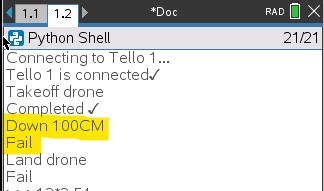
Mini project 2: The One Where Tello Tours the Room
Download documentsAfter successfully completing mini-project 1 your TI-Nspire™ CX II-to-Tello system is properly set up and ready to fly. In this project your Tello will depart its landing zone and take a tour of your room based on your measurements.
Remember the caution from project 1: the surface that Tello flies over needs to be a varied pattern. If the ‘floor’ is a smooth, uniform color then Tello will have difficulty maintaining its ‘Vision Positioning System’ and could possibly wander off course. And be sure the flight area (the entire room) is well-lit so that Tello can ‘see’ the ground. Keep a watchful eye on Tello as it flies around the room. Tello does not look forward.
Also be aware of the surroundings. Ensure your flying space is clear of people and objects.
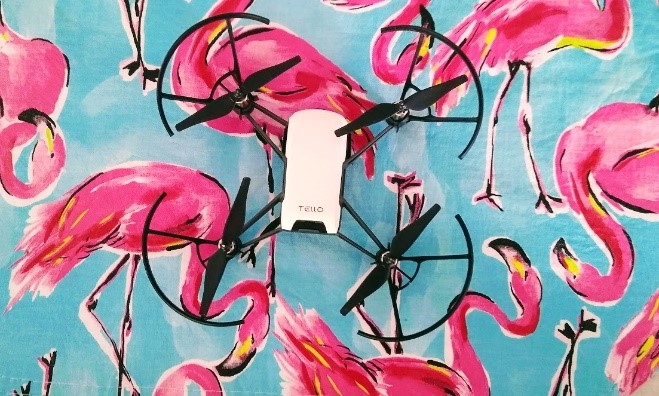
Intro 2
The room you are working in is likely in the shape of a rectangle. Let’s start the Tello in the center of the room, fly toward one wall, then fly along the four walls and return to the center of the room. See the red route pictured here.
In order to perform this task, you need to measure the dimensions of the room in feet. Use a regular carpenter's tape measure to measure the room. Write down your measurements to use in the program.
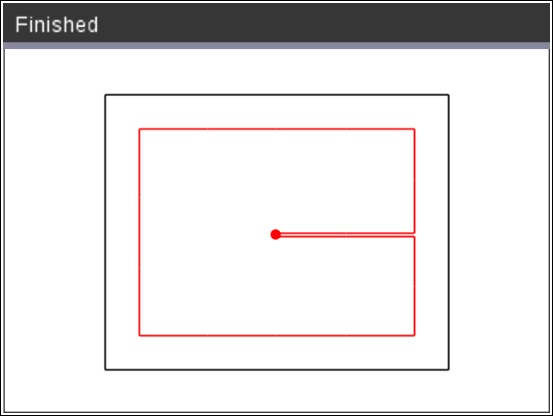
Step 1
Begin a new Python program (ours is called tello_tour.py). Import the Tello module from [menu] > More Modules > Tello
from tello import *
and perform a pre-flight check of the battery level before flying:
b = tello.battery( )
found on [menu] More Modules > Tello > Flight data. Use a variable in place of the var placeholder. If the battery is too low (10% or less) Tello will refuse to take off. If you think there is not enough charge to complete your mission, you can stop the program by pressing [on] on the keypad.
Tello will eventually land.
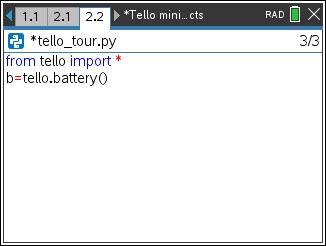
Step 2
Since you measured the room in feet and Tello only accepts measurements in centimeters, define a function to convert feet to centimeters. Get
def ( ) :
from [menu] > Built-ins > Functions
Also, get the return statement from the same menu and be sure it is indented to be the last statement of the function. We fill in the details next…
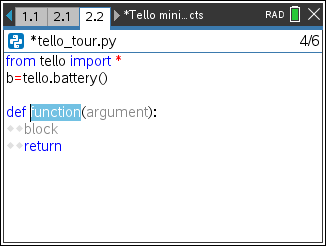
Step 3
The function name can be any identifier, such as f. We prefer to use a meaningful name, so we’ll use ft_to_cm and use the argument ft to represent the incoming value, a distance measured in feet.
