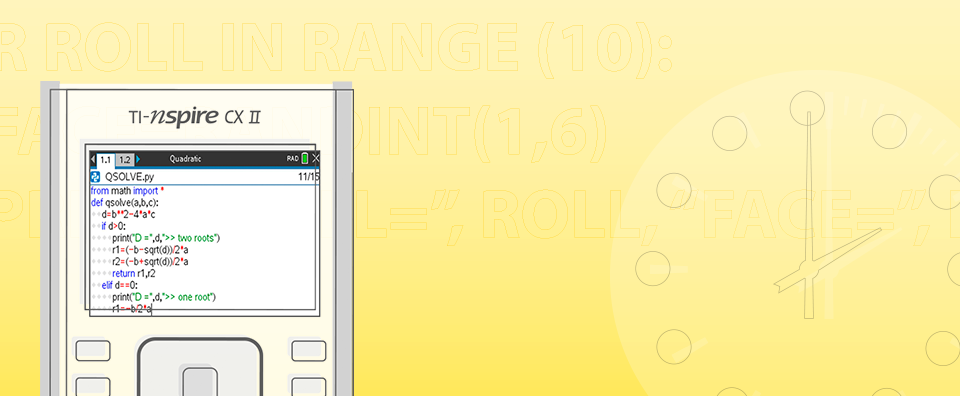
10 Minutes of Code: Python
Explore the basics of Python with these short, easy-to-master lessons that include everything beginners need to get started and succeed.
Unit 1: Getting Started with Python
Skill Builder 1: Introducing the Python Apps
Download Teacher/Student DocsIn this lesson, you will learn how to start the Python Editor and Shell apps and get to know the purpose of each of these new features.
Objectives:
- New Page > Add Python
- Use Editor and Shell
- Write your first bit of code in the Python Editor and run it
- Practice within the Python Shell
Step 1
Welcome to the Python experience on your TI-Nspire™ CX II. These lessons require no prior knowledge of programming and will help you get started with coding in the Python programming language in a friendly, easy-to-use platform in the palm of your hand. Get ready to tap the power of programming in Python…
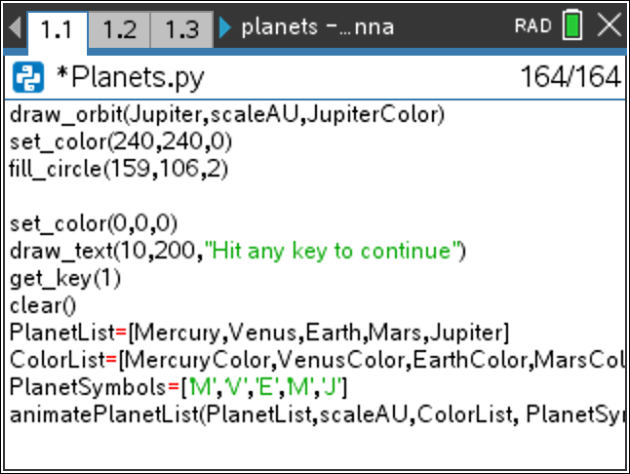
Step 2
Start a new document or press ctrl+doc to insert a page in your current document. A list of applications appears. Select
Add Python and choose New…
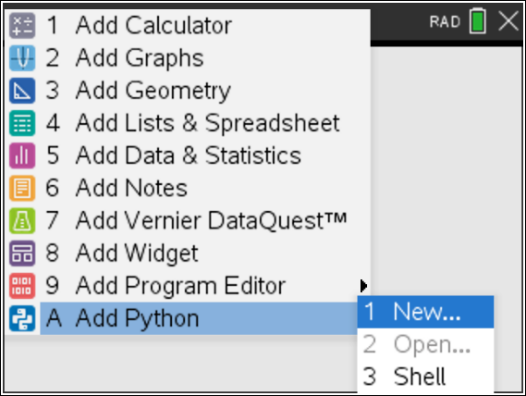
Step 3
- You are creating a Python file. The file will contain your Python code (programs, functions, and system statements). Your file Name: cannot contain spaces; keep it simple.
Name: firstWe will deal with the ‘Type:’ option later. Press enter to go to the Python Editor. If you type an invalid Python filename you will see an error message about valid names.
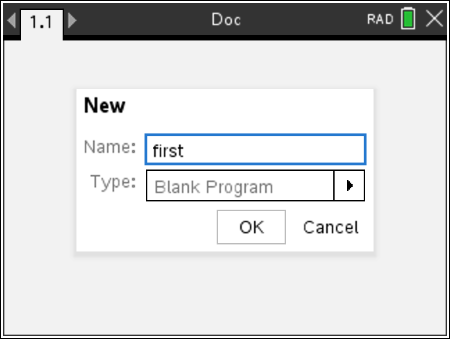
Step 4
Your very first program must simply print(something). To print plain text you must use quotes around it in addition to the parentheses:
print(“Hello, World”)
You could type the entire statement or get the print() function from
Menu > Built-ins > I/O > print()
For quotation marks, you can use either single quotes ‘ ‘ or double quotes “ “ .
The double quote is easier to locate on the keypad: ctrl+x (the multiplication key) but you only get one at a time (not like the TI-Nspire “□” template).
The single quote is on the punctuation key to the right of the letter ‘G’ on the alpha keyboard. In these lessons you will see both.
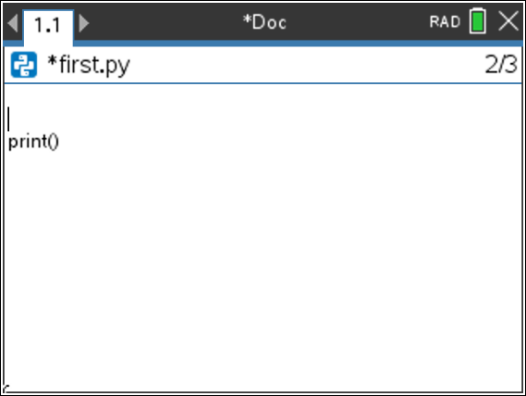
Step 5
Notice that the editor is color coded: literal strings (the text in quotes) show up as “green text” in the Editor. Use either single or double quotes. Both statements in this image are valid.
When you have the one-line statement completed, go to the next step to run the program.
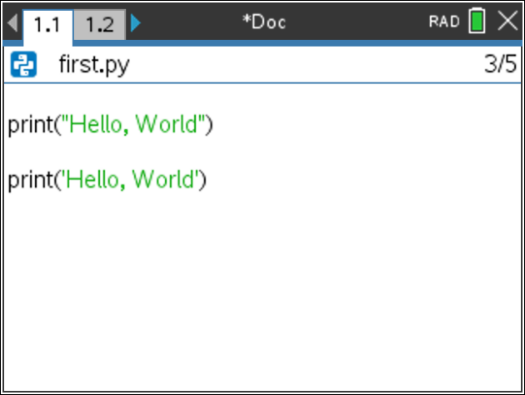
Step 6
To run the program select
menu > Run > Run
or simply press ctrl+R, which we will use from now on to run a program.
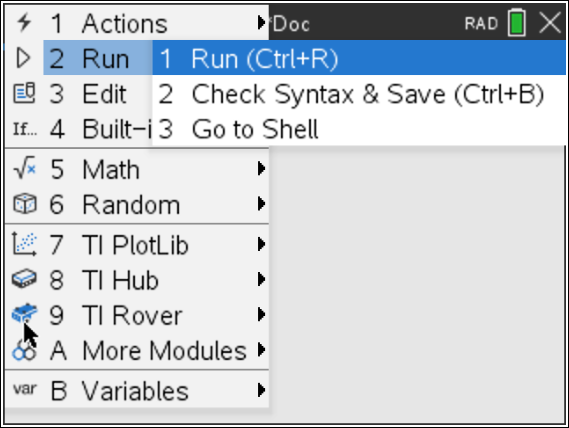
Step 7
Running the program brings you to the Python Shell on a new page in the document. The Shell is a separate app on the TI-Nspire and is responsible for interpreting your Python code and responding to Shell commands. You should see the words Hello, World on the screen, as this is what you told the computer to print().
>>> This is the Shell ‘command prompt’ indicating that the Shell is waiting to process your next command.
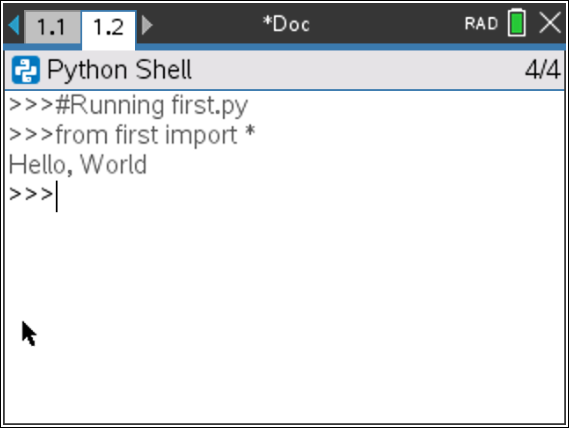
Step 8
At the Shell command prompt, >>>, you can perform calculations and test some short Python instructions.
Try some calculations: (Press enter on each line to see the effect.)
>>>2 + 3
>>>5 * 5
>>>6 * (4 - 2)
>>>3 ** 5 (raise to a power: 35)
>>>x = 13
>>>2 * x + 4
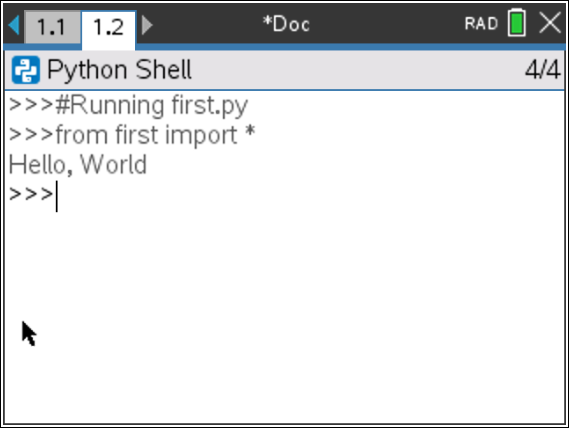
Step 9
-
Save your TI-Nspire document.
We will discuss mathematical operations and some variables in the next lesson as we write in the Editor again.
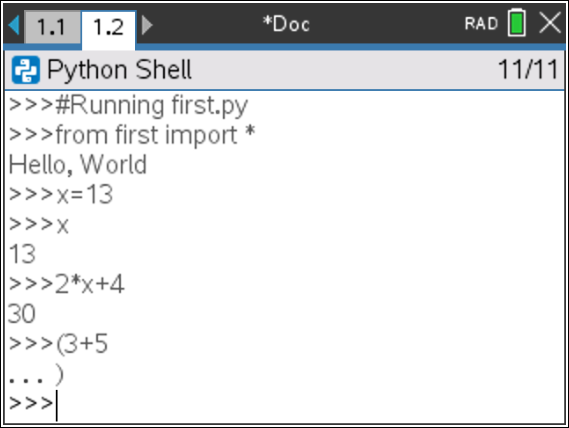
Skill Builder 2: Editing, Variables, Expressions
Download Teacher/Student DocsIn this lesson, you will work in the Python Editor, investigate the menus, use some mathematics operations, experience color highlighting, note special keypad changes, and work with different data types.
Objectives:
- Explore the menus
- Use some operators
- Learn about several of the basic types of variables
- Use the assignment statement (=)
- Experience the keyboard differences in the Editor
Step 1
Either use the TI-Nspire document you created in Skill Builder 1 or start a new document and add a Python Editor. print(‘Hello, World’) is left over from the previous lesson.
In the Editor, write the following statements:
x = 4 + 5
y = 3*x + 7
z = 5*x - 2*y
print(x, y, z)The comma (,) is to the left of the letter ‘O’ on the keypad.
Notice that none of these statement produces any results...yet.
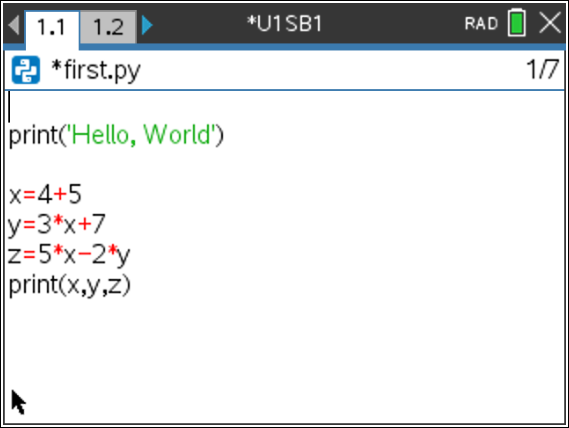
Step 2
-
Variables are letters or words that hold values. = is the ‘assignment operator’. It stores the result of the expression to its right in the variable name on the left:
x = 4 + 5
stores the value 9 in the variable named x. The variable x can then be used in other expressions, like z=5*x - 2*y and the value of x is used.
Note: Python Is case-sensitive. X and x are two different variables!
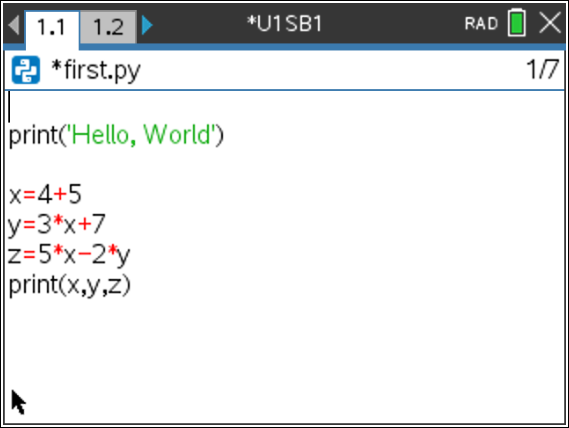
Step 3
-
What happens if you try:
4 + 5 = x
Press ctrl+B
Note: Python Is case-sensitive. X and x are two different variables!
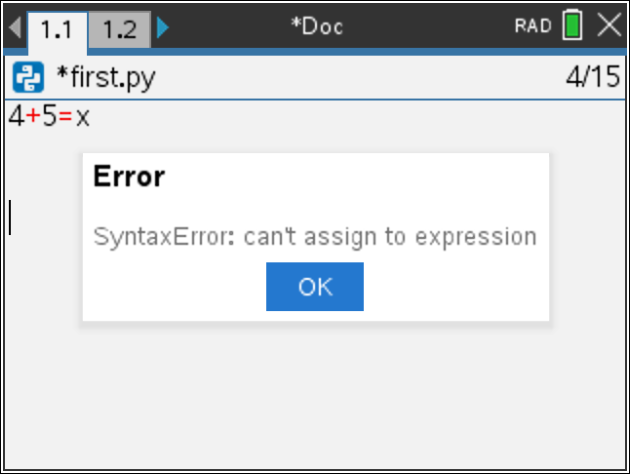
Step 4
Press ctrl+R to run this code. If you used the exact same expressions, you will see:
Hello, World
9 34 -23
>>>
That is: x is 9, y is 34, and z is -23. Do the math!
Press ctrl+left arrrow to go back to the Editor and try other expressions.
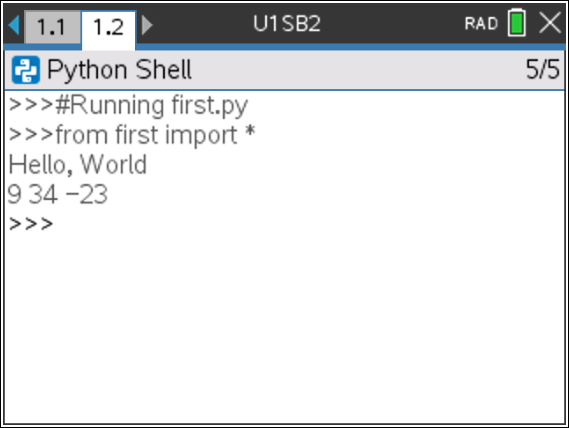
Step 5
Try the following:
x = 7
y = 3x
print(x, y)What do you get when you run this code?
Welcome to the world of computer programming! A ‘Syntax Error’ is an error in the text of the code. The English teacher calls it a grammatical error. ‘3x’ is not allowed in Python even though TI-Nspire allows it. It must be 3*x.
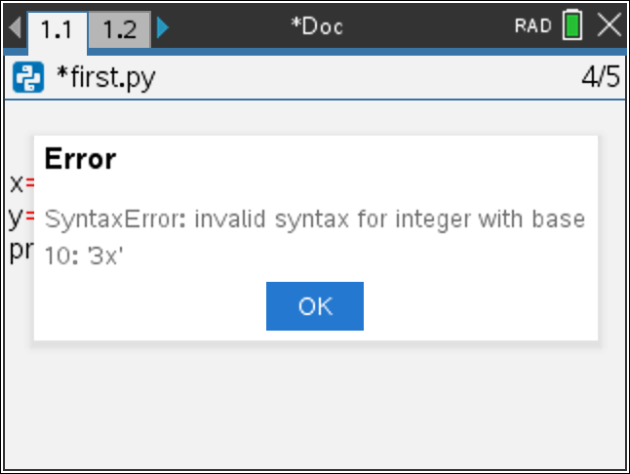
Step 6
Programming errors come in three flavors. Interpreter errors are usually reported as a ‘syntax error’ and runtime errors are detected by the computer. The third kind of error is in the head of the programmer, e.g., entering an incorrect expression (like misuse of order of operations) or logical structure (like using ‘and’ where ‘or’ is required).
Example: 5+3/7+1 vs. (5+3)/(7+1) (order of operations). Both are evaluated properly but give different results. What did the programmer intend?
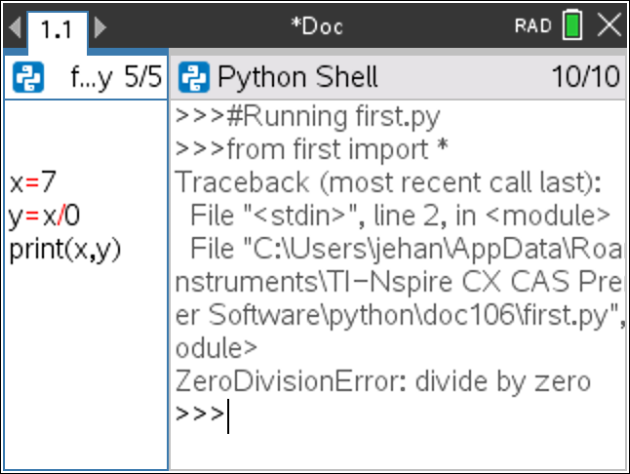
Step 7
-
Return your code to the three expressions and the print() statement from step 1.
To improve the appearance of the output, add a ‘message’ (such as x=) to each of the values:
print(“x=”,x,” y=”,y,” z=”,z)
There are some spaces in front of y= and z= inside the quotes.
Spaces are ignored by the interpreter but if they are inside quotes they will be printed as written.
Be very careful when entering this statement: the positions of the commas and the quotes is crucial. If any symbol is in the wrong position, you will see a ‘Syntax Error’ message when you press ctrl+R. You will have to find and correct the error, which is usually near or right above the cursor.
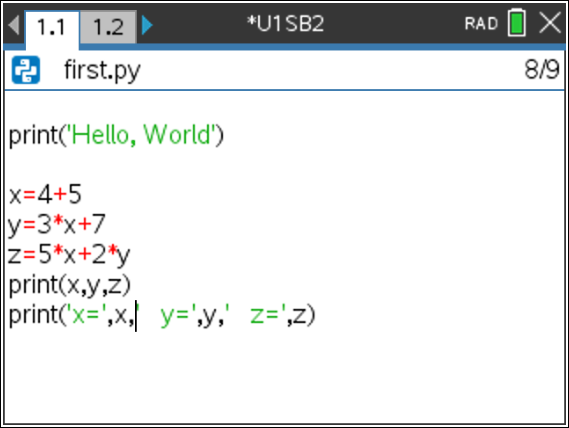
Step 8
-
When you run this program, you should now see:
x= 9 y= 34 z= -23
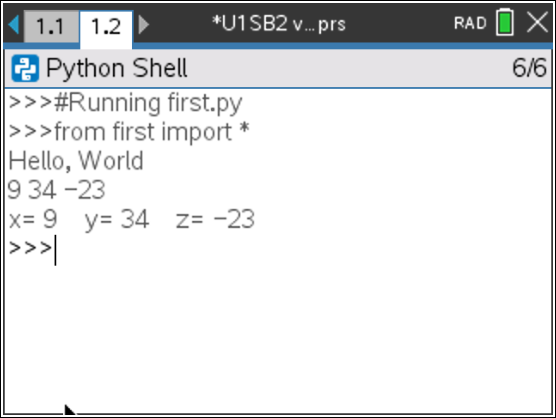
Skill Builder 3: Introducing the Python Function
Download Teacher/Student DocsIn this lesson, you will define a function and use the function to evaluate expressions. You will experience the purpose of indentation in Python and the assistance of inline prompts in the Editor.
Objectives:
- Define a function
- Use the function in evaluating expressions
- Use the input() function
Step 1
Functions play a big role in Python programming as in mathematics. Functions can be used to generate many different kinds of values and functions can serve as Python subroutines which help to break a complex process into smaller, manageable parts.
A program is a step-by-step recipe for solving a problem: an algorithm. All programs are algorithms underneath.