The block of the function is the formula that converts feet to centimeters:
cm = ft * 12 * 2.54
and the return statement uses the variable cm to send its value back to the program. The completed function is:
def ft_to_cm( ft ):
cm = ft * 12 * 2.54
return cm
You will use this function in the Tello Fly methods that require a distance. Now to code the main flight plan.
Step 4
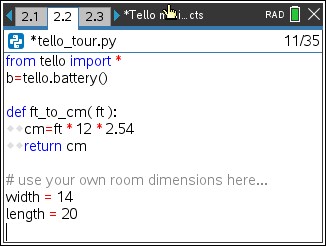
Note: To make a more user-friendly program you can use two input functions to enter these values, but who wants to type in two numbers every time you test the program?
Note that Tello’s maximum distance for a leg is 500cm, or about 16.4 feet. If your room is larger, then limit the dimensions so that Tello does not exceed this travel limit in any one leg of the journey.
Step 5
Recall that our flight plan has Tello start in the center of the facing one wall (width in our code). The front of Tello has the tiny status LED. The back holds the battery.
answer: length/2
but we don’t want Tello to crash into the wall, so we stop short of the wall, say leaving 2 feet of space to the wall:
length / 2 – 2 Note: The drawing to the right was made with Turtle graphics.
Step 6
In your program, issue the takeoff function, then use the forward function, both found on [menu] > More Modules > Tello > Fly
The forward(distance) argument is a value in centimeters, but our distance to fly, length / 2 – 2, is in feet so we use our conversion function as part of the argument:
tello.forward( ft_to_cm(length / 2 – 2) )
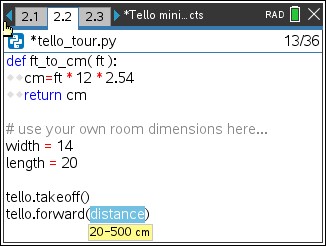
Step 7
For testing purposes, temporarily use the tello.backward( ) function to return Tello to its starting location to make sure that Tello behaves properly. Use the same argument as in the tello.forward( ) function.
Then use the tello.land( ) function.
Remember that all tello.fly methods are on[menu] > More Modules > Tello > Fly Double-check your code, press ctrl-B to check syntax, stand back, make sure the flight path is clear, then run the program (ctrl-R).
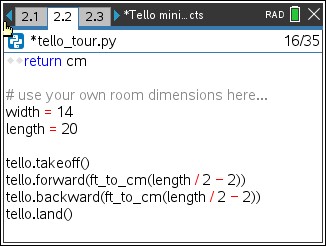
Step 8
If Tello returns to (approximately) its starting position, then you can complete the program to fly around the room. The next portion of the path travels along the wall (the bold red line seen here).
What is the length of this segment?
Answer: width / 2 - 2
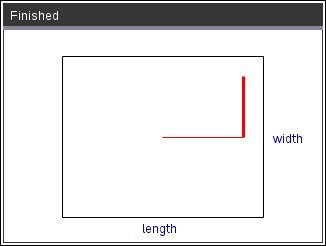
Step 9
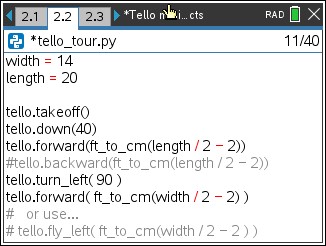
tello.fly_left( distance ) makes Tello fly to its left without turning.
If it is safe to land in the corner, then use the tello.land( ) function and test your flight now. And try both methods mentioned above as shown in the image as a #comment.
For the rest of the tour, you should stick with the method you use here: either (turning and forward) or just (flying in different directions).
Step 10
The third leg of the trip is along the wall. How long is this segment?
Answer: length – 4
Remember that we must stay 2 feet from all walls.
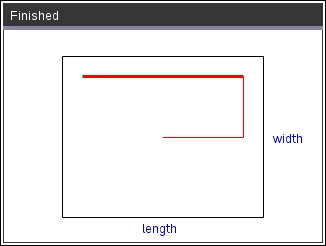
Step 11
tello.turn_left(90)
tello.forward( distance ) assuming Tello is always flying forward
or
tello.backward( distance ) assuming Tello is not turning.
In either case, the distance is ft_to_cm(length – 4) determined in the previous step.
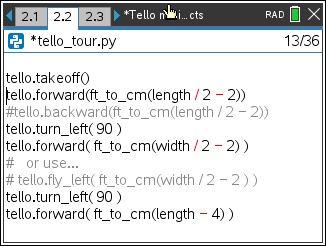
Step 12
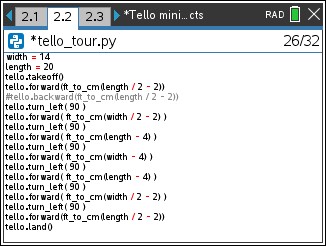
Font size adjusted in ‘Actions/Settings’ .
tello.forward(ft_to_cm(length / 2 - 2))
#tello.backward(ft_to_cm(length / 2 - 2))
tello.turn_left( 90 )
tello.forward( ft_to_cm(width / 2 - 2) )
tello.turn_left( 90 )
tello.forward( ft_to_cm(length - 4) )
tello.turn_left( 90 )
tello.forward( ft_to_cm(width - 4) )
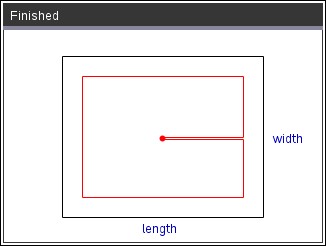
tello.forward( ft_to_cm(length - 4) )
tello.turn_left( 90 )
tello.forward( ft_to_cm(width / 2 - 2) )
tello.turn_left( 90 )
tello.forward(ft_to_cm(length / 2 - 2))
tello.land()
Step 13
To travel the same route without turning use the Tello flying methods .forward( ), .fly_left( ), .backward( ), and .fly_right( ) in the proper sequence using the proper distances. The final program will be much shorter because there is no need to turn.
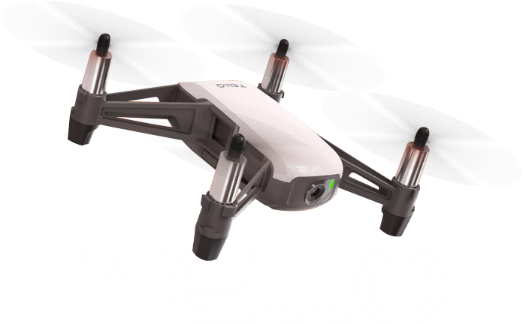
Mini project 3: Tello Search and Rescue
Download documentsOn Thanksgiving (USA) 2022, a cruise passenger fell overboard in the Gulf of Mexico. The US Coast Guard set out on a rescue mission to locate the passenger. Their search area was over 7,000 square miles. They located and rescued the passenger after he spent 20 hours treading water in the middle of the Gulf. How did they do that?
In December 2022, two men started sailing a boat from New Jersey to Florida. The Coast Guard started a search after reports the men were overdue in their journey. Eventually, the agency searched a combined 21,164 square miles from northern Florida to New Jersey. The men were rescued after 10 days at sea. How did they do that?
Intro 2
In this mini-project, you will write a Python program to conduct a coordinated ‘search’ of an area using Tello. There are several search patterns (routes) that professionals use to conduct a systematic search of a region depending on the situation.