Step 2
-
Write a program that lets the user enter a number for x and the program will use that value to evaluate the function
f(x)=x2 + 3x – 1.First, define a function using menu > Built-ins > Function > def function(). The function template appears and the inline prompts function, argument, and block are provided and must be replaced with your own code.
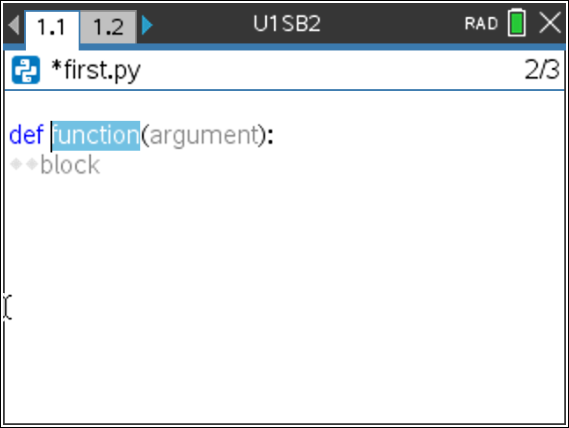
Step 3
-
Notice that function is currently selected. Replace function with f by typing the letter f, press tab to replace argument with x and tab again to change block to:
return x**2 + 3*x - 1
return is found on menu > Built-ins > Function.
x**2 is Python notation for x2, or x*x
and you must write 3*x, not 3x.Notice that block is indented two spaces. The light gray diamonds are ‘placeholders’ for you to keep track of the amount of indentation in more complex programs. Indenting is very important in Python.
We have finished defining the function. When you run this program, this function definition is not executed right away but the Shell ‘knows’ that it exists.
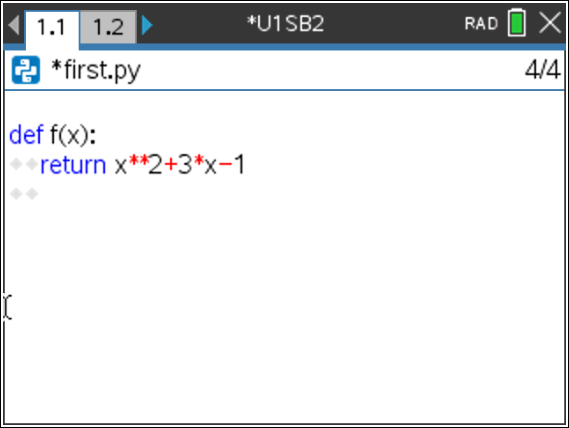
Step 4
-
Use an input() statement to allow the user to enter a number for x.
Backspace (press the del key) to the beginning of a new, blank line and write:
x=input(“Enter a value for x: “)
Use the = key on the keypad.
For input() press menu > Built-ins > I/O (or just type it in)
You can use either single (punctuation key) or double (ctrl+x (multiplication symbol)) quotes.
The green text in quotes is entered character by character from the keypad.
The colon (:) character is on the punctuation key. It is not required but makes the prompt look nice.
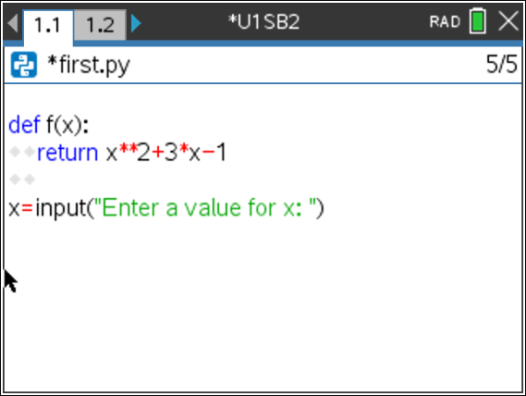
Step 5
-
On the next line write the print() statement:
print(“f(x) equals “ , f(x))
Recall that print() is on menu > Built-ins > I/O.
The f(x) in quotes will be printed as f(x) but the f(x) after the comma is a function call and will be replaced with the value that is returned by the function.
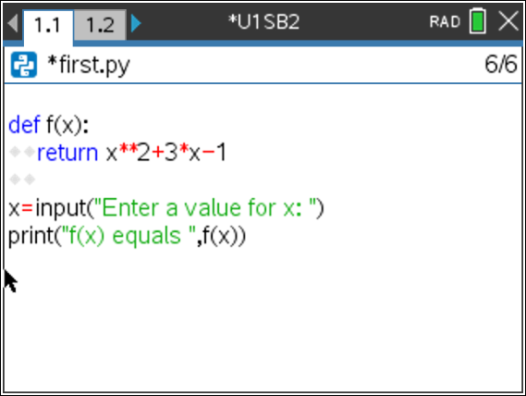
Step 6
-
Press ctrl+R to run the program. At the prompt, enter a number for x and press enter.
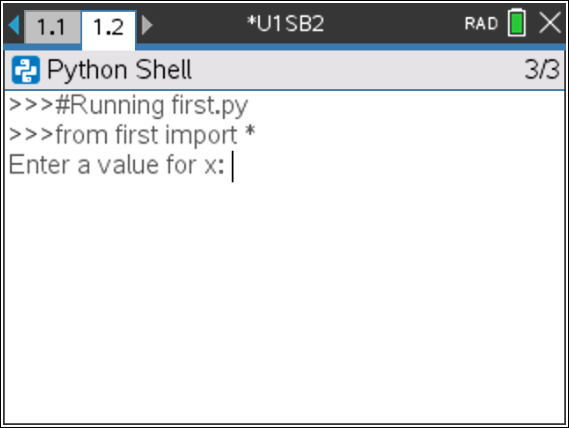
Step 7
-
Welcome to (possibly) your first ‘runtime’ error. The message is lengthy but the important part is in the last two lines. Note the last line number and the TypeError: “can’t convert…”
The error happens because the input() function returns a string, not a numeric value. The programmer (that’s you) must convert the string to a numeric value.
There are five simple types of data in Python: int (integer), str (string), float (numbers with decimals), complex (complex numbers) and bool (Booleans are either True or False).
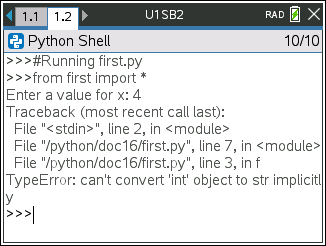
Step 8
-
Fixing the error: There are several convert functions built into Python. They are found on menu > Built-ins > Type.
They are int(), float(), str() and complex().
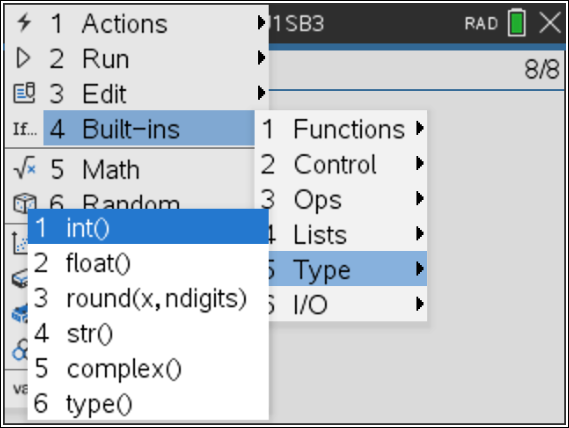
Step 9
-
To convert the string x to a number, use
x=float(x)
or
x=int(x)
just before the print statement. Try both and see the difference.
We chose float() in this example. Why?
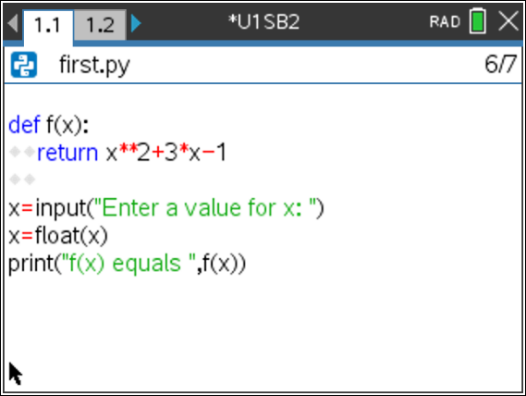
Step 10
-
Run the program again and it will work as intended.
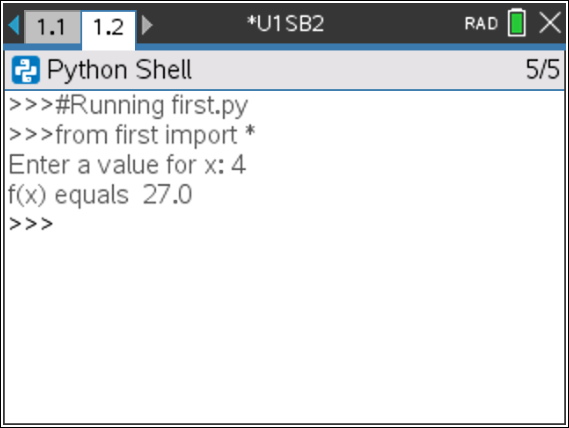
Application: Two Functions Are Better Than One
Download Teacher/Student DocsIn this application, you will make a second function and use both functions to investigate some of the mathematical properties of functions.
Objectives:
- Editing a Python file
- Copying a Python file
- Adding a function
- Evaluating function expressions in the Shell
- Creating an inverse function.
Intro 2
In the last lesson (Unit 1, Skill Builder 3) you defined a function f(x). In this application you will add another function to that file and then evaluate some expressions using those functions.
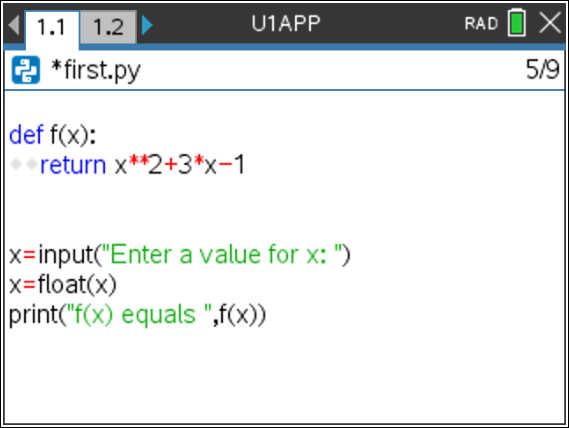
Step 1
Begin with the TI-Nspire document with the Python program you used in Skill Builder 3. See the image.
Save the TI-Nspire document using a different name by pressing doc > File > Save As… and use a different name. The title bar in the image shows the new name U1APP.
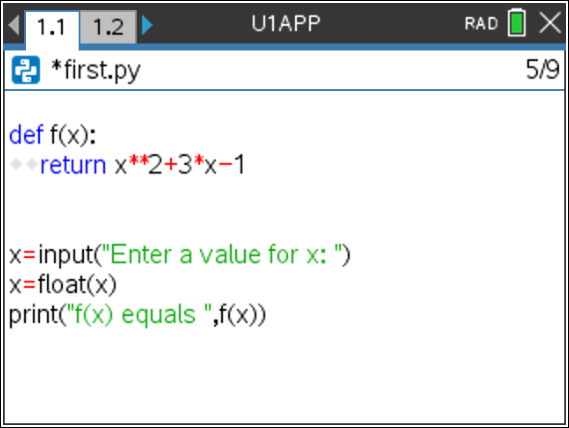
Step 2
Make a copy of the Python program by pressing menu > Actions > Create Copy… in the Python Editor.
Give the copy another name (the default adds a 1 to the end of the name).
(if Create Copy… is unavailable, press ctrl+B in the program to store it. There should be no asterisk in front of the Python filename on the top of the Editor).
This creates another Python Editor app containing the duplicate code in the document.
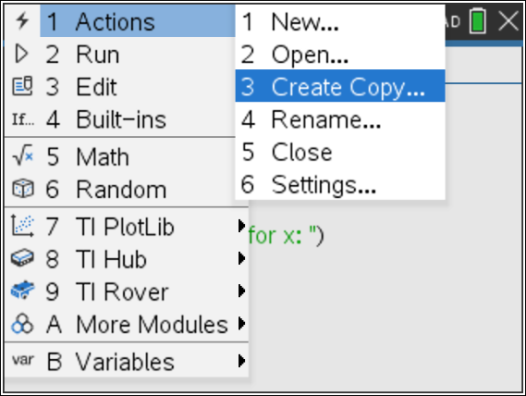
Step 3
Our new Python filename is second.py.
Now add a second function template below the function f(x):
On a blank line press menu > Built-ins > Functions and select def function().
Again, the syntax for the function structure includes a colon (:) at the end of the def line. This indicates that the following code is the definition of the function and the block is indented.
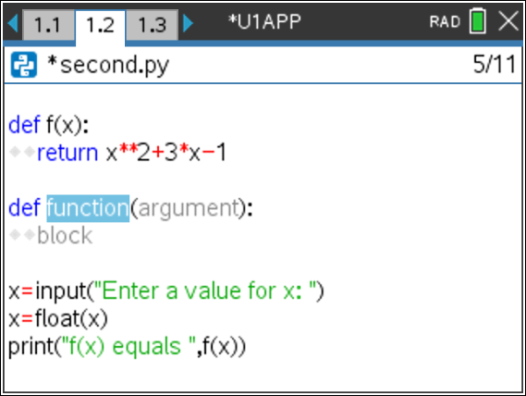
Step 5
Change f(x) by removing x**2+ from the function so that
f(x) = 3 * x - 1.
Define g(x):
def g(x):
return -2*x - 4
Delete the 3 lines of code at the bottom of the program leaving only the two functions.
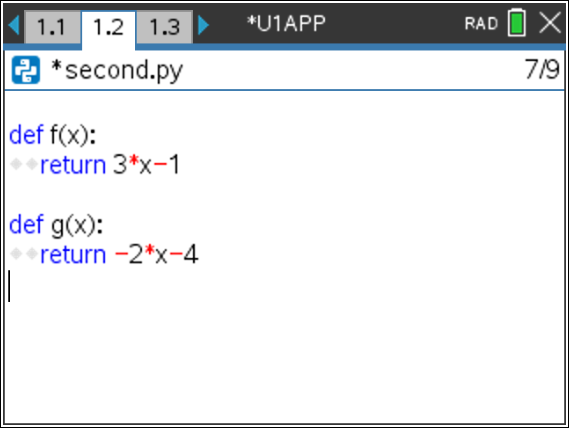
Step 6
Press ctrl+R and enter the expression f(1)+g(1).
Try other expressions using both functions like:
f(4)+g(1), f(5)+g(2), (f+g)(4), f(g(6))
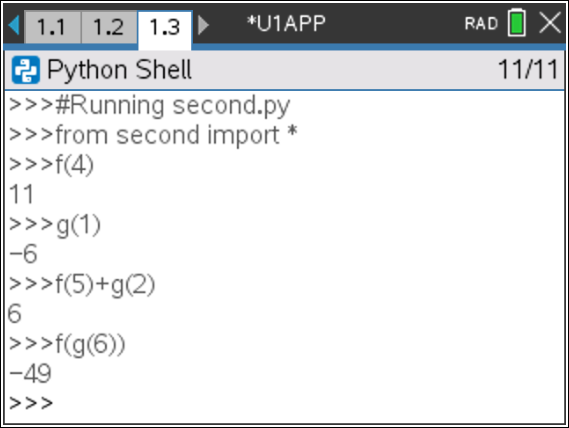
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 2: Input, Output and Functions
Skill Builder 1: A Tale of Two Means
Download Teacher/Student DocsIn this lesson, you will write a program that requires a mathematical function that is not part of Python’s Built-ins.
Objectives:
- Use import for additional functions
- Write a program using the menus
- Use multiple units in one problem
Step 1
The arithmetic mean (average, pronounced ‘arithMETIC’) | |
of two numbers is: | ![]() |
The geometric mean is: | ![]() |
Write a program to calculate and display both means to compare them for several examples. Begin a new Python program and name it TwoMeans.
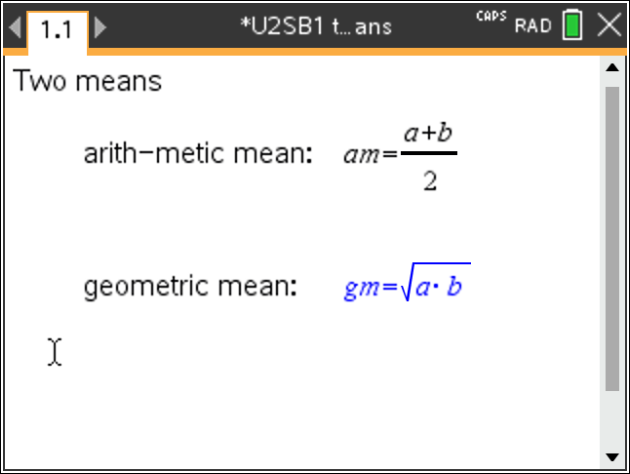
Step 2
- Start a line with the # sign (‘pound’, ‘number’, or ‘hashtag’) found on the punctuation key. This is used for making a comment which is ignored when the program runs. After the # sign write a sentence explaining the purpose of the program. You can also type ctrl+T to turn any line into a comment. Comments are useful in two ways:
- They allow the programmer to document the purpose for different chunks of code. This makes longer programs easier to read and debug if there are errors.
- They are useful in debugging because you can ‘comment out’ a line so it does not execute when the code runs. That allows the programmer to systematically isolate where the error occurs.
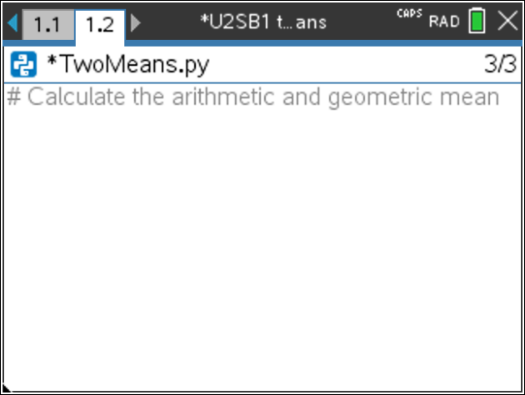
Step 3
- This program requires the square root function which is not part of Python’s built-in operations. Square root (sqrt) and other mathematics functions are found in the standard Python module called math. To use this function, you must ‘import’ the math module to your code. On menu > math, select the statement at the top:
from math import *
The * means import ‘everything’.
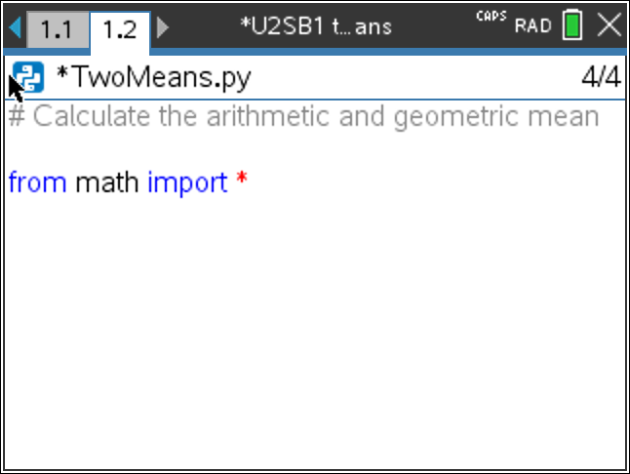
Step 4
-
Next we use the input statement to enter the first number. First type the variable a and the = sign.
Recall that input returns a string and we must convert it to a number. We’ll combine those two steps into one by writing:
a = float(input( ))
First get float() from menu > Built-ins > Type,
then look on menu > Built-ins > I/O for the input() function.
For the prompt inside the parentheses, write ‘First number?‘
Write a second statement to enter the second number (not shown).
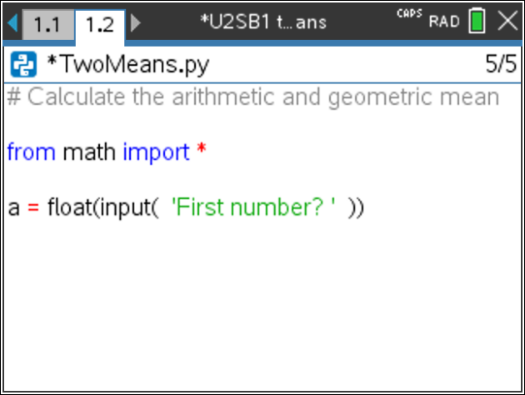
Step 5
-
Write two assignment statements, one for the arithmetic mean and one for the geometric mean:
am = (a + b) / 2
gm = sqrt(a * b)sqrt() is on menu > math.