Get your TI-Nspire™ CX II + Tello system ready to fly…
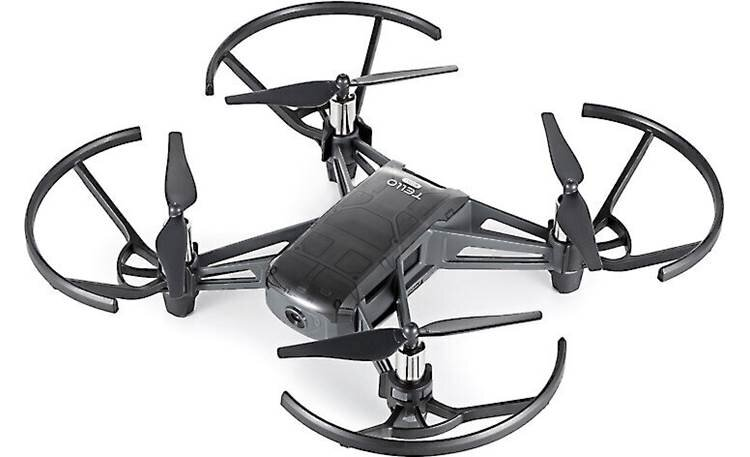
Step 1
Here is your first pattern, called the ‘parallel track search’. A drone starts in the lower left corner and flies the indicated path. At the end of the search, the drone can either land or return to its starting position.
Inputs to the program will be the length and width of the rectangular search area plus one other important measurement.
The program will determine the lengths of each of the flight segments and the number of steps needed to complete the search and then use a loop to conduct the flight.
Step 2
For the purpose of this project, length is the horizontal distance (the parallel tracks) and width is the vertical distance in the image shown here.
The other important measurement in this search pattern is called the “sweep width”, the distance between the (horizontal) flight segments.
Using length, width and sweep_width you can create an algorithm for Tello to complete this mission.
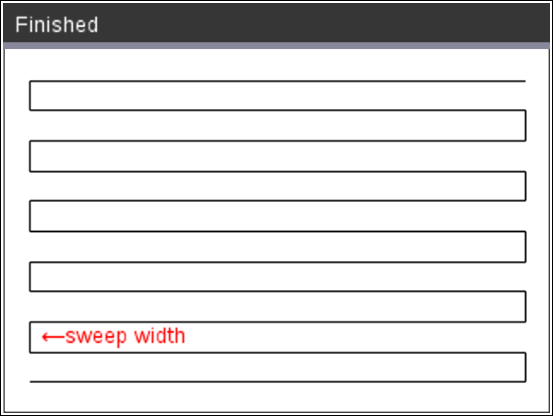
Step 3
Begin a new Python program (our is named search0.py). Import the Tello module and perform a battery check.
Use [menu] More Modules> Tello > for all the Tello functions.
Create three variables to define the search pattern:
width = 80length = 100
sweep_width = 20 # minimum distance*
These three values are all that is needed to conduct the search.
* Note: Tello flight distances are limited to be between 20 and 500 centimeters.
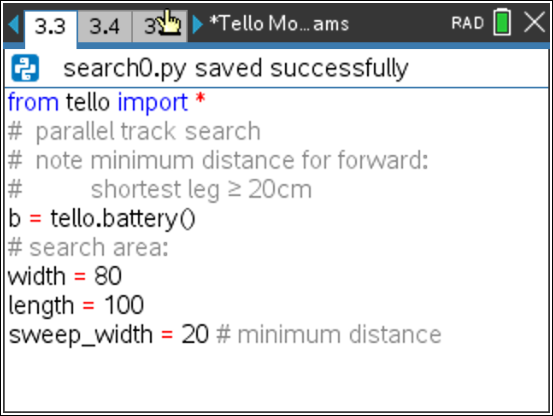
Step 4
One 'step' of the search consists of two horizontal segments and two short sweep_width segments as seen here. At the end of this path, Tello will be pointing in the same direction as it started (facing to the right in the image) so that this pattern can be repeated to complete the entire search.
We need to calculate the number of these steps needed to complete the search.