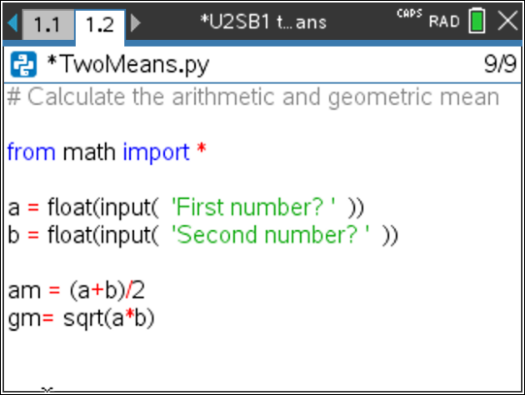
Step 6
-
The last task is to write the print() statements to display the two calculated values. Use your imagination! A sample is shown here:
print( "am = ", am)
print( "gm = ", gm)
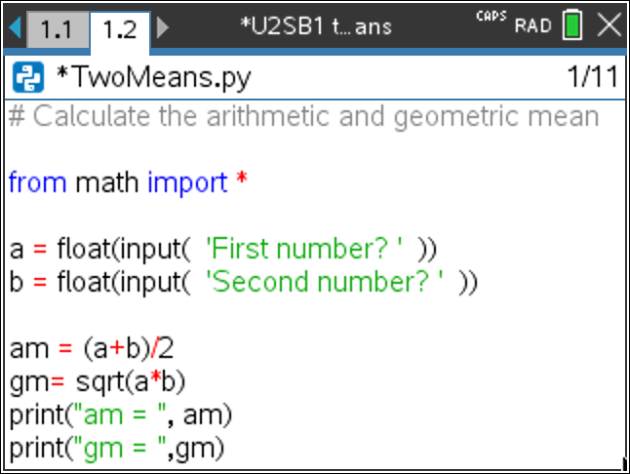
Step 7
-
Run the program by pressing ctrl+R and enter two numbers for which you know the answers…TEST, TEST, TEST.
After trying many examples, do you notice a relationship between the two means? Is one always larger than the other? Are they ever equal? How are they related to the two numbers you enter? Are there any values which cause an error?
Check your guesses with the teacher! Can you prove it?
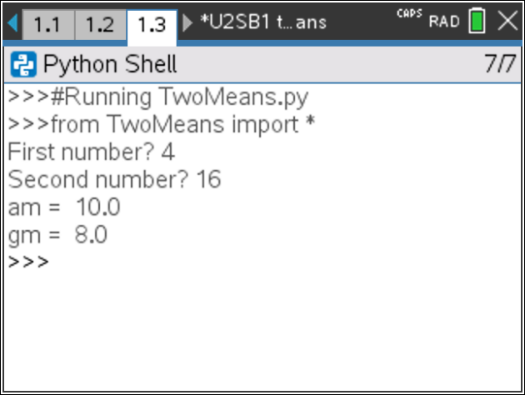
Step 7
-
Run the program by pressing ctrl+R and enter two numbers for which you know the answers…TEST, TEST, TEST.
After trying many examples, do you notice a relationship between the two means? Is one always larger than the other? Are they ever equal? How are they related to the two numbers you enter? Are there any values which cause an error?
Check your guesses with the teacher! Can you prove it?
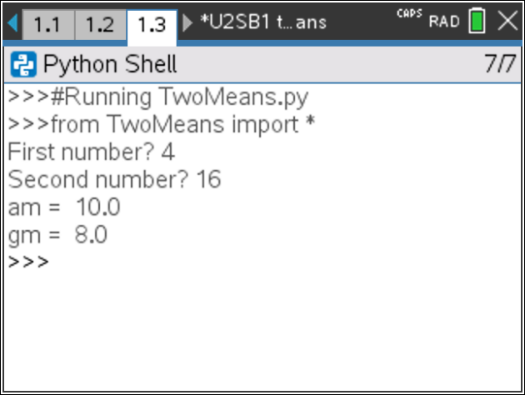
Step 7
-
Run the program by pressing ctrl+R and enter two numbers for which you know the answers…TEST, TEST, TEST.
After trying many examples, do you notice a relationship between the two means? Is one always larger than the other? Are they ever equal? How are they related to the two numbers you enter? Are there any values which cause an error?
Check your guesses with the teacher! Can you prove it?
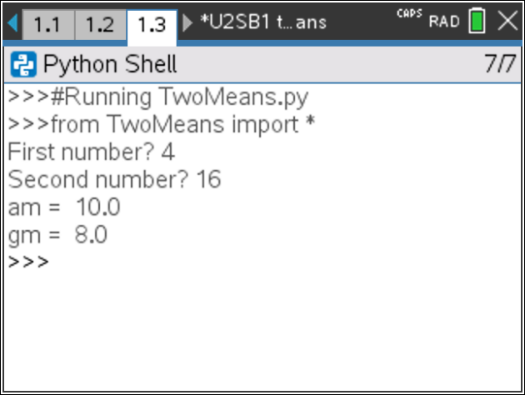
Step 7
-
Run the program by pressing ctrl+R and enter two numbers for which you know the answers…TEST, TEST, TEST.
After trying many examples, do you notice a relationship between the two means? Is one always larger than the other? Are they ever equal? How are they related to the two numbers you enter? Are there any values which cause an error?
Check your guesses with the teacher! Can you prove it?
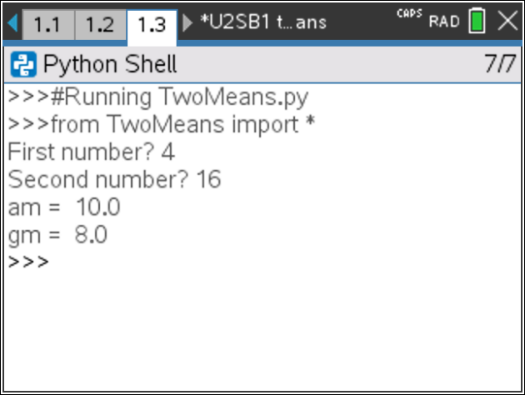
Skill Builder 2: Heron’s Formula
Download Teacher/Student DocsIn this lesson, you will use one of the program templates, create a function to evaluate and return a value, and explore the mathematical operators.
Objectives:
- Use a program template (Type:)
- Create a function for Heron’s Formula
Step 1
You are given a triangle with only the side measurements. Can you determine the area? YES, using Heron’s Formula.
In this lesson you will create a function to determine the area of a triangle using the three side lengths and then complete the program that uses that function.
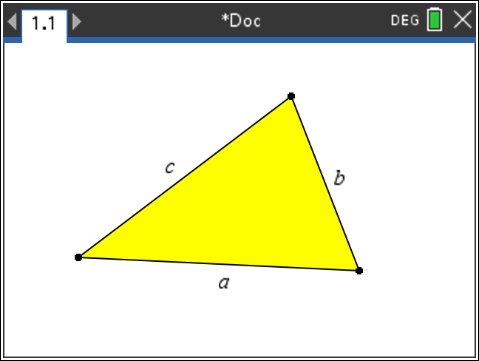
Step 2
-
This program, like the last one, requires the sqrt() function. This time we will use one of the included Python ‘templates’ which pre-loads the most commonly needed functions for the project.
When you select Add Python > New and are entering the name of the Python file (we use ‘area’ for this name) there is a field under the name labeled ‘Type:’. The default type is ‘Blank Program’. Clicking on the pop-up arrow on the right exposes the other types of programming projects available (there are many!).
For this project select the Type: Math Calculations and click OK or press enter.
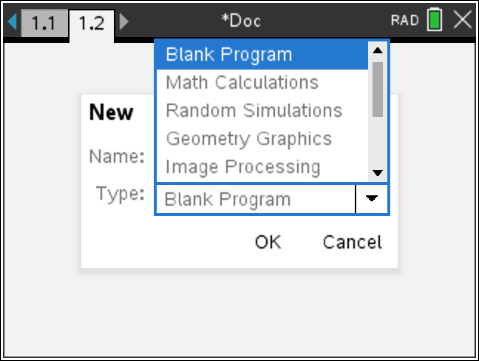
Step 3
The Math Calculations template provides the statement
from math import *
for you.
Next get the def function() statement from menu > Built-ins > Function.
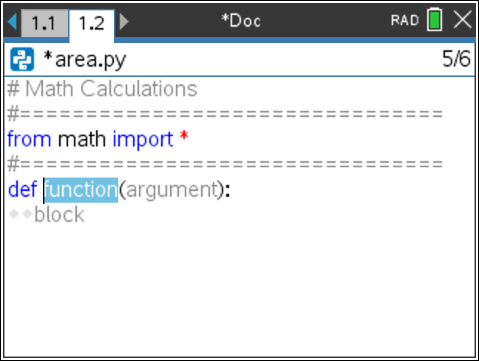
Step 4
- Make the name of the function heron. There are three arguments, a, b, and c, which represent the three sides of the triangle.
See the next step for the code for the block.
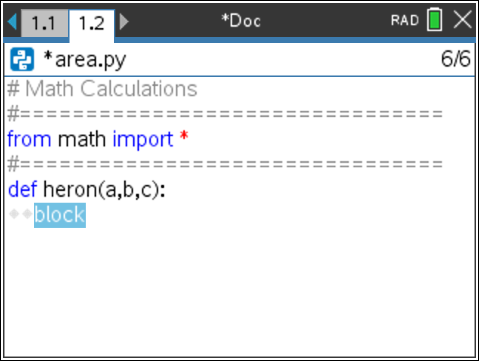
Step 5
Heron’s Formula is a two-step calculation:
First, calculate half the perimeter (the semi-perimeter):
s = (a + b + c) / 2
Then the area is:
area = sqrt(s*(s - a)*(s - b)*(s - c))
Remember that both statements in this function block are indented.
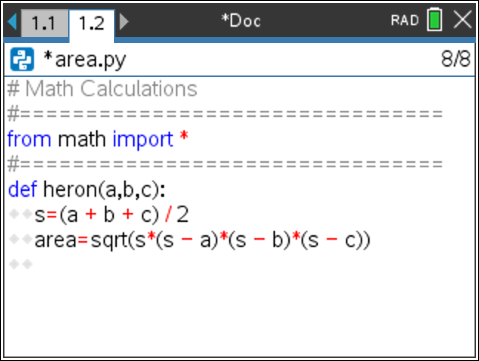
Step 6
Finish the function by providing the return statement
return area
found on menu > Built-ins > Function.
As in mathematics, functions have arguments and ‘produce’ a value. The return statement is needed to ‘send’ the value back to the main program where it can be used.
Important: Move the insertion cursor back to the beginning of a new line using del or shift+tab. You can also skip one or more lines for clarity.
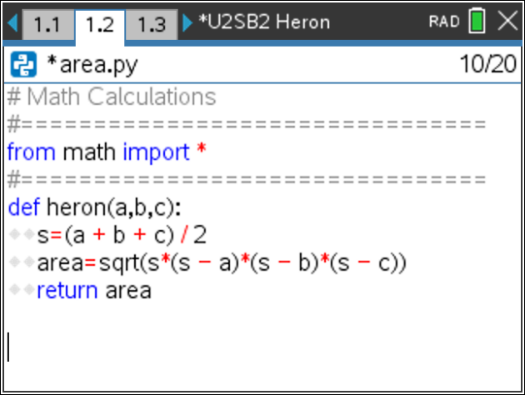
Step 7
-
Now it’s time to write the main program using just the statements:
input() (3 times for the three sides)
print() (to print the area)Before going to the next step, try writing them yourself.
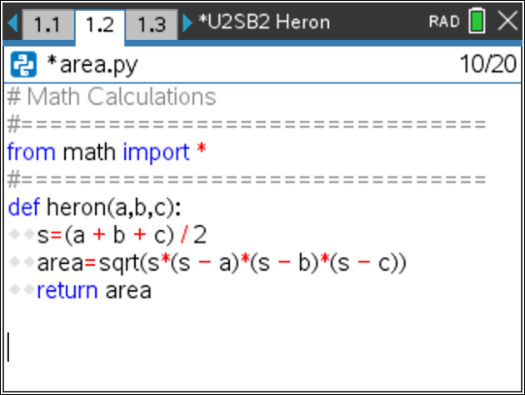
Step 8
-
The three input() statements request the lengths of the three sides, convert each length to a number, and store the values in three variables. We use x, y, and z.
x = float(input(“Enter first side: “))
The other two are similar.
The print() statement prints the value of the heron function with the three variables as arguments:
print(“Area = “ , heron(x,y,z))
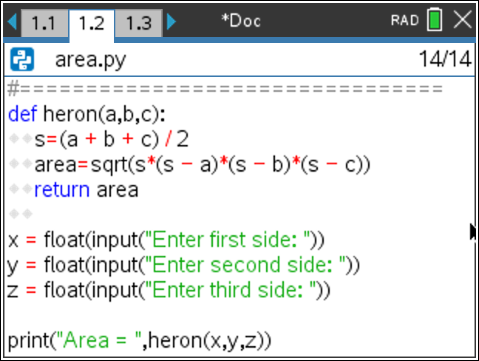
Step 9
-
Test your program with numbers for which you know the area, like 3, 4, and 5. Why is the area 6? What other triangles have areas that are easy to compute when given the three sides?
Remember to save your work.
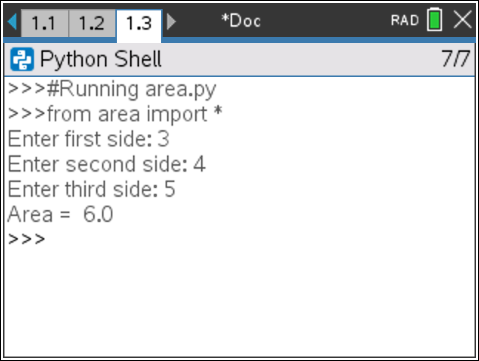
Skill Builder 3: The Midpoint Formula
Download Teacher/Student DocsIn this lesson, you will write and use a function that is used more than once.
Objectives:
- Review the midpoint formula
- Use uppercase in a variable
- Store the result of a function in a variable
- Return two values from a function
Step 1
In coordinate geometry there is a method for determining the coordinates of the midpoint of a line segment given the coordinates of the endpoints. Write a program that determines the midpoint’s coordinates.
There are two midpoint formulas that are really the same formula (average of two numbers) using different variables:
midX = (x1 + x2) / 2
midY = (y1 + y2) / 2
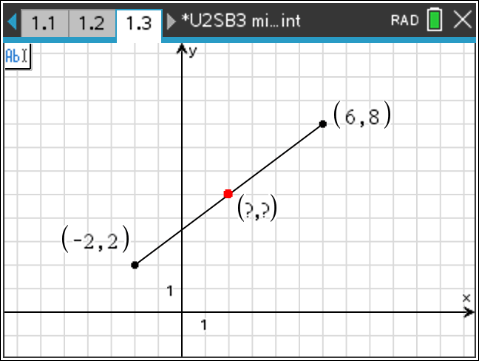
Step 2
-
Start a new Python file as a ‘Blank Program’ (we named it midpoint). Insert the def function() template from menu > Built-ins > Function.
Name the function midpt.
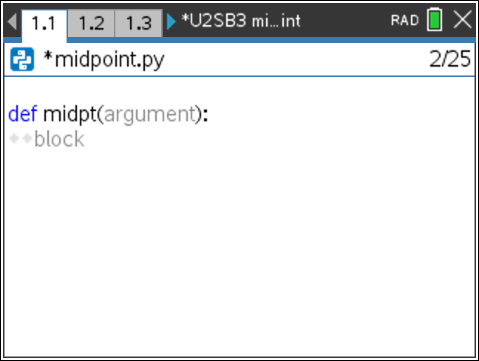
Step 3
-
This time write the main program first; then come back to code the midpt function last. Place your cursor at the beginning of a blank line below the function name. Write the four input statements to enter the four coordinates of the endpoints using x1, y1, x2, y2 as the four variables. Try it before moving to the next step.
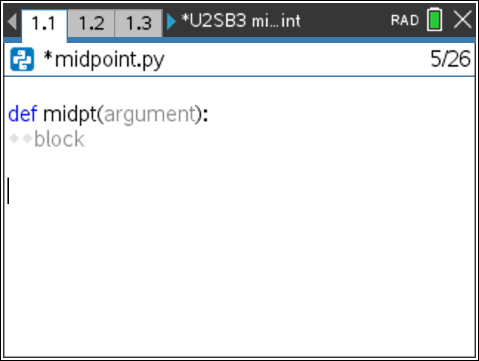
Step 4
-
The image on the right shows all four input statements. The first is:
x1 = float(input(“x1 = “))
Copy and paste comes in handy here! Select text using shift+arrowkeys, and use ctrl+C to copy and ctrl+V to paste just like on a computer. Be sure to edit the pasted code to suit.
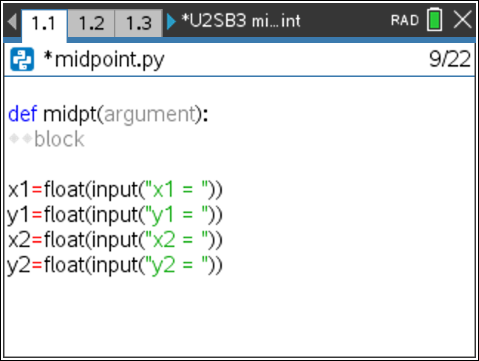
Step 5
-
Write TWO assignment statements to store the result of the midpt function into TWO DIFFERENT variables:
midX = midpt(x1, x2)
midY = midpt(y1, y2)(We are using the same function for two different sets of variables.)
Write the print statement to display the complete midpoint:
print(‘midpoint = ‘, )
Can you complete this statement so that the output appears as an ordered pair? For example: midpoint = (13, 5)
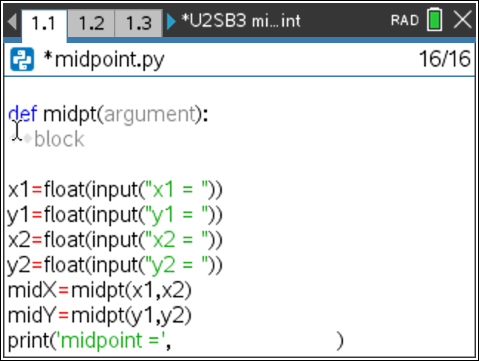
Step 6
-
Now go back to complete the midpt() function.
Use just two arguments. It’s better to use two new variables as arguments:
midpt(a,b)
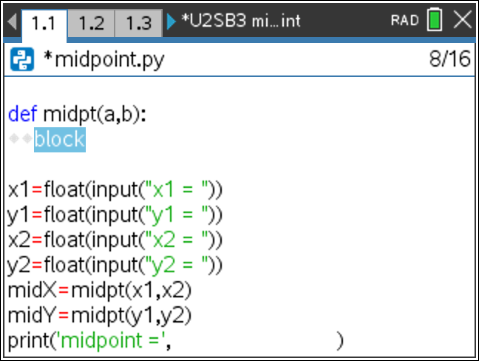
Step 7
-
The body of the function consists of one calculation:
mid = (a + b) / 2
and the return statement:
return mid
Make sure these two statements are indented the same amount.
return is found on menu > Built-ins > Function.
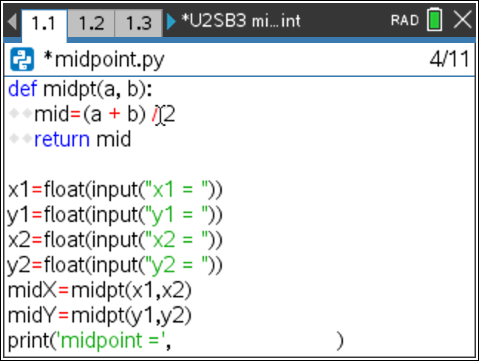
Step 8
-
Run the program and enter four values. Does your output resemble this?
Summary: Python functions can be used many times during a program.
Displaying output in a pleasing format can be tricky. Our print statement is:
print(‘midpoint = ( ‘, midX, ’, ‘, midY, ’)’ )
with extra spacing to see where the quotes and commas belong.
Remember to save your project.
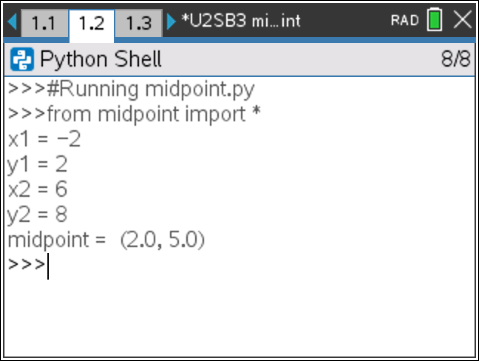
Application: Distance to the Horizon
Download Teacher/Student DocsIn this Application, you will write a program that that determines the distance to the horizon from a given altitude.
Objectives:
- Use import for additional functions
- Write a program using the menus
- Use multiple units in one problem
Step 1
When a plane is flying, how far away is the horizon to the pilot? The higher she flies, the further the distance to the horizon. How does she determine the distance to the horizon given the plane’s altitude?
The radius of the earth is 3,958.8 miles.
Write a program to input the altitude of the plane in feet and produce the distance to the horizon in miles.
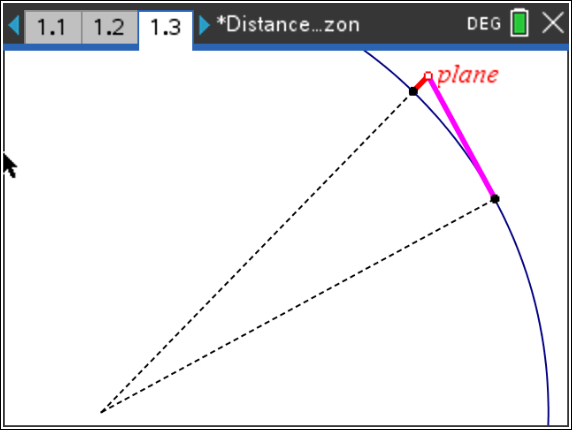
Step 2
-
Start a new Python file (we call it horizon).
Start with a comment explaining the purpose of the program.
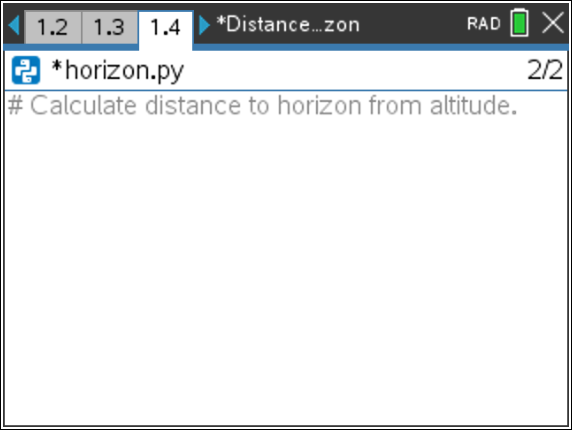
Step 3
-
This program uses the Pythagorean Theorem and requires the square root function which is not part of Python’s built-in operations. This function and others are found in a standard Python module called math. To use this function, you must import the math module to your code. On menu > math, select the statement at the top:
from math import *
The asterisk (*) means ‘everything’. You will see how to use the tools in this module using the menus.
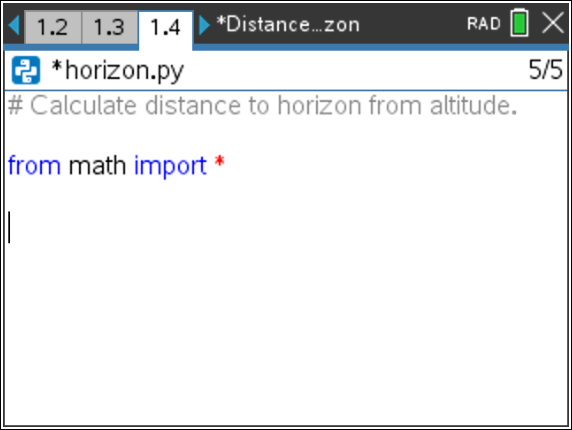
Step 4
-
Use the input statement to enter the altitude of the plane.
First type the variable alt and the = sign.
Then look on menu > Built-ins > I/O for the input() function.
For the prompt inside the parentheses, write: “Altitude of plane? “
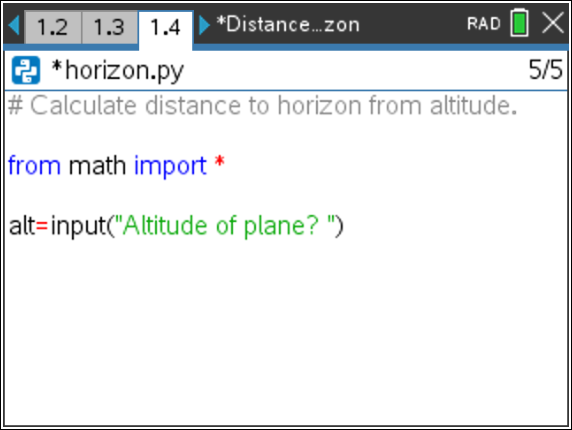
Step 5
-
From our labeled diagram we see a right triangle where one leg is the radius of the earth, the other leg is the distance from the plane to the horizon and the hypotenuse is (the radius of earth plus the altitude of the plane). The Pythagorean Theorem states that, in a right triangle
(leg1)2 + (leg2)2 = hyp2 or radius2 + leg22 = (radius+alt)2
Solving for leg2 gives: leg2 = sqrt((radius+alt)2 – radius2)
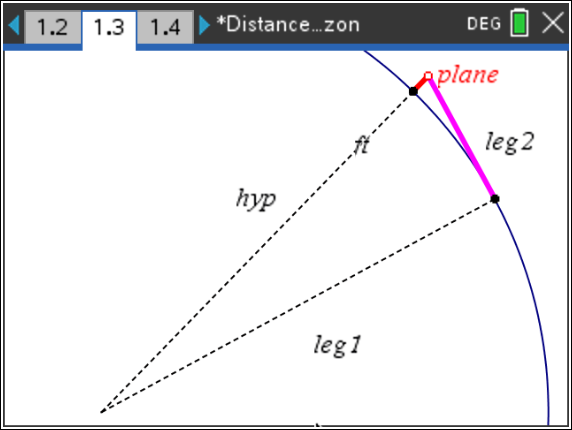
Step 6
-
We enter this formula into our program:
leg2 = sqrt((radius + alt)**2 – radius**2)
Be careful with the parentheses and be sure to use **2 for squaring. We also use the variable radius to represent the radius of the earth and set it equal to 3958.8:
radius = 3958.8
There are two more details to handle before we can test the program.
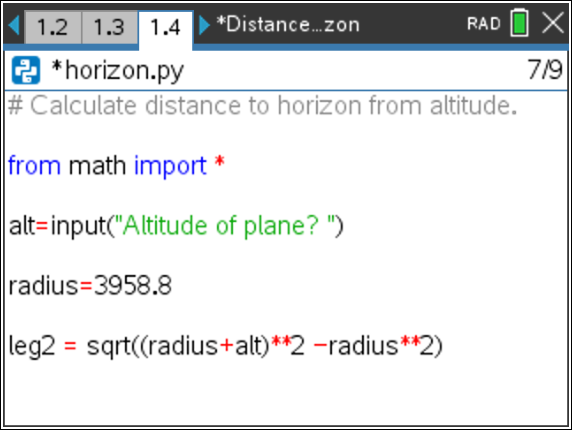
Step 7
-
Recall that input() produces a string. We need a number, so add the float function to the input statement:
alt = float(input(“Altitude of plane? “))
Also, the altitude is in feet but the radius is in miles. Convert the altitude into miles using the statement
alt = alt / 5280
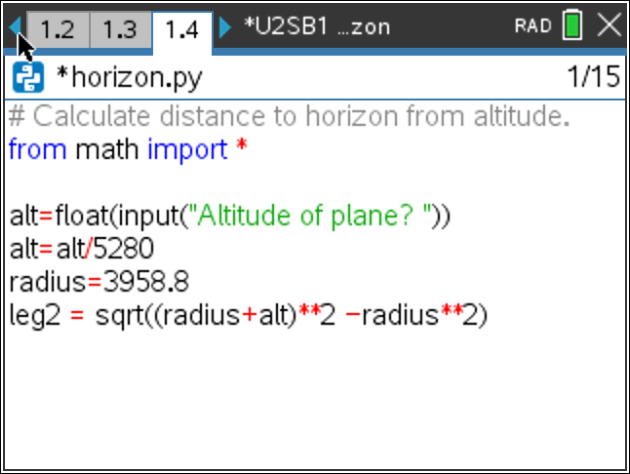
Step 8
-
We’re just about ready but we still have to print the answer!
print(leg2)
gives the answer, but a more informative message would be:
print(‘Distance to horizon: ‘, leg2’, miles.’)When ready, press ctrl+R to run the program. Try various altitudes.
Remember to save your document.
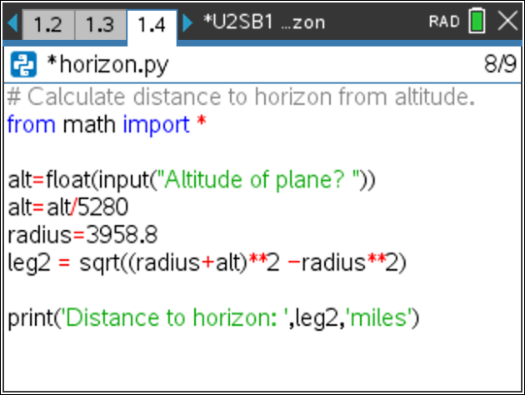
Step 9
-
Summary: The order of the statements in the final program is important but the order in which they are entered into the program does not matter. This is the power of a text editor. This program and all the others in this unit are examples of the ‘sequence’ structure of the language, which processes statements from top to bottom.
Comments, extra spaces, and blank lines in the program are ignored when you run the program. ‘import’ statements bring in additional functions from separate modules that are included in the TI-Nspire Python programming language.
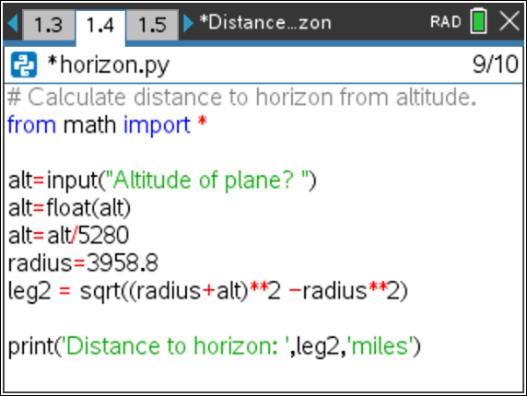
Step 10
-
Question 1: The International Space Station is 254 miles above the surface of the earth. How far is it to the horizon for the crew?
Question 2: If you are standing on the beach at the edge of the water looking out over the ocean, how far away is the horizon?
Hint: Enter the height of your eyes above the ground (in feet).
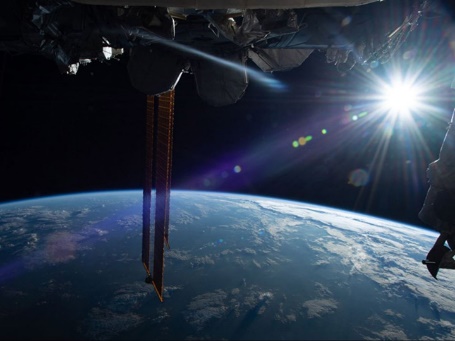
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 3: Conditions, if and while
Skill Builder 1: Collatz
Download Teacher/Student DocsIn this lesson, you will ‘branch’ out into the world of conditional programming. You will also investigate an as yet unproven conjecture.
Objectives:
- Learn the relational and logical operators
- Learn the integer operators (% and //)
- Write programs using if statements and while loops
Step 1
Choices, choices, choices. Our life is one long series of decisions: Are you hungry? What to eat? Is it cold? What to wear? Are we there yet? This decision-making process in programming is handled with if statements and while loops, which both depend on conditions. Examples of if and while in action are seen in in this image.
A condition is an expression that results in the value True or False:
X > Y A+B <= C Qty > 0 5 != 3
are all examples of conditions. (does not equal)
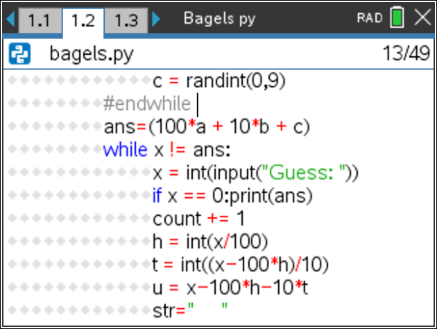
Step 2
A condition includes one or more of these relational operators:
== > < != >= <=
Compound conditions are made using the logical operators:
and or not
Conditions are used in if structures and while loops.
Caution: Remember to use == when writing a condition, not =. Using the wrong symbol will result in a syntax error. if x==5:, not if x=5:. Using the ctrl+= menu can help.
This lesson introduces you to these powerful programming tools.
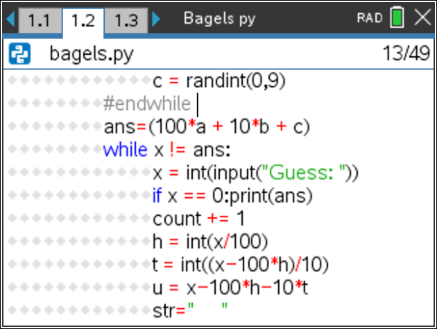
Step 3
-
The Collatz Conjecture
Algorithm: Take a positive integer: if it is even, divide it by 2,
otherwise multiply it by 3 and add 1.
Repeat with the result.
What happens to the sequence?
Begin with a blank Python file (we called it Collatz).
Input an integer to the variable num using the statement:
num = int( input( "Enter a positive integer: "))
int( is found on menu > Built-ins > Type.
input( is found on menu > Built-ins > I/O.
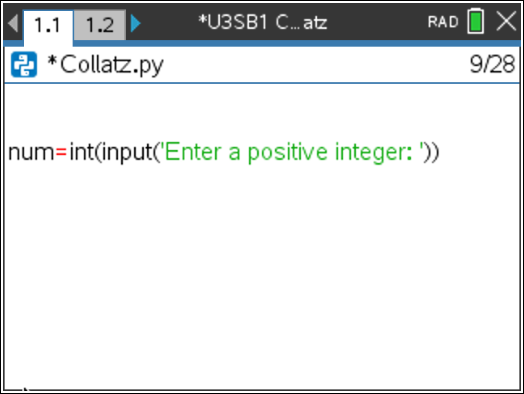
Step 4
-
if statements come in three flavors: if.., if..else.., and if..elif..else... They are all found on menu > Built-ins > Control. Note that there is no ‘then’ in Python.
(They are on the ‘Control’ menu because these statements control the flow of your program.)If.. Use when there is no ‘otherwise’ action. if..else.. Use when there are exactly two alternative actions for True and False.
(We will use this one soon.)if..elif..else.. Use these when there are three or more actions to be taken based on several conditions.
elif is short for ‘else if…’ and requires a condition like if.
You can add as many elifs as your algorithm requires.
(This one is used in the Application for this unit.)
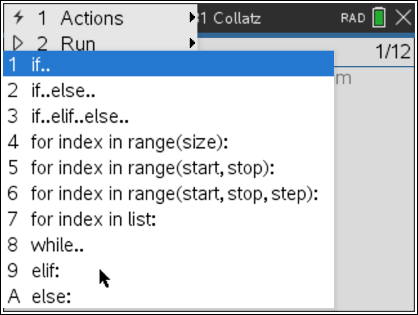
Step 5
-
Insert the if..else statement from menu > Built-ins > Control.
Press tab or right-arrow to move from prompt to prompt.
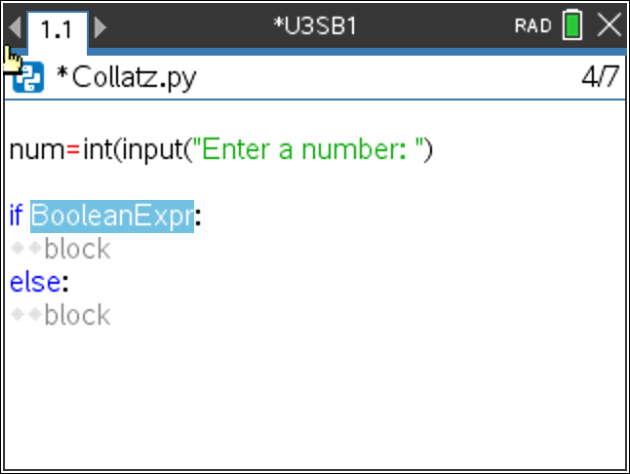
Step 6
-
The condition (BooleanExpr) is…
if num % 2 == 0:
% is called ‘mod’ and is the mathematical operator (like +, - *, and /) that gives the remainder when the first number is divided by the second. ‘mod’ is short for ‘modulus’.
% is found on the punctuation key (next to the letter ‘G’).
If the remainder when num is divided by 2 is zero, then the num is even.
Note the two equal signs!
For == just press the = key twice. All the relational operators are on ctrl+=.
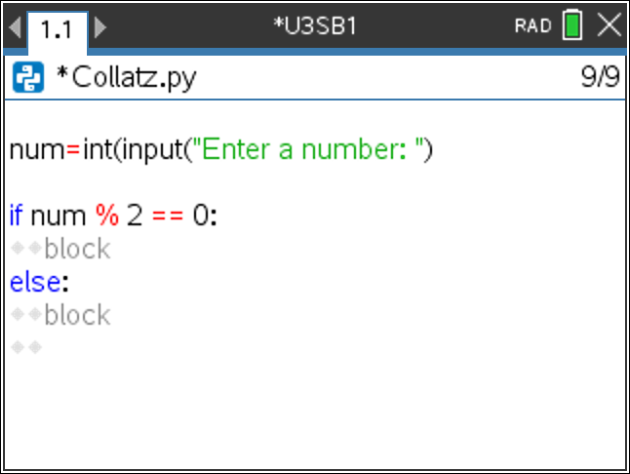
Step 7
-
The if : (when the number is even)
block (the top one)
becomes:
num = num // 2
// (two division signs) is integer division (no decimal and truncates to just the whole number). If you use / you will see a decimal point even if the number is an integer. Just use two / signs (the ÷ key).
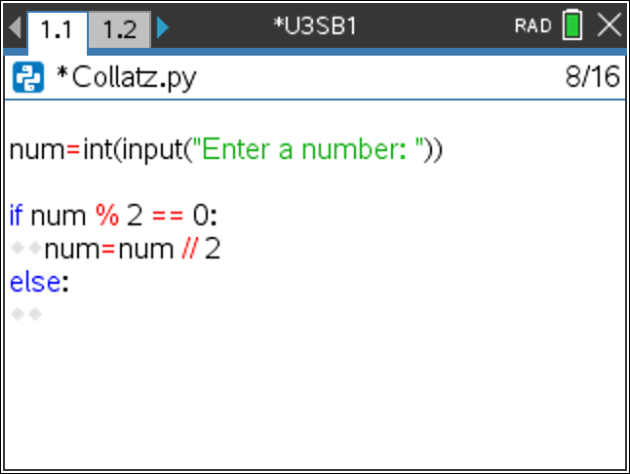
Step 8
-
The else: (when the number is odd)
block is:
num = 3 * num + 1
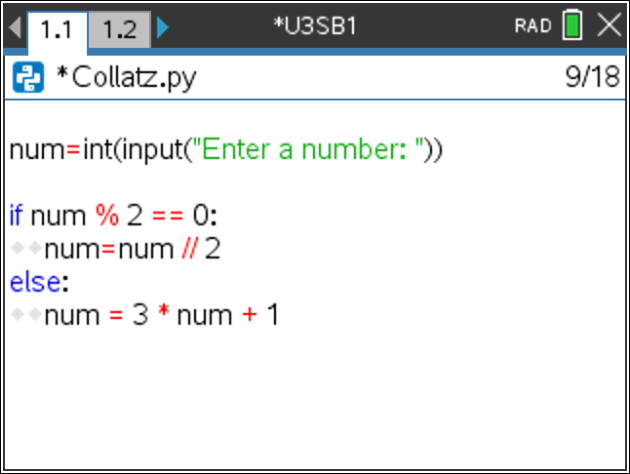
Step 9
-
After the if..else: statement block, backspace to the beginning of a line (erase the indent characters) and write the print statement:
print(num)
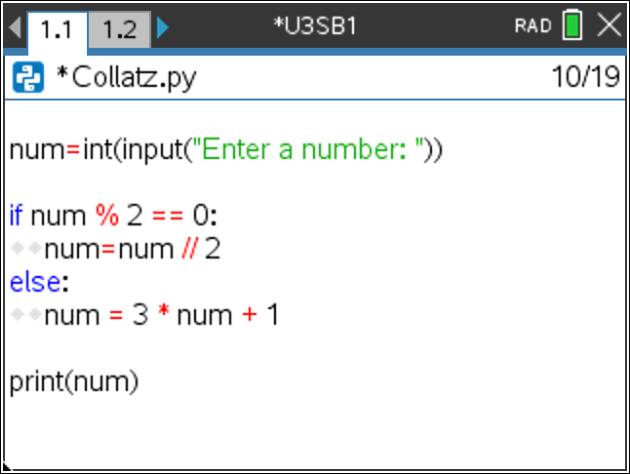
Step 10
-
Running the program:
Press ctrl+R to run the program. Enter a positive integer. An answer appears.
Press ctrl+R again and this time enter the last answer.
Repeat running the program, each time entering the previous answer.
Next you will add a loop to the program so that the process runs repeatedly by itself instead of having to run the program over and over…
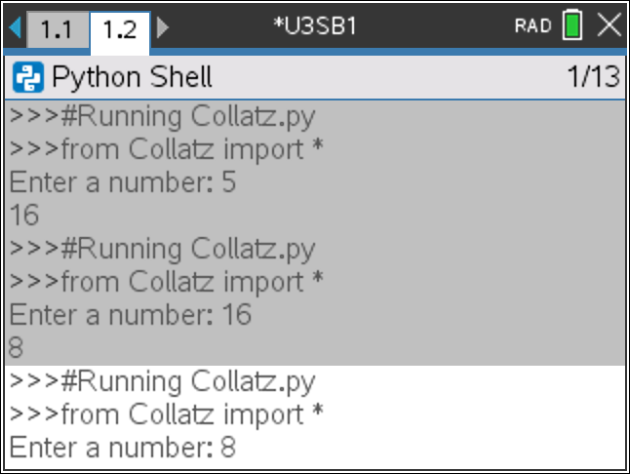
Step 11
-
Place your cursor right below the input statement and above the if statement as shown in this image. (Do not type: ‘<<< cursor here’)
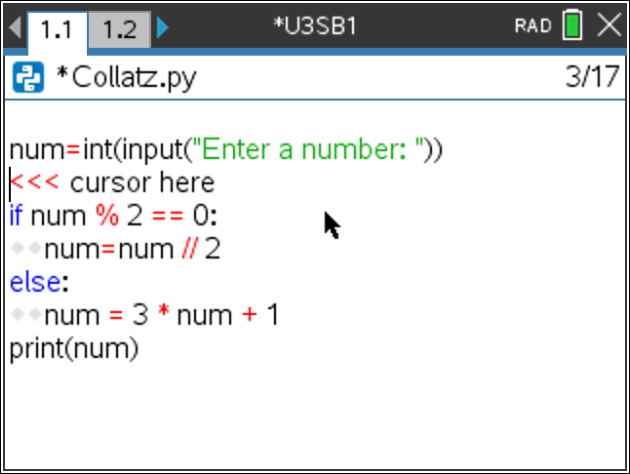
Step 12
-
On this blank line add the while statement found on
menu > Built-ins > Control.