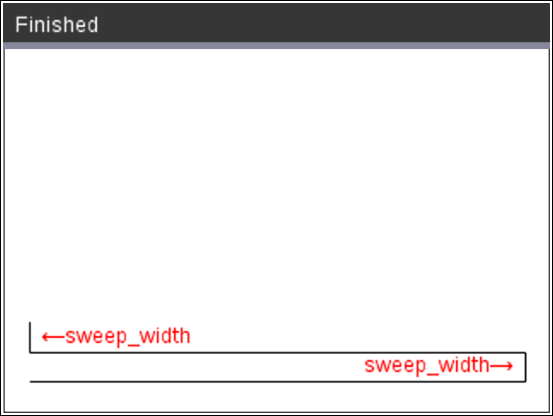
Step 5
In the image shown here, each flight segment is shown in a different color. The last (top) segment is added separately. How many steps are needed? steps depend only on the sweep_width and the width of the search area. Notice that there are two sweep_widths in each segment, so the number of steps needed is:
steps = width // (2 * sweep_width)
We use integer division (//) because this value will be used in a for loop.
Parentheses are required to control the order of operations.
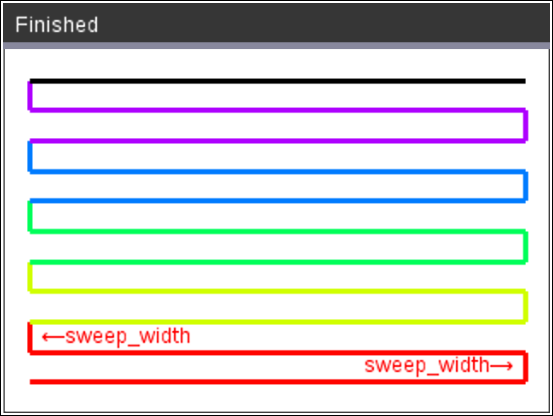
Step 6
In your program, calculate the number of steps, launch Tello, and start a for loop to conduct the search:
steps = width // (2 * sweep_width)
tello.takeoff( )
for i in range(steps):
♦ ♦
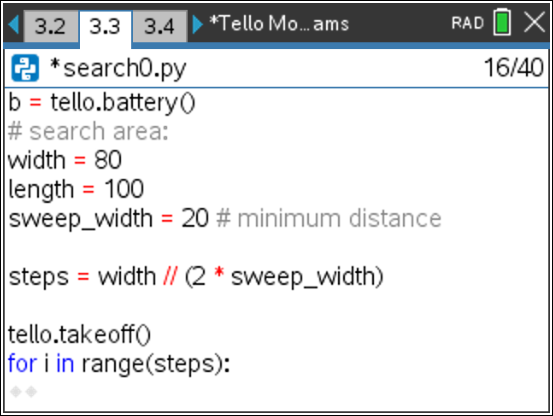
Step 7
The loop body (indented) will contain two long flights (the length of the area) and two short ones, the sweep_width. Recall from the last mini-project (Tour the Room) that there are two methods you can use: turn at each corner or just fly in the proper direction. Since we used the turn method in the last project, let’s use only the proper fly routines here.
Start by flying forward the length of the area:
♦ ♦ tello.forward(length)
Try completing the loop yourself before looking at the code in the next step…
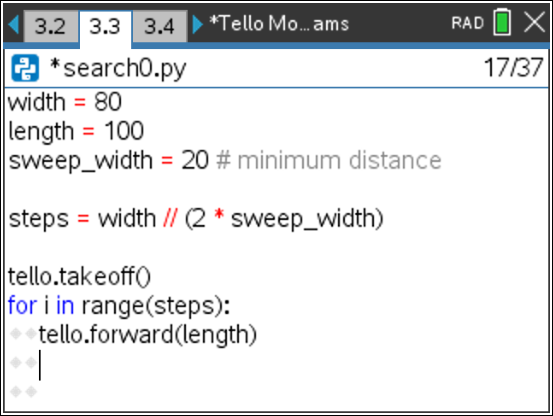
Step 8
The complete loop is:
for i in range(steps):
♦ ♦ tello.forward(length)
♦ ♦ tello.fly_left(sweep_width)
♦ ♦ tello.backward(length)
♦ ♦ tello.fly_left(sweep_width)
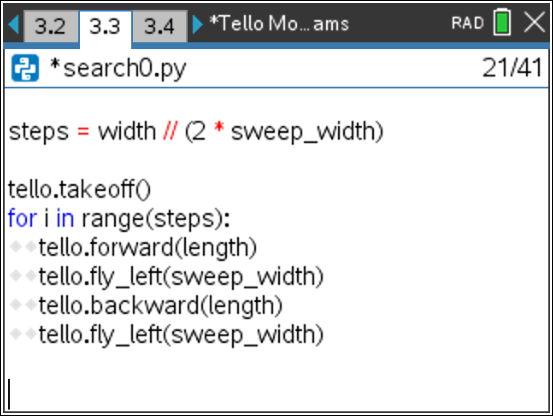
Step 9
This loop will leave Tello in the upper left corner. You have two options: quit searching here or complete the top segment to go to the upper right corner (the bold black segment pictured here). In either case, you must make sure Tello returns to the landing zone where it started because it might not be safe to land anywhere else.