You will see
while BooleanExpr:
blockpasted into your program.
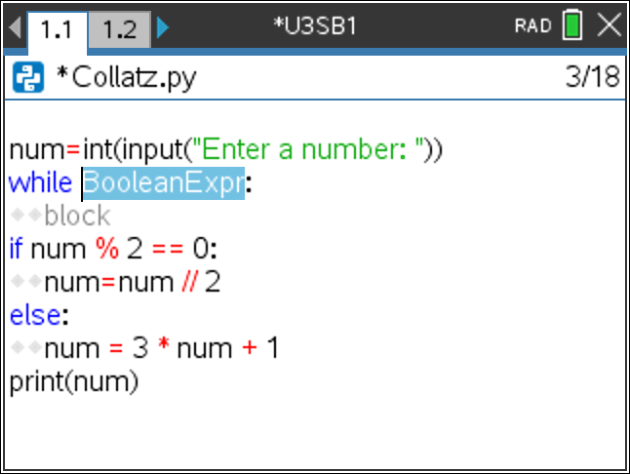
Step 13
-
Erase the entire line that says ‘block’.
Select the entire if structure and print statement using shift+down arrow. Press tab to indent all these lines two spaces to become the while block.
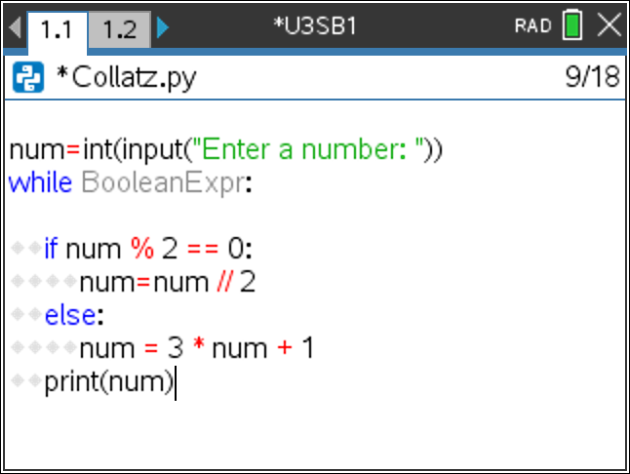
Step 14
-
Now write the condition by replacing BooleanExpression.
The Collatz Conjecture states that all sequences will eventually become 1.
As long as the number is greater than 1, continue processing; so write:
while num > 1 :
Be sure to leave the colon (:) at the end of this line.
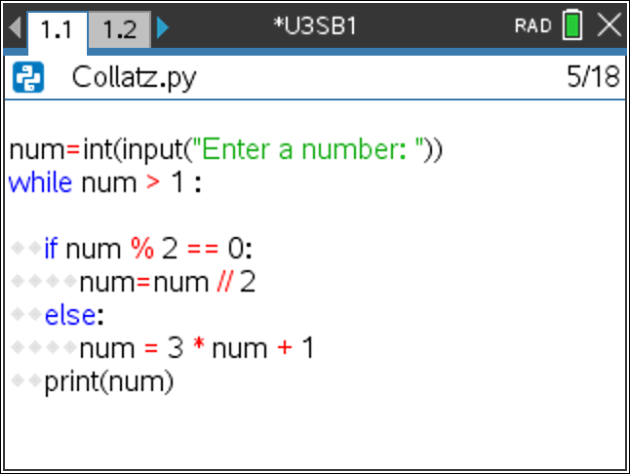
Step 15
-
Run the program. Enter 20 as the number. Follow the logic of the program: Odd numbers get larger and even numbers get smaller.
20 is even ➜ 10
10 is even ➜ 5
5 is odd ➜ 16
16 is even ➜ 8
and so on…
… and the program ends when the number reaches 1.Note that it only took one line of code to create a loop!
Can you find a number that causes the program to NEVER end? Try a large number. Notice how fast the numbers fly by! When the program ends you can scroll upward through the Shell history to examine the numbers.
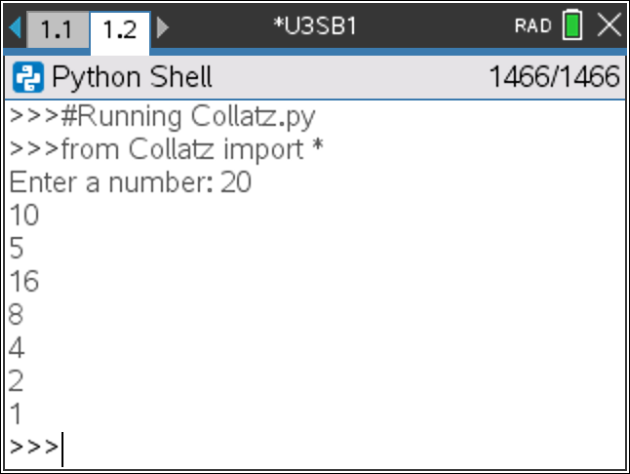
Skill Builder 2: Flag Waving
Download Teacher/Student DocsIn this lesson, you will learn about counters and accumulators by calculating the number and the total of numbers entered and determine the average of the numbers.
Objectives:
- Counter statement
- Accumulator statement
- While loop with a flag
- Calculating an average (mean)
Step 1
You will write a program that will count and add up a set of numbers. You do not know in advance how many numbers there will be. This lesson introduces the concept of a counter statement, an accumulator statement, a ‘flag’ value to end a loop, and the calculation of the average of the entered numbers.
Step 2
-
Begin a new Python file and name it count_ttl_avg.py.
Make two variables and set them both equal to zero:
count = 0
total = 0count keeps track of how many numbers are entered and
total keeps a running total of the numbers that have been entered.
A third variable, num, stores each number entered one at a time.
Rather than setting it equal to 0, use an input statement to get the first number from the user:
num=float(input(“Enter a number: “))
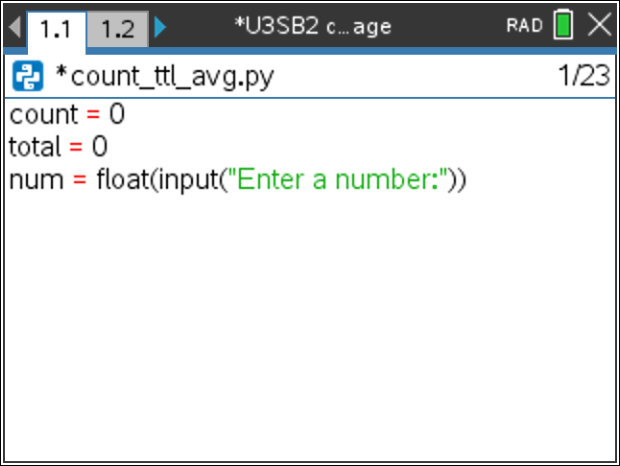
Step 3
-
Now start a while loop that will end when a certain number is entered. Some options here are 0, -999, or -1. Keep in mind that this unique number cannot be part of the set of numbers you are trying to process. We will use -999 as our ‘flag’ value to signal that we have finished entering numbers.
Select the while structure from menu > Built-ins > Control.
The ‘does not equal’ sign (!=) is on ctrl+=. We use it because we want the loop to continue as long as num does not equal -999.
Remember to leave the colon (:) at the end of the line!
Next we will fill in the while block…
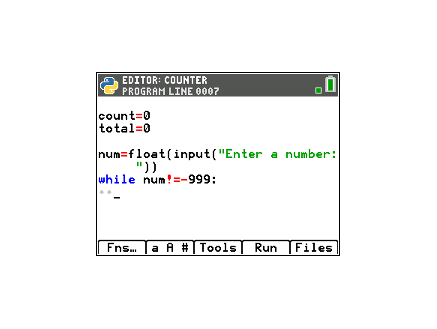
Step 4
-
The loop block will:
- Count the number of numbers entered.
- Add them up.
- Then, ask for another number.
Counting is done with the statementcount = count + 1
which adds 1 to the variable count each time it is processed.
Adding them up, or totaling, is done with the statement:
total = total + num
Asking for another number is an input statement like the first one
num=float(input(“Enter a number (-999 to end): “))
but here we add a message telling how to end the loop.
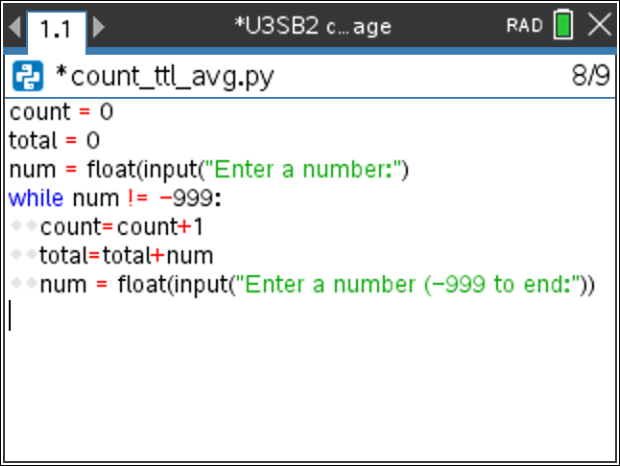
Step 5
-
We have finished with the loop. Skip a line or two and erase (backspace) the indent spaces.
Now is the time to process the inputted numbers. We kept track of the count and the total so we can calculate the average.
Try it yourself before looking at the next step…
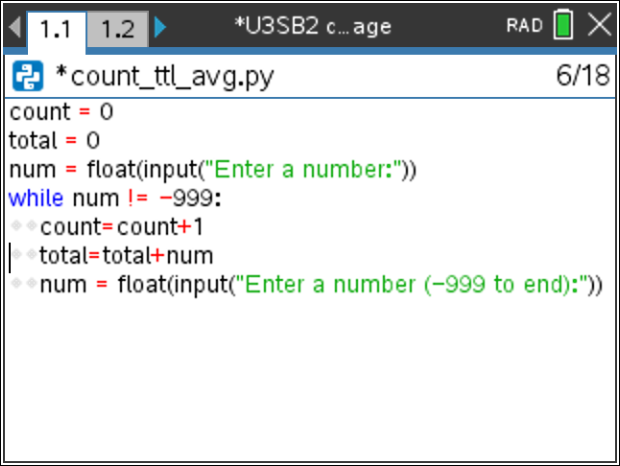
Step 6
-
The statement
avg = total / count
calculates the average and stores the result in the variable avg.
Ponder this: Why do we use / here and not // as in the last lesson?
Finally, write the print statement(s) that report the count, total and average to the user. You may wish to use more than one print statement. Our code is shown in the next step…
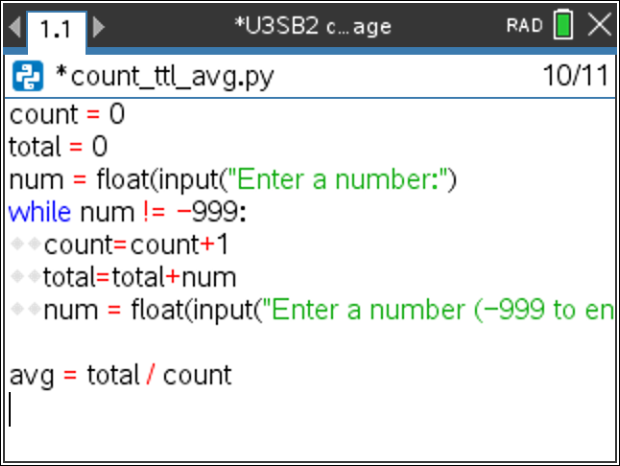
Step 7
-
We use three print statements to report the results:
print(" Count = ", count)
print(" Total = ",total)
print(" Average = ",avg)
A sample run of the program is shown.
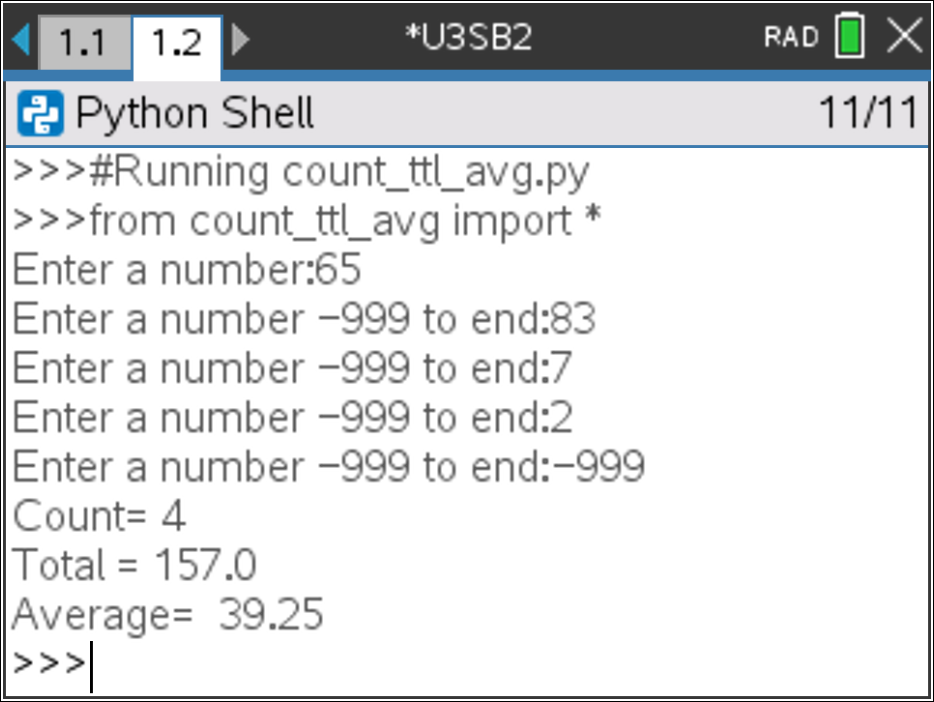
Skill Builder 3: Press a key
Download Teacher/Student DocsIn this lesson, you will use a function that looks for a keypress to terminate a loop. This feature is unique to the TI-Nspire so you will need to import one (or more) special modules.
Objectives:
- Use get_key() to end a loop
- Examine powers of 2 and powers of 1/2.
- Use sleep(n) to pause code execution for n seconds
Step 1
Now that you’ve experienced the while loop, let’s visit a special and powerful feature of the TI-Nspire Python system: get_key(). We will write a program that will display powers of 2 (2, 4, 8, 16, 32, 64, …) continuously and will end only when the esc key is pressed.
Step 2
Begin a new Python ‘blank program’ and name it ‘powers_of_2’.
Use menu > More Modules > TI System to get the statement
from ti_system import *
located at the top of the list.
On the same menu, select the special statement:
while get_key() != “esc”:
block
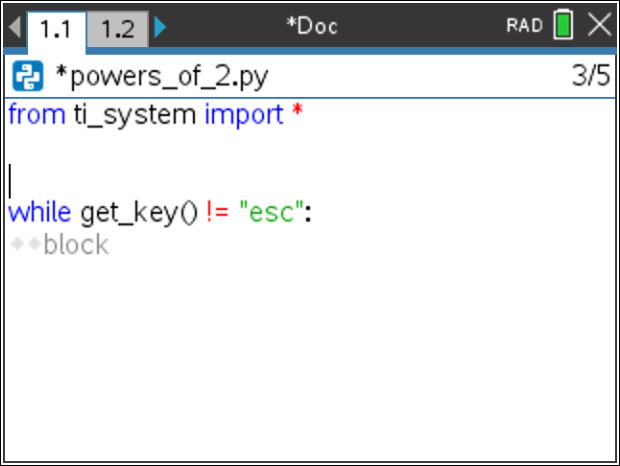
Step 3
- Now introduce a variable num = 2 just before the while statement.
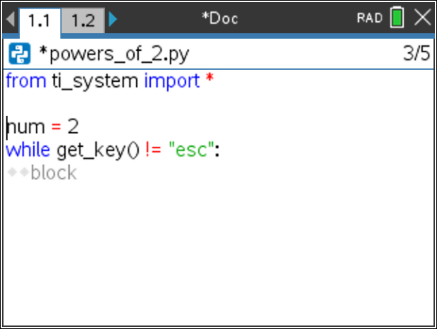
Step 4
-
In the loop block, print the number and then make an assignment statement that will multiply it by 2. Be sure your statements are indented.
Try it yourself before checking the code in the next step…
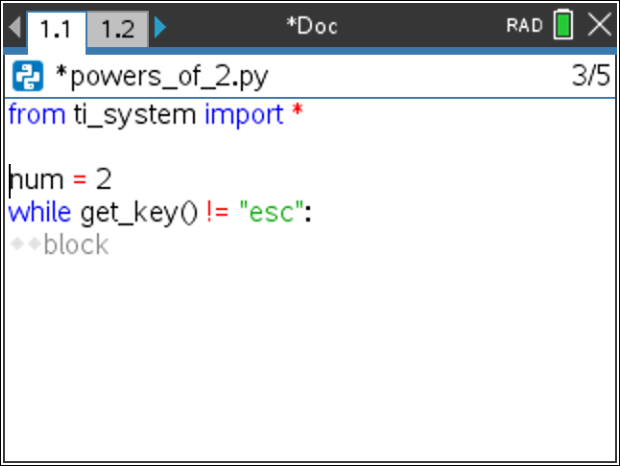
Step 5
-
Here you go…:
while get_key() != “esc”:
print(num)
num = num * 2Run the program and press esc to stop it.
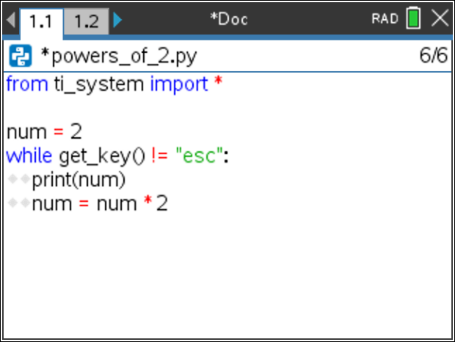
Step 6
-
The numbers grow really fast!
Two interesting features of Python:
It’s really fast and
there’s no upper limit to the integers.
However, you are limited by the capacity of the computer’s memory.Your next task is to slow things down so that you can read the numbers.
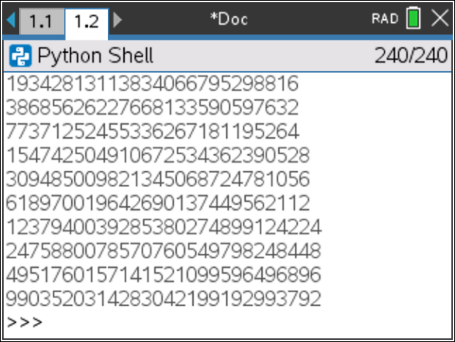
Step 7
-
You need a function called sleep() that is found in the Python time module.
At the top of your program add the statement
from time import sleep
located on the top of menu > More Modules > Time.
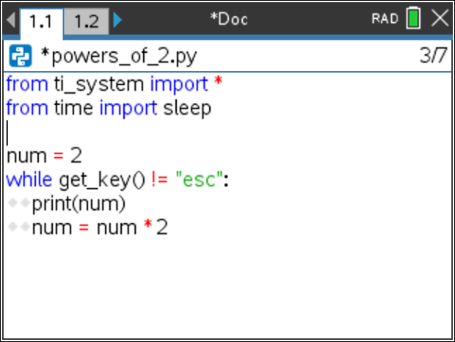
Step 8
-
Below the statement num = num * 2 add the statement
sleep(1)
inside the while block (indented like the other two statements above it).
Run the program again. This time, after each number is displayed, the computer sleeps (waits) for one second before proceeding to the next step in the loop. Change the sleep number to speed things up a bit. Again, press esc to end the program. Try other sleep() values to vary the speed of the display.
At the end of the program, print “Done”.
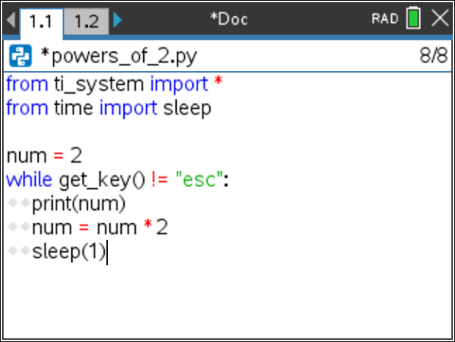
Step 9
-
Change each 2 in the program to 0.5.
What happens?
Try other numbers.
When do the numbers grow and when do they shrink?
How about negative numbers?
Would it make sense to try 0 or 1 instead of 2?
Now try this: Change num = num * 2 to num *= 2
This shortcut operation does the same thing and works for many other mathematical operators.