We’ll take on the challenge of completing the entire pattern and then returning home…
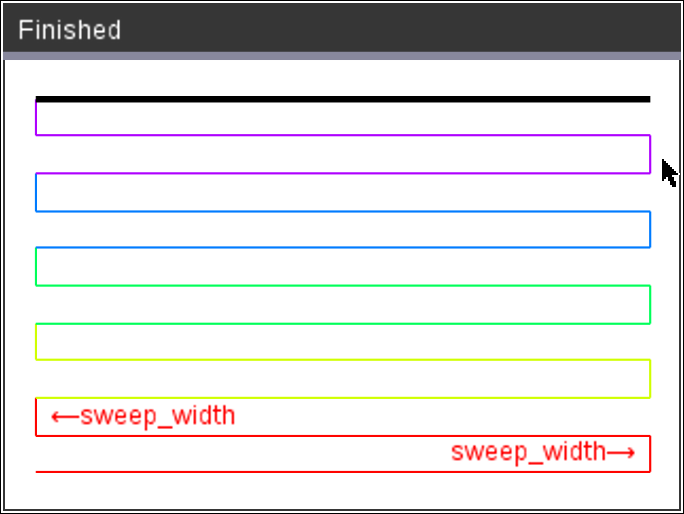
Step 10
tello.forward(length)
to complete the pattern.
This statement (and the ones that will follow) is not indented because it is not part of the for loop.
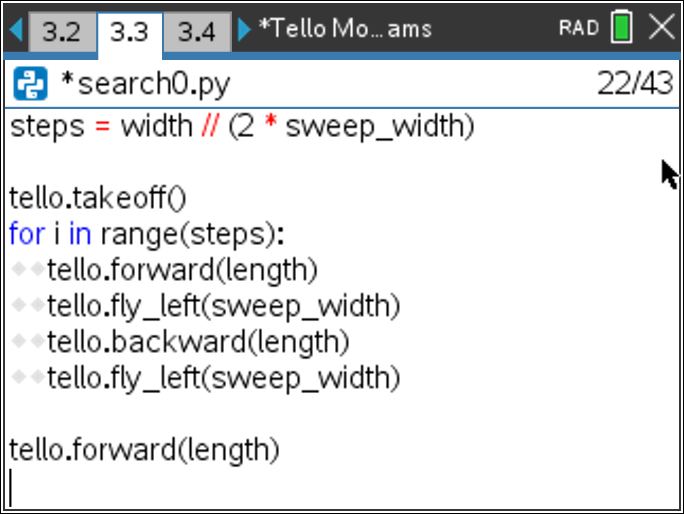
Step 11
To get Tello back to the landing zone use:
tello.fly_right(width)tello.backward(length)
tello.land()
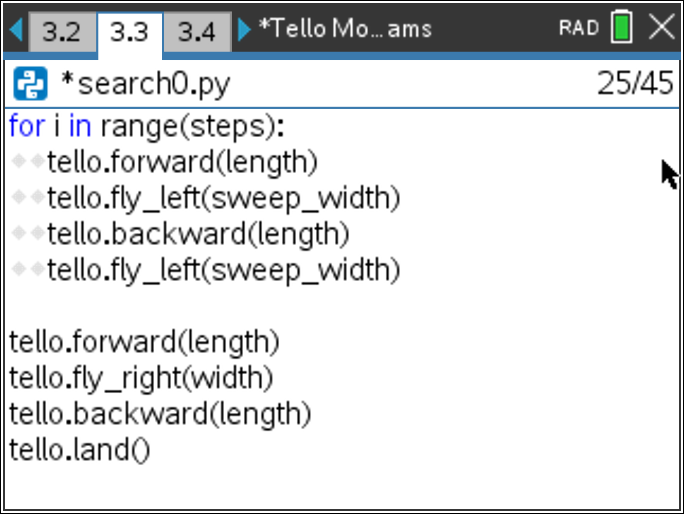
Step 12
This flight can be tricky. Start with a small search area. Since the sweep_width must be at least 20 cm, try length = 50, width = 60, sweep_width = 30 to test your code. Use a large, safe, open area (a square meter or more) for testing, Start Tello in one corner of the region, and give it a try. You should get the pattern seen here and Tello should return to its takeoff site.