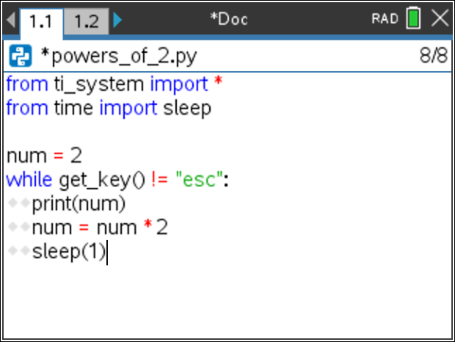
Application: FizzBuzz
Download Teacher/Student DocsIn this Application, you will write a program to illustrate the amazing ‘FizzBuzz’ phenomenon.
Objectives:
- Make a while loop that terminates in two ways
- Define a function that examines numbers for ‘divisibility’
Step 1
FizzBuzz
Write a program to print some of the natural numbers (1,2,3,…) but…
If the number is divisible by 3, print “Fizz” instead.
If the number is divisible by 5, print “Buzz” instead.
If the number is divisible by both 3 and 5, print “FizzBuzz” instead.
The program should end when the esc key is pressed.
‘Divisibility’ is tested using the % operator (mod or remainder).
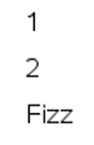
Step 2
There is one other form of the if statement you will find useful:
if <condition>:<true block1>
elif <another condition>: there can be many elif blocks
<true block2> elif is short for ‘else if’
<more elifs?>
else:
<false block> this block is processed when none of the others are true
Step 3
-
Begin a new Python ‘blank program’ file.
The get_key() is going to be used so add
from ti_system import *
found on menu > More Modules > TI System.
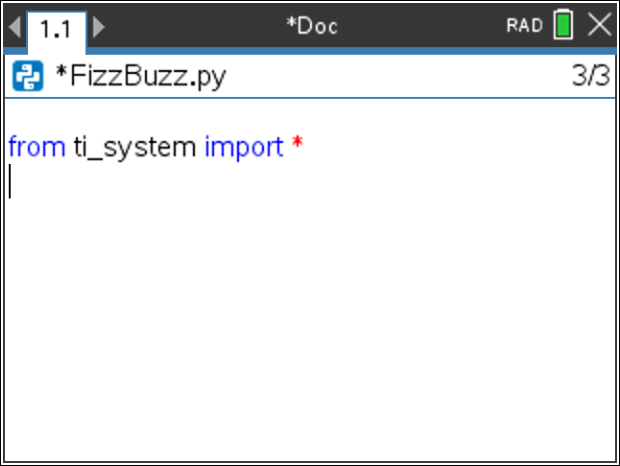
Step 4
-
Set up a variable that represents the counting numbers starting with 1.
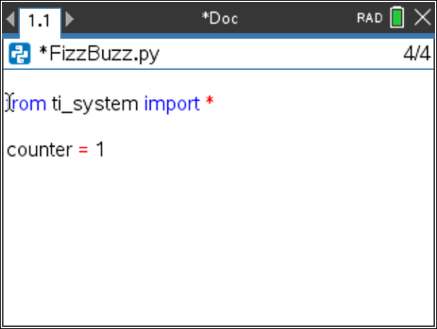
Step 5
-
Use the while get_key()!=0: structure found on
menu > More Modules > TI System.
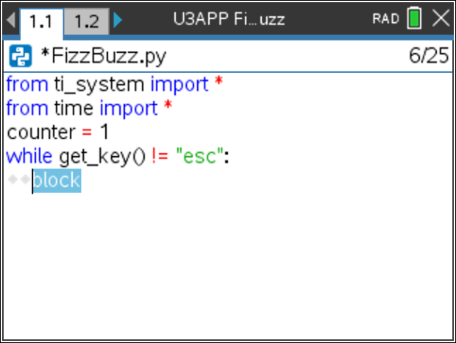
Step 6
-
Use the if..elif..else structure found on
menu > Built-ins > Control.Remember, it works like this:
if <this is true>:
<do this>
elif <this is true>: (stands for ‘else if…’)
<do this>
(there may be more elifs in here)
else:
<do this> (when none of the others are true)Caution: Remember to use == when writing a condition, not =. Using the wrong symbol will result in a syntax error. if x==5:, not if x=5:.
Using the ctrl+= menu can help.
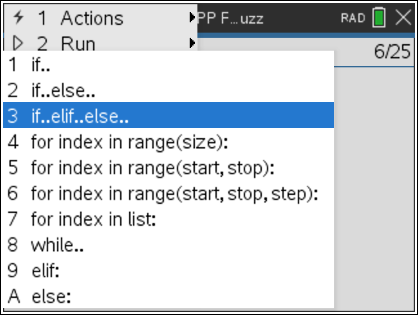
Step 7
-
Here is a peek at the if..elif..else structure but some information has been omitted.
The if and each elif has a condition to be met and a block to be processed when the condition is true. else: has no condition and its block will be processed when none of the others are true.
counter+=1 is shorthand for counter = counter + 1.Hint: Recall that A % B gives the remainder when A is divided by B.
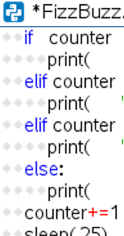
Step 8
-
A sample run of the program might look like the image to the right.
If your numbers go by too fast, use a sleep() function at the bottom to slow things down.
sleep() is in the time module so be sure to include:
from time import sleep
What is the first number replaced by ‘FizzBuzz’?
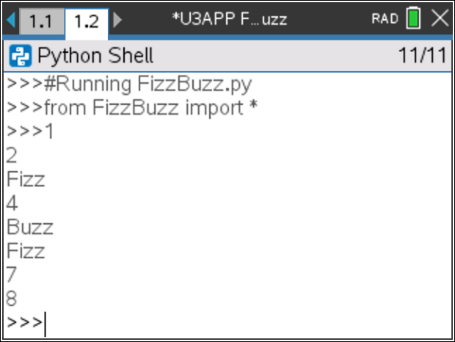
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 4: for loops and lists
Skill Builder 1: Home on the range()
Download Teacher/Student DocsIn this lesson, you will create your first of many for loops and examine the many advantages of iterations.
Objectives:
- Explore the range() function
- Use in to test for membership
- Write a for loop
Step 1
Python’s built-in range() function is used to generate a sequence of numbers. In this lesson you will use the range() function to make a for loop.
Open any Python Shell. If you do not have a Shell in your document, press ctrl+doc > Add Python > Shell.
Make a range using the assignment statement:
r = range(10,20,2)
range() is found on menu > Built-ins > Lists.
Now use the in operator to test certain values, like 5 in r.
Note that the results are either True or False.
Try some other values, as pictured. range() does not actually contain the numbers but rather creates them when needed. Printing a range() function just results in the range() function itself.
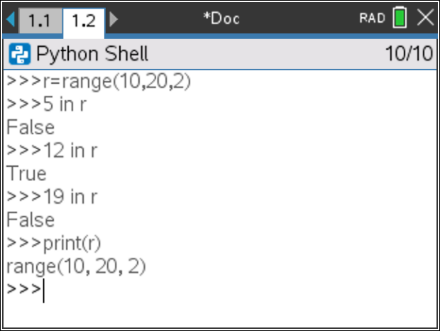
Step 2
-
Still in the Shell (since this is a good place to try things out without writing a program):
If you type r enter or print(r) enter, all you see is range(…).
To see all the values in the range() use the for statement:
Press menu > Built-ins > Control to select:
for index in range(start, stop, step)
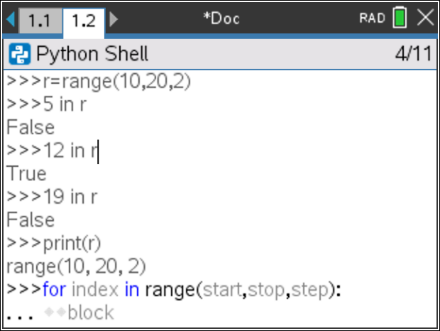
Step 3
-
Change the prompts (press tab to jump from one prompt to the next):
index change to i
start, stop, step change to 10,20,2
block change to print(i)
See the image to the right. Notice the indenting of the print() function.
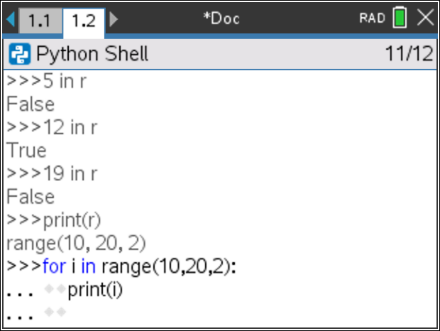
Step 4
-
Press enter a couple of times until the command is processed. You should see the numbers 10, 12, 14, 16, and 18 printed. 20 is not printed.
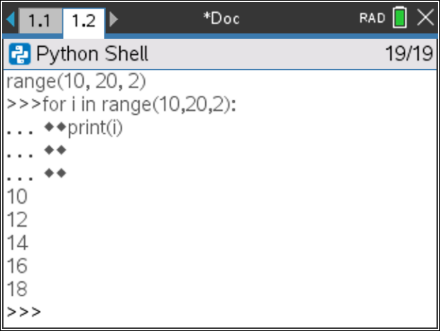
Step 5
-
Write a program using a for statement. Start a new Python file and add the simplest for statement
for index in range(size)
located in menu > Built-ins > Control.
Replace the three prompts with your code as before (size is just one value). Try it yourself…
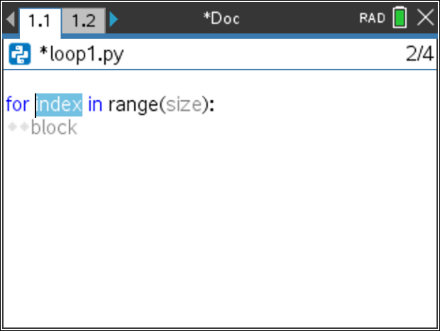
Step 6
-
See if your code is similar to that shown in the image.
i can be any variable, the size can be any positive integer, and the block can be any code. Try print(“hello”) instead.
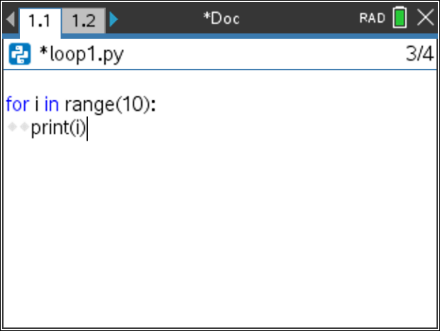
Step 7
-
Run the program. Your output should print some integers.
Without a start value, the loop begins with 0. The loop stops one short of the size (we used 10). size is the upper limit of the range and is not part of the range values.
Without a step value, the step size is 1.
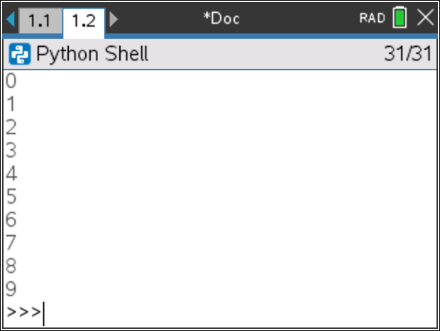
Step 8
-
There are two other for loop options that use range() found on the Control menu.
Can you make a ‘countdown’ loop that goes from 10 to 0 by 1’s? Try it now and then go to the next step…
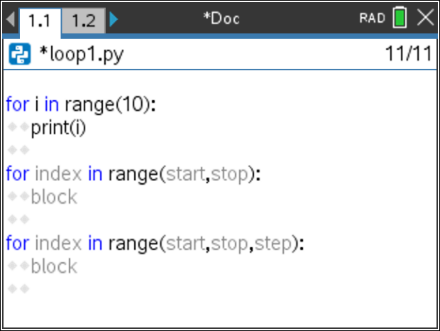
Step 9
-
Did you try something like this?
This loop stops at 1 (still 1 short of the stop value).
Tip: This split screen was achieved by pressing ctrl+4 (Group) in the Editor. The Shell history is cleared; to run the program again press ctrl+R in the Editor.
Again, the stop value of a loop is not included in the loop body (block).
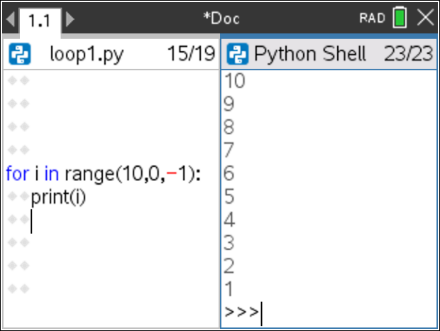
Step 10
-
Write a program that prints the squares of numbers between (and including) two entered numbers by using input().
In a new document write the two statements to input a lower and upper bound of the range.
Next write the for loop using the (start, stop) template.
The loop block should just print the number and its square.
Compare your program to the one shown in the next step…
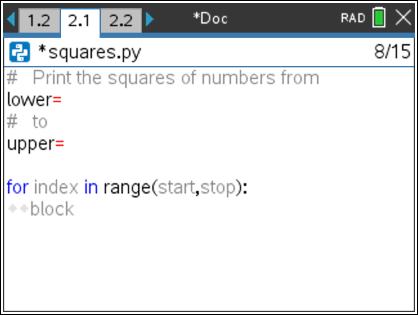
Step 11
-
Did you make the same mistake we did? The two final values, the upper value and its square (10 and 100, respectively), are not included in the printout. ☹
The range() should be:
range(lower, upper + 1)
Did you use num*num or num**2?
Remember to save your work!
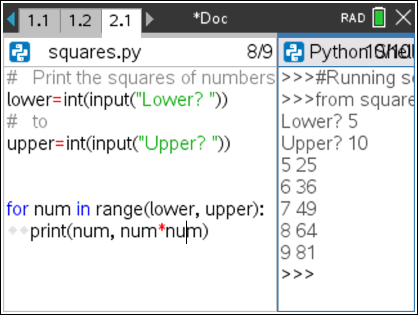
Skill Builder 2: Welcome to Lists
Download Teacher/Student DocsThis lesson introduces you to Python lists, which are similar to, but not the same as TI-Nspire lists.
Objectives:
- Create a list in the Shell
- Write a program to make a list
- List functions: .append()
- Add elements to a list
- List tools on the menu
- Analyze a list: mean
Step 1
-
Our first venture into the world of lists takes place in the Python Shell. In any Shell app see menu > Built-ins > Lists for the major list functions like list(), .append(), and others.
The assignment statement
a = list(range(0,5,1))
or
a = list(range(5))stores the numbers from 0 to 4 into the variable a as a list.
range() provides the numbers and list() arranges them into a list. Both functions are found on menu > Built-ins > Lists.
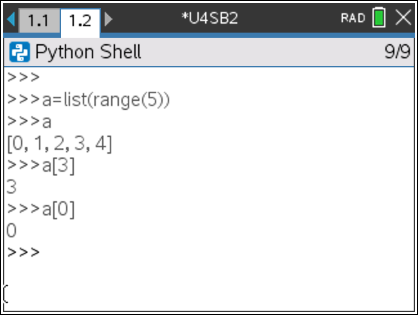
Step 2
-
Pressing enter only stores the list in the variable.
To see the list, just type the variable a then press enter.
The elements of the list are accessible by their index, BUT the first element of the list has index 0.
The list a has 5 elements: 0, 1, 2, 3, and 4 and the indices are 0, 1, 2, 3, and 4. There is no element a[5] in a 5-element list. List a looks like this:
Index: 0 1 2 3 4 Element: 0 1 2 3 4
The index and the element can both be represented by variables as you will see next…
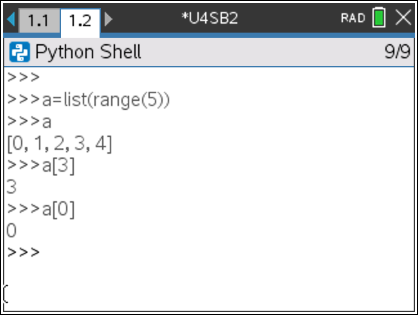
Step 3
-
Write a program that makes use of a list of numbers that we will enter. But first, tell the program how many numbers there will be.>
In a new Python file, use an input() statement to enter the number of numbers (we used the variable amt).
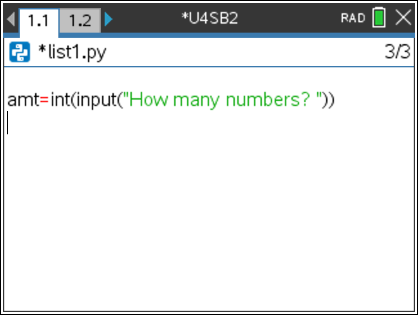
Step 4
-
Create an empty list using the assignment statement:
nums=[ ]
This list will be used to store all the numbers entered.
You can easily type the two square brackets using ctrl+( or select them from menu > Built-ins > Lists.
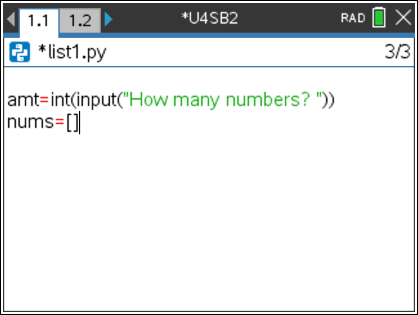
Step 5
-
Add a for loop to enter the numbers.
Use the loop index i. The range value is amt, the variable that holds the number of numbers to enter.
For the block…
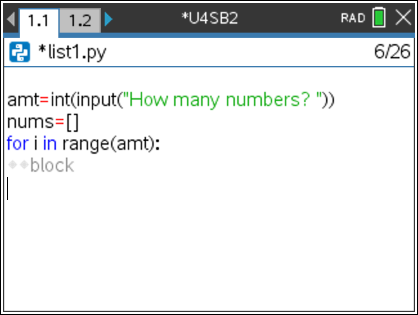
Step 6
-
…print the index value i. This represents the index of the list or the ‘Element number’.
Then write an input statement to enter the number. Use a float() function to allow a decimal value:
value = float(input(“ Enter value: “))
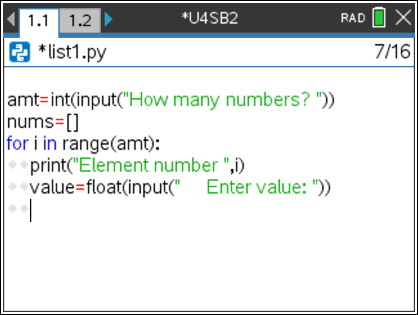
Step 7
-
To add the values to the list, use the Python function:
nums.append(value)
This is the last statement of the loop block.
Press backspace (del) to the beginning of the next line to write the summary code.
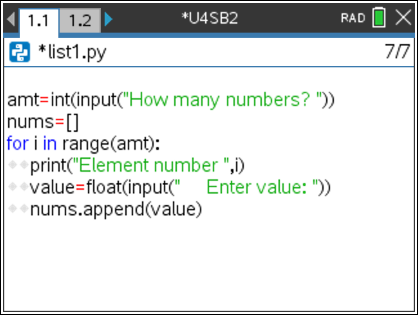
Step 8
-
Print the list with the single statement:
print(nums)
This would be a good time to test your program. When you run the program, enter the number of numbers to enter and then enter each value one at a time. The print statement tells you which element you are entering but remember that the count starts with 0.
After entering the numbers, you should see the list displayed in square brackets like this: [45, 43, 89, 25] (Your numbers will be different.)
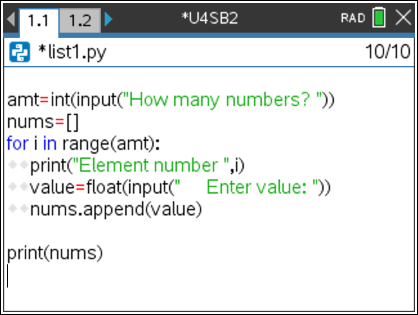
Step 9
-
There are many useful list functions in Python.
See menu > Built-ins > Lists again.
Scroll all the way down.
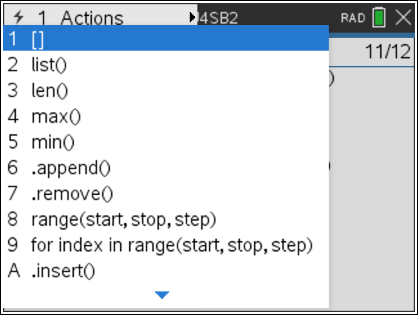
Step 10
-
Get the sum() function from the Lists menu. To calculate the mean of the list, use the formula:
mean = sum(nums) / amt
What additional summary information can you display?
Is there a ‘geometric mean’ as well?
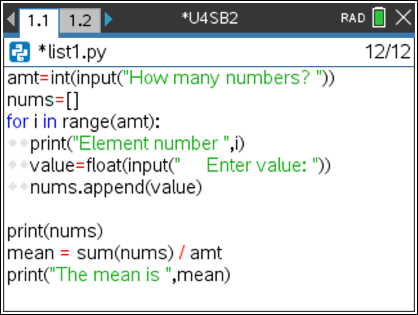
Skill Builder 3: Throwing in Some Randomness
Download Teacher/Student DocsIn this lesson, you will make a list of random numbers to investigate patterns.
Objectives:
- Use randint(a,b)
- Create a list of random integers
- Analyze properties of the list
Step 1
Lists (or arrays) are a convenient way to store many different values in a single variable. Python is very flexible with the contents of a list (it can hold different types of values, but this is rare) and has several ways of making a list.
One expression worth noting is [0] * 3 which ‘replicates’ the element 3 times.