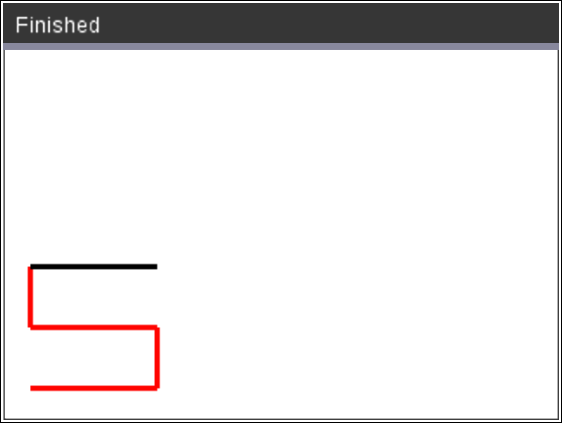
Step 13
There are other search and rescue patterns that can be found online.
Another interesting one is the “expanding box” pattern pictured (created using Turtle Graphics). Can you make Tello fly this ‘square spiral” pattern? How about other spirals?
Special thanks to the United States Coast Guard for their courage, talent, and inspiration.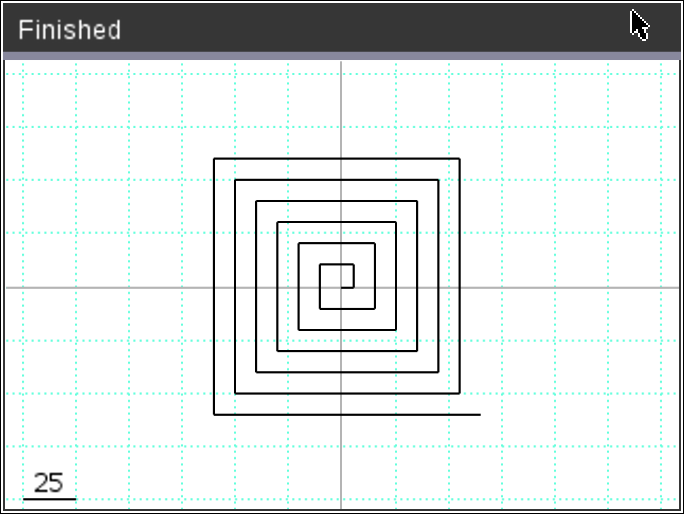
Step 14
Extensions (optional)
For additional activities to use Tello, check out the downloads here:
- Tello's Data: Tello contains sensors that can detect some information about its surroundings and position. This mini-project introduces some of these sensors and explores patterns among the data collected.
- Fly the Cube: Tello flies forward, backward, leftward, rightward, upward, & downward. It can fly in THREE DIMENSIONS (3D, also known as space). Using a special Tello “Mission Pad” assigns Tello a 3D coordinate system so that you can tell Tello to directly .goto( ) a particular point in space by giving the point’s three coordinates as a location. Note: The Tello EDU model is required.
- MP 1
- MP 2
- MP 3