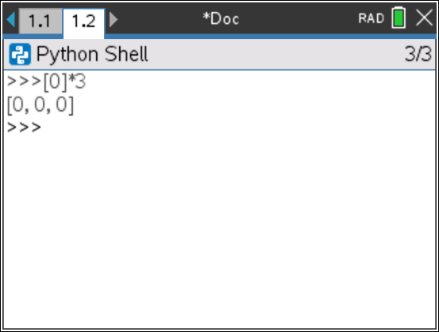
Step 2
What does 3 * [1,2,3] produce? The same expression in the Calculator app gives a completely different result as shown.
Use caution when working with lists in Python because the result might not be what you are accustomed to!
We will stick with the basics in this lesson…
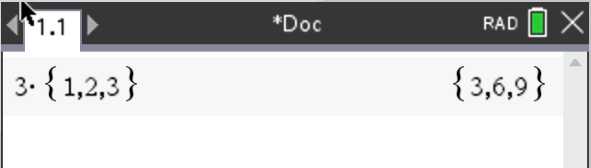
Step 3
-
For this project, when creating the new Python program, choose ‘Random Simulations’ from the Type: dropdown list.
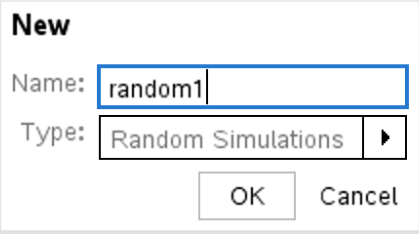
Step 4
-
The Random Simulations template provides you with two modules: math and random. You’ve seen that math includes functions like sqrt(), trig functions, exp() and log(), and others.
The random module contains functions that work with random numbers.
One useful function is randint(a, b) which returns a random integer between and including the values of a and b. The arguments can be numbers, variables or expressions that yield a number.
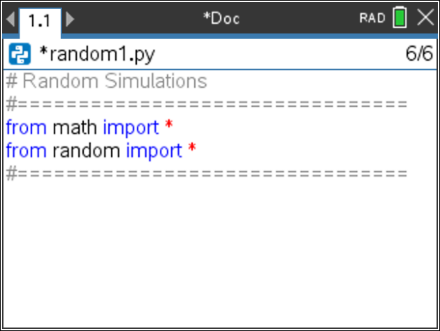
Step 5
-
First, create an empty list:
nums=[ ] (with nothing between the brackets)
Use a for loop to make a list of 100 random numbers, each selected from the range 0 to 25 (you supply the index variable and range size).
The loop block is:
nums.append(randint(1,25))
Pay attention to the two right parentheses at the end of this statement: a common syntax error.
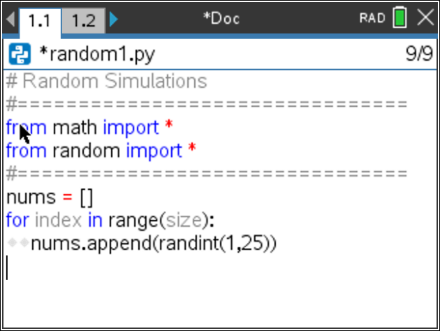
Step 6
-
Add a print statement to the program (after the for loop) to print the list after the numbers have been created:
print(nums)
Run the program now to make sure it works. Pressing
ctrl+R in the Shell will re-run the program and you will see a different set of numbers in each run.
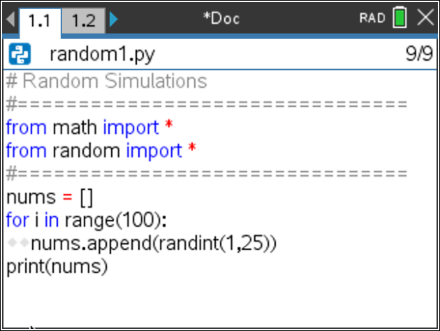
Step 7
-
Here’s a sample run.
Add code to your program to determine the average of the numbers. Try it yourself first. (Remember the last lesson!)
Display the minimum and maximum values in the list.
(Hint: See menu > Built-ins > Lists.)Can you sort the elements?
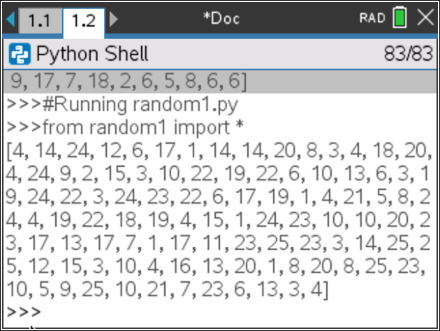
Step 8
-
About sorting…
Python has two tools for sorting a list:
nums.sort() arranges the elements into ascending order.
sorted(n) returns a list that is sorted but does not change the original list.
Use nums2 = sorted(n) to keep the original list and make a new, sorted list named nums2.
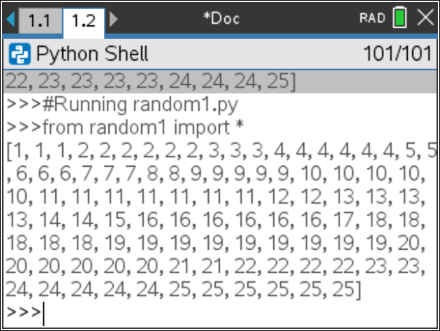
Step 9
-
(Optional) After sorting the list, it is now possible to determine the median (middle) value and the range (difference between minimum and maximum) of the data set.
Add statements to your program to display these values. Note: If the length (size) of the list is an even number, then the median is the average of the two ‘middle’ numbers.
Use the len(<list>) function found on menu > Built-ins > Lists to get the length of the list.
Can you also determine the Q1(first quartile) and Q3 (third quartile) values?
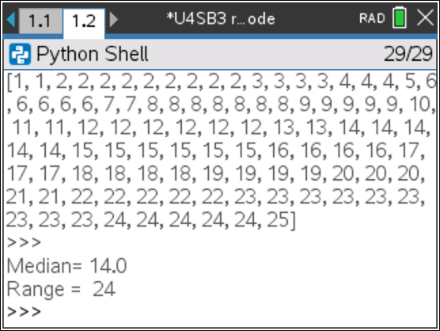
Application: A Toss of the Dice
Download Teacher/Student DocsIn this lesson, you will make a simulation of dice tossing using random numbers.
Objectives:
- Use randint() to simulate dice tosses
- Use a list to keep track of totals
- Analyze the totals
Step 1
When you toss a pair of fair 6-sided dice, the sum of the two dice will be a value from 2 to 12. Which sum is most likely? Least likely? Write a program to simulate the dice tosses and analyze the frequency of each of the eleven sums.
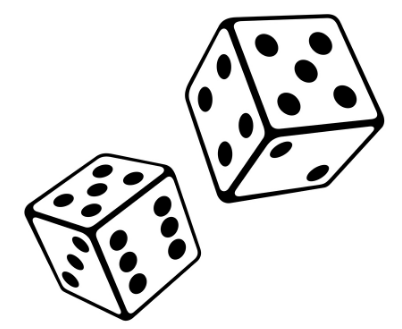
Step 2
-
Start a new Python file using the Random Simulations template as in the last lesson.
Use a list of 11 elements, all 0, to keep track of the 11 sums (2…12). An effortless way to create this list in Python is:
totals = [0] * 11
This means ‘make a list of 11 elements, all 0’. Remember ‘replication’ from the last lesson?
(Try this expression in a Python Shell to see that it works.)
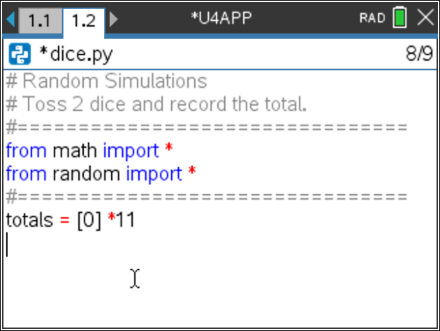
Step 3
-
Use an input statement to ask how many times to toss the dice:
trials = int(input(“# of trials?”))
You can find ‘#’ and ‘?’ on the punctuation key.
Now make a for loop to perform each trial (dice toss):
for i in range(trials):
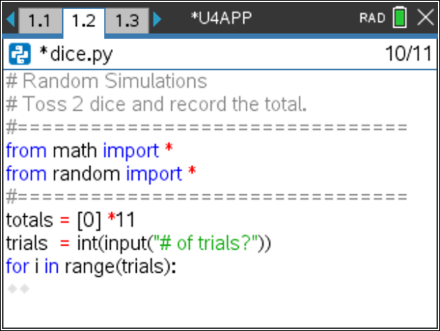
Step 4
-
Now, in the loop block, ‘toss the dice’. Use two variables, die1 and die2. Assign each a random integer from 1 to 6. Try it yourself first and then move to the next step…
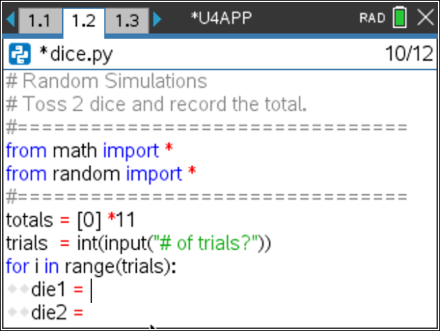
Step 5
-
Did you write = randint(1,6) for each?
Add the two die values together:
sum = die1 + die2
The sum ranges from 2 to 12 but the index of the list totals ranges from 0 to 10. We must subtract 2 from the sum to get the proper list index. To add 1 to the corresponding element in the list of totals looks like this:
totals[sum - 2] = totals[sum - 2] + 1
You may recognize this statement as a counter like c=c+1. Each element of the list is a separate counter.
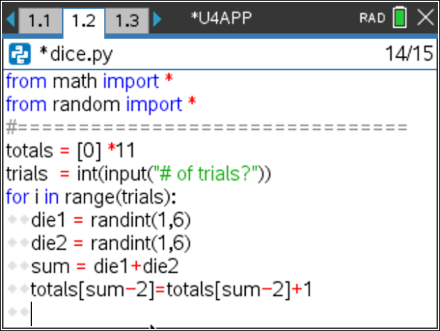
Step 6
-
That’s it for the loop block. When the trials are complete, we want to see the list of totals.
Dedent (un-indent) to the beginning of the next line and print the totals list:
print(totals)
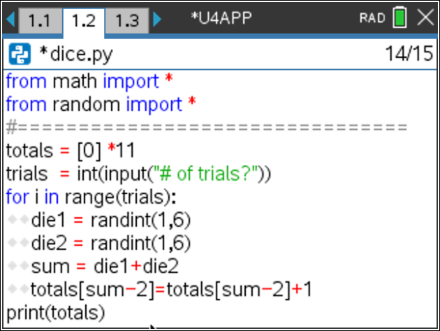
Step 7
-
Test the program now: When you run the program, try a small number like 10 for the number of trials. Check to make sure the sum of the elements in the list is 10. You will probably not get these same numbers (they are random, right?) but the sum of the numbers should equal the number of trials. Re-run with the same number of trials and you will see a different list.
When you are satisfied that the program runs as expected, try a large number of trials, say, 500, 1000, 5000. Which element of the totals list is the largest? Smallest?
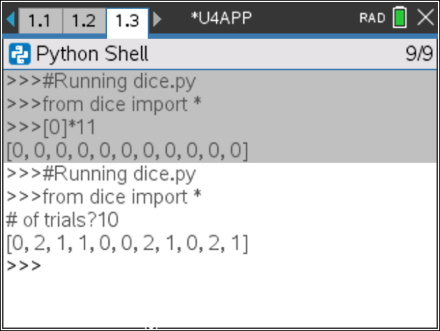
Step 8
-
Your task: Write a for loop that divides each value in the list by the number of trials. Print the result. What is the significance of these numbers?
Start with:
for i in range(11):
You might want to round the long decimals to a fewer number of places. What would be appropriate? Use round(n, #places).
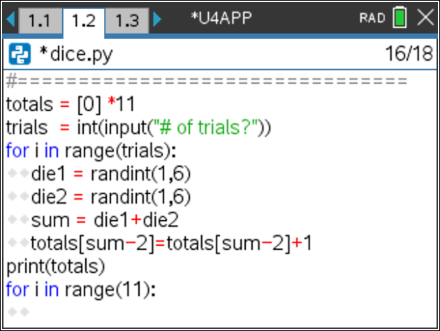
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
Unit 5: The TI Modules
Skill Builder 1: The Plot Thickens
Download Teacher/Student DocsThe TI-Nspire™ CX II Python system contains some special TI-developed modules. One that is useful for plotting data is ti_plotlib and another is ti_draw, which is used for creating graphical displays of all kinds.
(Note: You can find additional activities for other ti_ modules in the [+] Python Modules section of TI Codes.)
In this lesson, you will create a scatter plot of the dice totals from Unit 4 Application using the ti_plotlib module.
Objectives:
- Introduce ti_plotlib
- Make a scatter plot
- Adjust the window
Step 1
In the Unit 4 Application you made a simulation of tossing two dice and logging the experiment’s totals in a list. Here you will continue with that program and see how easy it is to make a scatter plot of that data using Python.
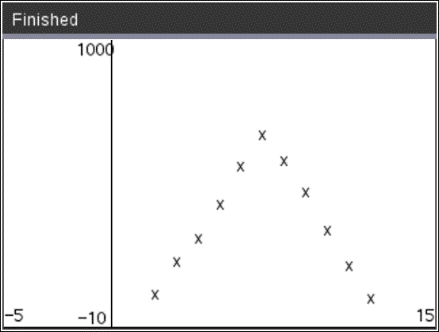
Step 2
Rather than starting from scratch, load the dice project from the Unit 4 Application. All the necessary code is shown in this image. You may have written more code below the print(totals) statement, but you will not need that for this project.
You can make a copy of this program in the document by using:
menu > Actions > Create Copy…
If ‘Create Copy…’ is not available, go back to the program Editor and press ctrl+B to store it. There should not be an asterisk (*) in front of the filename at the top of the page (as pictured).
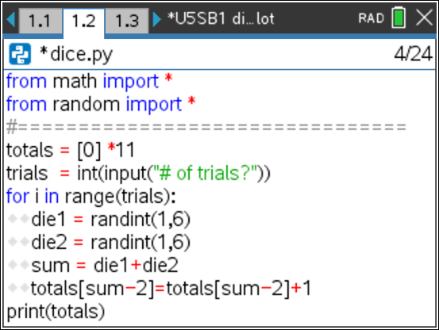
Step 3
-
To create a graphical plot of the data the program created, we need to import another custom TI module. At the top of your program, below ‘from random…’, add the following import statement:
import ti_plotlib as plt
You can get this entire statement from menu > TI Plotlib.
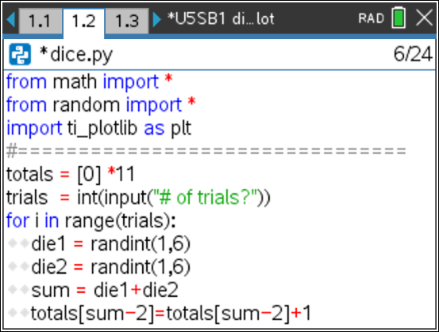
Step 4
-
Scroll to the bottom of the program (below print(totals)).
To make a scatter plot we need two lists, an xlist and a ylist. totals is going to be the ylist. For the xlist, we use the 11 possible sum values:
sums = [2,3,4,5,6,7,8,9,10,11,12]
(There are other, clever ways of making this list but just typing it in is fast and simple.)
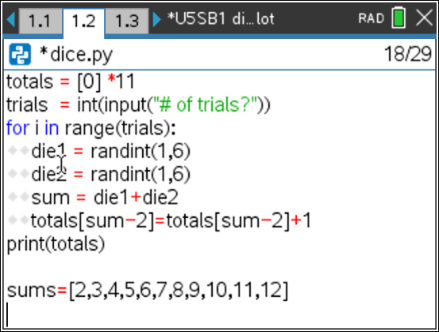
Step 5
-
Now we can set up and display the scatter plot of (sums, totals).
Setup statements are taken from menu > TI Plotlib > Setup.
The two we’ll use here are:
plt.window( )
The window settings depend on the data. Use -5,15 for the x-axis and 10,1000 for the y-axis (we plan on tossing a lot of dice).plt.axes( )
The choices for the “mode” pop up immediately. Select “on”.
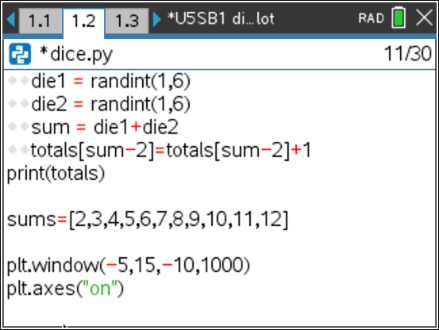
Step 6
-
To make the scatter plot, from menu > TI-Plotlib > Draw select:
scatter(xlist,ylist,"mark")
(plt. is added to the front of the function.)For xlist, type sums.
For ylist, type totals.
For mark, choose* from the four that are allowed.
*It is not so simple to choose a different mark once you have chosen one. There are only four to choose from. They are o, +, x, . (period). If you want to use a different mark, you must type one of these symbols to replace the one you originally chose. You do not get another list to choose from. To see the list again you must paste the function again from the menu.
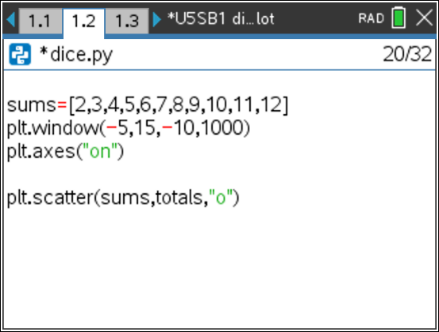
Step 7
-
Ready? Run the program with 1000 trials. Do you see something like this image?
Press any key to close the graph. Try again with 6000 trials.
Can you explain the picture?You can customize the window for the number of trials. In the window function change ymax to 1.1*max(totals).
Note that we only added four new statements to the program to make the plot!Remember to save your work!
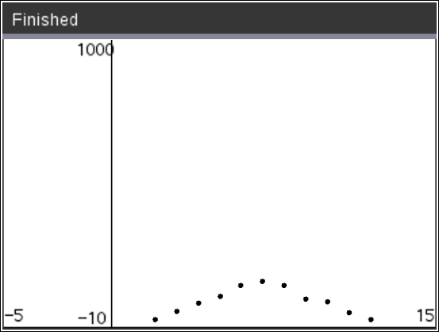
Skill Builder 2: Drawing with Python
Download Teacher/Student DocsIn this lesson, you will experience the thrill of graphical programming using the drawing tools.
Objectives:
- Draw an emoji (smiley face)
- Use set_window, set_color, and set_pen options
- Drawing shapes
Step 1
Graphics are on every electronic device we touch. The ability to make our own graphical designs and interactive animations gives us a tool to put our imaginations to work and give us an outlet for creative expression while learning programming.
Your project will be to draw an emoji: the smiley face…
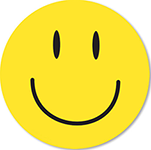
Step 2
-
Start a new Python file using the Type: Geometry Graphics
This template provides the ti_draw module in your program. It contains many tools to create custom graphical displays.
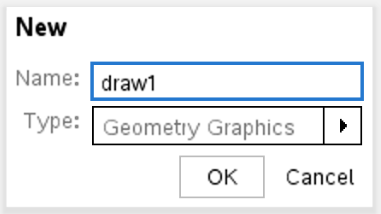
Step 3
-
When you use any of the ti_draw commands a drawing ‘canvas’ appears on top of the Python Shell. The canvas does not go away until the program is ‘Finished’.
Your first ti_draw command establishes the canvas coordinate system.
From menu > More Modules > TI Draw > Control, select set_window().
Use -10, 10, -7, 7 for the window:
set_window(-10, 10, -7, 7)
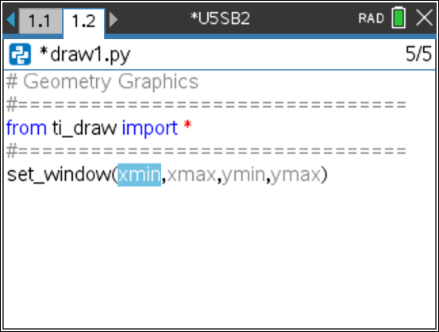
Step 4
-
Run the program now and you will see the blank canvas with a title bar that says ‘Finished’. Press any key to see the Shell again. Go back to the program…
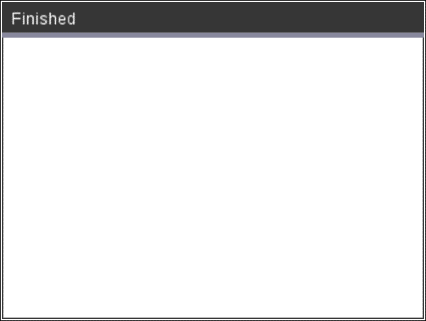
Step 5
-
Draw a yellow filled circle. This requires two statements: one to make the color and one to draw the filled circle.
Get set_color from menu > More Modules > TI Draw>Control.
(Notice the pop-up tool tips explaining the range of values allowed.)Use the color (255,255,0) to make yellow:
set_color(255,255,0)
The three values are the amount of red, green, and blue, respectively, to mix together.
Each value is limited to the range 0…255 as shown in the three tool tips.
Try some other number combinations. There are over 16 million different colors available (256**3)! We are mixing red and green to get yellow! Go figure.
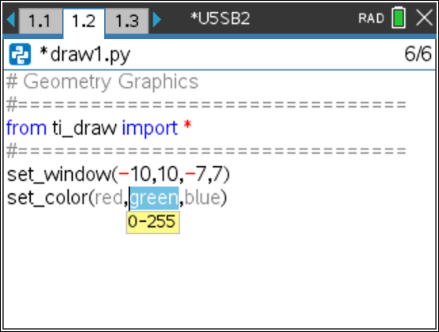
Step 6
-
The circle comes next.
From menu > More Modules > TI-Draw > Shape, select fill_circle().
Now, thinking about the window setting, make a nice big circle with center (x, y) at the origin and use a radius so that the entire circle is on the screen.
Try it now…
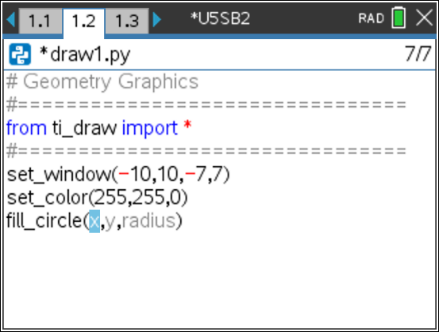
Step 7
-
We chose:
fill_circle(0,0,6)
Run the program to see the result so far.
Congratulations: your first drawing!
Try other numbers in the fill_circle( ) statement. Try mixing other colors in the set_color( ) statement.
Let’s do more…
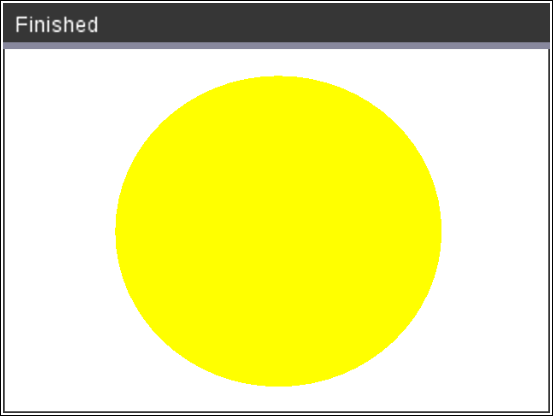
Step 8
-
Before we draw the smile and the eyes, let’s take a look at draw_rect.
Add the two statements:
set_color(0,0,0) (This is black.)
draw_rect(-4,-4,8,4)Run the program.
draw_arc() draws an arc inside this rectangle using the exact same arguments plus a startangle and arcangle (in degrees). To see an arc, add
draw_arc(-4,-4,8,4,0,270)
to your program below draw_rect. Then, run again to see the effect.
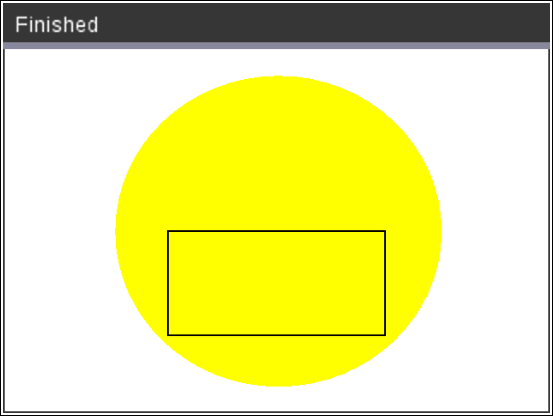
Step 9
-
Explanations:
In draw_rect and draw_arc, the first two numbers (x,y) or (-4,-4) are the lower left corner of the rectangle. 8 is the width (left-to-right) and 4 is the height (bottom-to-top).
draw_arc draws an elliptical arc inscribed in the rectangle. The center of the ellipse is the center of the rectangle. The entire ellipse is drawn when the startangle is 0° (or any number) and the arcangle is 360° (or more). A part of an ellipse is drawn when the arcangle is less than 360°.
Our choices of a startangle of 0° and an arcangle of 270° illustrate that 0° is ‘east’ (to the right) and the measurement goes counterclockwise, thus drawing ¾ of the ellipse.
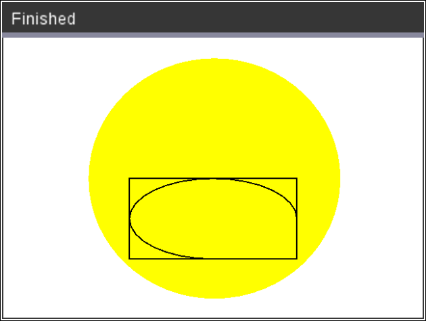
Step 10
-
Change the draw_arc statement to
draw_arc(-4,-4,8,4,180,180)which means ‘start at 180 degrees and go 180 degrees’ around (counterclockwise).
(0 degrees is to the right, 180 is to the left)This draws the lower half of the ellipse. Try other angles.
Now, do not erase but…turn the draw_rect statement into a comment by pressing ctrl+T anywhere on the line. This removes the rectangle from the drawing without removing the code from the program... just in case you need it again.
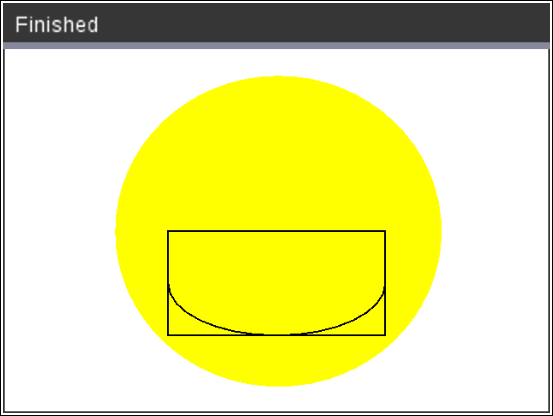
Step 11
-
To make the smile thicker, use (just before draw_arc):
set_pen("thick","solid")
This is found on menu > More Modules > TI Draw > Control.
set_pen has two arguments:
- pen thickness can be one of thin, medium, or thick
- pen style can be one of solid, dashed, or dotted
You can also use the numbers 0, 1, or 2 for both arguments.
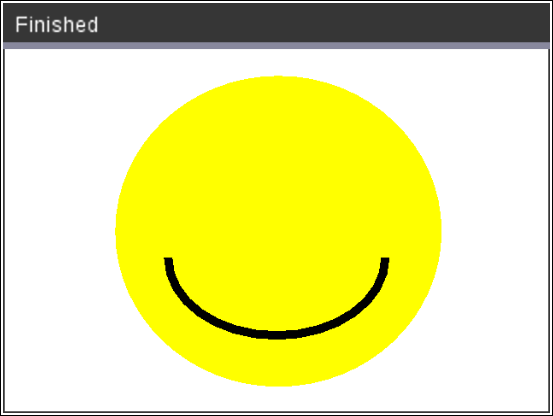
Step 12
-
Draw the eyes using two fill_arc statements. Remember that the structure is:
fill_arc(lower-left-x, lower-left-y, width-right, height-up, 0, 360)
Finally, use draw_circle() to surround the yellow circle with a black border. Try it yourself!
What emoji can you make? How about a cartoon character?
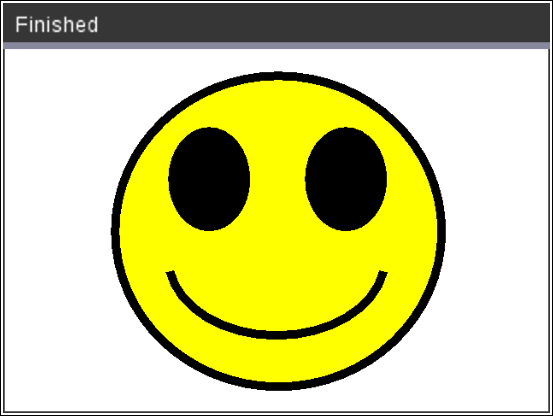
Skill Builder 3: Circles Everywhere
Download Teacher/Student DocsIn this lesson, you will make use of several separate included modules to create a ‘screen saver’ style animation.
Objectives:
- Use several imports
- Use random numbers
- Stop a program with a keypress
- Draw filled circles
Step 1
Your next project makes random circles on the screen until a key is pressed. This is a sort of ‘screen saver’ (a type of program that was used in the old days to prevent ‘burning in’ of a CRT display by fixed images).
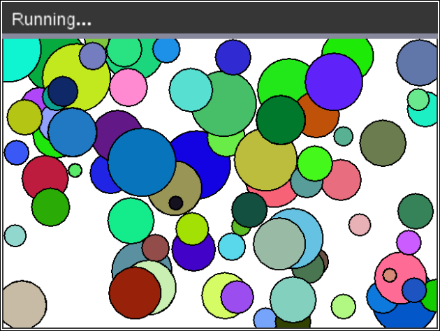
Step 2
Start a new Python file using the Type: Geometry Graphics This template provides the ti_draw module. You will also need two more modules in this project: one for random numbers and one for a ‘press esc to end’ feature. Random number functions are found in the random module and get_key is in the ti_system module, so import both modules. They are found on different menus.
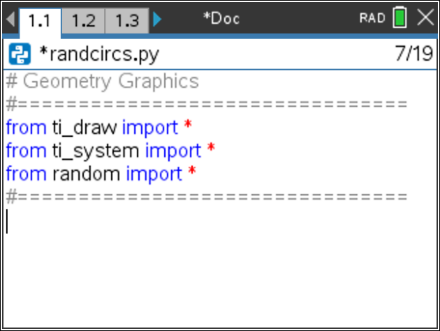
Step 3
In this project, do not use set_window. Use the ‘default’ graphics window where (0,0) is in the upper left corner. The screen is 318 x 212 pixels. The coordinates of the four corners and the center of the canvas are shown in this image.
An important difference between this screen and one designed using set_window is:
In the statement draw_rect(x, y, width, height), the (x,y) pair is now the upper-left corner and the height value measures from top-to-bottom because the y-values increase going down the screen.
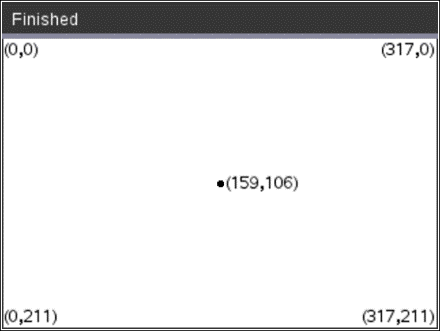
Step 4
-
The main program consists of a loop that stops when esc is pressed. This while statement is on menu > More Modules > TI System.
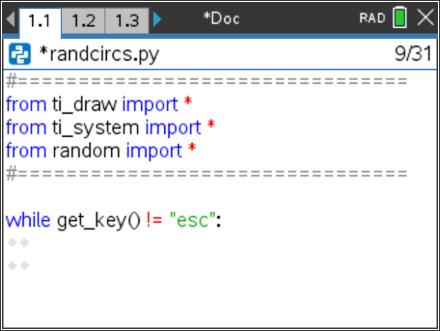
Step 5
-
To draw random circles in random colors, we need to set up several variables with random values. For the circles we need values for x, y, radius and for the colors we need red, green, and blue values.
Each of these six variables is assigned like this one:
x = randint(0,317)
Assign each variable a random integer in an appropriate range. Keep in mind the screen dimensions and color value restrictions.
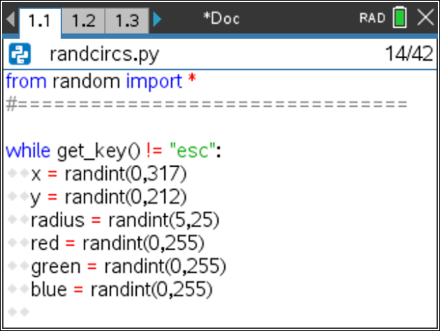
Step 6
-
Add the set_color() and fill_circle() functions from:
menu > More Modules > TI Draw
Do you see that the gray inline prompts for the arguments are the same words as the variable names? Unfortunately, you cannot leave them like that. The inline prompt red is not the variable red. You must type the variable names in place of the inline prompts.
Replace the prompts in these two statements with the variable names.
Run the program when ready and press esc to stop the program.
Feel free to modify the random number ranges. Suppose you want the circles to appear more reddish… what would you do?
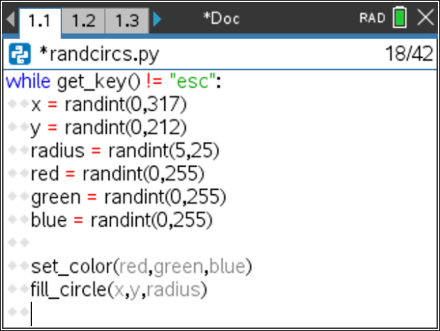
Step 7
-
And, just in case you want to control the speed of the drawing process, use the sleep() function in the time module again. Import sleep from the time module using
from time import sleep
and add sleep(1) at the bottom of the loop (after the fill_circle function and still indented).
This statement pauses processing for 1 second. Too slow? You can make things go faster or slower by using smaller or larger numbers in sleep().
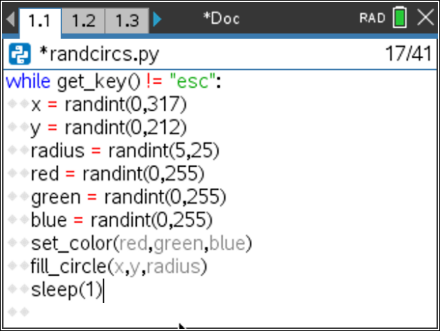
Step 8
-
If you look at the image at the beginning of this lesson, you’ll notice that each circle has a black edge. Can you make that happen, too?
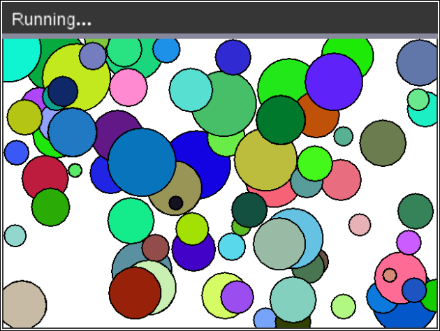
Application: Move it!
Download Teacher/Student DocsIn this Application, you will build the core of an interactive graphical program by using the arrow keys on the keypad to move an object on the screen.
Objectives:
- Combine skills and concepts from this course in a rich and engaging graphical programming project
Step 1
Putting it all together…
Write a program that ‘listens’ for your keypresses and draws something that moves on the screen in the direction of the key.
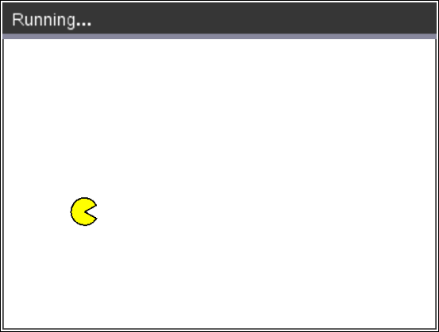
Step 2
-
We'll need get_key() to monitor keypresses and we’ll need the drawing functions to make an picture of something that will move around.
In a blank Python file, import both ti_system and ti_draw. Or, use one of the templates and add the missing imports.
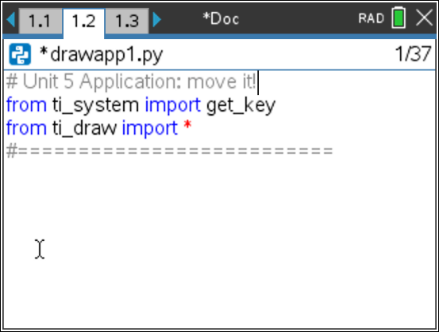
Step 3
-
We need to know what key is pressed so that we can act on it, so we store the key value in a variable:
k = get_key()
We start our object (a circle) at the center of the screen:
x=159
y=106This can also be written: x,y = 159,106
Pressing an arrow key will change one of these values and redraw the object in a new position, making it appear to move.
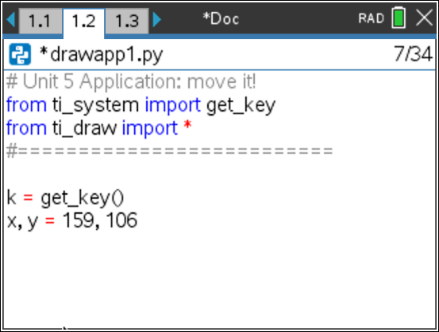
Step 4
-
Begin the main loop:
while k != ”esc”:
Create a colorful circle. Use a color and a radius of your choice.
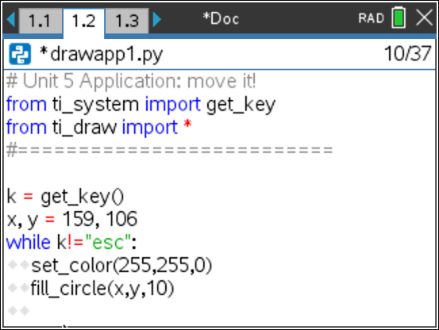
Step 5
-
Check the key that was pressed.
if k == “up”:
y = y – 10 (or y -= 10)This means: If up-arrow is pressed, subtract 10 from the y-value. This causes the circle to move up 10 pixels on the screen.
Write three more if statements for the other three arrow keys (“down”, “left”, and “right”, changing x and y appropriately.
All the if statements are part of the while block.
At the bottom of the block, read the keypress again (k = get_key()) inside the while loop since the first k = get_key() statement is not part of the loop.
When done, test your program.
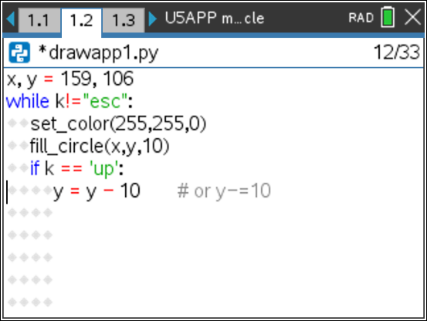
Step 6
-
Use the arrow keys to move the circle. Does the circle move? If so, congratulations!
But it leaves a trail behind.
Erase the old circle before drawing the new one by using:
set_color(255,255,255)
fill_circle(x,y,10)before changing the values of x and y.
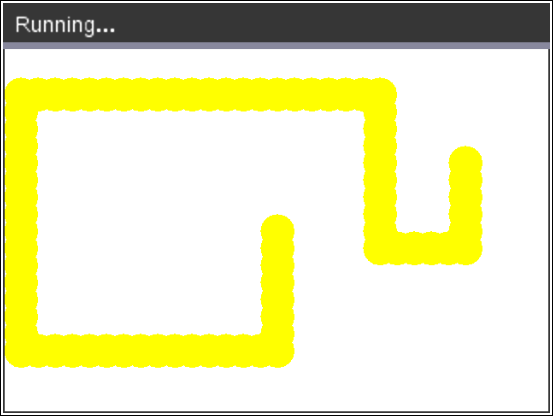
Step 7
-
The circle now ‘blinks’ because it is being constantly drawn in yellow, and then immediately in white. We only want the screen to be updated when the yellow circle is drawn.
So, at the top of your program (before the while), place the statement
use_buffer()
found in TI Draw > Control.
And, right after the yellow circle is drawn, place the statement
paint_buffer()
and run the program again.
Better? The screen is repainted only after the yellow circle is drawn.
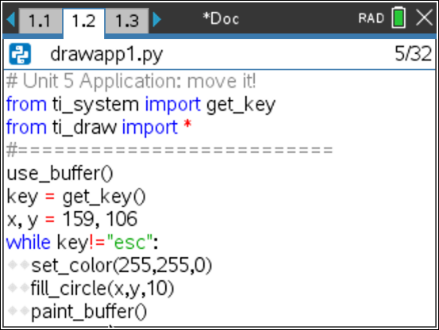
Step 8
-
Finally (for now), what happens when the circle goes off the screen? It just keeps going, going, going. Modify your program with more if statements to detect the edge of the screen and act. You have two options here:
-
When you get to the edge, stay there, and do not go off the screen
(if x<0 then make x=0, etc.). -
When you go off one edge of the screen, wrap around to the opposite side
(if x<0 then make x=317, etc.).
Good luck!
Extra challenge: Modify your program to let the circle keep moving in the last direction even when a key is not pressed.
-
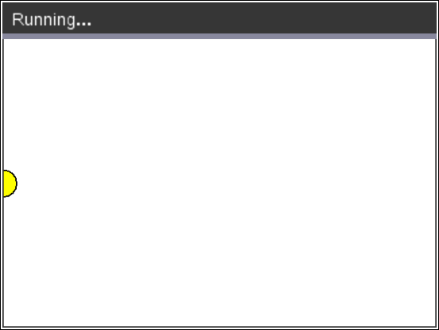
- Skill Builder 1
- Skill Builder 2
- Skill Builder 3
- Application
End of Course Projects
Extensions and More Practice
Download Student DocReady for more? Now that you have completed the course, you are ready to try your hand at some more challenges. Make sure you have completed all the Units prior to starting this section. The projects here will have you apply what you have already learned. Download the document here for challenges to choose from.
If you get stuck, refer back to the previous Units in the course. For an extra challenge, design your own program from scratch. If it’s really cool, post your work to social media and tag it @TICalculators. We would love to see your creativity. Good luck and get coding!